Learn all the possible ways to remove a new line (\n) from a string in Python, including strip()
, rstrip()
, lstrp()
, replace()
, split()
& join()
, splitlines()
and regular expressions.
How To Remove a New Line From a String in Python?
If you’re working with strings in Python, you may often need to strip newlines (\n
). Whether it’s cleaning up text files, formatting output, or processing user input, there are multiple ways to remove newlines from a string.
Let’s discuss all of the methods in detail with example codes and visual analogies.
Use the rstrip() Method
One of the easiest ways to remove new lines from a string in Python is by using the rstrip()
method, which is a pre-defined function. It stands for right strip, that’s why it removes (strips) the newlines (\n) and spaces from the right i-e the end of the string.
Code
multi_line_string="\n\n\n How to \n remove \n new line \n\n from string \n in Python \n\n\n" cleaned_string=multi_line_string.rstrip() print(cleaned_string)
Output
How to remove new line from string in Python
Apply the lstrip() Method
The lstrip()
method stands for left-strip that removes the leading spaces i-e spaces and new lines from the left (start) of the string while rstrip()
strips newline from the end of the string.
Code
multi_line_string="\n\n\n How to \n remove \n new line \n\n from string \n in Python \n\n\n" cleaned_string=multi_line_string.lstrip() print(cleaned_string)
Output
How to remove new line from string in Python
Use the strip() Method
The strip()
method strips newlines and spaces from both ends of the string. It is equivalent to using both lstrip()
and rstrip()
together.
Code
multi_line_string="\n\n\n How to \n remove \n new line \n\n from string \n in Python \n\n\n" cleaned_string=multi_line_string.strip() print(cleaned_string)
Output
How to remove new line from string in Python
Real-World Example
Imagine your car got dirty after a long trip, and now you want to clean it. If you clean only the front, it’s like using lstrip()
. If you clean only the back, it’s like using rstrip()
. But if you clean both the front and the back, while leaving the middle dirty, it’s like using strip()
.
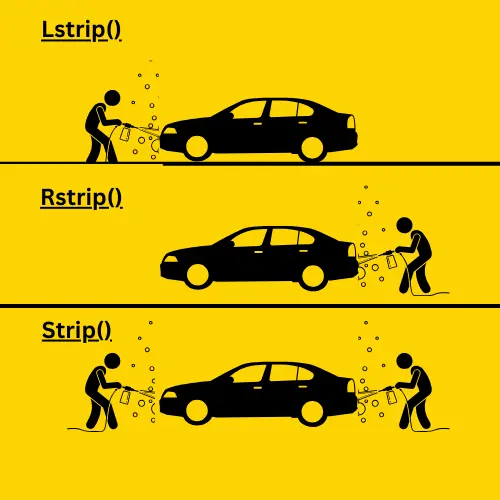
Remove Newline Using the Replace() Method
The replace()
method can remove all occurrences of \n
from anywhere in the string, not just the beginning or end.
To use the replace() method, we need to call the method on the string from which we want to remove the newlines. The first parameter will be the substring we want to replace (in this case, the newline character), and the second parameter will be the new string (which, in this case, will be an empty string to replace the new lines).
Code
multi_line_string="\n\n\n How to \n remove \n new line \n\n from string \n in Python \n\n\n" cleaned_string=multi_line_string.replace("\n","") print(cleaned_string)
Output
How to remove new line from string in Python
split() and join() Method
Imagine you have a chain made of a mix of steel and copper links. You want to get rid of the copper links, so you start by breaking the chain apart, separating the steel and copper. After you’ve done that, you set the copper links aside and reattach the steel parts, creating a chain that’s entirely made of steel, just like you wanted.
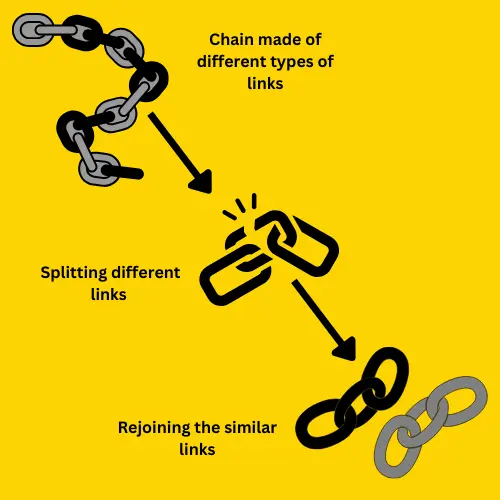
In Python, the split()
and join()
methods work in a similar way. The split()
method takes a parameter and breaks the string wherever it finds the word or character passed in the parameter. When the split()
method is called, it divides the string into smaller substrings and returns a list. Each substring is an element of the string.
On the other hand, the join()
method takes a list of strings and combines them into a single string. We will use the list created by the split()
method. To do this, we need to call the join()
function with a specified separator. Python places the separator between each substring when joining them. In our case, since we don’t want to add anything between the substrings, we will use an empty string as a separator. The list will be passed as a parameter to the join function and will return a string
Code
multi_line_string="\n\n\n How to \n remove \n new line \n\n from string \n in Python \n\n\n" strings_list=multi_line_string.split("\n") cleaned_string="".join(strings_list) print(cleaned_string)
Output
How to remove new line from string in Python
The splitlines() Method
The splitlines()
method works similarly to the split()
method. The main difference is that in Python, when we use the split()
method, we pass a parameter to the split()
function and the string is trimmed into substrings, wherever that parameter is found. If no parameter is provided, it splits the string at every blank space by default.
On the other hand, if the splitlines()
method is called without parameters, it breaks the string into smaller pieces based on line breaks (e.g., \n
, \r
). Just like the split() method, when the splitlines()
method is called on a string, it breaks the string into smaller chunks and returns a list, with each substring as an element of that list. We can then combine the elements of that list back into a single string using the join() method just like we did in the split() and join() method.
Code
multi_line_string="\n\n\n How to \n remove \n new line \n\n from string \n in Python \n\n\n" strings_list=multi_line_string.splitlines() cleaned_string="".join(strings_list) print(cleaned_string)
Output
How to remove new line from string in Python
Regular Expressions to Remove Newlines
A regular expression is a special pattern of characters which can be used to manipulate strings. It is a very powerful and useful tool when it comes to string manipulation.
Regular expressions can be used to remove all unnecessary lines from a string using the sub()
method. In the sub()
method we will have to pass three parameters. The first parameter is the element that we want to replace, second is the element that we want to replace it with and third is the string from which we want to manipulate. Basically, it works like the replace()
method which we have discussed earlier. Before using regular expressions, we have to make sure that the ‘re
’ module is imported use ‘import re
‘ statement.
Once the re module is imported, we can use the sub()
method by placing a dot after re
. It will return a string which we will be storing in a string variable. The following statement can be used to call the sub()
method ‘re.sub(r'\n','',multi_line_string)
‘. The ‘r
’ stands for ‘raw string’ which is used to ignore escape sequences. It treats ‘\’
as a normal character rather than treating it as an escape character. Hence by utilizing regular expressions in this way, we can remove all the new line characters from string in Python.
Code
import re multi_line_string="\n\n\n How to \n remove \n new line \n\n from string \n in Python \n\n\n" cleaned_string=re.sub(r'\n','',multi_line_string) print(cleaned_string)
Output
How to remove new line from string in Python
Comparison Table for All Methods
Method | Removes New Line from Start | Removes New Line from Middle | Removes New Line from End | Splits String | Rejoins String |
rstrip() | No | No | Yes | No | No |
lstrip() | Yes | No | No | No | No |
strip() | Yes | No | Yes | No | No |
replace() | Yes | Yes | Yes | No | No |
sub() (from re) | Yes | Yes | Yes | No | No |
split() + join() | Yes | Yes | Yes | Yes | Yes |
splitlines() + join() | Yes | Yes | Yes | Yes | Yes |
Why Remove New Lines from a String?
Removing newlines from a string in Python is often necessary for various reasons. Here are some common scenarios:
- Data Cleaning: When processing input data (like user input or file reading), newlines can interfere with further analysis or processing. Removing them ensures clean data.
- Formatting Output: When displaying text, extra newlines can create unwanted gaps or formatting issues. Stripping newlines helps maintain a neat presentation.
- String Comparison: When comparing strings, newlines can cause unexpected results. Removing them ensures accurate comparisons.
- Parsing and Tokenization: In tasks like parsing CSV files or other structured data, newlines may separate rows or entries. Stripping them can help in correctly splitting and processing data.
- Logging and Debugging: When logging messages, newlines may lead to confusing outputs. Removing them helps create concise log entries.
- Joining Strings: When concatenating strings, newlines can result in unintended breaks. Stripping them allows for smooth concatenation.
- User Input Validation: In user interfaces, stripping newlines can help enforce input rules (e.g., preventing empty lines in forms).
Conclusion
Unnecessary new lines are a problem in Python which can be solved using different methods. The rstrip()
removes the spaces and new lines from the end of the string, the lstrip()
removes from the beginning of the string, while the strip()
method removes both, from beginning and ending of the string. Similarly, the replace()
and sub()
method(from regular expressions) can be used to replace the new line character with empty space. The split()
and splitlines()
can be used to split the string into substring whenever the new line character is read and then rejoin the substrings to form a string using join()
method.