In this article, you’ll learn how to use loops in the Mojo language, specifically focusing on “for” and “while” loops. We’ll go over the workings of each loop with real-world examples you can relate to, along with simple code snippets.
Real World Analogy of Loops
Imagine you’re stacking, packing, and labeling boxes one by one repeatedly until your goal is met. That’s how loops work in real life: they help you perform repetitive tasks efficiently. In programming, loops serve the same purpose by running a block of code over and over until a condition is met, making tasks like processing lists or automating actions simpler and quicker.
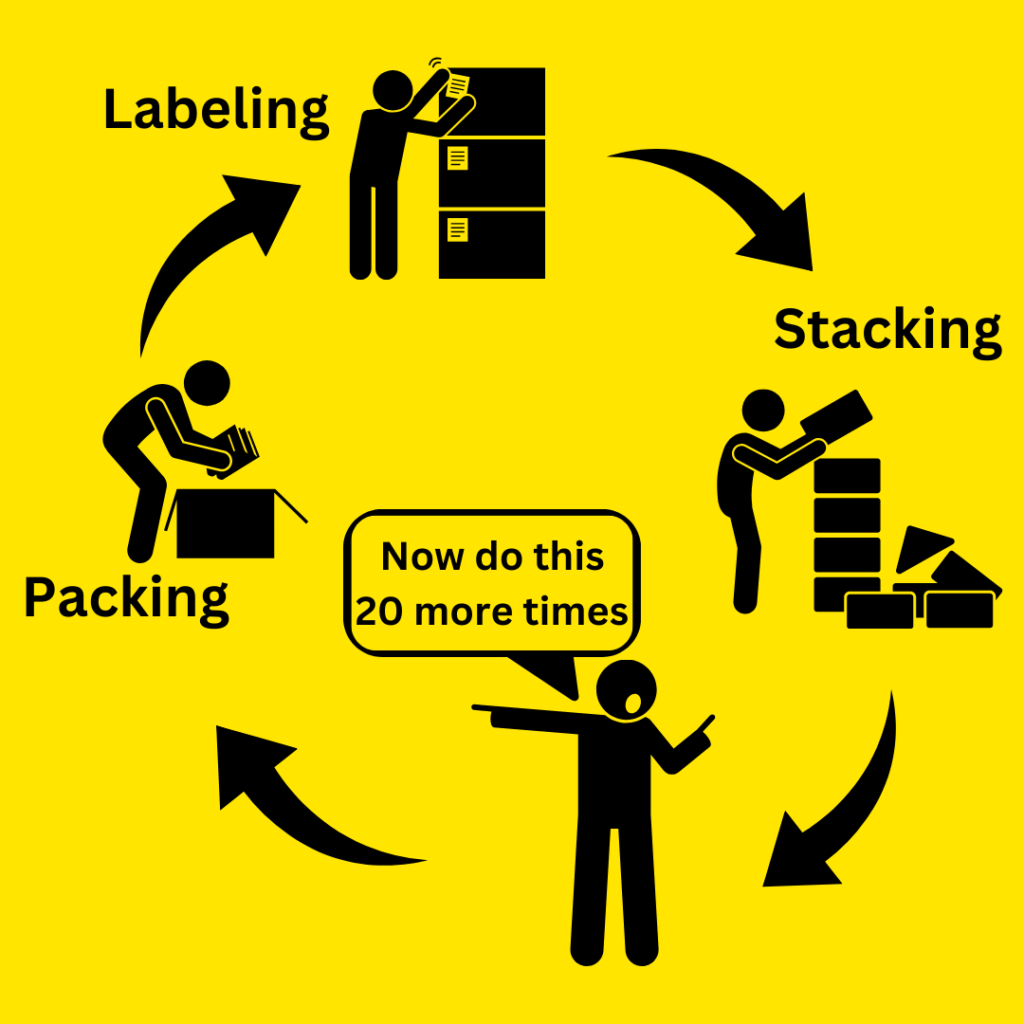
Loops in Mojo Programming
In Mojo programming, loops are used to repeat a block of code multiple times, which is helpful when performing the same task till a specific condition is met. Instead of writing the same code over and over, loops automate the repetition, making the program more short and organized. Sometimes, they are also used alongside conditional statements to make your code even more flexible.
Types of Loops in Mojo
Mojo programming language provides two types of loops for repeating specific tasks.
These are:
- for loop
- While loop
For loop in Mojo
A for
loop in Mojo repeats a certain part of code a fixed number of times that are known in advanced. Instead of manually keeping track of conditions, the loop automatically handles it for you, running the task for each item until it’s done. Once the loop has processed all the items, it stops.
The loop works with an iterator, which iterates over a list or an object and provides one item at a time. In a for
loop, the expression after the in
keyword must be iterable. An iterable is anything you can iterate by providing an iterator when asked. When the for
loop runs, it calls the iterable’s iter()
method, which gives the iterator. Each time the loop runs, the iterator’s next()
method provides the next item, and the loop uses that item to perform its task. The loop continues this process until no more items are left to process.
Real World Example of For Loop
Imagine you’re a teacher grading papers. The process involves Making a test, taking a test, and checking the test. Instead of checking each paper one by one without any structure, you can think of using a for loop. The loop would automatically move from one paper to the next, repeating the same grading process for each assignment, until all papers are graded. Just like a for loop goes through each item in a list, you go through each paper in the stack, performing the same task for everyone.
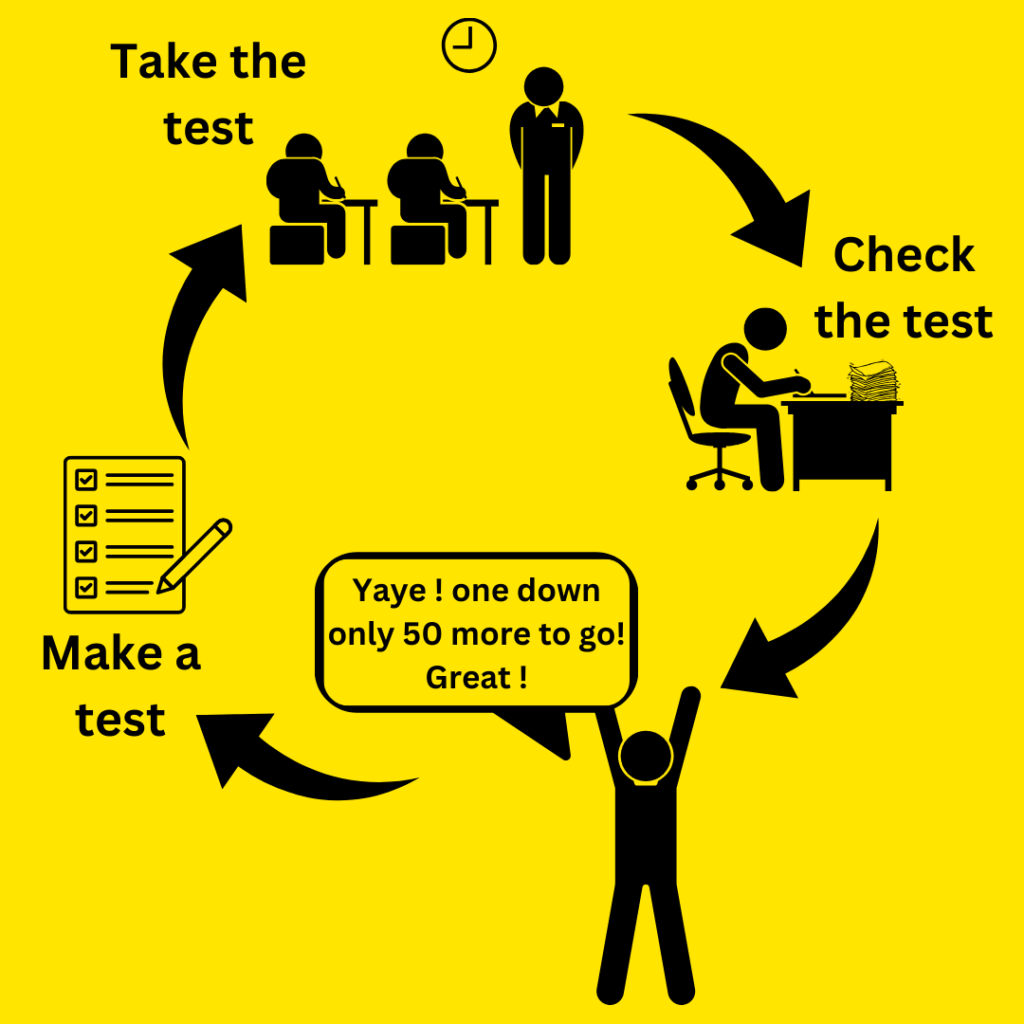
Flowchart of For Loop in Mojo
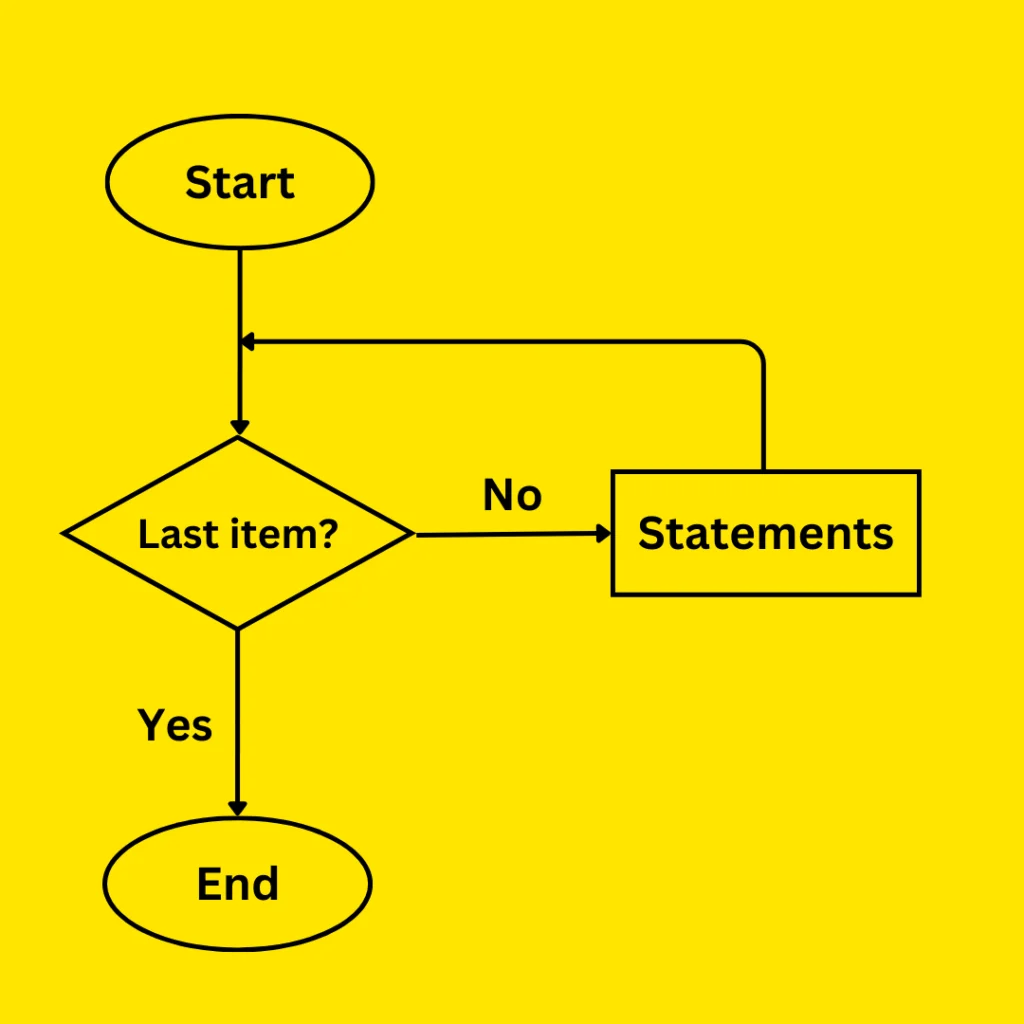
Syntax
for item in collection: # Code block to execute for each item
Example code
The is a code that prints numbers from 1-5 using a for loop in Mojo.
- The for loop iterates through a sequence of numbers generated by range(1, 6), which produces the numbers 1, 2, 3, 4, and 5.
- The loop variable i takes on each of these values, one at a time, during each iteration.
- print(i) is used inside the loop to output the current value of i to the console.
- The loop runs 5 times, printing each value of i from 1 to 5.
- The final result is each number from 1 to 5 printed on a new line.
for i in range(1, 6): print(i)
Output
1 2 3 4 5
While Loop in Mojo
A while
loop in Mojo runs a block of code repeatedly as long as a condition is true. Once the condition becomes false, the loop stops, and no further code is executed. This allows you to repeat tasks automatically without manually calling the same code again and again. While loops are used in situations where the exact number of iterations is not known in advanced.
Real World Example of While Loop
Imagine you’re filling a bottle with water, but you only want to stop once the bottle is full. You keep adding water until it reaches the desired level. In this case, the action of adding water is repeated as long as the bottle is not full, similar to how a while
loop repeats a task as long as a condition remains true. Once the bottle is full (condition becomes false), you stop adding water, just like a while
loop stops when the condition no longer holds.
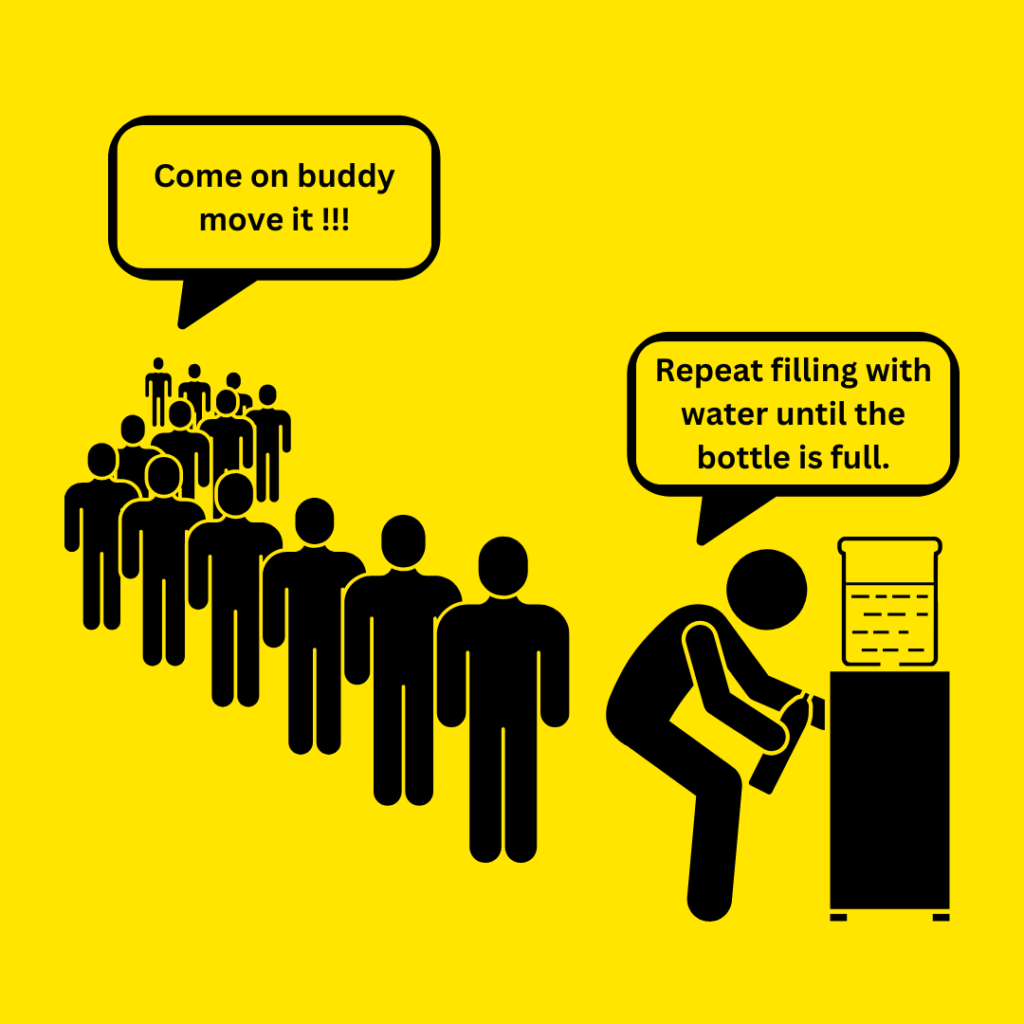
Flowchart of While Loop
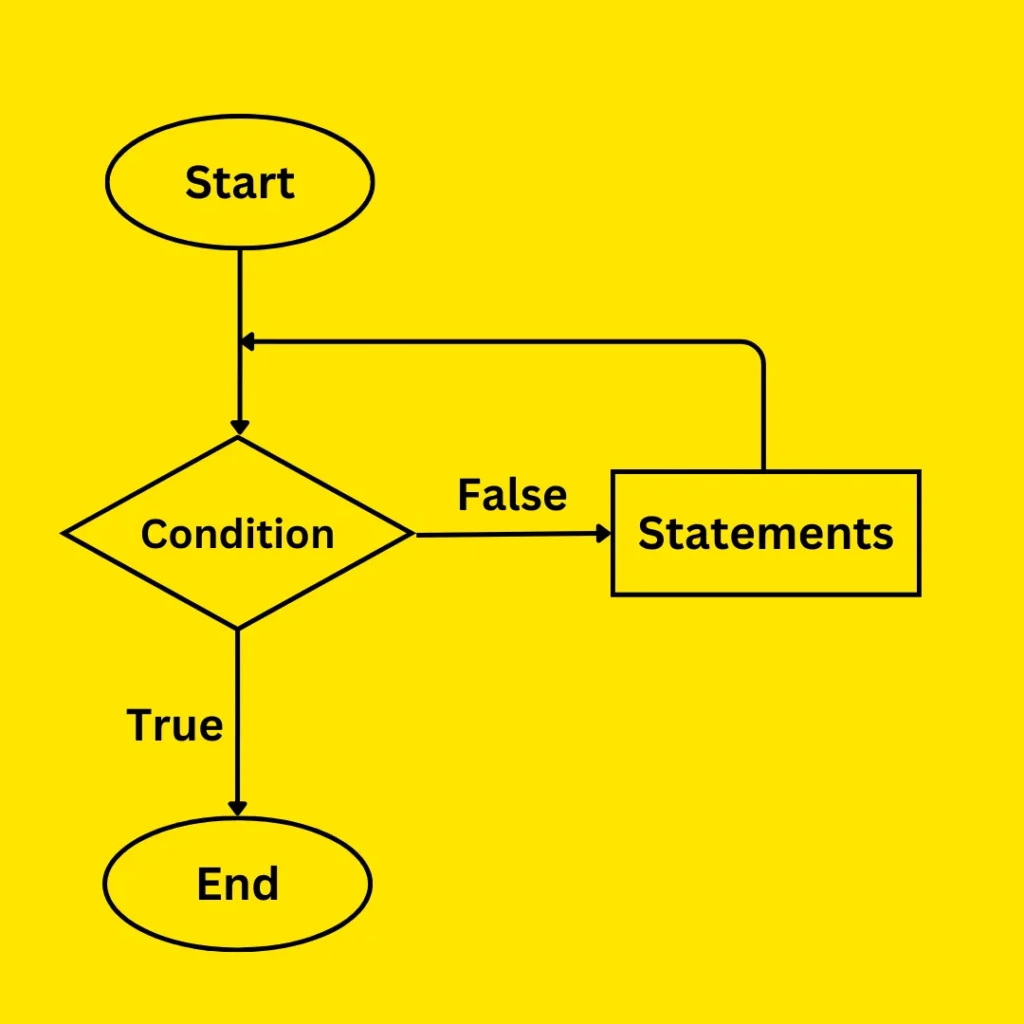
Syntax
while condition: # Code to execute repeatedly
Example Code
The is a code that prints numbers from 1-5 using a while loop in Mojo programming language
- The loop starts with n = 1.
- The condition n <= 5 is checked. If it’s True, the loop prints the value of n.
- After printing, the value of n is increased by 1 (n += 1).
- The loop continues until n becomes greater than 5, at which point it stops.
n = 1 while n <= 5: print(n) n += 1
Output
1 2 3 4 5
Jump Statements in Mojo
There are two basic Jump statements that are used in Mojo to control the loops behaviour.
These are:
- Continue Statement
- Break Statement
Continue Statement in Mojo
The continue
statement in Mojo loops is used to skip the current cycle and jump straight to the next one. If Mojo encounters continue
in a loop, it ignores any code that comes after it for that cycle and moves on to the next cycle of the loop. This allows you to skip certain conditions without ending the loop completely.
Break Statement Mojo
The break
statement in Mojo loops lets you exit the loop immediately. When Mojo encounters a break
, it immediately stops the loop, regardless of whether the loop’s condition is still true. This allows you to stop the loop early, which is useful when a specific condition is met and there’s no need to continue further cycles.
Using the Jump Statements in for Loop
The following code prints numbers from 1-5 but skips when the number 3 arrives and breaks if the number equals to 5.
- The loop cycles through the numbers 1 to 4.
- When i equals 3, the continue statement skips the print(i) statement and moves to the next iteration.
- The break statement completely stops the loop when i becomes 5.
for i in range(1, 6): if i == 3: continue # Skip printing 3 if i == 5: break # Stop the loop when i is 5 print(i)
Output
1 2 4
Using Jump Statements in While Loop
Here, code that prints numbers from 1-5 but skips when the number 3 arrives and breaks if the number equals to 5.
- We start by initialising i = 1.
- The while loop runs as long as i <= 5.
- When i == 3, the continue statement skips the print statement and goes to the next iteration.
- When i == 5, the break statement stops the loop entirely.
- The variable i is incremented at the end of each loop cycle to move to the next number.
i = 1 while i <= 5: if i == 3: i += 1 # Skip printing 3 continue if i == 5: break # Stop the loop when i is 5 print(i) i += 1
Output
1 2 4
Conclusion
In conclusion, you’ve learned about loops in Mojo, including how to use for
and while
loops effectively. We explored how loops help automate repetitive tasks and discussed important concepts like iterators and control statements such as continue
and break
. You also saw real-world examples and simple codes to solidify your understanding. Remember, loops are a powerful tool in programming, making your code more efficient and flexible.
As Syntax Scenarios aims to provide you with the best content that is easy to understand for beginners, you can explore our step-by-step guide to install Mojo and start exploring Mojo tutorials to learn more about this emerging language.