Loops are an important part of programming that lets you repeat tasks easily. In Mojo, loops help make your programs more efficient by automating repeated actions. In this article, you will learn how to use loops through a series of questions, starting from easy ones and moving to harder challenges. This will help you understand how loops can solve real-life problems and make your programs better.
Printing Numbers
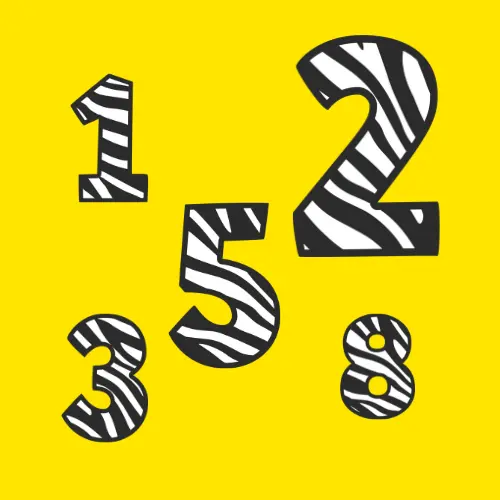
A teacher wants to teach students how to count. Write a Mojo program where the user inputs a number, and the program prints all numbers from 1 to the given number.
Test Case
Input:
Enter the maximum number: 10
Expected Output:
Counting from 1 to 10:
1
2
3
4
5
6
7
8
9
10
Explanation:
This program allows the user to input a maximum number (max_number
) and then counts and displays all the numbers from 1
up to max_number
, inclusive. It uses a for
loop to iterate through the range of numbers and prints each number on a new line.
- First, the program prompts the user to enter a number, which is then stored in the
max_number
. - The
for
loop is responsible for generating numbers starting from1
and ending atmax_number
. Therange(1, max_number + 1)
function creates a sequence of numbers from1
up to (and including)max_number
. Inside the loop, each number in the sequence is assigned to the variablei
, and theprint(i)
statement outputs the current value ofi
to the console.
max_number = int(input("Enter the maximum number: ")) print(f"Counting from 1 to {max_number}:") for i in range(1, max_number + 1): # Loop from 1 to the maximum number print(i)
Counting Down for a Rocket Launch
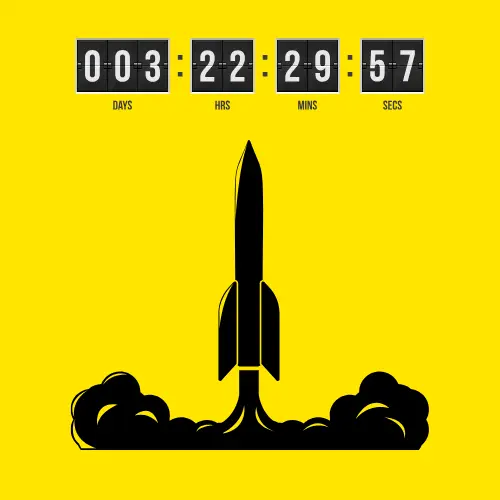
You are building a countdown program for a rocket launch. Write a Mojo program that starts from a user-provided number (e.g., 10) and counts down to 1, displaying “Liftoff!” at the end. Use a while loop.
Test Case
Input:
Enter the starting countdown number: 5
Expected Output:
Countdown begins:
5
4
3
2
1
Liftoff!
Explanation:
This program performs a countdown starting from a user-defined number and continues decrementing until it reaches 0.
- The program begins by asking the user to enter a number, which is stored in
count
. - The
while
loop ensures the countdown continues as long as the value ofcount
is greater than0
. Thecount -= 1
decreases the value ofcount
by1
after each iteration. - Once the
while
loop conditioncount > 0
is no longer true, the loop exits.
count = int(input("Enter the starting countdown number: ")) print("Countdown begins:") while count > 0: print(count) count -= 1 print("Liftoff!")
The sum of Digits of a Number
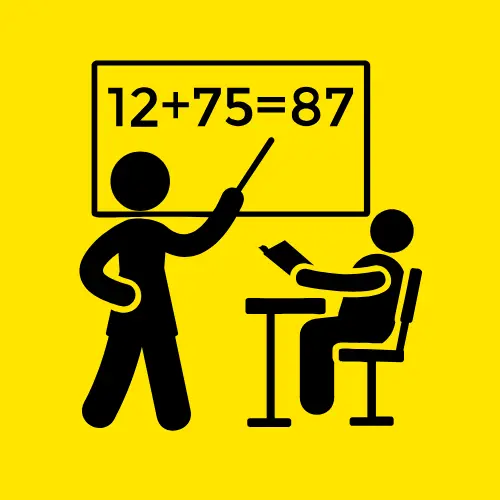
A cashier needs a program to calculate the sum of the digits of a number entered by a user. Write a Mojo program to find the sum of digits using a while loop.
Test Case
Input:
Enter a number: 1234
Expected Output:
Sum of digits in 1234 is: 10
Explanation:
This program calculates the sum of the digits of a number entered by the user.
- It begins by prompting the user to input a number and store it in the variable
num
. - A variable
sum_digits
is initialized to0
to keep track of the cumulative sum of the digits. The program uses awhile
loop that continues as long as the value ofnum
is greater than0
. - Inside the loop, the last digit of
num
is extracted using the modulus operation (num % 10
) and added tosum_digits
. The value ofnum
is then reduced by removing its last digit, achieved by integer division (num //= 10
). This process repeats until all the digits of the number have been processed, at which point the loop ends.
num = int(input("Enter a number: ")) sum_digits = 0 while num > 0: sum_digits += num % 10 num //= 10 print(f"Sum of digits is: {sum_digits}")
Multiplication Table
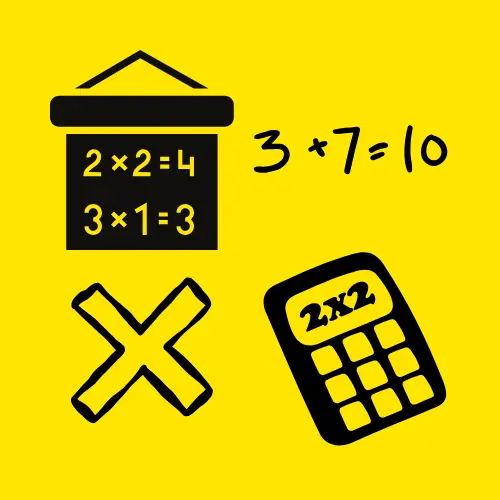
Write a Mojo program where the user enters a number, and the program prints its multiplication table up to 10.
Test Case
Input:
Enter a number: 7
Expected Output:
Multiplication table for 7:
7 x 1 = 7
7 x 2 = 14
7 x 3 = 21
7 x 4 = 28
7 x 5 = 35
7 x 6 = 42
7 x 7 = 49
7 x 8 = 56
7 x 9 = 63
7 x 10 = 70
Explanation:
This program generates the multiplication table for a number provided by the user.
- It begins by prompting the user to enter a number which is then stored in the variable
num
. - A
for
loop is used to iterate through the numbers from1
to10
. During each iteration, the program multiplies the input numbernum
by the current loop variablei
and prints the result in the format{num} x {i} = {num * i}
.
num = int(input(Enter a number: ")) print(f"Multiplication table for {num}:") for i in range(1, 11): print(f"{num} x {i} = {num * i}")
The sum of Odd Numbers
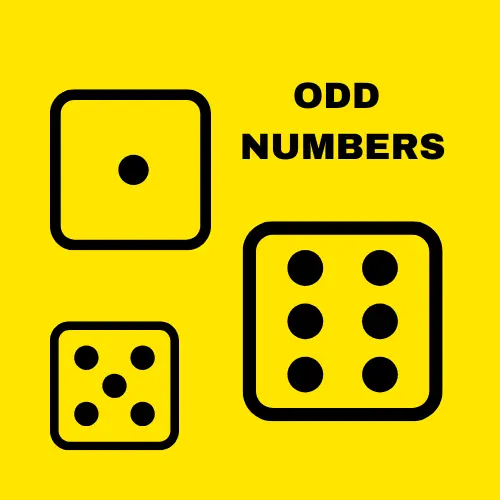
Write a Mojo program where the user enters a number. The program should calculate and display the sum of all odd numbers between 1 and the entered number.
Test Case
Input:
Enter a number: 10
Expected Output:
Sum of odd numbers between 1 and 10 is: 25
Explanation:
This program calculates the sum of all odd numbers between 1
and a user-provided number.
- The program begins by prompting the user to input a number.
- A variable
sum_odd
is initialized to0
to hold the cumulative sum of odd numbers. - The
for
loop,for i in range(1, num + 1, 2):
, is designed to iterate from1
tonum
, incrementing by2
to only include odd numbers. During each iteration, the current odd number (i
) is added tosum_odd
. - Once the loop completes, the program prints the total sum of odd numbers between
1
andnum
.
num = int(input("Enter a number: ")) sum_odd = 0 for i in range(1, num + 1, 2): sum_odd += i print(f"Sum of odd numbers between 1 and {num} is: {sum_odd}")
Counting Days to Reach a Savings Goal
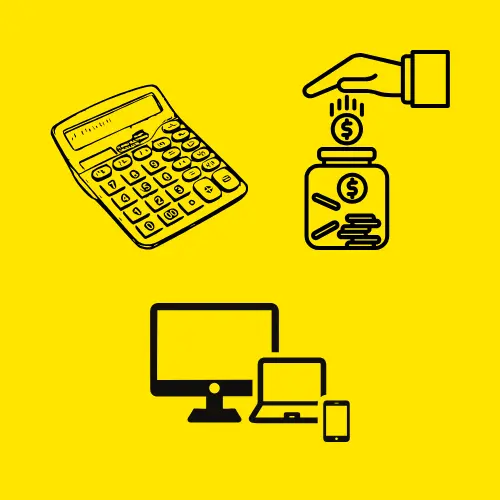
You want to save money for a new gadget that costs $100. You plan to save $3 every day. Write a Mojo program that calculates how many days it will take to reach or exceed your savings goal.
Test Case
Input:
Enter the cost of the gadget: 100
Enter your daily savings amount: 3
Expected Output:
It will take 34 days to save $100.
Explanation:
This program calculates the days required to save enough money to purchase a gadget based on the user’s daily savings.
- The program begins by asking the user to enter the cost of the gadget and daily savings amount which is then stored in the variables
goal
anddaily_savings
respectively. - Two variables,
days
andtotal_savings
, are initialized to0
to keep track of the number of days and the cumulative savings. - The
while
loop,while total_savings < goal:
, continues to execute as long as thetotal_savings
is less than thegoal
. Inside the loop, the variabledays
is incremented by1
for each day, and the daily savings amount is added tototal_savings
. Once the total savings are equal to or greater than the goal, the loop terminates.
goal = int(input("Enter the cost of the gadget:")) daily_savings = int(input("Enter your daily savings amount: ")) days = 0 total_savings = 0 while total_savings < goal: days += 1 total_savings += daily_savings print(f"It will take {days} days to save ${goal}.")
Plant Growth Tracker
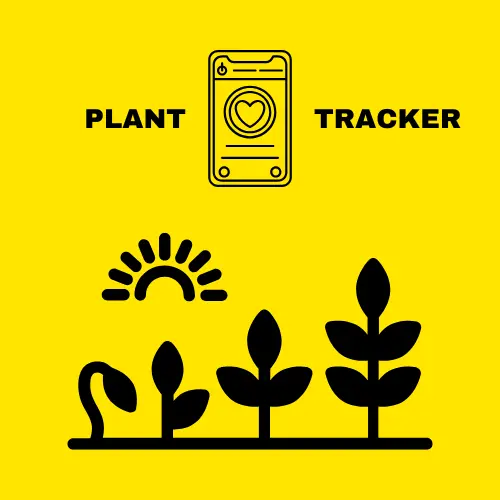
You are tracking the growth of a plant. Every day, the plant grows by 2 cm. On weekends (Saturdays and Sundays), the plant grows 3 cm. Write a Mojo program that tracks the plant’s growth over 30 days and displays the total growth after 30 days.
Test Case
Input:
Enter the number of days to track: 30
Expected Output:
Total growth after 30 days: 75 cm
Explanation:
This program tracks the growth of a plant over a user-specified number of days, considering different growth rates for weekdays and weekends.
- The program starts by asking the user to input the number of days they want to track.
- It then initializes two variables,
growth
(set to0
to store the cumulative growth) and the daily growth rates:daily_growth
(set to2
cm for weekdays) andweekend_growth
(set to3
cm for weekends). - The
for
loop, iterates over each day from1
todays_to_track
. Inside the loop, it checks if the current day is a weekend by evaluatingif day % 7 == 6 or day % 7 == 0:
. This condition checks if the day is either the 6th or 7th day of the week (Saturday or Sunday, based on zero-indexing). If it is a weekend, the plant’s growth is incremented byweekend_growth
(3 cm). Otherwise, for weekdays, the growth is incremented bydaily_growth
(2 cm).
days_to_track = int(input("Enter the number of days to track:")) growth = 0 daily_growth = 2 weekend_growth = 3 for day in range(1, days_to_track + 1): if day % 7 == 6 or day % 7 == 0: growth += weekend_growth else: growth += daily_growth print(f"Total growth after {days_to_track} days: {growth} cm")
Managing Weekly Grocery Shopping
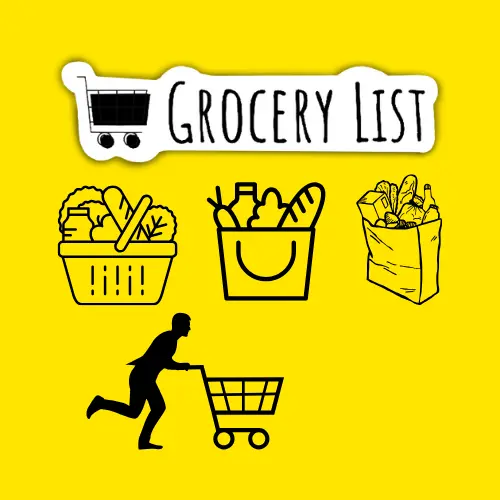
You have a weekly grocery budget of $100. Each item you buy has a specific price, and you want to calculate how many items you can buy without exceeding your budget. Write a Mojo program that will keep track of the total cost and stop when the total exceeds $100. Display the number of items you can buy and the total cost.
Test Case
Input:
Enter the price of item 1: 25
Enter the price of item 2: 30
Enter the price of item 3: 50
Enter the price of item 4: 10
Expected Output:
Item 1: $25
Item 2: $30
Item 3: $50
You can buy 3 items without exceeding your budget.
Total cost: $105
Explanation:
This program helps the user track the number of items they can purchase without exceeding a predefined budget.
- The program starts by setting the
budget
to100
, initializingtotal_cost
anditem_count
to0
. Thewhile
loop continues as long astotal_cost
is less than or equal to thebudget
. - Inside the loop, the program asks the user to enter the price of the next item. It then checks if adding the price of the current item to the
total_cost
would exceed the budget. If so, thebreak
statement stops the loop, preventing the user from purchasing the item. Otherwise, it adds the price tototal_cost
and incrementsitem_count
to track how many items have been bought.
budget = 100 total_cost = 0 item_count = 0 while total_cost <= budget: print("Enter the price of item", item_count + 1, ":") item_price = int(input()) if total_cost + item_price > budget: break total_cost += item_price item_count += 1 print(f"Item {item_count}: ${item_price}") print(f"You can buy {item_count} items without exceeding your budget.") print(f"Total cost: ${total_cost}")
The Mysterious Fibonacci Sequence
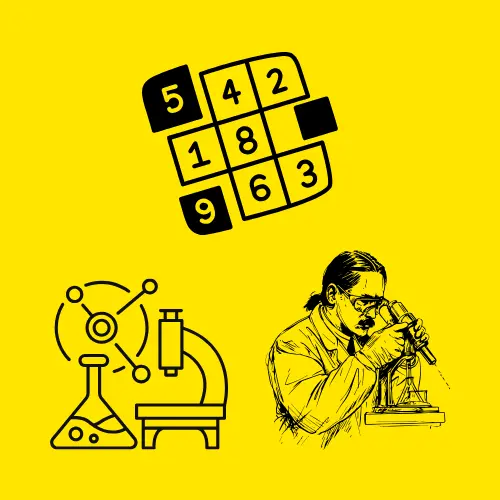
A scientist is studying a sequence of mysterious numbers related to the Fibonacci series, but there’s a twist. The Fibonacci sequence is modified:
The first number is user-defined (let’s call it X
).
The second number is also user-defined (let’s call it Y
).
Every subsequent number is calculated as the sum of the last two numbers in the sequence, similar to Fibonacci, but with the given X
and Y
.
You are required to calculate the numbers in this modified Fibonacci sequence, but you must stop the sequence when the numbers exceed 100.
Test Case
Input:
Enter the first number (X): 2
Enter the second number (Y): 3
Expected Output:
Fibonacci Sequence: 2, 3, 5, 8, 13, 21, 34, 55, 89
Explanation:
This program generates a Fibonacci sequence starting with two numbers provided by the user.
- The program begins by prompting the user to input the two numbers. These numbers are stored in the variables
X
andY
, which are then used to initialize two other variables,a
andb
, representing the first two numbers of the sequence. - The sequence begins by printing the values of
a
andb
. Awhile
loop is used to generate the next number in the sequence by addinga
andb
. The sum is stored in variablec
, and this value is printed. Ifc
exceeds100
, the loop breaks and the sequence generation stops. - Otherwise, the values of
a
andb
are updated for the next iteration, witha
being assigned the value ofb
, andb
being assigned the value ofc
. The program continues to print the numbers in the sequence until the condition is met.
X = int(input("Enter the first number (X): ")) Y = int(input("Enter the second number (Y): ")) # Initialize the sequence with X and Y a = X b = Y print("Fibonacci Sequence:") print(a, end=", ") print(b, end=", ") # Generate the next numbers in the sequence while True: c = a + b if c > 100: break print(c, end=", ") a = b b = c
Validate a Transaction ID
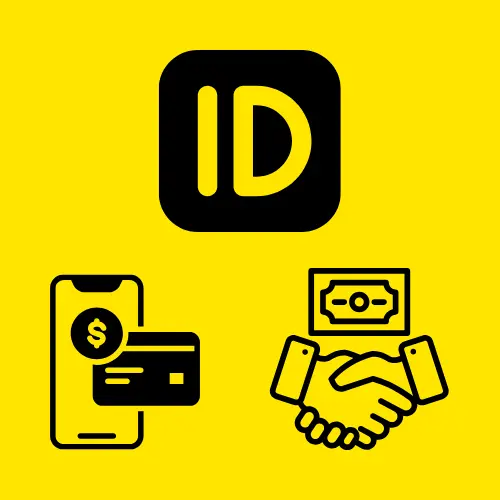
In a banking application, each transaction is assigned a unique transaction ID. However, to enhance security, the system requires that:
The transaction ID must be a positive integer greater than 1000.
The transaction ID must not contain any zeros, as the system identifies zero as a placeholder for invalid entries.
If the transaction ID contains a zero, the system should alert the user and reject the reversal request.
The system then reverses the transaction ID after validating it and prints the reversed ID.
Test Case
Input:
Enter the transaction ID: 87654321
Expected Output:
The reversed transaction ID is: 12345678
Explanation:
This program processes a transaction ID entered by the user, validating and reversing the ID if it meets the necessary conditions.
- The program starts by prompting the user to enter a transaction ID.
- It then checks if the transaction ID is less than or equal to 1000. If the ID is valid, the program proceeds with the validation check to ensure it doesn’t contain any zeros. This is done using a
while
loop that checks each digit by taking the modulus of 10 (temp_id % 10
). If any digit is zero, the ID is marked as invalid and the loop breaks. If no zeros are found, the program moves on to reverse the digits of the transaction ID. - To reverse the ID, the program uses another
while
loop. In each iteration, the last digit oftransaction_id
is added toreversed_id
, and the transaction ID is updated by removing its last digit. The reversed ID is printed once the loop completes.
transaction_id = int(input("Enter the transaction ID: ")) if transaction_id <= 1000: print("Invalid Transaction ID - Must be greater than 1000") else: temp_id = transaction_id is_valid = True while temp_id > 0: if temp_id % 10 == 0: is_valid = False break temp_id //= 10 if not is_valid: print("Invalid Transaction ID - Contains Zero") else: reversed_id = 0 while transaction_id > 0: reversed_id = reversed_id * 10 + transaction_id % 10 transaction_id //= 10 print("The reversed transaction ID is: ", reversed_id)
Diamond Star Pattern for Event Decoration
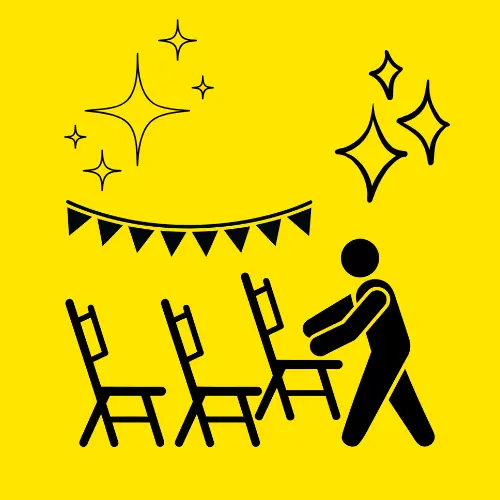
You are tasked with designing a diamond-shaped star pattern for event decoration. The height of the diamond’s top half is determined by a user input n
. The number of stars increases and then decreases as you move from the top row to the bottom, creating a diamond shape.
The user will provide an integer n
representing the height of the diamond’s top half.
The program should print a diamond pattern where:
The first half consists of rows of stars increasing in number.
The second half consists of rows of stars decreasing in number.
The number of stars in each row follows a sequence:
Row 1: 1 star
Row 2: 3 stars
Row 3: 5 stars, and so on for the first half.
The second half reverses this sequence.
The program should print the pattern using loops without doing any calculations in the print
statement.
Test Case
Input:
Enter the number of rows for the top half of the diamond: 4
Expected Output:
*
***
*****
*******
*********
*******
*****
***
*
Explanation:
This program generates a diamond pattern using stars (*
) based on the number of rows specified by the user for the top half of the diamond.
- It begins by asking the user to input the number of rows for the top half, where
n
determines the height and width of the top half of the diamond. - The program then uses two loops: the first loop generates the top half, and the second generates the bottom half. In the first loop, for each row from
1
ton
, the program calculates the number of spaces and stars. The number of spaces (spaces = n - i
) ensures that the stars are centered, and the number of stars (stars = 2 * i - 1
) starts with 1 star and increases by 2 stars with each row. - The second loop, which runs from
n - 1
down to1
, mirrors the first loop, decreasing the number of stars and spaces symmetrically to create the bottom half of the diamond. The final result is a diamond shape where the top half gradually increases in size and the bottom half symmetrically decreases.
n = int(input("Enter the number of rows for the top half of the diamond: ")) # Loop to print the top half of the diamond for i in range(1, n + 1): spaces = n - i stars = 2 * i - 1 print(' ' * spaces + '*' * stars) # Loop to print the bottom half of the diamond for i in range(n - 1, 0, -1): spaces = n - i stars = 2 * i - 1 print(' ' * spaces + '*' * stars)
Employee ID Armstrong Validation
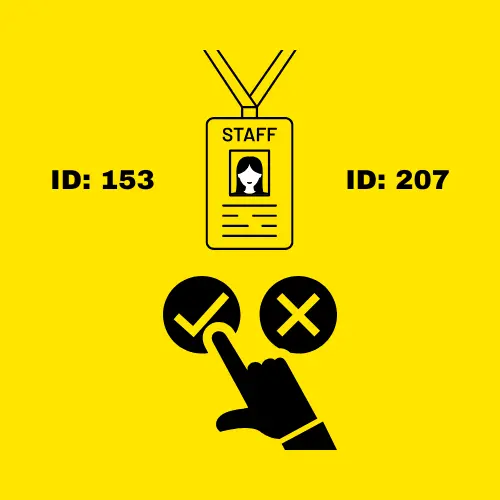
You work for a tech company that uses special Employee IDs. The company follows a unique rule for generating valid IDs. For a 3-digit Employee ID, the number must be an Armstrong Number. This means that the sum of the cubes of the digits of the ID must be equal to the ID itself.
As part of your validation system, you need to write a Mojo program to:
Take a 3-digit Employee ID from the user.
Check if the entered ID number is a valid Armstrong Number.
Print whether the ID is valid or invalid.
This validation ensures that only special IDs are issued, and the system uses the Armstrong Number property to enforce the rule.
Test Case
Input:
Enter the Employee ID (3-digit): 153
Expected Output:
The Employee ID 153 is valid (Armstrong Number).
Explanation:
This program checks whether a given employee ID (a 3-digit number) is a valid Armstrong number.
- The program starts by asking the user to enter a 3-digit employee ID. If the entered ID is not a 3-digit number (i.e., less than 100 or greater than 999), it prints a message asking the user to enter a valid 3-digit ID.
- If the ID is valid, the program proceeds to calculate the sum of the cubes of its digits. It extracts each digit of the ID using the modulus operator (
temp % 10
), cubes the digit, and adds it toarmstrong_sum
. - The ID is then divided by 10 to remove the last digit and continue with the next one. After calculating the sum of cubes, the program compares this sum with the original employee ID. If they are equal, it prints that the employee ID is valid and an Armstrong number; otherwise, it prints that the ID is invalid.
employee_id = int(input("Enter the Employee ID (3-digit): ")) if employee_id < 100 or employee_id > 999: print("Please enter a valid 3-digit Employee ID.") else: temp = employee_id armstrong_sum = 0 while temp > 0: digit = temp % 10 armstrong_sum += digit ** 3 temp = temp // 10 if armstrong_sum == employee_id: print(f"The Employee ID {employee_id} is valid (Armstrong Number).") else: print(f"The Employee ID {employee_id} is invalid (Not an Armstrong Number).")
Resource Scheduling for Project Teams
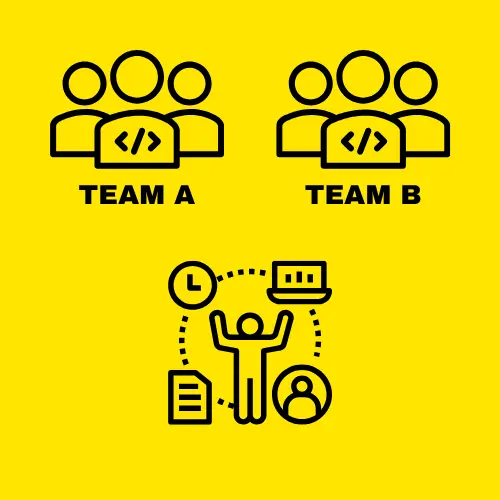
You are a project manager working on a multi-phase project with several teams. Each team has different work cycle times for completing their tasks, but you need to synchronize two specific teams’ tasks for resource allocation. You want to find out two key things:
LCM (Least Common Multiple): When both teams will complete their tasks at the same time again, i.e., the next time both teams will finish their tasks simultaneously.
HCF (Highest Common Factor): The greatest common factor of the two-cycle times, which helps identify the largest chunk of time that can be divided equally between both teams.
By calculating the LCM, you can plan when both teams will be able to align their schedules. On the other hand, calculating the HCF can help optimize resource allocation to avoid bottlenecks.
Test Case
Input:
Enter the 1st team cycle time: 12
Enter the 2nd team cycle time: 18
Expected Output:
LCM is: 36
HCF is: 6
Explanation:
This program calculates both the Least Common Multiple (LCM) and the Highest Common Factor (HCF) of two numbers, representing the cycle times of two teams.
- The program begins by asking the user to input two numbers,
num1
andnum2
, which represent the cycle times of two teams. - It then calculates the LCM by starting from the larger of the two numbers. It keeps increasing this value (
max_val
) until it finds a number that is divisible by bothnum1
andnum2
. Once such a number is found, it breaks out of the loop and prints the LCM. - After calculating the LCM, the program proceeds to calculate the HCF. It checks the smaller of the two numbers (
num1
ornum2
) and iterates down from this value to 1. For each iteration, it checks if bothnum1
andnum2
are divisible by the current value (i
). Once it finds the largest suchi
, it assigns this value tohcf
and breaks the loop. Finally, the program prints the HCF.
num1 = int(input("Enter the 1st team cycle time: ")) num2 = int(input("Enter the 2nd team cycle time: ")) if num1 > num2: max_val = num1 else: max_val = num2 while True: if max_val % num1 == 0 and max_val % num2 == 0: break max_val += 1 print(f"LCM is: {max_val}") if num1 < num2: smaller = num1 else: smaller = num2 for i in range(smaller, 0, -1): if num1 % i == 0 and num2 % i == 0: hcf = i break print(f"HCF is: {hcf}")
Menu-Driven Banking System
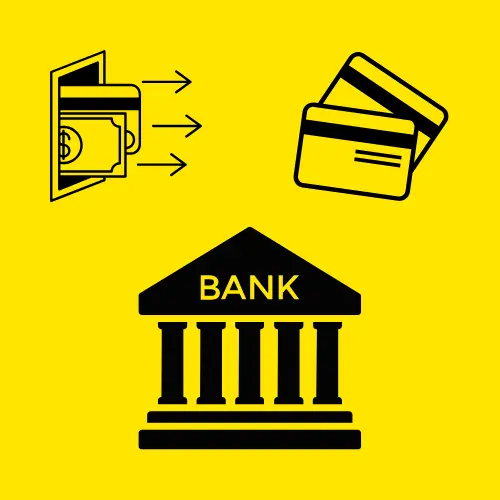
You are working on a simple banking system simulation for a small bank, where customers can interact with their accounts. The system should allow customers to perform various operations like checking account balances, depositing funds, withdrawing funds, and calculating simple interest for a given principal amount. The system will continue to offer these options until the customer decides to exit.
The program will:
Display a menu with operations.
Prompt the user to select an operation.
Continuously ask for the user’s input until the user selects Exit.
Test Case
Input:
Enter your choice: 1
Expected Output:
Account Balance: 1000
Explanation:
This program simulates a simple banking system where the user can check their balance, deposit money, withdraw money, calculate interest, or exit the system.
- The program starts with an initial balance of
balance = 1000
and presents the user with a menu of options. If the user chooses to check their balance (option1
), it simply displays the current balance. If the user selects the deposit option (2
), the program asks for the deposit amount (deposit
), adds it to the current balance, and prints the updated balance. - For withdrawals (option
3
), the program prompts the user to enter the withdrawal amount (withdraw
). It checks whether the withdrawal is possible by comparing it with the available balance. If the withdrawal is less than or equal to the balance, the system deducts the amount and shows the new balance. If not, it displays an error message. - When calculating interest (option
4
), the program asks for the principal (principal
), the interest rate (rate
), and the time period (time
). It computes the simple interest using the formula(principal * rate * time) / 100
and displays the result. If the user selects the exit option (5
), the program prints a message and terminates the loop. If the user enters an invalid choice, the program asks them to try again. The loop continues until the user chooses to exit.
balance = 1000 while True: print("\nChoose an operation:") print("1. Check Balance") print("2. Deposit") print("3. Withdraw") print("4. Calculate Interest") print("5. Exit") choice = int(input("Enter your choice: ")) if choice == 1: print(f"Account Balance: {balance}") elif choice == 2: deposit = int(input("Enter amount to deposit: ")) balance += deposit print(f"Deposited: {deposit}, New Balance: {balance}") elif choice == 3: withdraw = int(input("Enter amount to withdraw: ")) if withdraw <= balance: balance -= withdraw print(f"Withdrawn: {withdraw}, New Balance: {balance}") else: print("Insufficient funds. Withdrawal cannot be processed.") elif choice == 4: principal = int(input("Enter the principal amount: ")) rate = int(input("Enter the rate of interest: ")) time = int(input("Enter the time period: ")) interest = (principal * rate * time) / 100 print(f"Simple Interest: {interest}") elif choice == 5: print("Exiting the banking system.") break else: print("Invalid choice. Please try again.")
Challenge Bad Game
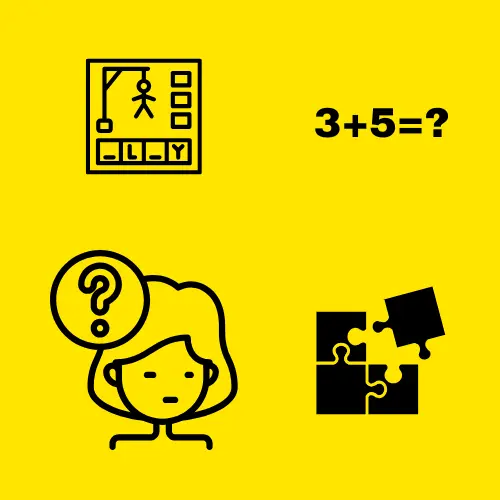
You’ve been selected to compete in a high-stakes competition hosted by a billionaire, who promises a hidden treasure to the first person to complete all three challenges. But there’s a catch: You’re out of the game if you fail any challenge.
Challenge 1: Guess a secret number between 1 and 100 within 5 attempts. You’ll get feedback after each guess to guide you.
Challenge 2: Type the word “Python” correctly within 3 attempts. If you make any mistake, you’ll have to start over.
Challenge 3: Solve a simple math problem: “What is 7 + 8?” within 3 attempts.
If you succeed in all challenges, the treasure is yours. Fail, and the game ends. Will you be able to pass the tests and claim the treasure, or will you fall short?
Test Case
Input:
Attempt 1/5: Guess the number: 30
Attempt 2/5: Guess the number: 50
Attempt 3/5: Guess the number: 60
Attempt 4/5: Guess the number: 20
Attempt 5/5: Guess the number: 40
Expected Output:
Too low! Try again.
Too high! Try again.
Too high! Try again.
Too low! Try again.
Too low! Try again.
Sorry, you’ve failed Challenge 1. The correct number was 45
Explanation:
This program is a fun “Challenge Bad Game” where the user has to complete three different challenges to win.
- Challenge 1: Guess the Number
The program asks the user to guess a number between 1 and 100. The target number is predefined astarget_number = 45
. The user has a maximum ofmax_attempts_1 = 5
attempts to guess the number correctly. If the user guesses too high or too low, they are prompted to try again. If the user runs out of attempts without guessing the correct number, the game exits, showing the correct number. - Challenge 2: Type the Word Correctly
The user is asked to type the word “Python” correctly. They are givenmax_attempts_2 = 3
attempts to input the correct word. If they input the wrong word, they are prompted to try again. If they fail after all attempts, the game exits. - Challenge 3: Solve a Math Problem
The user needs to solve the math problem7 + 8
. The correct answer is stored incorrect_answer = 15
. The user hasmax_attempts_3 = 3
attempts to input the correct answer. If they fail after all attempts, the game exits. - If the user passes all three challenges, they win the game and are congratulated with a message. The game is designed with clear feedback, allowing the user to retry until they either succeed or exhaust their attempts.
# Challenge 1: Guess the number target_number = 45 max_attempts_1 = 5 attempt_count_1 = 0 # Challenge 2: Type a word correctly correct_word = "Python" max_attempts_2 = 3 attempt_count_2 = 0 # Challenge 3: Solve a simple math problem correct_answer = 15 # 7 + 8 max_attempts_3 = 3 attempt_count_3 = 0 # Start the game print("Welcome to the Challenge Bad Game!") print("You will face 3 challenges. Pass them to win!") # Challenge 1: Guess the number print("\nChallenge 1: Guess the number between 1 and 100.") while attempt_count_1 < max_attempts_1: attempt_count_1 += 1 guess = int(input(f"Attempt {attempt_count_1}/{max_attempts_1}: Guess the number: ")) if guess == target_number: print("Correct! You've passed Challenge 1!") break elif guess < target_number: print("Too low! Try again.") else: print("Too high! Try again.") if attempt_count_1 == max_attempts_1: print("Sorry, you've failed Challenge 1. The correct number was", target_number) exit() # Challenge 2: Type a word correctly print("\nChallenge 2: Type the word 'Python' correctly.") while attempt_count_2 < max_attempts_2: attempt_count_2 += 1 word = input(f"Attempt {attempt_count_2}/{max_attempts_2}: Type the word: ") if word == correct_word: print("Correct! You've passed Challenge 2!") break else: print("Incorrect! Try again.") if attempt_count_2 == max_attempts_2: print("Sorry, you've failed Challenge 2. The correct word was 'Python'.") exit() # Challenge 3: Solve a simple math problem print("\nChallenge 3: Solve the math problem: 7 + 8.") while attempt_count_3 < max_attempts_3: attempt_count_3 += 1 answer = int(input(f"Attempt {attempt_count_3}/{max_attempts_3}: What is 7 + 8? ")) if answer == correct_answer: print("Correct! You've passed Challenge 3!") break else: print("Incorrect! Try again.") if attempt_count_3 == max_attempts_3: print("Sorry, you've failed Challenge 3. The correct answer was 15.") exit() # If all challenges are passed print("\nCongratulations! You've passed all challenges and won the game!")
In conclusion, loops in Mojo make it easy to handle tasks that repeat, like adding up numbers or checking conditions. By using loops in real-life situations, such as multiplication table or managing tasks, we can create simple programs that save time and effort. This shows how useful loops are in solving everyday problems in a clear and efficient way.