Learn how to solve real-world problems using 1D arrays in C with these interesting scenario-based coding questions. This article features challenges like managing warehouse resources, securing passwords, tracking missing numbers, and more. If you’ve already mastered operators, if-else statements, and loops, these exercises will help you level up your C programming skills.
Graduation Eligibility Checker
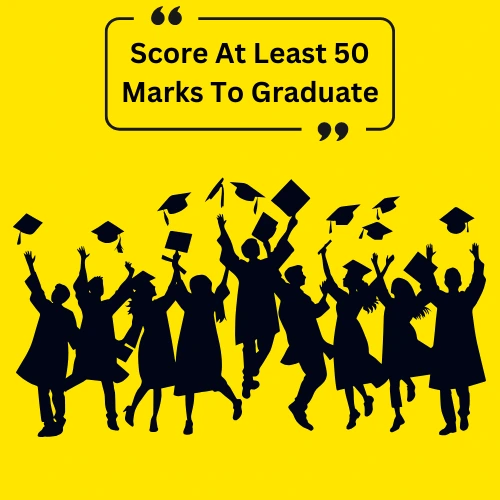
The graduation ceremony at your school is coming up soon. The headmaster has asked you to find out which students are eligible to graduate. To graduate, a student needs to score at least 50 marks in their final exams. Write a C program that takes the marks of 10 students and calculates how many of them have qualified.
Test Case
Input
Enter the marks of 10 students:
Student 1: 43
Student 2: 59
Student 3: 68
Student 4: 25
Student 5: 95
Student 6: 41
Student 7: 39
Student 8: 76
Student 9: 84
Student 10: 49
Expected Output
Total number of students who qualified for graduation: 5
- The program begins by prompting the user to enter the marks of 10 students. These inputs are stored in the
marks
array. - The variable
qualifiedCount
is initialized to zero to keep track of the number of students who qualify for graduation. - The program uses a
for
loop to read and process the marks of each student. - For each iteration, the program displays a message prompting the user to input the marks for a specific student and stores the entered value in
marks[i]
. - After reading each mark, the program checks if the mark is 50 or greater using an
if
statement. If the condition is true, it increments thequalifiedCount
variable by one to record the number of qualified students. - After processing all 10 marks, the program prints the total number of students who qualified for graduation by displaying the value stored in
qualifiedCount
.
#include <stdio.h> int main() { int marks[10], qualifiedCount = 0; printf("Enter the marks of 10 students:\n"); for (int i = 0; i < 10; i++) { printf("Student %d: ", i + 1); scanf("%d", &marks[i]); if (marks[i] >= 50) { qualifiedCount++; } } printf("\nTotal number of students who qualified for graduation: %d\n", qualifiedCount); return 0; }
User Registration System
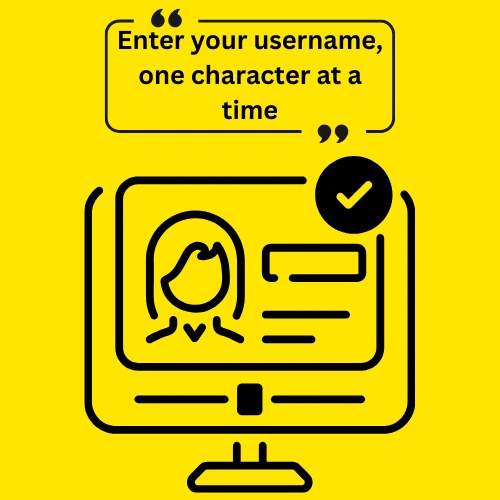
You are building a simple user registration system where the user needs to input their username. The user first provides the length of the username and then enters each character one by one. The system displays the complete username once all characters are entered. Write a C program that takes the length of the username as input, allows the user to input that many characters one by one, and then displays the complete username.
Test Case
Input
Enter the length of your username: 4
Enter your username, one character at a time:
J
h
o
n
Expected Output
Your username is: Jhon
- The program begins by asking the user to enter the length of their username. This tells the program how many characters it should expect. The length is stored in a variable called
length
. - Next, the program uses a
while
loop to collect each character of the username. The loop goes on until the program has collected the number of characters the user specified. Inside the loop, it asks for one character at a time and stores each one in theusername
array. - Once all the characters are collected, the program adds a special character (
'\0'
) to the end of the string to mark the end of the username. This is important for C strings. - After the username is fully entered, a
for
loop is used to print each character from theusername
array one by one. This loop will print the complete username by going through each character and displaying it on the screen.
#include <stdio.h> int main() { int length; char username[100], ch; int i = 0; printf("Enter the length of your username: "); scanf("%d", &length); printf("Enter your username, one character at a time:\n"); while (i < length) { scanf(" %c", &ch); username[i] = ch; i++; } username[i] = '\0'; printf("Your username is: "); for (int j = 0; j < length; j++) { printf("%c", username[j]); } printf("\n"); return 0; }
Temperature Tracker
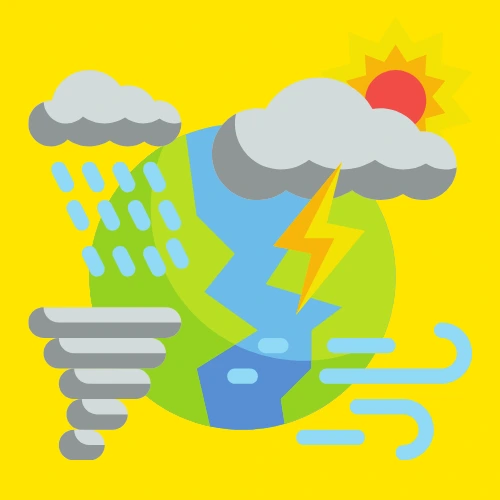
Imagine you’re assisting a meteorologist in keeping track of the week’s weather. Each day, you note down the temperature. By the end of the week, the meteorologist needs your help to find three things, the highest temperature recorded, the lowest, and the average for all seven days. Write a C program that takes the temperatures as input, then calculates and displays the highest, lowest, and average temperatures.
Test Case
Input
Enter the temperatures for 7 days:
Day 1: 25
Day 2: 28
Day 3: 26
Day 4: 27
Day 5: 29
Day 6: 24
Day 7: 30
Expected Output
Highest temperature: 30.00
Lowest temperature: 24.00
Average temperature: 27.00
- The program begins by asking the user to input the temperatures for seven days. These temperatures are stored in an array called
temperatures
. - Next, the program enters the
for
loop where it prompts the user to enter the temperature for each of the seven days. Each day’s input is saved into thetemperatures
array. - After all temperatures are inputted, the program enters another
for
loop. This loop performs three tasks, calculating the sum of all temperatures, finding the highest temperature, and finding the lowest temperature. - It starts by initializing the
highest
andlowest
temperatures to the value of the first day. Then, as the loop iterates through each day’s temperature, it compares the value to the current highest and lowest. - If a temperature higher than the current highest is found, it updates the
highest
. Similarly, if a temperature lower than the current lowest is found, it updates thelowest
. - In the same loop, the program also calculates the sum of all the temperatures by adding each temperature to the
sum
variable. - Once all calculations are done, the program calculates the average temperature by dividing the total
sum
by 7. - Finally, the program displays the highest temperature, lowest temperature, and average temperature for the week.
#include <stdio.h> int main() { float temperatures[7]; float sum = 0, highest, lowest, average; printf("Enter the temperatures for 7 days:\n"); for (int i = 0; i < 7; i++) { printf("Day %d: ", i + 1); scanf("%f", &temperatures[i]); } highest = lowest = temperatures[0]; for (int i = 0; i < 7; i++) { sum += temperatures[i]; if (temperatures[i] > highest) { highest = temperatures[i]; } if (temperatures[i] < lowest) { lowest = temperatures[i]; } } average = sum / 7; printf("Highest temperature: %.2f\n", highest); printf("Lowest temperature: %.2f\n", lowest); printf("Average temperature: %.2f\n", average); return 0; }
Treasure Hunt Validator
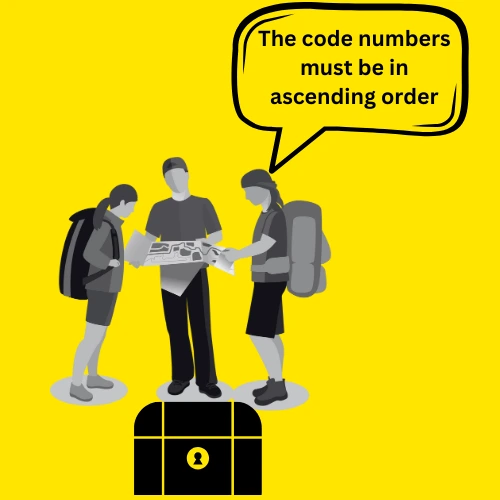
You are helping organize a fun treasure hunt for your friends. To unlock the treasure, players need to follow a series of numbered clues. These clue numbers must always be in a strictly increasing order. If the numbers are out of order, the treasure hunt might get messed up. Your job is to Write a C program that checks whether the clue numbers are correctly ordered. If they are, the hunt can continue smoothly, otherwise, an error message should be shown.
Test Case
Input
Enter the number of treasure codes: 5
Enter the treasure codes one by one:
Code 1: 50
Code 2: 100
Code 3: 150
Code 4: 200
Code 5: 250
Expected Output
The treasure codes are valid. Here’s the sequence:
Code 1: 50
Code 2: 100
Code 3: 150
Code 4: 200
Code 5: 250
- The program begins by asking the user how many treasure codes they want to enter, storing the value in the variable
numCodes
. - An integer array
clueCodes[numCodes]
is created to store the unique treasure codes. - A
for
loop is used to collect each code from the user. - Another
for
loop is used to validate whether the codes are in strictly increasing order. - If the sequence is valid, the program displays the treasure codes in order. Otherwise, it shows an error message and stops further execution.
#include <stdio.h> int main() { int numCodes; printf("Enter the number of treasure codes: "); scanf("%d", &numCodes); int clueCodes[numCodes]; printf("Enter the treasure codes one by one:\n"); for (int i = 0; i < numCodes; i++) { printf("Code %d: ", i + 1); scanf("%d", &clueCodes[i]); } int isValid = 1; for (int i = 1; i < numCodes; i++) { if (clueCodes[i] <= clueCodes[i - 1]) { isValid = 0; break; } } if (isValid == 1) { printf("\nThe treasure codes are valid. Here's the sequence:\n"); for (int i = 0; i < numCodes; i++) { printf("Code %d: %d\n", i + 1, clueCodes[i]); } } else { printf("\nError: The treasure codes are not in strictly increasing order.\n"); } return 0; }
Vault Security Check
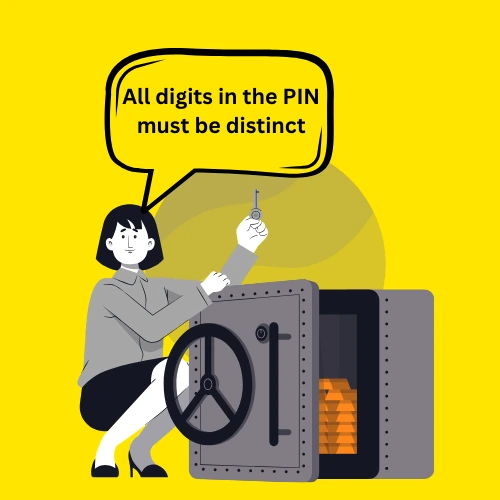
Imagine you’re in charge of securing a vault that opens with a secret 8-digit PIN. For extra safety, the vault won’t open if any of the digits are repeated. Your task is to write a program in C language that takes the 8-digit PIN from the user, checks if any digits are repeated, and then tells whether the PIN is valid or invalid.
Test Case
Input
Enter the 8 digits of the PIN:
1
3
3
2
4
2
5
6
Expected Output
Invalid PIN: Digits must be unique.
- The program begins by asking the user to input 8 digits for the PIN. Each digit is entered one by one, and these digits are stored in an array called
pin[8]
. - The program uses a loop to go through each digit of the PIN. For each digit, the program compares it with all the digits that come after it using another loop. This nested loop checks if any digit appears more than once.
- If a duplicate digit is found, the program sets the variable
isValid
to 0 and breaks out of the loop, marking the PIN as invalid. - If no duplicates are found, the program proceeds to check all the digits, and if everything is fine, it prints “
Valid PIN.
“
#include <stdio.h> int main() { int pin[8]; int isValid = 1; printf("Enter the 8 digits of the PIN:\n"); for (int i = 0; i < 8; i++) { scanf("%d", &pin[i]); } for (int i = 0; i < 8; i++) { for (int j = i + 1; j < 8; j++) { if (pin[i] == pin[j]) { isValid = 0; break; } } if (isValid == 0) { break; } } if (isValid == 1) { printf("Valid PIN.\n"); } else { printf("Invalid PIN: Digits must be unique.\n"); } return 0; }
Cinema Seat Booking System
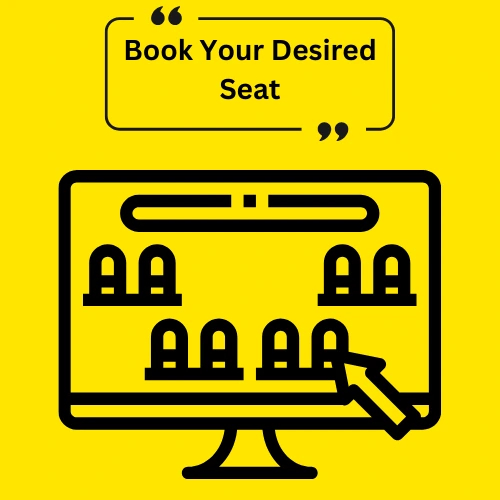
Imagine that you are designing a cinema ticket booking system. The theater has a row of seats, and customers can book a seat by entering a seat number. The system should allow booking a seat only if it’s available and prevent double booking. Write a C program that initializes a list of available seats, allows the user to book seats, and prevents booking an already reserved seat. The program should run in a loop until the user decides to stop.
Test Case
Input
Welcome to Cinema Seat Booking System (10 seats available).
Enter seat number to book (1-10): 3
Do you want to book another seat? (1-Yes / 0-No): 1
Enter seat number to book (1-10): 3
Do you want to book another seat? (1-Yes / 0-No): 1
Enter seat number to book (1-10): 5
Do you want to book another seat? (1-Yes / 0-No): 0
Expected Output
Seat 3 successfully booked!
Sorry! Seat 3 is already booked.
Seat 5 successfully booked!
Thank you for using the Cinema Booking System!
- The program begins by declaring an array
seats[10]
and initializing all elements to0
, indicating that all 10 seats are available. - The program enters a
do-while
loop to repeatedly allow users to book a seat.The user is prompted to enter a seat number (between 1 and 10). This input is stored inseatNumber
. - The program checks whether the entered seat number is within the valid range (1 to 10).
- If the seat number is out of range, an error message is displayed, and the user is asked to try again.
- If the seat is already booked (the corresponding index in
seats
is1
), the program notifies the user that the seat is unavailable. - If the seat is available (
0
), it is marked as booked by settingseats[seatNumber - 1] = 1
, and a confirmation message is displayed. - The program then asks the user if they want to book another seat.
- If the user enters
1
, the loop continues. If the user enters0
, the loop terminates, and a farewell message is displayed.
#include <stdio.h> int main() { int seats[10] = {0}; int seatNumber, choice; printf("Welcome to Cinema Seat Booking System (10 seats available).\n"); do { printf("Enter seat number to book (1-10): "); scanf("%d", &seatNumber); if(seatNumber < 1 || seatNumber > 10) { printf("Invalid seat number! Please enter between 1 and 10.\n"); } else if(seats[seatNumber - 1] == 1) { printf("Sorry! Seat %d is already booked.\n", seatNumber); } else { seats[seatNumber - 1] = 1; printf("Seat %d successfully booked!\n", seatNumber); } printf("Do you want to book another seat? (1-Yes / 0-No): "); scanf("%d", &choice); } while(choice == 1); printf("Thank you for using the Cinema Booking System!\n"); return 0; }
Festival Lights Controller
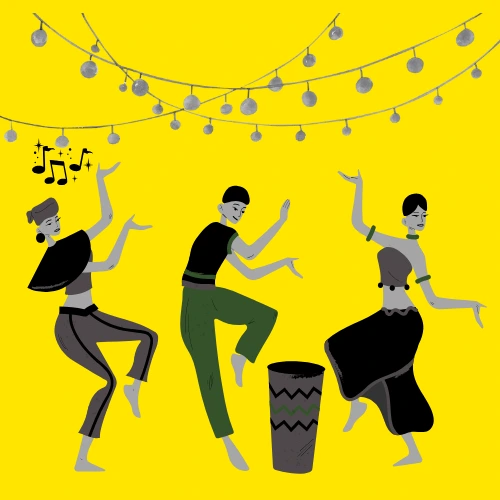
Imagine you are responsible for setting up festival lights for a grand event. The lights are arranged in a straight line, and each light has two states, ON (1) or OFF (0). Due to a power surge, some lights have randomly turned off. Your task is to toggle (reverse) the state of all light, turn ON lights OFF and turn OFF lights ON to restore the festival’s beauty. Write a C program that takes the number of lights and their initial states as input, toggles their states, and displays the updated sequence.
Test Case
Input
Enter the number of festival lights: 5
Enter the initial states (0 for OFF, 1 for ON):
1
1
0
0
1
Expected Output
Updated light sequence:
0 0 1 1 0
- The program begins by asking the user to enter the number of lights. This input is stored in
n
. - It then declares an array
lights[n]
to store the initial states of the lights. - A
for
loop is used to take the initial state of each light (0
for OFF,1
for ON). - Another
for
loop is used to toggle each light by flipping0
to1
and1
to0
. This is achieved using the formulalights[i] = 1 - lights[i]
. - If
lights[i]
is1
(ON), then1 - 1 = 0
(turns OFF).Iflights[i]
is0
(OFF), then1 - 0 = 1
(turns ON). - Finally, the program prints the updated sequence of light states.
#include <stdio.h> int main() { int n; printf("Enter the number of festival lights: "); scanf("%d", &n); int lights[n]; printf("Enter the initial states (0 for OFF, 1 for ON):\n"); for(int i = 0; i < n; i++) { scanf("%d", &lights[i]); } for(int i = 0; i < n; i++) { lights[i] = 1 - lights[i]; } printf("Updated light sequence:\n"); for(int i = 0; i < n; i++) { printf("%d ", lights[i]); } printf("\n"); return 0; }
The Mysterious Missing Number
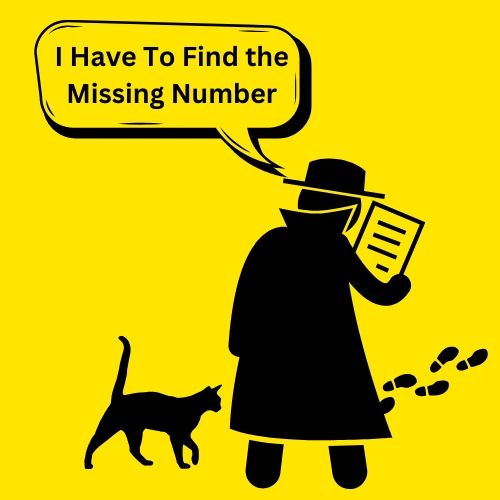
Imagine that you are a detective solving a case. A sequence of consecutive numbers from 1 to N was written down, but one number is missing from the list. Your job is to identify the missing number. Write a C program that takes a list of numbers from 1 to N (with one missing) as input and finds the missing number.
Test Case
Input
Enter the total sequence size: 5
Enter 4 numbers (one number missing):
1
2
3
5
Expected Output
The missing number is: 4
- The program starts by asking the user to enter
N
, which represents the full sequence from1
toN
. - An integer array
numbers[N-1]
is declared to store the given numbers. - The program calculates the expected sum of numbers from
1
toN
using the formula,sum_expected=N×(N+1)/2
- A
for
loop is used to take theN-1
numbers as input while simultaneously calculating the actual sum of the entered numbers. - The missing number is determined by subtracting
sum_actual
fromsum_expected
. - Finally, the program prints the missing number.
#include <stdio.h> int main() { int N; printf("Enter the total sequence size: "); scanf("%d", &N); int numbers[N-1], sum_actual = 0; int sum_expected = (N * (N + 1)) / 2; printf("Enter %d numbers (one number missing):\n", N-1); for(int i = 0; i < N-1; i++) { scanf("%d", &numbers[i]); sum_actual += numbers[i]; } int missing = sum_expected - sum_actual; printf("The missing number is: %d\n", missing); return 0; }
Secure Password Strength Validator
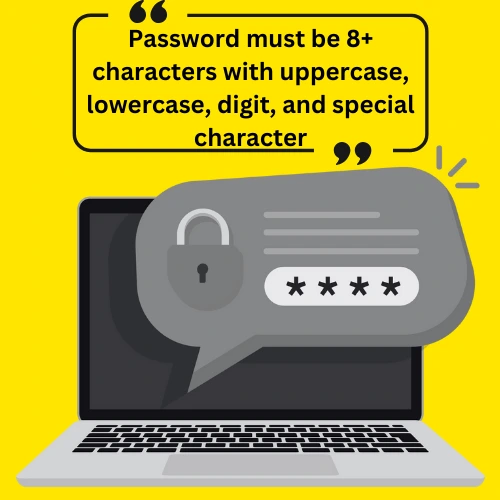
Imagine you are developing a password strength validator for an online banking system. A user is required to enter a password one character at a time, just like when entering a PIN on an ATM screen. The system will check whether the password meets the security criteria to be considered strong. To be considered strong, the password must contain at least one uppercase letter, one lowercase letter, one digit (0-9), and one special character such as @, #, $, %, &, *, or !. Additionally, the password must be at least 8 characters long to ensure a reasonable level of security. Your task is to take password input character by character, check if it meets the security conditions, and display whether the password is Strong or Weak.
Test Case
Input
Enter the length of your password: 12
Enter your password character by character:
P
a
s
s
w
o
r
d
@
3
2
1
Expected Output
Password is Strong.
- The program starts by asking the user to enter the length of the password, which is stored in
n
. - If the entered length is less than 8, the program immediately prints
"Password is too short!"
and exits. - The program then declares a character array
password[n+1]
to store the password. - Four integer flags (
hasUpper
,hasLower
,hasDigit
,hasSpecial
) are initialized to0
to track whether each condition is met. - A
for
loop is used to take password input one character at a time, storing each character inpassword[i]
. - Inside the loop, each character is checked.
- if it is an uppercase letter (
A-Z
), thenhasUpper = 1
. - if it is a lowercase letter (
a-z
), thenhasLower = 1
. - if it is a digit (
0-9
), thenhasDigit = 1
. - if it matches any special character (
@, #, $, %, &, *, !
), thenhasSpecial = 1
by iterating through a predefined list of special characters. - After taking all characters, the program validates the password.
- If all four flags are
1
, the password is Strong. Otherwise, it is Weak. - Finally the program prints whether the password is Strong or Weak.
#include <stdio.h> int main() { int n; printf("Enter the length of your password: "); scanf("%d", &n); if(n < 8) { printf("Password is too short! Must be at least 8 characters.\n"); return 0; } char password[n+1]; int hasUpper = 0, hasLower = 0, hasDigit = 0, hasSpecial = 0; char specialChars[] = "@#$%&*!?"; printf("Enter your password character by character:\n"); for(int i = 0; i < n; i++) { scanf(" %c", &password[i]); if(password[i] >= 'A' && password[i] <= 'Z') hasUpper = 1; else if(password[i] >= 'a' && password[i] <= 'z') hasLower = 1; else if(password[i] >= '0' && password[i] <= '9') hasDigit = 1; else { for(int j = 0; j < 8; j++) { if(password[i] == specialChars[j]) { hasSpecial = 1; break; } } } } password[n] = '\0'; if(hasUpper==1 && hasLower==1 && hasDigit==1 && hasSpecial==1) printf("Password is Strong.\n"); else printf("Password is Weak.\n"); return 0; }
The Lost Parcel Tracker
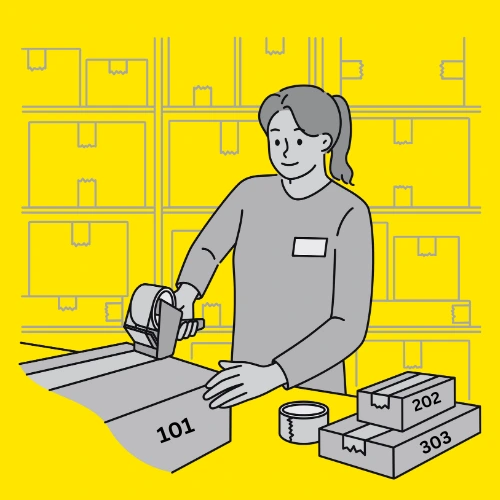
Imagine you are working at a parcel delivery company. The company tracks parcels using unique tracking numbers, but sometimes parcels get misplaced or duplicate entries are recorded in the system. You have been given a list of tracking numbers recorded in a day, and your task is to identify if there are any duplicate tracking numbers. Write a C program that takes the number of parcels and their tracking numbers as input, then checks if any tracking numbers appear more than once and displays them.
Test Case
Input
Enter the number of parcels: 7
Enter the tracking numbers:
101
202
303
101
404
505
202
Expected Output
Duplicate Tracking Numbers:
101
202
- The program starts by asking the user to enter the number of parcels. This input is stored in the variable
n
. - The program then declares an integer array
tracking[n]
to store the tracking numbers and another arrayfound[n]
to keep track of which numbers have already been detected as duplicates. - A for loop is used to take the tracking numbers as input from the user and store them in the
tracking
array. - The program initializes the
found
array with 0, marking all numbers as “not found” initially. - A nested for loop is used to compare each tracking number with every other number in the array.
- If a duplicate is found, it is printed and marked in the
found
array to prevent re-printing the same duplicate. - If no duplicate tracking numbers are found, the program displays a message indicating that all tracking numbers are unique.
#include <stdio.h> int main() { int n; printf("Enter the number of parcels: "); scanf("%d", &n); int tracking[n], found[n]; printf("Enter the tracking numbers:\n"); for(int i = 0; i < n; i++) { scanf("%d", &tracking[i]); found[i] = 0; } int hasDuplicate = 0; printf("Duplicate Tracking Numbers:\n"); for(int i = 0; i < n; i++) { for(int j = i + 1; j < n; j++) { if(tracking[i] == tracking[j] && found[j] == 0) { printf("%d\n", tracking[i]); found[j] = 1; hasDuplicate = 1; } } } if(hasDuplicate == 0) { printf("No duplicate tracking numbers found.\n"); } return 0; }
Treasure Map Decryption
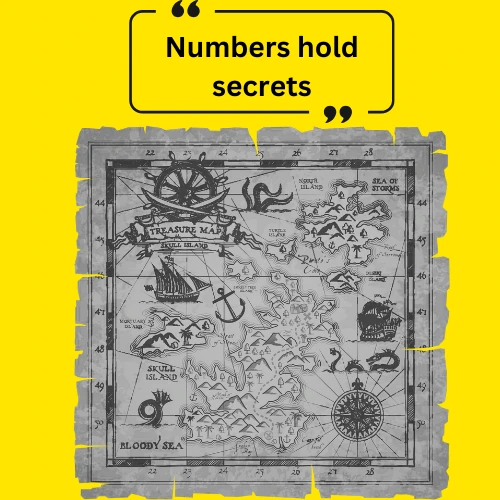
Imagine you are an explorer who has discovered an ancient treasure map written in coded numerical sequences. The map consists of a series of numbers, and hidden within them is a secret pattern that reveals the treasure’s location. However, the map is encoded using a special rule: Each even-indexed number represents steps forward, and each odd-indexed number represents a steps backward. The sum of these movements determines the final position of the treasure on a coordinate scale. Your task is to write a C program that takes an encoded map sequence, deciphers it, and prints the final position of the treasure.
Test Case
Input
Enter the number of encoded steps: 6
Enter the encoded map sequence:
5
3
8
2
7
4
Expected Output
The treasure is located at position: 11
- The program starts by asking the user to enter the number of encoded steps. This input is saved in the
n
variable. - Next, the program declares an integer array
steps[n]
to store the encoded map sequence. - It then prompts the user to enter each number in the sequence using a
for
loop, storing each value in thesteps
array. - The program initializes a variable
position
to 0, which represents the starting coordinate of the treasure. - The program then processes the encoded sequence using another
for
loop. - If the index of the number is even, the value is added to
position
, indicating a step forward. - If the index is odd, the value is subtracted from
position
, indicating a step backward. - This process continues for all numbers in the sequence. Once all numbers have been processed, the final computed
position
represents the treasure’s location. - Finally the program then prints the final
position
.
#include <stdio.h> int main() { int n; printf("Enter the number of encoded steps: "); scanf("%d", &n); int steps[n], position = 0; printf("Enter the encoded map sequence:\n"); for(int i = 0; i < n; i++) { scanf("%d", &steps[i]); } for(int i = 0; i < n; i++) { if(i % 2 == 0) { position += steps[i]; } else { position -= steps[i]; } } printf("The treasure is located at position: %d\n", position); return 0; }
Mysterious Faulty Elevator System
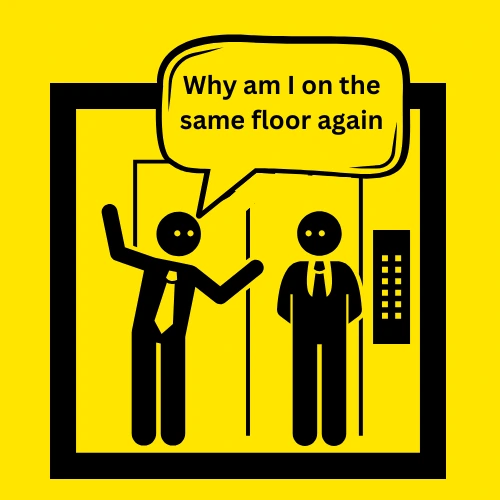
A skyscraper in the city has been experiencing strange elevator malfunctions. The building’s automated system keeps a log of all the floors visited by the elevator, but due to a bug in the software, the elevator sometimes skips floors or revisits old ones multiple times. Your task is to analyze the elevator log and determine two key details, how many unique floors were visited and which floor was visited the most times. By doing this, you can help the engineers diagnose the issue and fix the faulty system. Write a C program that takes the elevator’s movement log, processes the data, and outputs both the number of unique floors visited and the most frequently visited floor.
Test Case
Input
Enter the number of floor visits: 10
Enter the floor visit log:
5
3
8
3
7
5
3
2
5
8
Expected Output
Total unique floors visited: 5
Most frequently visited floor: 3 (visited 3 times)
- The program starts by asking the user to enter the number of floor visits recorded in the elevator log. This number is stored in the
n
variable. - Next, the program declares an integer array
floors[n]
to store the list of visited floors. - A
for
loop prompts the user to enter each floor number, storing them in thefloors
array. - To make it easier to count unique floors and find the most frequently visited floor, the program first sorts the array using Bubble Sort. Sorting ensures that duplicate floor visits appear next to each other, simplifying the counting process.
- After sorting, the program initializes
uniqueCount
to1
. - It also initializes
maxFloor
tofloors[0]
,maxCount
to1
, andcurrentCount
to1
to keep track of visit frequencies. - A second
for
loop then scans the sorted array. If the current floor is different from the previous one, it means a new unique floor was encountered, souniqueCount
is increased. - If the current floor is the same as the previous one,
currentCount
is increased to track how many times the floor has been visited. - If
currentCount
becomes greater thanmaxCount
, the program updatesmaxFloor
to reflect the most frequently visited floor. - Once the loop finishes, the program prints the total number of unique floors visited and the most frequently visited floor along with how many times it was visited.
#include <stdio.h> int main() { int n; printf("Enter the number of floor visits: "); scanf("%d", &n); int floors[n]; printf("Enter the floor visit log:\n"); for(int i = 0; i < n; i++) { scanf("%d", &floors[i]); } for(int i = 0; i < n - 1; i++) { for(int j = 0; j < n - i - 1; j++) { if(floors[j] > floors[j + 1]) { int temp = floors[j]; floors[j] = floors[j + 1]; floors[j + 1] = temp; } } } int uniqueCount = 1, maxFloor = floors[0], maxCount = 1, currentCount = 1; for(int i = 1; i < n; i++) { if(floors[i] != floors[i - 1]) { uniqueCount++; currentCount = 1; } else { currentCount++; } if(currentCount > maxCount) { maxCount = currentCount; maxFloor = floors[i]; } } printf("Total unique floors visited: %d\n", uniqueCount); printf("Most frequently visited floor: %d (visited %d times)\n", maxFloor, maxCount); return 0; }
The Alien Transmission Decoder
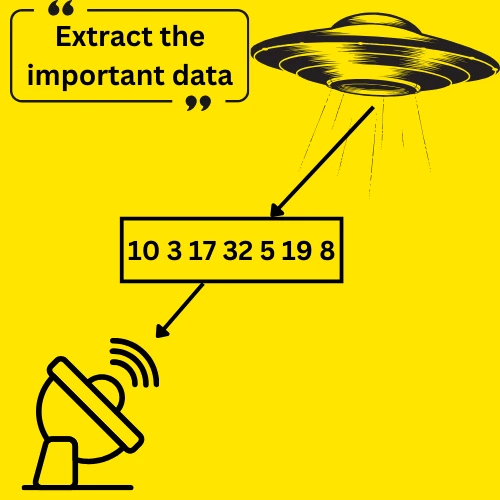
Astronomers at a space research center recently picked up a mysterious radio signal from deep space. The signal is made up of a series of numbers, and while it seems to hide an important message, it’s mixed with extra data. After careful analysis, scientists discovered a pattern: each prime number in the sequence is a crucial piece of the puzzle, while the composite numbers are just background noise. To reveal the hidden message, you need to extract all the prime numbers from the list and add them together. The sum you get is the final decoded value. Your task is to write a C program that takes in this sequence of numbers, identifies which ones are prime, and then prints out the sum of these prime numbers.
Test Case
Input
Enter the number of intercepted data points: 7
Enter the transmission data:
10
3
17
22
5
19
8
Expected Output
Decoded Message Value: 44
- The program starts by asking the user to enter the number of intercepted data points. This input is stored in the variable
n
. - Next, the program declares an integer array
data[n]
to store the intercepted numbers. - A
for
loop is used to taken
numbers as input from the user, storing them one by one in thedata
array. - After the input is received, the program initializes a variable
sumPrimes
to0
. This variable will store the sum of all prime numbers in the sequence. - The program then uses another
for
loop to process each number in the array. For every number, a prime check is performed. - If the number is less than 2, it is ignored because only numbers 2 and above can be prime.
- If the number is 2, it is immediately considered prime and added to
sumPrimes
. - For numbers greater than 2, the program checks whether they are divisible by any number from
2
tonum/2
. If a divisor is found, the number is marked as not prime and ignored. - If a number passes the prime check, it is added to
sumPrimes
. - Once all numbers in the array have been analyzed, the program prints the final decoded message, which is the total sum of all prime numbers found in the sequence.
#include <stdio.h> int main() { int n; printf("Enter the number of intercepted data points: "); scanf("%d", &n); int data[n], sumPrimes = 0; printf("Enter the transmission data:\n"); for (int i = 0; i < n; i++) { scanf("%d", &data[i]); int isPrime = 1; if (data[i] < 2) { isPrime = 0; } else { for (int j = 2; j <= data[i] / 2; j++) { if (data[i] % j == 0) { isPrime = 0; break; } } } if (isPrime) { sumPrimes += data[i]; } } printf("Decoded Message Value: %d\n", sumPrimes); return 0; }
Flood Relief Supply Tracker
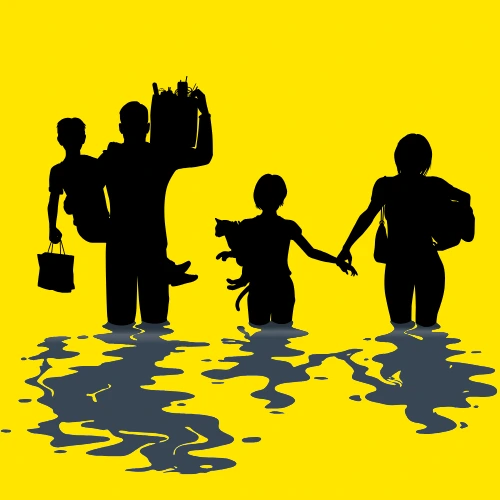
During a flood relief effort, a team is responsible for distributing essential supplies to several relief centers. Each center receives shipments that include four important items: food, water, medicine, and blankets. As the supplies are used, the team keeps a record of how much has been consumed at each center. To manage resources properly, they need a way to calculate how much of each item is left at every location. More importantly, they need to identify any center where the stock of an item has dropped below 20 units so they can arrange for a resupply. Your task is to write a C program that helps with this. The program should take the initial stock of supplies at each center, the amount used, and calculate what remains. It should also check if any supply is running low and flag those centers so that restocking can be done quickly.
Test Case
Input
Enter the number of relief centers: 2
Enter initial supply for each relief center (Food, Water, Medicine, Blankets):
Relief Center 1:
Food: 100
Water: 80
Medicine: 50
Blankets: 60
Relief Center 2:
Food: 90
Water: 70
Medicine: 30
Blankets: 40
Enter used supply for each relief center:
Relief Center 1:
Used Food: 85
Used Water: 50
Used Medicine: 40
Used Blankets: 50
Relief Center 2:
Used Food: 75
Used Water: 55
Used Medicine: 25
Used Blankets: 30
Expected Output
Remaining supplies at each relief center:
Relief Center 1:
Food: 15 units remaining
Water: 30 units remaining
Medicine: 10 units remaining
Blankets: 10 units remaining
Relief Center 2:
Food: 15 units remaining
Water: 15 units remaining
Medicine: 5 units remaining
Blankets: 10 units remaining
Relief Centers that need urgent resupply:
Relief Center 1 – Food (Only 15 units left!)
Relief Center 1 – Medicine (Only 10 units left!)
Relief Center 1 – Blankets (Only 10 units left!)
Relief Center 2 – Food (Only 15 units left!)
Relief Center 2 – Water (Only 15 units left!)
Relief Center 2 – Medicine (Only 5 units left!)
Relief Center 2 – Blankets (Only 10 units left!)
- The program starts by asking the user to enter the number of relief centers. This input is stored in the variable
r
, which represents how many relief centers are being tracked. - Next, the program declares several 1D arrays to store data for each relief center. These arrays include:
initialFood
,initialWater
,initialMedicine
, andinitialBlankets
to store the initial supply quantities of each type of supply for every relief center.usedFood
,usedWater
,usedMedicine
, andusedBlankets
to store the amount of each supply that has been used by the relief centers.remainingFood
,remainingWater
,remainingMedicine
, andremainingBlankets
to store the remaining supplies after usage is deducted from the initial supply.
- The program then prompts the user to enter the initial supplies for each relief center. Using a
for
loop, it iterates through all relief centers (from0
tor-1
) and takes input for the quantity of food, water, medicine, and blankets for each relief center. The input for each type of supply is stored in the correspondinginitial
array. - After recording the initial supplies, the program prompts the user to enter the used supplies for each relief center. Another
for
loop iterates through all relief centers, and the user is asked to input the quantity of food, water, medicine, and blankets that have been used at each center. These values are stored in the correspondingused
arrays. - Once the program has both the initial and used supply data, it calculates the remaining supplies for each type of resource at every relief center. A third
for
loop performs the calculation: remainingSupply = initialSupply−usedSupply. - This calculation is done separately for food, water, medicine, and blankets. The results are stored in the respective
remaining
arrays. The program then displays the remaining quantities of each supply type for every relief center. - Next, the program checks if any relief center has low supplies that need urgent resupply. The threshold for resupply is set to 20 units. For every relief center, the program compares the remaining quantities of each supply type with the threshold. If the remaining quantity is below 20, it prints a message indicating which relief center and supply type need urgent resupply.
- The program keeps track of whether any relief center needs resupply using a variable
needResupply
. If no center requires resupply, the program prints a message stating that all centers have sufficient supplies. - Finally, the program ends after displaying the remaining supplies for each relief center and listing the centers that need urgent resupply.
#include <stdio.h> int main() { int r; printf("Enter the number of relief centers: "); scanf("%d", &r); int initialFood[r], initialWater[r], initialMedicine[r], initialBlankets[r]; int usedFood[r], usedWater[r], usedMedicine[r], usedBlankets[r]; int remainingFood[r], remainingWater[r], remainingMedicine[r], remainingBlankets[r]; printf("\nEnter initial supply for each relief center (Food, Water, Medicine, Blankets):\n"); for (int i = 0; i < r; i++) { printf("Relief Center %d:\n", i + 1); printf("Food: "); scanf("%d", &initialFood[i]); printf("Water: "); scanf("%d", &initialWater[i]); printf("Medicine: "); scanf("%d", &initialMedicine[i]); printf("Blankets: "); scanf("%d", &initialBlankets[i]); } printf("\nEnter used supply for each relief center:\n"); for (int i = 0; i < r; i++) { printf("Relief Center %d:\n", i + 1); printf("Used Food: "); scanf("%d", &usedFood[i]); printf("Used Water: "); scanf("%d", &usedWater[i]); printf("Used Medicine: "); scanf("%d", &usedMedicine[i]); printf("Used Blankets: "); scanf("%d", &usedBlankets[i]); } printf("\nRemaining supplies at each relief center:\n"); for (int i = 0; i < r; i++) { remainingFood[i] = initialFood[i] - usedFood[i]; remainingWater[i] = initialWater[i] - usedWater[i]; remainingMedicine[i] = initialMedicine[i] - usedMedicine[i]; remainingBlankets[i] = initialBlankets[i] - usedBlankets[i]; printf("Relief Center %d:\n", i + 1); printf("Food: %d units remaining\n", remainingFood[i]); printf("Water: %d units remaining\n", remainingWater[i]); printf("Medicine: %d units remaining\n", remainingMedicine[i]); printf("Blankets: %d units remaining\n", remainingBlankets[i]); } printf("\nRelief Centers that need urgent resupply:\n"); int needResupply = 0; for (int i = 0; i < r; i++) { if (remainingFood[i] < 20) { printf("Relief Center %d - Food (Only %d units left!)\n", i + 1, remainingFood[i]); needResupply = 1; } if (remainingWater[i] < 20) { printf("Relief Center %d - Water (Only %d units left!)\n", i + 1, remainingWater[i]); needResupply = 1; } if (remainingMedicine[i] < 20) { printf("Relief Center %d - Medicine (Only %d units left!)\n", i + 1, remainingMedicine[i]); needResupply = 1; } if (remainingBlankets[i] < 20) { printf("Relief Center %d - Blankets (Only %d units left!)\n", i + 1, remainingBlankets[i]); needResupply = 1; } } if (!needResupply) { printf("All relief centers have sufficient supplies.\n"); } return 0; }
Resource Management for Warehouses
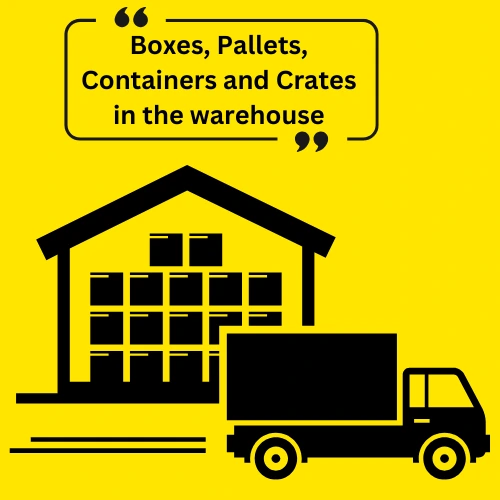
As the logistics manager of a large supply chain company, you are responsible for overseeing multiple warehouses spread across different locations. Each warehouse stores four essential types of storage units that help in managing goods and shipments efficiently. These include boxes, which are used for packaging and small shipments; pallets, which are essential for stacking and bulk transportation; containers, which handle large-scale freight shipments; and crates, which are specifically used for fragile or specialized goods. To ensure smooth operations, you need a system that can track, manage, and optimize the distribution of these resources across all warehouses. The system should allow you to add a new warehouse to the network, initializing its stock of boxes, pallets, containers, and crates. Additionally, it must provide the ability to update the stock levels whenever shipments arrive or resources are used. The system should also display the inventory of all warehouses, making it easier to monitor stock levels. Analyzing the most stocked resource across all warehouses will help determine which storage unit has the highest total quantity. In cases where stock levels need balancing, the system should allow for the transfer of resources between warehouses. If there is a need to find the warehouse with the highest quantity of a specific resource, the system should be able to identify it quickly. Furthermore, when a warehouse is shut down or no longer needed, the system should provide an option to remove it. Since each warehouse is identified by a numeric warehouse ID, the system will track all resources using these IDs. Your task is to write a menu-driven C program that implements this system.
Test Case
Input
Enter the maximum number of warehouses: 3
— Warehouse Resource Management System —
1- Add a New Warehouse
2- Update Resource Quantities
3- Display All Warehouses
4- Analyze the Most Stocked Resource Across All Warehouses
5- Transfer Resources Between Warehouses
6- Find the Warehouse with the Highest Stock
7- Remove a Warehouse
8- Exit
Enter your choice: 1
Enter the initial quantity of boxes: 100
Enter the initial quantity of pallets: 50
Enter the initial quantity of containers: 30
Enter the initial quantity of crates: 20
Enter your choice: 1
Enter the initial quantity of boxes: 150
Enter the initial quantity of pallets: 80
Enter the initial quantity of containers: 40
Enter the initial quantity of crates: 30
Enter your choice: 1
Enter the initial quantity of boxes: 200
Enter the initial quantity of pallets: 100
Enter the initial quantity of containers: 60
Enter the initial quantity of crates: 40
Enter your choice: 2
Enter the warehouse ID (1 to 3) to update: 2
Enter the new quantity of boxes: 180
Enter the new quantity of pallets: 90
Enter the new quantity of containers: 50
Enter the new quantity of crates: 35
Enter your choice: 3
Enter your choice: 4
Enter your choice: 5
Enter the source warehouse ID (1 to 3): 3
Enter the target warehouse ID (1 to 3): 1
Select resource type to transfer (0: Boxes, 1: Pallets, 2: Containers, 3: Crates): 2
Enter the quantity to transfer: 10
Enter your choice: 6
Select resource type to find the warehouse with the highest stock (0: Boxes, 1: Pallets, 2: Containers, 3: Crates): 0
Enter your choice: 7
Enter the warehouse ID (1 to 3) to remove: 2
Enter your choice: 8
Expected Output
Warehouse added successfully!
Warehouse added successfully!
Warehouse added successfully!
Resource quantities updated successfully!
— Warehouse Details —
Warehouse 1:
Boxes: 100
Pallets: 50
Containers: 30
Crates: 20
Warehouse 2:
Boxes: 180
Pallets: 90
Containers: 50
Crates: 35
Warehouse 3:
Boxes: 200
Pallets: 100
Containers: 60
Crates: 40
— Resource Analysis —
Total Boxes: 480
Total Pallets: 240
Total Containers: 140
Total Crates: 95
Most Stocked Resource: Boxes (480 units)
Warehouse 3 has the highest stock: 200 units
Warehouse removed successfully!
Exiting the program. Goodbye!
- The program starts by asking the user to enter the maximum number of warehouses that can be managed. This input is stored in the
maxWarehouses
variable. - It then declares four arrays,
boxes
,pallets
,containers
, andcrate
, each of sizemaxWarehouses
. These arrays are used to store the respective quantities of resources in each warehouse. - Another variable,
warehouseCount
, is initialized to 0 to keep track of how many warehouses have been added. - A
do-while
loop is used to continuously display the menu options and take user input until the user chooses to exit by selecting option8
. - If the user selects option
1
, the program checks whether the maximum number of warehouses has been reached by comparingwarehouseCount
withmaxWarehouses
. If the limit is reached, a message is displayed stating that no more warehouses can be added. - If there is space, the program prompts the user to enter the initial quantity of
boxes
,pallets
,containers
, andcrates
for the new warehouse. These values are stored in their respective arrays at indexwarehouseCount
, and thenwarehouseCount
is incremented to reflect the addition of the new warehouse. - If the user selects option
2
, the program asks for the warehouse ID that needs updating. Since warehouses are stored in arrays starting from index0
, the entered warehouse ID is decremented by1
to match the correct array index. - The program checks whether the given warehouse ID is valid by ensuring it falls within the range of existing warehouses. If valid, the user is prompted to enter the new quantities of
boxes
,pallets
,containers
, andcrates
, which are then updated in the corresponding arrays. - If the ID is invalid (outside the range of
0
towarehouseCount - 1
), an error message is displayed. - If the user selects option
3
, the program first checks if there are any warehouses available. IfwarehouseCount
is0
, it displays a message stating that no warehouses exist. - Otherwise, the program uses a
for
loop to iterate over all existing warehouses, printing theirboxes
,pallets
,containers
, andcrates
values. - If the user selects option
4
, the program calculates the total quantity of each resource across all warehouses. Four integer variablestotalBoxes
,totalPallets
,totalContainers
, andtotalCrates
are initialized to0
. - The program iterates through all warehouses using a
for
loop, adding up the quantities of each resource. - To determine which resource is stocked in the largest quantity, a variable
maxStock
is initially set tototalBoxes
, and a character arrayresourceName
is set to"Boxes"
. - Then, three
if
conditions comparetotalPallets
,totalContainers
, andtotalCrates
withmaxStock
. If any of these have a higher total,maxStock
is updated to that resource’s total, andresourceName
is updated accordingly. - Finally, the program prints the total quantity of each resource and the most stocked resource.
- If the user selects option
5
, the program asks for the source warehouse ID and the target warehouse ID where the transfer will take place. The IDs are decremented by1
to match the correct array index. - The program ensures that the IDs are valid and prompts the user to select a resource type to transfer (
0
for boxes,1
for pallets,2
for containers,3
for crates). - The user is then asked to enter the quantity to be transferred. The program checks if the source warehouse has enough stock for the transfer. If the quantity is valid, the resource is deducted from the source warehouse and added to the target warehouse.
- If the source warehouse has less stock then the required stock, an error message is displayed.
- If the user selects option
6
, the program asks them to choose a resource type (boxes, pallets, containers, or crates). - A variable
maxStock
is initialized to-1
andmaxIndex
is set to-1
to keep track of the warehouse with the highest stock. - The program iterates through all warehouses, checking the selected resource’s stock in each one. If a warehouse has more of that resource than
maxStock
,maxStock
is updated, andmaxIndex
stores that warehouse’s index. - After the loop, the program displays the warehouse ID with the highest stock of the selected resource. If no valid resource type was entered, an error message is shown.
- If the user selects option
7
, the program asks for the warehouse ID to be removed. The ID is decremented by1
to match the correct array index. - The program ensures the ID is valid before proceeding. If valid, all warehouses after the removed one are shifted left in the arrays to fill the gap. This ensures that the warehouses remain in sequence.
- The
warehouseCount
is then decremented to reflect the removal, and a success message is displayed. If the warehouse ID is invalid, an error message is shown. - If the user selects option
8
, the program prints a goodbye message and terminates the loop.
#include <stdio.h> int main() { int maxWarehouses; printf("Enter the maximum number of warehouses: "); scanf("%d", &maxWarehouses); int boxes[maxWarehouses], pallets[maxWarehouses], containers[maxWarehouses], crates[maxWarehouses]; int warehouseCount = 0; int choice; do { printf("\n--- Warehouse Resource Management System ---\n"); printf("1. Add a New Warehouse\n"); printf("2. Update Resource Quantities\n"); printf("3. Display All Warehouses\n"); printf("4. Analyze the Most Stocked Resource Across All Warehouses\n"); printf("5. Transfer Resources Between Warehouses\n"); printf("6. Find the Warehouse with the Highest Stock\n"); printf("7. Remove a Warehouse\n"); printf("8. Exit\n"); printf("Enter your choice: "); scanf("%d", &choice); if (choice == 1) { if (warehouseCount >= maxWarehouses) { printf("Maximum number of warehouses reached. Cannot add more warehouses.\n"); } else { printf("Enter the initial quantity of boxes: "); scanf("%d", &boxes[warehouseCount]); printf("Enter the initial quantity of pallets: "); scanf("%d", &pallets[warehouseCount]); printf("Enter the initial quantity of containers: "); scanf("%d", &containers[warehouseCount]); printf("Enter the initial quantity of crates: "); scanf("%d", &crates[warehouseCount]); warehouseCount++; printf("Warehouse added successfully!\n"); } } else if (choice == 2) { int warehouseID; printf("Enter the warehouse ID (1 to %d) to update: ", warehouseCount); scanf("%d", &warehouseID); warehouseID--; if (warehouseID >= 0 && warehouseID < warehouseCount) { printf("Enter the new quantity of boxes: "); scanf("%d", &boxes[warehouseID]); printf("Enter the new quantity of pallets: "); scanf("%d", &pallets[warehouseID]); printf("Enter the new quantity of containers: "); scanf("%d", &containers[warehouseID]); printf("Enter the new quantity of crates: "); scanf("%d", &crates[warehouseID]); printf("Resource quantities updated successfully!\n"); } else { printf("Invalid warehouse ID.\n"); } } else if (choice == 3) { if (warehouseCount == 0) { printf("No warehouses available.\n"); } else { printf("\n--- Warehouse Details ---\n"); for (int i = 0; i < warehouseCount; i++) { printf("Warehouse %d:\n", i + 1); printf("Boxes: %d\n", boxes[i]); printf("Pallets: %d\n", pallets[i]); printf("Containers: %d\n", containers[i]); printf("Crates: %d\n\n", crates[i]); } } } else if (choice == 4) { int totalBoxes = 0, totalPallets = 0, totalContainers = 0, totalCrates = 0; for (int i = 0; i < warehouseCount; i++) { totalBoxes += boxes[i]; totalPallets += pallets[i]; totalContainers += containers[i]; totalCrates += crates[i]; } int maxStock = totalBoxes; char resourceName[10] = {'B', 'o', 'x', 'e', 's', '\0'}; if (totalPallets > maxStock) { maxStock = totalPallets; resourceName[0] = 'P'; resourceName[1] = 'a'; resourceName[2] = 'l'; resourceName[3] = 'l'; resourceName[4] = 'e'; resourceName[5] = 't'; resourceName[6] = 's'; resourceName[7] = '\0'; } if (totalContainers > maxStock) { maxStock = totalContainers; resourceName[0] = 'C'; resourceName[1] = 'o'; resourceName[2] = 'n'; resourceName[3] = 't'; resourceName[4] = 'a'; resourceName[5] = 'i'; resourceName[6] = 'n'; resourceName[7] = 'e'; resourceName[8] = 'r'; resourceName[9] = 's'; resourceName[10] = '\0'; } if (totalCrates > maxStock) { maxStock = totalCrates; resourceName[0] = 'C'; resourceName[1] = 'r'; resourceName[2] = 'a'; resourceName[3] = 't'; resourceName[4] = 'e'; resourceName[5] = 's'; resourceName[6] = '\0'; } printf("\n--- Resource Analysis ---\n"); printf("Total Boxes: %d\n", totalBoxes); printf("Total Pallets: %d\n", totalPallets); printf("Total Containers: %d\n", totalContainers); printf("Total Crates: %d\n", totalCrates); printf("Most Stocked Resource: %s (%d units)\n", resourceName, maxStock); } else if (choice == 5) { int sourceID, targetID, resourceType, quantity; printf("Enter the source warehouse ID (1 to %d): ", warehouseCount); scanf("%d", &sourceID); printf("Enter the target warehouse ID (1 to %d): ", warehouseCount); scanf("%d", &targetID); sourceID--; targetID--; if (sourceID >= 0 && sourceID < warehouseCount && targetID >= 0 && targetID < warehouseCount) { printf("Select resource type to transfer (0: Boxes, 1: Pallets, 2: Containers, 3: Crates): "); scanf("%d", &resourceType); printf("Enter the quantity to transfer: "); scanf("%d", &quantity); if (resourceType == 0 && boxes[sourceID] >= quantity) { boxes[sourceID] -= quantity; boxes[targetID] += quantity; } else if (resourceType == 1 && pallets[sourceID] >= quantity) { pallets[sourceID] -= quantity; pallets[targetID] += quantity; } else if (resourceType == 2 && containers[sourceID] >= quantity) { containers[sourceID] -= quantity; containers[targetID] += quantity; } else if (resourceType == 3 && crates[sourceID] >= quantity) { crates[sourceID] -= quantity; crates[targetID] += quantity; } else { printf("Invalid transfer. Check resource type or available stock.\n"); } } else { printf("Invalid warehouse ID(s).\n"); } } else if (choice == 6) { int resourceType; printf("Select resource type to find the warehouse with the highest stock (0: Boxes, 1: Pallets, 2: Containers, 3: Crates): "); scanf("%d", &resourceType); int maxStock = -1, maxIndex = -1; for (int i = 0; i < warehouseCount; i++) { int stock = 0; if (resourceType == 0) { stock = boxes[i]; } else if (resourceType == 1) { stock = pallets[i]; } else if (resourceType == 2) { stock = containers[i]; } else if (resourceType == 3) { stock = crates[i]; } if (stock > maxStock) { maxStock = stock; maxIndex = i; } } if (maxIndex != -1) { printf("Warehouse %d has the highest stock: %d units\n", maxIndex + 1, maxStock); } else { printf("Invalid resource type.\n"); } } else if (choice == 7) { int warehouseID; printf("Enter the warehouse ID (1 to %d) to remove: ", warehouseCount); scanf("%d", &warehouseID); warehouseID--; if (warehouseID >= 0 && warehouseID < warehouseCount) { for (int i = warehouseID; i < warehouseCount - 1; i++) { boxes[i] = boxes[i + 1]; pallets[i] = pallets[i + 1]; containers[i] = containers[i + 1]; crates[i] = crates[i + 1]; } warehouseCount--; printf("Warehouse removed successfully!\n"); } else { printf("Invalid warehouse ID.\n"); } } else if (choice == 8) { printf("Exiting the program. Goodbye!\n"); } else { printf("Invalid choice. Please try again.\n"); } } while (choice != 8); return 0; }