Learning the basics is one thing, but applying those concepts practically is where the real challenge lies. After getting hands-on experience with if-else, nested if-else, and loops in Python, it’s time to take the next step and level up your game. Let’s tackle some real-world, easy-to-understand, and interesting coding problems with nested loops in Python and put your skills to the test here at Syntax Scenarios.
Note: You can view the answer code and its explanation by clicking on the question (or the drop-down icon).
Chessboard Pattern
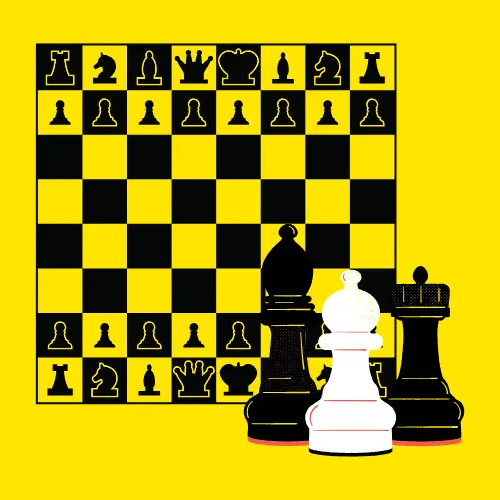
Imagine setting up a chessboard for a game, but this time you need to create it digitally using Python. The board will have alternating black (#) and white (.) squares, and you want to customize the number of rows and columns. You can ask the user for the size of the chessboard and then use Python to print the pattern with alternating squares in each row.
How can you print a chessboard pattern using nested loops in Python where the user specifies the number of rows and columns, and each row alternates between # and .?
Test Case:
Input:
rows= 8
cols= 8
Expected Output:
# . # . # . # .
. # . # . # . #
# . # . # . # .
. # . # . # . #
# . # . # . # .
. # . # . # . #
# . # . # . # .
. # . # . # . #
Explanation:
The code uses nested loops to print a chessboard pattern based on user input for rows and columns.
- First, it asks the user for the number of rows and columns, storing them in
rows
andcols
, respectively, as integers usingint()
. - The outer
for
loop iterates over each row, runningrows
times. Each time the loop runs, it represents one row of the pattern. - Inside the outer loop, there is another inner loop (the nested loop) that runs for each column in the current row, iterating
cols
times. This means that for each row, the inner loop controls how many times the pattern will be printed horizontally. - In the inner loop:
- The code checks if the sum of the current row and column numbers (
row + col
) is even or odd using the condition(row + col) % 2 == 0
.- If the sum is even, it prints a
#
symbol. - If the sum is odd, it prints a
.
symbol.
- If the sum is even, it prints a
- The
print()
statement hasend=" "
to avoid starting a new line after each symbol. This keeps the symbols in the same row until the inner loop finishes.
- The code checks if the sum of the current row and column numbers (
- After completing one row (when the inner loop finishes), the
print()
statement without any arguments moves to the next line, so the next row begins from a new line. - The process repeats until all rows and columns are printed, creating a chessboard-like pattern.
rows = int(input("Enter the number of rows: ")) cols = int(input("Enter the number of columns: ")) for row in range(rows): for col in range(cols): if (row + col) % 2 == 0: print("#", end=" ") else: print(".", end=" ") print() # Move to next row
Parking Lot Management
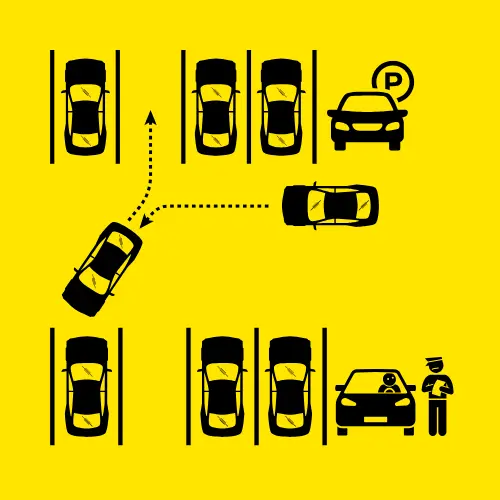
Imagine you’re managing a parking lot with multiple sections. Each section has several parking spots. Some sections are for compact cars and others are for regular cars. You need to check whether a car can park in a specific section based on its size. A compact car can only park in compact spots, but a regular car can park in both compact and regular spots. You need to check multiple sections and spots in a loop to determine if a car can park in any available spot.
Develop a Python code for this scenario.
Test Case:
Input:
Number of sections= 2
Number of spots= 6
car size (compact/regular)= compact
Expected Output:
Checking Section 1…
Compact car can park in Spot 1 of Section 1.
Compact car can park in Spot 2 of Section 1.
Compact car can park in Spot 3 of Section 1.
Compact car can park in Spot 4 of Section 1.
Checking Section 2…
Compact car can park in Spot 1 of Section 2.
Compact car can park in Spot 2 of Section 2.
Compact car can park in Spot 3 of Section 2.
Compact car can park in Spot 4 of Section 2.
Explanation:
This code helps to check where compact and regular cars can park in a parking lot with multiple sections and spots.
- The code first asks how many sections (
num_sections
) and spots (num_spots
) are in the parking lot, and what size the car is (car_size
). - It uses the
.lower()
function to make sure the car size is checked in lowercase, regardless of how the user enters it. - The outer
while
loop starts withsection = 1
and repeats for each section of the parking lot. - Inside this loop, another
while
loop starts withspot = 1
and checks each parking spot in the current section. - If the car is compact, the code checks if the spot is in the first half of the section (
num_spots // 2
). Compact cars can park only there. - The code allows regular cars to park in any spot, as regular cars can park anywhere.
- After checking each spot, the code moves to the next spot using
spot += 1
. - Once all spots in a section are checked, the code moves to the next section by increasing the
section
number withsection += 1
. - This process continues until all sections and spots are checked, showing where each car size can park.
num_sections = int(input("Enter the number of sections in the parking lot: ")) num_spots = int(input("Enter the number of spots in each section: ")) car_size = input("Enter the car size (compact/regular): ").lower() section = 1 while section <= num_sections: print(f"Checking Section {section}...") spot = 1 while spot <= num_spots: # Iterate through each spot in the section if car_size == "compact" and spot <= num_spots // 2: # Compact cars can only park in half of the spots print(f"Compact car can park in Spot {spot} of Section {section}.") elif car_size == "regular" and (spot <= num_spots // 2 or spot > num_spots // 2): # Regular cars can park in any spot print(f"Regular car can park in Spot {spot} of Section {section}.") spot += 1 # Move to the next spot section += 1 # Move to the next section
Pirate Treasure Hunt
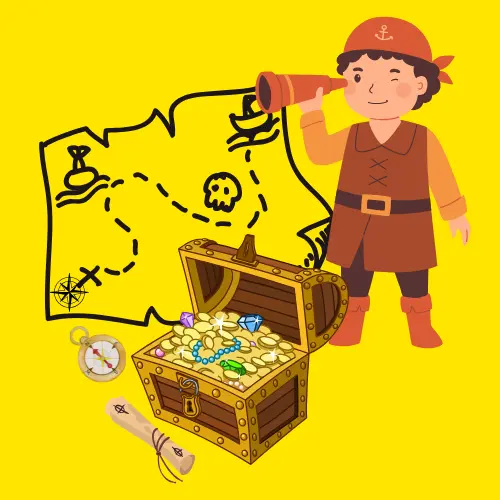
You’re the captain of a pirate ship, leading your crew on a treasure hunt. The treasure is hidden somewhere in a 4×5 grid of sand. To make it exciting, ask a crew member to secretly pick a row and column for the treasure’s location. Now, you need to check every spot in the grid. As you search, if you find the treasure at the chosen spot, print “Found treasure!” For all other spots, print “Try again.”
How can you write a Python program using nested loops to search the grid and find the treasure based on the user’s input for its location?
Test Case:
Input:
Treasure row (1-4)= 3
Treasure column (1-5)= 2
Expected Output :
Try again.
Try again.
Try again.
Try again.
Try again.
Try again.
Try again.
Try again.
Try again.
Try again.
Found treasure!
Try again.
Try again.
Try again.
Try again.
Explanation:
This code simulates a grid-based treasure hunt where a player searches for a treasure at specific coordinates.
- The code first asks the user to input the
treasure_row
andtreasure_col
to specify where the treasure is hidden on the grid. - It then defines the grid dimensions using
grid_rows
andgrid_cols
, set to 4 rows and 5 columns. - Using nested loops:
- The outer loop goes through each row (
row
) in the grid, from 1 to 4. - For each row, the inner loop iterates through each column (
col
), from 1 to 5.
- The outer loop goes through each row (
- Inside the loops:
- The
if
condition checks if the currentrow
andcol
match thetreasure_row
andtreasure_col
provided by the user. - If a match is found, it prints “Found treasure!” to indicate the treasure is located at that position.
- Otherwise, it prints “Try again.” for all other grid positions.
- The
- This continues until the entire grid is checked, ensuring the treasure can be found if the user provides valid coordinates.
treasure_row = int(input("Enter the treasure row (1-4): ")) treasure_col = int(input("Enter the treasure column (1-5): ")) grid_rows, grid_cols = 4, 5 for row in range(1, grid_rows + 1): # Loop through each row for col in range(1, grid_cols + 1): # Loop through each column if row == treasure_row and col == treasure_col: print("Found treasure!") else: print("Try again.")
Prime Numbers Grid Puzzle
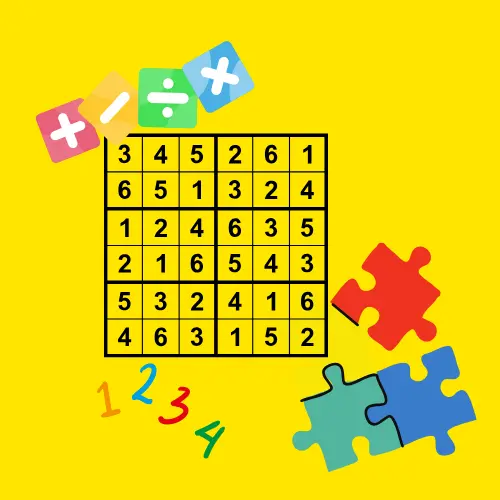
Imagine you’re designing a math puzzle for students, where they need to find prime numbers in a grid. The grid contains numbers generated by multiplying the row and column indices, and you want to highlight only the prime numbers. To make it easier to visualize, any number that isn’t prime will be replaced with a dash (-). The goal is to programmatically create this grid and display it so students can spot the prime numbers.
How can you use nested loops in Python to generate this grid, check if each number is prime, and print the grid where prime numbers remain, and non-prime numbers are replaced with a dash (-)?
Test Case:
Input:
Rows= 4
Columns= 4
Expected Output:
– – – –
– 2 3 –
– 3 – –
– – – –
Explanation:
This code creates a grid and checks whether each number in the grid is prime or not.
- The code first asks the user to input the number of
rows
andcols
to define the size of the grid. - Then, two nested
for
loops are used:- The outer loop goes through each
row
from 1 to the total number of rows (rows
). - The inner loop goes through each
col
from 1 to the total number of columns (cols
).
- The outer loop goes through each
- For each cell in the grid, the number at that position is calculated by multiplying the
row
andcol
values, storing it in the variablenum
. - Then, the code checks if
num
is a prime number:- If
num
is less than 2, it cannot be prime, so the variableis_prime
is set toFalse
. - Otherwise, the code checks if
num
is divisible by any number between 2 and the square root ofnum
. If it finds a factor, it setsis_prime
toFalse
and breaks the loop.
- If
- If
is_prime
isTrue
, the number is printed. Otherwise, a hyphen (“-“) is printed to represent a non-prime number. - After each row is processed, the code moves to the next row using
print()
.
rows = int(input("Enter the number of rows: ")) cols = int(input("Enter the number of columns: ")) for row in range(1, rows + 1): for col in range(1, cols + 1): num = row * col # Calculate the number in the grid is_prime = True if num < 2: # Numbers less than 2 are not prime is_prime = False for i in range(2, int(num ** 0.5) + 1): # Check for factors if num % i == 0: is_prime = False break print(f"{num}" if is_prime else "-", end=" ") print() # Move to the next row
Student Grading System
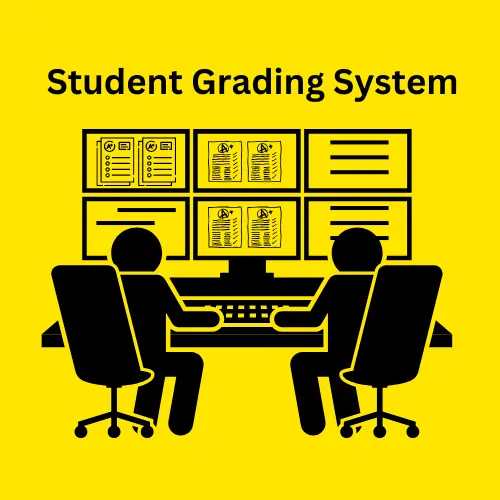
Suppose you’re building a grading system for a class of students. Each student has marks for multiple subjects, and based on their average score, you need to assign them a grade. If their average score is 80 or more, they get an “A”, between 60 and 79 they get a “B”, and below 60 means they get a “C”. You want the system to ask for each student’s marks, calculate their average score, and then display the grade accordingly.
Develop a Python script to calculate the average score for each student, assign grades based on the average, and display the results.
Test Case:
Input:
Number of students= 3
For each student, input their subject scores:
For Student 1:
Enter the number of subjects for Student 1: 3
Enter score for Subject 1: 85
Enter score for Subject 2: 90
Enter score for Subject 3: 88
For Student 2:
Enter the number of subjects for Student 2: 3
Enter score for Subject 1: 75
Enter score for Subject 2: 80
Enter score for Subject 3: 70
For Student 3:
Enter the number of subjects for Student 3: 3
Enter score for Subject 1: 55
Enter score for Subject 2: 60
Enter score for Subject 3: 58
Expected Output:
Student 1: Average Score = 87.67, Grade = A
Student 2: Average Score = 75.00, Grade = B
Student 3: Average Score = 57.67, Grade = C
Explanation:
This code calculates the average score for each student and assigns a grade based on the average score.
- First, the code asks for the number of
students
and stores it in the variablenum_students
. - The
for
loop runs once for each student, from 1 to the total number of students.- Inside the loop, it initializes
total_score
to 0 for each student to keep track of their total score. - Then, the code asks how many
subjects
the student has and stores it innum_subjects
. - Another
for
loop runs for each subject, asking for the score and adding it tototal_score
.
- Inside the loop, it initializes
- After collecting all the scores, the code calculates the
avg_score
by dividingtotal_score
by the number of subjects (num_subjects
). - Based on the average score, it assigns a grade:
- If the average score is 80 or higher, the grade is “A”.
- If it’s between 60 and 79, the grade is “B”.
- Otherwise, the grade is “C”.
- Finally, the code prints the student’s average score and their assigned grade.
num_students = int(input("Enter the number of students: ")) for student in range(1, num_students + 1): total_score = 0 num_subjects = int(input(f"Enter the number of subjects for Student {student}: ")) for subject in range(1, num_subjects + 1): score = int(input(f"Enter score for Subject {subject}: ")) total_score += score avg_score = total_score / num_subjects if avg_score >= 80: grade = "A" elif avg_score >= 60: grade = "B" else: grade = "C" print(f"Student {student}: Average Score = {avg_score:.2f}, Grade = {grade}")
Talent Show Pyramid
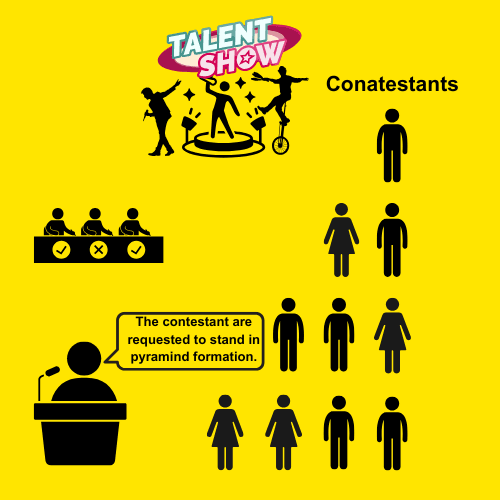
Imagine you’re organizing a talent show, and participants need to stand in a pyramid formation for their final performance. The first row will have 1 person, the second row will have 2 people, the third row will have 3, and so on, forming a pyramid shape. To plan this, you decide to create a program that prints a pyramid of numbers, where each row represents the number of people in that row. The user will specify how many rows the pyramid should have.
Create a Python script to print this pyramid of numbers based on the user’s input for the number of rows?
Test Case:
Input:
Rows= 5
Expected Output:
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
Explanation:
This code generates a number pyramid where each row displays an increasing sequence of numbers, starting from 1.
- The code first asks for the number of
rows
in the pyramid and stores it in the variablerows
. - A
for
loop runs from 1 to the total number of rows (rows + 1
). Each iteration corresponds to a row in the pyramid.- Inside the first loop, another
for
loop runs from 1 to the current row number (row + 1
). This loop generates the numbers for that row. - The
print(num, end=" ")
statement displays the current number in the sequence, keeping it on the same line with a space in between.
- Inside the first loop, another
- After printing all the numbers for a row, the outer loop executes a
print()
statement to move to the next line, creating the pyramid structure.
rows = int(input("Enter the number of rows: ")) for row in range(1, rows + 1): for num in range(1, row + 1): print(num, end=" ") print()
Vending Machine
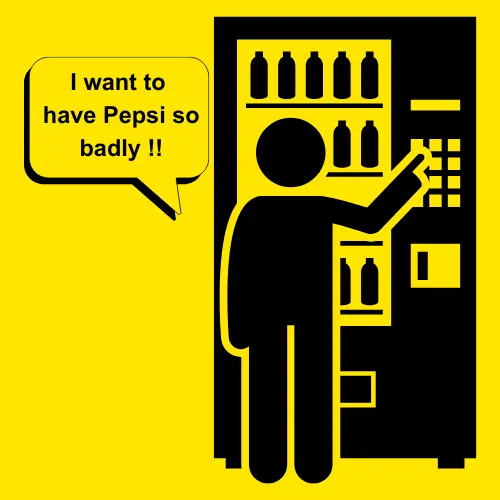
A vending machine that sells drinks for $1. It accepts coins of 25 cents, 10 cents, and 5 cents. The user can insert any combination of coins. The machine should keep accepting coins until the total amount inserted is greater than or equal to $1.
Develop a Python code to mimic this scenario.
Test Cases:
Input:
Coins = 25 cents
Coins = 25 cents
Coins = 10 cents
Coins = 10 cents
Coins = 5 cents
Expected Output:
Enjoy your drink!
Explanation:
This code simulates a vending machine that accepts coins until the total amount reaches or exceeds the price of a drink.
- The
total_amount
is initialized to 0, representing the money inserted by the user. - The
drink_price
is set to 100 cents (equivalent to $1), which is the cost of the drink. - A
while
loop runs as long astotal_amount
is less thandrink_price
. This ensures the loop continues until the user inserts enough money. - Inside the loop:
- The user is prompted to insert a coin by choosing from three options:
1
for 25 cents,2
for 10 cents, and3
for 5 cents. - If the user enters an invalid option (anything other than 1, 2, or 3), a nested
while
loop asks the user to try again until a valid option is chosen. - Depending on the user’s choice:
- If
coin_option
is1
, 25 cents are added tototal_amount
. - If
coin_option
is2
, 10 cents are added. - If
coin_option
is3
, 5 cents are added.
- If
- The user is prompted to insert a coin by choosing from three options:
- Once the
total_amount
equals or exceedsdrink_price
, the loop ends. - After the loop:
- If the total amount is sufficient, the message
"Enjoy your drink!"
is displayed. - Otherwise, the message
"Please insert more coins."
is displayed (though this case will not occur due to the loop’s condition).
- If the total amount is sufficient, the message
total_amount = 0 drink_price = 100 # Price in cents while total_amount < drink_price: coin_option = int(input("Insert a coin (1: 25 cents, 2: 10 cents, 3: 5 cents): ")) while (coin_option != 1) and (coin_option != 2) and (coin_option != 3): print("Invalid coin option. Please choose 1, 2, or 3.") coin_option = int(input("Insert a coin (1: 25 cents, 2: 10 cents, 3: 5 cents): ")) if coin_option == 1: total_amount += 25 elif coin_option == 2: total_amount += 10 else: total_amount += 5 if total_amount >= drink_price: print("Enjoy your drink!") else: print("Please insert more coins.")
Countdown for Workout Session
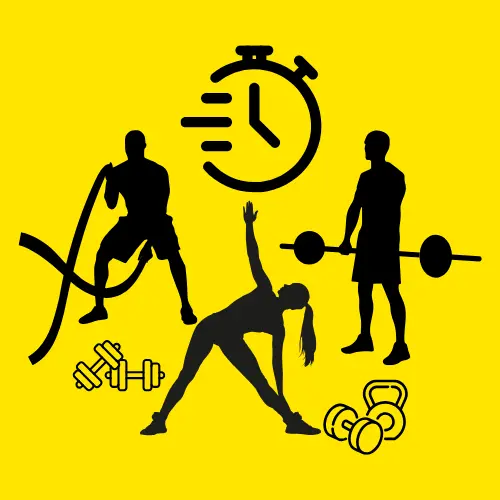
Imagine you’re organizing a workout session and need a timer to keep track of intervals. Each exercise has a set duration in minutes and seconds, and you want a program to display the countdown in real time. The timer should show the remaining time in minutes and seconds, and once it reaches zero, it should display “Time’s up!” to signal the end of the interval.
How can you create a countdown timer in Python that lets the user input the minutes and seconds, and displays the countdown until the time runs out?
Test Case
Input:
Minutes: 0
Seconds: 10
Expected Output:
00:10
00:09
00:08
00:07
00:06
00:05
00:04
00:03
00:02
00:01
Time’s up!
Explanation:
The code is a countdown timer that counts down from a specified number of minutes and seconds to zero.
- First the user enters the timer’s duration in minutes (
minutes
) and seconds (seconds
) usinginput()
, and these values are converted to integers withint()
. - Then a
while
loop runs as long as there are any minutes or seconds left (minutes > 0 or seconds > 0
). - Inside the loop, if
seconds > 0
, it displays the remaining time in theMM:SS
format usingprint(f"{minutes:02d}:{seconds:02d}")
, ensuring two-digit formatting. - The program pauses for 1 second using
time.sleep(1)
(from thetime
library which is used here to add a one-second pause between each countdown step, making the timer function realistically.) and decreasesseconds
by 1. - When seconds reach
0
and minutes remain,minutes
decreases by 1, andseconds
resets to59
to start counting down the new minute. - Once both minutes and seconds reach
0
, the program ends and displays"Time's up!"
.
import time # Input the duration of the timer in minutes and seconds minutes = int(input("Enter minutes: ")) seconds = int(input("Enter seconds: ")) while minutes > 0 or seconds > 0: # Outer loop runs until the timer reaches 0 while seconds > 0: # Inner loop counts down the seconds print(f"{minutes:02d}:{seconds:02d}") # Display the time in MM:SS format time.sleep(1) # Wait for 1 second seconds -= 1 # Decrease seconds by 1 if minutes > 0: # When seconds reach 0 and minutes are remaining minutes -= 1 # Decrease minutes by 1 seconds = 59 # Reset seconds to 59 for the new minute print("Time's up!")
Auto-Driving Cars and Traffic Signals
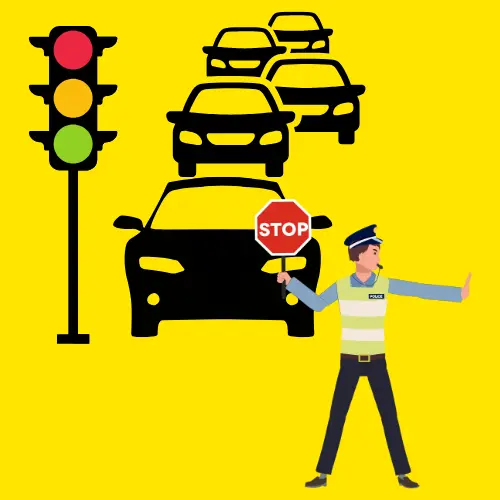
Suppose you are developing a system for an autonomous car to navigate through a grid of streets. Each street has a traffic signal at the intersections, which can be red, yellow, or green. The car needs to check each intersection in its path. The car can only move forward when the signal is green. If it’s yellow, the car waits for the light to turn green, and if it’s red, the car must stop and turn back.
Your program has to simulate the car’s journey through the grid using nested loops.
Test Case:
Input:
Rows= 1
Columns= 5
Enter signals for row 1 (use ‘red’, ‘yellow’, ‘green’, separated by spaces):
green green green green green
Output:
Car’s journey through the grid:
Intersection (1, 1) has a GREEN signal.
Car moves forward.
Intersection (1, 2) has a GREEN signal.
Car moves forward.
Intersection (1, 3) has a GREEN signal.
Car moves forward.
Intersection (1, 4) has a GREEN signal.
Car moves forward.
Intersection (1, 5) has a GREEN signal.
Car moves forward.
Explanation:
The code simulates a car’s journey through a grid of intersections, where each intersection has a traffic signal (red
, yellow
, or green
), and the car’s movement depends on the signals.
- The user is prompted to input the number of rows (
rows
) and columns (columns
) for the grid. These values define the grid size. - A message
"Car's journey through the grid:"
is displayed to indicate the start of the simulation. - For each row in the grid (
for i in range(1, rows + 1)
), the user provides the traffic signals for that row as a space-separated string (e.g.,"green red yellow"
). These signals are stored as a list using thesplit()
method. - Next, the program iterates through each column in the current row (
for j in range(1, columns + 1)
), processing one signal at a time.- The signal for the current intersection (
signal = row_signals[j - 1]
) is retrieved, and the intersection is identified by its coordinates (i
,j
). - The signal is displayed in uppercase for clarity using
signal.upper()
. - Based on the signal:
- If the signal is
"green"
, the car moves forward, and a corresponding message is printed. - If the signal is
"yellow"
, the car waits at the intersection, and this is communicated to the user. - If the signal is
"red"
, the car stops and turns back. In this case, thebreak
statement exits the inner loop (columns), and the outer loop (rows) also terminates due to the nested structure.
- If the signal is
- The signal for the current intersection (
- The
else
block after the inner loop ensures the car continues to the next row only if nored
signal is encountered. However, encountering ared
signal stops the journey entirely.
rows = int(input("Enter the number of rows in the grid: ")) columns = int(input("Enter the number of columns in the grid: ")) print("\nCar's journey through the grid:") for i in range(1, rows + 1): print(f"Enter signals for row {i} (use 'red', 'yellow', 'green', separated by spaces):") row_signals = input().split() for j in range(1, columns + 1): # Loop through each column in the current row signal = row_signals[j - 1] # Access the corresponding signal print(f"Intersection ({i}, {j}) has a {signal.upper()} signal.") if signal == "green": print("Car moves forward.") elif signal == "yellow": print("Car waits at the intersection.") elif signal == "red": print("Car stops and turns back.") break else: continue break
PUBG Loot Collection
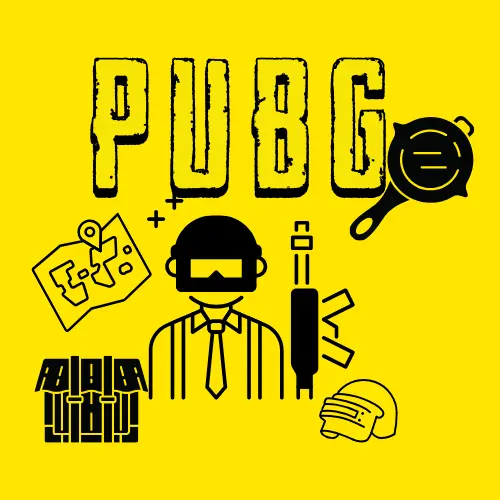
Write a program that calculates the BMI and prints the result in float (up to 3 decimal places) on the screen. Formula: BMI = kg/m²Imagine you are designing a simple simulation for PUBG loot collection in different buildings of a game map. Each building has several floors, and each floor has specific loot items. Your job is to write a program that goes through each floor of each building to display the loot items.
The player can decide to take the loot or leave it. If they take the loot, it is added to their inventory.
Test Case
Input:
Number of Buildings= 2
Number of floors= 2
Expected Output:
Entering Building 1…
Enter loot item for Building 1, Floor 1: Gold
Loot found on Floor 1: Gold
Do you want to take this loot? (yes/no): yes
Gold added to inventory.
Enter loot item for Building 1, Floor 2: Silver
Loot found on Floor 2: Silver
Do you want to take this loot? (yes/no): no
Silver left behind.
Finished checking all floors in Building 1.
Entering Building 2…
Enter loot item for Building 2, Floor 1: Diamond
Loot found on Floor 1: Diamond
Do you want to take this loot? (yes/no): yes
Diamond added to inventory.
Enter loot item for Building 2, Floor 2: Ruby
Loot found on Floor 2: Ruby
Do you want to take this loot? (yes/no): no
Ruby left behind.
Finished checking all floors in Building 2.
Loot collection completed!
Explanation:
This code simulates collecting loot from different buildings and floors in a game.
- First, the code takes the number of buildings (
num_buildings
) and the number of floors per building (num_floors
) as input using theinput()
function. - Then, it prints a message to start the loot simulation.
- The code loops through each building using
for building in range(1, num_buildings + 1)
and prints a message when entering a building. - Inside each building loop, another loop runs through each floor of that building, using
for floor in range(1, num_floors + 1)
, and asks for a loot item found on that floor. - After collecting loot information, the program asks if the user wants to take the loot using
input("Do you want to take this loot? (yes/no): ").lower()
. theinput()
function is used for user input, and.lower()
ensures that the user’s response is in lowercase to avoid mismatches. - It then checks if the user answers “yes” and adds the loot to the inventory or leaves it behind.
- After finishing a building’s floors, it prints that the floors in the building are checked.
- Finally, after all buildings are processed, the code prints “Loot collection completed!”
num_buildings = int(input("Enter the number of buildings in the map: ")) num_floors = int(input("Enter the number of floors in each building: ")) print("\nLoot Simulation Begins:\n") print("Your inventory contains the following loot:") for building in range(1, num_buildings + 1): print(f"Entering Building {building}...") for floor in range(1, num_floors + 1): loot = input(f"Enter loot item for Building {building}, Floor {floor}: ") print(f"Loot found on Floor {floor}: {loot}") take_loot = input("Do you want to take this loot? (yes/no): ").lower() if take_loot == "yes": print(f"{loot} added to inventory.") else: print(f"{loot} left behind.") print(f"Finished checking all floors in Building {building}.\n") print("Loot collection completed!")
Robot Cleaning Robot
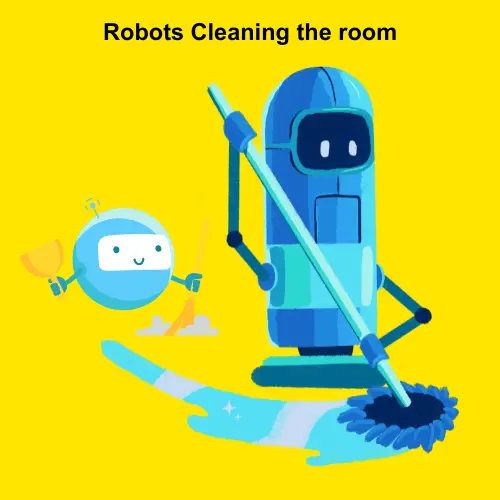
You are programming a robot to clean a large room, which is divided into a grid of smaller sections. Some sections are clean, while others are dirty. The robot moves from one section to the next, cleaning the dirty sections it encounters.
The robot stops if it encounters a clean section twice in a row, as it has finished cleaning.
Test Case
Input:
Rows= 2
Columns= 3
Enter the cleanliness of row 1 (use ‘dirty’ or ‘clean’, separated by spaces):
dirty
clean
dirty
Enter the cleanliness of row 2 (use ‘dirty’ or ‘clean’, separated by spaces):
clean
dirty
clean
Expected Output:
Robot encounters section (1, 1) which is dirty.
Cleaning the section…
Robot encounters section (1, 2) which is clean.
Section is already clean.
Robot encounters section (1, 3) which is dirty.
Cleaning the section…
Robot encounters section (2, 1) which is clean.
Section is already clean.
Robot stopped cleaning. All clean sections checked.
Explanation:
This code simulates a robot cleaning a room, checking the cleanliness of sections in a grid-like room, and stopping once two sections are clean.
- The code first takes the number of rows (
rows
) and columns (columns
) of the room as input. - The variable
clean_count
is initialized to 0 to keep track of how many sections are clean. - The outer
for
loop,for i in range(1, rows + 1)
, loops through each row of the room. - Inside this loop, it asks the user to input the cleanliness status for each section in the row (either “dirty” or “clean”).
- The inner
for
loop,for j in range(1, columns + 1)
, loops through each column in the current row, and checks each section’s cleanliness. - The code then checks if the section is “dirty”. If it is, it prints that the robot is cleaning the section, and it resets the
clean_count
to 0 (indicating the robot is cleaning a section). - If the section is already “clean”, it increments the
clean_count
by 1 and prints that the section is clean. - When
clean_count
reaches 2 (meaning two clean sections have been checked), it prints that the robot has stopped cleaning, and all clean sections are checked, then breaks out of the inner loop. - The
else
in the inner loop ensures the outer loop continues until the robot finishes cleaning. - The
break
in both loops ensures the robot stops as soon as two clean sections are checked.
rows = int(input("Enter the number of rows in the room: ")) columns = int(input("Enter the number of columns in the room: ")) clean_count = 0 for i in range(1, rows + 1): # Loop through each row print(f"Enter the cleanliness of row {i} (use 'dirty' or 'clean', separated by spaces):") for j in range(1, columns + 1): # Loop through each column in the current row section = input() # Input the cleanliness status of each section print(f"Robot encounters section ({i}, {j}) which is {section}.") if section == "dirty": print("Cleaning the section...") clean_count = 0 # Reset clean count when robot cleans else: clean_count += 1 print("Section is already clean.") if clean_count == 2: print("Robot stopped cleaning. All clean sections checked.") break else: continue break
A table in Mathematical Contest
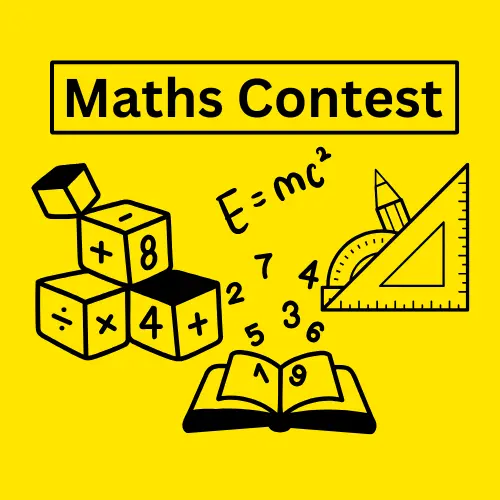
A mathematical contest asks participants to create a unique pattern using nested loops. The task is to take an integer input n
from the user and generate the following pattern, where each number in a row is the product of its row number and column number. However, the first number of every row should be aligned properly based on the maximum width of the last number in the table.
Test Case:
Input:
size of the table (n)= 4
Output:
1 2 3 4
2 4 6 8
3 6 9 12
4 8 12 16
Explanation:
This code generates and displays a multiplication table in a well-aligned format, where each number is padded with spaces to ensure all numbers have equal width for neat alignment.
- The code first takes an integer
n
as input, representing the size of the multiplication table (i.e., the table will haven
rows andn
columns). - The variable
max_number
is set ton * n
, which is the highest number in the table (the result of multiplyingn
by itself). - The variable
max_width
is initialized to 0. This will hold the maximum number of digits in any number of the table. - The
while temp > 0
loop is used to count how many digits are in themax_number
. It repeatedly dividestemp
by 10, increasingmax_width
by 1 each time, untiltemp
becomes 0. - After determining the width of the largest number, the program enters a nested
for
loop. The outer loop iterates through each row from1
ton
, and the inner loop iterates through each column from1
ton
. - Inside the inner loop, the product of the current row and column (
row * col
) is calculated and stored inproduct
. - The code then calculates the width (number of digits) of the current
product
using a similar process as before (looping through and dividing by 10). - To ensure the numbers align correctly, the code prints the necessary spaces. The number of spaces printed is equal to
max_width - width
, wherewidth
is the number of digits in the current product. - Finally, the product is printed, followed by a space. After printing all columns for a row,
print()
is called to move to the next line.
n = int(input("Enter the size of the table (n): ")) max_number = n * n max_width = 0 # Find the number of digits in max_number temp = max_number while temp > 0: max_width += 1 temp //= 10 for row in range(1, n + 1): for col in range(1, n + 1): product = row * col # Determine the number of digits in the current product width = 0 temp = product while temp > 0: width += 1 temp //= 10 for _ in range(max_width - width): print(" ", end="") print(product, end=" ") print()
Maze Exploration
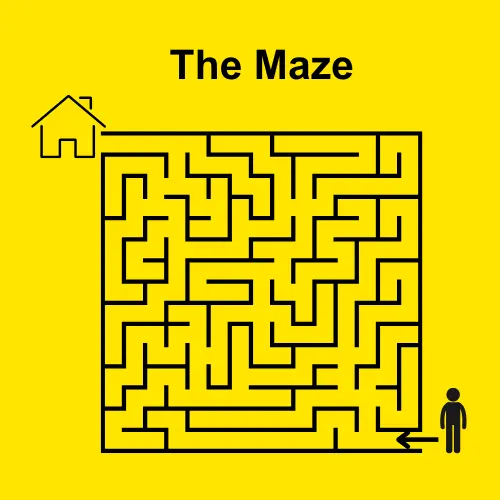
You are navigating through a maze, and you need to check whether each step in the maze is a valid path or a wall. You have a series of rooms in the maze, and you check each one by one. If the room is a wall, you can’t proceed; if it’s an open path, you move forward. Your goal is to track how many rooms you can pass through before encountering a wall.
Write a Python code for this scenario.
Test Case:
Input:
Rooms= 4
Is room 1 an open path or a wall? (Enter ‘open’ or ‘wall’): open
Is room 2 an open path or a wall? (Enter ‘open’ or ‘wall’): open
Is room 3 an open path or a wall? (Enter ‘open’ or ‘wall’): wall
Expected Output:
Room 1 is an open path. You can move forward.
Room 2 is an open path. You can move forward.
Room 3 is a wall. You can’t move forward.
Total open rooms passed: 2
Explanation:
This code simulates navigating through a maze, where the user encounters rooms that are either open paths or walls. The goal is to count how many open rooms the user passes until encountering a wall.
- The code starts by asking the user to input the number of rooms in the maze (
rooms
). - It initializes a variable
open_rooms_passed
to 0, which will keep track of the number of open rooms the user passes. - The
room_number
is set to 1, representing the first room in the maze. - The
while
loop runs as long asroom_number
is less than or equal torooms
, allowing the user to check each room in the maze. - Inside the loop, the user is asked to input the status of the current room (whether it is an open path or a wall). The input is converted to lowercase using
.lower()
to handle any case variations. - If the room is an open path,
open_rooms_passed
is incremented by 1, and a message is printed saying the room is an open path and the user can move forward. - If the room is a wall, the program prints a message saying the user cannot move forward and breaks out of the loop, stopping further room checks.
- After each iteration, the
room_number
is incremented by 1 to move to the next room. - Once the loop ends, the code prints the total number of open rooms the user passed (
open_rooms_passed
).
rooms = int(input("Enter the number of rooms in the maze: ")) open_rooms_passed = 0 room_number = 1 while room_number <= rooms: room_status = input(f"Is room {room_number} an open path or a wall? (Enter 'open' or 'wall'): ").lower() if room_status == "open": open_rooms_passed += 1 print(f"Room {room_number} is an open path. You can move forward.") elif room_status == "wall": print(f"Room {room_number} is a wall. You can't move forward.") break room_number += 1 print(f"Total open rooms passed: {open_rooms_passed}")
Animal Counting in a Zoo
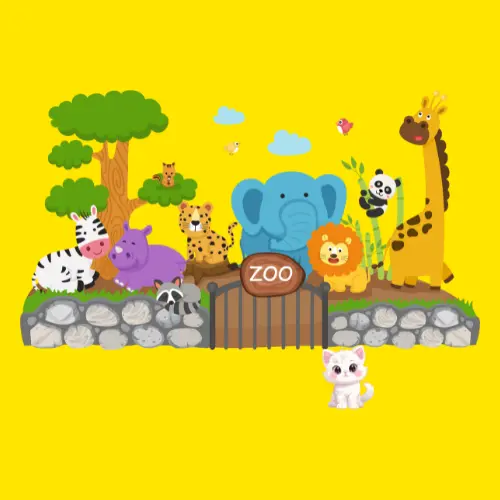
You are counting animals in a zoo that has multiple sections. Each section has a different number of animals. You need to count how many animals there are in total by checking each section. If a section has more animals, you need to count them one by one.
Develop a Python script for this scenario.
Test Case:
Input:
Enter the number of sections in the zoo: 3
Enter the number of different animal types in section 1: 2
Enter the number of animals of type 1 in section 1: 5
Enter the number of animals of type 2 in section 1: 3
Enter the number of different animal types in section 2: 1
Enter the number of animals of type 1 in section 2: 4
Enter the number of different animal types in section 3: 3
Enter the number of animals of type 1 in section 3: 6
Enter the number of animals of type 2 in section 3: 2
Enter the number of animals of type 3 in section 3: 1
Expected Output:
Counting 8 animals in section 1.
Counting 4 animals in section 2.
Counting 9 animals in section 3.
Total animals in the zoo: 21
Explanation:
This code calculates the total number of animals in a zoo, where the zoo is divided into several sections. For each section, it asks for the number of different animal types and the number of animals of each type.
- The user is first asked to input the number of sections in the zoo (
sections
). - The variable
total_animals
is initialized to 0. This will hold the total number of animals in the zoo. - A
for
loop starts withsection_number
ranging from 1 tosections
, representing each section of the zoo. - Inside the loop, the user is asked how many different animal types are in the current section (
types_of_animals
). - Then, for each animal type in that section, another
for
loop asks for the number of animals of that type (animals_of_this_type
). - The number of animals of that type is added to
animals_in_section
, which tracks the total number of animals in the current section. - After processing all animal types in the current section,
animals_in_section
is added tototal_animals
, which keeps track of the overall total number of animals in the zoo. - A message is printed to show the total number of animals counted in the current section.
- This process repeats for all sections in the zoo.
- Finally, the code prints the total number of animals in the zoo, stored in
total_animals
.
sections = int(input("Enter the number of sections in the zoo: ")) total_animals = 0 for section_number in range(1, sections + 1): animals_in_section = 0 types_of_animals = int(input(f"Enter the number of different animal types in section {section_number}: ")) for animal_type in range(1, types_of_animals + 1): animals_of_this_type = int(input(f"Enter the number of animals of type {animal_type} in section {section_number}: ")) animals_in_section += animals_of_this_type # Add to section total total_animals += animals_in_section # Add to overall total for the zoo print(f"Counting {animals_in_section} animals in section {section_number}.") print(f"Total animals in the zoo: {total_animals}")
Space Mission Progress Tracker
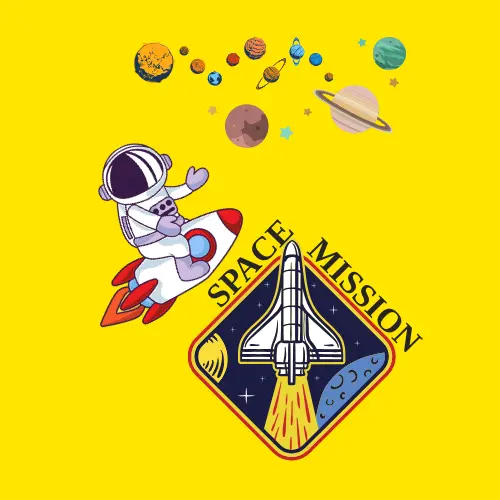
You’re managing a space mission, and you need to track the progress of astronauts completing various tasks across different mission phases. Each phase has several tasks that must be completed. You will go through each phase and check how many tasks have been completed in each phase through Python script.
Test Case:
Input:
Phases= 2
Enter the number of tasks in phase 1: 3
Is task 1 in phase 1 completed? (Enter ‘yes’ or ‘no’): yes
Is task 2 in phase 1 completed? (Enter ‘yes’ or ‘no’): no
Is task 3 in phase 1 completed? (Enter ‘yes’ or ‘no’): yes
Enter the number of tasks in phase 2: 2
Is task 1 in phase 2 completed? (Enter ‘yes’ or ‘no’): yes
Is task 2 in phase 2 completed? (Enter ‘yes’ or ‘no’): no
Expected Output:
Task 1 in phase 1 is completed.
Task 2 in phase 1 is not completed.
Task 3 in phase 1 is completed.
Task 1 in phase 2 is completed.
Task 2 in phase 2 is not completed.
Total tasks completed in the mission: 3
Explanation:
This code keeps track of the completed tasks in a space mission, divided into different phases. For each phase, the user inputs the number of tasks, and the code checks whether each task has been completed.
- The user is asked to input the number of phases in the space mission (
phases
). - A variable
completed_tasks
is initialized to 0. This will store the count of tasks that are completed throughout the mission. - A
while
loop is used to go through each phase in the mission. The loop runs as long asphase_number
is less than or equal tophases
. - For each phase, the user is asked how many tasks are there in that phase (
tasks
). - Then, a second
while
loop goes through each task in the current phase. It checks the status of the task: whether it’s completed or not. - If the task is completed (the user enters ‘yes’), the code adds 1 to
completed_tasks
and prints a message confirming the task is completed. - If the task is not completed (the user enters ‘no’), the code prints a message saying the task is not completed.
- After checking all tasks in the current phase, the code moves on to the next phase by increasing
phase_number
. - Finally, the code prints the total number of tasks completed in the mission, stored in
completed_tasks
.
phases = int(input("Enter the number of phases in the space mission: ")) completed_tasks = 0 phase_number = 1 while phase_number <= phases: tasks = int(input(f"Enter the number of tasks in phase {phase_number}: ")) task_number = 1 while task_number <= tasks: status = input(f"Is task {task_number} in phase {phase_number} completed? (Enter 'yes' or 'no'): ").lower() if status == "yes": completed_tasks += 1 print(f"Task {task_number} in phase {phase_number} is completed.") else: print(f"Task {task_number} in phase {phase_number} is not completed.") task_number += 1 phase_number += 1 print(f"Total tasks completed in the mission: {completed_tasks}")
For more Python topics, solutions to common problems, and scenario-based questions on various Python concepts, visit Python at Syntax Scenarios.