Learn how to solve tough real-world problems using C programming. This article includes detailed examples and code for challenging scenarios, like analyzing cricket matches or managing spacecraft safety. Step-by-step explanations make these complex tasks easy to understand for beginners as well as advanced coders.
Exam Result
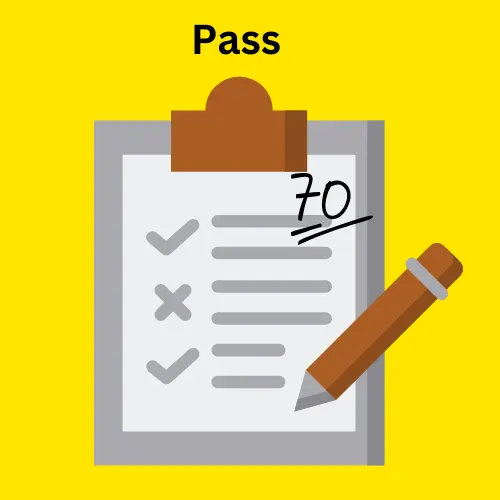
Imagine you’re developing a program for an online education platform. The platform needs to check if a student has passed an exam based on their score. A score of 40 or higher is considered a passing grade. Write a C program that takes the student’s exam score as input and outputs whether they have passed or failed.
Test Case
Input
Enter your exam score: 42
Expected Output
Pass
- The program starts by asking the user to input their exam score. The input is stored in the
score
variable. - Using an
if
statement, the program checks whether the score is greater than or equal to 40. - If the condition is false, the
else
block executes, and the program prints “Fail
” usingprintf
. - If the condition is true, the program prints “
Pass
“.
#include <stdio.h> int main() { int score; printf("Enter your exam score: "); scanf("%d", &score); if (score >= 40) { printf("Pass\n"); } else { printf("Fail\n"); } return 0; }
Eligibility for Shop Discounts
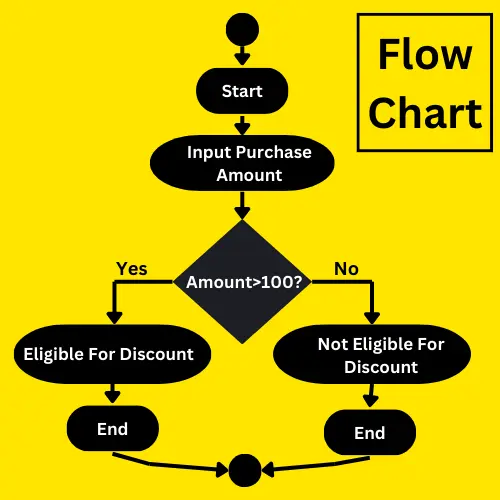
Imagine you’re a developer for an e-commerce platform. The platform provides a 10% discount on purchases over $100. Write a program in C language that takes the total purchase amount as input and tells the user whether they are eligible for the discount or not.
Test Case
Input
Enter your total purchase amount: 120
Expected Output
You are eligible for a 10% discount!
- The program starts by prompting the user to input the total purchase amount, which is stored in the
purchase
variable. - An
if
statement checks if the entered purchase amount is greater than 100. - If the condition is true, the program prints “
You are eligible for a 10% discount!
“. The reason for using10%%
in the code is to correctly display the percentage symbol (%
) in the output. In C language,%%
is used to escape the percent symbol when printing something withprintf
statement. Without this, the compiler would misinterpret%
as a placeholder for a variable. - If the condition is false, the program executes the
else
block and prints “No discount available.
“.
#include <stdio.h> int main() { float purchase; printf("Enter your total purchase amount: "); scanf("%f", &purchase); if (purchase > 100) { printf("You are eligible for a 10%% discount!\n"); } else { printf("No discount available.\n"); } return 0; }
Train Ticket Discount Check
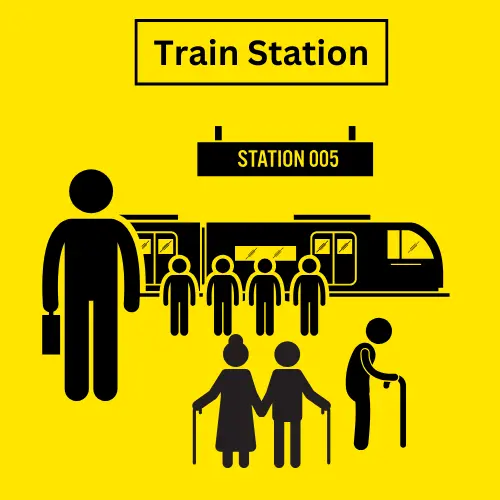
Imagine you’re building a ticket booking system for a train service. The system needs to check if a passenger qualifies for a discounted ticket. Passengers aged 60 or above are eligible for the discount. Write a C program that takes the passenger’s age as input and displays ‘Eligible for discount’ if they qualify; otherwise, display ‘Not eligible for discount.
Test Case
Input
Enter your age: 59
Expected Output
Not eligible for discount
- The program starts by prompting the user to input their age, which is stored in the
age
variable. - Using an
if
statement, the program checks if the age is 60 or above. - If the condition is true, it prints “
Eligible for discount.
“ - If the condition is false, the program executes the
else
block, printing “Not eligible for discount.
“
#include <stdio.h> int main() { int age; printf("Enter your age: "); scanf("%d", &age); if (age >= 60) { printf("Eligible for discount"); } else { printf("Not eligible for discount"); } return 0; }
Even or Odd Number Detector
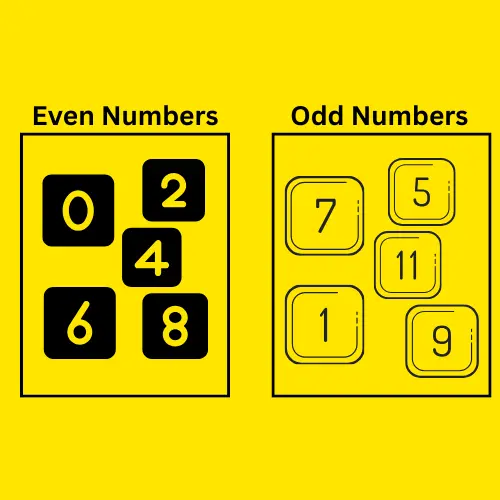
Imagine that you are designing a utility app for basic number analysis. One of its features is to identify if a given number is even or odd. Write a C program that takes a number as input and outputs whether it is even or odd.
Test Case
Input
Enter a number: 23
Expected Output
The number is Odd
- The program starts by asking the user to enter a number, which is stored in the variable
number
. - It then checks if the number is even using an
if
condition. The%
operator is used to find the remainder when the number is divided by 2. If the remainder is zero, then it means that the number is even. - If the number is not even, the
else
block runs and displays a message saying the number is odd.
#include <stdio.h> int main() { int number; printf("Enter a number: "); scanf("%d", &number); if (number % 2 == 0) { printf("The number is Even\n"); } else { printf("The number is Odd\n"); } return 0; }
Age Group Classifier
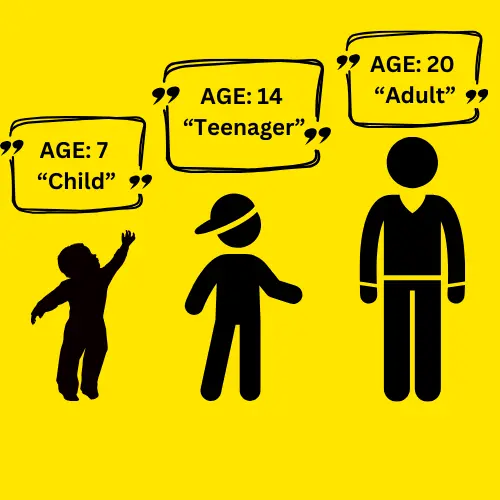
You’re working on a new feature for a health app that sorts users into different age groups. The app should classify users as a child if they are under 12, a teenager if they are between 12 and 17, and an adult if they are 18 or older. Write a C program to handle this classification based on the user’s age.
Test Case
Input
Enter your age: 15
Expected Output
You are a Teenager
- The program begins by asking the user to enter their age, which is stored in the
age
variable. - It then checks the value of
age
to find out the user’s age category. - First, it checks if the age is less than 12 using the condition
(age < 12)
. If this condition is true, the program displays “You are a Child
” using aprintf
. - If the first condition is false, it moves to the next condition
(age <= 17)
. If this condition is true, the program displays “You are a Teenager
“. - If neither of the above conditions is true, it means the user is 18 or older. In this case, the
else
block is executed and the program displays “You are an Adult
“.
#include <stdio.h> int main() { int age; printf("Enter your age: "); scanf("%d", &age); if (age < 12) { printf("Your are a Child\n"); } else if (age <= 17) { printf("You are a Teenager\n"); } else { printf("Your are an Adult\n"); } return 0; }
Speeding Ticket Calculator
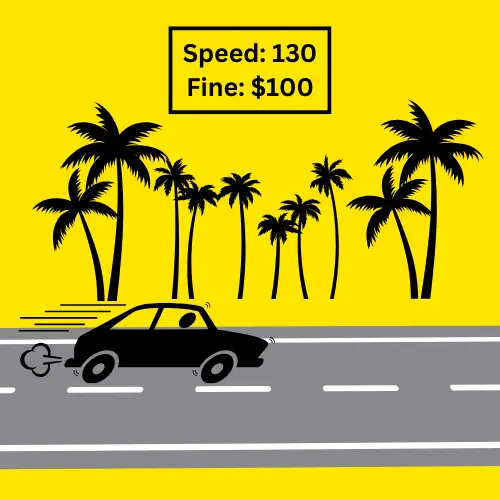
Imagine you are designing a program for traffic police to calculate fines for speeding. The speed limit on a highway is 100 km/h. If a vehicle’s speed is greater than the limit but less than or equal to 120 km/h, the fine is $50. If the speed exceeds 120 km/h, the fine is $100. Write a C program that takes the speed of the vehicle as input and calculates the fine accordingly. If the speed is within the limit, then the program should display “No fine.”
Test Case
Input
Enter the speed of the vehicle (km/h): 112
Expected Output
Fine: $50.
- The program begins by asking the user to input the vehicle’s speed, which is stored in the
speed
variable. - An
if
statement checks whether the speed is within the limit (less than or equal to 100). If this condition is true, the program prints"No fine."
- If the
if
condition is not met, anelse if
statement evaluates whether the speed is greater than 100 but less than or equal to 120. If this condition is satisfied, the program prints"Fine: $50."
- If neither of the previous conditions is true, the
else
block is executed, indicating that the speed exceeds 120. The program then prints"Fine: $100."
#include <stdio.h> int main() { int speed; printf("Enter the speed of the vehicle (km/h): "); scanf("%d", &speed); if (speed <= 100) { printf("No fine.\n"); } else if (speed <= 120) { printf("Fine: $50.\n"); } else { printf("Fine: $100.\n"); } return 0; }
Mountain Adventure Health Check
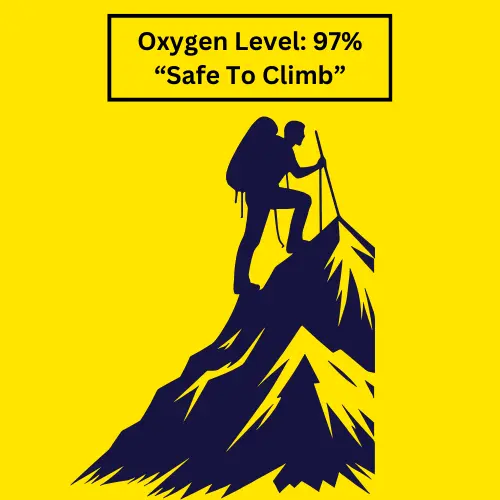
Imagine you are leading a mountain climbing adventure. Before allowing participants to join, you need to ensure their oxygen levels are safe. Participants with oxygen levels of 95% or above are considered “Safe to climb.” Those with levels between 85% and 94% receive a warning to “Consult a doctor before climbing,” while levels below 85% are categorized as “Not safe to climb.” Write a C program that takes a participant’s oxygen level as input and prints the corresponding safety advice.
Test Case
Input
Enter your oxygen saturation level percentage: 88
Expected Output
Caution: Consult a doctor before climbing.
- The program begins by prompting the user to input their oxygen saturation level, which is stored in the
oxygen
variable. - The program uses an if-else structure to evaluate the oxygen level and display the appropriate message:
- An
if
statement checks if the oxygen level is 95% or higher. If this condition is true, the program prints “Safe to climb
“. - An
else if
is used to check if the oxygen level is between 85% and 94%, if the if condition fails. If this condition is true, the program prints “Caution: Consult a doctor before climbing
“. - When the
if
andelse if
conditions fails, theelse
block is executed. This means that the oxygen level is below 85%.. The program prints “Not safe to climb
” usingprintf
statement.
#include <stdio.h> int main() { int oxygen; printf("Enter your oxygen saturation level percentage: "); scanf("%d", &oxygen); if (oxygen >= 95) { printf("Safe to climb.\n"); } else if (oxygen >= 85) { printf("Caution: Consult a doctor before climbing.\n"); } else { printf("Not safe to climb.\n"); } return 0; }
Treasure Hunt Score Checker
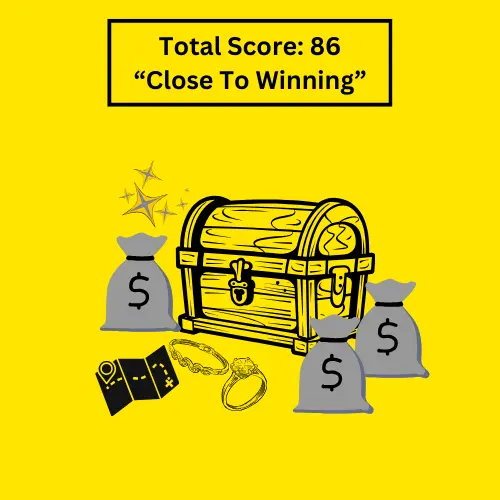
Imagine you are making a treasure hunt game. In this game, players collect points from treasures and bonuses. A player’s total points is equal to the sum of their treasure points and bonus points. If their total score is 100 or more, they win the game. If the score is between 50 and 99, they are ‘Close to winning.’ If the score is less than 50, they ‘Lose the game.’ Write a program in C language that takes the treasure points and bonus points as input and shows the result.
Test Case
Input
Enter your treasure points: 40
Enter your bonus points: 30
Expected Output
Close to winning.
- The program starts by asking the user to input their treasure points and bonus points. These values are stored in the
treasure
andbonus
variables respectively. - The total score is calculated using the formula
total = treasure + bonus
. This operation will add the treasure points and bonus points to calculate the player’s total score. - First, the program uses an
if
condition to check if the total score is 100 or more. If this condition is true, it prints “You win the game!
” usingprintf
statement. - If the
if
condition fails, the program moves to theelse if
condition to check if the total score is between 50 and 99. If this condition is true, it prints “Close to winning
“. - Finally, if both the if and else if conditions fail, then the
else
block is executed, printing “You lose the game
“.
#include <stdio.h> int main() { int treasure; int bonus; int total; printf("Enter your treasure points: "); scanf("%d", &treasure); printf("Enter your bonus points: "); scanf("%d", &bonus); total = treasure + bonus; if (total >= 100) { printf("You win the game!\n"); } else if (total >= 50) { printf("Close to winning.\n"); } else { printf("You lose the game.\n"); } return 0; }
Challenge Timer
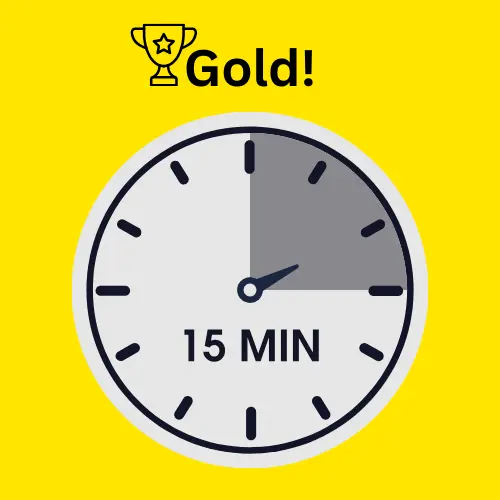
Imagine you are designing a timer for an online challenge game where players are ranked based on how fast they complete the challenge. If a player finishes in 30 seconds or less, they achieve ‘Gold’ status. If they complete it in 31 to 60 seconds, they achieve ‘Silver’ status. If they take 61 to 120 seconds, they get ‘Bronze’ status. However, if their time exceeds 120 seconds, they fail the challenge. Write a C program that takes the completion time in seconds as input and shows the player’s result.
Test Case
Input
Enter the challenge completion time (in seconds): 29
Expected Output
Gold status
- The program starts by asking the user to input the challenge completion time, which is stored in the
time
variable. - First, the program checks if the time is 30 seconds or less using
if
condition. If true, it prints “Gold status.
“ - If the first condition fails, the program checks if the time is between 31 and 60 seconds using
else if
condition. If true, it prints “Silver status.
“ - If the second condition fails, it checks if the time is between 61 and 120 seconds using another
else if
condition. If true, it prints “Bronze status.
“ - Finally, if none of the above conditions are met, the program executes the
else
block and prints “Failed the challenge
.”
#include <stdio.h> int main() { int time; printf("Enter the challenge completion time (in seconds): "); scanf("%d", &time); if (time <= 30) { printf("Gold status\n"); } else if (time <= 60) { printf("Silver status\n"); } else if (time <= 120) { printf("Bronze status\n"); } else { printf("Failed the challenge\n"); } return 0; }
Resource Management in a Video Game
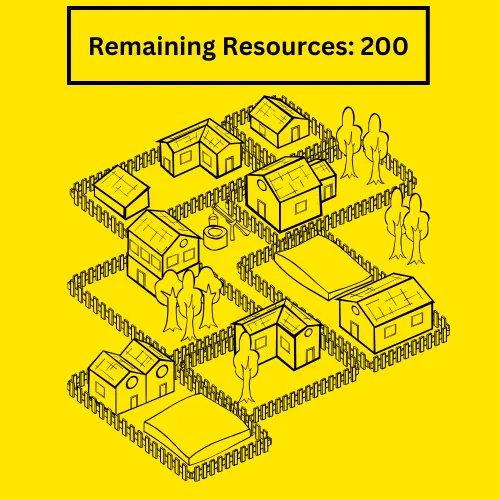
Imagine you are creating a video game where players collect resources to build a village. Each building requires a certain amount of resources, and players need to check if they have enough resources to construct it. The program should first take the total number of resources the player has and then the cost of the building they want to construct. If the player has enough resources, the program should subtract the cost from the total and display the remaining resources. If the player don’t have enough resources, the program should notify the player that they lack the required resources. Write a program in C language to perform this task.
Test Case
Input
Enter your total resources: 500
Enter the building cost: 300
Expected Output
Building constructed. Remaining resources: 200
- The program starts by asking the user to input their total resources and the cost of constructing a building. These values are stored in the
totalResources
andbuildingCost
variables respectively. - The program uses an
if
condition to check iftotalResources
is greater than or equal tobuildingCost
. - If the condition is true, the program subtracts
buildingCost
fromtotalResources
to calculate the remaining resources and prints the updated amount. - If the condition is false, the
else
block is executed and the program informs the player that they do not have enough resources to construct the building.
#include <stdio.h> int main() { int totalResources; int buildingCost; printf("Enter your total resources: "); scanf("%d", &totalResources); printf("Enter the building cost: "); scanf("%d", &buildingCost); if (totalResources >= buildingCost) { totalResources = totalResources - buildingCost; printf("Building constructed. Remaining resources: %d\n", totalResources); } else { printf("Not enough resources to construct the building.\n"); } return 0; }
Autonomous Drone Delivery System
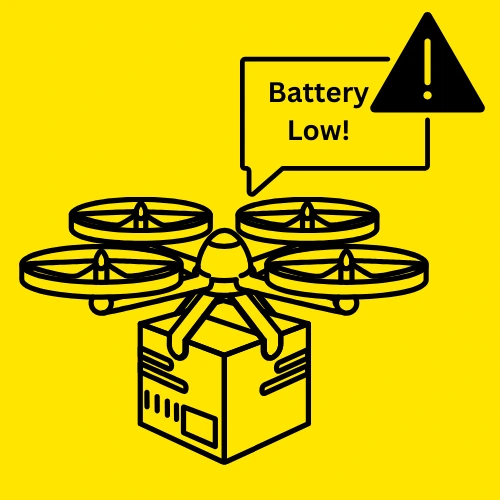
Imagine you are building a program for an autonomous drone delivery system. The drones have a limit on how much weight they can carry and how far they can travel on a full charge. The program should take the total weight of the packages, the drone’s weight capacity, and the distance to the delivery location. If the weight exceeds the drone’s capacity, it should calculate how many trips are needed. It should also check if the drone can complete a round trip on a full charge. If not, the program should figure out how many stops the drone will need for recharging. Write a program in C to handle these tasks.
Test Case
Input
Enter total weight of packages (kg): 120
Enter drone weight capacity (kg): 50
Enter one-way distance to delivery location (km): 20
Enter drone range on full charge (km): 50
Expected Output
Trips required: 3
Stops required for recharging: 0
- The program starts by asking the user to input the total weight of the packages, the drone’s weight capacity, the one-way distance to the delivery location , and the drone’s range on a full charge. These inputs are stored in
totalWeight
,capacity
,distance
andrange
variables respectively. - The number of trips required to transport the packages is initially calculated using
trips = totalWeight / capacity
. This determines how many full trips are needed to carry the total weight. - If the total weight is not perfectly divisible by the drone’s capacity
(totalWeight % capacity != 0)
, then the program increments trips by 1 so that there is an additional trip for the leftover weight. - The program calculates whether the drone needs to recharge for the round trip. The total distance to be covered is
2 * distance
. - If the round-trip distance is less than or equal to the drone’s range (
if (2 * distance <= range)
), then no stops are required, andstops
variable is set to 0. - Otherwise, the number of full recharges required is calculated using
stops = (2 * distance) / range
. This determines how many completerange
cycles the drone needs to complete the round trip. - If there is any remaining distance that doesn’t fit into the drone’s full range (
if (2 * distance) % range != 0)
), the program incrementsstops
by 1. - Finally, The program prints the total number of trips required and the number of stops for recharging using
printf
.
#include <stdio.h> int main() { int totalWeight, capacity, trips, range, distance, stops; printf("Enter total weight of packages (kg): "); scanf("%d", &totalWeight); printf("Enter drone weight capacity (kg): "); scanf("%d", &capacity); printf("Enter one-way distance to delivery location (km): "); scanf("%d", &distance); printf("Enter drone range on full charge (km): "); scanf("%d", &range); trips = totalWeight / capacity; if (totalWeight % capacity != 0) { trips += 1; } if (2 * distance <= range) { stops = 0; } else { stops = (2 * distance) / range; if ((2 * distance) % range != 0) { stops += 1; } } printf("Trips required: %d\n", trips); printf("Stops required for recharging: %d\n", stops); return 0; }
Smart Farming Irrigation System
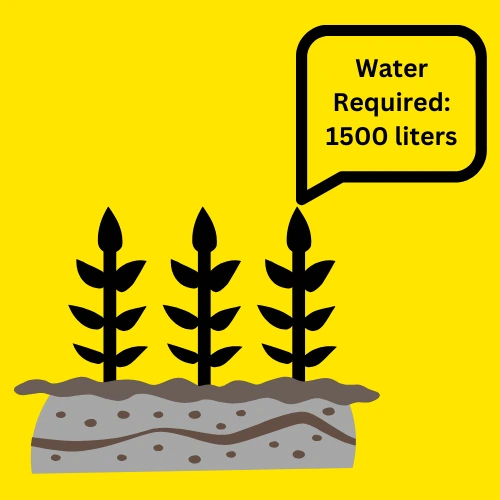
Imagine you are designing an irrigation system for a smart farm. The system calculates how much water is needed based on the type of crop, the size of the field, and the soil’s moisture level. Different crops need different amounts of water. Wheat needs 20 liters per square meter, rice needs 25 liters, and corn needs 15 liters.
If the soil moisture level is more than 70%, the system reduces the water needed by 30%. If the moisture level is less than 30%, it increases the water needed by 20%.
Write a program in C language that calculates the total water required based on these conditions.
Test Case
Input
Enter the type of crop (W for Wheat, R for Rice, C for Corn): R
Enter the size of the field (in sq. meters): 50
Enter the soil moisture level (percentage): 25
Expected Output
Total water required: 1500.00 liters
- The program starts by prompting the user to enter the type of crop they want to irrigate, which is stored in the
cropType
variable. The user can inputW
for Wheat,R
for Rice, orC
for Corn. - Next, the program takes size of the field in square meters and soil moisture level in percentage as input from the user. These values are stored in the
fieldSize
andmoistureLevel
variables respectively. - The program uses the value of
cropType
to determine the amount of water required per square meter, assigning 20 liters for Wheat, 25 liters for Rice, and 15 liters for Corn. If an invalid crop type is entered, the program outputs “Invalid crop type.
” and terminates. - The total water required is calculated by multiplying the
fieldSize
by the water requirement per square meter, and the result is stored in thetotalWater
variable. - If the soil moisture level is above 70%, the program reduces the total water requirement by 30% by multiplying
totalWater
by0.7
. If the soil moisture level is below 30%, it increases the total water requirement by20%
by multiplyingtotalWater
by1.2
. - Finally, the program outputs the total water required in liters, rounded to two decimal places, using the
printf
function.
#include <stdio.h> int main() { char cropType; float fieldSize; float moistureLevel; float waterPerSqMeter; float totalWater; printf("Enter the type of crop (W for Wheat, R for Rice, C for Corn): "); scanf(" %c", &cropType); printf("Enter the size of the field (in sq. meters): "); scanf("%f", &fieldSize); printf("Enter the soil moisture level (percentage): "); scanf("%f", &moistureLevel); if (cropType == 'W') { waterPerSqMeter = 20; } else if (cropType == 'R') { waterPerSqMeter = 25; } else if (cropType == 'C') { waterPerSqMeter = 15; } else { printf("Invalid crop type.\n"); return 1; } totalWater = fieldSize * waterPerSqMeter; if (moistureLevel > 70) { totalWater = totalWater * 0.7; } else if (moistureLevel < 30) { totalWater = totalWater * 1.2; } printf("Total water required: %.2f liters\n", totalWater); return 0; }
Tiered Data Billing System
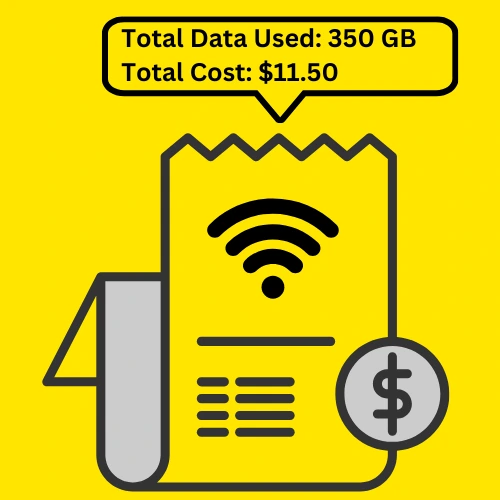
Imagine you’re building a billing system for a cloud data center. The cost of data usage is based on three tiers. For the first 100 GB, the rate is $0.05 per GB. For the next 200 GB (from 101 to 300 GB), the rate is $0.03 per GB. Any usage beyond 300 GB is charged at $0.01 per GB.
Your task is to write a program in C language that takes the total data usage as input and calculates the total cost using this tiered pricing system.
Test Case
Input
Enter the total data usage (GB): 350
Expected Output
Total cost: $11.50
- The program starts by asking the user to input the total data usage in gigabytes (GB). This value is stored in the variable
usage
. - The
cost
variable is initialized to0
to store the calculated total cost. - The program checks how much data has been used and calculates the cost based on the tiered pricing.
- If the usage is 100 GB or less (
usage <= 100
), the cost is calculated asusage * 0.05
, where $0.05 is the price per GB for the first 100 GB. - If the usage is more than 100 GB but less than or equal to 300 GB (
usage <= 300
), then thecost
is calculated by adding two components. the first 100 GB is charged at $0.05 per GB, which is(100 * 0.05)
, and the remaining usage above 100 GB is charged at $0.03 per GB. This is calculated as((usage - 100) * 0.03)
. These two results are then added to get the the totalcost
. - If the usage is more than 300 GB, the cost is calculated by adding three components. The first 100 GB is charged at $0.05 per GB, which is
(100 * 0.05)
. The next 200 GB (from 101 to 300 GB) is charged at $0.03 per GB, calculated as(200 * 0.03)
. The remaining number of GB is charged at $0.01 per GB, calculated as((usage - 300) * 0.01)
. The totalcost
is the sum of these three components. - After calculating the total
cost
, the program displays it to the user usingprintf
and formats it to two decimal places using%.2f
.
#include <stdio.h> int main() { float usage; float cost = 0; printf("Enter the total data usage (GB): "); scanf("%f", &usage); if (usage <= 100) { cost = usage * 0.05; } else if (usage <= 300) { cost = (100 * 0.05) + ((usage - 100) * 0.03); } else { cost = (100 * 0.05) + (200 * 0.03) + ((usage - 300) * 0.01); } printf("Total cost: $%.2f\n", cost); return 0; }
Real-Time Cricket Match Analysis
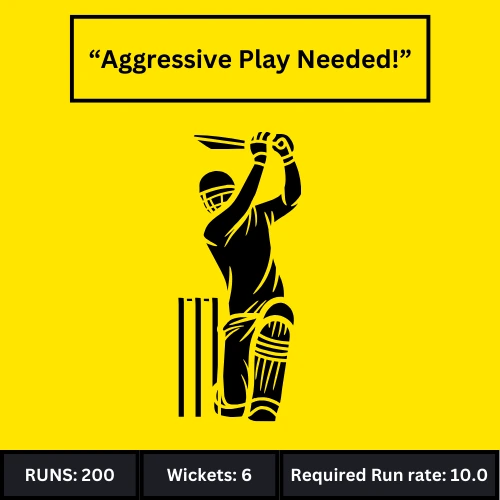
Imagine you’re writing a program to help analyze a cricket match in real time. The program will look at the target score, the current score, the number of overs left, and how many wickets remain to evaluate the situation and give strategic advice to the team. If the required run rate (calculated as remaining runs divided by overs left) is above 8.0, the program will suggest ‘Aggressive Play Needed.’ If the required run rate is between 4.0 and 8.0, it will suggest ‘Maintain Steady Scoring.’ If the required run rate is below 4.0, it will advise ‘Defensive Play Possible.’ If 8 or more wickets have fallen, the program will warn ‘High Risk of Losing.’
Write a C program that takes all these inputs, calculates the required run rate, and then provides an appropriate evaluation of the match situation.
Test Case
Input
Enter the target score: 250
Enter the current score: 200
Enter the overs left: 5
Enter the wickets remaining: 4
Expected Output
Required Run Rate: 10.00
Aggressive Play Needed
- The program asks the user to enter the target score, the current score, the number of overs left, and the wickets remaining. These values are stored in variables called
target
,current
,oversLeft
, andwicketsRemaining
. - Then the program calculates the required run rate using the formula
(target - current) / oversLeft
. This tells how many runs the team needs to score per over to reach the target. The result is saved in the variablerequiredRunRate
and shown to the user with two decimal places usingprintf
function. - Based on the number of wickets left and the required run rate, the program gives advice on how the team should play.
- First, an
if
condition is used to check if thewicketsRemaining
is less than 3. If this condition is true, the program displays “High Risk of Losing
“. - If the first condition is false then, an
else if
condition is used to check if the required run rate is greater than 8.0. If this condition is true, the program suggests “Aggressive Play Needed
” to advise faster scoring. - Another
else if
condition checks if the required run rate is between 4.0 and 8.0. If this is true, the program recommends “Maintain Steady Scoring
“ - If all the previous conditions are false then an
else
condition executes to handle the cases where the required run rate is below 4.0, and in this scenario, the program advises “Defensive Play Possible
“. - Finally he program outputs both the calculated required run rate and the advice for the team based on the situation using
printf
function.
#include <stdio.h> int main() { int target, current, wicketsRemaining; float oversLeft, requiredRunRate; printf("Enter the target score: "); scanf("%d", &target); printf("Enter the current score: "); scanf("%d", ¤t); printf("Enter the overs left: "); scanf("%f", &oversLeft); printf("Enter the wickets remaining: "); scanf("%d", &wicketsRemaining); requiredRunRate = (target - current) / oversLeft; printf("Required Run Rate: %.2f\n", requiredRunRate); if (wicketsRemaining < 3) { printf("High Risk of Losing\n"); } else if (requiredRunRate > 8.0) { printf("Aggressive Play Needed\n"); } else if (requiredRunRate >= 4.0) { printf("Maintain Steady Scoring\n"); } else { printf("Defensive Play Possible\n"); } return 0; }
Spacecraft Mission Critical System
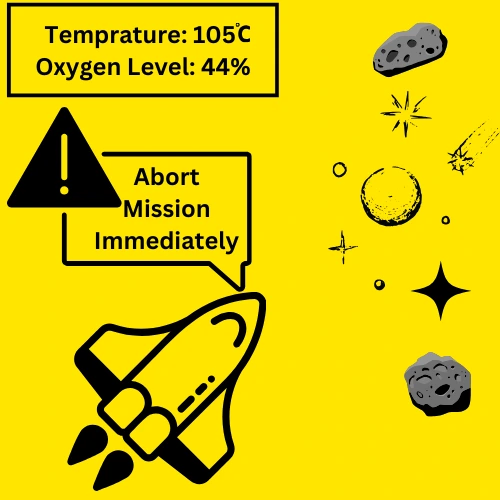
Imagine you are building a decision system for a spacecraft to ensure the mission runs safely and successfully. The system observes key factors like oxygen levels, fuel reserves, and external temperature to decide the next steps.
If oxygen levels drop below 50% and the external temperature goes above safe limits (greater than 100°C), then the system advises “Abort Mission Immediately.” If fuel reserves are less than 25% or oxygen levels are between 50% and 70%, it suggests “Switch to Emergency Mode.” When all conditions are normal, the system advises “Continue Normal Operations.”
Write a program in C language that asks the user to enter the oxygen level, fuel reserve percentage, and external temperature. Based on these inputs the program should give the correct advice for the spacecraft.
Test Case
Input
Enter oxygen level percentage: 45
Enter fuel reserve percentage: 30
Enter external temperature in Celsius: 105
Expected Output
Abort Mission Immediately
- The program starts by asking the user to enter the oxygen level (as a percentage), the fuel reserve (as a percentage), and the external temperature (in Celsius). These inputs are stored in variables called
oxygenLevel
,fuelReserve
, andexternalTemperature
. - The program then uses
if-else
conditions to analyze the situation and provide advice. First, it checks if the oxygen level is below 50% and the external temperature is above 100°C usingif
condition. If both conditions are true, it prints “Abort Mission Immediately
“. - If the first condition isn’t met, it checks if the fuel reserve is below 25% or if the oxygen level is between 50% and 70% using
else if
condition. If either of these conditions is true, it prints “Switch to Emergency Mode
“. - If none of the above conditions are met, it means everything is normal, so the program prints “
Continue Normal Operations
“.
#include <stdio.h> int main() { float oxygenLevel; float fuelReserve; float externalTemperature; printf("Enter oxygen level percentage: "); scanf("%f", &oxygenLevel); printf("Enter fuel reserve percentage: "); scanf("%f", &fuelReserve); printf("Enter external temperature in Celsius: "); scanf("%f", &externalTemperature); if (oxygenLevel < 50 && externalTemperature > 100) { printf("Abort Mission Immediately\n"); } else if (fuelReserve < 25 || (oxygenLevel >= 50 && oxygenLevel <= 70)) { printf("Switch to Emergency Mode\n"); } else { printf("Continue Normal Operations\n"); } return 0; }