In this tutorial, you will learn about data types and variables in C++, and how to declare, initialize, access, and update the variables with practical code snippets and engaging visuals.
Table of Contents
Real-World Analogy
Just like you have a container in the kitchen for storing ingredients, let’s say sugar, the variable is like that container for storing the data. Consider the analogy:
- First, you have an empty container => variable declared
- You add some sugar => assign value to the variable
- You take out the sugar => access the value in the variable
- You replace the amount of sugar => update the value of the variable.
A variable in programming has the same purpose to store, use, and update the data.
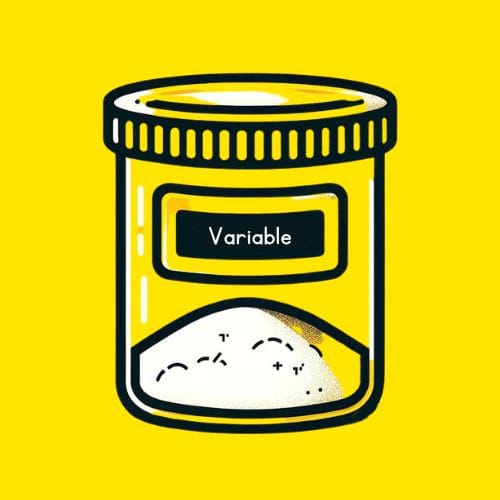
Technically, a variable is a named memory location in RAM that stores the data.
Data Type in C++
A variable can be of different types. Just like you have different containers to store different types and amounts of ingredients, so are the variables.
Data type determines the type, size, and range of the value stored by the variable.
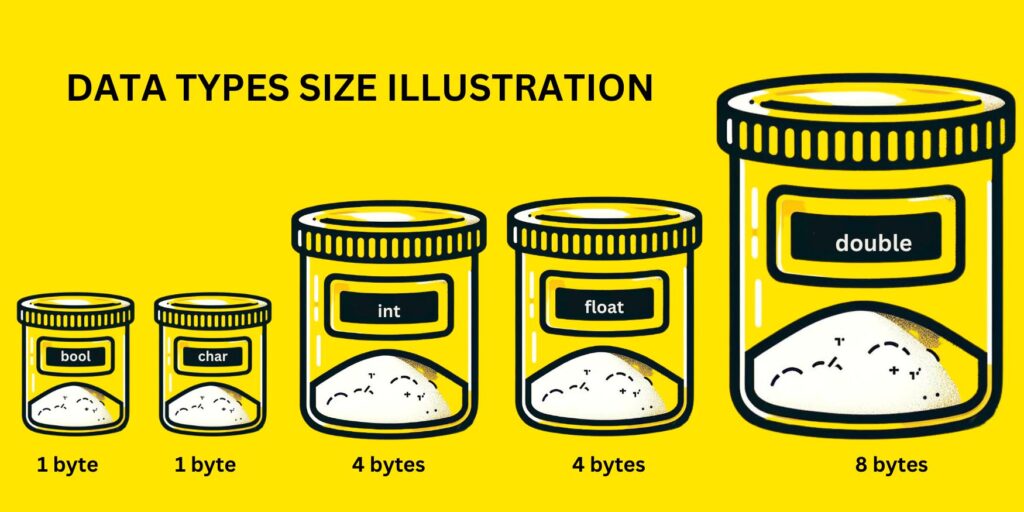
Commonly Used Data Types in C++
Type | Purpose | Size |
bool | Stores either true (1) or false (0) | 1 byte |
char | Stores a single character e.g. ‘a’ | 1 byte |
int | Stores a whole number eg. 123 | 4 bytes |
float | Stores single precision value (up to 7 decimal places) | 4 bytes |
double | Stores single precision value (up to 16 decimal places) | 8 bytes |
Declare a Variable in C++
Declaring a variable tells the compiler to reserve its space in RAM depending on the data type.
To declare a variable write the data type with variable name.
Syntax
Datatype variablename;
Example
int x;
You can also declare multiple variables of the same type together in one line.
Example
int a, b, c;
on the back end
Initialize a Variable in C++
A variable is initialized when you assign a value to it. You can initialize a variable at the time of declaration or later.
Syntax
Datatype variablename = value
Example
int x = 2; // initialization at the time of declaration
or
int x; x = 2; // initialization after declaration
Similarly, multiple variables can also be initialized in a single line.
Example
int a = 1, b = 2, c = 3;
Update a Variable in C++
Updating a variable means changing the value stored in it.
Syntax
Variablename = newvalue;
Example
int x = 2; x = 3 // updating value
Rules for naming a variable in C++
When naming a variable in programming, follow these simple rules:
- Start with a letter or underscore: For example,
score
or_count
. - No spaces allowed: Use underscores instead, like
high_score
. - Case sensitive:
totalCost
andtotalcost
are different variables. - No special characters: Only letters, numbers, and underscores, such as
total3
ordata_value
. - Avoid reserved words: Don’t use language keywords like
int
,float
. - Make it descriptive: Use names that describe the data’s purpose, like
userName
.
Short, clear variable names help others understand what data is being stored just by looking at the name.
Valid Variable Names
int age;
// Can use letters.int _score;
// Can start with an underscore.int total5;
// Can mix letters and numbers (but not start with numbers).
Invalid Variable Names
int 2cool;
// Cannot start with a number.int user name;
// Cannot contain whitespace.int float;
// Cannot use C++ reserved keywords.
C++ Code Demonstrating Variable Operations
The below code implements all the operations discussed. The variable ‘number’ is declared, initialized with a value of 5, accessed and displayed using the cout statement, and also gets updated.
#include <iostream> int main() { // Declaration of a variable int number; // Initialization of the variable number = 5; // Accessing the value of the variable std::cout << "Initial value of number: " << number << std::endl; // Changing the value of the variable number = 10; // Accessing the new value of the variable std::cout << "New value of number: " << number << std::endl; return 0; }
Remember! The best way you can learn programming is through visualization of code. Go to Python tutor, copy and paste the code there, and start visualizing the code.
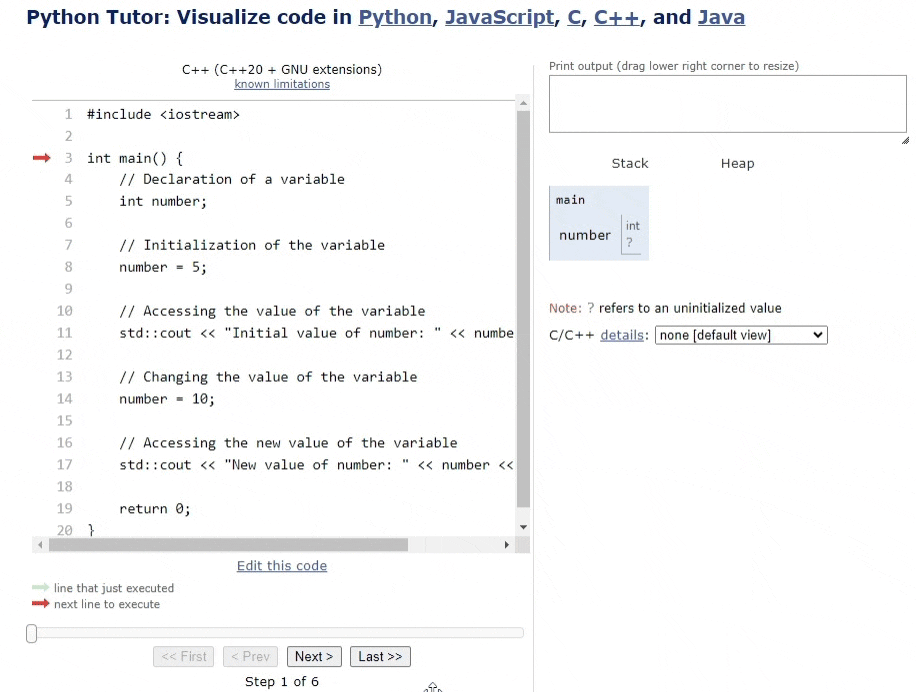