Learn how to check if an object has an attribute in Python using hasattr(), getattr(), and try-except in our beginner friendly guide with easy code snippets, real-world analogies, and visuals.
In Python, objects have attributes that store their data and methods. Checking if an object has a specific attribute is a common task. This guide will cover different methods to check if an object has an attribute, including practical examples and use cases.
Understanding Attributes and Objects
An attribute is a characteristic or property of an object, like its color or size. An object is an instance of a class containing attributes and methods that define its behavior and state.
Ways to Check if an Object Has an Attribute in Python
There are multiple ways to find out if an object contains attributes. Let’s discuss the top three ways.
1. Check Attributes with hasattr()
The hasattr()
function in Python is used to check if an object has a specific attribute. Think of it like checking if a car has wheels. If that property exist, the hasattr()
will return true, and if the attribute does not exist, the function will return false. So, using hasattr()
, you can find out whether an object has a specific attribute or not.
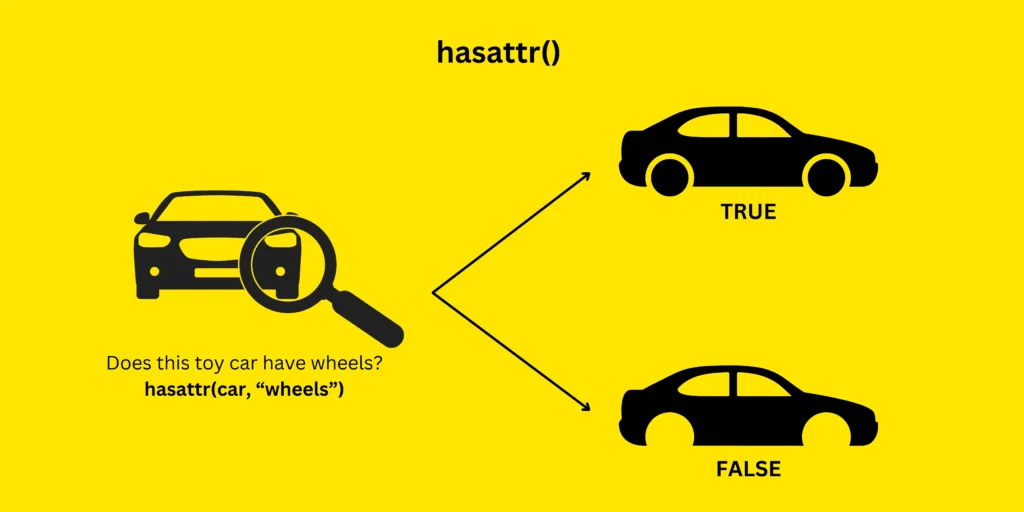
Syntax
hasattr(object, attribute_name)
- object: The object you want to check.
- attribute_name: The name of the attribute you want to check, written as a string.
The function returns True
if the object has the attribute, and False
otherwise.
Example
The code defines a Car
class with attributes wheels
and color
, and creates an instance of this class with 4 wheels
and color red
. The hasattr()
function is then used to check whether the toy_car
object has specific attributes. For wheels
, hasattr()
returns True
since the attribute exists, and for sunroof
, it returns False
since the attribute is not defined. This demonstrates how hasattr()
efficiently checks for the presence of an attribute, returning True
if it exists and False
if it doesn’t.
# Define the Car class with attributes wheels and color class Car: def __init__(self, wheels, color): self.wheels = wheels self.color = color # Create an instance of Car with 4 wheels and color red toy_car = Car(wheels=4, color='red') # Check if the car has the attribute 'wheels' and 'sunroof' has_wheels = hasattr(toy_car, 'wheels') has_sunroof = hasattr(toy_car, 'sunroof') # Output the return values of hasattr() print("Wheels: ", has_wheels) # Expected output: True print("Sunroof: ", has_sunroof) # Expected output: False
Output
Wheels: True Sunroof: False
2. Use getattr() to Access Attributes in Python
In Python, you can get the value of a particular attribute from an object with a built-in function known as getattr()
. If the attribute does not exist, it can return a default value or raise an error if no default value is provided. Think of getattr()
like checking if a car has wheels. If it has, it will return the value of the wheels attribute i-e 4. If the attribute wheels do not exist, it will either raise an error or give a default value provided by the user eg. “The car has no wheels”.
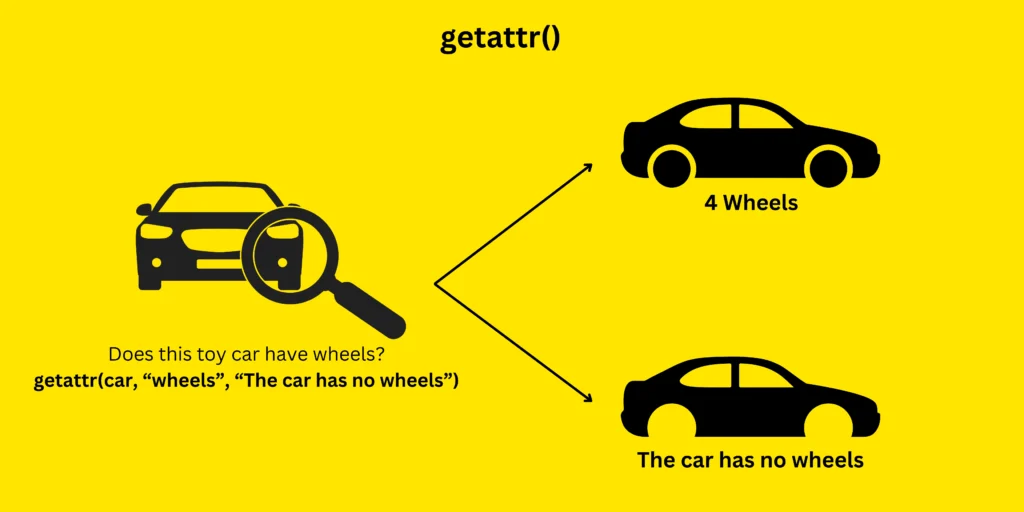
Syntax
getattr(object, attribute_name[, default])
- object: The object from which you want to access the attribute.
- attribute_name: The name of the attribute you want to check, written as a string.
- default (optional): A value to return if the attribute is not found. if not provided and the attribute is missing,
getattr()
will raise anAttributeError
, which occurs when you try to access or call an attribute that does not exist on an object.
Example
The following code uses the same example provided earlier but this time uses the getattr()
function. The getattr()
is used to retrieve the value of the wheels
attribute from the toy_car
object. Since the wheels
attribute exists, getattr()
returns the value 4
.
Next, getattr()
is used to check for a sunroof
attribute that doesn’t exist in the Car
class. In this case, getattr()
returns the default value "The car has no sunroof"
. This demonstrates how getattr()
can access existing attributes and provide a fallback value when an attribute is not present.
# Define the Car class with attributes wheels and color class Car: def __init__(self, wheels, color): self.wheels = wheels self.color = color # Create an instance of Car with 4 wheels and color red toy_car = Car(wheels=4, color='red') # Use getattr() to check if the car has wheels attribute # If the attribute doesn't exist, it will return "The car has no wheels" wheels = getattr(toy_car, 'wheels', "The car has no wheels") print(f"Wheels: {wheels}") # Use getattr() to check if the car has a sunroof attribute (which doesn't exist) # Since sunroof is not defined, it will return "The car has no sunroof" sunroof = getattr(toy_car, 'sunroof', "The car has no sunroof") print(f"Sunroof: {sunroof}")
Output
Wheels: 4 Sunroof: The car has no sunroof
3. Use try and except to Check for Attributes
The try
and except
block in Python helps you manage errors smoothly. Imagine you’re trying to check if a car has a specific feature, like a sunroof. If the feature is missing, instead of getting frustrated, you calmly find another way to proceed. Similarly, you can attempt to access an attribute like sunroof
, within the try
block, and if it doesn’t exist, Python will raise an AttributeError
. This error is caught in the except
block, allowing you to handle the missing attribute without causing your program to fail.
Syntax
try: # Attempt to access an attribute except AttributeError: # This code runs if the attribute is missing
try
block: Place the code where you attempt to access the attribute.except AttributeError
block: Specify what should happen if the attribute does not exist.
Example
In this scenario, the try
and except
block is used to handle potential errors when accessing attributes of a car. In the first try
block, accessing the wheels
attribute works successfully since it exists, so no error occurs. In the second try
block, accessing the sunroof
attribute, which does not exist, raises an AttributeError
. The except
block catches this error and prints a message stating that the car has no sunroof, allowing the program to continue running smoothly without crashing.
# Define the Car class with attributes wheels and color class Car: def __init__(self, wheels, color): self.wheels = wheels self.color = color # Create an instance of Car with 4 wheels and color red toy_car = Car(wheels=4, color='red') # Try to access the 'wheels' attribute try: print(f"Wheels: {toy_car.wheels}") # This will work as 'wheels' attribute exists except AttributeError: print("The car has no wheels.") # Try to access the 'sunroof' attribute try: print(f"Sunroof: {toy_car.sunroof}") # This will raise an AttributeError except AttributeError: print("The car has no sunroof.")
Output
Wheels: 4 The car has no sunroof.
Once you find out if an object has attributes, you may want to print all properties of attributes of that object.
Why It’s Important to Check If Certain Attributes Exist for an Object?
- Avoid Errors: Checking object attributes helps prevent errors, like
AttributeError
, that can crash your program if you try to access something that doesn’t exist. - Handle Missing Attributes: You can provide a fallback or alternative action if an attribute is missing, ensuring your code runs smoothly.
- Improve Code Reliability: By checking attributes, your code becomes more reliable and less prone to unexpected failures.
- Control Flow: It allows you to control the flow of your program, deciding what to do if an attribute is present or absent.
- Enhance User Experience: Properly handling missing attributes can improve user experience by preventing abrupt program terminations and providing meaningful messages.
Conclusion
Checking if an object has an attribute helps you avoid errors and write better Python code. By using methods like hasattr()
, getattr()
, try
blocks, dir()
, __dict__
, and vars()
, you can make sure that attributes are present before you try to use them. This practice leads to code that is less likely to crash and easier to understand. It also helps in debugging and ensures that your programs work smoothly with different objects. Overall, these techniques make your code more reliable and maintainable.