If you frequently work with data or manipulate arrays in Python, you’re more likely to be familiar with boolean arrays. These arrays contain only True
or False
values and help filter data, perform comparisons, and execute element-wise logical operations. In this article, we’ll explore how to create a boolean array and the various ways to combine two boolean arrays in NumPy. Let’s get started!
Why Combine Boolean Arrays
Imagine you’re organizing a sports event with a bunch of people, and you have two lists:
- List 1 shows who’s available to play
- List 2 shows who have the required skills.
Now, to form your team, you need to combine these lists. If someone is both available and has the required skills, they’ll play. However, if they’re available but lack the necessary skills, they’ll simply enjoy the game by watching it. If they have the skills but aren’t available, sadly, they won’t be able to enjoy the match either.
Combining boolean arrays in NumPy is similar to filtering data based on multiple conditions. This helps you quickly identify which elements meet all (or some) of the criteria.
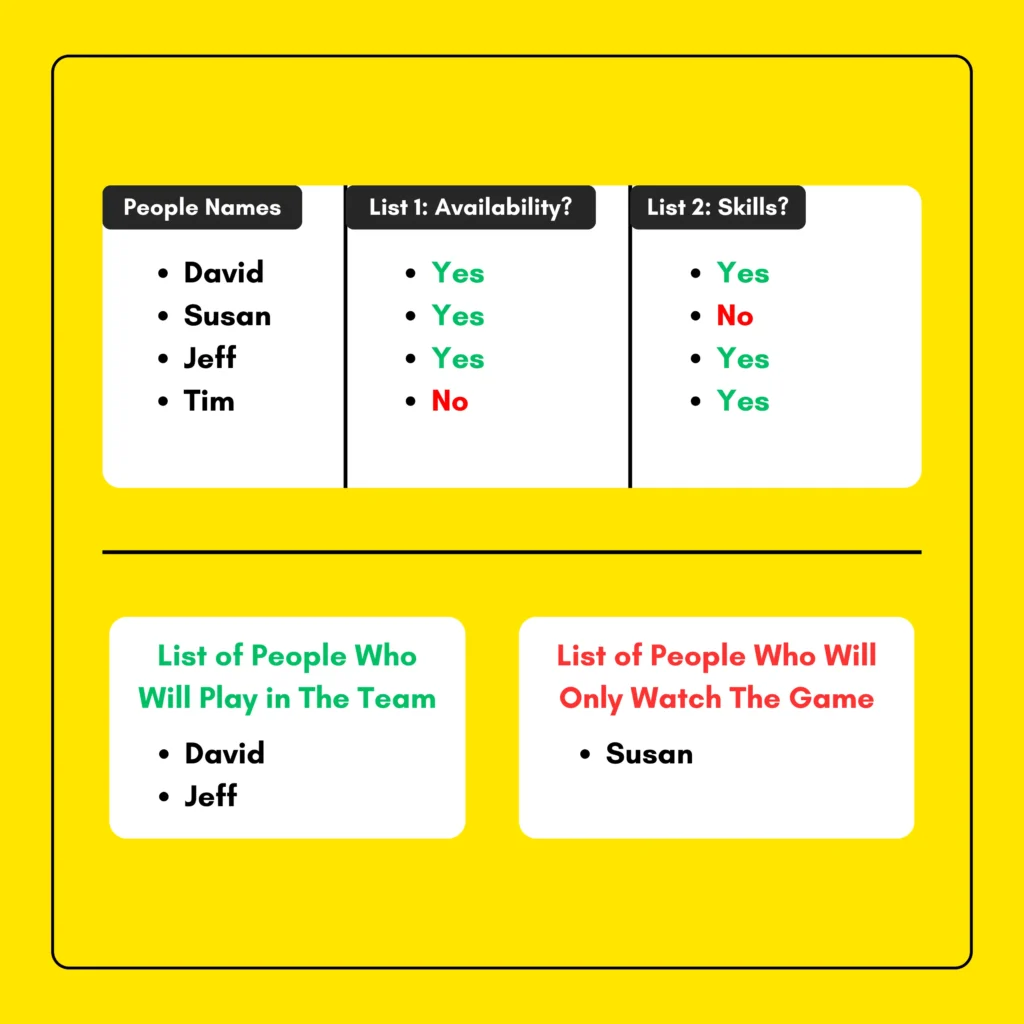
Creating a Boolean Array with Numpy’s np.array()
Before combining boolean arrays, you first need to create them. Python’s NumPy library provides the np.array()
function for this. You can initialize a Boolean array by passing a list of True
and False
values directly to np.array()
.
Example Code
As you can see in the example below, we use np.array()
to create a boolean array from a list of True
and False
values and print it to the output screen to see our newly created boolean array.
import numpy as np # Create a boolean array with True and False values bool_array = np.array([True, False, True, False]) print(bool_array)
Output
[ True False True False ]
3 Different Ways to Combine Two Boolean Arrays in NumPy
1. Using Bitwise OR Operator
If you want an element to be True
when at least one of the corresponding elements in two arrays is True
, you can use the OR (|
) operator. Simply put, the OR operator is useful when you want at least one of your conditions to be True
.
Example Code
- In this example code, we first create two boolean arrays,
array1
andarray2
. - Then, we use the OR (
|
) operator to combine these arrays. - Finally, we print the result, which is a new boolean array.
import numpy as np # Create two boolean arrays array1 = np.array([True, False, True, False]) array2 = np.array([False, False, True, True]) # Combine arrays using the OR operator result = array1 | array2 print(result)
Output
[ True False True True ]
2. Using Bitwise AND Operator
The AND (&
) operator combines two boolean arrays and returns True
only if both elements at a particular position are True
. Unlike the OR operator, The AND operator is useful when you want both of your conditions to be True
.
Example Code
- Similar to the OR operation, we first create two boolean arrays.
- Next, we use the AND (
&
) operator to combine them. - Finally, we print the resulting boolean array.
import numpy as np # Create two boolean arrays array1 = np.array([True, False, True, False]) array2 = np.array([False, False, True, True]) # Combine arrays using the AND operator result = array1 & array2 print(result)
Output
[ False False True False ]
The AND operator works like finding common elements between two lists. If a value is True
in both lists, the result is True
. However, if either list has False
for that value, the result is False
, much like how we identify common values when comparing lists.
3. Using np.logical_or() and np.logical_and() for More Readable Code
If you prefer a more readable approach, you can use Numpy’s built-in np.logical_or()
and np.logical_and()
functions. These functions work the same way as the |
and &
operators but can improve code readability, especially in more complicated scenarios where you’re combining multiple boolean arrays.
Example Code
- Here, we first create two boolean arrays,
array1
andarray2
. - The
np.logical_or(array1, array2)
function performs the OR operation between the arrays. - The
np.logical_and(array1, array2)
function performs the AND operation between the arrays. - Finally, we print the resulting boolean arrays.
import numpy as np # Create two boolean arrays array1 = np.array([True, False, True, False]) array2 = np.array([False, False, True, True]) # Combine arrays using np.logical_or result_or = np.logical_or(array1, array2) # Combine arrays using np.logical_and result_and = np.logical_and(array1, array2) print("OR result:", result_or) print("AND result:", result_and)
Output
OR result: [ True False True True ] AND result: [ False False True False ]
A Practical Example of Combining Boolean Arrays in NumPy
Boolean arrays are often used in data filtering. For example, let’s say you have two arrays containing the employees’ ages and salaries, and you want to filter employees who meet two conditions:
- Employees older than 30
- Employees with a salary over $50,000
We can combine these two boolean arrays to find the employees that meet both criteria. Here’s how:
import numpy as np # Sample data ages = np.array([25, 45, 30, 35, 40, 28]) salaries = np.array([45000, 60000, 55000, 40000, 70000, 48000]) # Conditions age_condition = ages > 30 salary_condition = salaries > 50000 # Combine conditions eligible_employees = age_condition & salary_condition print("Eligible employees:", eligible_employees)
Output
The output shows which employees are eligible: False
means not eligible, while True
means eligible. For example, Employee 1 is not eligible, while Employees 2, 4, and 5 meet both conditions.
Eligible employees: [ False True False True True False ]
After filtering the data, you can also write the resulting list to a file if you need to store it for further processing.
Wrapping Up
In this article, we learned how to create boolean arrays using NumPy and why they are useful for various data processing tasks, such as data filtering. We also covered different methods to combine two boolean arrays:
- Bitwise OR operator (
|
) combines two boolean arrays and returnsTrue
if at least one element isTrue
at each position. - Bitwise AND operator (
&
) combines two boolean arrays and returnsTrue
only if both elements areTrue
at each position. np.logical_or()
performs the OR operation between two boolean arrays, providing a more readable approach.np.logical_and()
performs the AND operation between two boolean arrays, offering clear code for multiple conditions.
So, the next time you work with boolean arrays in NumPy, these techniques will make your life much easier!
For more beginner-friendly tutorials on Python programming, don’t forget to check out the dedicated Python series at Syntax Scenarios.