Struggling to convert a list into a tuple in Python? Whether you’re optimizing performance or working with immutable data, learn quick and easy ways to do this in just a few lines of code!
What is a List in Python? A Real-world Analogy
Imagine you’re writing on a whiteboard. A list is like that whiteboard where you can erase and rewrite anything while the board itself remains unchanged. Similarly, a mutable object’s memory address stays the same, but its contents can be updated.
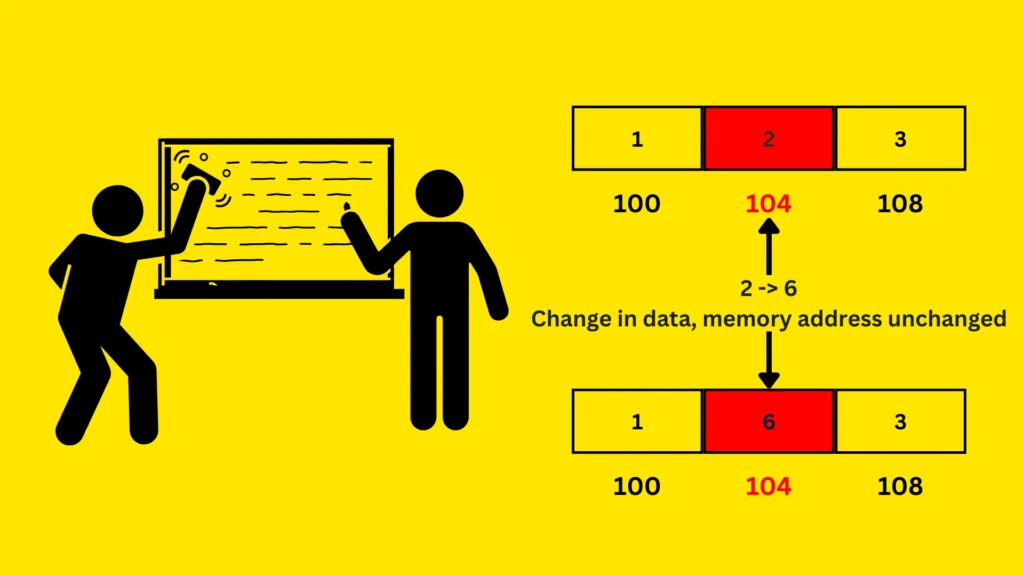
Similarly, a Python list is an ordered, mutable collection of values. You can change the list after creating it by adding, removing, or modifying items. Lists are mutable, meaning their memory address stays the same even when the content changes.
Example of a list:
my_list = [1, 2, 3, "apple", "banana"]
What is a Tuple in Python? A Real-world Analogy
Now, imagine you have a printed photo. Once it’s printed, you can’t change the photo itself (you can’t remove or add something to the picture without printing a new one).
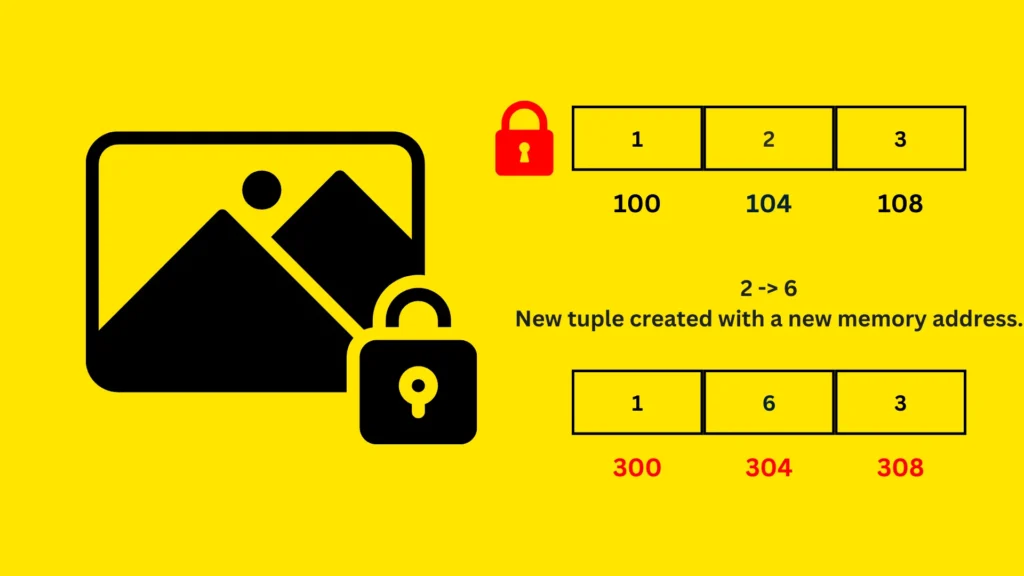
Similarly, in Python, a tuple is a collection of values that are ordered but immutable (unchangeable). Once you define it, the values inside it stay the same forever. When you create a tuple, the system locks its contents. This makes tuples more memory-efficient for data that doesn’t need to change. Their unchangeable nature helps the system optimize for speed and storage.
my_tuple = (1, 2, 3, "apple", "banana")
How to Convert a List into a Tuple?
Here is a list of ways to convert a list into a tuple in Python.
tuple()
constructor: Simple and efficient for most cases.- List comprehension: Useful when you need to transform or filter elements.
- Unpacking operator: Concise and clean for direct conversion.
- map() function: Ideal for applying transformations before conversion.
- Generator expression: Memory-efficient for large datasets.
- *Using args: Best when dealing with function arguments.
- For loop: Gives you full control but is less efficient.
1. Using the tuple() Constructor
Using the tuple()
constructor. This method directly converts any iterable (like a list) into a tuple, making it the most commonly used approach.
Syntax
tuple(iterable)
iterable
: The list (or any other iterable) that you want to convert into a tuple.
Example Code
In this code, the tuple()
constructor is used to convert a list into a tuple. The list [1, 2, 3]
is passed to the constructor, and the result is a tuple (1, 2, 3)
. This method is the most efficient and straightforward when you just need to convert a list to a tuple without any transformations.
my_list = [1, 2, 3] my_tuple = tuple(my_list) print(my_tuple)
Output
(1, 2, 3)
2. Using a List Comprehension with tuple()
Using list comprehension along with the tuple()
constructor is a good way to convert a list while applying transformations to the list elements. This method is useful when you need to perform operations on the elements before converting them.
For example, if your list contains None values, you can filter None from the list before converting into a tuple
Syntax
tuple([x for x in iterable])
[x for x in iterable]
: A list comprehension that can apply transformations or filtering.tuple()
: Converts the resulting list into a tuple.
Example Code
Here, the list comprehension [x for x in my_list]
simply loops over the elements of my_list
and passes them to the tuple()
constructor, resulting in a tuple (1, 2, 3)
. This method is useful when you need to perform operations like filtering or modifying the list elements before conversion.
my_list = [1, 2, 3] my_tuple = tuple([x for x in my_list]) print(my_tuple)
Output
(1, 2, 3)
3. Using the * (Unpacking) Operator
The unpacking operator *
can be used to unpack the elements of a list directly into a tuple. This is a clean and concise method for converting a list to a tuple without using the tuple()
constructor.
Syntax
(*iterable,)
*iterable
: Unpacks the list elements.- The trailing comma creates a tuple from the unpacked elements.
Example Code
In this example, the unpacking operator *
is used to unpack the elements of my_list
into a tuple. This is a shorthand way of converting the list into a tuple. This method is a more concise alternative to using tuple()
, particularly when you want to unpack values into a tuple without explicitly calling tuple()
.
my_list = [1, 2, 3] my_tuple = (*my_list,) print(my_tuple)
Output
(1, 2, 3)
4. Using the map() Function (For Specific Transformations)
The map()
function applies a specific transformation to each item in an iterable before converting it to a tuple. This method is helpful when you need to apply a function to the list elements, such as converting all numbers to strings.
Syntax
tuple(map(function, iterable))
function
: A function that transforms each element of the iterable.iterable
: The list to be converted.map()
: Applies the transformation function to each element in the iterable.
Example Code
Here, map(str, my_list)
is used to convert all elements in my_list
to strings before converting the result to a tuple. This method is great for cases where you need to perform a specific transformation, such as converting numbers to strings.
my_list = [1, 2, 3] my_tuple = tuple(map(str, my_list)) print(my_tuple)
Output
('1', '2', '3')
5. Using a Generator Expression
A generator expression is a memory-efficient way to create a tuple from a list. Unlike list comprehension, a generator expression does not create an intermediate list, making it more efficient for large datasets.
Syntax
tuple(expression for item in iterable)
expression for item in iterable
: A generator expression that yields each element of the iterable.tuple()
: Converts the generator output into a tuple.
Example Code
In this example, the generator expression (x for x in my_list)
is used to iterate through my_list
, and the results are converted into a tuple. This is a memory-efficient way of converting the list to a tuple. This method is ideal when working with large datasets, as it avoids the overhead of creating an intermediate list.
my_list = [1, 2, 3] my_tuple = tuple(x for x in my_list) print(my_tuple)
Output
(1, 2, 3)
6. Using *args in Function Definition (For Multiple Elements)
When dealing with functions that accept variable numbers of arguments, the *args
syntax can be used to pass a list and convert it into a tuple.
Syntax
def function_name(*args): return args
*args
: Accepts multiple arguments and collects them into a tuple.- The function can then return the tuple.
Example Code
In this case, the convert_to_tuple()
function accepts multiple arguments and returns them as a tuple. The *my_list
syntax unpacks the elements of my_list
and passes them as individual arguments to the function. This method is particularly useful when working with functions that need to accept a variable number of arguments.
Make sure not use list as a function, otherwise you will encounter TypeError: list object is not callable .
def convert_to_tuple(*args): return args my_list = [1, 2, 3] my_tuple = convert_to_tuple(*my_list) print(my_tuple)
Output
(1, 2, 3)
7. Using a For Loop (Manual Method)
If you want full control over the conversion process, you can use a for loop to manually iterate over the list and add each element to a tuple.
Syntax
tuple = () for item in iterable: tuple += (item,)
tuple = ()
: Initializes an empty tuple.- The loop iterates over the list, adding each element to the tuple.
Example Code
Here, a for loop is used to manually add each element from my_list
into my_tuple
. This method allows you to handle more complex logic if needed. Although this method gives you full control, it’s less efficient than the built-in methods like tuple()
or unpacking.
my_list = [1, 2, 3] my_tuple = () for item in my_list: my_tuple += (item,) print(my_tuple)
Output
(1, 2, 3)
Use Cases for Converting a List to a Tuple
Now, let’s go over more real-world scenarios where converting a list to a tuple might be useful:
- Protecting Data from Changes (Immutability): You are managing a list of server IP addresses in a network configuration. You want to ensure that no one can accidentally change or delete an IP address.
- Faster Access with Fixed Data: You have a list of countries that doesn’t change, and you want to improve performance when accessing this data. You convert the list to a tuple for faster, more efficient access.
- Storing Data as Dictionary Keys: You need to use a collection of items (like coordinates or a date) as keys in a dictionary. But dictionaries require the keys to be immutable.
- Reducing Memory Usage for Fixed Data: You’re dealing with a large set of data that doesn’t change. You want to reduce the memory usage, so you convert your list to a tuple.
Conclusion
Converting a list to a tuple in Python is a simple yet powerful technique that can help improve performance and ensure data immutability. Whether you’re optimizing code or working with fixed data, knowing these conversion methods will enhance your Python skills and make your code more efficient. You’ve explored several methods to convert a list to a tuple in Python, each with its unique advantages. Choose the method that best fits your use case based on simplicity, efficiency, and transformation requirements.
For more interesting and engaging articles on Python, you can visit our Python tutorial series by Syntax Scenarios.