Imagine you’re working on a project, and you need a Data Frame to store data dynamically. However, at the start, there’s no data available. So, how do you create an empty DataFrame that can later be populated with data? In this article, we’ll explore several ways to create a Pandas DataFrame without any initial values and show how you can append rows and columns as your data grows over time.
What is an Empty DataFrame and Why Do You Need It?
An empty DataFrame is like an empty notebook—you create it first, then add content as needed. It is useful when:
- You don’t have data at the start but need a structured DataFrame.
- You want to append data dynamically later.
- You need a DataFrame with specific column names but no initial rows.
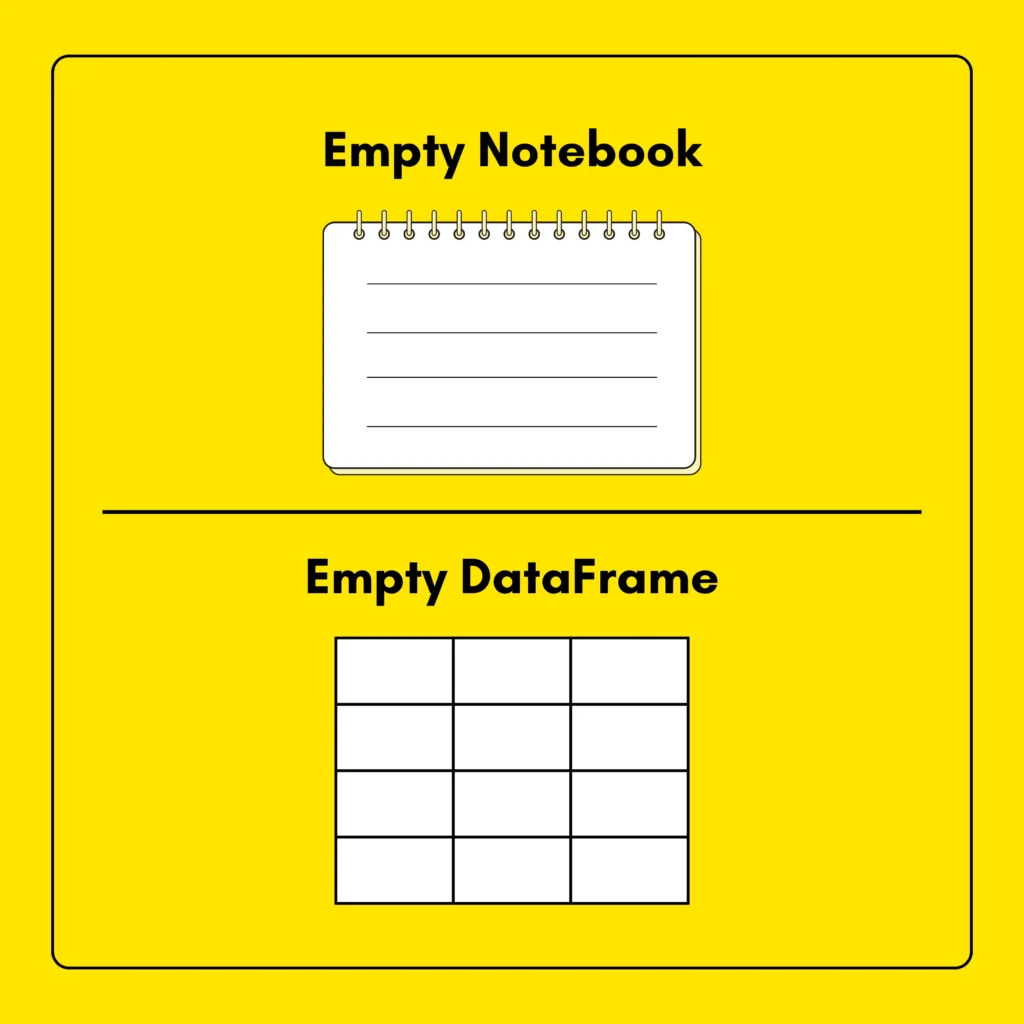
Multiple Ways to Initialize an Empty DataFrame in Pandas
1. Using pd.DataFrame()
The most basic way to create an empty pandas DataFrame is by using the pd.DataFrame()
function without passing any arguments or parameters.
Example Code
In this example, we first import pandas
library. Then we call pd.frame()
without any arguments. Finally, our empty DataFrame is printed to the console with no rows and columns.
import pandas as pd # Create an empty DataFrame empty_df = pd.DataFrame() # Show the DataFrame print(empty_df)
Output
Empty DataFrame Columns: [] Index: []
2. Creating an Empty DataFrame with Column Names
Sometimes, you already know the column names you want to work with but don’t have any data yet. In this case, you can initialize an empty DataFrame with predefined columns.
Example Code
Here, we pass a list of column names ("Name"
, "Age"
, "City"
) to the columns
parameter. The result is an empty DataFrame with three columns but no rows. The DataFrame is then displayed as the output.
import pandas as pd # Create an empty DataFrame with predefined columns empty_df = pd.DataFrame(columns=["Name", "Age", "City"]) # Show the DataFrame print(empty_df)
Output
Empty DataFrame Columns: [Name, Age, City] Index: []
3. Initializing an Empty DataFrame with Index Labels
If you need an empty DataFrame but with a predefined index, this method is helpful.
Example Code
In this example code, we pass an index
parameter with a list of row labels ("Row1"
, "Row2"
, "Row3"
) to pd.DataFrame
. After that, we print our DataFrame, which contains only the row labels.
import pandas as pd # Create an empty DataFrame with predefined index empty_df = pd.DataFrame(index=["Row1", "Row2", "Row3"]) # Show the DataFrame print(empty_df)
Output
Empty DataFrame Columns: [] Index: [Row1, Row2, Row3]
4. Creating an Empty DataFrame with Both Columns and Index
You can also combine both the columns
and index
parameters to create an empty DataFrame that’s pre-structured with both column names and row indices.
Example Code
Here, we use the columns
parameter to define the column names as a list ("Name"
, "Age"
, "City"
) and the index
parameter to set the row labels as a list ("Row1"
, "Row2"
, "Row3"
). Finally, we print the resulting DataFrame.
import pandas as pd # Create an empty DataFrame with columns and index empty_df = pd.DataFrame(columns=["Name", "Age", "City"], index=["Row1", "Row2", "Row3"]) # Show the DataFrame print(empty_df)
Output
Name Age City Row1 NaN NaN NaN Row2 NaN NaN NaN Row3 NaN NaN NaN
In this output, NaN
indicates missing values. The Pandas library can also be used to check if a value is NaN
in a list.
How to Append Data to an Empty DataFrame
The .loc[]
method allows you to add rows to the empty DataFrame with a specific index. It’s a more efficient way to add data, especially when working with large datasets because it avoids the overhead of reindexing the entire DataFrame as .append()
does.
Note: append()
method is deprecated in Pandas 1.3+.
Example Code
In this example, we first create an empty DataFrame using the columns
parameter. Then, we use .loc[0]
to add a row at index 0
by assigning the values ["Peter", 30, "Chicago"]
. The result is an updated DataFrame with a single row at index 0
, which is then printed on the screen.
import pandas as pd # Create an empty DataFrame empty_df = pd.DataFrame(columns=["Name", "Age", "City"]) # Add a row using loc empty_df.loc[0] = ["Peter", 30, "Chicago"] # Show the updated DataFrame print(empty_df)
Output
Name Age City 0 Peter 30 Chicago
Wrap Up
In this tutorial, we covered different ways to create an empty DataFrame in Pandas. Whether you need a simple blank DataFrame, one with predefined columns, or a structured placeholder for future data, you now have multiple methods to choose from.
Each approach serves a specific purpose, so pick the one that best fits your needs. If you’re working on a project where data will be added dynamically, initializing an empty DataFrame correctly can save you time and effort later.
For more beginner-friendly Python tutorials, don’t forget to explore our Python Series at Syntax Scenarios!