Learning programming is like solving puzzles; it’s all about figuring out the right pieces to make things work. In Python, we use lists for 1D arrays, and they are a powerful tool for handling ordered collections of data. Let’s look into some fun and engaging scenarios where you’ll use 1D arrays in Python to tackle real-world problems. These scenarios will not only help you learn but also give you practical experience in solving everyday problems with coding. Ready to get started?
Here are some scenario-based questions, ranging from easy to hard, that will help you strengthen your understanding of 1D arrays in Python.
Saving a Princess in a Maze
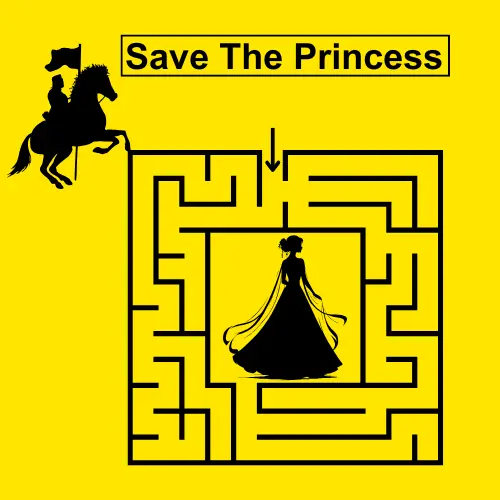
You are a knight trying to rescue a princess in a maze. The maze is a 1D array, where each value tells you how far to move (positive or negative). The goal is to reach the princess, who is at the last index.
Write a Python program to find the number of steps you need to take.
Test Case:
Input:
Input Steps= 3 -1 2 1 -3 2 1
Expected Output:
Total steps taken: 7
Explanation:
Explanation:
This code calculates how many steps it takes to reach the end of a path based on given steps that can take you forward by a certain number of positions. The code uses a list and a while
loop to simulate this process.
Bulleted Explanation:
- The code begins by asking for input with the message
Enter the steps (space-separated values):
, where the user inputs a sequence of steps, separated by spaces. - The input is split into separate values using
.split()
, which breaks the input into a list of strings, then converted into integers usingint(i)
within a list comprehension:[int(i) for i in input_steps.split()]
. - The list
steps
contains the number of steps to take from each position in the path, starting at position 0. - The variables
current_position
andsteps_taken
are initialized to 0.current_position
keeps track of where you are, andsteps_taken
counts how many steps you’ve taken. - Inside the
while
loop, the code keeps adding the number of steps from the current position to thecurrent_position
. This is done usingcurrent_position += steps[current_position]
. - After each step, the
steps_taken
counter is increased by 1. - The loop continues as long as the
current_position
is less than the length of thesteps
list. - The loop stops when the
current_position
reaches the last position (current_position == len(steps) - 1
), and it breaks out of the loop. - Finally, the total number of steps taken is printed:
Total steps taken: {steps_taken}
.
input_steps = input("Enter the steps (space-separated values): ") steps = [int(i) for i in input_steps.split()] current_position = 0 steps_taken = 0 while current_position < len(steps): current_position += steps[current_position] steps_taken += 1 if current_position == len(steps) - 1: break print(f"Total steps taken: {steps_taken}")
Weather Data Analysis
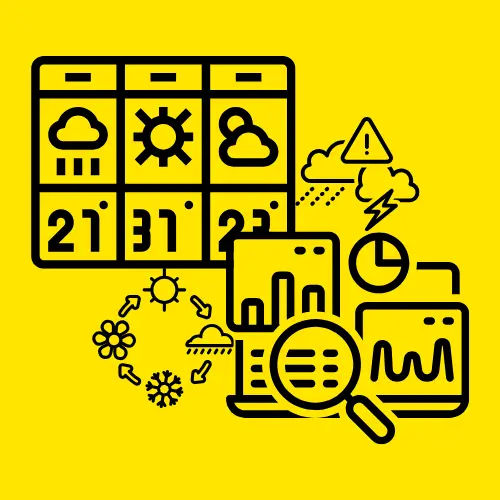
Imagine you are working for a weather forecasting company, and you’ve been tasked with analyzing the temperatures for a specific month. You have a list of daily temperatures for that month, and your job is to calculate three key things: the average temperature for the month, the number of days the temperature was above average, and the difference between the highest and lowest temperatures recorded.
You need to write a Python program that performs these calculations and displays the results, so the company can get a clear understanding of the temperature trends for that month.
Test Case:
Input:
Temperatures = [32, 34, 29, 31, 28, 33, 35, 36, 30, 28, 31, 32, 34, 35, 29, 30]
Expected Output:
Average Temperature = 31.5
Days Above Average = 8
Temp Difference = 8
Explanation:
This code calculates the average temperature from a list of daily temperatures, counts how many days had temperatures above the average, and calculates the difference between the highest and lowest temperatures.
- First, the code takes input for daily temperatures as space-separated values using
input()
. The input string is split into individual temperature values using.split()
and converted into integers usingint()
. This list of temperatures is stored in the variabletemperatures
. - The code then calculates the average temperature by summing all the temperatures using
sum(temperatures)
and dividing it by the number of temperatures withlen(temperatures)
. The result is stored inaverage_temp
. - After that, the code counts how many days had temperatures above average. It does this by using a generator expression
sum(1 for temp in temperatures if temp > average_temp)
, which counts the number of days where the temperature is greater than theaverage_temp
. - The code then calculates the difference between the highest and lowest temperatures using
max(temperatures) - min(temperatures)
, and stores it intemp_diff
. - Finally, the code prints the average temperature, the number of days above the average temperature, and the temperature difference.
input_temperatures = input("Enter daily temperatures (space-separated values): ") temperatures = [int(temp) for temp in input_temperatures.split()] average_temp = sum(temperatures) / len(temperatures) above_average_days = sum(1 for temp in temperatures if temp > average_temp) temp_diff = max(temperatures) - min(temperatures) print(f"Average Temperature = {average_temp}") print(f"Days Above Average = {above_average_days}") print(f"Temperature Difference = {temp_diff}")
Stock Market Investment
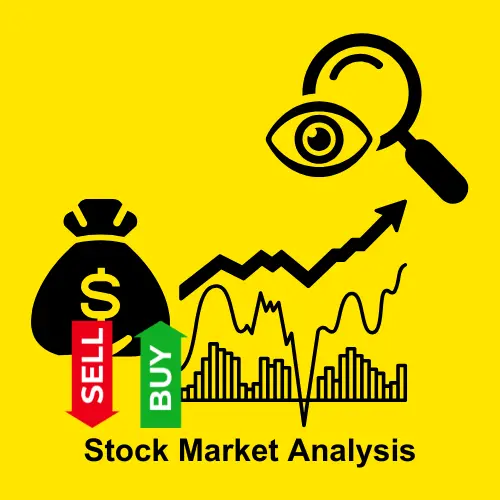
Imagine you are an investor who invests a fixed amount monthly in the stock market. You track a company’s stock prices over several months to evaluate your gains or losses based on percentage changes in the stock prices. A rise or fall in stock price directly affects your investment, like a 10% increase yielding a 10% gain.
To analyze your performance, you write a Python program to calculate the monthly percentage change, determine the gain or loss for each month, and compute the total profit or loss over the period.
Test Case:
Input:
Stock Prices = [100, 110, 105, 120, 125]
Investment per Month = 1000
Expected Output:
Total gain/loss: 4000.0
Explanation:
This code calculates the total gain or loss from monthly stock investments based on changes in stock prices.
- First, the user inputs monthly stock prices as a space-separated string, which is converted to a list of floating-point numbers using
input()
and a list comprehension withfloat()
.- Example:
"100 110 105"
becomes[100.0, 110.0, 105.0]
.
- Example:
- Next, the user specifies the amount they invest each month, which is read and converted to a float using
float(input())
. - The main logic uses a
for
loop starting from the second month (i = 1
) to calculate the monthly price change percentage:- The formula
(stock_prices[i] - stock_prices[i - 1]) / stock_prices[i - 1]
computes the percentage change between the current month’s price and the previous month’s price.
- The formula
- This percentage change (
price_change
) is then multiplied byinvestment_per_month
to calculate the gain or loss for that month. - The
gain_or_loss
for each month is added tototal_gain
to keep track of the overall gain or loss across all months. - Finally, the total gain or loss is printed.
input_prices = input("Enter stock prices for each month (space-separated values): ") stock_prices = [float(price) for price in input_prices.split()] investment_per_month = float(input("Enter the amount invested each month: ")) total_gain = 0 for i in range(1, len(stock_prices)): price_change = (stock_prices[i] - stock_prices[i - 1]) / stock_prices[i - 1] gain_or_loss = investment_per_month * price_change total_gain += gain_or_loss print(f"Total gain/loss: {total_gain}")
Freelance Earning Calculation
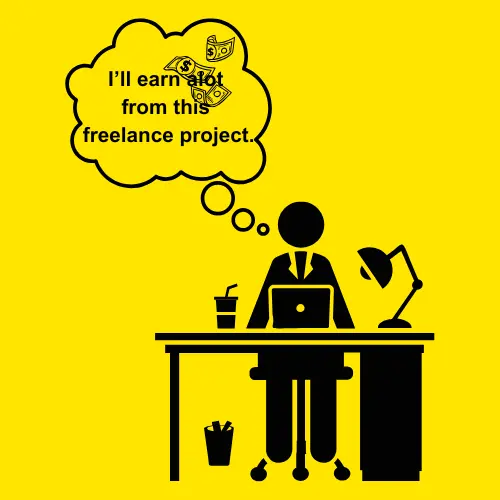
As a freelancer, you work on several client projects, each with a different hourly rate. You track the hours worked for each client. At the end of the month, you need to calculate your total income based on the hours worked and the hourly rates for each client.
Write a Python program that takes three inputs: a list of client names, a list of hours worked, and a list of hourly rates, then calculates and outputs your total income for the month.
Test Case:
Input:
Client Names= Client A, Client B, Client C
Hours Worked for each client= 40, 30, 25
Hourly Rates for each client= 50, 60, 70
Expected Output:
Total income: $4250
Explanation:
This code calculates the total income based on the number of hours worked for each client and their respective hourly rates.
- First, the user inputs a comma-separated list of client names, which is split into individual elements using
.split(',')
. The.strip()
method is then used to remove any extra spaces around each client name. - Next, the user inputs the hours worked for each client, again as a comma-separated string. The string is split and converted into a list of integers using
.split(',')
andint()
, with.strip()
to remove any extra spaces. - Similarly, the user inputs the hourly rates for each client. This is also processed by splitting the string, converting each value into an integer, and stripping any spaces.
- Then a
for
loop iterates through each client:- For each client, the code calculates the total income by multiplying the number of hours worked (
hours_worked[i]
) by the hourly rate (hourly_rates[i]
). - This value is added to
total_income
, which accumulates the total income over all clients.
- For each client, the code calculates the total income by multiplying the number of hours worked (
- Finally, the total income is printed out using
print(f"Total income: ${total_income}")
.
clients = input("Enter client names (comma-separated): ").split(',') clients = [client.strip() for client in clients] hours_worked = input("Enter hours worked for each client (comma-separated): ").split(',') hours_worked = [int(hour.strip()) for hour in hours_worked] hourly_rates = input("Enter hourly rates for each client (comma-separated): ").split(',') hourly_rates = [int(rate.strip()) for rate in hourly_rates] total_income = 0 for i in range(len(clients)): total_income += hours_worked[i] * hourly_rates[i] print(f"Total income: ${total_income}")
Loan Repayment Schedule
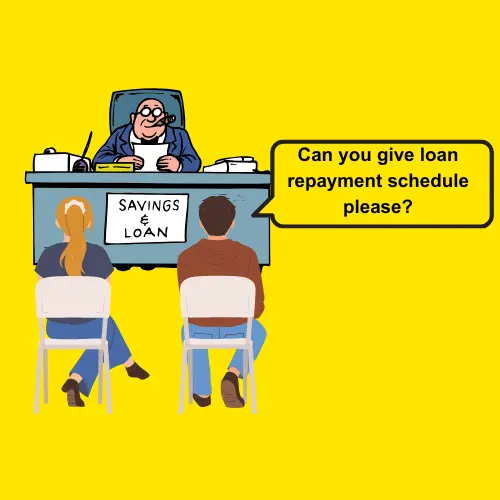
You took out a loan with a fixed monthly interest rate. You have a list of loan balances after each repayment. Your task is to calculate the total interest paid. The interest rate stays the same each month. To do this, calculate how much interest is paid based on the remaining balances.
Write a Python script to find the total interest.
Test Case:
Input:
Loan Balances for each month= 10000, 9000, 8000, 7000, 6000
Monthly Interest rate= 0.05
Expected Output:
Total interest paid: 2250.0
Explanation:
This code calculates the total interest paid on a loan over several months.
- First, the user inputs the loan balances for each month as a comma-separated string. The
.split(',')
method divides the input into a list of strings, and.strip()
removes any extra spaces from the values. These values are then converted tofloat
to represent the loan balances correctly. - Next, the user provides the monthly interest rate as a decimal (e.g., 0.05 for 5%). This value is converted to a
float
to ensure it’s in numerical format. - Then, the variable
total_interest_paid
is initialized to 0. This will keep track of the total interest paid across all months. - The code uses a
for
loop that starts from the second month (i = 1
) and loops through the rest of the months. For each month:- The interest is calculated by multiplying the previous month’s loan balance (
loan_balances[i-1]
) by the monthly interest rate (monthly_interest_rate
). - This interest is added to the
total_interest_paid
.
- The interest is calculated by multiplying the previous month’s loan balance (
- Finally, the total interest paid is printed out using
print(f"Total interest paid: {total_interest_paid}")
.
loan_balances = input("Enter the loan balances for each month (comma-separated): ").split(',') loan_balances = [float(balance.strip()) for balance in loan_balances] monthly_interest_rate = float(input("Enter the monthly interest rate (as a decimal): ")) total_interest_paid = 0 for i in range(1, len(loan_balances)): interest = loan_balances[i-1] * monthly_interest_rate total_interest_paid += interest print(f"Total interest paid: {total_interest_paid}")
Twitter Hashtag Trending
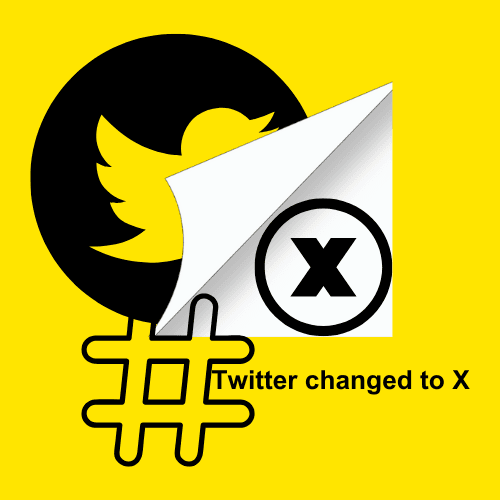
You are analyzing a set of tweets to find the most frequently used hashtag. The array of strings represents tweets, and each tweet contains hashtags. You need to find out which hashtag has the highest frequency across all tweets using a Python code.
Test Case:
Input:
Enter the number of tweets: 5
Enter tweet 1: #Python is amazing
Enter tweet 2: I love #Python and #AI
Enter tweet 3: #Python is great for data science
Enter tweet 4: #AI is the future
Enter tweet 5: #Python #AI #DataScience
Expected Output:
The most frequent hashtag is: #Python
Explanation:
This code finds the most frequent hashtag from multiple tweets.
- The user inputs the number of tweets (
n
), and the code collects them into thetweets
list using a list comprehension:[input(f"Enter tweet {i+1}: ") for i in range(n)]
. - The code initializes two empty lists:
hashtags
for storing unique hashtags andfrequencies
for storing their counts. - It loops through each tweet, splits it into words with
.split()
, and checks if a word starts with#
using.startswith('#')
to identify hashtags. - If the hashtag already exists in
hashtags
, the code updates its frequency by finding its index with.index()
and incrementing the corresponding value infrequencies
. If not, the code adds the hashtag with a frequency of 1 using.append()
. - After processing all the tweets, the code finds the index of the most frequent hashtag using
frequencies.index(max(frequencies))
and retrieves it fromhashtags
. - Finally, it prints the most frequent hashtag.
n = int(input("Enter the number of tweets: ")) tweets = [input(f"Enter tweet {i+1}: ") for i in range(n)] hashtags = [] frequencies = [] for tweet in tweets: words = tweet.split() for word in words: if word.startswith('#'): if word in hashtags: index = hashtags.index(word) # Find the index of the existing hashtag frequencies[index] += 1 else: hashtags.append(word) # Add the new hashtag frequencies.append(1) # Set the initial frequency to 1 max_index = frequencies.index(max(frequencies)) most_frequent_hashtag = hashtags[max_index] print(f"The most frequent hashtag is: {most_frequent_hashtag}")
Find the Highest Score
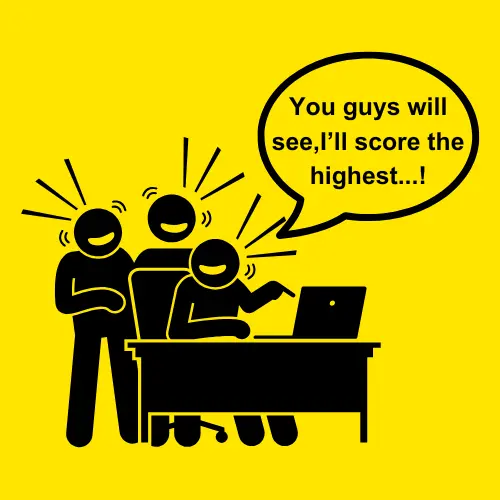
You and your friends are competing in an online game, and everyone is eager to know who scored the highest. Each player submits their score, and you want to find the highest score using Python.
Test Case:
Input:
Number of players: 5
Enter the score for player 1: 85
Enter the score for player 2: 92
Enter the score for player 3: 88
Enter the score for player 4: 78
Enter the score for player 5: 90 Space Exploration Crew Selection
Expected Output:
The highest score is: 92
Explanation:
This code determines the highest score among a group of players.
- First, the code prompts the user to input the number of players (
num_players
). Then, it initializes an empty list calledscores
to store each player’s score. - Next, using a
for
loop, the code collects scores for each player by asking for input. It adds these scores to thescores
list using the.append()
method. - After collecting all the scores, the code initializes
highest_score
with the first score in the list (scores[0]
). - Then, it iterates through the
scores
list. During each iteration, it checks if the current score is greater thanhighest_score
using anif
condition. If this condition is true, the code updateshighest_score
to the current score. - Finally, after the loop completes, the code uses
print()
to display the highest score.
num_players = int(input("Enter the number of players: ")) scores = [] for i in range(num_players): score = int(input(f"Enter the score for player {i + 1}: ")) scores.append(score) # Initialize the highest score to the first score highest_score = scores[0] for score in scores: if score > highest_score: highest_score = score print(f"The highest score is: {highest_score}")
Letter Puzzle Challenge
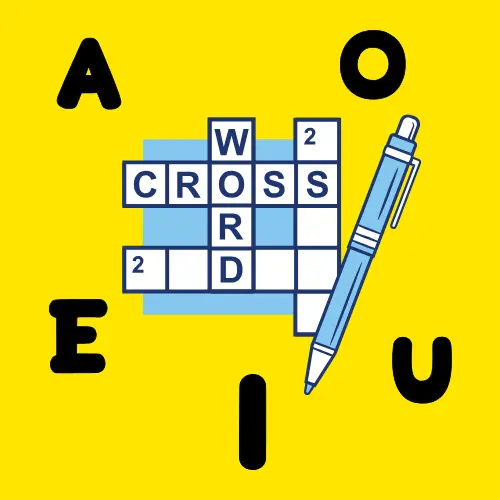
You’re participating in a letter puzzle game where you receive a random list of letters. Your challenge is to count how many vowels (a, e, i, o, u) are present in the given list. To ensure fairness and accuracy, you decide to write a Python program that will count the vowels for you.
Test Case:
Input:
Number of letters: 6
Enter the letters one by one:
Letter 1: a
Letter 2: b
Letter 3: e
Letter 4: f
Letter 5: i
Letter 6: o
Expected Output:
Number of vowels in the puzzle: 4
Explanation:
This code counts the number of vowels in a given set of letters.
- First, the code prompts the user to enter the number of letters in the puzzle (
n
). Then, it initializes an empty list namedletters
to store the input letters. - Next, the code asks the user to enter each letter one by one using a
for
loop. As each letter is entered, it converts the letter to lowercase with.lower()
to ensure consistency (since vowels can appear in uppercase or lowercase) and adds it to theletters
list. - After collecting all the letters, the code defines a list of vowels,
vowels = ['a', 'e', 'i', 'o', 'u']
, and initializes a variablevowel_count
to zero to keep track of the number of vowels. - Then, it uses a nested
for
loop to compare each letter inletters
with the vowels in thevowels
list. If a match is found (if letter == vowel
), it increasesvowel_count
by 1 and immediately breaks out of the inner loop usingbreak
. This ensures each letter is only counted once, even if it matches a vowel multiple times. - Finally, the code prints the total count of vowels using the
print()
function.
n = int(input("Enter the number of letters in the puzzle: ")) letters = [] print("Enter the letters one by one:") for i in range(n): letter = input(f"Letter {i + 1}: ").lower() # Convert to lowercase for consistency letters.append(letter) vowels = ['a', 'e', 'i', 'o', 'u'] vowel_count = 0 # Count the vowels for letter in letters: for vowel in vowels: if letter == vowel: vowel_count += 1 break # Exit inner loop once matched print(f"Number of vowels in the puzzle: {vowel_count}")
The Monster Hunt
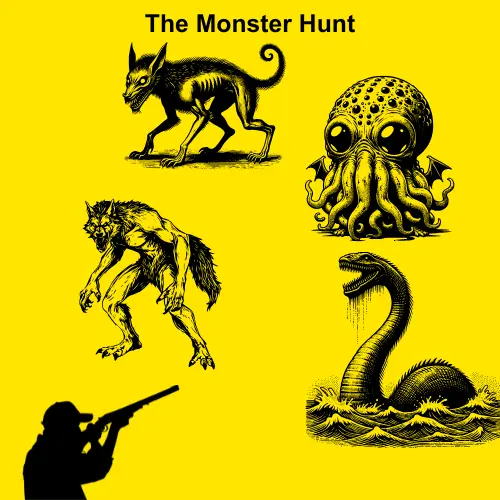
In a dark forest, you’re hunting monsters. Each monster has a different health value. Your goal is to calculate how many monsters you can defeat with your weapon’s attack power. The weapon’s power decreases by 10% after each attack. You stop when your weapon’s power is zero or less.
Write a Python script to calculate how many monsters you can defeat.
Test Case:
Input:
Health of Monsters separated by spaces= 100 150 200 50 120
Initial Weapon Power= 500
Output:
Monsters defeated: 4
Explanation:
This code simulates a battle with monsters where the weapon power decreases as each monster is defeated.
- The code starts by prompting the user to enter the health of monsters, separated by spaces, using
input()
. It splits the input into individual health values with.split()
and stores them in themonsters
list as strings. - A
for
loop then goes through each health value in themonsters
list and convert each string to an integer usingint()
. The converted health values are stored in themonster_healths
list. - Next, the user is prompted to enter the initial weapon power using
input()
, and this value is converted to an integer. - The
defeated_count
variable is initialized to 0, which will keep track of how many monsters the weapon defeats. - Then, a
for
loop starts to process each monster’s health:- If the
weapon_power
is 0 or below, the loop breaks, meaning no more monsters can be defeated. - Otherwise, the weapon power is reduced by 10% of the current monster’s health (i.e.,
weapon_power -= (health * 0.1)
). - The defeated monster count (
defeated_count
) is incremented by 1 each time the weapon defeats a monster.
- If the
- Finally, the total number of defeated monsters is printed.
monsters = input("Enter the health of monsters separated by spaces: ").split() monster_healths = [] for health in monsters: monster_healths.append(int(health)) weapon_power = int(input("Enter the initial weapon power: ")) defeated_count = 0 for health in monster_healths: if weapon_power <= 0: break weapon_power -= (health * 0.1) # Decrease weapon power by 10% of monster's health defeated_count += 1 print(f"Monsters defeated: {defeated_count}")
Sorting Student Scores
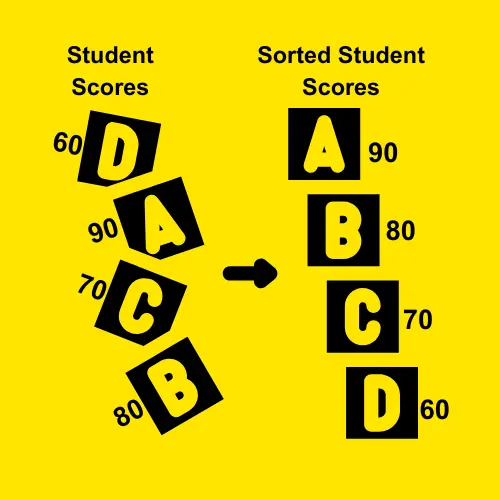
In a school, you are responsible for organizing student scores. You have a list of student scores. You need to sort them in descending order (from highest to lowest) without using pre-defined sorting functions. You will implement your sorting logic using a simple algorithm in Python.
Test Case:
Input:
Number of students: 5
Enter the score for student 1: 90
Enter the score for student 2: 72
Enter the score for student 3: 85
Enter the score for student 4: 96
Enter the score for student 5: 88
Expected Output:
Sorted scores in descending order: [96, 90, 88, 85, 72]
Explanation:
The code sorts a list of student scores in descending order using the bubble sort algorithm.
- First, the program asks for the number of students, which is stored in
n
. - It then asks for each student’s score, collecting them into a list called
scores
using a list comprehension. - Next, the code applies the bubble sort algorithm to arrange the scores in descending order:
- The outer
for
loop runs forn
iterations, where each iteration moves the largest element to the end. - Inside the outer loop, the inner loop goes through the list from the start to the last unsorted element (
n-i-1
). - If the current score is smaller than the next score (
scores[j] < scores[j+1]
), the two scores are swapped. This ensures that the larger score moves towards the beginning of the list.
- The outer
- Finally, after sorting, the program prints the sorted scores in descending order.
n = int(input("Enter the number of students: ")) scores = [int(input(f"Enter the score for student {i+1}: ")) for i in range(n)] # Bubble Sort logic to sort scores in descending order for i in range(n): # After each iteration, the largest element will be in its correct position for j in range(0, n-i-1): if scores[j] < scores[j+1]: # Swap if the element is smaller than the next element scores[j], scores[j+1] = scores[j+1], scores[j] print(f"Sorted scores in descending order: {scores}")
Grocery Store Price List
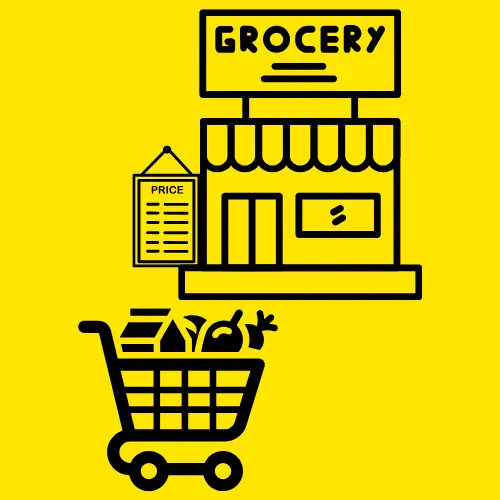
Suppose you work as an inventory manager at a local grocery store. The store receives weekly shipments of different food items, and you keep track of their prices in an array. This time, the store is offering a special discount on all the items, where the price of each item should be reduced by 10%. You need to apply this discount to the current prices of all items in the listby the help of a Python code.
Test Case:
Input:
Number of items: 4
Enter the price of item 1: 15.0
Enter the price of item 2: 7.5
Enter the price of item 3: 25.0
Enter the price of item 4: 9.0
Expected Output:
Updated price list after discount:
13.5
Free
22.5
Free
Explanation:
The code updates the prices of items in a grocery list, applying a discount and marking some items as “Free.”
- First, the program asks for the number of items in the grocery list (
n
). - Then, it asks for the price of each item and stores them in a list called
prices
. - After collecting all the prices, the code moves to the next step:
- The first
for
loop goes through each item in the list. - It applies a 10% discount on each price by multiplying the price by 0.9 (
prices[i] * 0.9
). - If the price after the discount is below 10, the item is marked as “Free.”
- The first
- Finally, the program prints the updated list of prices, showing either the discounted price or “Free” for the items.
n = int(input("Enter the number of items in the grocery list: ")) prices = [] for i in range(n): price = float(input(f"Enter the price of item {i+1}: ")) prices.append(price) for i in range(n): # Applying 10% discount prices[i] = prices[i] * 0.9 # If the price is below 10, mark it as "Free" if prices[i] < 10: prices[i] = "Free" print("Updated price list after discount:") for price in prices: print(price)
Facebook Like Counts
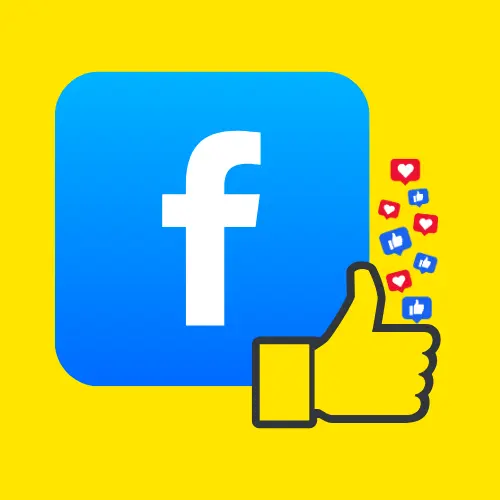
You are building a feature for a social media app. Each post has a number of likes stored in a list. Add 10 extra likes to every post. Then, find which posts have more than 100 likes and mark them as “Trending.” Write a Python program to do this.
Test Case:
Input:
Number of posts: 3
Enter the likes for post 1: 95
Enter the likes for post 2: 50
Enter the likes for post 3: 101
Expected Output:
Updated like counts:
Trending
60
Trending
Explanation:
This code updates the like counts for a series of posts and marks posts with high likes as “Trending.”
- The program begins by asking for the number of posts (
n
). - Next, it collects the like counts for each post and stores them in a list called
likes
. - Then, it processes each post’s likes:
- It adds 10 likes to the existing count for each post (
likes[i] += 10
). - If the updated likes exceed 100, the program marks the post as “Trending” by setting its value to
"Trending"
.
- It adds 10 likes to the existing count for each post (
- Finally, the code prints the updated like counts, showing either the new number of likes or “Trending” for posts with over 100 likes.
n = int(input("Enter the number of posts: ")) likes = [] for i in range(n): count = int(input(f"Enter the likes for post {i+1}: ")) likes.append(count) for i in range(n): likes[i] += 10 if likes[i] > 100: likes[i] = "Trending" print("Updated like counts:") for like in likes: print(like)
Freelancer Ratings
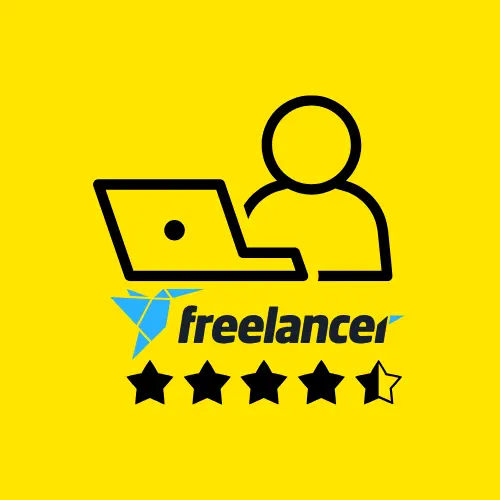
You manage ratings for freelancers. Freelancer ratings are stored in an array, where each rating is between 0 and 5. A new policy deducts 0.5 from the rating of each freelancer who hasn’t worked in the last 6 months. Any freelancer with a rating less than 2 will receive a “Low Priority” tag.
Develop a Python script for this scenario.
Test Case:
Input:
Enter the number of freelancers: 3
Enter the rating for freelancer 1 (0 to 5): 2.5
Enter the rating for freelancer 2 (0 to 5): 4.0
Enter the rating for freelancer 3 (0 to 5): 1.8
Expected Output:
Updated ratings:
2.0
3.5
Low Priority
Explanation:
This code adjusts the ratings of freelancers and categorizes those with low ratings as “Low Priority.”
- It starts by asking for the number of freelancers (
n
). - Then, it collects the ratings (on a scale of 0 to 5) for each freelancer and stores them in a list called
ratings
. - Next, it processes each freelancer’s rating:
- It deducts 0.5 from each rating (
ratings[i] -= 0.5
). - If the updated rating falls below 2, the program marks the freelancer as
"Low Priority"
by replacing their rating with this label.
- It deducts 0.5 from each rating (
- Finally, it prints the updated ratings list, showing either the adjusted rating or
"Low Priority"
for freelancers with ratings under 2.
n = int(input("Enter the number of freelancers: ")) ratings = [] for i in range(n): rating = float(input(f"Enter the rating for freelancer {i+1} (0 to 5): ")) ratings.append(rating) for i in range(n): ratings[i] -= 0.5 # Deducting 0.5 if ratings[i] < 2: ratings[i] = "Low Priority" print("Updated ratings:") for rating in ratings: print(rating)
Candy Crush High Scores
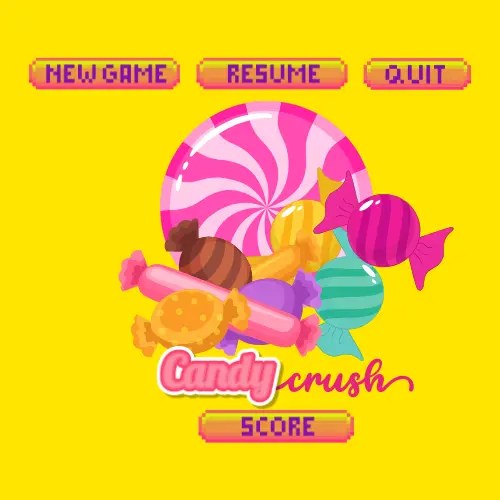
You are developing a scoring system for a game like Candy Crush in Python. Players’ scores are stored in an array. If a player’s score exceeds 1000, they receive a “Gold Medal” badge.
Test Case:
Input:
Enter the number of players: 3
Enter the score for player 1: 950
Enter the score for player 2: 1200
Enter the score for player 3: 850
Expected Output:
Updated player scores:
950
Gold Medal
850
Explanation:
This code updates the scores of players and awards a “Gold Medal” to those with high scores in a game.
- It starts by asking for the number of players (
n
). - Then, it collects the scores of all players, storing them in a list called
scores
. - After that, it processes each player’s score:
- If a player’s score exceeds 1000, the program replaces their score with
"Gold Medal"
.
- If a player’s score exceeds 1000, the program replaces their score with
- Finally, it prints the updated scores list, showing either the original score or
"Gold Medal"
for high-scoring players.
n = int(input("Enter the number of players: ")) scores = [] for i in range(n): score = int(input(f"Enter the score for player {i+1}: ")) scores.append(score) for i in range(n): if scores[i] > 1000: scores[i] = "Gold Medal" print("Updated player scores:") for score in scores: print(score)
Space Exploration Crew Selection
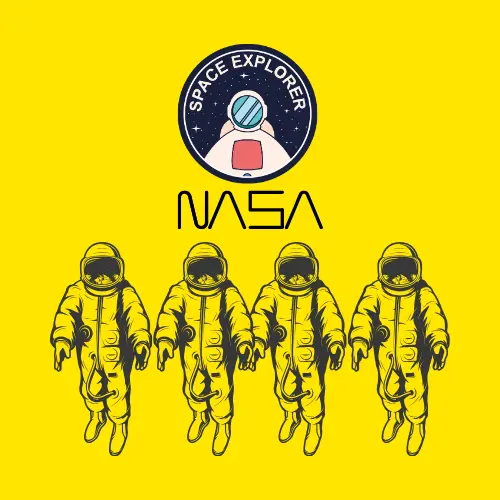
Imagine you are working for the Galactic Exploration Agency, which is selecting a crew for a mission to Mars. Each candidate has scores from physical, technical, and psychological tests. The goal is to find the top 3 candidates with the highest scores.
Write a Python program to take the number of candidates and their scores as input, sort the scores in descending order without using built-in sort functions, and display the top 3 scores as “Selected for Crew.”
Test Case:
Input:
Number of candidates: 5
Enter the score for candidate 1: 78
Enter the score for candidate 2: 85
Enter the score for candidate 3: 90
Enter the score for candidate 4: 88
Enter the score for candidate 5: 95
Expected Output:
Top 3 candidates selected for the crew:
Candidate 1: 95 (Selected for Crew)
Candidate 2: 90 (Selected for Crew)
Candidate 3: 88 (Selected for Crew)
Explanation:
This code selects the top 3 candidates for a crew based on their scores.
- First, it asks for the number of candidates (
n
). - Then, it collects the scores of all candidates and stores them in the
scores
list. - Next, the code uses the Bubble Sort technique to sort the scores in descending order. This means that the highest score comes first, and it keeps swapping the scores until they are in the correct order.
- After sorting, the program prints the top 3 candidates’ scores. It prints up to 3 candidates, but if there are fewer than 3 candidates, it will print all of them.
- Finally, it shows the selected candidates and their scores.
n = int(input("Enter the number of candidates: ")) scores = [] for i in range(n): score = int(input(f"Enter the score for candidate {i+1}: ")) scores.append(score) for i in range(n): for j in range(0, n-i-1): if scores[j] < scores[j+1]: # Swap elements scores[j], scores[j+1] = scores[j+1], scores[j] print("\nTop 3 candidates selected for the crew:") for i in range(3 if n >= 3 else n): print(f"Candidate {i+1}: {scores[i]} (Selected for Crew)")
For more engaging and informative articles on Python, check out our Python tutorial series at Syntax Scenarios.