Understand the Python error “EOL while scanning string literal” and learn all the possible ways to fix this error.
EOL While Scanning String Literal in Python – A Real-world Analogy
Imagine a train running smoothly on its railway track, but suddenly, the track ends without reaching a station. The train has to stop right there, leaving the passengers confused. This is similar to what happens in Python when you get the syntaxerror: EOL while scanning string literal.
Python is reading your code, but if it doesn’t find the proper ending, like a closing quotation mark, or encounters a line break, it gets confused and stops, just like the train on the unfinished track.
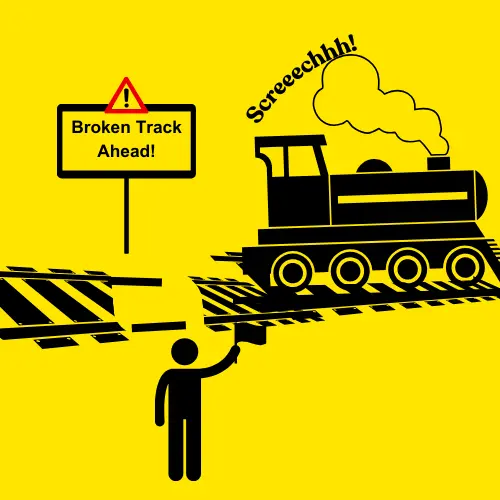
“EOL” stands for “End of Line” in Python. The error SyntaxError: EOL while scanning string literal
happens when Python reaches the end of a line but expects more to finish a string. It’s like starting a sentence and stopping before finishing it. When you see this error, it means there’s an unfinished string in your code that needs fixing.
How to Avoid the EOL Error in Python?
Here are some quick simple tips to avoid the EOL error:
- Check Your Quotes: Make sure every string starts and ends with matching quotes.
- Handle Escape Sequences Properly: Use double backslashes or raw strings for file paths.
- Use Triple Quotes for Long Strings That Span Multiple Lines: Using triple quotes makes it easy for Python interpreter to know in advance that the string will span multiple lines.
- Avoid Extra Spaces: Ensure there are no unwanted spaces or tabs in your string.
Common Causes And Solutions of the “EOL Error” in Python
Let’s discuss the common causes that lead to the Python EOL error along with their solutions explained with real-world examples and visuals.
Cause 1: Using Escape Characters Incorrectly
In Python, special characters like \n
(newline) or \t
(tab) have specific meanings. Misusing them confuses the Python Interpreter which results in Python EOL errors in your code.
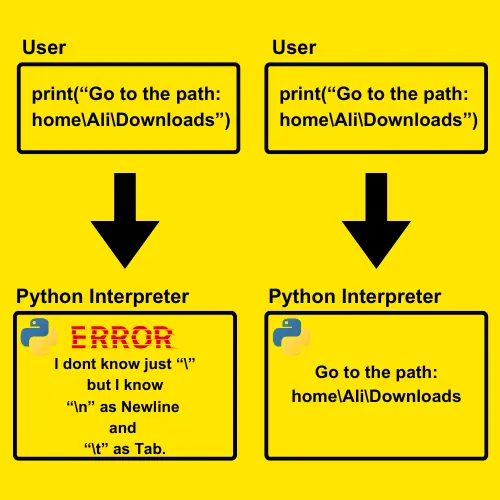
Example
In the code file_path = "C:\Users\Ahmad\Documents"
, the backslashes are supposed to be part of the file path. This causes an “EOL while scanning string literal
” error because Python misinterprets the backslashes as special characters, expecting more characters to complete the string.
# Defining a string variable file_path = "C:\Users\Ahmad\Documents" print(file_path)
Output:
SyntaxError: EOL while scanning string literal
Solution: Use Backslashes or Raw String
To fix this, use double backslashes (\\
) or a raw string (r"..."
), like this:
# using double backslashes file_path = "C:\\Users\\Ahmad\\Documents" print(f’The file path is: {file_path}’)
Output:
The file path is: C:\Users\John\Documents
OR
# using raw string file_path = r"C:\Users\Ahmad\Documents" print(f’The file path is: {file_path}’)
Output:
The file path is: C:\Users\John\Documents
Cause 2: Missing Closing Quotes
Missing closing quotes often cause the python SyntaxError: EOL while scanning string literal
. It’s like having a bike with one tire missing which shows it isn’t ready to use. In Python, starting a string with a quote but not closing it with the same quote leaves the code incomplete, causing errors.
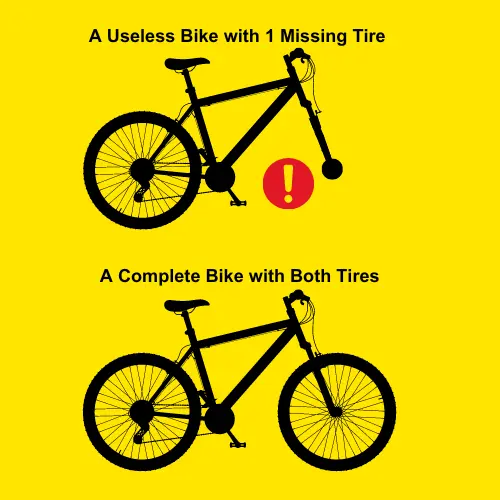
Example
The code starts a string variable with a quote but doesn’t end it, which causes an EOL error. Python can’t understand the string because it’s incomplete.
# Defining a variable with a string # Forget to close the quote message = "I am wearing my new shoes # Dislplaying the variable print(message)
Output
SyntaxError: EOL while scanning string literal
Solution: Check the Quotes of a String
Ensure that every string you create starts and ends with the same type of quote. If you start with a single quote ('
), make sure it ends with it too, and the same for double quotes ("
).
# Defining a variable with a string message = "I am wearing my new shoes" # Corrected String with finished quotes # Displaying the variable print(message)
Output
I am wearing my new shoes
Cause 3: Using the Wrong Type of Quotes
Starting a string with a double quote (“) and ending it with a single quote (‘) or the other way around will cause a Python error. This is similar to wearing a sandal on one foot and a Loafer on the other; it just doesn’t fit together properly!
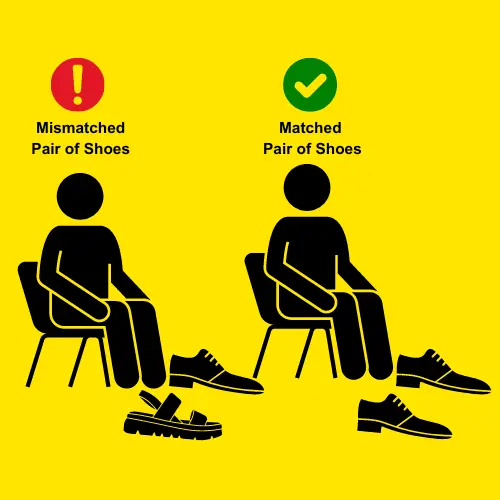
Example
The code uses mismatched quotes ("
and '
) to start and end the string, which causes a Python EOL error. Python can’t interpret the string correctly due to the incorrect quote pairing.
# Defining a variable with a string message = "I am wearing my new shoes’ # using mismatched quotes # Displaying the variable print(message)
Output
SyntaxError: EOL while scanning string literal
Solution: Use Matched Quotes
To fix this error, use the same type of quote at the beginning and end of the string. Starting a string with a double quote (“) must end it with a double quote. Similarly, starting with a single quote ('
) and ending with a single quote. This way, your string will be complete and you won’t get the SyntaxError: EOL while scanning string literal
.
# Defining a variable with a string # Double quotes message = "I am wearing my new shoes" # Displaying the variable print(message)
Output
I am wearing my new shoes
OR
# Single quotes message = 'I am wearing my new shoes' # Displaying the variable print(message)
Output
I am wearing my new shoes
Cause 4: Line Breaks Within a String
If you press ‘Enter‘ in the middle of a string without proper handling, Python will show an EOL Error. Imagine sending a text message to your friend and hitting ‘Enter‘ by mistake, splitting your sentence into random lines. This will create confusion for your friend in understanding the message because it’s broken up. In the same way, line breaks in a Python string make it hard for the code to work right.
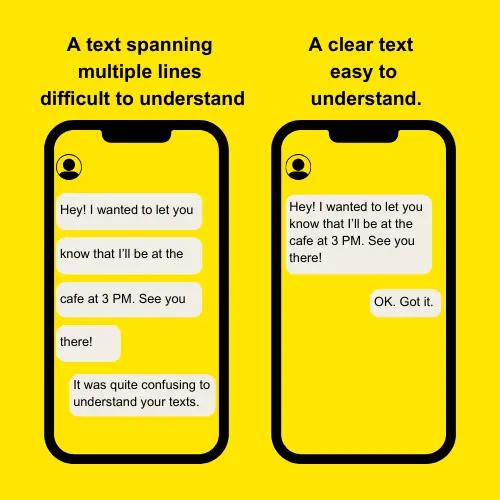
Example
The line break inside the string within the code causes a Python EOL error. It’s like sending a text message that is split up, making it hard for the Python Interpreter to understand it.
# Defining a variable with a string message = "This is a long message that covers two lines" # Printing the variable print(message)
Output:
SyntaxError: EOL while scanning string literal
Solution: Escape Line Breaks or Use Triple Quotes
If your string is long and spans multiple lines, you can either use triple quotes ('''
or """
) to enclose the string or escape the line break with a backslash (\
).
message = """This is a long message that covers two lines""" # Uisng triple quotes print(message)
Output:
This is a long message that covers two lines
OR
message = "This is a long message \ that covers two lines" # Using Escape line print(message)
Output:
This is a long message that covers two lines
Conclusion
Facing errors is part of the coding journey, they are like speed bumps on the road to mastering code. They slow us down but also sharpen our skills. The “EOL While Scanning” error is like missing punctuation or signs in everyday writing. It might seem confusing at first, but the solution is usually simple. By carefully checking your string literals, quotes, and escape sequences, you’ll quickly learn how to fix this error. With practice, it will become easy to handle.