In everyday life, we often do the same tasks over and over, like calculating the total price of multiple items in a shopping cart. Loops help us do these tasks easily in programming. In Python, loops let you repeat tasks without writing the same code repeatedly. In this article, Syntax Scenarios provides fun, real-world examples of how loops work and teach you how to use them in Python. By the end, you’ll see how loops can make your code faster and simpler!
Stargazing Logbook
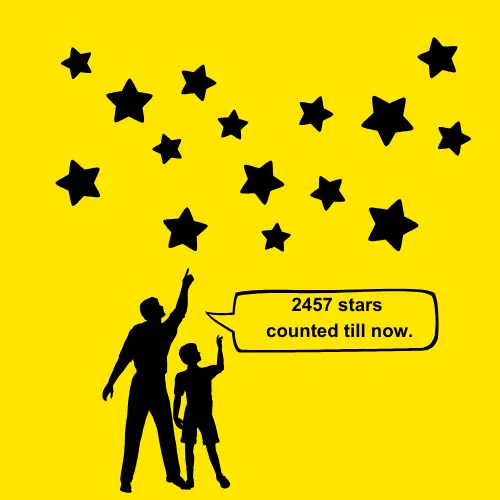
You love stargazing and decide to count the stars in the sky every night. Each night, the number of stars you see is different because of changes in the weather and how clear the sky is. You want to write a program that lets you enter the number of stars you see each night, keeps a running total, and shows you the total number of stars after several nights.
Test Case:
Input:
Nights: 4
Stars counted each night: 20, 35, 25, 40
Expected Output:
Total stars counted: 120
Explanation:
This code calculates the number of stars counted over a specified number of nights.
- It asks you to input the number of nights you will count stars, storing this value in the
nights
variable as an integer usingint(input())
. - The
total_stars
variable starts at 0 to keep track of the overall star count. - A
for
loop runs from 1 tonights + 1
, where each iteration represents a single night. - Inside the loop, the code asks you to input the number of stars counted on the current night, storing the value in the
stars
variable as an integer. - The code adds the stars counted that night to the
total_stars
usingtotal_stars += stars
. - After updating the total, the code prints the cumulative total of stars so far along with the night number.
- When the loop ends, the code prints the total number of stars you counted across all the nights, formatted in a complete sentence.
nights = int(input("How many nights will you count stars? ")) total_stars = 0 for night in range(1, nights + 1): stars = int(input(f"How many stars did you count on night {night}? ")) total_stars += stars # Add this night's stars to the total print(f"After night {night}, total stars so far: {total_stars}") print(f"In {nights} nights, you counted a total of {total_stars} stars!")
Water Intake Tracker
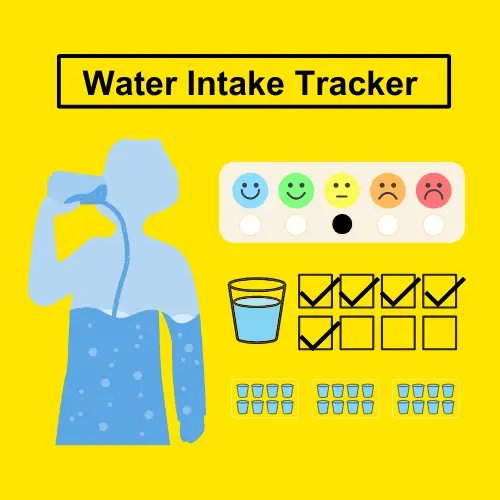
Do you want to track how much water you drink every day for a week? For that, you want to know the total amount of water you’ve consumed by the end of the week.
Write a Python program to calculate the total water intake for the week based on how much you drink each day.
Test Case:
Input:
Day 1: 2.5 liters
Day 2: 3 liters
Day 3: 2 liters
Day 4: 2.5 liters
Day 5: 3 liters
Day 6: 3.5 liters
Day 7: 2 liters
Expected Output:
Total water consumed: 18.5 liters
Explanation:
This code calculates the total water consumed over 7 days.
- First, the
total_water
variable is initialized to 0 to keep track of the running total of water consumed. - Then, a
for
loop is used to repeat the process of asking for daily water intake. It runs for 7 days (from day 1 to day 7) using therange(1, 8)
. - Inside the loop, the
input()
function asks the user to enter the amount of water they drank on each day. This input is converted into a float usingfloat()
to handle decimal values, representing liters. - The
total_water
is updated by adding the daily intake to it after each day’s input. - Finally, after the loop finishes, the
print()
function displays the total water consumed over the 7 days.
total_water = 0 for day in range(1, 8): daily_water = float(input(f"Enter water intake for Day {day} (in liters): ")) total_water += daily_water print(f"Total water consumed: {total_water} liters")
Book Reading Challenge
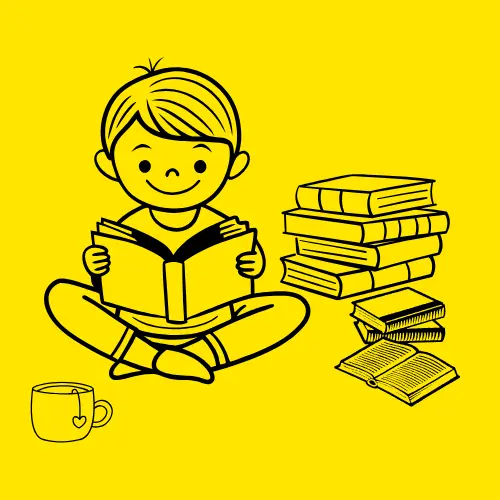
You’ve set a goal to read a certain number of pages every day for a month, but you plan to increase the number of pages you read each week. The first week, you read a fixed number of pages every day. In the second week, you decide to read 5 more pages each day compared to the previous week. In the third week, you decide to increase it by another 5 pages per day, and so on for the entire month.
Write a Python program that calculates the number of pages you will read in a month based on this increasing pattern.
Test Case:
Input:
Pages to read in the first week: 20
Days in a month: 30
Expected Output:
Total pages read: 1200
Explanation:
This code calculates the total number of pages read in a month, considering that the number of pages read per day increases each week.
- First, it asks the user to enter the number of pages read per day during the first week, and the total number of days in the month. These inputs are converted into integers using
int()
. - The
total_pages
variable is initialized to 0 to keep track of the total pages read. The variablepages_per_day
is set to the pages read in the first week andweeks_in_month
is calculated by dividing the total days by 7 to get the number of full weeks. The remaining days after the full weeks are calculated using the modulus operator (%
). - Then, a
for
loop runs through each week in the month. For each full week, it adds the pages read tototal_pages
by multiplying the pages read per day by 7. After each week, the pages per day are increased by 5. - After the loop, if there are any remaining days (less than a full week), it adds the pages read for those remaining days to
total_pages
. - Finally, the
print()
function displays the total pages read over the month.
pages_per_day_first_week = int(input("Enter the number of pages you read per day in the first week: ")) days_in_month = int(input("Enter the number of days in the month: ")) total_pages = 0 pages_per_day = pages_per_day_first_week weeks_in_month = days_in_month // 7 # Calculate number of full weeks remaining_days = days_in_month % 7 # Days left after full weeks # Loop through each week for week in range(1, weeks_in_month + 1): total_pages += pages_per_day * 7 # Add pages read in each full week pages_per_day += 5 # Increase pages per day by 5 for the next week # Handle the remaining days in the last partial week if remaining_days > 0: total_pages += pages_per_day * remaining_days print(f"Total pages read: {total_pages}")
Weekly Grocery Budget
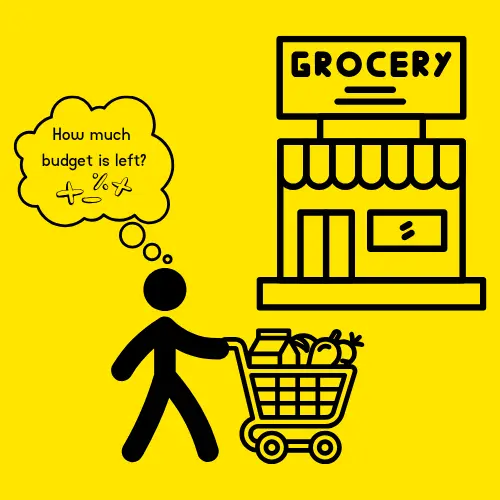
You have a weekly grocery budget of $150. Each time you shop, you enter the cost of the items purchased. If your spending exceeds your budget, the program warns you. Use a while
loop to track your expenses until you reach the budget limit.
Test Case:
Input:
Shopping 1: $50
Shopping 2: $40
Shopping 3: $30
Shopping 4: $50
Expected Output:
Total spent: $50. You have $100 remaining.
Total spent: $90. You have $60 remaining.
Total spent: $120. You have $30 remaining.
Total spent: $170. Warning: You exceeded your budget by $20.
Explanation:
This code helps track spending during shopping and alerts the user if they exceed their budget.
- The
total_spent
variable is initialized to 0 to keep track of the total amount spent, and thebudget
is set to $150. - A
while
loop runs as long astotal_spent
is less than or equal to the budget. Inside the loop, theinput()
function asks the user for the cost of their shopping, and thefloat()
function converts this input into a decimal number to handle monetary values. - After each expense is entered, it is added to the
total_spent
. - If the updated
total_spent
is still within the budget, the remaining amount is calculated by subtractingtotal_spent
frombudget
. The program then prints the total spent and the remaining budget. - If the updated
total_spent
exceeds the budget, the amount exceeded is calculated by subtractingbudget
fromtotal_spent
. The program warns the user that they have exceeded the budget and displays how much they went over.
total_spent = 0 budget = 150 while total_spent <= budget: expense = float(input("Enter the cost of your shopping: ")) total_spent += expense if total_spent <= budget: remaining = budget - total_spent print(f"Total spent: ${total_spent:.2f}. You have ${remaining:.2f} remaining.") else: exceeded = total_spent - budget print(f"Total spent: ${total_spent:.2f}. Warning: You exceeded your budget by ${exceeded:.2f}.")
Designing a Gift Wrap Pattern
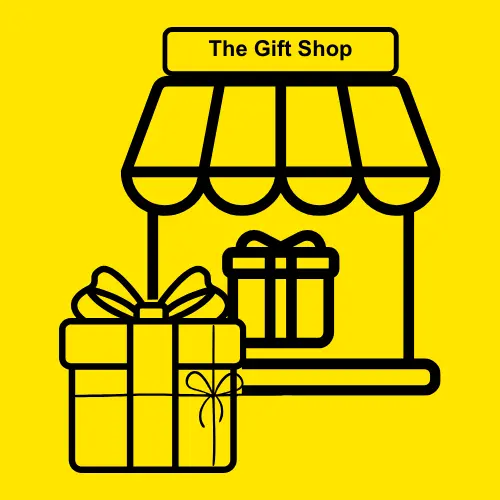
A gift shop owner wants to design a unique wrapping paper with a triangular pattern of stars. The wrapping paper should have 5 rows of stars, with each row having one more star than the previous row. Write a Python program to create this design using loops.
Test Case:
Input:
No input required.
Expected Output:
*
**
***
****
*****
Explanation:
This code generates a simple pattern of stars (*
) where each row has an increasing number of stars, starting from 1 star in the first row.
- The variable
row
is initialized to 1 to represent the starting row, andtotal_rows
is set to 5, defining the total number of rows in the pattern. - A
while
loop runs as long as the currentrow
number is less than or equal tototal_rows
. - Inside the loop, the
print()
function prints a string of stars, with the number of stars determined by multiplying the*
character by the current value ofrow
. For example, whenrow
is 1, it prints one star, and whenrow
is 2, it prints two stars. - After printing the stars for the current row, the
row
variable is incremented by 1 (row += 1
), moving to the next row. - The loop continues until all rows are printed.
row = 1 total_rows = 5 while row <= total_rows: print("*" * row) # Print stars equal to the current row number row += 1 # Move to the next row
Reversing a Lottery Ticket Number
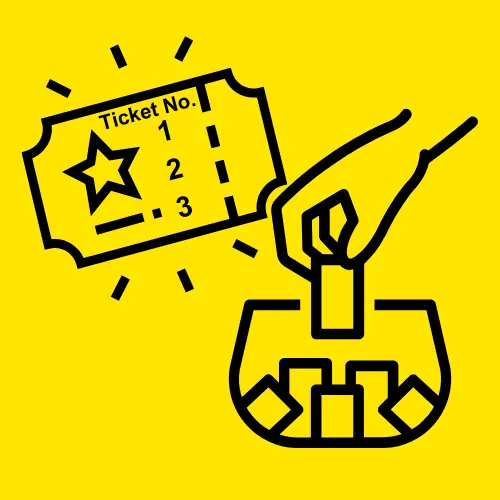
Imagine you are a lottery organizer. Each lottery ticket has a unique 3-digit number, and you want to create a special reward code by reversing the digits of the ticket number. For example, if the ticket number is 123, the reward code will be 321. This reversed number will be used to determine bonus prizes for the lucky participants.
How can you reverse the digits of a 3-digit lottery ticket number using Python?
Test Case:
Input:
123
Expected Output:
The reversed reward code is: 321
Explanation:
This code reverses a 3-digit lottery ticket number and displays it as a “reward code.”
- The
ticket_number
variable is initialized by taking the user’s input, which is converted into an integer usingint()
. - The
reversed_number
variable is initialized to 0 and will store the reversed ticket number as the digits are extracted. - A
while
loop runs as long as theticket_number
is greater than 0. Inside the loop, the last digit ofticket_number
is extracted using the modulus operator (% 10
) and stored in the variabledigit
. - The extracted digit is then added to
reversed_number
, but first,reversed_number
is multiplied by 10 to shift its digits left (making space for the new digit). - After adding the digit, the last digit is removed from
ticket_number
using integer division (// 10
). - The loop continues until all digits are reversed, and then the
print()
function displays the final reversed reward code.
ticket_number = int(input("Enter a 3-digit lottery ticket number: ")) reversed_number = 0 while ticket_number > 0: digit = ticket_number % 10 # Extract the last digit reversed_number = reversed_number * 10 + digit # Add it to the reversed number ticket_number //= 10 # Remove the last digit print(f"The reversed reward code is: {reversed_number}")
Parking Lot Earnings
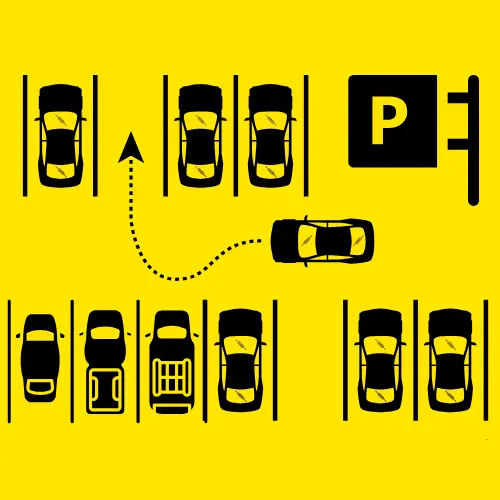
You run a busy parking lot that charges customers based on how long they park. The first hour costs $5, and every additional hour costs $3. You want to calculate how much money your parking lot earns from 10 cars based on their parking durations.
For example, if one car parks for 1 hour, it will pay $5. If another car parks for 4 hours, it will pay $5 for the first hour and $9 for the remaining 3 hours (3 hours × $3). By adding up the earnings from all the cars, you can figure out how much your parking lot has made.
Write a Python program that calculates the total earnings from 10 cars, given their parking durations.
Test Case:
Input:
Enter parking duration for car 1: 1
Enter parking duration for car 2: 2
Enter parking duration for car 3: 3
Enter parking duration for car 4: 4
Enter parking duration for car 5: 5
Enter parking duration for car 6: 1
Enter parking duration for car 7: 2
Enter parking duration for car 8: 3
Enter parking duration for car 9: 4
Enter parking duration for car 10: 5
Expected Output:
Total earnings: 95
Explanation:
This code calculates the total earnings from parking fees for 10 cars, based on the duration of their parking.
- The
total_earnings
variable is initialized to 0 to keep track of the total amount earned. - A
for
loop runs 10 times, once for each car, usingrange(1, 11)
. For each iteration, the program asks the user to input the parking duration (in hours) for the current car using theinput()
function, which is converted into an integer usingint()
. - If the parking duration is more than 1 hour, the parking fee is calculated as $5 for the first hour, and $3 for each additional hour (calculated by subtracting 1 from the total hours and multiplying by 3
(hours - 1) * 3
). - If the parking duration is 1 hour or less, the fee is simply $5.
- The calculated fee for each car is added to the
total_earnings
. - After all 10 cars have been processed, the
print()
function displays the total earnings from the parking fees.
total_earnings = 0 for car in range(1, 11): hours = int(input(f"Enter parking duration for car {car} (in hours): ")) if hours > 1: fee = 5 + (hours - 1) * 3 # $5 for the first hour, $3 for each additional hour else: fee = 5 # $5 for the first hour total_earnings += fee print("Total earnings:", total_earnings)
Discounted Price for Bulk Purchases
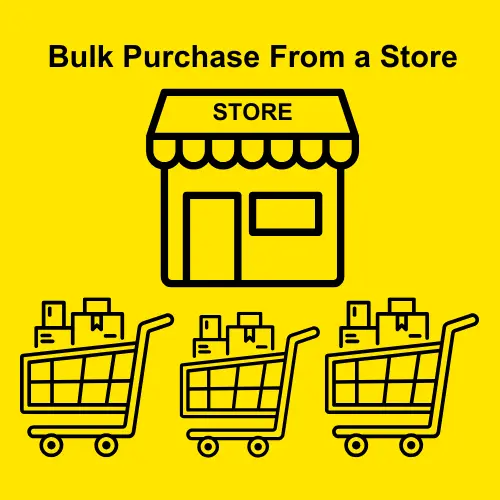
You run a small shop that sells products in bulk. The price per unit decreases by $0.50 for each additional unit purchased. Write a Python program to calculate the total cost for purchasing between 1 and 10 units, applying the discount for each additional unit.
Test Case:
Input:
Enter the price per unit: 5
Expected Output:
Units: 1, Price per Unit: $5.00, Total Cost: $5.00
Units: 2, Price per Unit: $4.50, Total Cost: $9.00
Units: 3, Price per Unit: $4.00, Total Cost: $12.00
Units: 4, Price per Unit: $3.50, Total Cost: $14.00
Units: 5, Price per Unit: $3.00, Total Cost: $15.00
Units: 6, Price per Unit: $2.50, Total Cost: $15.00
Units: 7, Price per Unit: $2.00, Total Cost: $14.00
Units: 8, Price per Unit: $1.50, Total Cost: $12.00
Units: 9, Price per Unit: $1.00, Total Cost: $9.00
Units: 10, Price per Unit: $0.50, Total Cost: $5.00
Explanation:
The code calculates the total cost of buying units of a product, applying a discount for each additional unit.
- The user inputs the
price_per_unit
, which is then converted into a floating-point number usingfloat()
. This represents the price for one unit of the product. - A
for
loop runs from 1 to 10, iterating through the number of units being bought. For each loop iteration, the current number of units is stored in theunits
variable. - For each unit, the code calculates the discounted price by subtracting $0.50 for every additional unit beyond the first. The formula used is
price_per_unit - (units - 1) * 0.5
. The first unit has no discount, while the price decreases for each additional unit. - The code calculates the total cost for the current number of units by multiplying the number of units by the discounted price.
- The
print()
function displays the current number of units, the discounted price per unit, and the total cost for that quantity. The price and cost are formatted to two decimal places using:.2f
.
price_per_unit = float(input("Enter the price per unit: ")) for units in range(1, 11): # Calculate the discounted price for each additional unit discount_price = price_per_unit - (units - 1) * 0.5 total_cost = units * discount_price # Total cost for the number of units print(f"Units: {units}, Price per Unit: ${discount_price:.2f}, Total Cost: ${total_cost:.2f}")
The Magical Potion Brewer
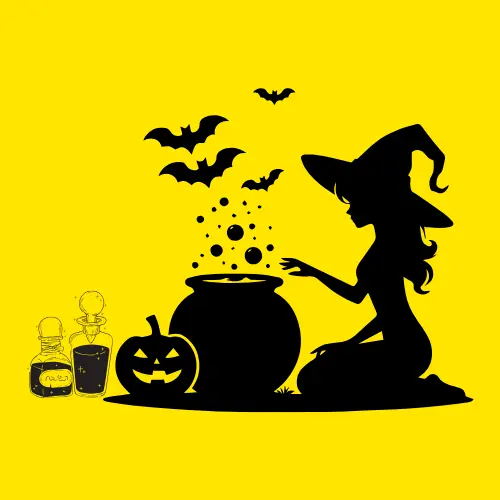
In the kingdom of Azura, a potion maker starts with 5 units of magical herb and 10 units of moonstone. After each batch, the herb goes down by 1 unit and the moonstone goes up by 2 units. After the 10th batch, the potion maker adds 3 more units of herb to each batch to make the potion stronger. The potion maker continues brewing until the total ingredients used is more than 100.
Write a Python program that uses a while loop to track how many ingredients are used in each batch, shows how much herb and moonstone is used, and stops when the total ingredients are over 100. The output of the code should be in the structure defined below.
Test Case:
Input:
Initial herb = 5 units
Initial moonstone = 10 units
Brew until total ingredients exceed 100 units.
Expected Output:
Brewing Batch 1:
Herb used: 5 units
Moonstone used: 10 units
Total ingredients used in this batch: 15 units
Total ingredients so far: 15 units
Brewing Batch 2:
Herb used: 4 units
Moonstone used: 12 units
Total ingredients used in this batch: 16 units
Total ingredients so far: 31 units
Brewing Batch 3:
Herb used: 3 units
Moonstone used: 14 units
Total ingredients used in this batch: 17 units
Total ingredients so far: 48 units
Brewing Batch 4:
Herb used: 2 units
Moonstone used: 16 units
Total ingredients used in this batch: 18 units
Total ingredients so far: 66 units
Brewing Batch 5:
Herb used: 1 unit
Moonstone used: 18 units
Total ingredients used in this batch: 19 units
Total ingredients so far: 85 units
Brewing Batch 6:
Herb used: 0 units
Moonstone used: 20 units
Total ingredients used in this batch: 20 units
Total ingredients so far: 105 units
Total ingredients used after all batches: 105 units
Explanation:
The code simulates making potion batches, changing the ingredients each time while keeping track of how much is used.
- It starts with 5 units of
herb
and 10 units ofmoonstone
. Thetotal_ingredients
starts at 0 to keep track of all the ingredients used, andbatch_number
starts at 1 to count the batches. - A
while
loop keeps running as long astotal_ingredients
is less than or equal to 100. - Inside the loop, the code adds the
herb
andmoonstone
for each batch and updates thetotal_ingredients
. - The code prints details of each batch: how much
herb
andmoonstone
were used, how many ingredients in total for that batch, and the running total. - After each batch, the code reduces
herb
by 1 and adds 2 tomoonstone
for the next batch. It also increases thebatch_number
by 1. - The loop keeps going until the
total_ingredients
is more than 100. - When the loop finishes, the code prints the
total_ingredients
used after all the batches.
herb = 5 moonstone = 10 total_ingredients = 0 batch_number = 1 while total_ingredients <= 100: batch_ingredients = herb + moonstone total_ingredients += batch_ingredients print(f"Brewing Batch {batch_number}:") print(f" Herb used: {herb} units") print(f" Moonstone used: {moonstone} units") print(f" Total ingredients used in this batch: {batch_ingredients} units") print(f" Total ingredients so far: {total_ingredients} units\n") # Update the ingredients for the next batch herb -= 1 moonstone += 2 batch_number += 1 print(f"Total ingredients used after all batches: {total_ingredients} units")
Printing Patterns for a Tree Trunk
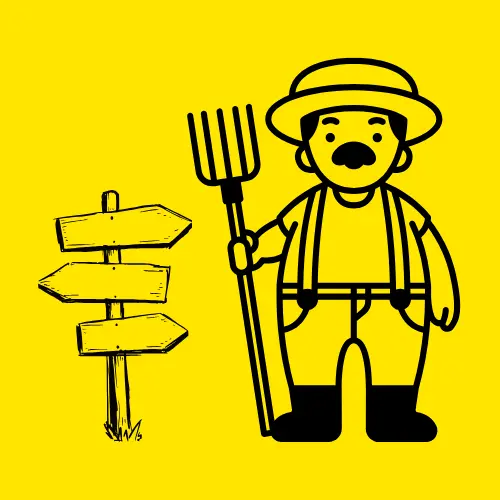
A farmer is making a wooden post for his farm. The post needs to be printed with a simple design, with 5 rows and 3 wooden pieces in each row. He wants to use a program to help him know how the post will look before cutting the wood.
Write a Python program to print the design of the wooden post. The post should have 5 rows, and each row should have 3 pieces of wood (represented by #
symbols).
Test Case:
Input:
Height: 5, Width: 3
Output:
###
###
###
###
###
Explanation:
This code draws a rectangle shape made of #
symbols to represent the wooden post.
- The variables
height
andwidth
are set to 5 and 3, respectively.height
represents the number of rows, andwidth
represents the number of#
symbols printed in each row. - A
for
loop runs forheight
times, which is 5 in this case. The loop variablerow
changes with each iteration, but it isn’t used in the loop itself. - Inside the loop, the code prints
#
symbols. The expression"#" * width
repeats the#
symbolwidth
(3) times in each row. - The loop prints 5 rows of
#
symbols, each containing 3 symbols, creating a rectangle.
height = 5 width = 3 for row in range(height): print("#" * width) # Print width times in each row
A Rare Number Experiment
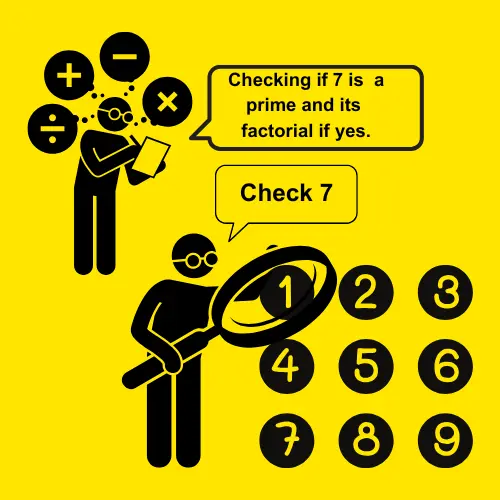
In a mathematics research competition, participants are tasked with exploring special numbers. For each number they select, they must determine whether it is a prime number. If it is prime, they must find its factorial and count the total digits in the factorial. This information will help the researchers understand the relationship between prime numbers and the complexity of their factorials.
Write a Python program to determine if a number is prime, calculate its factorial if it is prime, and count the total digits in the factorial.
Test Cases
Input:
Enter a number: 7
Expected Output:
7 is a prime number.
Factorial of 7 is 5040.
Total digits in the factorial: 4.
Explanation:
This code determines whether a number is prime, calculates its factorial if it is prime, and counts the digits in the factorial.
- First, the user inputs a number using
int(input())
, which is stored in the variablenumber
. - The code initializes
is_prime
asTrue
,factorial
as 1, anddigits
as 0. - A
for
loop runs from 1 to the value ofnumber
(inclusive). Inside the loop:- It checks if
number
is divisible by any value other than 1 and itself. If it finds such a divisor, it setsis_prime
toFalse
. - If the number is still considered prime and
i
is less than or equal tonumber
, the code multipliesfactorial
byi
to calculate the factorial.
- It checks if
- After the loop finishes, the code checks the value of
is_prime
:- If the number is prime and greater than 1, it prints that the number is prime, displays the factorial, and counts the digits in the factorial. To count digits, it converts the factorial to a string using
str()
and finds its length usinglen()
. - If the number is not prime, it simply prints that the number is not prime.
- If the number is prime and greater than 1, it prints that the number is prime, displays the factorial, and counts the digits in the factorial. To count digits, it converts the factorial to a string using
number = int(input("Enter a number: ")) is_prime = True factorial = 1 digits = 0 for i in range(1, number + 1): # Check for primality (only runs for numbers greater than 1) if i > 1 and number % i == 0 and i != number: is_prime = False # Calculate factorial if is_prime and i <= number: factorial *= i # Check the result of primality if is_prime and number > 1: print(f"{number} is a prime number.") print(f"Factorial of {number} is {factorial}.") # Count digits in the factorial factorial_str = str(factorial) digits = len(factorial_str) print(f"Total digits in the factorial: {digits}.") else: print(f"{number} is not a prime number.")
Decode a Hidden Message in Number
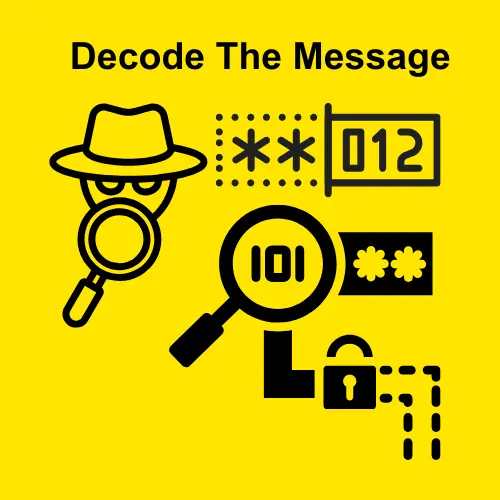
Imagine you’re a mysterious scribe you’re asked to decode a hidden number message. To do this, you first take the given number and multiply it by 3. Then, you look at each digit of the resulting number and transform it: if the digit is odd, you increase it by 1 (for example, 1 becomes 2, 3 becomes 4), and if the digit is even, you decrease it by 1 (for example, 2 becomes 1, 4 becomes 3). Finally, you add all the transformed digits together to get the final decoded number, which holds the secret message.
Your task is to follow these steps to figure out the hidden message using Python script!
Test Case:
Input:
number= 123
Expected Output:
The decoded number is: 19
Explanation:
This code takes a number, applies some transformations to it, and calculates a decoded value based on its digits.
- The user inputs a number, and it is stored in the variable
number
. - The code multiplies
number
by 3 and stores the result innew_number
. - The code then converts
new_number
into a string (new_number_str
) to easily access each individual digit. - A
for
loop iterates through each digit in the string:- It converts each
digit
back to an integer. - If the digit is even (i.e., divisible by 2), the code subtracts 1 from it and adds the result to
decoded_value
. - If the digit is odd, the code adds 1 to it and then adds the result to
decoded_value
.
- It converts each
- After processing all digits, the code prints the final
decoded_value
.
number = int(input("Enter a number to decode: ")) new_number = number * 3 #Convert to string to access each digit new_number_str = str(new_number) decoded_value = 0 for digit in new_number_str: digit = int(digit) if digit % 2 == 0: # If the digit is even decoded_value += (digit - 1) else: # If the digit is odd decoded_value += (digit + 1) print(f"The decoded number is: {decoded_value}")
The Bank’s Compound Interest Calculation
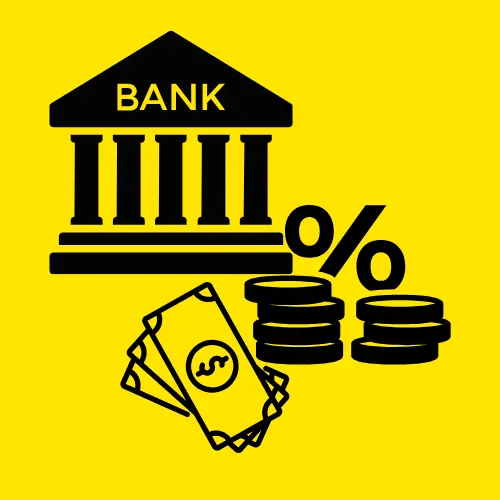
Imagine you’re a financial advisor helping a client calculate how their $1000 investment grows in a savings account with an annual interest rate of 5%, compounded annually. The client wants to know the value of their investment over the next 10 years. Using the compound interest formula A=P(1+100r)t, where P is the principal, r is the interest rate, and t is the time in years.
Write a Python program that uses a for loop to calculate the amount at the end of each year and prints the result. For example, after year 1, the amount will be the original $1000 increased by 5%, and this process continues until the 10th year.
Test Case:
Input:
Principal: $1000
Annual interest rate: 5%
Years: 10
Expected Output:
Year 1: Amount = $1050.00
Year 2: Amount = $1102.50
Year 3: Amount = $1157.63
Year 4: Amount = $1215.51
Year 5: Amount = $1276.28
Year 6: Amount = $1340.09
Year 7: Amount = $1407.09
Year 8: Amount = $1477.45
Year 9: Amount = $1551.32
Year 10: Amount = $1628.88
Explanation:
This code calculates and displays the amount of money accumulated after each year with compound interest.
- The
principal
is set to 1000, therate
is set to 5%, and theyears
is set to 10. - A
for
loop runs from 1 toyears
(inclusive), where each iteration represents a year. - Inside the loop, the code calculates the
amount
using the compound interest formula:- It multiplies the
principal
by(1 + rate / 100) ** year
, whererate / 100
is the annual interest rate, and** year
raises it to the power of the current year to account for compounding.
- It multiplies the
- The code then prints the year and the accumulated amount, formatting it to two decimal places.
principal = 1000 rate = 5 # Annual interest rate in percentage years = 10 for year in range(1, years + 1): amount = principal * (1 + rate / 100) ** year # Compound interest formula print(f"Year {year}: Amount = ${amount:.2f}")
Electricity Bill Calculation
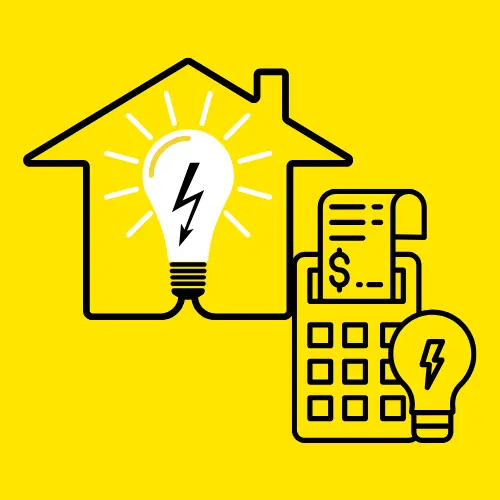
Imagine you’re managing the electricity billing for several households in your neighborhood. Each month, a household reports how much electricity they’ve consumed, and you need to calculate their electricity bill. The first 100 kWh of electricity is charged at $0.10 per kWh, and any usage beyond 100 kWh is charged at $0.15 per kWh.
Your task is to calculate and print the electricity bill for each household based on their monthly consumption.
Test Case:
Input:
Enter household 1 electricity consumption (kWh): 120
Enter household 2 electricity consumption (kWh): 80
Enter household 3 electricity consumption (kWh): 150
Enter household 4 electricity consumption (kWh): 90
Enter household 5 electricity consumption (kWh): 200
Expected Output:
Household 1: Consumption = 120 kWh, Bill = $13.00
Household 2: Consumption = 80 kWh, Bill = $8.00
Household 3: Consumption = 150 kWh, Bill = $16.50
Household 4: Consumption = 90 kWh, Bill = $9.00
Household 5: Consumption = 200 kWh, Bill = $23.00
Explanation:
This code calculates the electricity bill for multiple households based on their consumption and a tiered pricing system.
- The rates for the first 100 kWh and above 100 kWh are set as
rate_1
(0.10) andrate_2
(0.15), respectively. The number of households is set to 5, withnum_households = 5
. - The
for
loop iterates over each household from 1 tonum_households
. - For each household, the code prompts the user to input their electricity consumption (
consumption
), measured in kWh. - If the consumption is less than or equal to 100 kWh, the bill is calculated by multiplying
consumption
byrate_1
. - However, if the consumption exceeds 100 kWh, the code incorrectly calculates the bill by adding two parts: the first 100 kWh at
rate_1
and any additional consumption atrate_2
. - After the calculation, the code prints the consumption and the bill for the household, formatted to two decimal places.
rate_1 = 0.10 # Rate for first 100 kWh rate_2 = 0.15 # Rate for above 100 kWh num_households = 5 for household in range(1, num_households + 1): consumption = int(input(f"Enter household {household} electricity consumption (kWh): ")) if consumption <= 100: bill = consumption * rate_1 bill = (100 * rate_1) + ((consumption - 100) * rate_2) print(f"Household {household}: Consumption = {consumption} kWh, Bill = ${bill:.2f}")
The Squid Game Round
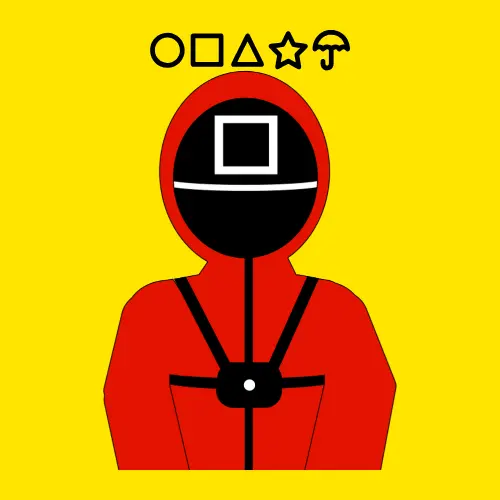
Imagine you’re a contestant in a tough round of Squid Game. In this game, you start with a number, and in each round, the number changes. Sometimes, it gets bigger by multiplying by 2, or it gets bigger by adding a certain value. If the number ever has the digit “7” in it, you lose and the game ends. But if the number is divisible by 4, you get a second chance, and the number will be cut in half. Your job is to see how many rounds you can survive before the number breaks the rules, and count the total rounds it takes for the game to end. Write a Python program that simulates the game and tells you if you survive each round or not.
Test Case:
Input:
Enter the starting number: 5
Enter the number of rounds: 6
Expected Output:
Round 1: You survive with the number 8.
Round 2: The number 16 was divisible by 4, halved!
Round 3: You survive with the number 13.
Round 4: You survive with the number 26.
Round 5: The number 52 was divisible by 4, halved!
Round 6: You survive with the number 20.
You survived 6 rounds before failing!
Explanation:
This code simulates a game where you start with a number and perform actions over several rounds.
- First, the code asks the user for the
initial_number
(starting number) androunds
(number of rounds to play). A variablecount_rounds
is initialized to keep track of the rounds you survive. - The
for
loop runs for each round, starting from 1 torounds
. In each round: - The code checks if the round number is even or odd.
- If it’s even, the
number
is doubled; if it’s odd, thenumber
is increased by 3. - The next step checks if the
number
contains the digit ‘7’. This is done by converting the number to a string usingstr(number)
. Thestr()
function allows us to treat the number as a string so that we can check if ‘7’ is in the string. If ‘7’ is found, the game ends. The message"You lose!"
is printed, and thebreak
statement is used to exit the loop, ending the game early. - If the
number
doesn’t contain ‘7’, the code checks if it’s divisible by 4. If it is, thenumber
is halved, and a message is shown. This is done using integer division (//
), which divides the number and keeps it as an integer. - If the
number
isn’t divisible by 4, a message is displayed saying you survive the round, and thecount_rounds
is incremented. - Finally, the code prints how many rounds you survived before losing.
initial_number = int(input("Enter the starting number: ")) rounds = int(input("Enter the number of rounds: ")) count_rounds = 0 for round_num in range(1, rounds + 1): number = initial_number if round_num % 2 == 0: number *= 2 else: number += 3 # Check if the number contains '7' if '7' in str(number): print(f"Round {round_num}: The number {number} contains '7'. You lose!") break #the game ends # Check if the number is divisible by 4 if number % 4 == 0: number = number // 2 print(f"Round {round_num}: The number {number} was divisible by 4, halved!") else: print(f"Round {round_num}: You survive with the number {number}.") count_rounds += 1 # Increment rounds survived print(f"\nYou survived {count_rounds} rounds before failing!")
For more Python topics, solutions to common problems, and scenario-based questions on various Python concepts, visit Python at Syntax Scenarios.