This article is full of fun and simple programming tasks to help you practice C language. You’ll find examples like calculating bills at a bookshop, figuring out road trip distances, splitting vacation costs, or even solving math puzzles. Each example comes with clear steps, sample inputs and outputs, and complete C code to make it easy to follow. Whether you’re just starting out or looking for hands-on practice, these real-life scenarios are a great way to learn and improve your coding skills.
Bookshop Bill Calculator
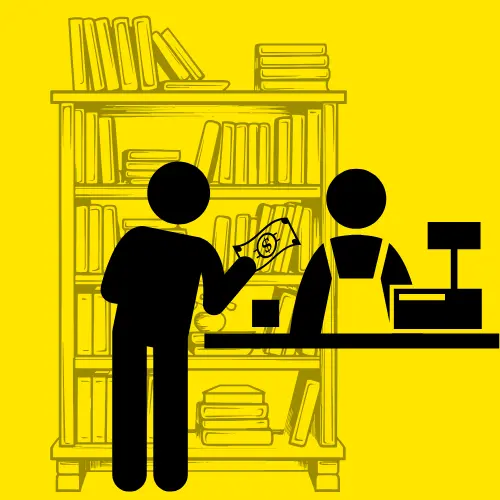
Imagine you are creating a program for a bookshop. Each book costs $10. You need to calculate the total cost for a customer based on the number of books they want to purchase. Write a C program to calculate the total bill.
Test Case
Input
Enter the number of books: 3
Expected Output
The total cost is: $30
Explanation
To calculate the total bill, first store the price of a single book, $10, in a variable. Then, prompt the user to enter the number of books they wish to purchase. After receiving the input, calculate the total cost by multiplying the number of books with the price of a single book
- First, the
book_cost
variable is initialized to store the fixed price of a single book, which is $10. - The user is then prompted to enter the number of books they wish to purchase.
-
quantity
variable is used to store the number of books input by the user. - The program calculates the total cost by multiplying
book_cost
with quantity, and the result is stored in thetotal_cost
variable. - Finally, the program displays the calculated total cost to the user using the
printf
function.
#include <stdio.h> int main() { int book_cost = 10; int quantity; int total_cost; printf("Enter the number of books: "); scanf("%d", &quantity); total_cost = book_cost * quantity; printf("The total cost is: $%d\n", total_cost); return 0; }
Road Trip Distance Calculator
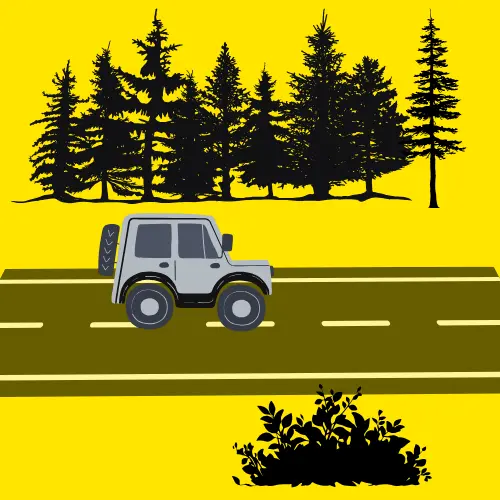
You’re planning a road trip and want to calculate how far you’ll travel. You also have to consider the time spent on breaks. The car travels at a constant speed of 60 kilometers per hour. Write a C program to calculate the total distance traveled after subtracting the time spent on stops.
Test Case
Input
Enter the total travel time in hours: 5
Enter the total stop time in hours: 1
Expected Output
The total distance traveled is: 240.00 km
- The program starts by defining the car’s speed as 60 km/h.
- The user is prompted to input the total travel time in hours and the total time spent on stops in hours.
- The adjusted travel time is calculated by subtracting the stop time from the total travel time. The formula used in the program is
adjustedTime = totalTime - stopTime
. - The distance traveled is then calculated using the formula
distance = speed * adjustedTime
. - The program displays the total distance traveled, formatted to two decimal places using
printf
.
#include <stdio.h> int main() { float speed = 60.0; float totalTime, stopTime, adjustedTime, distance; printf("Enter the total travel time in hours: "); scanf("%f", &totalTime); printf("Enter the total stop time in hours: "); scanf("%f", &stopTime); adjustedTime = totalTime - stopTime; distance = speed * adjustedTime; printf("The total distance traveled is: %.2f km\n", distance); return 0; }
Salary Calculator
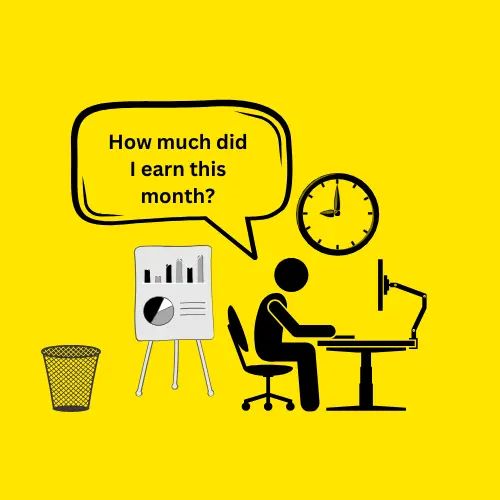
Imagine you work in a company on an hourly basis and are paid based on your hourly rate. Write a C program to calculate your weekly salary based on the hourly rate and the number of hours worked during the week. The salary should also include a fixed bonus.
Test Case
Input
Enter your hourly rate: 25
Enter the number of hours worked: 40
Expected Output
Your weekly salary is: $1050.00
- The program begins by asking the user to input their hourly rate and the number of hours worked during the week.
- The
hourlyRate
variable is used to store the hourly rate entered by the user. - The
hoursWorked
variable is used to store the number of hours worked entered by the user. - The
base_salary
is calculated using the formulabase_salary
=hourlyRate
*hoursWorked
. - A fixed bonus of $50 is added to the
base_salary
, regardless of hours worked. The result is stored infinal_salary
variable. - The program then displays the calculated weekly salary, formatted to two decimal places using
printf
.
#include <stdio.h> int main() { float hourlyRate; float hoursWorked; float baseSalary, finalSalary; printf("Enter your hourly rate: "); scanf("%f", &hourlyRate); printf("Enter the number of hours worked in a week: "); scanf("%f", &hoursWorked); baseSalary = hourlyRate * hoursWorked; finalSalary = baseSalary + 50; printf("Your weekly salary is: $%.2f\n", finalSalary); return 0; }
Bank Balance Calculation
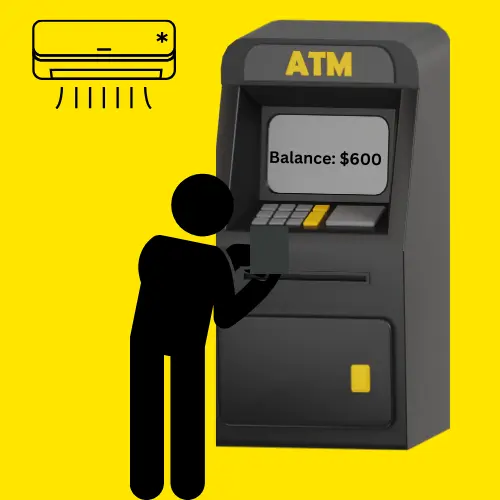
Imagine that you are managing a simple bank account, and you need to calculate the balance after a deposit and a withdrawal. Write a program in C language that takes the initial balance, the amount to deposit, and the amount to withdraw as inputs from the user, and then calculates and displays the final balance.
Test Case
Input
Enter the initial balance: 500
Enter the deposit amount: 200
Enter the withdrawal amount: 100
Output
Your final balance is: $600
- The program starts by asking the user to input the initial balance, the deposit amount, and the withdrawal amount.
- The
initialBalance
variable stores the amount of money the user currently has in their account. - The
depositAmount
variable stores the amount the user wants to deposit into their account. - The
withdrawalAmount
variable stores the amount the user wants to withdraw. - The final balance is calculated using the formula
finalBalance
=initialBalance
+depositAmount
–withdrawalAmount
, where the deposit amount is added to the initial balance, and the withdrawal amount is subtracted from it. - The program then displays the final balance to the user using printf.
#include <stdio.h> int main() { int initialBalance; int depositAmount; int withdrawalAmount; int finalBalance; printf("Enter the initial balance: "); scanf("%d", &initialBalance); printf("Enter the deposit amount: "); scanf("%d", &depositAmount); printf("Enter the withdrawal amount: "); scanf("%d", &withdrawalAmount); finalBalance = initialBalance + depositAmount - withdrawalAmount; printf("Your final balance is: $%d\n", finalBalance); return 0; }
Candy Party
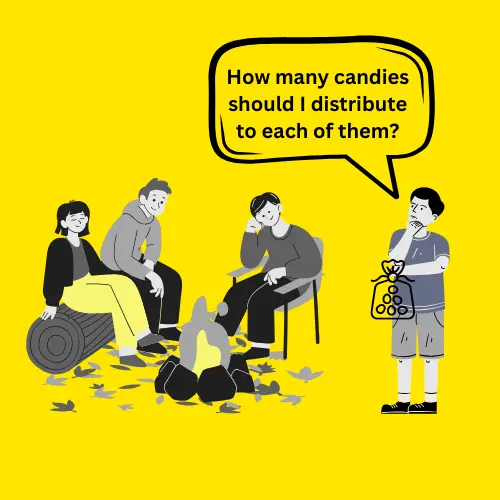
Imagine you have a bag full of candies, and you want to share them equally among your friends so that everyone gets the same number of candies. After sharing, some candies might remain that cannot be distributed equally. Write a C program to calculate how many candies each friend will receive and how many candies will be left over.
Test Case
Input
Enter the total number of candies: 25
Enter the number of friends: 4
Expected Output
Each friend gets 6 candies.
Leftover candies: 1
- The program starts by asking the user to input the total number of candies and the number of friends they want to distribute them to.
- The
totalCandies
variable stores the total number of candies the user has. - The
totalFriends
variable stores the total number of friends who will receive candies. - The number of candies each friend will receive is calculated using integer division,
candiesPerFriend
=totalCandies
/totalFriends
. This operation divides the total number of candies by the total number of friends and gives the result as the number of full candies each friend gets. - The leftover candies are calculated using the modulus operator,
leftoverCandies
=totalCandies
%totalFriends
. This operation gives the remainder when dividing the total number of candies by the total number of friends, representing the leftover candies. - Finally, the program displays the number of candies each friend gets and the leftover candies.
#include <stdio.h> int main() { int candies; int friends; int candies_per_person; int leftover_candies; printf("Enter the total number of candies: "); scanf("%d", &candies); printf("Enter the number of friends: "); scanf("%d", &friends); candies_per_person = candies / friends; leftover_candies = candies % friends; printf("Each friend gets %d candies.\n", candies_per_person); printf("Leftover candies: %d\n", leftover_candies); return 0; }
Vacation Cost Calculation
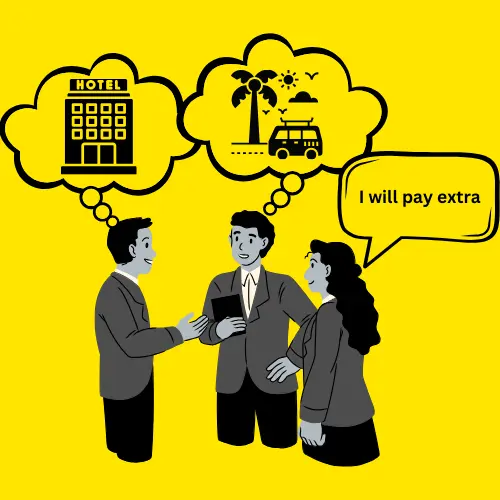
Imagine you and your friends plan a vacation. The total cost of the vacation includes accommodation, food, and transportation expenses. The group decides to split the total cost equally among all members, but one person volunteers to pay an extra amount to cover tips and other small expenses. Write a C program to calculate how much each person has to pay and the total amount paid by the person covering the extra expenses.
Test Case
Input
Enter the cost of accommodation: 500
Enter the cost of food: 300
Enter the cost of transportation: 200
Enter the number of friends: 5
Enter the extra contribution amount: 50
Output
Each person owes: $200
The contributing person owes: $250
- The program begins by prompting the user to input the costs of accommodation, food, and transportation, which are stored in
accommodation
,food
andtransportation
respectively. - Then the user is asked to input the number of friends and the extra contribution amount which is stored in
friends
andextraContribution
variables respectively. - The total cost of the vacation is calculated by adding the values of
accommodation
,food
, andtransportation
and is stored intotalCost
variable. - The
totalCost
is divided by thefriends
variable to calculate the per-person share, and the result is stored inperPersonShare
- The program then calculates the final amount for the person making the extra contribution by adding the
extraContribution
to their share of the cost. The result is stored infinalAmount
. - Finally, the program displays the per-person share for everyone else and the total amount paid by the contributing person using
printf
.
#include <stdio.h> int main() { int accommodation; int food; int transportation; int friends; int extraContribution; int totalCost; int perPersonShare; int finalAmount; printf("Enter the cost of accommodation: "); scanf("%d", &accommodation); printf("Enter the cost of food: "); scanf("%d", &food); printf("Enter the cost of transportation: "); scanf("%d", &transportation); printf("Enter the number of friends: "); scanf("%d", &friends); printf("Enter the extra contribution amount: "); scanf("%d", &extraContribution); totalCost = accommodation + food + transportation; perPersonShare = totalCost / friends; finalAmount = perPersonShare + extraContribution; printf("Each person owes: $%d\n", perPersonShare); printf("The contributing person owes: $%d\n", finalAmount); return 0; }
Coffee Break Time
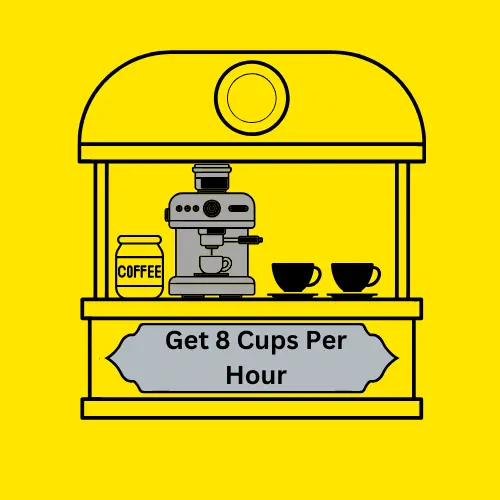
Imagine you are automating a coffee production system for a cafe. The machine produces 8 cups of coffee per hour. Write a program in C language to calculate how many full hours it will take to produce a certain number of cups, and how many cups will be left incomplete.
Test Case
Input
Enter the total cups needed: 25
Output
Full hours: 3
Remaining cups: 1
- The program takes the total number of cups needed as input and stores it in
total_cups
. - The number of total cups is divided by 8 to find the
full_hours
since 8 cups can be made in one hour. - The modulus operator ‘
%
‘ gives the remainder. The remaining cups are calculated using the modulus operator and stored inremaining_cups
. - The
full_hours
andremaining_cups
are displayed to the user usingprintf
.
#include <stdio.h> int main() { int total_cups; printf("Enter the total cups needed: "); scanf("%d", &total_cups); int full_hours = total_cups / 8; int remaining_cups = total_cups % 8; printf("Full hours: %d\n", full_hours); printf("Remaining cups: %d\n", remaining_cups); return 0; }
Marathon Calories Burned
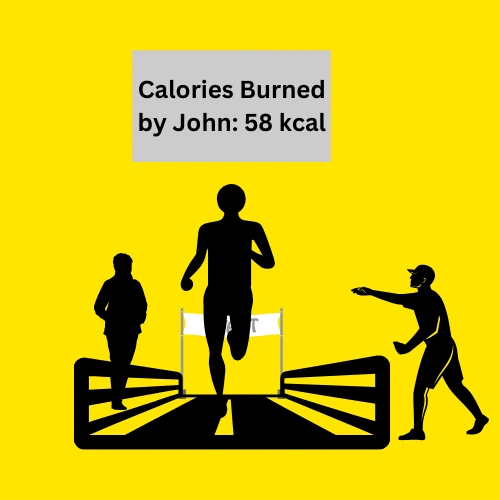
Imagine you’re developing a fitness tracking app for marathon runners. In this app, the goal is to calculate how many calories a runner burns based on the distance they run. A person burns 50 calories for every kilometer they run. Write a C program that takes the distance traveled in meters and calculates the calories burned by the marathon runner.
Test Case
Input
Enter the distance you ran (in meters): 7500
Output
You burned 375.00 calories by running 7.50 kilometers.
- In this program, first a constant variable
calories_per_km
is defined to store the value of calories burned per kilometer. - The program takes the distance traveled in meters as input using the
scanf
function and stores it in the variabledistance_meters
. - The distance in meters is converted to kilometers by dividing
distance_meters
by 1000, as 1 kilometer equals 1000 meters. The result is stored in the variabledistance_kilometers
. - The total calories burned is calculated by multiplying
distance_kilometers
bycalories_per_km
. The result is stored in the variabletotal_calories
. - The program displays the total calories burned and the distance in kilometers using the
printf
function.
#include <stdio.h> int main() { float distance_meters; float distance_kilometers; float total_calories; const int calories_per_km = 50; printf("Enter the distance you ran (in meters): "); scanf("%f", &distance_meters); distance_kilometers = distance_meters / 1000.0; total_calories = distance_kilometers * calories_per_km; printf("You burned %.2f calories by running %.2f kilometers.\n", total_calories, distance_kilometers); return 0; }
Jungle Adventure Supplies
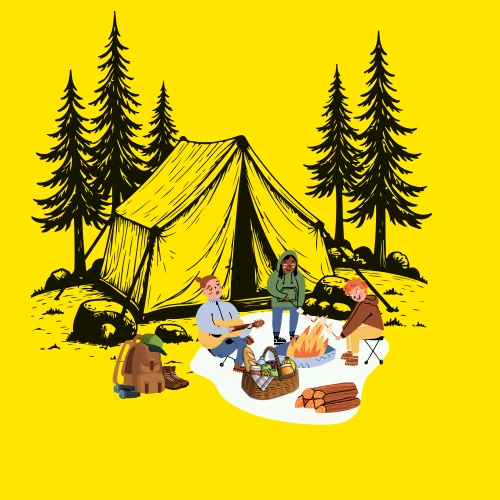
You and your friends are going on an exciting jungle adventure. Each person needs a food pack to stay energized. Each food pack can feed 4 people. Write a program to calculate how many food packs you need for your group and how many people will have to share from an additional pack.
Test Case
Input
Enter the total number of people: 13
Output
Food packs needed: 3
People without full packs: 1
- The program takes the total number of people in your group as input and stores it in the
total_people
variable. - The number of full food packs needed is calculated by dividing the total people by 4 using integer division and the result is stored in
packs_needed
. - The number of people left without a full pack is calculated using the modulus operator and stored in
extra_people
. - Finally, a two
printf
statements are used to display the results.
#include <stdio.h> int main() { int total_people; printf("Enter the total number of people: "); scanf("%d", &total_people); int packs_needed = total_people / 4; int extra_people = total_people % 4; printf("Food packs needed: %d\n", packs_needed); printf("People without full packs: %d\n", extra_people); return 0; }
River Crossing Weight Calculation
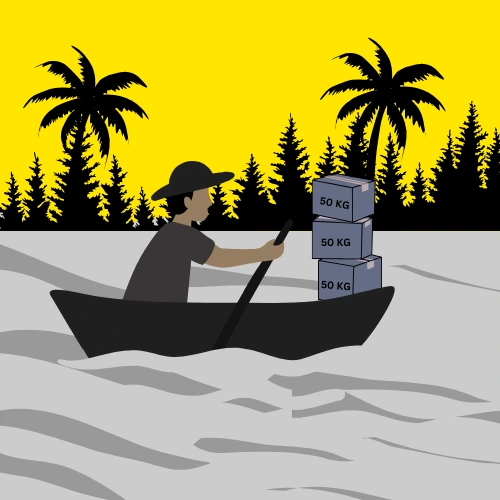
You are transporting cargo across a river using boats. Each boat has a maximum weight limit of 200 kilograms, and each cargo box weighs exactly 50 kilograms. You need to calculate how many boats are required to transport all the cargo boxes safely. Write a program to calculate this based on the total number of cargo boxes provided by the user.
Test Case
Input
Enter the total number of cargo boxes: 9
Output
Total boats needed: 3
- The program takes the total number of cargo boxes as input and stores it in
total_boxes
. - Each boat can carry 200 kg, which is equivalent to 4 cargo boxes.
- The number of boats needed is calculated by dividing the total number of cargo boxes by 4 and rounding up. This is done by adding 3 to the total number of boxes and then dividing by 4.
- The total boats needed are displayed using a
printf
.
#include <stdio.h> int main() { int total_boxes; printf("Enter the total number of cargo boxes: "); scanf("%d", &total_boxes); int boats_needed = (total_boxes + 3) / 4; printf("Total boats needed: %d\n", boats_needed); return 0; }
Desert Water Distribution
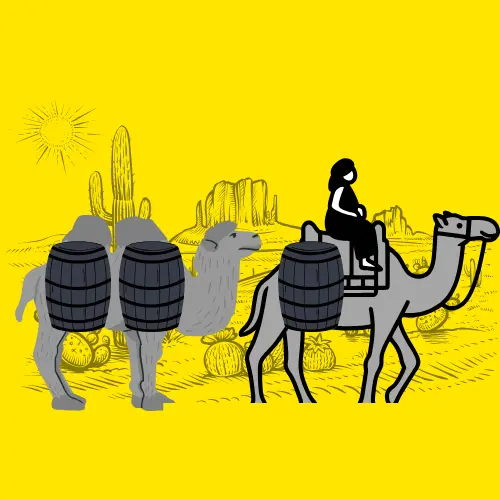
You are distributing water in a desert outpost. Each barrel holds exactly 60 liters of water, and each camel can carry 180 liters. Write a program to calculate how many camels are needed to transport a given number of barrels of water.
Test Case
Input
Enter the total number of barrels: 10
Output
Total camels needed: 4
- The program takes the total number of barrels as input and stores it in
total_barrels
. - Each camel can carry 3 barrels (180/60 = 3).
- The total number of camels required is calculated by dividing the
total_barrels
by 3 and then rounding up. - For rounding up, 2 is added to
total_barrels
before dividing it by 3. This ensures there is an extra camel for any remainder.
#include <stdio.h> int main() { int total_barrels; printf("Enter the total number of barrels: "); scanf("%d", &total_barrels); int camels_needed = (total_barrels + 2) / 3; printf("Total camels needed: %d\n", camels_needed); return 0; }
Treasure Game Scoring
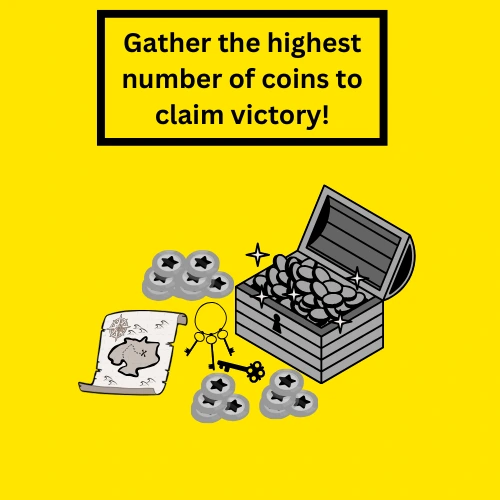
You are designing a scoring system for a treasure hunt game. Each player collects gold coins during the game, and their final score is calculated based on the number of coins they collect. Each gold coin contributes 10 points to the player’s score. Additionally, players earn a bonus of 50 points for every full set of 25 coins they gather. Write a program in C language that calculates the total score for a player.
Test Case
Input
Enter the number of coins collected: 15
Output
Total score: 150
- The program takes the number of coins collected by the player as input and stores it in the
coins_collected
variable. - Then the program calculates the
base_score
by multiplying thecoins_collected
by 10. - The
bonus_points
are calculated by first dividing the number of coins by 25. This will find out that how many complete sets of 25 coins are earned. Then these complete sets are multiplied by 50 to calculate the total bonus points. Players that have collected less than 25 coins will not get any bonus points. - The
total_score
is calculated by adding thebase_score
and thebonus_points
. - Then the
total_score
is displayed usingprintf
.
#include <stdio.h> int main() { int coins_collected; printf("Enter the number of coins collected: "); scanf("%d", &coins_collected); int base_score = coins_collected * 10; int bonus_points = (coins_collected / 25) * 50; int total_score = base_score + bonus_points; printf("Total score: %d\n", total_score); return 0; }
Space Mission Fuel Optimization
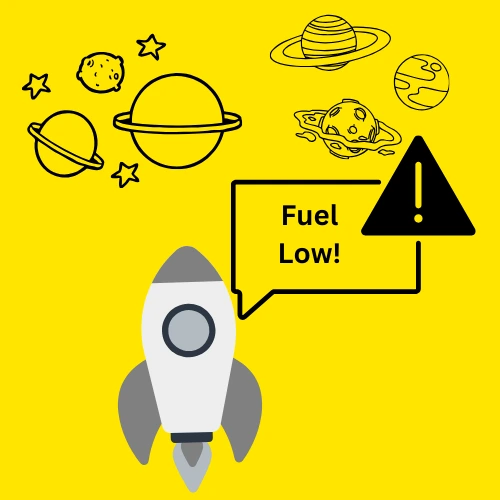
You are a scientist working on a space mission, and your job is to figure out how much fuel the spacecraft needs to reach a distant planet. The process has a few steps. First, you calculate the base fuel by multiplying the spacecraft’s weight (in tons) by the square of the distance to the planet (in million kilometers). Then, you adjust this base fuel by dividing it by the engine’s efficiency. To make sure the spacecraft is safe, you add a margin, which is found by multiplying the weight, distance, and efficiency together. Finally, you find the total fuel needed by subtracting the sum of the weight, distance, and efficiency from the adjusted fuel. Write a C program to do these calculations and find the total fuel required for the mission.
Test Case
Input
Enter the weight of the spacecraft (in tons): 10
Enter the distance to the planet (in million kilometers): 5
Enter the efficiency factor of the engine: 2
Expected Output
Total fuel required: 208
- First of all, the program takes the weight, distance and efficiency of the space craft from the user and stores it in
weight
,distance
andefficiency
variables respectively. - To find the
base_fuel
the program multiplies weight with the square of distance. The square of an integer variable can be calculated by multiplying it with itself. - Then the
base_fuel
is divided byefficiency
factor and the result is stored inadjusted_fuel
. - The
safety_margin
is calculated by multiplyingweight
,distance
and theefficiency
factor of the space craft. - The
total_fuel
required is calculated by adding theadjusted_fuel
andsafety_margin
and then subtracting the sum ofweight
,distance
andefficiency
from it.
#include <stdio.h> int main() { int weight; int distance; int efficiency; printf("Enter the weight of the spacecraft (in tons): "); scanf("%d", &weight); printf("Enter the distance to the planet (in million kilometers): "); scanf("%d", &distance); printf("Enter the efficiency factor of the engine: "); scanf("%d", &efficiency); int base_fuel = weight * (distance * distance); int adjusted_fuel = base_fuel / efficiency; int safety_margin = weight * distance * efficiency; int total_fuel = adjusted_fuel + safety_margin - (weight + distance + efficiency); printf("Total fuel required: %d\n", total_fuel); return 0; }
Bank Transaction Code Analyzer
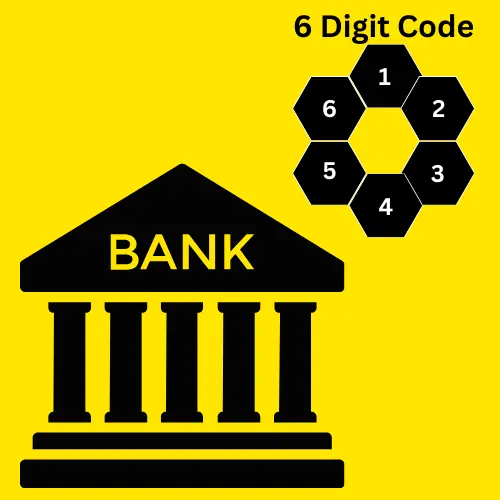
Imagine that you are working for a banking system, and your job is to make it easier to analyze transaction codes. Each transaction code is a six-digit number, where every digit holds specific information about the transaction. To simplify the process, you need a way to break down these codes, understand their details, and perform basic calculations. Write a program in C that reads a six-digit transaction code from the user, separates it into individual digits, then calculates the sum and product of those digits, and finally displays the results.
Test Case
Input
Enter a six-digit transaction code: 123456
Expected Output
Digits: 1 2 3 4 5 6
Sum of digits: 21
Product of digits: 720
- First of all, the program takes the six-digit transaction code as input from the user and stores it in the
code
variable - To find the first digit, the program divides the
code
by 100000. This gives the digit in the hundred-thousands place. - To find the second digit, the program divides the
code
by 10000 and then calculates the remainder when divided by 10. This gives the digit in the ten-thousands place. - The third digit is found by dividing the
code
by 1000 and then taking the remainder when divided by 10. This gives the digit in the thousands place. - To find the fourth digit, the program divides the
code
by 100 and takes the remainder when divided by 10. This gives the digit in the hundreds place. - For the fifth digit, the program divides the
code
by 10 and takes the remainder when divided by 10. This gives the digit in the tens place. - Finally, the sixth digit is obtained by taking the remainder of the
code
when divided by 10. This gives the digit in the units place. - The sum of all six digits is calculated by adding them together and the product of the digits is calculated by multiplying all six digits.
- Finally, the program displays each digit, the sum of the digits, and the product of the digits using
printf
.
#include <stdio.h> int main() { int code; printf("Enter a six-digit transaction code: "); scanf("%d", &code); int d1 = code / 100000; int d2 = (code / 10000) % 10; int d3 = (code / 1000) % 10; int d4 = (code / 100) % 10; int d5 = (code / 10) % 10; int d6 = code % 10; int sum = d1 + d2 + d3 + d4 + d5 + d6; int product = d1 * d2 * d3 * d4 * d5 * d6; printf("Digits: %d %d %d %d %d %d\n", d1, d2, d3, d4, d5, d6); printf("Sum of digits: %d\n", sum); printf("Product of digits: %d\n", product); return 0; }
Math Puzzle Challenge
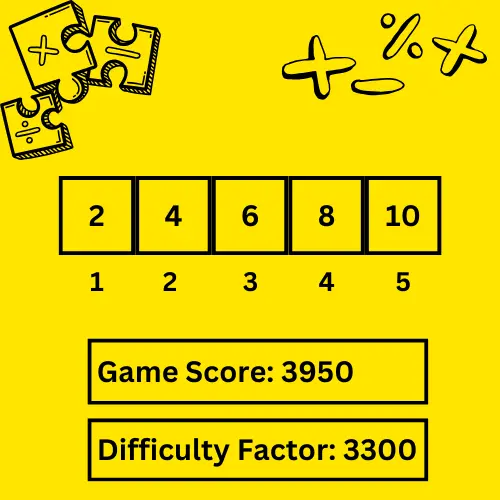
Imagine that you are designing a math-based game for students in which they will solve puzzles by entering a sequence of numbers. The game will evaluate the sequence to find two outcomes. The first outcome is the game score and the second outcome is the difficulty factor. This program should work on a sequence of 5 numbers. The weighted sum of the sequence is calculated by multiplying each number by its fixed position in the sequence and adding these values together. Additionally, the program calculates the product of all five numbers. The game score is then determined by adding the weighted sum and the product, and taking the remainder when divided by 100003 (a prime number). The difficulty factor is found by multiplying the total of all the numbers by their weighted sum, and then dividing the result by 99991 to get the remainder.
Write a program in C that calculates and displays the game score and difficulty factor based on the sequence of numbers entered.
Test Case
Input
Enter the first positive integer: 2
Enter the second positive integer: 4
Enter the third positive integer: 6
Enter the fourth positive integer: 8
Enter the fifth positive integer: 10
Expected Output
Game Score: 3950
Difficulty Factor: 3300
- The program starts by asking the user to input five positive integers at a time. Each number is stored in a separate variable.
- Then the
weighted_sum
is calculated by multiplying each number by its position (1 to 5) and summing up these values. - The
product
of the numbers is calculated by multiplying all five values together. - The
game_score
is calculated by adding theweighted_sum
and theproduct
. After that we find it’s reminder when divided by100003. - The
difficulty_factor
is determined by multiplying thetotal_sum
of the numbers by theirweighted_sum
, then taking the remainder when divided by 99991. - Finally, the program outputs both the
game_score
and thedifficulty_factor
usingprintf
.
#include <stdio.h> int main() { int n1, n2, n3, n4, n5; printf("Enter the first positive integer: "); scanf("%d", &n1); printf("Enter the second positive integer: "); scanf("%d", &n2); printf("Enter the third positive integer: "); scanf("%d", &n3); printf("Enter the fourth positive integer: "); scanf("%d", &n4); printf("Enter the fifth positive integer: "); scanf("%d", &n5); int weighted_sum = n1 * 1 + n2 * 2 + n3 * 3 + n4 * 4 + n5 * 5; int total_sum = n1 + n2 + n3 + n4 + n5; int product = n1 * n2 * n3 * n4 * n5; int game_score = (weighted_sum + product) % 100003; int difficulty_factor = (total_sum * weighted_sum) % 99991; printf("Game Score: %d\n", game_score); printf("Difficulty Factor: %d\n", difficulty_factor); return 0; }