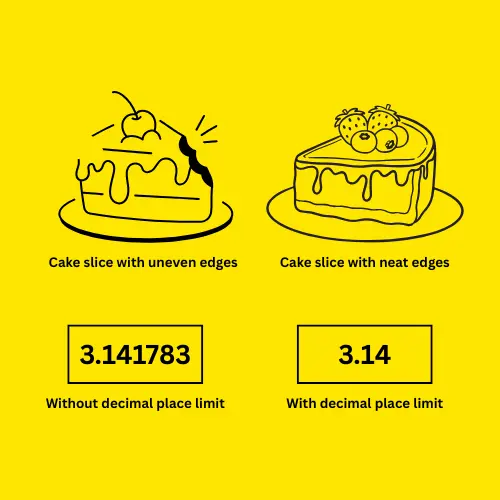
Limiting decimal places in Python is like trimming a cake to make it look neat and presentable. Just as you wouldn’t serve a cake with uneven edges, you don’t want to display numbers with too many decimal places. Python provides multiple ways to “trim” extra decimals in numbers
Why Limit Decimal Places?
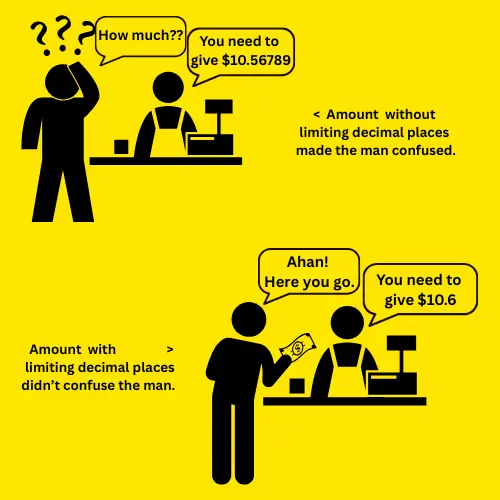
Imagine you’re at a bakery, and the cashier tells you your total is 10.56789. Wouldn’t you prefer to hear 10.57 instead? Limiting decimal places makes numbers easier to read, understand, and use. In Python, this is especially useful for:
- Financial calculations (e.g., currency).
- Scientific data (e.g., measurements).
- Displaying clean and concise results.
Methods to Limit Decimal Places in Python
Let’s explore all the possible methods to limit decimal places in Python in a fun and easy way, along with their general syntax, examples, and outputs.
Method 1: Using the round() Function
The round() function is like a cookie-cutter. It neatly shapes your number to the desired decimal places and rounds the number to the nearest value with the specified decimal places. It’s simple and quick for basic rounding tasks.
Syntax:
rounded = round(number, decimal_places)
round()
: Round a number.number
: The value to round.decimal_places
: Digits to keep after the decimal.
Example:
number = 3.14159 rounded = round(number, 2) # Round to 2 decimal places print(rounded)
Output:
3.14
Method 2: Using String Formatting
String formatting is like a fancy label on a product. It doesn’t change the product itself but makes it look better. It displays the number with fixed decimal places without changing its actual value. It’s ideal for displaying clean and readable results.
Syntax with f-strings (Python 3.6+):
formatted = f"{number:.decimal_placesf}"
f""
: An f-string for formatting.number
: The value to format.:.decimal_placesf
: Specifies decimal places (f for float).
Example:
number = 3.14159 formatted = f"{number:.2f}" # Format to 2 decimal places print(formatted)
Output:
3.14
Method 3: Using the decimal Module
The decimal module is like a precision scale. It ensures your calculations are accurate and consistent, especially for financial data. It avoids the inaccuracies of floating-point arithmetic.
Syntax:
getcontext().prec = decimal_places
getcontext().prec = decimal_places
: Sets the precision for decimal operations.
Example:
from decimal import Decimal, getcontext getcontext().prec = 3 result = Decimal('1') / Decimal('7') print(result)
Output:
0.143
Method 4: Using the math Module
The math module is like a toolbox. It has tools to manipulate numbers in specific ways. The math.floor() function rounds down to the nearest integer. It’s useful for truncating numbers without rounding.
Syntax with math.floor():
floored = math.floor(number * 10**decimal_places) / 10**decimal_places
math.floor(value)
: Rounds down the value to the nearest integer.number * 10**decimal_places
: Shifts the decimal point right to preserve precision.math.floor(...)
: Removes extra decimal places by truncating./ 10**decimal_places
: Shifts the decimal point back to its original position.
Example:
import math number = 3.14159 floored = math.floor(number * 100) / 100 # Limit to 2 decimal places print(floored)
Output:
3.14
Method 5: Using numpy for Arrays
If you’re working with a list of numbers, numpy is like a factory machine. It processes everything at once and rounds all numbers in an array efficiently. It’s ideal for scientific or data analysis tasks.
Syntax:
rounded_numbers = np.round(array, decimal_places)
np.round(array, decimal_places)
: Rounds each element in array to the specified number of decimal places.array
: A NumPy array containing numbers to be rounded.decimal_places
: The number of decimal places to round each value to.
Example:
import numpy as np numbers = np.array([3.14159, 2.71828, 1.41421]) rounded_numbers = np.round(numbers, 2) # Round all numbers to 2 decimal places print(rounded_numbers)
Output:
[3.14 2.72 1.41]
Method 6: Using Custom Functions
Sometimes, you need a custom solution like baking a cake with a unique recipe. Custom functions allow you to define your logic for limiting decimal places. They’re flexible and can be tailored to specific needs.
Syntax:
def limit_decimals(number, decimals): factor = 10 ** decimals return int(number * factor) / factor
- def limit_decimals(number, decimals): Defines a function to limit decimal places.
- factor = 10 ** decimals: Creates a scaling factor (e.g., 10^2 = 100 for 2 decimals).
- int(number * factor) / factor: Multiplies number by factor, converts to int (truncating extra decimals), then divides back to restore the decimal places.
Example:
def limit_decimals(number, decimals): factor = 10 ** decimals return int(number * factor) / factor number = 3.14159 limited = limit_decimals(number, 2) # Limit to 2 decimal places print(limited)
Output:
3.14
Which Method to Use When?
Method | Best Use Case | 1-Line Syntax | Key Feature |
round() | Simple rounding of numbers. | rounded = round(number, decimal_places) | Rounds the number to the nearest value with specified decimal places. |
String Formatting | Displaying numbers with fixed decimals. | formatted = f"{number:.decimal_placesf}" | Formats the number as a string without changing its actual value. |
decimal Module | High-precision calculations (e.g., finance). | result = Decimal('number') / Decimal('divisor') | Ensures accurate decimal arithmetic with customizable precision. |
math Module | Truncating or flooring numbers. | floored = math.floor(number * 10**decimal_places) / 10**decimal_places | Rounds down or truncates without rounding. |
numpy | Rounding arrays of numbers efficiently. | rounded_numbers = np.round(array, decimal_places) | Works on entire arrays at once, ideal for scientific or data analysis tasks. |
Custom Function | Custom logic for limiting decimals. | limited = int(number * 10**decimals) / 10**decimals | Allows you to define your logic for limiting decimal places. |
FAQs
1. What’s the difference between round() and string formatting?
- round() changes the actual value of the number.
- String formatting only changes how the number is displayed.
2. Which method is best for financial calculations?
Use the decimal module for high precision and accuracy.
3. Can I limit decimal places without rounding?
Yes, use math.trunc() or a custom function to truncate the number.
4. How do I limit decimal places in an array?
Use numpy.round() for efficient array-wide operations.
5. What’s the easiest way to format numbers for display?
Use f-strings (e.g., f”{number:.2f}”) for clean and modern code.
Conclusion
Limiting decimal places in Python is a crucial skill for making your data clean, readable, and precise. Whether you’re using round(), string formatting, the decimal module, or numpy, each method has its use case. By following this guide, you’ll be able to limit decimal places in Python like a pro!
Remember, the key to mastering this skill is practice. Try these methods in your next project and see which one works best for you.
For more Python tutorials, check out the Python Series on Syntax Scenarios and level up your coding skills!