Working on problems that use arithmetic operators in Mojo helps you learn how to do simple calculations with different types of data while managing values through variables. These problems include examples like calculating the total cost of groceries, calculating distances, or figuring out profits. Each problem comes with an easy explanation and example code to show how to use variables to store and work with data, making it clear how arithmetic operations are used in everyday situations. This way, you can practice and improve your programming skills.
Calculating a Restaurant Bill
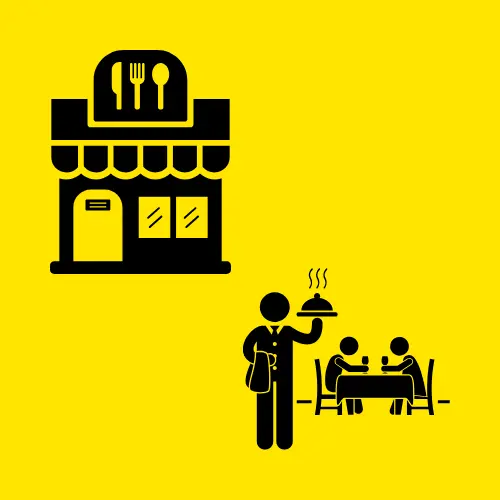
Imagine dining at a restaurant where you ordered a meal costing $25 and a drink for $5. The restaurant also applies a 10% service charge. Write a Mojo program to determine the total amount of your bill.
Test Case
Input:
Please give the cost of the meal you ordered: 25
Please give the cost of the drink you ordered: 5
Enter the service charge percentage: 10
Expected Output:
Final bill is: 33.0
Explanation:
This program calculates the final bill for a restaurant meal. It takes the cost of the meal, the cost of a drink, and a service charge percentage entered by the user.
- The program first asks the user to enter the cost of the
meal
,drink
, andservice_charge_percentage
. - After getting the inputs, the program adds the cost of the
meal
and thedrink
to find the total cost. This total is stored in thetotal
variable. - Next, it calculates the service charge by dividing the percentage by 100 to convert it into a decimal (e.g., 10% becomes 0.1), which is needed to calculate the portion of the total based on the percentage, and then multiply it by the
total
. The result is saved in theservice_charge
variable. - Finally, the program adds
service_charge
to thetotal
cost to find the final bill. This amount is stored in thefinal_bill
variable. The program then displays the final bill to the user.
meal = Float(input("Please give the cost of the meal you ordered: ")) drink = Float(input("Please give the cost of the drink you ordered: ")) service_charge_percent = Float(input("Enter the service charge percentage: ")) total = meal + drink service_charge = (service_charge_percent / 100) * total final_bill = total + service_charge print(f"Final bill is: {final_bill}")
Temperature Conversion
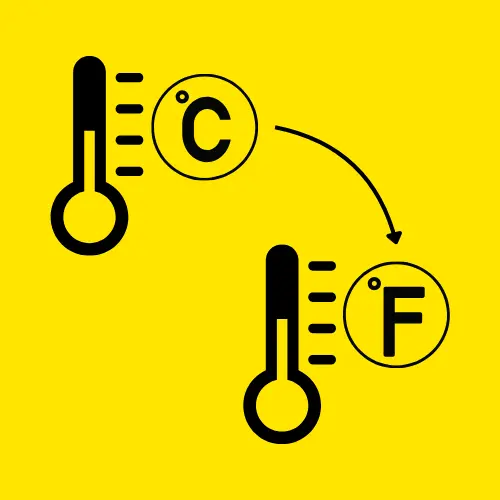
Write a Mojo program that takes a temperature value in Celsius and converts it into Fahrenheit. Use the formula Fahrenheit = (Celsius * 9/5) + 32 to perform the conversion and display the result.
Test Case
Input:
Enter temperature in Celsius: 25
Expected Output:
Temperature in Fahrenheit is: 77.0
Explanation:
This program takes a temperature given in Celsius and calculates its equivalent value in Fahrenheit using a simple formula.
- The program prompts the user to input the temperature in Celsius, which is then saved in the
celsius
variable. - To convert Celsius to Fahrenheit, the program multiplies the Celsius temperature by 9, divides the result by 5, adds 32, and then stores the final value in the
fahrenheit
variable.
celsius = Float(input("Enter temperature in Celsius: ")) fahrenheit = (celsius * 9 / 5) + 32 print(f"Temperature in Fahrenheit is: {fahrenheit}")
Sharing Candies
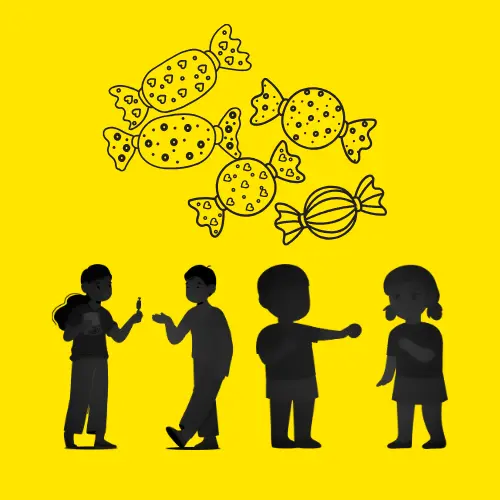
There are 50 candies that need to be shared equally among 5 friends. Write a Mojo program to find out how many candies each friend will get and calculate if there are any leftover candies after dividing them equally.
Test Case
Input:
Kindly enter the total number of candies available: 50
Enter the number of friends: 5
Expected Output:
Each friend gets 10 candies.
Candies left over: 0
Explanation:
This program divides a certain number of candies equally among friends and calculates if there are any leftover candies.
- The program first asks the user to input the total number of candies and the number of friends. The inputs are converted to integers (using
Int()
) and stored in the variablestotal_candies
andfriends
. - Next, the program calculates how many candies each friend should get by dividing
total_candies
byfriends
. The result is stored in thecandies_per_friend
variable. - Then, the program calculates how many candies will be left over after dividing them. It uses the modulo operator (
%
) to find the remainder when dividingtotal_candies
byfriends
. This remainder is stored in theleftover_candies
variable.
total_candies = Int(input("Kindly enter the total number of candies available: ")) friends = Int(input("Enter the number of friends: ")) candies_per_friend = total_candies / friends leftover_candies = total_candies % friends print(f"Each friend gets {candies_per_friend} candies.") print(f"Candies left over: {leftover_candies}")
Distance Calculation
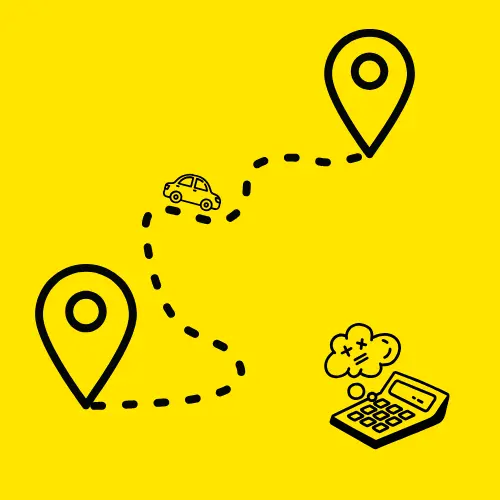
A car covers a distance of 60 miles per hour. Write a Mojo program to calculate how far it will travel in 5 hours.
Test Case
Input:
Input the car’s speed in miles per hour: 60
Enter the time in hours: 5
Expected Output:
Distance is: 300
Explanation:
This program calculates a car’s distance based on its speed and the time it travels.
- The program begins by prompting the user to enter the car’s speed in miles per hour and the time traveled in hours, which are saved in the
speed
andtime
variables, respectively. - After getting the inputs, the program calculates the distance the car has traveled by multiplying the
speed
by thetime
. This result is stored in thedistance
variable.
speed = Int(input("Input the car's speed in miles per hour: ")) time = Int(input("Enter the time in hours: ")) distance = speed * time print(f"Distance is: {distance}")
Perimeter of a Rectangle
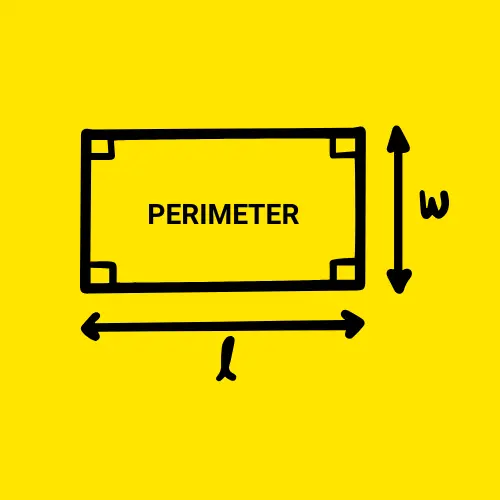
Given the length and width of a rectangle, write a Mojo program to calculate its perimeter
Test Case
Input:
Enter the rectangle’s length: 10
Enter the rectangle’s width: 5
Expected Output:
Perimeter of a rectangle is: 30
Explanation:
The program calculates how to find the perimeter of a rectangle.
- It first asks the user to enter the length and width of the rectangle, which are stored in the
length
andwidth
variables. - To calculate the perimeter of a rectangle, you first add the
length
andwidth
together. Then, you multiply the sum by 2 to get the total perimeter. This final result is stored in theperimeter
variable.
length = Int(input("Enter the rectangle's length: ")) width = Int(input("Enter the rectangle's width: ")) perimeter = 2 * (length + width) print(f"Perimeter of a rectangle is: {perimeter}")
Fuel Cost Calculator
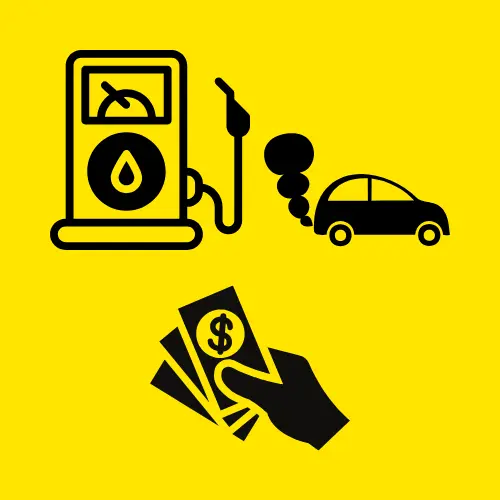
A car consumes 5 liters of fuel to travel 100 kilometers. Calculate the total cost of fuel needed to travel 350 kilometers if fuel costs $2 per liter.
Test Case
Input:
Enter fuel consumption per 100 km: 5
Enter the distance to travel in km: 350
Enter the cost of fuel per liter: 2
Expected Output:
Total cost: 35
Explanation:
This program calculates the total cost of fuel for a trip.
- The program asks the user to input the fuel consumption per 100 km, the distance to travel in kilometers, and the cost of fuel per liter, storing these values in the
fuel_per_100km
,distance
, andfuel_cost
variables, respectively. - To calculate how much fuel is needed for the trip, the program divides the
distance
by 100 and then multiplies it by the fuel consumption per 100 km (fuel_per_100km
). This result is stored in thefuel_needed
variable. - After that, the program calculates the total fuel cost by multiplying the fuel needed (
fuel_needed
) by the cost per liter of fuel (fuel_cost
). The total cost is saved in thetotal_cost
variable.
fuel_per_100km = Float(input("Enter fuel consumption per 100 km: ")) distance = Float(input("Enter the distance to travel in km: ")) fuel_cost = Float(input("Enter the cost of fuel per liter: ")) fuel_needed = (distance / 100) * fuel_per_100km total_cost = fuel_needed * fuel_cost print(f"Total cost: {total_cost}")
Average Score
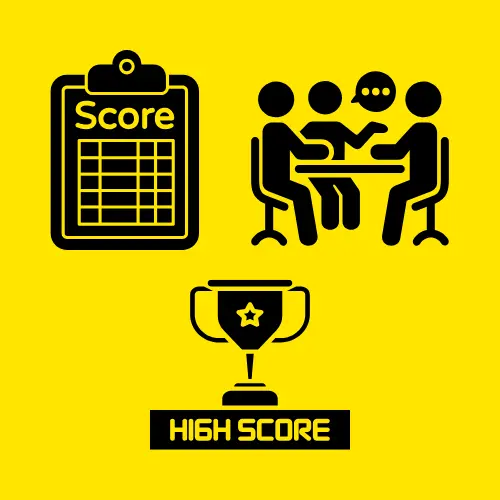
Write a Mojo program to calculate the average score of a student from 5 courses.
Test Case
Input:
Enter the score for course 1: 80
Enter the score for course 2: 75
Enter the score for course 3: 90
Enter the score for course 4: 85
Enter the score for course 5: 70
Expected Output:
Average is: 80.0
Explanation:
This program calculates the average score of five courses.
- It first asks the user to enter the score for each of the five courses, and these values are stored in variables
course_score1
,course_score2
,
,course_score
3
, andcourse_score
4
.course_scor
e5 - Then, the program adds all the scores together to find the
total
. - After that, it divides the
total
by 5 to calculate theaverage
score.
course_score1 = Float(input("Enter the score for course 1: ")) course_score2 = Float(input("Enter the score for course 2: ")) course_score3 = Float(input("Enter the score for course 3: ")) course_score4 = Float(input("Enter the score for course 4: ")) course_score5 = Float(input("Enter the score for course 5: ")) total = course_score1 + course_score2 + course_score3 + course_score4 + course_score5 average = total / 5 print(f"Average is: {average}")
Grocery Budget Check
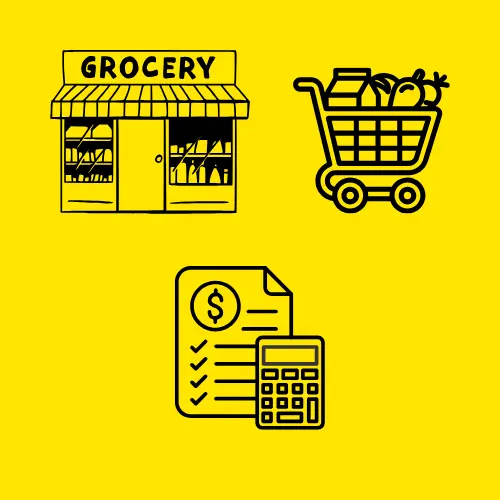
You have $50 to spend. Write a Mojo program to calculate how much money remains after buying 3 products priced at $15, $20, and $10.
Test Case
Input:
Enter your total budget: 50
Enter the price of product 1: 15
Enter the price of product 2: 20
Enter the price of product 3: 10
Expected Output:
Remaining Budget: 5
Explanation:
This program helps you figure out how much money you’ll have left after shopping.
- The program starts by asking you to enter the total amount of money
budget
and the prices of three productsproduct_price1
,product_price2
, andproduct_price3
. - Then program calculates the total cost of these products by adding their prices together and stores the result in the
total_cost
variable. - After that, it calculates the remaining money by subtracting the
total_cost
from yourbudget
and stores this value in theremaining_budget
variable.
budget = Float(input("Enter your total budget: ")) product_price1 = Float(input("Enter the price of product 1: ")) product_price2 = Float(input("Enter the price of product 2: ")) product_price3 = Float(input("Enter the price of product 3: ")) total_cost = product_price1 + product_price2 + product_price3 remaining_budget = budget - total_cost print(f"Remaining Budget: {remaining_budget}")
Splitting the Bill
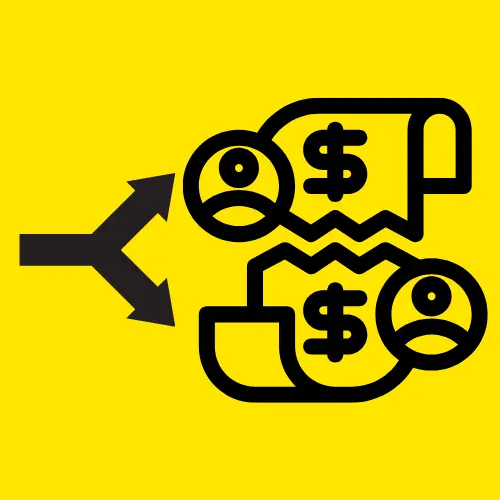
Four friends split a restaurant bill of $120 equally. Each also leaves a 10% tip. Write a Mojo program to calculate the total each friend pays.
Test Case
Input:
Enter the total bill amount: 120
Enter the number of friends: 4
Enter the tip percentage: 10
Expected Output:
Total per person: 33.0
Explanation:
This program helps calculate how much each person needs to pay when splitting a bill, including a tip.
- User start by entering the total bill amount (
bill
), the number of friends (people
), and the tip percentage (tip_percent
). - The program first divides the bill by the number of people (
bill / people
) to find out how much each person has to pay. - The program calculates the tip for each person by converting the tip percentage into a decimal (
tip_percent / 100
) and then multiplying it by the amount each person has to pay (per_person
). This gives thetip
amount for each individual. - Finally, the program adds the calculated tip (
tip
) to the amount each person has to pay (per_person
). This gives the total amount each person needs to pay, including the tip.
bill = Float(input("Enter the total bill amount: ")) people = Int(input("Enter the number of friends: ")) tip_percent = Float(input("Enter the tip percentage: ")) per_person = bill / people tip = (tip_percent / 100) * per_person total_per_person = per_person + tip print(f"Total per person: {total_per_person}")
Profit Calculation
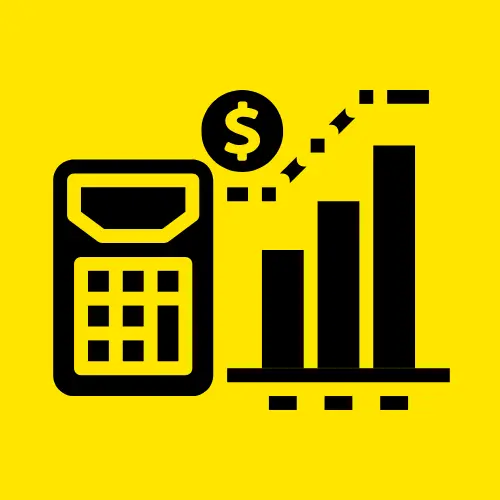
A company produces 1000 units of a product. The cost to produce each unit is $15.50, and the fixed overhead cost for the factory is $5000. The product is sold for $25.00 each. Write a program to calculate the total profit made by the company.
Test Case
Input:
Enter the number of units produced: 1000
Enter the cost to produce each unit: 15.50
Enter the fixed overhead cost: 5000
Enter the selling price per unit: 25.00
Expected Output:
Total profit: 5000.0
Explanation:
This program helps calculate the total profit made from producing and selling units of a product.
- First, it asks the user to enter the number of units produced (
units_produced
), the cost to produce each unit (cost_per_unit_produced
), the fixed overhead cost (fixed_costs
), and the selling price per unit (selling_price_per_unit
). - It then calculates the total cost (
total_cost
) by multiplying the number of units (units_produced
) by the cost per unit (cost_per_unit_produced
) and adding the fixed costs (fixed_costs
). - The total revenue (
total_revenue
) is calculated by multiplying the number of units (units_produced
) by the selling price per unit (selling_price_per_unit
). - Finally, the program finds the
profit
by subtracting the total cost (total_cost
) from the total revenue (total_revenue
), and prints out the total profit.
units_produced = Int(input("Enter the number of units produced: ")) cost_per_unit_produced = Float(input("Enter the cost to produce each unit: ")) fixed_costs = Float(input("Enter the fixed overhead cost: ")) selling_price_per_unit = Float(input("Enter the selling price per unit: ")) total_cost = (units_produced * cost_per_unit_produced) + fixed_costs total_revenue = units_produced * selling_price_per_unit profit = total_revenue - total_cost print(f"Total profit: {profit}")
Annual Salary Calculation
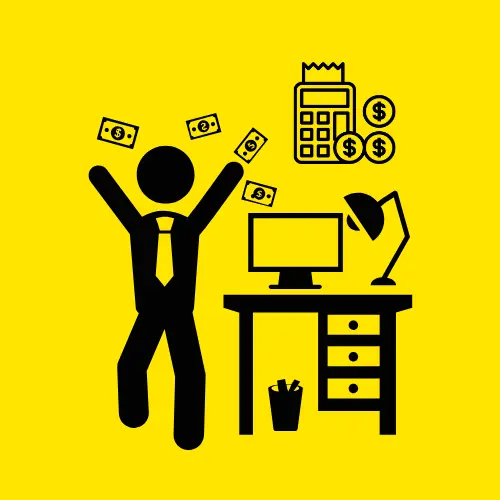
A person earns a monthly salary of $3000. They receive a yearly bonus based on their salary. The bonus is 5% of the total salary for the year, but an additional 2% bonus is given for each year of service. Calculate the total salary including the bonus if they have worked for 3 years.
Test Case
Input:
Enter the monthly salary: 3000
Enter the annual bonus percentage (in %): 5
Enter the additional bonus percentage per year of service (in %): 2
Enter the number of years of service: 3
Expected Output:
Total Salary: 38700.0
Explanation:
This program calculates the total salary including annual bonus and additional bonus based on years of service.
- First, it asks for the monthly salary (
monthly_salary
), the annual bonus percentage (annual_bonus_percentage
), the additional bonus percentage per year of service (yearly_bonus_additional
), and the number of years of service (years_of_service
). - Next, it computes the annual salary (
annual_salary
) by multiplying the monthly salary (monthly_salary
) by 12. Multiplying themonthly_salary
by 12 gives the total salary for the whole year. - The annual bonus is calculated by multiplying the annual salary (
annual_salary
) by the bonus percentage (annual_bonus_percentage
) divided by 100 to convert it to a decimal. This gives the actualbonus
amount. - The
additional_bonus
is calculated by multiplying the annual salary (annual_salary
) by the additional bonus percentage (yearly_bonus_additional
) (converted to decimal form by dividing by 100), and then multiplying by the number of years of service (years_of_service
) to get the total bonus based on experience. - Finally, it adds the
annual_salary
,bonus
, andadditional_bonus
together to determine the total salary (total_salary
).
monthly_salary = Float(input("Enter the monthly salary: ")) annual_bonus_percentage = Float(input("Enter the annual bonus percentage (in %): ")) yearly_bonus_additional = Float(input("Enter the additional bonus percentage per year of service (in %): ")) years_of_service = Int(input("Enter the number of years of service: ")) # Calculate annual salary annual_salary = monthly_salary * 12 # Calculate total bonus bonus = annual_salary * (annual_bonus_percentage / 100) additional_bonus = annual_salary * (yearly_bonus_additional / 100) * years_of_service # Total salary with bonus total_salary = annual_salary + bonus + additional_bonus print(f"Total Salary: {total_salary}")
Profit from Selling Stocks
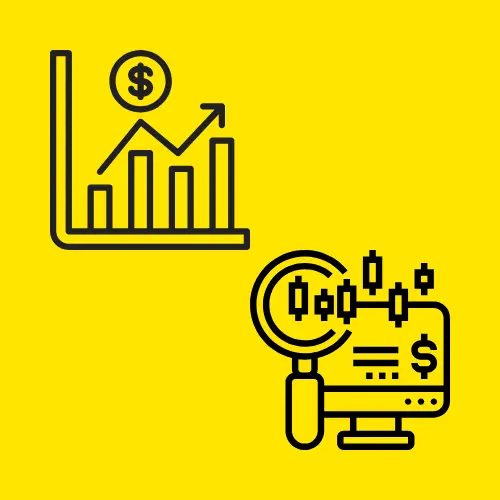
You buy 200 shares of a stock at $40 each. After 6 months, the stock price increases by 12%. You sell the stock. Calculate the profit you make from selling all the shares.
Test Case
Input:
Enter the purchase price per share: 40
Enter the percentage increase in price: 12
Enter the number of shares bought: 200
Expected Output:
Total profit: 960.0
Explanation:
This program calculates the profit made from selling shares after a price increase.
- First, you input the purchase price per share (
purchase_price_per_share
), the percentage increase in price (price_increase_percentage
), and the number of shares bought (number_of_shares_bought
). - The new price per share (
new_price_per_share
) is calculated by multiplying thepurchase_price_per_share
by(1 + price_increase_percentage / 100)
, which adds the price increase to the original price. - Next, the profit per share (
profit_per_share
) is calculated by subtracting the originalpurchase_price_per_share
from thenew_price_per_share
, giving the gain on each share. - Finally, the total profit (
total_profit
) is found by multiplying theprofit_per_share
by the number of shares (number_of_shares_bought
), showing the overall profit from all the shares.
purchase_price_per_share = Float(input("Enter the purchase price per share: ")) price_increase_percentage = Float(input("Enter the percentage increase in price: ")) number_of_shares_bought = Int(input("Enter the number of shares bought: ")) new_price_per_share = purchase_price_per_share * (1 + price_increase_percentage / 100) profit_per_share = new_price_per_share - purchase_price_per_share total_profit = profit_per_share * number_of_shares_bought print(f"Total profit: {total_profit}")
Volume of Liquid in Different Containers
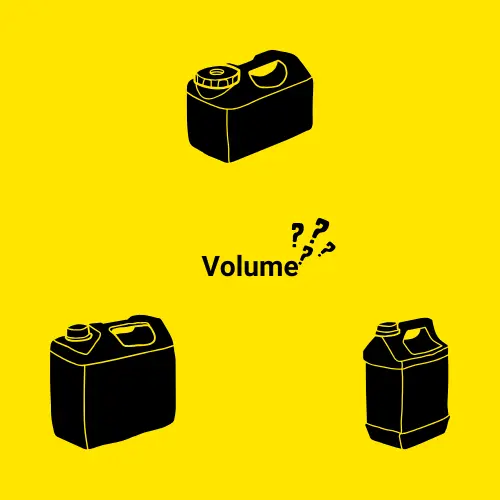
You have 3 cylindrical containers. The first has a radius of 5 cm and height 10 cm, the second has a radius of 7 cm and height 15 cm, and the third has a radius of 6 cm and height 12 cm. Calculate the total volume of liquid these three containers can hold.
Test Case
Input:
Please provide the radius of the first container: 5
Please provide the height of the first container: 10
Please provide the radius of the second container: 7
Please provide the height of the second container: 15
Please provide the radius of the third container: 6
Please provide the height of the third container: 12
Expected Output:
The total volume of all three containers is: 1300.21
Explanation:
This program calculates the total volume of three cylindrical containers by taking user inputs for their radius and height, and then applying the formula for the volume of a cylinder: V = π * r² * h
.
- The program asks the user to input the radius and height of three containers. These values are stored in the variables
radius_1
,height_1
,radius_2
,height_2
,radius_3
, andheight_3
. These inputs are taken as floating point numbers usingFloat(input())
to allow for decimal values, as the measurements could be non-integer. - The volume of each cylinder is calculated using the formula
V = π * r² * h
. In this case, we use an approximate value ofπ
as3.14159
.- For the first container, the volume is calculated as
volume_1 = pi * (radius_1 ** 2) * height_1
, where the radius is squared (radius_1 ** 2
), then multiplied by the height (height_1
), and finally multiplied by π. - Similarly, the volumes for the second and third containers are stored in
volume_2
andvolume_3
, calculated in the same way.
- For the first container, the volume is calculated as
radius_1 = Float(input("Please provide the radius of the first container: ")) height_1 = Float(input("Please provide the height of the first container: ")) radius_2 = Float(input("Please provide the radius of the second container: ")) height_2 = Float(input("Please provide the height of the second container: ")) radius_3 = Float(input("Please provide the radius of the third container: ")) height_3 = Float(input("Please provide the height of the third container: ")) # Volume of cylinder formula: V = π * r^2 * h pi = 3.14159 volume_1 = pi * (radius_1 ** 2) * height_1 volume_2 = pi * (radius_2 ** 2) * height_2 volume_3 = pi * (radius_3 ** 2) * height_3 total_volume = volume_1 + volume_2 + volume_3 print(f"The total volume of all three containers is: {total_volume}")
Hostel Rent Calculation
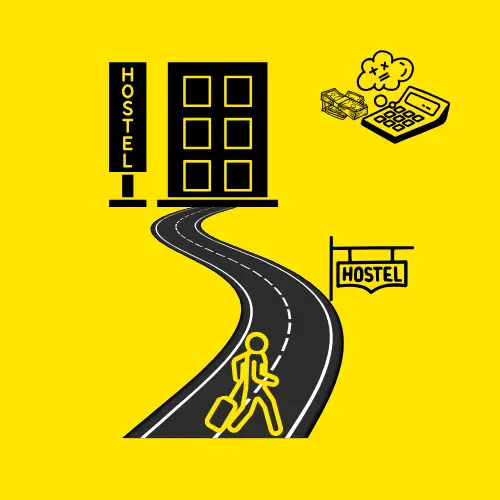
There are 50 hostelites staying in 10 rooms, and they went home for the summer break. Each room has 5 students. The rent for each room is divided in the following way:
The first 3 rooms receive the rent from 4 students who paid full rent, and the 5th student pays half rent.
The next 5 rooms receive rent from 3 students who paid full rent, and the 2 remaining students pay half rent.
The last 2 rooms receive rent from 2 students who paid full rent, and the 3 remaining students pay half rent.
Write a Mojo program to calculate the total rent collected by the hostel, but the rent for each room is taken as input from the user.
Test Case
Input:
Enter the rent for a student paying full rent: 10000
Enter the rent for a student paying half rent: 5000
Expected Output:
Total rent collected by the hostel: 95000
Explanation:
This program calculates the total rent collected by a hostel from multiple rooms, based on the number of students paying full rent and half rent.
- The program asks the user to input the rent values, where
full_rent
stores the rent for a student paying the full amount, andhalf_rent
stores the rent for a student paying half the rent. - Rooms 1 to 3: These rooms have 4 full rent students and 1 half rent student.
- The total rent collected from these rooms is calculated by multiplying
4 * full_rent
to get the rent from full rent students (full_rent_students_room_1_3
) and addinghalf_rent
for the one half rent student (half_rent_students_room_1_3
). The total rent for rooms 1 to 3 is stored intotal_rent_room_1_3
.
- The total rent collected from these rooms is calculated by multiplying
- Rooms 4 to 8: These rooms have 3 full rent students and 2 half rent students.
- Similarly, the rent for these rooms is calculated by multiplying
3 * full_rent
for the full rent students (full_rent_students_room_4_8
) and2 * half_rent
for the half rent students (half_rent_students_room_4_8
). The total rent for rooms 4 to 8 is stored intotal_rent_room_4_8
.
- Similarly, the rent for these rooms is calculated by multiplying
- Rooms 9 to 10: These rooms have 2 full rent students and 3 half rent students.
- Again, the rent for these rooms is calculated by multiplying
2 * full_rent
for the full rent students (full_rent_students_room_9_10
) and3 * half_rent
for the half rent students (half_rent_students_room_9_10
). The total rent for rooms 9 to 10 is stored intotal_rent_room_9_10
.
- Again, the rent for these rooms is calculated by multiplying
- After calculating the rent for each set of rooms, the program adds the rents from all the rooms together. The total rent for all rooms is calculated as:
total_rent = total_rent_room_1_3 * 3 + total_rent_room_4_8 * 5 + total_rent_room_9_10 * 2
. This formula multiplies the rent for each set of rooms by the number of rooms (3 rooms in the first set, 5 rooms in the second set, and 2 rooms in the last set) and adds them to get the total rent.
full_rent = Int(input("Enter the rent for a student paying full rent: ")) half_rent = Int(input("Enter the rent for a student paying half rent: ")) # Calculate rent for the first 3 rooms full_rent_students_room_1_3 = 4 * full_rent half_rent_students_room_1_3 = half_rent total_rent_room_1_3 = full_rent_students_room_1_3 + half_rent_students_room_1_3 # Calculate rent for the next 5 rooms full_rent_students_room_4_8 = 3 * full_rent half_rent_students_room_4_8 = 2 * half_rent total_rent_room_4_8 = full_rent_students_room_4_8 + half_rent_students_room_4_8 # Calculate rent for the last 2 rooms full_rent_students_room_9_10 = 2 * full_rent half_rent_students_room_9_10 = 3 * half_rent total_rent_room_9_10 = full_rent_students_room_9_10 + half_rent_students_room_9_10 # Calculate total rent total_rent = total_rent_room_1_3 * 3 + total_rent_room_4_8 * 5 + total_rent_room_9_10 * 2 print("Total rent collected by the hostel: ", total_rent)
Sales Target Calculation
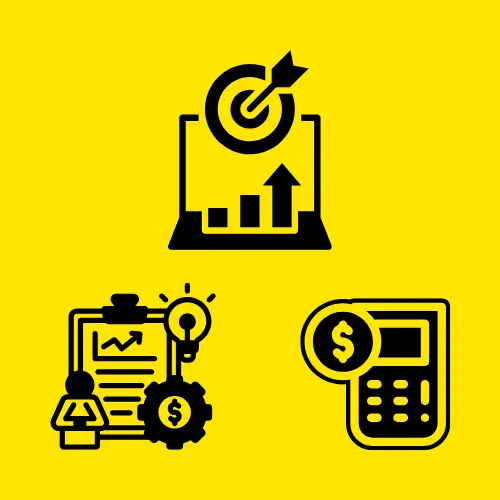
A store is selling a product for $120 each. The store has a sales target of $36,000 for the month. However, the store has a discount offer where for every 4 products sold, the 5th product is given for free.
You need to calculate how many products need to be sold in order to meet the sales target.
Given:
The price of one product is $120.
The store wants to earn $36,000 in total sales.
For every 5 products sold, the store earns money for only 4 products because 1 is free.
Calculate the minimum number of products that must be sold to meet the sales target.
Test Case
Input:
Enter the price of each product: 120
Enter the sales target: 36000
Expected Output:
Total products to be sold: 150
Explanation:
- The program begins by asking the user to enter the price of each product and the sales target. The price per product is stored in the variable
price_per_product
, and the sales target is stored in the variablesales_target
. - Then, the program calculates the total value of 4 products (since the 5th one is free). This is done by multiplying the
price_per_product
by 4, and the result is saved in the variablevalue_per_5_products
. - Next, the program calculates how many sets of 5 products are needed to reach the sales target. To do this, the program divides the
sales_target
by thevalue_per_5_products
(the total value of 4 products) and stores the result in the variableproducts_needed
. This gives the number of product sets required to meet or exceed the sales target. - Finally, the program calculates the total number of products that must be sold, including the free ones. Since each set contains 5 products (4 paid and 1 free), the program multiplies the number of sets (
products_needed
) by 5. The result is saved in the variabletotal_products_sold
.
price_per_product = Int(input("Enter the price of each product: ")) sales_target = Int(input("Enter the sales target: ")) # Calculate the total value of 4 products (since the 5th one is free) value_per_5_products = 4 * price_per_product # Calculate how many sets of 5 products are needed to meet the sales target products_needed = sales_target / value_per_5_products # Calculate the total number of products (including free ones) total_products_sold = products_needed * 5 print("Total products to be sold: ", total_products_sold)
Using arithmetic operators in coding problems that relate to real-life situations in Mojo is a great way to improve your programming skills. By solving these problems, you’ll get better at doing different types of calculations, making your code more useful and efficient. Keep practicing and trying new challenges to get better at solving real-world problems.