Working with data in pandas often involves adjusting your DataFrame’s columns – adding new ones, renaming them for clarity, changing their order, or removing those you no longer need. For beginner Python programmers, these operations are fundamental to data cleaning and feature engineering.
In this tutorial, we’ll walk through how to add, rename, reorder, and remove columns in a pandas DataFrame with simple examples. By the end, you’ll know how to manage your DataFrame’s columns effectively – a skill that is useful whether you’re prepping data for analysis, cleaning up a dataset, or creating new features for a machine learning model.
We’ll use a small sample DataFrame to illustrate each operation. Let’s create a simple DataFrame:
Example Code:
import pandas as pd # Sample DataFrame of people df = pd.DataFrame({ 'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35], 'City': ['New York', 'Paris', 'London'] }) print(df)
Output:
Name Age City 0 Alice 25 New York 1 Bob 30 Paris 2 Charlie 35 London
This DataFrame has three columns: Name, Age, and City. Next, we’ll go through each column operation one by one.
Adding Columns to a Pandas DataFrame
Adding a new columns is useful whenever you derive new information from existing data or bring in additional data. For example, you might compute a new column for total sales from price and quantity, or add a country column based on a city column. In our example, let’s add a new column called “Country” to provide more information about each person’s location.
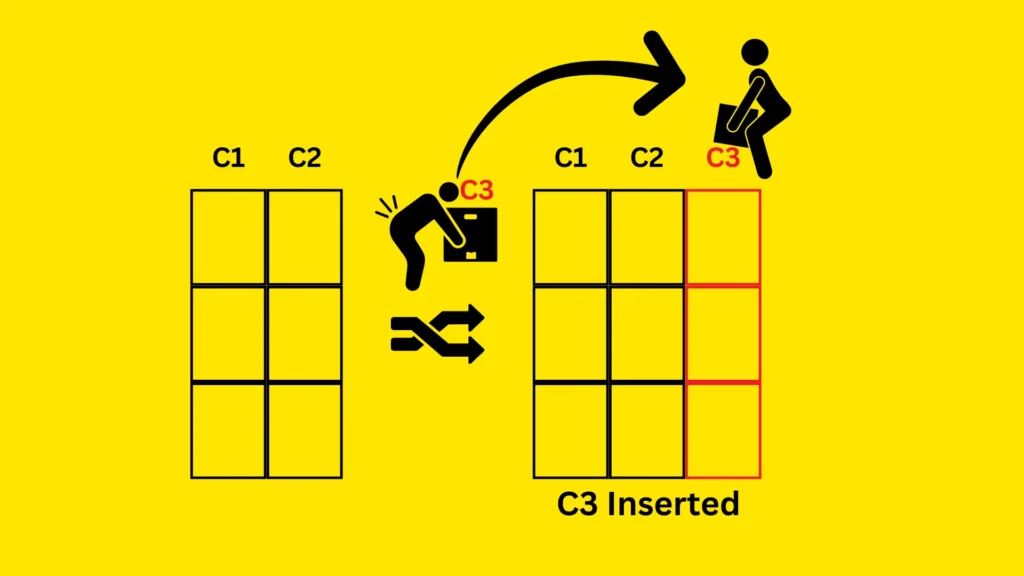
Using Assignment to Add a Column
The easiest way to add a column is by assignment with the bracket notation. You specify the new column name in df[...]
and assign a list or Series of values to it. For instance, to add a Country column:
Example Code:
df['Country'] = ['USA', 'France', 'UK'] print(df)
Output:
Name Age City Country 0 Alice 25 New York USA 1 Bob 30 Paris France 2 Charlie 35 London UK
We simply did df['Country'] = [...]
to add the column. Pandas added Country as a new column at the end of the DataFrame, and each row got the corresponding value from the list. Make sure the list (or array/Series) you assign has the same number of entries as the DataFrame’s rows.
Inserting a Column at a Specific Position (Optional)
By default, the new column appears at the end. If you need to insert a column at a specific position (say as the first column), you can use the DataFrame’s .insert()
method. For example, to insert a column named “ID” at position 0 with values [1, 2, 3]
:
Example Code:
df.insert(0, 'ID', [1, 2, 3])
This would put the ID column as the first column in the DataFrame. Using .insert()
is handy when the order of columns matters immediately upon creation (for instance, if you’re preparing data for output where a certain order is required). We’ll talk more about reordering columns in a moment.
Renaming Columns in a Pandas DataFrame
Column names might need to change for clarity or consistency. Perhaps the original dataset had cryptic column names, or you want to standardize naming conventions (e.g., make them all lowercase, or remove spaces). Renaming columns makes your DataFrame easier to understand and prevents confusion in later analysis.
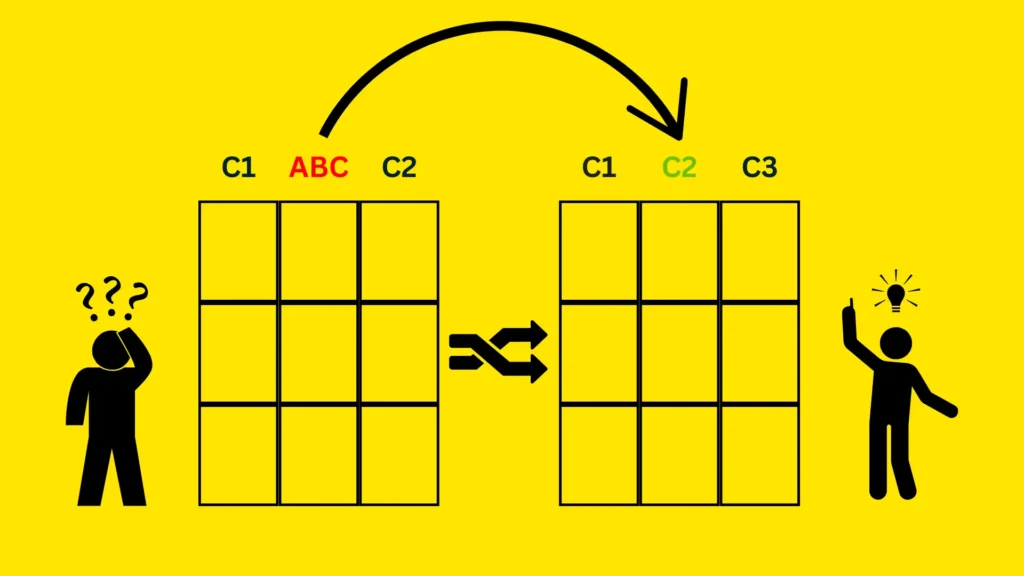
In pandas, you can rename columns using the .rename()
method or by assigning to df.columns
. The .rename()
method is more flexible and is commonly used to rename one or several columns.
Using the .rename()
Method
To rename columns, use df.rename()
with the columns
parameter. Pass a dictionary where keys are current column names and values are the new names. For example, let’s rename the “City” column to “City_Name” for clarity:
Example Code:
# Rename the 'City' column to 'City_Name' df = df.rename(columns={'City': 'City_Name'}) print(df)
Output:
Name Age City_Name Country 0 Alice 25 New York USA 1 Bob 30 Paris France 2 Charlie 35 London UK
Now the column formerly called City is labeled City_Name. We assigned the result back to df
because df.rename()
returns a new DataFrame by default. If you don’t assign it (or use inplace=True
), the original DataFrame won’t be modified – it would just display the renamed version. In this example, we chose to update df
with the renamed columns.
You can rename multiple columns at once by adding more entries to the dictionary. For instance:
df.rename(columns={'Name': 'Full Name', 'Age': 'Age (Years)'})
would rename Name to Full Name and Age to Age (Years) in one go.
Re-ordering Columns in Pandas DataFrame
Sometimes the default order of columns isn’t the most convenient for analysis or presentation. You might want certain columns next to each other for easier comparison (e.g., putting “Country” next to “City_Name”), or you may want the target variable in a dataset to be the last column. Reordering columns doesn’t change the data, but it can make a DataFrame more readable and logical.
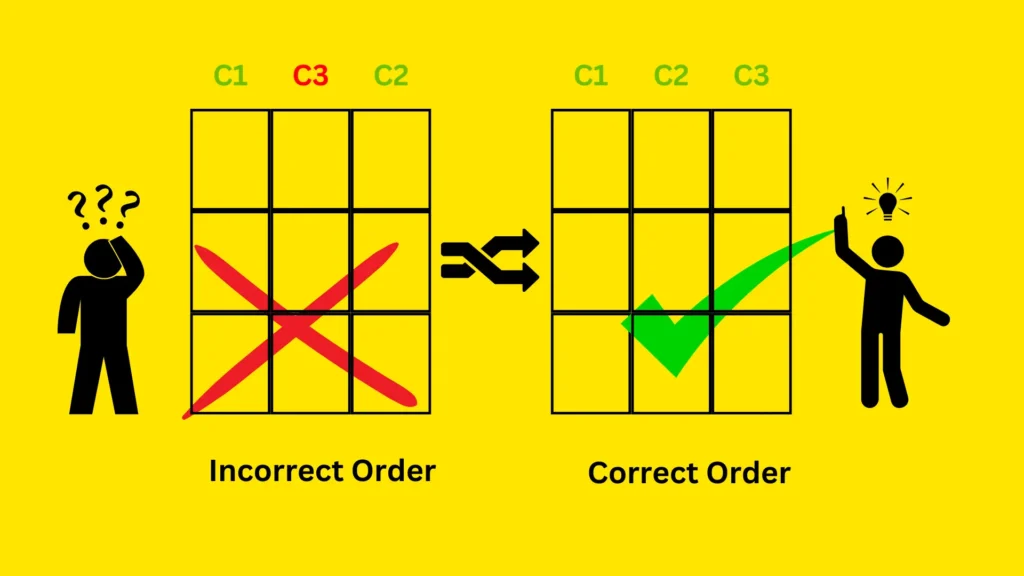
The simplest way to rearrange columns is to reassign the DataFrame with a list of columns in the desired order. Essentially, you’re selecting the columns in the new order using bracket notation.
Using Column Selection to Reorder
Let’s rearrange our DataFrame columns so that Country comes right after Name. Right now our df.columns
are ['Name', 'Age', 'City_Name', 'Country']
. We want ['Name', 'Country', 'City_Name', 'Age']
instead. We can achieve this by slicing the DataFrame with the new column order:
Example Code:
# Reorder columns: put 'Country' after 'Name' df = df[['Name', 'Country', 'City_Name', 'Age']] print(df)
Output:
Name Country City_Name Age 0 Alice USA New York 25 1 Bob France Paris 30 2 Charlie UK London 35
Now the Country column appears immediately after Name, and Age has moved to the end. We created a new ordering by providing a list of column names in the desired sequence.
Another way to reorder is by using df.reindex()
. For example, df = df.reindex(columns=['Name','Country','City_Name','Age'])
accomplishes the same rearrangement. Use whichever approach feels clearer. The direct selection with df[...]
is quick and easy for manual reordering, while reindex
can be useful if you’re programmatically generating the new order.
Removing Columns from a Pandas DataFrame
Removing (dropping) dataframe columns is essential when you have irrelevant or redundant data. Maybe a column is not needed for your analysis (e.g., an extra identifier or an empty column), or you want to drop sensitive information. In pandas, you can delete columns using the .drop()
method or the del
statement. The .drop()
method is versatile and the most commonly used for this purpose.
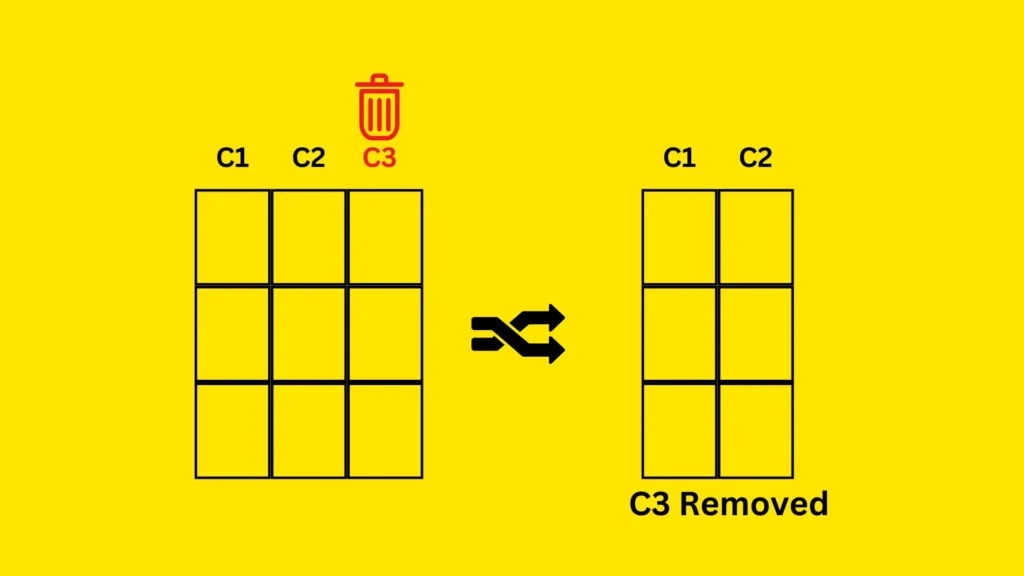
Using the .drop()
Method
To drop columns, use df.drop()
and specify the column name(s). You should also indicate that you’re dropping from columns (not rows) by using axis=1
or the columns
parameter. We will drop the Age column from our DataFrame as an example:
Example Code:
# Remove the 'Age' column df = df.drop(columns=['Age']) print(df)
Output:
Name Country City_Name 0 Alice USA New York 1 Bob France Paris 2 Charlie UK London
Now the DataFrame only has Name, Country, and City_Name; the Age column is gone. We used columns=['Age']
to be explicit that we are dropping a column. (Alternatively, we could write df.drop('Age', axis=1)
for the same result.) Just like with renaming, we assigned the result back to df
because .drop()
returns a new DataFrame by default. If you prefer, you can call df.drop(columns=['Age'], inplace=True)
to drop in place, but be cautious – using inplace=True
means the original DataFrame is modified without returning a new one.
To drop multiple columns in one go, provide a list of column names. For example, df.drop(columns=['Age', 'City_Name'])
would remove both Age and City_Name at once. This is useful in cleaning datasets where you identify several columns to discard (like dropping several low-value features in a machine learning preprocessing step).
Best Practices for Managing DataFrame Columns
Working with pandas DataFrames effectively comes with experience. Here are some best practices and tips for managing columns:
- Use Meaningful Names: Name your columns descriptively. Clear names like
Price_USD
orCustomerID
are better than single letters or unclear abbreviations. This makes your code self-documenting and easier to maintain. - Consistency: Keep a consistent format (all lowercase, or CamelCase, etc.). For example, don’t mix spaces and underscores in names; pick one style (many prefer underscores, e.g.,
first_name
). Consistency helps prevent typos and confusion. - Adding Data: When adding new columns based on calculations, leverage pandas’ vectorized operations (like arithmetic on series) instead of Python loops. It’s not only more concise but also faster. For adding many columns at once, consider using
df.assign()
which can add multiple new columns in one call and is useful in method chaining. - Renaming Carefully: If you plan to use certain columns later (for example, in a plot or merge), make sure to rename them early on for clarity. Keep track of original names in case you need to refer back to documentation or source data. Pandas won’t complain if you accidentally rename to an existing name – it will overwrite, so ensure new names are unique or intended.
- Dropping vs Selecting: To create a DataFrame without certain columns, you can drop the unwanted columns or select the ones you need. For instance,
df[['col1','col2']]
will keep only col1 and col2. This approach can sometimes be clearer when you want to focus on a subset of columns. - Check Results: After any column operation, print out
df.head()
(the first few rows) to verify the DataFrame looks as expected. It’s easy to catch mistakes (like a mis-typed column name leading to no change, or dropping the wrong column) by inspecting the outcome immediately.
By following these practices, you’ll reduce errors and make your data manipulation code more robust and readable.
Conclusion
In this beginner-friendly guide, we covered how to add new columns to a pandas DataFrame, rename existing columns, reorder columns, and remove columns you don’t need. These operations are fundamental for transforming raw data into a cleaner form for analysis or machine learning. As you work with pandas, you’ll find yourself using these column operations frequently – whether it’s creating a new feature column, cleaning up column names, organizing data for output, or dropping irrelevant information.
With the examples above, you should be able to confidently manipulate DataFrame columns. Feel free to experiment with your own datasets: try adding calculated columns, renaming for clarity, reordering columns for different views, and dropping those that aren’t useful. Happy data wrangling with pandas!