If you’ve ever worked with lists in Python, you’ve probably needed to compare them to see what’s different. Whether you’re looking for missing items, checking for duplicates, or verifying that the values in two lists match, comparing lists is a common task in many real-world applications. In this article, we’ll explore different ways to compare lists in Python and identify their differences.
Why Do We Need to Compare Lists in Python?
Let’s say you run an online bookstore. You have a list of books currently in stock and another list of books that customers have ordered. To fulfil the orders correctly, you need to identify:
- Books that customers ordered but aren’t in stock.
- Extra books in stock that no one ordered.
This is exactly where Python list comparison is useful. It helps automate such comparisons quickly!
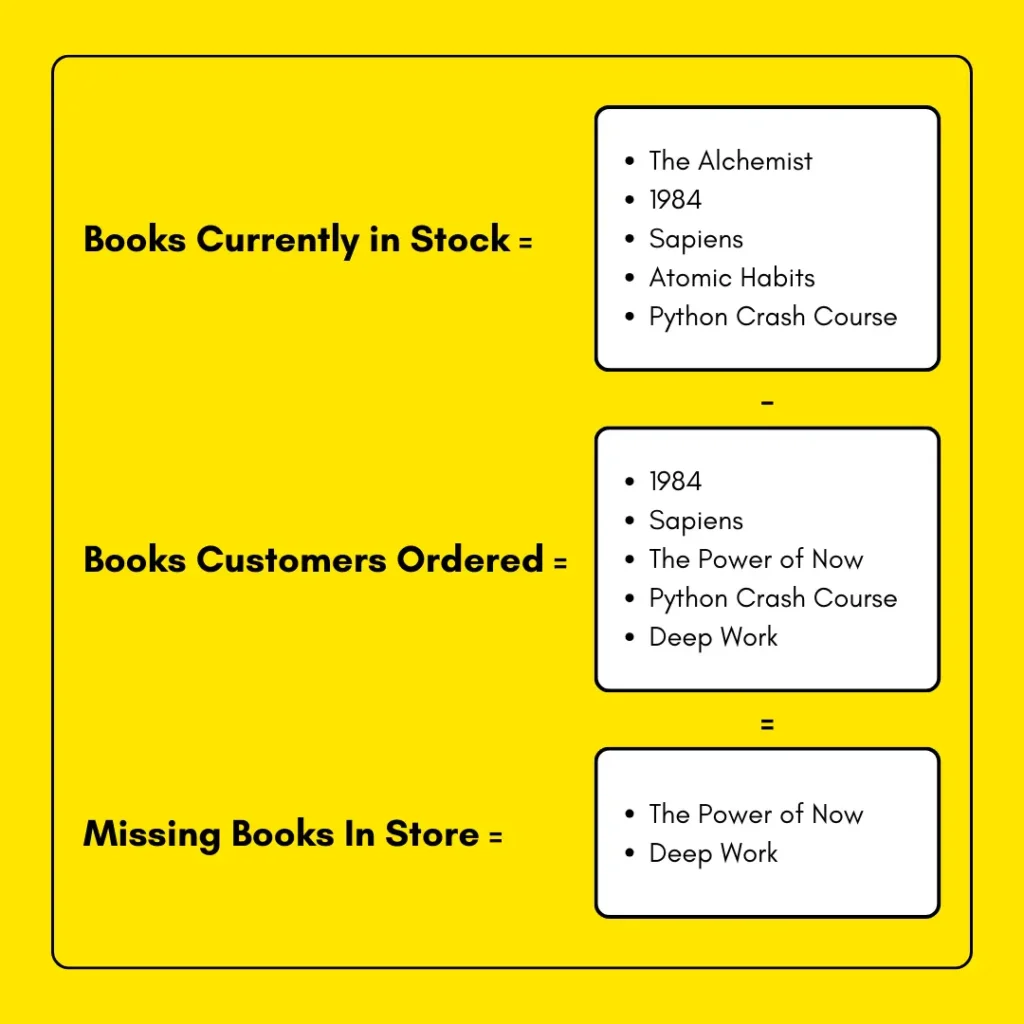
Different Methods to Compare Two Lists and Find Their Differences
1. Using set() for Symmetric and Asymmetric Differences
One of the simplest and fastest ways to compare two lists in Python is by converting them into sets. The power of sets comes from their ability to eliminate duplicates and directly compare the values between them. This method is ideal for Python list difference operations, as it is simple and efficient.
- Asymmetric difference finds items present in one list but not in the other.
- Symmetric difference finds items that are unique in both lists.
Example Code
In this example, we first create two lists, list1
and list2
, containing a few numbers. The expression set(list1) ^ set(list2)
returns items that are not common to both lists. The expression set(list1) - set(list2)
returns items present in list1
but not in list2
. We then assign the results to symmetric_diff
and asymmetric_diff
, respectively, and finally print the results.
list1 = [1, 2, 3, 4, 5] list2 = [4, 5, 6, 7, 8] # Symmetric difference (elements unique to both lists) symmetric_diff = set(list1) ^ set(list2) # Asymmetric difference (elements in list1 but not in list2) asymmetric_diff = set(list1) - set(list2) # Print the differences print("Symmetric Difference:", symmetric_diff) print("Asymmetric Difference:", asymmetric_diff)
Output
Symmetric Difference: {1, 2, 3, 6, 7, 8} Asymmetric Difference: {1, 2, 3}
2. Using filter() Function
The filter() function allows for a more controlled way to compare list A and B and extract non-matching values. This approach works best when you want to define a custom rule to filter out matching or non-matching values from the lists.
Example Code
Here, we have two lists, list1
and list2
. The filter()
function uses a lambda to check if an item from list1
is not in list2
, returning the items that are only in list1
and printing the result.
list1 = [1, 2, 3, 4, 5] list2 = [4, 5, 6, 7, 8] # Finding items in list1 that aren't in list2 diff = list(filter(lambda item: item not in list2, list1)) # Print the difference print("Items in list1 but not in list2:", diff)
Output
Items in list1 but not in list2: [1, 2, 3]
3. Using collections.Counter
For lists with duplicate elements, Counter from the collections module is a great tool for list comparison in Python.
Example Code
In this example code, we first import Counter
from the collections
module. Two lists, list1
and list2
, are defined, containing some overlapping values. The Counter
function counts the occurrences of each element in both lists. The expression counter1 - counter2
calculates the difference between the two counters, returning the elements that are in list1
but not in list2
, which are stored in diff
. The result stored in diff
is then printed.
from collections import Counter list1 = [1, 2, 3, 4, 4] list2 = [3, 4, 4, 5, 6] # Count occurrences of each element in both lists counter1 = Counter(list1) counter2 = Counter(list2) # Finding the difference between two counters diff = counter1 - counter2 # Print the difference print("Items in list1 but not in list2:", diff)
Output
Items in list1 but not in list2: Counter({1: 1, 2: 1})
As you can see in the output, Counter({1: 1, 2: 1})
shows that the elements 1
and 2
are present in list1
but not in list2
, and each element occurs once.
4. Using List Comprehension
List comprehension is one of the most Pythonic ways to filter and compare two lists. It’s concise, easy to read, and perfect for tasks like comparing values between lists.
Example Code
The following code iterates through each element in list1
and checks if the item is not present in list2
using the condition if item not in list2
. If the condition is true, the item is added to diff
, which is then printed.
list1 = [1, 2, 3, 4, 5] list2 = [4, 5, 6, 7, 8] # Items in list1 but not in list2 diff = [item for item in list1 if item not in list2] # Print the difference print("Items in list1 but not in list2:", diff)
Output
Items in list1 but not in list2: [1, 2, 3]
5. Using in Operator with a for Loop
A classic method to compare list A and B is using a for loop with the in operator.
Example Code
In this code, the for
loop iterates through each item in list1
and checks if it’s present in list2
using the in
operator. If the item is not found in list2
, it is added to the diff
list using the append()
method. Finally, the diff
list is printed to the console.
list1 = [1, 2, 3, 4, 5] list2 = [4, 5, 6, 7, 8] # Empty list to store the differences diff = [] # Iterate through each item in list1 for item in list1: # Check if the item is not in list2 if item not in list2: # Add the item to the diff list diff.append(item) # Print the difference print("Items in list1 but not in list2:", diff)
Output
Items in list1 but not in list2: [1, 2, 3]
6. Using NumPy for List Comparison
For handling large lists efficiently, NumPy provides an optimized way to perform list difference operations. This method is particularly useful when dealing with Python difference between two lists in large datasets.
Example Code
Here, we first import numpy
and define two lists, list1
and list2
. We then convert these lists into arrays, array1
and array2
, using np.array
. The function np.setdiff1d(array1, array2)
is then used to find the elements that are in array1
but not in array2
. The result is stored in the diff
variable, which is then printed to the screen.
import numpy as np list1 = [1, 2, 3, 4, 5] list2 = [4, 5, 6, 7, 8] # Convert lists into arrays array1 = np.array(list1) array2 = np.array(list2) # Finding differences using numpy setdiff1d diff = np.setdiff1d(array1, array2) # Print the difference print("Items in list1 but not in list2:", diff)
Output
Items in list1 but not in list2: [1 2 3]
Conclusion
Now you know multiple ways to compare two lists in Python and find their differences. Whether you prefer using sets, list comprehensions, or NumPy, each method has its own advantages.
- Use
set()
for quick unordered comparisons. - Use
filter()
for a functional approach. - Use
Counter
when working with duplicate values. - Use list comprehension or loops for simplicity.
- Use
NumPy
when working with large datasets.
For more easy-to-follow Python tutorials, be sure to check out our Python Series at Syntax Scenarios!