Loops make programming easier by handling repetitive tasks without extra effort, and in Mojo, nested loops take it a step further by solving complex problems that involve multiple conditions at once. This article will explore fun and practical scenarios, starting with simple cases and gradually tackling more advanced challenges. By the end, you’ll see how powerful loops can be in real-world coding and how they can sharpen your Mojo programming skills!
Scheduling the Office Workday
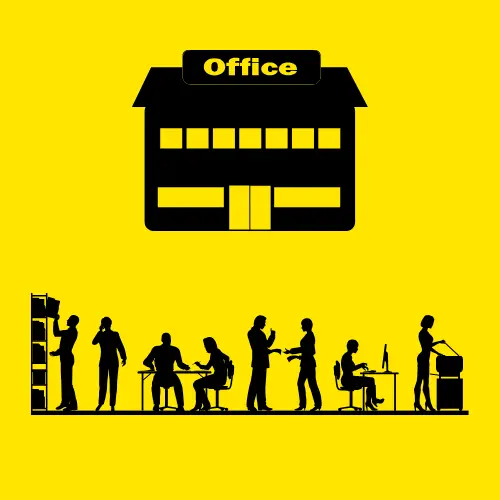
You are tasked with printing the work schedule for a small office, which operates over 5 working days (Monday to Friday). For each day, you need to print the tasks that need to be done: Checking Emails, Meeting with Team, and Documenting Work.
You need to create a Mojo program that asks for the total number of days and tasks and prints the schedule.
Test Case:
Input:
Enter the number of days: 5
Enter the number of tasks per day: 3
Expected Output:
Day 1: Checking Emails, Meeting with Team, Documenting Work
Day 2: Checking Emails, Meeting with Team, Documenting Work
Day 3: Checking Emails, Meeting with Team, Documenting Work
Day 4: Checking Emails, Meeting with Team, Documenting Work
Day 5: Checking Emails, Meeting with Team, Documenting Work
Explanation:
This code generates a schedule for each day, listing tasks based on the user’s input for days and tasks per day.
- First, the user is prompted to input the number of days and the number of tasks per day.
- The outer loop (
for day in range(1, days + 1)
) iterates over each day, printing the day number. - The inner loop (
for task in range(1, tasks + 1)
) iterates over the tasks. It checks the task number using conditions: if the task is 1, it prints"Checking Emails"
, if task 2, it prints"Meeting with Team"
, and for other tasks, it prints"Documenting Work"
. Aprint()
at the end ensures that the next day’s tasks appear on a new line.
days = int(input("Enter the number of days: ")) tasks = int(input("Enter the number of tasks per day: ")) for day in range(1, days + 1): print(f"Day {day}: ", end="") for task in range(1, tasks + 1): if task == 1: print("Checking Emails", end=", ") elif task == 2: print("Meeting with Team", end=", ") else: print("Documenting Work", end=", ") print()
Overtime Championship: Reward the Top Performers!
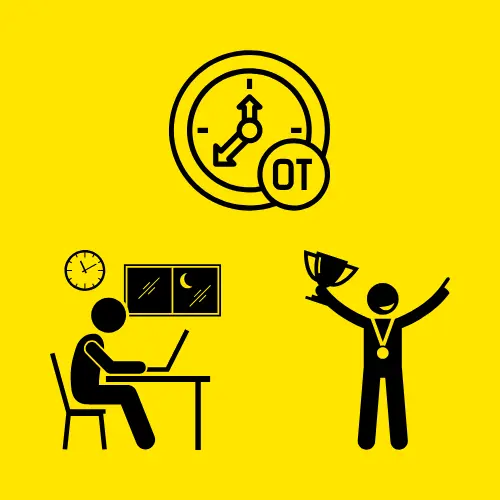
Your company has decided to host an Overtime Championship to motivate employees who go the extra mile! Over the past 3 working days, employees have been putting in extra effort, and now you need to calculate their total overtime hours and reward them based on their performance.
The rules are simple:
Each employee works a standard 8 hours per day, and any hours worked beyond that are counted as overtime.
Each overtime hour is paid $10.
The employee with the highest total overtime will be crowned the “Overtime Champion” and receive an additional $50 bonus.
Your task is to calculate the total overtime hours and payment for each employee and determine the champion.
Test Case:
Input:
Enter the number of employees: 3
Employee 1 Day 1 hours: 9
Employee 1 Day 2 hours: 10
Employee 1 Day 3 hours: 8
Employee 2 Day 1 hours: 8
Employee 2 Day 2 hours: 9
Employee 2 Day 3 hours: 9
Employee 3 Day 1 hours: 7
Employee 3 Day 2 hours: 8
Employee 3 Day 3 hours: 11
Expected Output:
Employee 1 Overtime Hours: 3
Employee 1 Overtime Pay: $30
Employee 2 Overtime Hours: 2
Employee 2 Overtime Pay: $20
Employee 3 Overtime Hours: 3
Employee 3 Overtime Pay: $30
The Overtime Champion is Employee 1 with a bonus of $50!
Explanation:
This code calculates the overtime hours and pay for multiple employees and determines the “Overtime Champion” based on who has the most overtime hours.
- The program first takes the number of employees (
employees
) as input. Two variables,max_overtime
andchampion
, are initialized to keep track of the highest overtime hours and the corresponding employee. - The outer loop runs for each employee. Inside this loop, the
total_overtime
for the employee is initialized to 0, and aday
counter is set to 1 to process each day’s overtime. The innerwhile
loop runs for each of the three days, prompting the user to enter the overtime hours worked by the employee for that day. - If the hours worked are greater than 8, the overtime hours (hours worked minus 8) are added to
total_overtime
. After processing all three days, the total overtime hours are used to calculate the overtime pay (overtime_pay = total_overtime * 10
), and the result is displayed for the employee. - The program then checks if the current employee has worked more overtime than the previous maximum (
if total_overtime > max_overtime:
). If so,max_overtime
is updated, and the current employee is marked as the newchampion
.
employees = int(input("Enter the number of employees: ")) max_overtime = 0 champion = 0 for employee in range(1, employees + 1): total_overtime = 0 day = 1 while day <= 3: hours = int(input("Employee " + str(employee) + " Day " + str(day) + " hours: ")) if hours > 8: total_overtime += hours - 8 day += 1 overtime_pay = total_overtime * 10 print("Employee", employee) print("Overtime Hours:", total_overtime) print("Overtime Pay: $", overtime_pay) if total_overtime > max_overtime: max_overtime = total_overtime champion = employee print("The Overtime Champion is Employee", champion, "with a bonus of $50!")
Classroom Grading Challenge: Average and Passing Status
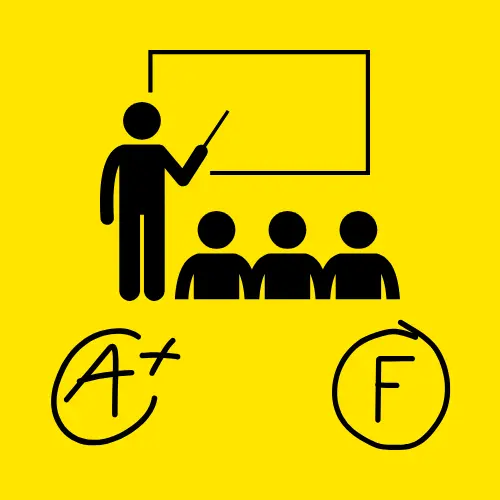
You are the class instructor of a school conducting multiple tests for students. Each student takes 3 tests to evaluate their performance. You need to calculate the average score for each student and determine whether they have passed or failed based on the average score.
Here are the rules:
Each student’s average score is calculated from their 3 test scores.
A student passes if their average score is 50 or above.
After processing all students, display their average scores and whether they passed or failed.
In the end, highlight how many students passed.
Your job is to calculate this using a for loop for the number of students and a while loop to handle the test scores.
Test Case:
Input:
Enter the number of students: 2
Enter Test 1 score for Student 1: 40
Enter Test 2 score for Student 1: 60
Enter Test 3 score for Student 1: 50
Enter Test 1 score for Student 2: 30
Enter Test 2 score for Student 2: 20
Enter Test 3 score for Student 2: 40
Expected Output:
Student 1 Average: 50.0
Student 1 Result: Pass
Student 2 Average: 30.0
Student 2 Result: Fail
Total Students Passed: 1
Explanation:
This code takes input for the number of students and their scores in three tests calculates the average score for each student, and determines if they passed or failed based on that average.
- It first asks the user to input the total number of students (
students
) and initializes a counter (passed_students
) to track how many students pass. - The outer loop (
for student in range(1, students + 1)
) iterates over each student. For each student, the code sets thetotal_score
to 0 and initializes the test counter (test = 1
). - Inside the loop, a
while
loop runs three times, prompting the user to input each test score (score
) and adding it tototal_score
. After all scores are entered, the average score is calculated by dividingtotal_score
by 3. - The program then checks if the average is greater than or equal to 50. If true, it prints a pass message and increments the
passed_students
counter otherwise, it prints a fail message. Finally, it outputs the total number of passed students.
students = int(input("Enter the number of students: ")) passed_students = 0 for student in range(1, students + 1): total_score = 0 test = 1 while test <= 3: score = float(input("Enter Test " + str(test) + " score for Student " + str(student) + ": ")) total_score += score test += 1 average = total_score / 3 print("Student", student, "Average:", average) if average >= 50: print("Student", student, "Result: Pass") passed_students += 1 else: print("Student", student, "Result: Fail") print("Total Students Passed:", passed_students)
Warehouse Inventory Tracker
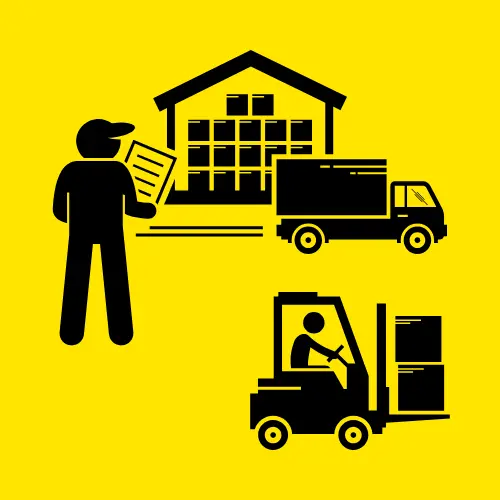
You manage a warehouse with 2 products: Product A and Product B. Each product is stored in 3 separate sections of the warehouse. Input the quantity of each product stored in each section, calculate the total quantity for each product, and display the results.
Test Case:
Input:
Enter quantity of Product A in Section 1: 50
Enter quantity of Product A in Section 2: 30
Enter quantity of Product A in Section 3: 20
Enter quantity of Product B in Section 1: 60
Enter quantity of Product B in Section 2: 40
Enter quantity of Product B in Section 3: 50
Expected Output:
Total Quantity of Product A: 100
Total Quantity of Product B: 150
Explanation:
This code calculates the total quantity of two products (A and B) in three sections and displays the result for each product.
- The
product
variable is initialized to 1, representing product A, and awhile
loop runs twice, once for each product (A and B). For each product, thetotal_quantity
is set to 0, and thesection
counter is initialized to 1 to represent the sections where the product is located. - Inside the loop, another
while
loop runs three times to handle input for each section. Theproduct_name
is assigned based on whether it’s product A or B, and thequantity
for the current section is entered by the user. The entered quantity is added tototal_quantity
, and thesection
counter is incremented. - After all three sections are processed for a product, the total quantity is displayed. The
product
counter is then incremented to process the next product.
product = 1 while product <= 2: total_quantity = 0 section = 1 while section <= 3: if product == 1: product_name = "A" else: product_name = "B" quantity = int(input("Enter quantity of Product " + product_name + " in Section " + str(section) + ": ")) total_quantity += quantity section += 1 print("Total Quantity of Product", product_name, ":", total_quantity) product += 1
Simple Number Pattern
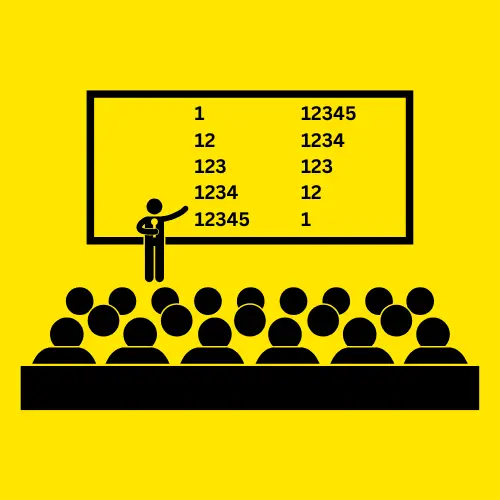
You are organizing a number pattern display for a school event. The pattern will be in the shape of a triangle. The first row will contain 1, the second row will contain 1 2, the third row will contain 1 2 3, and so on. However, the user will specify how many rows they want to display in the pattern.
Your task is to write a program that prints this number pattern based on the number of rows entered by the user.
Test Case:
Input:
Enter the number of rows for the pattern: 4
Expected Output:
1
1 2
1 2 3
1 2 3 4
Explanation:
This code generates a pattern of numbers based on the number of rows specified by the user.
- The program first takes the number of rows (
rows
) as input. - Then, the outer loop starts from row 1 and runs until the specified number of rows is reached. Inside the outer loop, the
num
variable is initialized to 1 at the beginning of each row. - The inner loop runs for each number in the current row, printing the numbers from 1 up to the current row number (
num += 1
). Theend=""
in theprint
function ensures that the numbers are printed on the same line, separated by a space. - After printing the numbers for the current row,
print("")
move the cursor to the next line. Therow
counter is then incremented to process the next row. This continues until the specified number of rows is printed.
rows = int(input("Enter the number of rows for the pattern: ")) row = 1 while row <= rows: num = 1 while num <= row: print(num, " ", end="") num += 1 print("") row += 1
Treasure Hunt Challenge
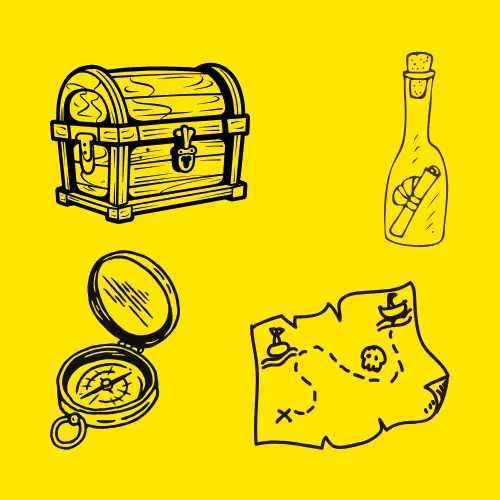
Imagine you are participating in a treasure hunt where the clues to the treasure are hidden in prime numbers. The treasure can only be found by collecting prime numbers within a specific range. You’ve been given a starting numberand an ending number. The challenge is to identify all the prime numbers in this range and find their sum. The more prime numbers you find, the closer you get to your treasure!
Your task is to calculate the sum of all prime numbers between the two numbers you are given. A prime number is a number that can only be divided by 1 and itself.
Test Case:
Input:
Enter the starting number: 10
Enter the ending number: 30
Expected Output:
The prime numbers between 10 and 30 are: 11 13 17 19 23 29
The sum of these prime numbers is: 122
Explanation:
This code finds all the prime numbers between a given start and end number, calculates their sum, and displays both the prime numbers and their total sum.
- First, the program takes the starting (
start
) and ending (end
) numbers as input from the user. It then initializes two variables:sum_primes
to store the sum of prime numbers andprime_numbers
to store the prime numbers as a string. - The
num
variable is initialized to the start value and the outerwhile
loop runs as long asnum
is less than or equal to the end value. - For each number (
num
) in the specified range, the program checks if it is prime. Theis_prime
variable is set toTrue
at the beginning of each iteration. An innerwhile
loop starts withdiv = 2
and checks ifnum
is divisible by any number between 2 andnum / 2
. - If any divisor is found (i.e.,
num % div == 0
), it setsis_prime
toFalse
and breaks the loop, meaning the number is not prime. If no divisors are found andnum
is greater than 1, it is considered prime. The prime number is then added to thesum_primes
, and the prime number is appended to theprime_numbers
string. - After processing all numbers in the range, the program prints the list of prime numbers and the sum of these primes.
start = int(input("Enter the starting number: ")) end = int(input("Enter the ending number: ")) sum_primes = 0 prime_numbers = "" num = start while num <= end: is_prime = True div = 2 while div <= num / 2: if num % div == 0: is_prime = False break div += 1 if is_prime and num > 1: sum_primes += num prime_numbers += str(num) + " " num += 1 print("The prime numbers between", start, "and", end, "are:", prime_numbers) print("The sum of these prime numbers is:", sum_primes)
Reverse Pyramid of Stars: Mountain Climbing Challenge
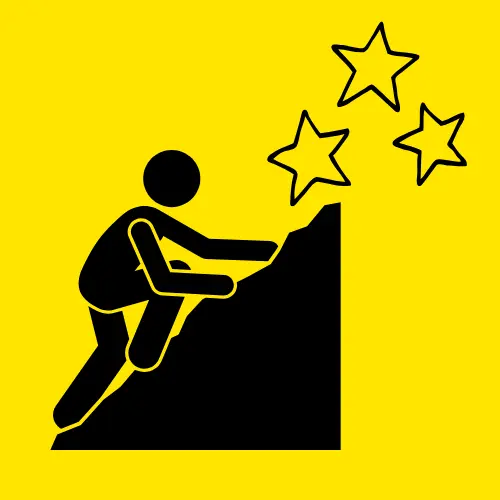
Imagine you are preparing for a mountain climbing challenge. The climb is difficult, but there’s a special symbol that marks each level of the mountain: a star. The higher you go, the fewer stars you’ll see because the air gets thinner. You need to print a reverse pyramid of stars that marks the levels of your climb. The number of stars will decrease as you move up, just like the difficulty of the climb increases!
Your task is to print a reverse pyramid of stars based on the number of levels you want to climb, and for each level, the number of stars will decrease from the base to the top. You will enter how many levels you want to climb, and the pyramid will form accordingly.
Test Case:
Input:
Enter the number of levels in your climb: 5
Expected Output:
* * * * *
* * * *
* * *
* *
*
Explanation:
This code generates an inverted triangle pattern of stars based on the number of levels specified by the user.
- The program first takes the number of levels (
levels
) as input. Thelevel
variable is initialized to the total number of levels and is used in the outerwhile
loop, which runs untillevel
reaches 1. - In each iteration of the outer loop, the program first prints spaces to align the stars in the correct position. The inner
while
loop prints the spaces, where the number of spaces decreases as the level number decreases. - After printing the spaces, the program prints the stars. The
star
variable is used to print stars (*
) for the current level. The inner loop runs for the number of stars equal to the current level. Each star is printed with a space after it (end=" "
). - After printing the stars for a particular level, the program moves to the next line (
print("")
) and decrements thelevel
counter to handle the next level of the pyramid. This process continues until the pattern is fully printed.
levels = int(input("Enter the number of levels in your climb: ")) level = levels while level >= 1: space = 1 while space <= (levels - level): print(" ", end="") space += 1 star = 1 while star <= level: print("*", end=" ") star += 1 print("") level -= 1
Alien Energy Generator
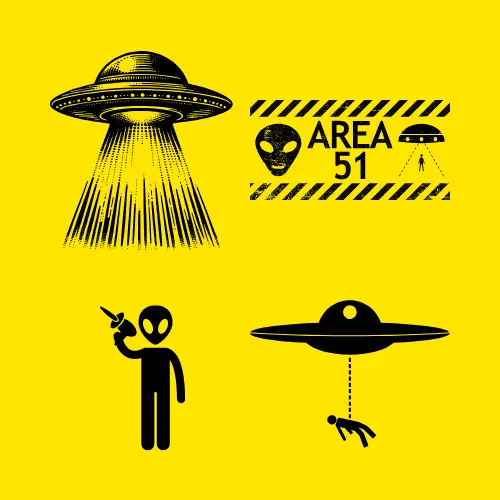
You’ve discovered an alien energy generator! It generates energy levels based on a mysterious formula: the energy level of each cell is the sum of its row and column numbers. You need to calculate the energy level for every cell in a grid.
The program should display the energy levels row by row, and then show the total energy produced by the grid.
Test Case:
Input:
Enter the number of rows: 3
Enter the number of columns: 3
Expected Output:
Energy levels:
2 3 4
3 4 5
4 5 6
Total energy: 36
Explanation:
This code calculates the “energy” of each cell in a grid and finds the total energy for all cells.
- The user provides the number of rows (
rows
) and columns (cols
), and the variabletotal_energy
is initialized to 0 to store the cumulative energy. - The outer loop starts with
row
at 1 and runs until it equals the number of rows, iterating over each row. Inside this loop, the inner loop begins withcol
at 1 and processes each column in the current row. - For each cell, the energy is calculated as the sum of
row
andcol
, printed on the same line, and added tototal_energy
. After completing one row, aprint()
moves to the next line, and the outer loop incrementsrow
to proceed to the next. Once the grid is fully processed, the program prints the total energy of all cells.
rows = int(input("Enter the number of rows: ")) cols = int(input("Enter the number of columns: ")) total_energy = 0 row = 1 while row <= rows: col = 1 while col <= cols: energy = row + col print(energy, " ", end="") total_energy += energy col += 1 print() row += 1 print("Total energy:", total_energy)
Magical Forest Matrix
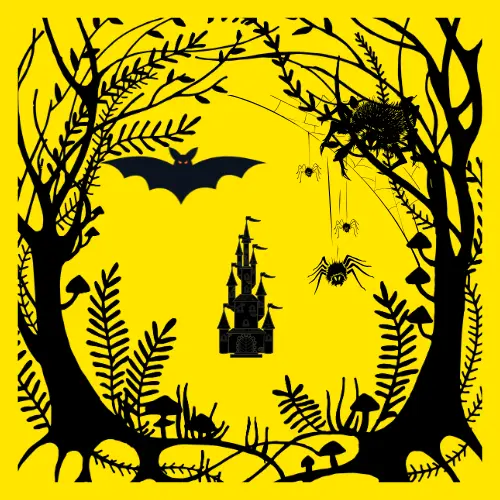
You’ve entered a magical forest represented as a grid. Each cell in the grid contains a magical energy level, which is the product of its row and column numbers. You need to calculate and display the magical energy levels for each cell in the matrix.
After calculating the energy levels, you must compute the forest’s total magical energy and identify the highest energy level in the grid.
Write a Mojo program to calculate and display the matrix of energy levels and perform the required calculations.
Test Case:
Input:
Enter the number of rows: 3
Enter the number of columns: 4
Expected Output:
Magical Forest Matrix:
1 2 3 4
2 4 6 8
3 6 9 12
Total Magical Energy: 60
Highest Energy Level: 12
Explanation:
This code calculates the “magical energy” for each cell in a grid, where the energy of a cell is determined by multiplying its row and column indices. It also computes the total magical energy of the grid and identifies the highest energy level.
- The program starts by asking the user to input the number of rows (
rows
) and columns (cols
). Two variables,total_energy
andhighest_energy
, are initialized to 0 to keep track of the cumulative energy and the maximum energy value, respectively. - The outer
while
loop begins withrow
set to 1 and iterates over each row. Inside this loop, the innerwhile
loop starts withcol
at 1 and processes each column in the current row. For each cell, the energy is calculated asrow * col
, printed on the same line, and added tototal_energy
. - If the current energy exceeds
highest_energy
, it is updated to reflect the new maximum value. After printing all columns for a row, aprint()
moves to the next line, and the outer loop incrementsrow
to process the next row.
rows = int(input("Enter the number of rows: ")) cols = int(input("Enter the number of columns: ")) total_energy = 0 highest_energy = 0 row = 1 while row <= rows: col = 1 while col <= cols: energy = row * col print(energy, " ", end="") total_energy += energy if energy > highest_energy: highest_energy = energy col += 1 print() row += 1 print("Total Magical Energy:", total_energy) print("Highest Energy Level:", highest_energy)
Perfect Number Detectives
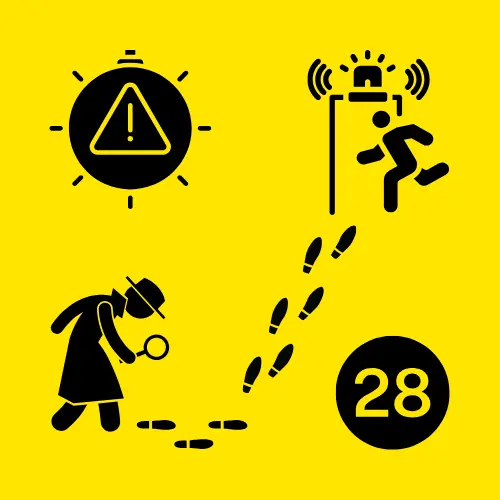
Imagine you’re part of a group of detectives called the “Number Sleuths.” Your team is tasked with solving the case of the “Perfect Numbers.” These special numbers are known to be equal to the sum of their divisors (except for the number itself). In this case, you need to gather evidence and determine whether a certain number is a “Perfect Number.”
Your mission is twofold:
You need to check if a given number is a perfect number.
You need to find and list all the perfect numbers up to a certain maximum, and present your findings to the agency.
The agency has received a tip that perfect numbers exist up to a certain point, but the exact numbers remain a mystery. Your team will need to investigate and solve the case!
Test Case:
Input:
Enter the number to investigate: 28
Investigate all perfect numbers up to: 1000
Expected Output:
Investigating number: 28
Sum of divisors of 28 (excluding itself): 28
28 is a perfect number!
Perfect numbers up to 1000 :
6
28
496
Explanation:
This code investigates whether a given number is a perfect number and then finds all perfect numbers up to a specified limit. A perfect number is a positive integer that is equal to the sum of its proper divisors (excluding itself).
- First, the program takes two inputs from the user:
number
andlimit
. - For the given
number
, it calculates the sum of its divisors using afor
loop, which checks every number from 1 to half ofnumber
. If a divisor divides the number evenly (number % divisor == 0
), it is added tosum_of_divisors
. - After the loop, if the
sum_of_divisors
is equal tonumber
, it means the number is perfect, and the program prints that it is a perfect number. If not, it prints that the number is not perfect. - After investigating the given number, the program then checks all numbers from 1 to the
limit
to identify perfect numbers. For each number, it calculates the sum of its divisors using the same method and checks if the sum equals the number. If it does, the number is printed as a perfect number.
number = int(input("Enter the number to investigate: ")) limit = int(input("Investigate all perfect numbers up to: ")) sum_of_divisors = 0 for divisor in range(1, number // 2 + 1): if number % divisor == 0: sum_of_divisors += divisor if sum_of_divisors == number: print("Investigating number:", number) print("Sum of divisors of", number, "(excluding itself):", sum_of_divisors) print(number, "is a perfect number!") else: print("Investigating number:", number) print(number, "is not a perfect number.") print("Perfect numbers up to", limit, ":") for num in range(1, limit + 1): sum_of_divisors = 0 for divisor in range(1, num // 2 + 1): if num % divisor == 0: sum_of_divisors += divisor if sum_of_divisors == num: print(num)
Garden Watering Schedule
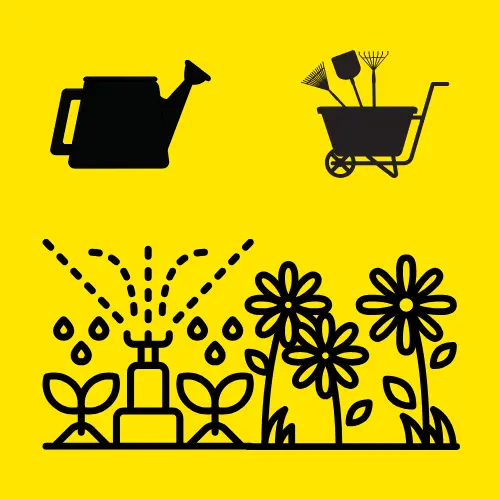
You are managing a community garden, and you have two different water sprinklers in the garden. Each sprinkler has a unique watering schedule: one sprinkler waters every certain number of days, and the other waters every different number of days. To optimize your watering schedule, you need to find out how often both sprinklers will water on the same day. This is done by calculating the Greatest Common Divisor (GCD) of the two watering intervals.
Your task is to compute the GCD of the watering intervals of both sprinklers and determine the optimal day when both sprinklers will water together.
Test Case:
Input:
Enter the number of sprinkler pairs: 2
Enter details for sprinkler pair 1:
Watering interval for sprinkler 1: 24
Watering interval for sprinkler 2: 36
Enter details for sprinkler pair 2:
Watering interval for sprinkler 1: 40
Watering interval for sprinkler 2: 60
Expected Output:
GCD of their watering intervals: 12
GCD of their watering intervals: 20
Explanation:
This code calculates the greatest common divisor (GCD) of the watering intervals of pairs of sprinklers.
- It first takes input for the number of sprinkler pairs (
num_pairs
). - For each pair, the program prompts the user to enter the watering intervals for both sprinklers. The intervals are stored in
sprinkler_1
andsprinkler_2
. The GCD is calculated by repeatedly dividing the larger number by the smaller number and replacing the larger number with the remainder until the remainder is 0. At this point, the smaller number is the GCD.
num_pairs = int(input("Enter the number of sprinkler pairs: ")) for i in range(1, num_pairs + 1): print(f"Enter details for sprinkler pair {i}:") sprinkler_1 = int(input("Watering interval for sprinkler 1: ")) sprinkler_2 = int(input("Watering interval for sprinkler 2: ")) a = sprinkler_1 b = sprinkler_2 while b != 0: temp = b b = a % b a = temp print(f"GCD of their watering intervals: {a}")
Menu-Driven Student Marks Tracker
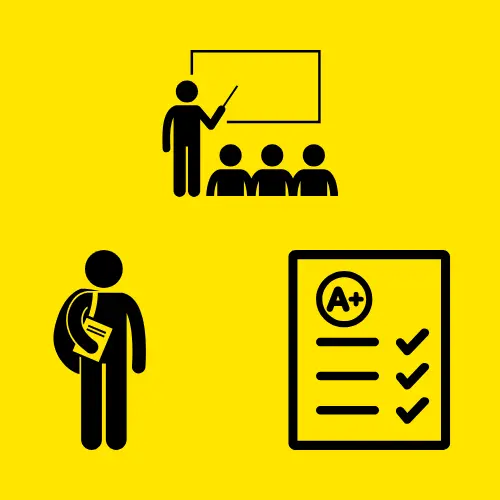
You are building a student marks tracker for a school, where you can:
Input marks for multiple students.
View marks of all students.
Calculate the average marks of each student.
Display the highest and lowest marks in each subject.
The user will input marks for each student and be able to choose from the following options from a menu.
Input:
The number of students
Marks for each student in each subject.
Test Case:
Input:
Enter the number of students: 2
Student 1 :
Enter Math marks: 85
Enter Science marks: 56
Enter English marks: 90
Student 2 :
Enter Math marks: 40
Enter Science marks: 65
Enter English marks: 80
Enter your choice: 4
Enter your choice: 5
Exiting the program.
Expected Output:
Highest and Lowest Marks in each subject:
Math: Highest = 40 , Lowest = 40
Science: Highest = 65 , Lowest = 65
English: Highest = 80 , Lowest = 80
Explanation:
This code represents a menu-driven program for managing student marks, where users can input student data, view marks, calculate averages, and find the highest and lowest marks in each subject.
- Initially, the program prompts the user for the number of students and then displays a menu with five options.
- The first option allows users to input marks for each student across three subjects: Math, Science, and English. However, an issue arises because the variables
math_marks
,science_marks
, andenglish_marks
are overwritten for each student, resulting in only the last student’s marks being stored. - The second option allows viewing all student marks, but again, due to overwriting, it only shows the last entered marks.
- The third option calculates the average marks for each student but encounters the same problem of storing only the last student’s marks.
- The fourth option displays the highest and lowest marks for each subject, but as with the other operations, it doesn’t track multiple students’ data properly. Lastly, the fifth option exits the program.
n = int(input("Enter the number of students: ")) # Menu-driven program while True: print("\nMenu:") print("1. Input student marks") print("2. View all student marks") print("3. Calculate average marks of each student") print("4. Display highest and lowest marks in each subject") print("5. Exit") choice = int(input("Enter your choice: ")) if choice == 1: # Input student marks print("Enter marks for ", n, " students:") student_counter = 1 while student_counter <= n: print("\nStudent ", student_counter, ":") math_marks = int(input("Enter Math marks: ")) science_marks = int(input("Enter Science marks: ")) english_marks = int(input("Enter English marks: ")) student_counter += 1 elif choice == 2: # View all student marks print("\nAll student marks:") student_counter = 1 while student_counter <= n: print("Student ", student_counter, ":") print("Math = ", math_marks, ", Science = ", science_marks, ", English = ", english_marks) student_counter += 1 elif choice == 3: # Calculate average marks of each student print("\nAverage marks of each student:") student_counter = 1 while student_counter <= n: total_marks = math_marks + science_marks + english_marks average_marks = total_marks / 3 print("Student ", student_counter, "Average: ", average_marks) student_counter += 1 elif choice == 4: # Display highest and lowest marks in each subject print("\nHighest and Lowest Marks in each subject:") student_counter = 1 math_highest = math_marks math_lowest = math_marks science_highest = science_marks science_lowest = science_marks english_highest = english_marks english_lowest = english_marks while student_counter <= n: # Math if math_marks > math_highest: math_highest = math_marks if math_marks < math_lowest: math_lowest = math_marks # Science if science_marks > science_highest: science_highest = science_marks if science_marks < science_lowest: science_lowest = science_marks # English if english_marks > english_highest: english_highest = english_marks if english_marks < english_lowest: english_lowest = english_marks student_counter += 1 print("Math: Highest = ", math_highest, ", Lowest = ", math_lowest) print("Science: Highest = ", science_highest, ", Lowest = ", science_lowest) print("English: Highest = ", english_highest, ", Lowest = ", english_lowest) elif choice == 5: # Exit print("Exiting the program.") break else: print("Invalid choice, please try again.")
The Grand Prize Ceremony
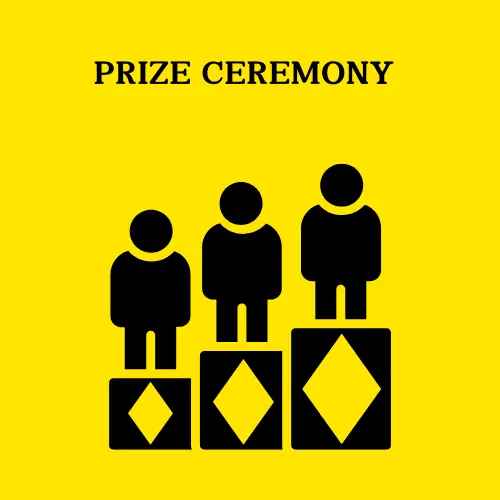
Imagine you are the event coordinator for a grand ceremony where participants are rewarded with special prizes based on their achievements. To add some excitement, you decide to create a diamond-shaped prize distribution pattern to award the prizes during the event.
The prize values are represented as square numbers, where the first prize is 1, the second prize is 4, the third is 9, and so on. The distribution follows a diamond pattern where each row represents a group of prizes being handed out.
The upper half of the ceremony represents the increasing number of participants who are receiving their prizes, while the lower half reflects the conclusion of the ceremony, where prizes are given back in reverse order. Write a Mojo program that generates this diamond-shaped prize distribution pattern, where the number of rows in the upper half of the diamond (i.e., the increasing number of participants) is provided by the user.
Test Case:
Input:
Enter the number of rows for the diamond: 4
Expected Output:
1
1 4
1 4 9
1 4 9 16
1 4 9
1 4
1
Explanation:
This code generates a diamond-shaped pattern using squares of numbers.
- The code begins by prompting the user to input the number of rows, stored in
n
, for the upper half of the diamond. - The program then uses a
while
loop to iterate through the rows, withrow
starting at 1 and increasing up ton
. For each row, the program first calculates the number of spaces required for center alignment by using anotherwhile
loop with the variablespaces
, where the number of spaces printed is determined by the difference betweenn
and the current row. - Following the spaces, another
while
loop is used to print the squares of numbers from1
to the currentrow
, with each square calculated ascolumn * column
, wherecolumn
starts at 1 and increases up to the currentrow
number. - Once the upper half is printed, the program moves to the lower half by decrementing
row
fromn - 1
and repeating the process. In this part, the number of spaces and the squares printed decreases in reverse order.
n = int(input("Enter the number of rows for the diamond: ")) # Upper half of the diamond row = 1 while row <= n: # Print leading spaces for center alignment spaces = 1 while spaces <= (n - row): print(" ", end=" ") spaces += 1 # Print the squares of the numbers for the current row column = 1 while column <= row: print((column * column), end=" ") column += 1 print() # Move to the next line after each row row += 1 # Lower half of the diamond (reverse of the upper half) row = n - 1 while row >= 1: # Print leading spaces for center alignment spaces = 1 while spaces <= (n - row): print(" ", end=" ") spaces += 1 # Print the squares of the numbers for the current row column = 1 while column <= row: print((column * column), end=" ") column += 1 print() # Move to the next line after each row row -= 1
The Curious Mathematician’s Experiment
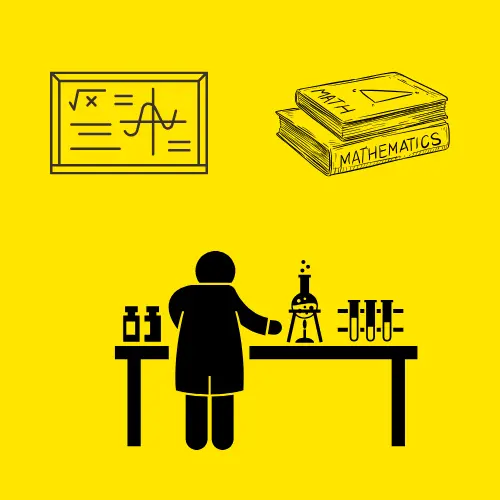
You are part of a team working on a groundbreaking mathematical experiment. The goal is to study factorials of numbers and understand their unique properties. A mysterious ancient mathematician left behind a hypothesis claiming that the sum of the digits of a factorial could reveal hidden patterns in numbers. To validate this, your team must:
Calculate the factorial of every number in a given range.
Determine the sum of the digits of each factorial.
Record the results to look for any potential patterns.
Your task is to write a program to perform this experiment. The program will take the starting and ending numbers of the range as input and display the factorial of each number and the sum of its digits.
Test Case:
Input:
Enter the starting number of the range: 4
Enter the ending number of the range: 6
Expected Output:
Number: 4
Factorial: 24
Sum of digits of factorial: 6
Number: 5
Factorial: 120
Sum of digits of factorial: 3
Number: 6
Factorial: 720
Sum of digits of factorial: 9
Explanation:
The program calculates the factorial of each number in a user-defined range and finds the sum of the digits of each factorial.
- The program begins by asking the user for a starting number (
start
) and an ending number (end
) to define a range of numbers. - It then uses a
while
loop to iterate through each number in the range, starting fromstart
and continuing untilend
. For each number in the range, the program calculates its factorial by initializing a variable calledfactorial
to1
and using anotherwhile
loop, where a counteri
starts from1
and increments up to the current number. In each iteration,factorial
is multiplied byi
, resulting in the factorial of the number. - After the factorial is calculated, the program determines the sum of the digits of the factorial. It initializes a variable
sum_of_digits
to0
and creates a copy of thefactorial
value in a variable namedtemp
. Using anotherwhile
loop, it repeatedly extracts the last digit oftemp
using the modulus operator (temp % 10
), adds it tosum_of_digits
, and removes the last digit fromtemp
by dividing it by10
using integer division (temp // 10
). This process continues until all digits are processed andtemp
becomes0
. - The program then displays the current number (
num
), its factorial (factorial
), and the sum of the digits of the factorial (sum_of_digits
). Finally, the loop incrementsnum
to move to the next number in the range.
start = int(input("Enter the starting number of the range: ")) end = int(input("Enter the ending number of the range: ")) num = start while num <= end: # Calculate the factorial factorial = 1 i = 1 while i <= num: factorial *= i i += 1 # Calculate the sum of the digits of the factorial sum_of_digits = 0 temp = factorial while temp > 0: digit = temp % 10 sum_of_digits += digit temp = temp // 10 print("Number:", num) print("Factorial:", factorial) print("Sum of digits of factorial:", sum_of_digits) print() num += 1
Transaction Calculator for Sales and Discounts
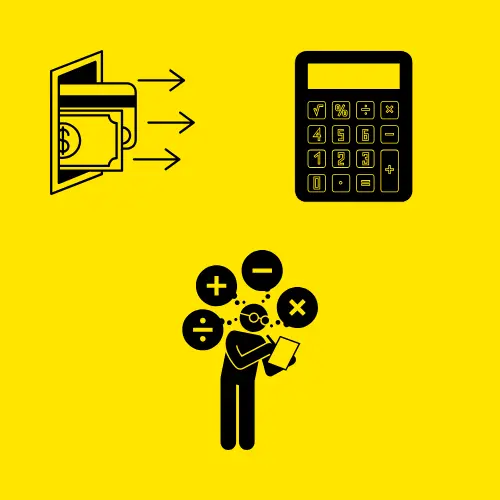
You are managing a small business where you handle various sales transactions daily. To assist in calculating the results of different transactions, you need to create a transaction calculator that can perform basic arithmetic operations like addition, subtraction, multiplication, and division.
Your task is to build a simple calculator that can take inputs from the user, perform calculations, and print the results. This calculator should be able to continue asking for more operations or exit based on user input. For example, the business owner might want to calculate the total price for products in a sale (addition), calculate the discount amount (multiplication or division), or check for returns (subtraction). They can continue calculating until they are done.
Test Case:
Input:
Enter the first number: 5
Enter the second number: 10
Enter the operation (+, -, *, /): +
Do you want to perform another operation (yes/no)? no
Exiting the calculator. Goodbye!
Expected Output:
Result: 15
Explanation:
This program is a simple calculator that performs basic arithmetic operations (addition, subtraction, multiplication, division) based on user input, and continues calculating until the user chooses to exit.
- The program begins by prompting the user to enter two numbers (
num1
andnum2
) and an arithmetic operation (operation
). - Based on the selected operation, the program calculates the result (
result
). If the operation is addition (+
), subtraction (-
), or multiplication (*
), the program directly performs the operation. - If the operation is division (
/
), it first checks ifnum2
is not zero to avoid division by zero errors. If the user inputs an invalid operation, the program prompts for the operation again. - Once the result is displayed, it asks whether the user wants to perform another operation. If the response (
continue_calculating
) is not “yes” or “no,” the program continues asking until a valid input is provided. If the user answers “no,” the program prints a goodbye message and exits. Here’s a flow of the program:
while True: num1 = int(input("Enter the first number: ")) num2 = int(input("Enter the second number: ")) operation = input("Enter the operation (+, -, *, /): ") # Perform the calculation based on the operation result = 0 if operation == "+": result = num1 + num2 elif operation == "-": result = num1 - num2 elif operation == "*": result = num1 * num2 elif operation == "/": if num2 != 0: result = num1 / num2 else: print("Error: Division by zero is not allowed.") continue else: print("Invalid operation! Please enter one of (+, -, *, /).") continue print("Result:", result) # Ask if the user wants to perform another operation continue_calculating = input("Do you want to perform another operation (yes/no)? ").lower() # Nested loop for the decision to continue or exit while continue_calculating != "yes" and continue_calculating != "no": print("Invalid input! Please type 'yes' or 'no'.") continue_calculating = input("Do you want to perform another operation (yes/no)? ").lower() # Break the outer loop if user decides to exit if continue_calculating == "no": print("Exiting the calculator. Goodbye!") break
Understanding nested loops in Mojo helps you solve complex problems more easily. By practicing with real-life examples, you can improve your problem-solving skills and write better, more efficient code. The more you use loops, the easier it becomes to handle challenging tasks in programming.