Strings play a big role in programming, but sometimes, you need to verify if a string is empty before using it. In this article, we’ll look at different approaches to check if a string is empty in Python. We’ll also discuss the differences between null, blank, and empty strings.
- Difference Between Empty, Blank, and Null Strings
- 9 Quick Methods to Determine If a String is Empty in Python
- Method 1: Using the NOT Operator
- Method 2: Using an Equality Operator (==)
- Method 3: Using Ternary Operators
- Method 4: Using Slicing
- Method 5: Using the len() Function
- Method 6: Using the bool() Function
- Method 7: Using Regular Expressions
- Method 8: Using a Try-Except Block
- Method 9: Using a Custom Function
- Working with None and Empty Strings in Python
- Handling Empty Strings with Extra Spaces (Blank Strings)
- Real-World Uses of Checking for Empty Strings
- Conclusion
- Related Python How-to Articles
Strings are a sequence of characters. They are the backbone of text manipulation in Python. Sometimes, we need to perform specific operations on strings to suit our needs, and one such task is handling empty strings. But what’s the difference between empty, blank, and null strings? Let’s dive in!
Difference Between Empty, Blank, and Null Strings
Imagine a box that can hold letters or numbers. If the box is empty, it doesn’t have anything inside. Similarly, an empty string means no characters are present, not even a space. In Python, it’s written as two quotes with nothing in between: ""
.
On the other hand, a null string doesn’t exist at all, and a blank string might only appear empty but could contain spaces or invisible characters.
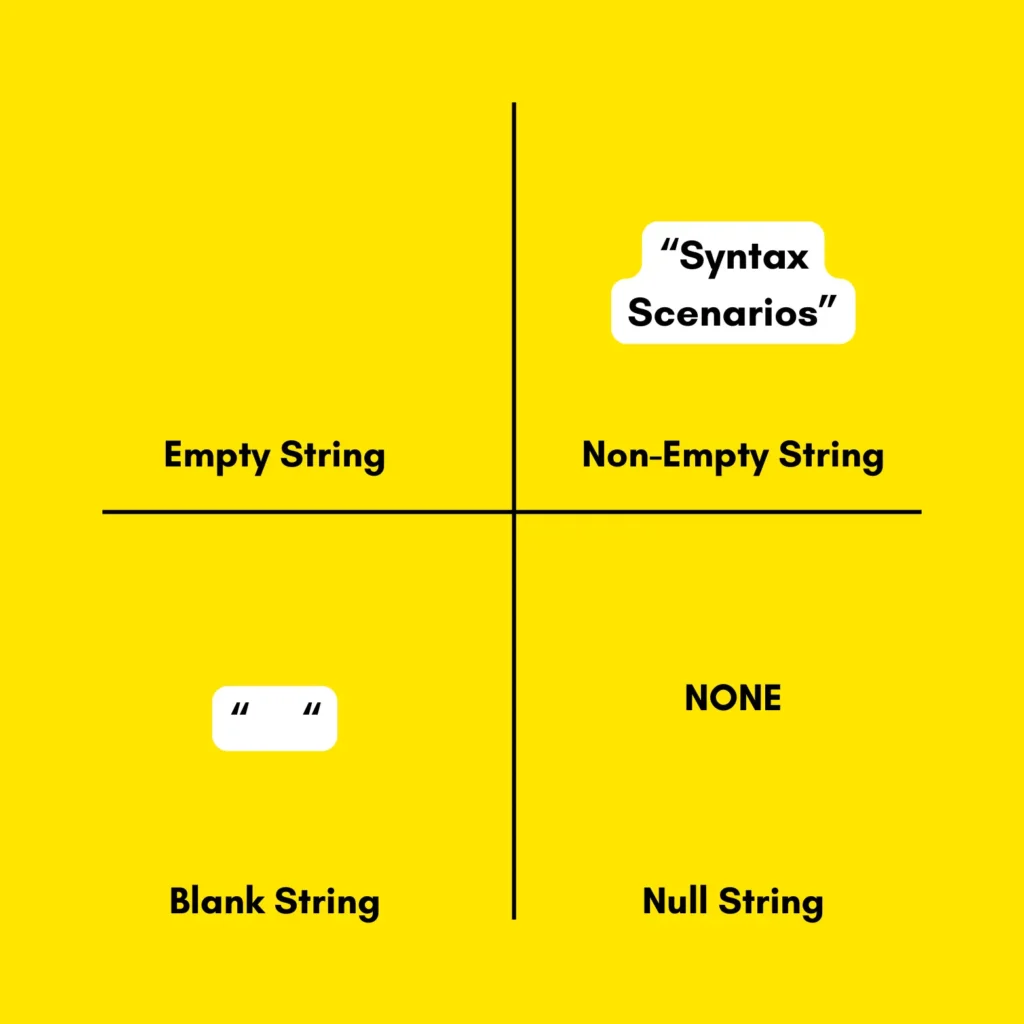
Example Code
This code shows three types of strings: an empty string (emptyString = ""
), a string with spaces (blankString = " "
), and a null value (nullString = None
). When we print emptyString
, nothing will show up, blankString
will show spaces and nullString
will print None
.
emptyString = "" blankString = " " nullString = None print("Output of emptyString:", emptyString) print("Output of blankString:", blankString) #Spaces can't be seen but they are present there print("Output of nullString:", nullString)
Output
Output of emptyString: Output of blankString: Output of nullString: None
An empty string is just one way to represent a lack of value, and understanding how variables store such values is key to mastering Python.
9 Quick Methods to Determine If a String is Empty in Python
You have plenty of options in Python to check whether a string is empty. Let’s go through each one with simple code examples.
Method 1: Using the NOT Operator
An empty string is considered False
in Python. This allows you to directly check if a string is empty by simply evaluating its truth value using the not
operator.
Example Code
Here, the not
operator flips the truth value of the variable str_text
. Since an empty string evaluates to False
, not str_text
becomes True
, and the program prints “Your string is empty.”
str_text = "" if not str_text: print("Your string is empty.") else: print("Your string is not empty.")
Output
Your string is empty.
Method 2: Using an Equality Operator (==)
Another straightforward method to check for an empty string is by comparing it with ""
. It explicitly shows what you are checking for. A string is treated as empty if it is the same as ""
.
Example Code
In this example, the program compares str_text
with an empty string using the equality operator ==
. Since the two strings are identical, Python would print “Your string is empty.”
str_text = "" if str_text == "": print("Your string is empty.") else: print("Your string is not empty.")
Output
Your string is empty.
Method 3: Using Ternary Operators
Ternary operators let you check conditions in a single-line format, making your code more concise and readable. They are especially useful when you want to write quick checks without using multiple lines of if-else statements.
Example Code
This code example uses a ternary operator to evaluate not str_text
and eventually, prints “Your string is empty.”
str_text = "" print("Your string is empty." if not str_text else "Your string is not empty.")
Output
Your string is empty.
Method 4: Using Slicing
You can use slicing to extract parts of a string or to check if it’s empty by comparing the slice to an empty string (""
).
Example Code
In this code, str_text[:]
creates a slice of the string from the start to the end. If the slice equals ""
, the program determines that the string is empty and prints “Your string is empty.”
str_text = "" if str_text[:] == "": print("Your string is empty.") else: print("Your string is not empty.")
Output
Your string is empty.
Method 5: Using the len() Function
The len()
function tells a string’s length (no. of characters in a string). A string would be empty if the len()
results in 0
.
Example Code
In the following code, len(str_text)
will return 0
because there are no characters in the string. That means the string is empty.
str_text = "" if len(str_text) == 0: print("Your string is empty.") else: print("Your string is not empty.")
Output
Your string is empty.
Method 6: Using the bool() Function
Python’s bool()
function evaluates the truth value of an object. In the case of an empty string, bool()
will return False
, and for non-empty strings, it will return True
.
Example Code
Here, in this code, bool(str_text)
checks the truth value of the string. In our case, that is going to be False
and the program prints, “Your string is empty.”
str_text = "" if not bool(str_text): print("Your string is empty.") else: print("Your string is not empty.")
Output
Your string is empty.
Method 7: Using Regular Expressions
While regular expressions (regex) are often used for complex patterns like truncating strings in Python, you can also use them for simple tasks like checking if a string is empty.
Example Code
As seen in the code below, the re.fullmatch(r"", str_text)
method is used to check if str_text
is exactly empty. The pattern r""
matches a string with no characters at all. While the match is successful, the program prints, “Your string is empty.”
import re str_text = "" if re.fullmatch(r"", str_text): print("Your string is empty.") else: print("Your string is not empty.")
Output
Your string is empty.
Method 8: Using a Try-Except Block
A try-except block can handle unexpected catches and errors. It can also be used to check if a string is empty in Python, ensuring your program doesn’t crash when something goes wrong.
Example Code
In the code below, the program checks the truth value of the variable txt_str
. Then, based on the truth value, the program prints whether the string is empty or not.
str_text = "" try: if not str_text: print("The string is empty.") else: print("The string is not empty.") except TypeError: print("An error occurred.")
Output
The string is empty.
Method 9: Using a Custom Function
A custom function allows you to create a centralised logic for checking if a string is empty. This is useful if you need to perform this check multiple times throughout your program, as it allows you to reuse the same logic without duplicating code.
Example Code
In the code provided below, the function is_empty()
checks whether a string is empty and then prints the result.
def is_empty(string): if string == "": return "The string is empty." else: return "The string is not empty." str_txt = "" print(is_empty(str_txt))
Output
The string is empty.
Working with None and Empty Strings in Python
Sometimes, a string might not just be empty; it could also be None
. This means the string doesn’t exist at all. You can handle both cases by checking for None
and then if the string is empty.
Imagine it as a shelf in a store. If the shelf doesn’t exist (None
), you can’t check whether it has any items on it. But if the shelf exists (not None
), you can check whether it’s empty or stocked with products.
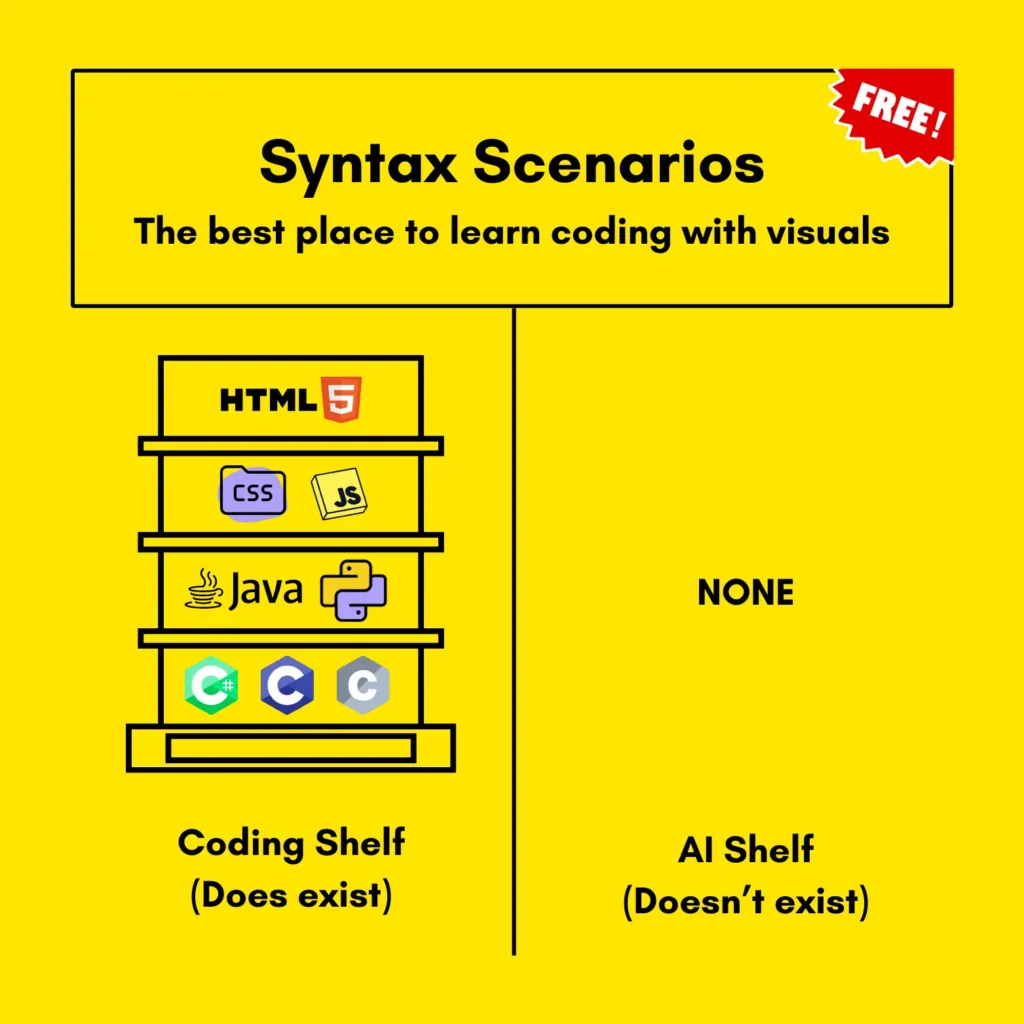
Example Code
In this code, the program first checks if str_txt
is None
. If not, then it checks if the string is empty. This handles both cases effectively.
str_txt = None if str_txt is None: print("Your string is null.") elif str_txt == "": print("Your string is empty.") else: print("The string isn't empty.")
Output
Your string is null.
Handling Empty Strings with Extra Spaces (Blank Strings)
Sometimes, strings contain extra spaces or unwanted characters like newline characters, which can make them seem empty. You can use the strip()
method to remove any spaces in the string before checking its emptiness. This technique is particularly useful for identifying blank strings when user input has accidental spaces.
Example Code
In this code, str_txt.strip()
removes all blank spaces from the string. The string becomes empty then.
str_text = " " if str_text.strip() == "": print("Your given string is empty.") else: print("Your given string is not empty.")
Output
Your given string is empty.
Real-World Uses of Checking for Empty Strings
In programming, checking for empty strings is useful in various real-world scenarios. It helps you ensure that your code runs smoothly and provides the correct output. Because you can:
- Ensure users don’t leave fields blank by checking for empty strings in forms.
- Skip empty lines while reading files to avoid processing unnecessary data.
- Remove empty strings from lists or logs to keep your data clean and meaningful.
- Avoid running unnecessary operations by checking if a string is empty before processing it.
- Prevent sending empty messages or emails by validating the content before sending.
Conclusion
Checking if a string is empty in Python is a simple yet essential task in programming. It helps in ensuring smooth operations, especially when working with user inputs, files, and data processing. You can easily handle empty strings and avoid potential errors by using various methods like the if statement, len(), regular expressions, and custom functions.
Now, you are more confident in choosing the best method for your specific needs, making your code more efficient and reliable.
For more easy-to-understand articles on Python programming with beginner-friendly analogies, explore our Python tutorial series at Syntax Scenarios.
I love your blog.. very nice colors & theme. Did you create
this website yourself or diid you hire someone to do
it for you? Plz answsr back as I’m looking to construct
my oown blog and would like to find out where u got this from.
cheers http://boyarka-Inform.com/
Thanks! It was made by my student: https://moosamuhyuddin.com/