Looking for exciting coding challenges on 2D arrays in C? This article presents real-world scenario-based problems, from cinema seat booking to smart warehouse inventory management and flood monitoring systems. Perfect for C programmers who have mastered loops, if-else statements, and 1D arrays, these problems will enhance logical thinking and problem-solving skills. Each question comes with a detailed explanation, test case and solution code.
Cinema Seat Booking System
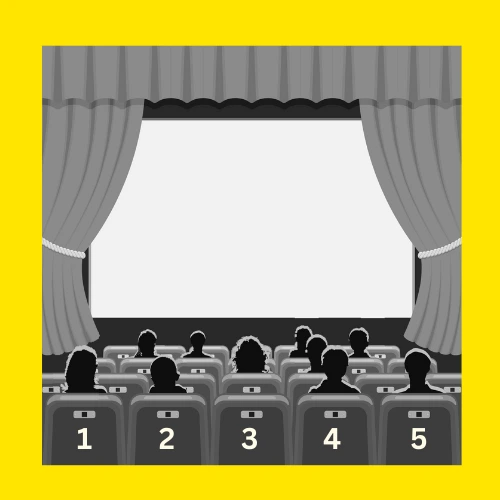
Imagine you are in charge of a small cinema hall with a 5×5 seating arrangement. Some seats are already booked, while others are still available. When a customer comes in, they ask for a specific seat. Write a C program that allows users to enter the row and column number of the seat they want. The program should check if the seat is available and book it if possible. If the seat is already booked, it should display a message informing the user.
Test Case
Input
Enter seat row (0-4): 2
Enter seat column (0-4): 1
Expected Output
Seat is available. Booking now…
- The program initializes a 5×5 2D array named
seats
, where0
represents an available seat and1
represents a booked seat. - The then program prompts the user to enter a row and column number (from 1 to 5).The entered values are stored in
row
andcol
. - As the array is zero-indexed, 1 is subtracted from
row
andcol
value to match the correct index. - The program checks if the input values are valid.
- If the seat is available (
seats[row][col] == 0
), the program books the seat by settingseats[row][col] = 1
and displays a confirmation message. - If the seat is already booked (
seats[row][col] == 1
), the program notifies the user.
#include <stdio.h> int main() { const int ROWS = 5, COLS = 5; int seats[5][5] = { {0, 1, 0, 0, 1}, {0, 0, 0, 1, 0}, {1, 0, 1, 0, 0}, {0, 1, 0, 1, 0}, {0, 0, 0, 0, 1} }; int row, col; printf("Enter seat row (1-5): "); scanf("%d", &row); printf("Enter seat column (1-5): "); scanf("%d", &col); row -= 1; col -= 1; if (row >= 0 && row < ROWS && col >= 0 && col < COLS) { if (seats[row][col] == 0) { printf("Seat is available. Booking now...\n"); seats[row][col] = 1; } else { printf("Sorry, this seat is already booked.\n"); } } else { printf("Invalid seat selection!\n"); } return 0; }
Chessboard Representation
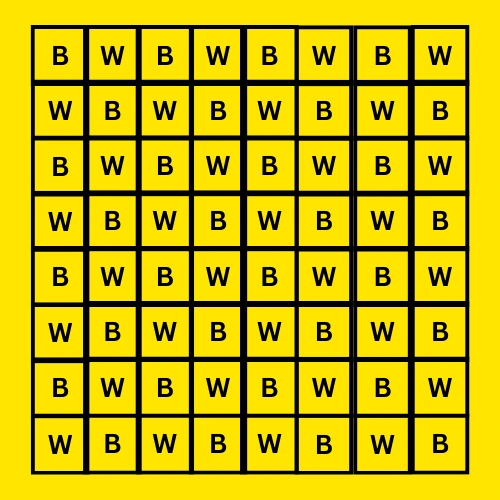
Imagine you are developing a chessboard visualization tool for a digital chess game. The chessboard consists of 8 rows and 8 columns, where each square alternates between black and white. The black squares are represented by ‘B’, and the white squares are represented by ‘W’. The game requires you to first generate the complete chessboard pattern and then display it exactly as it appears in a real chessboard. Write a C program that stores the chessboard pattern and then prints it while maintaining the correct alternating pattern.
Test Case
Expected Output
Chessboard Pattern:
B W B W B W B W
W B W B W B W B
B W B W B W B W
W B W B W B W B
B W B W B W B W
W B W B W B W B
B W B W B W B W
W B W B W B W B
- The program starts by defining the chessboard size as 8×8 and declares a 2D character array
board[8][8]
to store the chessboard pattern. - Next, the program uses a nested
for
loop to iterate through each cell of the 8×8 chessboard grid. The outer loop runs from0
to7
, representing the rows, while the inner loop runs from0
to7
, representing the columns. - For each cell, the program determines whether it should be a black (
'B'
) or white ('W'
) square based on the sum of the row index (i
) and column index (j
). - If the sum (i + j) is even, the square is assigned ‘
B
‘ for black. - If the sum (i + j) is odd, the square is assigned ‘
W
‘ for white. - Once the chessboard pattern is fully stored in the 2D array, the program proceeds to print the chessboard.
- To display the chessboard, another nested for loop iterates over the
board
array, printing each character with spaces in between for clear formatting. After printing each row, a newline character (\n
) moves to the next row to maintain the 8×8 grid structure. - After executing both loops, the program successfully prints a chessboard pattern with alternating black and white squares.
#include <stdio.h> int main() { int size = 8; char board[8][8]; for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { if ((i + j) % 2 == 0) { board[i][j] = 'B'; } else { board[i][j] = 'W'; } } } printf("Chessboard Pattern:\n"); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { printf(" %c ", board[i][j]); } printf("\n"); } return 0; }
Pixel Inversion
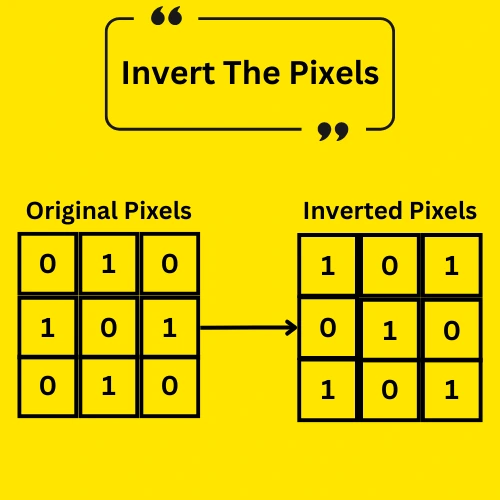
Imagine you are working on a simple image editing program that handles black-and-white images. These images are stored as a 5×5 grid, where 0 represents a black pixel and 1 represents a white pixel. Your task is to invert its colors by converting every 0 to 1 and every 1 to 0. After performing this transformation, you should display both the original and the inverted images so that the user can see the changes. Write a C program that takes a 5×5 binary image as input, applies the color inversion, and prints both the original and modified images.
Test Case
Input
Enter the 5×5 binary image (0 for black, 1 for white):
0 1 0 1 0
1 0 1 0 1
0 1 0 1 0
1 0 1 0 1
0 1 0 1 0
Expected Output
Original Image:
0 1 0 1 0
1 0 1 0 1
0 1 0 1 0
1 0 1 0 1
0 1 0 1 0
Inverted Image:
1 0 1 0 1
0 1 0 1 0
1 0 1 0 1
0 1 0 1 0
1 0 1 0 1
- The program declares a 5×5 2D array named
image
to store the user’s input. - The program prompts the user to enter each pixel value (
0
or1
) one by one using a nestedfor
loop. - A validation check ensures the user enters only
0
or1
. If invalid input is given, the user is asked to re-enter using a while loop. - After taking the input, it prints the original image by iterating through the
image
array. - Another loop iterates through the matrix and inverts each pixel using an
if-else
condition. - If the pixel is
0
, it is changed to1
. - If the pixel is
1
, it is changed to0
. - Finally, the new inverted image is displayed using nested
for
loops.
#include <stdio.h> int main() { const int SIZE = 5; int image[5][5]; printf("Enter the 5x5 binary image (0 for black, 1 for white):\n"); for (int i = 0; i < SIZE; i++) { for (int j = 0; j < SIZE; j++) { scanf("%d", &image[i][j]); while (image[i][j] != 0 && image[i][j] != 1) { printf("Invalid input! Enter 0 or 1: "); scanf("%d", &image[i][j]); } } } printf("\nOriginal Image:\n"); for (int i = 0; i < SIZE; i++) { for (int j = 0; j < SIZE; j++) { printf("%d ", image[i][j]); } printf("\n"); } for (int i = 0; i < SIZE; i++) { for (int j = 0; j < SIZE; j++) { if (image[i][j] == 0) { image[i][j] = 1; } else { image[i][j] = 0; } } } printf("\nInverted Image:\n"); for (int i = 0; i < SIZE; i++) { for (int j = 0; j < SIZE; j++) { printf("%d ", image[i][j]); } printf("\n"); } return 0; }
Daily Weather Tracker
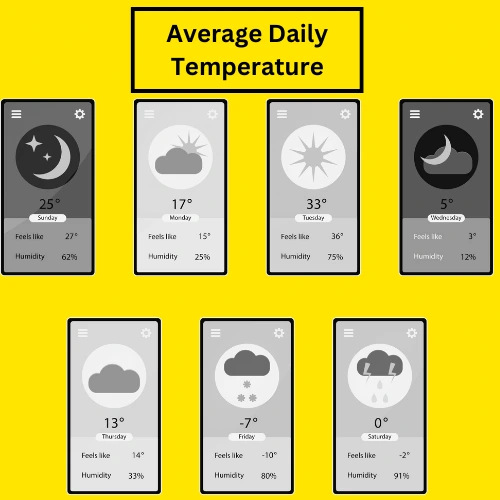
Imagine you are a weather analyst tracking temperature readings for a city. You record temperatures for 7 days at three different times of the day (Morning, Afternoon, and Evening). You need to analyze this data to find the average temperature for each day. Write a C program that takes daily temperature readings as input and calculates the average temperature for each day.
Test Case
Input
Enter temperatures for Day 1 (Morning, Afternoon, Evening): 20.5 25.3 22.1
Enter temperatures for Day 2 (Morning, Afternoon, Evening): 21.0 26.4 23.0
Enter temperatures for Day 3 (Morning, Afternoon, Evening): 18.9 24.5 21.3
Enter temperatures for Day 4 (Morning, Afternoon, Evening): 22.0 27.1 23.8
Enter temperatures for Day 5 (Morning, Afternoon, Evening): 19.5 23.9 22.4
Enter temperatures for Day 6 (Morning, Afternoon, Evening): 20.1 25.7 21.9
Enter temperatures for Day 7 (Morning, Afternoon, Evening): 21.3 26.2 22.5
Expected Output
Average Temperatures per Day:
Day 1: 22.63°C
Day 2: 23.47°C
Day 3: 21.57°C
Day 4: 24.30°C
Day 5: 21.93°C
Day 6: 22.57°C
Day 7: 23.33°C
- The program declares a 2D array named
temperatures
to store values for 7 days and 3 times per day. - A
for
loop iterates 7 times for each day. - Inside the loop, another
for
loop runs 3 times to take temperature inputs for morning, afternoon and evening. - The temperatures are stored in the array
temperatures[i][j]
wherei
represents the day, andj
represents the time of the day. - Another
for
loop is used to calculate the average temperature per day by summing up the three temperature readings and dividing by 3. - The calculated average is displayed for each day.
#include <stdio.h> int main() { const int DAYS = 7, TIMES = 3; float temperatures[7][3]; float dailyAverage; for (int i = 0; i < DAYS; i++) { printf("Enter temperatures for Day %d (Morning, Afternoon, Evening): ", i + 1); for (int j = 0; j < TIMES; j++) { scanf("%f", &temperatures[i][j]); } } printf("\nAverage Temperatures per Day:\n"); for (int i = 0; i < DAYS; i++) { dailyAverage = (temperatures[i][0] + temperatures[i][1] + temperatures[i][2]) / 3; printf("Day %d: %.2f°C\n", i + 1, dailyAverage); } return 0; }
Image Brightness Adjustment
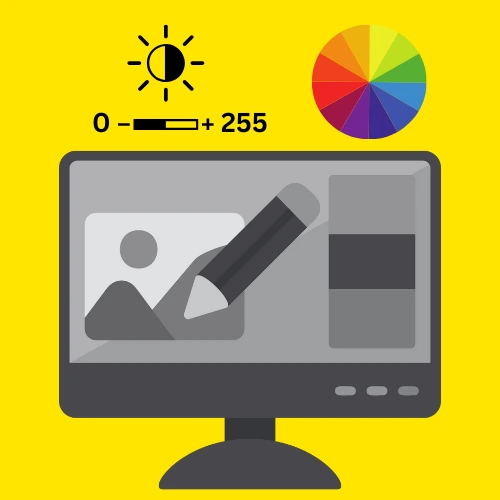
Imagine you are developing a digital photo editing application that allows users to enhance images by adjusting brightness levels. Each image is composed of small square blocks, each having a brightness value ranging from 0 (completely dark) to 255 (completely bright). Users can enter an adjustment value to increase the brightness of all blocks. However, since the brightness cannot exceed 255, any block that reaches this limit remains at 255, and brightness cannot go below 0. Write a C program that takes the image dimensions and pixel brightness values as input, displays the brightness values before modification, applies the brightness adjustment while ensuring values stay within the valid range, and then displays the updated brightness values after modification.
Test Case
Input
Enter number of rows: 2
Enter number of columns: 3
Enter the pixel brightness values (0-255):
120 200 150
80 50 30
Enter brightness increase value: 50
Expected Output
Original image brightness values:
120 200 150
80 50 30
Modified image brightness values:
170 250 200
130 100 80
- The program starts by asking the user to enter the number of rows and columns for the image. These inputs are saved in the variables
rows
andcols
, respectively. - Next, the program prompts the user to enter the brightness values for each pixel in the image. These values range from 0 (completely dark) to 255 (completely bright) and are stored in a 2D array called
image
. - A nested
for
loop is used to iterate over each position in the grid and take input from the user. - The program then asks the user to enter a brightness increase value, which is stored in the variable
brightnessIncrease
. This value determines how much each pixel’s brightness should be increased. - The program then displays the original brightness values by iterating through the
image
grid using another nestedfor
loop. - Next, the program adjusts the brightness values by iterating through the
image
grid again using a nestedfor
loop. - For each pixel the brightness value is increased by
brightnessIncrease
. - If the new brightness value exceeds
255
, it is set to255
to ensure it stays within the valid range. - If the new brightness value drops below
0
, it is set to0
to prevent underflow. - Once all brightness values have been adjusted, the program prints the modified image brightness values in grid format.
#include <stdio.h> int main() { int rows, cols, brightnessIncrease; printf("Enter number of rows: "); scanf("%d", &rows); printf("Enter number of columns: "); scanf("%d", &cols); int image[rows][cols]; printf("Enter the pixel brightness values (0-255):\n"); for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { scanf("%d", &image[i][j]); } } printf("Enter brightness increase value: "); scanf("%d", &brightnessIncrease); printf("Original image brightness values:\n"); for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { printf("%d ", image[i][j]); } printf("\n"); } for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { image[i][j] += brightnessIncrease; if (image[i][j] > 255) { image[i][j] = 255; } else if (image[i][j] < 0) { image[i][j] = 0; } } } printf("Modified image brightness values:\n"); for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { printf("%d ", image[i][j]); } printf("\n"); } return 0; }
Sales Tracker
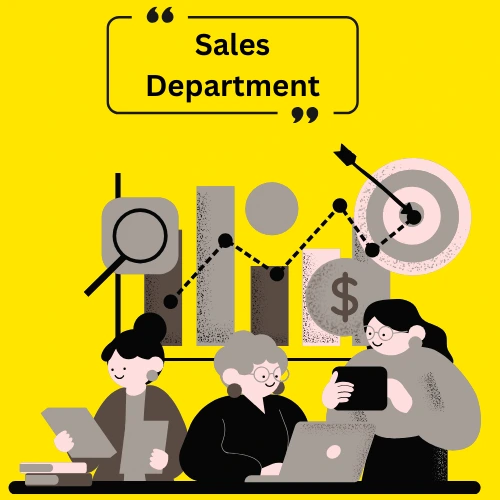
Imagine you work in a company’s sales department, and your job is to track monthly sales for different regions. The company operates in four regions: North, South, East, and West, and you need to record their sales for three months. Write a C program that takes the sales data for four regions over three months and calculates the total sales for each region.
Test Case
Input
Enter sales for Region 1 for 3 months: 100 200 150
Enter sales for Region 2 for 3 months: 250 300 280
Enter sales for Region 3 for 3 months: 180 210 190
Enter sales for Region 4 for 3 months: 300 320 310
Expected Output
Total Sales per Region:
Region 1: 450
Region 2: 830
Region 3: 580
Region 4: 930
- The program starts by defining two constants,
REGIONS
andMONTHS
, which store the number of sales regions and the number of months for which sales data is recorded. - The program then declares a 2D array named
sales
to store the monthly sales data for each region. It also declares a 1D array namedtotal
, which will store the total sales for each region. - Before collecting sales data, the program initializes all elements of the
total
array to 0 using afor
loop. - The program then prompts the user to enter sales data for each region over three months using a nested
for
loop. - The outer loop runs for each region, iterating from
0
toREGIONS - 1
. - The inner loop runs for each month, iterating from
0
toMONTHS - 1
. - For each region and month, the program takes an integer input from the user and stores it in the
sales
array. - The input sales value is added to the corresponding region’s total sales using
total[i] += sales[i][j]
. - After all sales data has been entered, the program calculates and displays the total sales per region using another
for
loop. - Once all regions have been processed, the program displays the total sales for each region.
#include <stdio.h> int main() { const int REGIONS = 4; const int MONTHS = 3; int sales[REGIONS][MONTHS]; int total[REGIONS]; for (int i = 0; i < REGIONS; i++) { total[i] = 0; } for (int i = 0; i < REGIONS; i++) { printf("Enter sales for Region %d for 3 months: ", i + 1); for (int j = 0; j < MONTHS; j++) { scanf("%d", &sales[i][j]); total[i] += sales[i][j]; } } printf("\nTotal Sales per Region:\n"); for (int i = 0; i < REGIONS; i++) { printf("Region %d: %d\n", i + 1, total[i]); } return 0; }
Top Scorer
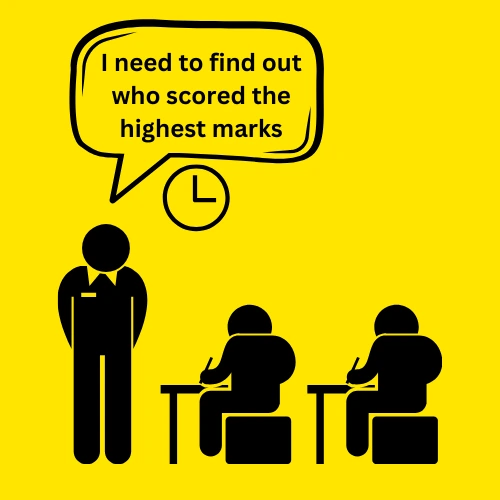
Imagine you are a teacher, and you need to analyze students’ scores in different subjects. You don’t know the exact number of students or subjects in advance, so you need a program that can handle any number of students and subjects. Write a C program that first takes the number of students and subjects as input, then collects scores, and finally finds and displays the highest marks in each subject.
Test Case
Input
Enter the number of students: 3
Enter the number of subjects: 2
Student 1:
Enter marks for Subject 1: 85
Enter marks for Subject 2: 90
Student 2:
Enter marks for Subject 1: 88
Enter marks for Subject 2: 92
Student 3:
Enter marks for Subject 1: 91
Enter marks for Subject 2: 89
Expected Output
Highest Marks in Each Subject:
Subject 1: 91
Subject 2: 92
- The program starts by asking the user to enter the number of students and subjects.
- The program then declares a 2D array named
scores
to store student marks for different subjects. - It also declares a 1D array
highestMarks
, which stores the highest score for each subject. - Before taking input, the program initializes all elements of
highestMarks
to 0. - The program then asks the user to enter marks for each student in each subject using a nested
for
loop: - The outer loop iterates through each student.
- The inner loop iterates through each subject.
- The program displays a message asking for marks for each subject. The input score is stored in the
scores
array. - The program compares the input score with the current highest score for that subject. If the new score is higher, it updates
highestMarks[j]
. - Once all scores are entered, the program displays the highest marks for each subject.
#include <stdio.h> int main() { int students, subjects; printf("Enter the number of students: "); scanf("%d", &students); printf("Enter the number of subjects: "); scanf("%d", &subjects); int scores[students][subjects]; int highestMarks[subjects]; for (int j = 0; j < subjects; j++) { highestMarks[j] = 0; } for (int i = 0; i < students; i++) { printf("\nStudent %d:\n", i + 1); for (int j = 0; j < subjects; j++) { printf("Enter marks for Subject %d: ", j + 1); scanf("%d", &scores[i][j]); if (scores[i][j] > highestMarks[j]) { highestMarks[j] = scores[i][j]; } } } printf("\nHighest Marks in Each Subject:\n"); for (int j = 0; j < subjects; j++) { printf("Subject %d: %d\n", j + 1, highestMarks[j]); } return 0; }
Flood Monitoring System
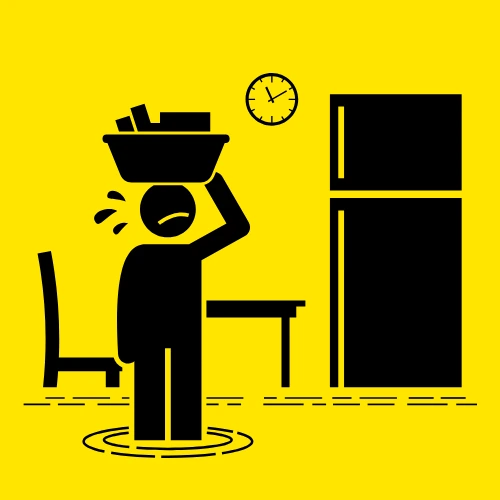
Imagine you are a disaster management officer responsible for monitoring flood risks in a city. The city is divided into small sections, like a grid, where each section has a specific elevation (height in meters). If any section’s elevation is lower than a given flood level, it is at risk of flooding. The flood control department provides you with the city’s grid size and the elevation values for each section. Your task is to create a flood risk map by marking the flooded areas with “X”, while the safe areas remain unchanged. Write a C program that takes the grid size, elevation values, and flood level as input and then displays the flood risk map.
Test Case
Input
Enter the number of rows: 3
Enter the number of columns: 3
Enter elevation values for each region:
10 12 8
15 5 18
7 14 9
Enter flood level threshold: 10
Expected Output
Flood Risk Map:
10 12 X
15 X 18
X 14 X
- The program begins by asking the user to enter the number of rows and columns in the city grid, storing these values in the
rows
andcols
variables. - Next, it initializes a 2D array
city[][]
to store the elevation values of each region. - The user is then prompted to enter the elevation values for every region in the city grid.
- After receiving the elevation data, the program asks for the flood level threshold, which is stored in the
flood_level
variable. - To generate the flood risk map, the program checks each cell in the grid.
- If the elevation value is below the flood level, it is marked as
X
to indicate flooding. - If the elevation value is above or equal to the threshold, it remains unchanged, indicating a safe region.
- Finally, the program prints the flood risk map using nested
for
loops.
#include <stdio.h> int main() { int rows, cols, flood_level; printf("Enter the number of rows: "); scanf("%d", &rows); printf("Enter the number of columns: "); scanf("%d", &cols); int city[rows][cols]; printf("Enter elevation values for each region:\n"); for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { scanf("%d", &city[i][j]); } } printf("Enter flood level threshold: "); scanf("%d", &flood_level); printf("\nFlood Risk Map:\n"); for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { if (city[i][j] < flood_level) { printf(" X "); } else { printf("%2d ", city[i][j]); } } printf("\n"); } return 0; }
Treasure Hunt Map
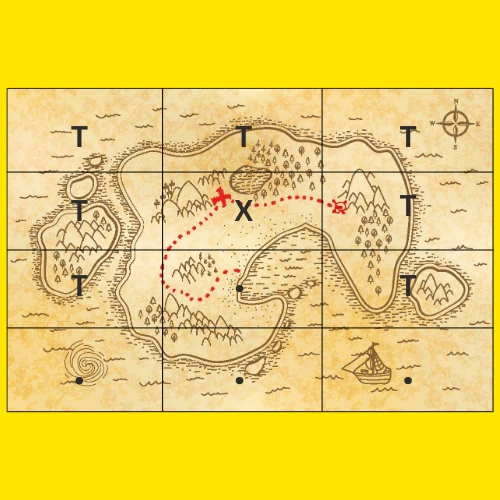
Imagine you are an adventurer who discovers an ancient treasure map hidden inside an old book. The map is a grid representing different locations on a mysterious island, where some spots have traps (T), some are empty (.), and one contains the hidden treasure (X). Your task is to create a system that takes input for the map’s size (rows and columns), stores it as a 2D grid, and allows the user to enter ‘T’ for traps, ‘.’ for empty spots, and ‘X’ for the treasure. The program should then search for the treasure’s location, display the entire map, and provide the exact coordinates of the treasure. Write a C program that allows users to input the treasure map, locate the treasure, and present the results in a clear format.
Test Case
Input
Enter the number of rows: 3
Enter the number of columns: 4
Enter the treasure map grid (3 x 4). Use only ‘T’ (trap), ‘.’ (empty), and ‘X’ (treasure):
Enter value for cell [1][1]: .
Enter value for cell [1][2]: T
Enter value for cell [1][3]: .
Enter value for cell [1][4]: T
Enter value for cell [2][1]: .
Enter value for cell [2][2]: X
Enter value for cell [2][3]: .
Enter value for cell [2][4]: T
Enter value for cell [3][1]: T
Enter value for cell [3][2]: .
Enter value for cell [3][3]: T
Enter value for cell [3][4]: .
Expected Output
Treasure Map:
. T . T
. X . T
T . T .
Treasure found at: Row 2, Column 2
- The program starts by asking the user to enter the number of rows and columns for the treasure map. These inputs are saved in the variables
rows
andcols
. - Next, the program declares a 2D character array
map[][]
to store the grid representation of the map. - The user is then prompted to enter a character for each cell in the grid using a nested
for
loop. - The program ensures that the user only enters valid characters (
'T'
for trap,'.'
for empty,'X'
for treasure) by using a while loop for input validation. If the user enters an invalid character, the program displays an error message and prompts for re-entry until a valid character is provided. - After storing the entire map, the program searches for the treasure (
'X'
) using another nestedfor
loop. - When the treasure is found, its row and column indices are stored in
treasureRow
andtreasureCol
, and the loop is exited to prevent unnecessary searches. - Once the treasure’s location is identified, the program displays the complete treasure map in its original format using another nested loop. Each element in the 2D array is printed with spaces in between to maintain the grid structure.
- Finally, the program checks if the treasure was found.
- If
treasureRow
andtreasureCol
contain valid indices (not-1
), it prints the exact location of the treasure in 1-based indexing by adding1
to the row and column values. - If no treasure is found, it displays a message indicating that the treasure is missing from the map.
#include <stdio.h> int main() { int rows, cols; printf("Enter the number of rows: "); scanf("%d", &rows); printf("Enter the number of columns: "); scanf("%d", &cols); char map[rows][cols]; printf("Enter the treasure map grid (%d x %d). Use only 'T' (trap), '.' (empty), and 'X' (treasure):\n", rows, cols); for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { while (1) { printf("Enter value for cell [%d][%d]: ", i + 1, j + 1); scanf(" %c", &map[i][j]); if (map[i][j] == 'T' || map[i][j] == '.' || map[i][j] == 'X') { break; } else { printf("Invalid input! Please enter 'T' for trap, '.' for empty, or 'X' for treasure.\n"); } } } } int treasureRow = -1, treasureCol = -1; for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { if (map[i][j] == 'X') { treasureRow = i; treasureCol = j; break; } } } printf("\nTreasure Map:\n"); for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { printf(" %c ", map[i][j]); } printf("\n"); } if (treasureRow != -1 && treasureCol != -1) { printf("\nTreasure found at: Row %d, Column %d\n", treasureRow + 1, treasureCol + 1); } else { printf("\nNo treasure found on the map!\n"); } return 0; }
Secret Spy Code Decryption
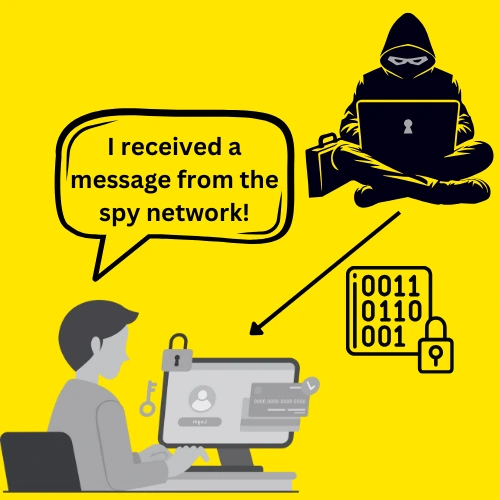
Imagine you are working as a cybersecurity expert for a secret intelligence agency. Your team has intercepted a coded message from an enemy spy network. The message is written in a grid-like format, where each row contains a sequence of letters. However, the enemy has used a mirror encryption technique, flipping the message horizontally to make it unreadable. Your task is to decode the message by reversing the order of characters in each row while keeping the structure intact. Once the message is correctly flipped, it will reveal the original secret communication. Write a C program that processes the encoded message and displays the decrypted version.
Test Case
Input
Enter the number of rows: 3
Enter the number of columns: 5
Enter the encoded message (one row at a time):
o l l e h
d l r o w
e r c e s
Expected Output
Decrypted Message:
h e l l o
w o r l d
s e c r e
- The program starts by asking the user to enter the number of rows and columns for the coded message. These inputs are stored in the variables
rows
andcols
. - Next, the program declares a 2D character array
message[][]
to store the encrypted message. - The user is prompted to input the coded message, character by character.
- Once the message is stored, the program applies horizontal flipping by reversing the order of characters in each row.
- A nested
for
loop is used, where the first column swaps with the last, the second swaps with the second-last, and so on. - This process is repeated for every row to fully decode the message.
- After reversing the message in each row, the decrypted message is displayed.
#include <stdio.h> int main() { int rows, cols; printf("Enter the number of rows: "); scanf("%d", &rows); printf("Enter the number of columns: "); scanf("%d", &cols); char message[rows][cols]; printf("Enter the encoded message (one row at a time):\n"); for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { scanf(" %c", &message[i][j]); } } for (int i = 0; i < rows; i++) { for (int j = 0; j < cols / 2; j++) { char temp = message[i][j]; message[i][j] = message[i][cols - j - 1]; message[i][cols - j - 1] = temp; } } printf("\nDecrypted Message:\n"); for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { printf("%c ", message[i][j]); } printf("\n"); } return 0; }
City Traffic Control System
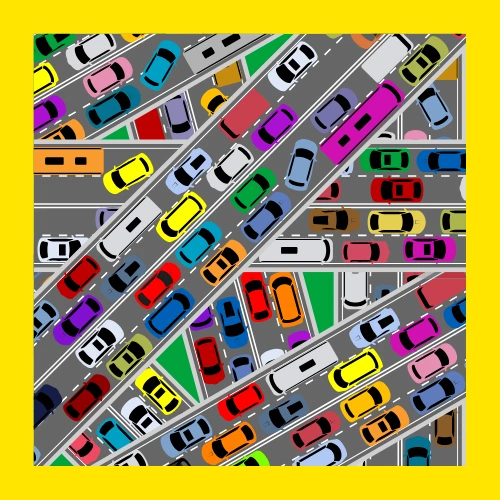
Imagine you are designing an intelligent traffic control system for a smart city. The city has multiple intersections, and each intersection has a set of traffic signals controlling the flow of vehicles. At any given time, vehicles may be waiting at these intersections, and the system needs to optimize traffic light durations based on congestion levels. Each intersection is monitored, and the number of waiting vehicles is recorded in a structured format. Additionally, the duration for which each traffic light stays green is maintained in a separate record. The challenge is to analyze the traffic density at each intersection and adjust the signal durations accordingly. If an intersection has more than 50 vehicles waiting, the system should increase the green light duration by 10 seconds to allow more vehicles to pass through and reduce congestion. If an intersection has fewer than 10 vehicles, the system should decrease the green light duration by 5 seconds, ensuring it does not drop below the minimum limit of 5 seconds. Intersections with moderate traffic between these two limits will remain unchanged. Write a C program that takes as input the number of vehicles waiting at each intersection and the current green light durations, adjusts the signal timings based on traffic density, and then displays the updated signal durations for all intersections.
Test Case
Input
Enter number of intersections (rows): 3
Enter number of roads per intersection (columns): 4
Enter the number of vehicles waiting at each intersection:
12 55 30 8
5 100 45 9
20 7 60 15
Enter the current green signal duration (in seconds) for each intersection:
30 40 25 20
15 50 35 10
20 10 45 30
Expected Output
Updated signal durations (in seconds):
30 50 25 15
10 60 35 5
20 5 55 30
- The program starts by asking the user to enter the number of intersections (rows) and the number of roads per intersection (columns). These values are stored in the variables
rows
andcols
, respectively. - Next, the program reads the traffic congestion data, which is stored in a 2D array called
trafficGrid
. Each value in this grid represents the number of vehicles currently waiting at a particular intersection. - After storing the traffic data, the program reads the signal timing data into another 2D array called
signalGrid
. Each value in this grid represents the current green light duration in seconds for the corresponding intersection. - Once both grids are populated, the program iterates over them using a nested for loop and applies the traffic optimization rules.
- If an intersection has more than 50 vehicles, then the corresponding signal timing in
signalGrid
is increased by 10 seconds. - If an intersection has fewer than 10 vehicles,then the corresponding signal timing in
signalGrid
is reduced by 5 seconds, ensuring that the minimum duration never goes below 5 seconds by usingif
condition. - Other intersections remain unchanged.
- After applying the optimization rules, the program prints the updated signal timings in a grid format using nested
for
loops.
#include <stdio.h> int main() { int rows, cols; printf("Enter number of intersections (rows): "); scanf("%d", &rows); printf("Enter number of roads per intersection (columns): "); scanf("%d", &cols); int trafficGrid[rows][cols], signalGrid[rows][cols]; printf("Enter the number of vehicles waiting at each intersection:\n"); for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { scanf("%d", &trafficGrid[i][j]); } } printf("Enter the current green signal duration (in seconds) for each intersection:\n"); for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { scanf("%d", &signalGrid[i][j]); } } for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { if (trafficGrid[i][j] > 50) { signalGrid[i][j] += 10; } else if (trafficGrid[i][j] < 10) { if (signalGrid[i][j] > 5) { signalGrid[i][j] -= 5; } } } } printf("Updated signal durations (in seconds):\n"); for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { printf("%d ", signalGrid[i][j]); } printf("\n"); } return 0; }
Wizard’s Enchanted Spell Grid
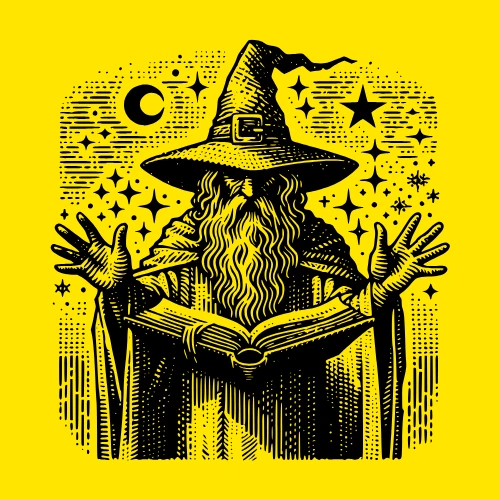
Imagine you are a young apprentice in the mystical land of Eldoria, training under the great wizard Alzareth. Your master has given you a challenging task to test your spellcasting abilities. In the ancient spellbook, spells are stored as magical symbols arranged in a structured pattern inside a sacred spell grid. The potency of each spell is determined by the energy values stored at various locations in the grid. However, over centuries, the magic has become unstable, and some spells have lost their balance, causing dangerous fluctuations in magical energy. To stabilize the magic, you must perform a powerful spell-rebalancing ritual. The spell grid is represented as a square formation of energy values, and a second grid contains magical weights that influence the spells’ stability. Your task is to compute a stability score for each spell by calculating the weighted sum of its surrounding elements. The more balanced a spell, the more stable the energy in the spell grid becomes. Each spell’s new energy value is determined by taking the sum of the products of its surrounding elements and their corresponding weights, ensuring that each spell’s power is correctly adjusted based on its neighbors. The outer edges of the spell grid are special boundary elements and must remain unchanged during the stabilization process. The final grid should reflect the newly stabilized magic values after the transformation. Write a C program that takes as input the initial spell grid and the corresponding weight grid, applies the rebalancing ritual using the weighted sum transformation, and then displays the stabilized spell grid after the ritual is complete.
Test Case
Input
Enter the size of the spell grid (N x N): 3
Enter the initial spell grid values:
2 3 4
1 5 6
7 8 9
Enter the weight grid values:
1 0 -1
2 1 -2
3 0 -3
Expected Output
Stabilized spell grid:
2 3 4
1 -13 6
7 8 9
- The program starts by asking the user to enter the size of the spell grid, which is saved in the
size
variable. - Next, the program prompts the user to enter the initial spell grid values. This input is stored in a 2D array called
spellGrid
. A nested for loop is used to iterate over the grid and take input for each element. - After that, the program asks the user to enter the magical weight grid values, which are stored in another 2D array called
weightGrid
. Again, a nested for loop is used to take input for each element in the weight grid. - Once both grids are populated, the program initializes a new 2D array called
stabilizedGrid
to store the transformed spell values after the spell-rebalancing ritual. - The program then processes the spell grid using a nested for loop.
- If the current element is on the boundary (first row, last row, first column, or last column), then the value remains unchanged and is copied directly to
stabilizedGrid
. - If the element is not on the boundary, its new value is computed using the weighted sum transformation.
- A sum variable is initialized to
0
. - A nested loop iterates through the neighboring elements (above, below, left, right, and diagonal neighbors).
- Each neighboring element is multiplied by its corresponding weight from
weightGrid
, and the product is added tosum
. - After processing all neighboring values, the computed sum is stored in
stabilizedGrid
.
- A sum variable is initialized to
- Once all elements in
stabilizedGrid
are updated, the program prints the final stabilized spell grid, showing the adjusted magical energy values.
#include <stdio.h> int main() { int size; printf("Enter the size of the spell grid (N x N): "); scanf("%d", &size); int spellGrid[size][size], weightGrid[size][size], stabilizedGrid[size][size]; printf("Enter the initial spell grid values:\n"); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { scanf("%d", &spellGrid[i][j]); } } printf("Enter the weight grid values:\n"); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { scanf("%d", &weightGrid[i][j]); } } for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { if (i == 0 || j == 0 || i == size - 1 || j == size - 1) { stabilizedGrid[i][j] = spellGrid[i][j]; } else { int sum = 0; for (int x = -1; x <= 1; x++) { for (int y = -1; y <= 1; y++) { sum += spellGrid[i + x][j + y] * weightGrid[x + 1][y + 1]; } } stabilizedGrid[i][j] = sum; } } } printf("Stabilized spell grid:\n"); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { printf("%d ", stabilizedGrid[i][j]); } printf("\n"); } return 0; }
The Labyrinth of the Ancient Guardians
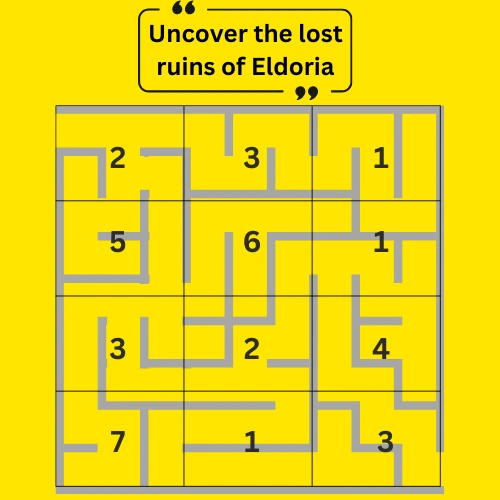
Imagine you are an explorer on a quest to uncover the lost ruins of Eldoria, an ancient civilization hidden deep within a mystical labyrinth. This underground maze is full of winding paths, magical walls, and powerful guardians that protect sacred treasures. The labyrinth is represented as a grid of magical tiles, each with a certain energy level that determines how difficult it is to pass through. Your mission is to find the least energy-consuming path from the entrance (top-left corner) to the treasure chamber (bottom-right corner). You can only move right or down, collecting energy from each tile you step on. Some tiles have traps that drain your energy, while others contain powerful relics that boost your strength. Write a C program that takes a grid of energy values as input and calculates the minimum energy required to reach the treasure. The program should also display the path taken.
Test Case
Input
Enter the number of rows: 4
Enter the number of columns: 4
Enter the energy values of the labyrinth:
2 3 1 4
5 6 1 2
3 2 4 1
7 1 3 2
Expected Output
Minimum energy required to reach the treasure: 12
Path taken (1 indicates the chosen path):
1 1 1 0
0 0 1 1
0 0 0 1
0 0 0 1
- The program starts by asking the user to enter the number of rows and columns for the labyrinth grid. These inputs are saved in the
rows
andcols
variables. - Next, the program prompts the user to enter the energy values for each tile in the labyrinth. These values are stored in a 2D array called
labyrinthGrid
. The input is taken using a nestedfor
loop, iterating over each row and column. - The program then initializes another 2D array called
energyGrid
, which is used to store the minimum energy required to reach each tile. The starting position (top-left corner) of theenergyGrid
is assigned the corresponding value fromlabyrinthGrid
, as this is where the explorer begins the journey. - The program then fills the first row of the
energyGrid
by accumulating energy from the left. Since the explorer can only move right in this row, the energy at each position is calculated as the sum of the energy at the previous position and the energy of the current tile fromlabyrinthGrid
. - Similarly, the program fills the first column by accumulating energy from above. Since the explorer can only move downward in this column, the energy at each position is calculated as the sum of the energy at the position above and the energy of the current tile from
labyrinthGrid
. - After processing the first row and first column, the program proceeds to fill the rest of the
energyGrid
. It iterates through each tile and calculates the minimum energy required to reach that position by choosing the smaller energy value from either the top or the left tile and adding the current tile’s energy value. - Once the entire
energyGrid
is filled, the program retrieves the minimum energy required to reach the treasure chamber, which is stored at the bottom-right corner ofenergyGrid
, and prints this value. - After calculating the minimum energy, the program traces back the optimal path taken to reach the treasure. Starting from the bottom-right corner, it moves backward to determine whether the explorer reached the current position from the left or from above, always choosing the tile with the lower energy cost. Each tile on the optimal path is marked as
1
in a separatepath
array, while the rest remain0
. - Finally, the program prints the path taken, where
1
indicates the chosen path from the entrance to the treasure chamber.
#include <stdio.h> int main() { const int MAX_SIZE = 100; int rows, cols; printf("Enter the number of rows: "); scanf("%d", &rows); printf("Enter the number of columns: "); scanf("%d", &cols); int labyrinthGrid[MAX_SIZE][MAX_SIZE], energyGrid[MAX_SIZE][MAX_SIZE], path[MAX_SIZE][MAX_SIZE]; printf("Enter the energy values of the labyrinth:\n"); for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { scanf("%d", &labyrinthGrid[i][j]); } } energyGrid[0][0] = labyrinthGrid[0][0]; for (int j = 1; j < cols; j++) { energyGrid[0][j] = energyGrid[0][j - 1] + labyrinthGrid[0][j]; } for (int i = 1; i < rows; i++) { energyGrid[i][0] = energyGrid[i - 1][0] + labyrinthGrid[i][0]; } for (int i = 1; i < rows; i++) { for (int j = 1; j < cols; j++) { if (energyGrid[i - 1][j] < energyGrid[i][j - 1]) { energyGrid[i][j] = energyGrid[i - 1][j] + labyrinthGrid[i][j]; } else { energyGrid[i][j] = energyGrid[i][j - 1] + labyrinthGrid[i][j]; } } } printf("Minimum energy required to reach the treasure: %d\n", energyGrid[rows - 1][cols - 1]); int i = rows - 1, j = cols - 1; path[i][j] = 1; while (i > 0 || j > 0) { if (i == 0) { j--; } else if (j == 0) { i--; } else if (energyGrid[i - 1][j] < energyGrid[i][j - 1]) { i--; } else { j--; } path[i][j] = 1; } printf("Path taken (1 indicates the chosen path):\n"); for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { printf("%d ", path[i][j]); } printf("\n"); } return 0; }
The Quantum Circuit Simulator
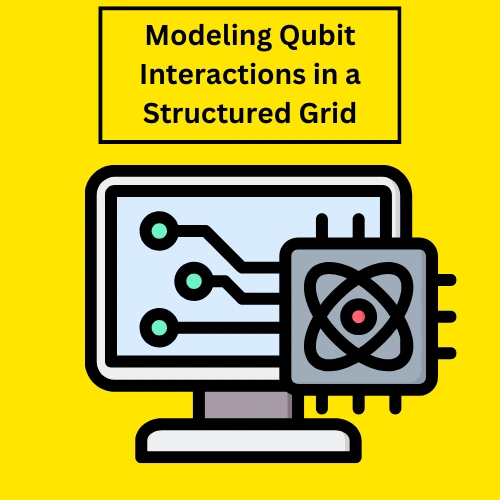
In a high-tech quantum computing lab, scientists are developing a Quantum Circuit Simulator to model how qubits (quantum bits) interact within a structured grid. Unlike regular circuits, quantum circuits rely on entanglement and superposition, making it essential to carefully track the state of each qubit. The circuit is represented as an N × N grid, where each cell holds a number representing a quantum energy level. As qubits interact, they exchange energy with their neighbors in a specific pattern, and to maintain stability, the energy levels must be updated based on a transformation rule. Every qubit inside the grid, except for those on the outer edges, recalculates its energy by taking the average of its four direct neighbors (top, bottom, left, and right), while the boundary qubits remain unchanged as they are linked to external stabilizers. Since all qubits must update their values simultaneously, the new values need to be stored first before modifying the grid, ensuring that previous calculations do not interfere with ongoing updates. The task is to write a C program that takes an integer N as input, reads the initial quantum energy levels, applies the transformation, and then displays the final stabilized quantum circuit grid.
Test Case
Input
Enter the size of the Quantum Circuit Grid (N): 4
Enter the quantum energy levels:
5 5 5 5
5 9 8 5
5 7 6 5
5 5 5 5
Expected Output
Stabilized Quantum Circuit Grid:
5 5 5 5
5 6 6 5
5 6 6 5
5 5 5 5
- The program begins by asking the user to enter the size of the quantum circuit grid (N × N) and stores this value in the variable
N
. Then, it prompts the user to input the quantum energy levels for each qubit in the circuit. These values are stored in a 2D array calledquantumGrid
. - To ensure the energy transformation is applied correctly, the program creates another 2D array called
updatedGrid
, which holds the new stabilized energy values. Since each qubit’s new energy depends on its surrounding qubits, this extra array prevents values from being overwritten before calculations are complete. - The next step is copying the boundary values from
quantumGrid
toupdatedGrid
, as boundary qubits remain unchanged due to their link to external stabilizers. A loop scans the outermost rows and columns and assigns these values directly without modification. - The program then updates the inner cells (excluding the boundaries). It goes through each inner qubit and calculates its new energy level by taking the average of its four direct neighbors (top, bottom, left, and right). These new values are stored in
updatedGrid
. - Once all updates are complete, the program copies the stabilized values from updatedGrid back into quantumGrid so the transformation is fully applied.
- Finally, it prints the updated quantum circuit grid using a nested loop, displaying the final energy levels after stabilization.
#include <stdio.h> int main() { int N; printf("Enter the size of the Quantum Circuit Grid (N): "); scanf("%d", &N); int quantumGrid[N][N], updatedGrid[N][N]; printf("Enter the quantum energy levels:\n"); for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { scanf("%d", &quantumGrid[i][j]); } } for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { if (i == 0 || j == 0 || i == N - 1 || j == N - 1) { updatedGrid[i][j] = quantumGrid[i][j]; } } } for (int i = 1; i < N - 1; i++) { for (int j = 1; j < N - 1; j++) { updatedGrid[i][j] = (quantumGrid[i - 1][j] + quantumGrid[i + 1][j] + quantumGrid[i][j - 1] + quantumGrid[i][j + 1]) / 4; } } for (int i = 1; i < N - 1; i++) { for (int j = 1; j < N - 1; j++) { quantumGrid[i][j] = updatedGrid[i][j]; } } printf("Stabilized Quantum Circuit Grid:\n"); for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { printf("%d ", quantumGrid[i][j]); } printf("\n"); } return 0; }
Smart Warehouse Inventory Management System
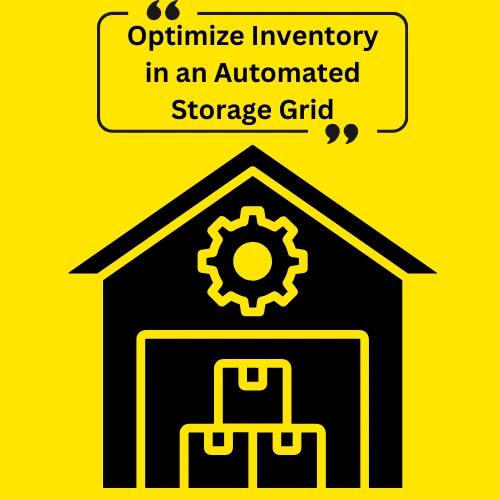
You are the chief inventory manager of a large automated warehouse, responsible for tracking and optimizing inventory levels across multiple storage zones. The warehouse is designed as an N × N grid, where each cell represents a storage unit containing a specific number of items. To help warehouse staff manage inventory efficiently, you need to create a menu-driven program that allows them to perform several key operations. The program should display the current inventory grid, showing the number of items in each storage unit. It should also allow staff to update inventory levels by modifying the item count at a specific storage unit when needed. Additionally, the program should identify which storage unit contains the highest number of items and display its location. To better understand the overall stock, it should calculate and display the average inventory across all storage units. For better organization, the program must also include a feature to redistribute inventory for balance. This means adjusting the inventory levels of inner storage units, excluding the boundary units, by averaging the number of items from their neighboring storage units. This ensures a more even distribution of stock across the warehouse. Finally, the program should provide an option to exit when no further actions are required. Your task is to write a C program that implements this Smart Warehouse Inventory Management System, making it easier for warehouse staff to monitor and manage inventory efficiently.
Test Case
Input
Enter the size of the warehouse grid (N): 4
Enter the number of items in each storage unit:
50 40 30 20
60 80 90 10
70 85 75 15
55 45 35 25
Smart Warehouse Inventory Management System
1- View Current Inventory Grid
2- Update Inventory Data
3- Find Storage Unit with Maximum Items
4- Find Average Inventory Across the Warehouse
5-Redistribute Inventory for Balance
6- Exit
Enter your choice: 1
Enter your choice: 2
Enter row index (0 to 3): 0
Enter column index (0 to 3): 1
Enter new inventory level: 15
Enter your choice: 3
Enter your choice: 4
Enter your choice: 5
Enter your choice: 6
Expected Output
Current Inventory Grid:
50 40 30 20
60 80 90 10
70 85 75 15
55 45 35 25
Most stocked storage unit is at (1, 2) with 90 items.
Average inventory: 47.50 items per unit
Inventory redistributed successfully.
Current Inventory Grid:
50 15 30 20
60 62 48 10
70 67 56 15
55 45 35 25
Exiting Warehouse Management System.
- The program starts by asking the user to enter the size of the warehouse grid (N × N). This input is stored in the variable
N
. - Next, the program prompts the user to enter the initial inventory levels for each storage unit in the warehouse. These values are stored in a 2D array called
warehouseGrid
. - A nested for loop is used to iterate over each row and column, taking user input to initialize the warehouse inventory.
- After initializing the grid, the program enters a menu-driven loop that allows the user to select different operations until they choose to exit.
- If the user selects option 1, the program prints the current state of the warehouse inventory.
- A nested for loop iterates through
warehouseGrid
and prints each value, displaying the inventory grid in a structured format.
- A nested for loop iterates through
- If the user selects option 2, the program allows them to modify the inventory at a specific storage unit.
- The program prompts the user to enter the row index and column index of the storage unit they want to update.
- The user then inputs the new inventory level, which is assigned to the corresponding position in
warehouseGrid
.
- If the user selects option 3, the program determines which storage unit has the highest inventory count.
- A variable
maxLevel
is initialized with the first element ofwarehouseGrid
, and variablesmaxRow
andmaxCol
are set to track its position. - A nested for loop iterates through the grid, updating
maxLevel
,maxRow
, andmaxCol
whenever a higher inventory value is found. - After scanning the entire grid, the program prints the location and inventory level of the most stocked storage unit.
- A variable
- If the user selects option 4, the program calculates the average number of items stored per unit.
- A variable
sum
is initialized to store the total number of items. - A nested for loop iterates through
warehouseGrid
, adding each element’s value tosum
. - The program then calculates the average inventory by dividing
sum
by the total number of storage units(N × N)
. - The result is displayed with two decimal places.
- A variable
- If the user selects option 5, the program balances the stock by redistributing the stock more evenly among inner storage units.
- A temporary 2D array called
optimizedGrid
is created to store the new inventory levels after redistribution. - The boundary storage units are copied directly into
optimizedGrid
since they remain unchanged. - A nested for loop iterates over the inner storage units (excluding boundary units), and each storage unit is updated based on the average inventory of its four direct neighbors (top, bottom, left, and right).
- The computed values are stored in
optimizedGrid
to prevent overwriting the original values before all updates are applied. - Once the calculations are complete, another nested loop copies the values from
optimizedGrid
back intowarehouseGrid
. - The program then prints a message indicating that inventory has been successfully redistributed.
- A temporary 2D array called
- If the user selects option 6, the program prints a termination message and exits the loop.
#include <stdio.h> int main() { int N, choice; printf("Enter the size of the warehouse grid (N): "); scanf("%d", &N); int warehouseGrid[N][N]; printf("Enter the number of items in each storage unit:\n"); for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { scanf("%d", &warehouseGrid[i][j]); } } while (1) { printf("\nSmart Warehouse Inventory Management System\n"); printf("1. View Current Inventory Grid\n"); printf("2. Update Inventory Data\n"); printf("3. Find Storage Unit with Maximum Items\n"); printf("4. Find Average Inventory Across the Warehouse\n"); printf("5. Redistribute Inventory for Balance\n"); printf("6. Exit\n"); printf("Enter your choice: "); scanf("%d", &choice); if (choice == 1) { printf("Current Inventory Grid:\n"); for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { printf("%d ", warehouseGrid[i][j]); } printf("\n"); } } else if (choice == 2) { int row, col, newLevel; printf("Enter row index (0 to %d): ", N - 1); scanf("%d", &row); printf("Enter column index (0 to %d): ", N - 1); scanf("%d", &col); printf("Enter new inventory level: "); scanf("%d", &newLevel); warehouseGrid[row][col] = newLevel; } else if (choice == 3) { int maxLevel = warehouseGrid[0][0]; int maxRow = 0, maxCol = 0; for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { if (warehouseGrid[i][j] > maxLevel) { maxLevel = warehouseGrid[i][j]; maxRow = i; maxCol = j; } } } printf("Most stocked storage unit is at (%d, %d) with %d items.\n", maxRow, maxCol, maxLevel); } else if (choice == 4) { int sum = 0; for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { sum += warehouseGrid[i][j]; } } printf("Average inventory: %.2f items per unit\n", (float)sum / (N * N)); } else if (choice == 5) { int optimizedGrid[N][N]; for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { optimizedGrid[i][j] = warehouseGrid[i][j]; } } for (int i = 1; i < N - 1; i++) { for (int j = 1; j < N - 1; j++) { optimizedGrid[i][j] = (warehouseGrid[i - 1][j] + warehouseGrid[i + 1][j] + warehouseGrid[i][j - 1] + warehouseGrid[i][j + 1]) / 4; } } for (int i = 1; i < N - 1; i++) { for (int j = 1; j < N - 1; j++) { warehouseGrid[i][j] = optimizedGrid[i][j]; } } printf("Inventory redistributed successfully.\n"); } else if (choice == 6) { printf("Exiting Warehouse Management System.\n"); break; } else { printf("Invalid choice! Please enter a number from 1 to 6.\n"); } } return 0; }