Scientific notation is often used in mathematics and science to handle very large or very small numbers. It also helps when precision is key, such as in astronomy, physics, and engineering. Learn how Python provides an easy way to handle these numbers as variables or constants.
What Is Scientific Notation? A Real-world Analogy
Scientific notation is a way to write large or small numbers more simply. It shows numbers as a product of a number between 1 and 10 and a power of 10. Think of it like a shortcut for writing addresses.
Imagine a big city with many streets. Writing full addresses for each place can take a lot of time. Instead, you use shorter forms. For example, instead of writing “123 Main Street, Apartment 4B,” you might write “123 Main St., Apt 4B.” This makes it quicker and easier to understand.
Similarly, scientific notation helps simplify numbers. For instance, instead of writing “0.000000123,” we can write “1.23 × 10⁻⁷.” This form is shorter and more useful for calculations.
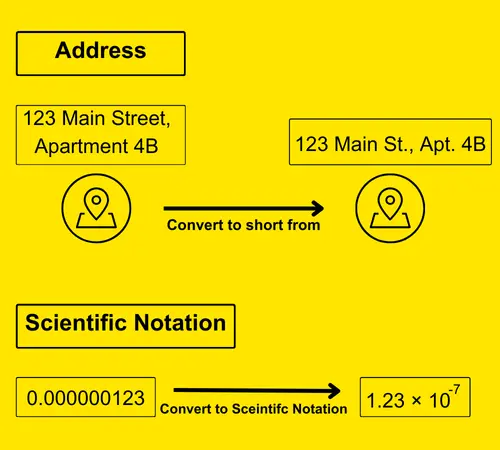
Python Syntax for Scientific Notation
In Python, we use the letter ‘e’ (or ‘E’) to show powers of 10. The ‘e’ stands for “exponent” and is used to work with large or small numbers.
Syntax
number = aEb
a
is the base (a float or integer).E
(or e) indicates “times ten raised to the power of”.b
is the exponent.
Default Behaviour Of Python in Displaying Numbers
By default, Python shows numbers in scientific notation based on their size. Here’s how it works:
- If a number is between 10-4 and 1015, Python will display it in decimal form.
- However, if a number is smaller than 10-4 or larger than 1015, Python will use scientific notation to show it.
Example
In the code snippet, the output of the first number is in decimal format as it is within the specified range, while the other numbers are displayed in scientific notation since they fall outside that range.
# This number is between 10^-4 and 10^15 print(f'Decimal number = {decimal_number}') decimal_number = 123456789.0 # This number is smaller than 10^-4 scientific_number_large = 1.23e16 # This number is larger than 10^15 scientific_number_small = 1.23e-5 scientific_number_large = 1.23e16 print(f'Decimal number = {decimal_number}') print(f'Small scientific number = {scientific_number_small}') print(f'Large scientific number = {scientific_number_large}')
Output
Decimal number = 123456789.0 Small scientific number = 1.23e-05 Large scientific number = 1.23e+16
Format Numbers in Scientific Notation and in Standard Form
In Python, you can format numbers in both scientific notation and standard form depending on your requirements. You can use methods such as str.format()
, f-strings, and libraries like NumPy and Pandas to switch between these forms for better control over the display of numbers.
1. str.format()
The str.format()
method allows you to display a number in both scientific notation and standard form. This method provides flexibility for formatting based on your needs.
Syntax
"{:e}".format(number)
{:}
: Placeholder for the value.e
: Format specifier for scientific notation..nf
: Specifies the number of decimal places for standard form.
Example
This code formats the number 9732
into scientific notation and standard form:
num = 9732 print("Scientific Notation:", "{:e}".format(num)) # Scientific form print("Standard Form:", "{:.2f}".format(num)) # Standard form with 2 decimal places
Output
Scientific Notation: 9.732000e+03 Standard Form: 9732.00
2. f-strings
With f-strings, you can format numbers into both scientific notation and standard form. F-strings make it easy to embed variables in strings while controlling the display format.
Syntax
f"{value:e}" # For scientific notation f"{value:.nf}" # For standard form (n specifies decimal places)
n
: Number of decimal places (e.g., in “.2f
”, “2” means two digits after the decimal).f
: Fixed-point notation, displaying the number in decimal form instead of scientific notation.
Example
This helps you control how numbers appear. For example, you can show ten decimal places using a format specifier. This format makes Python display the full number instead of using scientific notation, as shown in the code below:
number = 12300000 print(f"Scientific Notation: {number:e}") # Scientific form print(f"Standard Form: {number:.2f}") # Standard form with 2 decimal places
Output
Scientific Notation: 1.230000e+07 Standard Form: 12300000.00
3. NumPy’s np.set_printoptions()
In NumPy, you can control how numbers are displayed by using the np.set_printoptions()
function. By setting suppress=True
, you can prevent scientific notation and show the full decimal values instead.
Syntax
np.set_printoptions(precision=n, formatter={'float_kind':'{:e}'.format}) # For scientific notation np.set_printoptions(suppress=True, precision=n) # For standard form
precision=n
: Specifies the number of decimal places.suppress=True
:- Ensures numbers are shown in standard form instead of scientific notation.
Example
This code shows how to switch between scientific and standard form for a NumPy array:
import numpy as np array = np.array([0.00001, 200000]) # Scientific notation np.set_printoptions(precision=2, formatter={'float_kind':'{:e}'.format}) print("Scientific Notation:", array) # Standard form np.set_printoptions(suppress=True, precision=2) print("Standard Form:", array)
Output
Scientific Notation: [1.00e-05 2.00e+05] Standard Form: [0.00 200000.00]
4. Panda’s pd.set_option()
In Pandas, you can format DataFrame columns to control how numbers are displayed. By using the pd.set_option()
function, you can prevent scientific notation and show the full decimal values.
Syntax
pd.set_option('display.float_format', '{:e}'.format) # For scientific notation pd.set_option('display.float_format', '{:.nf}'.format) # For standard form (n specifies decimal places)
'display.float_format'
: Option that sets the format for displaying floating-point numbers.
Example
This code formats a Pandas DataFrame column to display numbers in both scientific notation and standard form:
import pandas as pd df = pd.DataFrame([0.00001, 200000], columns=['Value']) # Scientific notation pd.set_option('display.float_format', '{:e}'.format) print("Scientific Notation:\n", df) # Standard form pd.set_option('display.float_format', '{:.5f}'.format) print("Standard Form:\n", df)
Output:
Scientific Notation: Value 0 1.000000e-05 1 2.000000e+05 Standard Form: Value 0 0.00001 1 200000.00000
Convert Scientific Notation to Standard Form
Sometimes, you may need to remove or convert a scientific notation to standard form in Python. This is common for financial calculations or reports. For this, you can cast the number as an float
or decimal
or int
according to your needs. Python will automatically handle the conversion, but sometimes, for better readability, you might want to format it explicitly.
Example
The code below converts a scientific notation into a standard decimal and integer form using type casting.
import decimal # Number in scientific notation number = 1.23e5 # Convert to float float_value = float(number) # Convert to decimal decimal_value = decimal.Decimal(number) # Convert to integer integer_value = int(number) print(f"Float value: {float_value}") print(f"Decimal value: {decimal_value}") print(f"Integer value: {integer_value}")
Output
Float value: 123000.0 Decimal value: 123000 Integer value: 123000
Conclusion
Scientific notation is a helpful way to simplify numbers in Python. It makes working with both big and small numbers easier. By learning how to use it, you can improve your skills in handling data. This knowledge will make your Python projects and calculations more efficient and manageable.
FAQs
Why is scientific notation useful in Python?
Scientific notation is used to simplify very large or very small numbers, making them more manageable in both reading and calculations. This is useful in fields like science, engineering, and data analysis.
How do you write a number in scientific notation in Python?
In Python, scientific notation is written using the letter “e” to represent powers of ten. For example, 1.23e4
means 1.23 × 104
, which equals 12,300.
What happens if you don’t format numbers in Python?
Python automatically formats very small or large numbers in scientific notation to make them easier to read. Numbers between 10^-4
and 10^15
are shown in decimal form by default.
Can you prevent scientific notation in Python?
Yes, by using formatting techniques like f-strings
or libraries like NumPy and Pandas, you can force numbers to be displayed in full decimal form, even if they are very small or large.
What is the easiest way to convert scientific notation to standard form?
To change the scientific notation to standard form, just convert the number to a float. Python will automatically show the number in decimal form. So, when you cast the number to float, it becomes a regular decimal.