Have you ever needed to add an element at the beginning of a list in Python? You probably know how to append items to the end using .append()
, but what about inserting them at the start? Python doesn’t have a built-in .prepend()
method, but there are several ways to prepend elements to a list. In this article, we’ll explore each method and discuss the best use cases for each.
Why Do You Need to Prepend to a List?
Prepending elements to a list is a common task in programming, especially when working with queues, stacks, logs, and real-time data processing. If you’re managing event timelines, reversing operations, or structuring ordered data, you’ll often need to add elements at the beginning of a list rather than the end.
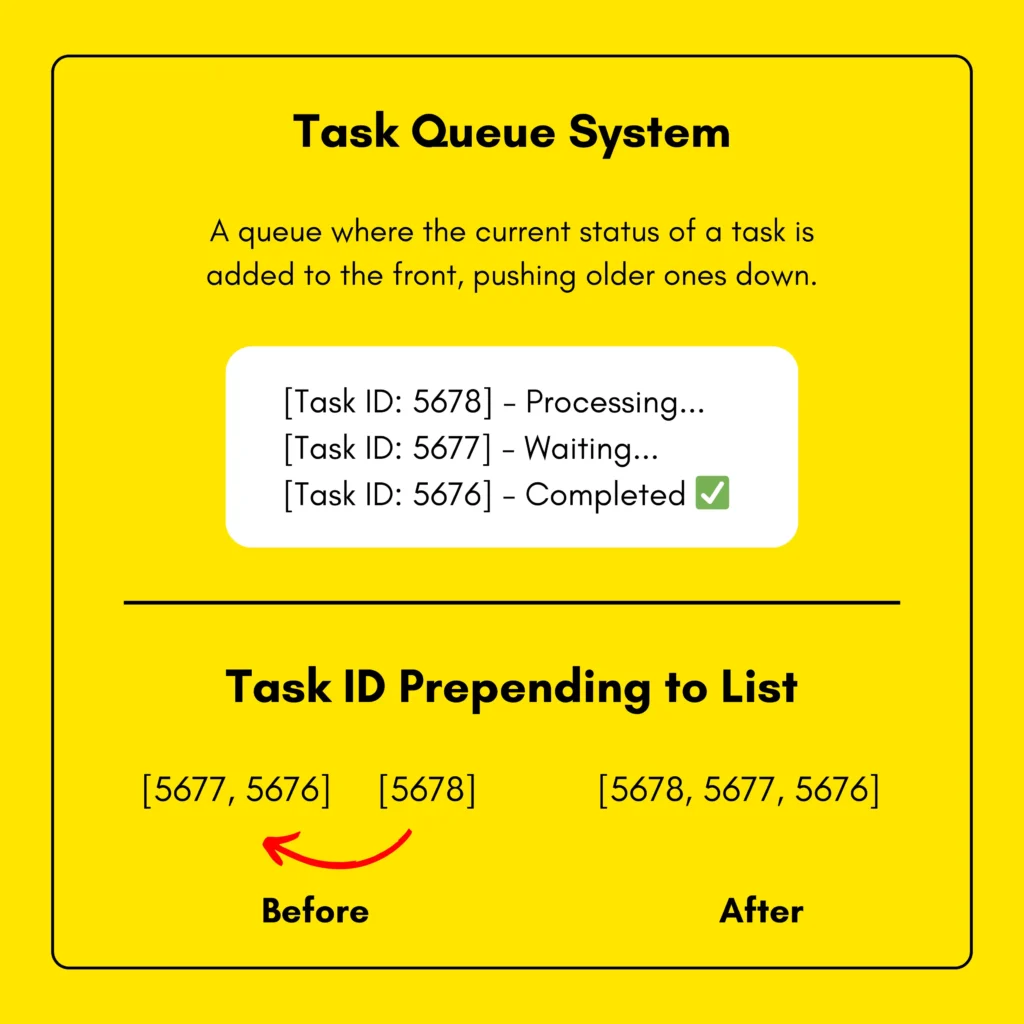
6 Different Ways to Prepend Elements to a List in Python
1. Using insert() Method
The .insert()
method is the most intuitive way to add an item at the beginning of a list. It allows inserting an element at any specific index.
Example Code
In this example, we first initialize a list whole_numbers
containing five whole numbers. The whole_numbers.insert(0, 0)
method inserts the value 0
at index 0
, which is the beginning of the list. After that, we print the updated list to the console.
whole_numbers = [1, 2, 3, 4, 5] # List of whole numbers whole_numbers.insert(0, 0) # Insert 0 at the beginning print(whole_numbers) # Print updated list
Output
[0, 1, 2, 3, 4, 5]
Best Use Case
The .insert()
method is ideal when you need to add a single item at the start of a list without modifying other elements.
2. Using + Operator (List Concatenation)
If you want to prepend multiple elements, the +
operator (concatenation) is a clean and effective way. However, note that it does not modify the original list but instead creates a new one.
Example Code
Here, we have a list called best_friends
. The expression ["Hermione"] + best_friends
creates a new list by prepending Hermione
to the original list, rather than modifying the original list itself. We then print the updated list.
best_friends = ["Harry", "Ron"] # Original list best_friends_updated = ["Hermione"] + best_friends # Prepend Hermione print(best_friends_updated) # Print new list
Output
['Hermione', 'Harry', 'Ron']
Best Use Case
List concatenation is particularly useful when you want to prepend multiple elements at once, all while keeping the original list intact.
3. Using * Operator
If you like concatenation but need flexibility, the *
operator can help unpack elements dynamically. This is an elegant way to prepend multiple elements in one go.
Example Code
In this example, we have two lists, stationery1
and stationery2
, each containing some items. The expression [*stationery2, *stationery1]
expands both lists and combines them, storing the result in a new list called pouch
. Finally, we print the result to the output screen.
stationery1 = ["pencil", "pen", "sharpener"] # List 1 stationery2 = ["eraser", "ruler"] # List 2 pouch = [*stationery2, *stationery1] # Prepend multiple elements print(pouch) # Print new list
Output
['eraser', 'ruler', 'pencil', 'pen', 'sharpener']
Best Use Case
The *
operator is great for dynamically prepending elements from another iterable, such as a generator or tuple.
4. Using collections.deque
If you’re dealing with large lists and need efficiency, collections.deque
is the way to go. It is optimized for fast insertions and deletions at both ends of the list.
Example Code
In the following code, we first import deque
from the collections
module. Then, we create a deque object called prime_numbers
and initialize it with some prime numbers. The appendleft(2)
method adds the number 2
to the left side (front) of the prime_numbers
deque. Finally, the list is printed on the screen.
from collections import deque # Importing deque prime_numbers = deque([3, 5, 7, 11]) # Initial list prime_numbers.appendleft(2) # Prepend 13 to the left print(list(prime_numbers)) # Print updated list
Output
[2, 3, 5, 7, 11]
Best Use Case
collections.deque
is the best method for prepending elements to a list in real-time applications, such as processing logs, maintaining history, or implementing queues.
5. Using Slicing
Slicing is another Pythonic way to prepend elements to a list. It lets you modify a list in place without creating a new object.
Example Code
Here, we have a list of items called inventory
. inventory[:0]
means we insert the new items, charger
and headphones
, at the start of the list without replacing any existing items. Lastly, we print the final updated list.
inventory = ["laptop", "mouse", "keyboard"] inventory[:0] = ["charger", "headphones"] # Prepend items to the list print(inventory) # Print updated list
Output
['charger', 'headphones', 'laptop', 'mouse', 'keyboard']
Best Use Case
Slicing is best for scenarios where you need to modify a list in place while keeping the syntax concise.
6. Using a Custom Function
If you want reusability in your code and make it cleaner, creating a custom function is the best approach for prepending elements to your list.
Example Code
In this example, we first define a function prepend
that takes two parameters, lst
and elements
, and returns the concatenation of the two lists. We then create two lists, prices
and new_prices
, each containing some values. Next, we call the prepend
function, passing these two lists as arguments. The function merges both lists and prints the result.
def prepend(lst, elements): return elements + lst prices = [19.99, 29.99, 39.99] new_prices = [9.99, 14.99] print(prepend(prices, new_prices))
Output
[9.99, 14.99, 19.99, 29.99, 39.99]
Best Use Case
This method is ideal for enhancing the reusability and maintainability of your code, especially when you need to prepend elements dynamically across multiple lists.
FAQs Related to Prepending Elements to a List in Python
1. What is the fastest way to prepend to a list in Python?
Using collections.deque.appendleft()
is the fastest method for prepending elements, especially for large datasets.
2. Can I use append() to prepend elements?
No, .append()
only adds elements to the end of a list. To add elements to the start, use .insert(0, element)
or deque.appendleft()
.
3. How do I prepend multiple elements at once?
You can use slicing (my_list[:0] = [1, 2, 3]
) or list concatenation (my_list = [1, 2, 3] + my_list
).
4. Is deque always faster than lists?
For frequent insertions at the beginning, yes. But for occasional prepends, a regular list is fine.
End Notes
Now that you know 6 different ways to prepend to a list in Python, choosing the right method depends on your specific use case. Each approach has its own strengths, so consider factors like performance, reusability, and the nature of your data to determine the most suitable option for your project.
For more amazing and beginner-friendly tutorials on Python programming, don’t forget to check out the dedicated Python series at Syntax Scenarios.