In programming, nested if-else statements help us make decisions based on multiple conditions. This article provides a series of scenario-based coding questions to help you practice using nested if-else in real-life situations. By going through these examples, you’ll learn how to solve problems like calculating grades or solving games while getting better at writing code that handles different conditions. These exercises will show you how nested if-else statements can make your code more flexible and effective.
Discount Calculator for Shoppers
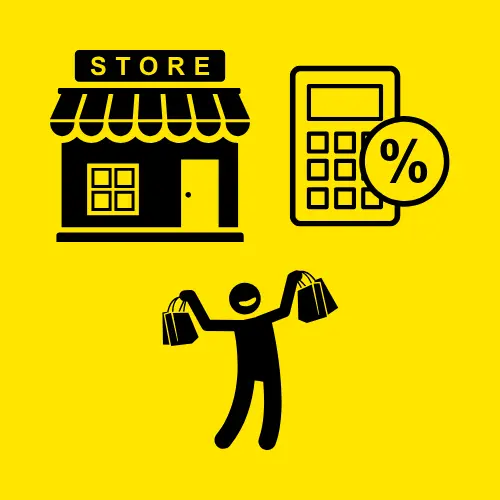
A retail store offers discounts based on the amount a customer spends:
No discount if the amount is less than $50.
10% discount for $50 to $100.
20% discount for more than $100.
Additionally, for customers who qualify for a discount, if the amount spent is an even number, apply an additional 5% discount to the already discounted price.
Write a Mojo program to calculate the final price after applying the discount.
Test Case
Input:
Enter the amount spent: 120
Expected Output:
Discounted Amount: 96.0
Explanation:
This program calculates the final price after applying a discount based on the amount spent.
- The program prompts the user to input the amount they have spent and stored in the variable
amount_spent
. - If the
amount_spent
is less than 50, no discount is applied, and the program directly prints theamount_spent
. - If the
amount_spent
is between 50 and 100 (inclusive), a discount of 10% is applied.- The program calculates the
discount
as 10% ofamount_spent
, and thediscounted_amount
is computed by subtracting thediscount
from theamount_spent
. - Additionally, if the
amount_spent
is even, an extra 5% discount is applied to thediscounted_amount
. The final discounted amount is then printed.
- The program calculates the
- If the
amount_spent
is greater than 100, a 20% discount is applied.- The program calculates the
discount
as 20% ofamount_spent
, and thediscounted_amount
is computed by subtracting thediscount
from theamount_spent
. - If the
amount_spent
is even, an extra 5% discount is applied to thediscounted_amount
. The final discounted amount is then printed.
- The program calculates the
amount_spent = float(input("Enter the amount spent: ")) if amount_spent < 50: print("No discount. Amount to pay:", amount_spent) elif amount_spent >= 50 and amount_spent <= 100: discount = amount_spent * 0.10 discounted_amount = amount_spent - discount if amount_spent % 2 == 0: extra_discount = discounted_amount * 0.05 discounted_amount -= extra_discount print(f"Discounted Amount: {discounted_amount}") elif amount_spent > 100: discount = amount_spent * 0.20 discounted_amount = amount_spent - discount if amount_spent % 2 == 0: extra_discount = discounted_amount * 0.05 discounted_amount -= extra_discount print(f"Discounted Amount: {discounted_amount}")
Grade Based on Marks and Subject Difficulty
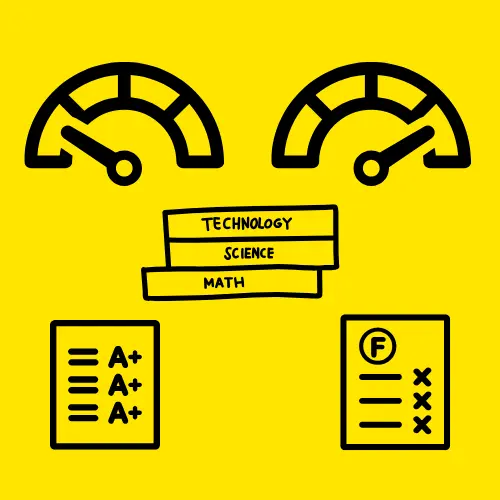
Write a Mojo program to check the following conditions:
If the subject difficulty is “Easy”:
If the marks are greater than or equal to 80, the grade will be A.
If the marks are between 60 and 79, the grade will be B.
If the marks are between 40 and 59, the grade will be C.
If the marks are below 40, the grade will be D.
If the subject difficulty is “Hard”:
If the marks are greater than or equal to 70, the grade will be A.
If the marks are between 50 and 69, the grade will be B.
If the marks are between 30 and 49, the grade will be C.
If the marks are below 30, the grade will be D.
Test Case
Input:
Enter subject (Easy/Hard): Easy
Enter marks: 85
Expected Output:
Grade: A
Explanation:
This program determines the grade based on the subject’s difficulty level and the marks obtained by the user.
- The user is prompted to input the
difficulty
(either “Easy” or “Hard”) and themarks
they received. - If the
difficulty
is “Easy”, the program checks if themarks
are 80 or above.- If this condition is true, it prints “Grade: A”. If the
marks
are between 60 and 79, the program prints “Grade: B”. If themarks
are between 40 and 59, it prints “Grade: C”. If themarks
are below 40, it prints “Grade: D”.
- If this condition is true, it prints “Grade: A”. If the
- If the
difficulty
is “Hard”, the program applies a stricter grading scale.- If the
marks
are 70 or above, it prints “Grade: A”. If themarks
are between 50 and 69, it prints “Grade: B”. If themarks
are between 30 and 49, it prints “Grade: C”. If themarks
are below 30, it prints “Grade: D”.
- If the
- If the input
difficulty
is neither “Easy” nor “Hard”, the program prints “Invalid subject difficulty”.
difficulty = input("Enter the subject difficulty (Easy/Hard): ") marks = float(input("Enter the marks: ")) if difficulty == "Easy": if marks >= 80: print("Grade: A") elif marks >= 60: print("Grade: B") elif marks >= 40: print("Grade: C") else: print("Grade: D") elif difficulty == "Hard": if marks >= 70: print("Grade: A") elif marks >= 50: print("Grade: B") elif marks >= 30: print("Grade: C") else: print("Grade: D") else: print("Invalid subject difficulty")
Number Categorization
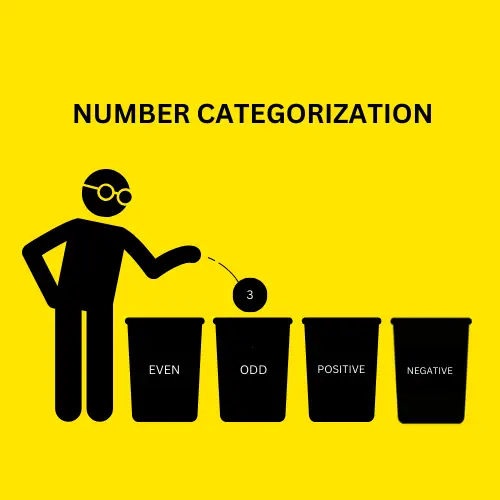
Write a Mojo program that classifies a number into one of the following categories:
Positive Even
Positive Odd
Negative Even
Negative Odd
Zero
Test Case
Input:
Enter a number: -2
Expected Output:
Negative Even
Explanation:
This program classifies a given number as either “Zero”, “Positive Even”, “Positive Odd”, “Negative Even”, or “Negative Odd”.
- The user is prompted to input a
number
. - The program first checks if the
number
is zero. If true, it prints “Zero”. If the number is not zero, it proceeds to check whether the number is positive or negative. - If the
number
is positive, the program further checks if it is even or odd using the modulus operator (%
).- If the number is divisible by 2, it prints “Positive Even”. If not, it prints “Positive Odd”.
- If the
number
is negative, the program similarly checks if it is even or odd.- If it is divisible by 2, it prints “Negative Even”, otherwise, it prints “Negative Odd”.
number = int(input("Enter a number: ")) if number == 0: print("Zero") else: if number > 0: if number % 2 == 0: print("Positive Even") else: print("Positive Odd") else: if number % 2 == 0: print("Negative Even") else: print("Negative Odd")
Hotel Room Price Calculator
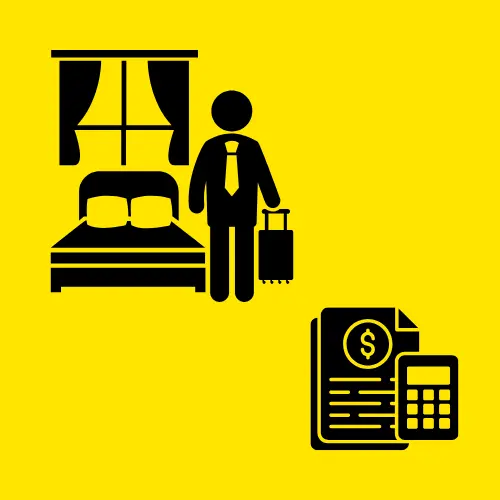
A hotel offers different room prices based on whether the customer is a member and how long they plan to stay. The pricing rules are as follows:
If the customer is a member: If they stay for more than 7 nights, they get a 25% discount.
If they stay for 7 nights or less, they get a 15% discount.
If the customer is not a member: If they stay for more than 7 nights, they get a 10% discount.
If they stay for 7 nights or less, they get no discount.
Write a Mojo program to calculate the room price after applying the discount based on the membership status and stay duration.
Test Case
Input:
Enter the room price: 200
Are you a member (yes/no): Yes
Enter the number of nights: 8
Expected Output:
Room Price after Discount: 150.0
Explanation:
This program calculates the final room price after applying a discount based on the user’s membership status and the stay duration.
- The user inputs the
price
of the room, whether they are amember
(Yes/No), and thestay_duration
(number of nights). - If the
membership
is “yes” (case-insensitive), the program checks if thestay_duration
is greater than 7 nights.- If true, a 25% discount is applied to the
price
. If the stay is 7 nights or fewer, a 15% discount is applied.
- If true, a 25% discount is applied to the
- If the
membership
is “no”, the program checks if thestay_duration
exceeds 7 nights.- If true, a 10% discount is applied. If the stay is 7 nights or fewer, no discount is given.
- The program then calculates the
final_price
by subtracting thediscount
from the originalprice
and prints thefinal_price
after discount.
price = float(input("Enter the room price: ")) membership = input("Are you a member (yes/no): ") stay_duration = int(input("Enter the number of nights: ")) if membership == "yes": if stay_duration > 7: discount = price * 0.25 else: discount = price * 0.15 else: if stay_duration > 7: discount = price * 0.10 else: discount = 0 final_price = price - discount print(f"Room Price after Discount: {final_price}")
Digital Clock Validity
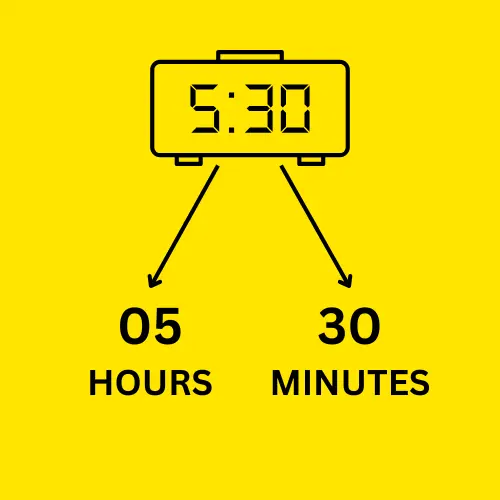
Write a Mojo program to check if a given time is valid, where the hour and minute are taken separately as inputs. The rules for a valid time are:
The hour must be between 00 and 23.
The minute must be between 00 and 59.
If both the hour and minute are valid, print “Valid Time”. Otherwise, print “Invalid Time”.
Test Case
Input:
Enter the hour (00-23): 23
Enter the minute (00-59): 45
Expected Output:
Valid Time
Explanation:
This program validates the time input provided by the user.
- The user is prompted to enter an
hour
(between 00 and 23) and aminute
(between 00 and 59). - The program first checks if the
hour
is within the valid range (0-23).- If this condition is true, it proceeds to check if the
minute
is also within the valid range (0-59). If both thehour
andminute
are valid, it prints “Valid Time”.
- If this condition is true, it proceeds to check if the
- If the
minute
is outside the valid range, it prints “Invalid Time: Minute must be between 00 and 59”. If thehour
is not within the valid range (0-23), the program prints “Invalid Time: Hour must be between 00 and 23”.
hour = int(input("Enter the hour (00-23): ")) minute = int(input("Enter the minute (00-59): ")) if hour >= 0 and hour <= 23: if minute >= 0 and minute <= 59: print("Valid Time") else: print("Invalid Time: Minute must be between 00 and 59.") else: print("Invalid Time: Hour must be between 00 and 23.")
Triangle Angle Validity
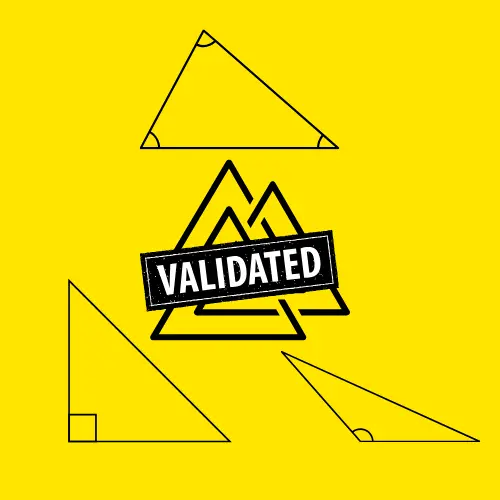
Write a program to check if three angles form a valid triangle and classify it based on the angles. The classifications are:
Acute Triangle: All angles are less than 90°.
Right Triangle: One angle is exactly 90°.
Obtuse Triangle: One angle is greater than 90°.
Conditions for a valid triangle:
The sum of the three angles must be 180°.
Each angle must be greater than 0°.
If the angles do not form a valid triangle, print “Invalid Triangle”.
Test Case
Input:
Enter the first angle: 60
Enter the second angle: 60
Enter the third angle: 60
Expected Output:
Acute Triangle
Explanation:
This program classifies a triangle based on the angles entered by the user.
- The user is asked to input three angles:
angle1
,angle2
, andangle3
. - The program first checks if the sum of the three angles equals 180 degrees.
- If the sum is valid, it proceeds to check if all the angles are greater than 0.
- If any angle is less than or equal to 0, it prints “Invalid Triangle: Angles must be greater than 0”.
- If all angles are valid, the program then checks the type of triangle:
- If
angle1 < 90 and angle2 < 90 and angle3 < 90
, it prints “Acute Triangle”. - If
angle1 == 90 or angle2 == 90 or angle3 == 90
, it prints “Right Triangle”. - If
angle1 > 90 or angle2 > 90 or angle3 > 90
, it prints “Obtuse Triangle”.
angle1 = int(input("Enter the first angle: ")) angle2 = int(input("Enter the second angle: ")) angle3 = int(input("Enter the third angle: ")) if angle1 + angle2 + angle3 == 180: if angle1 > 0 and angle2 > 0 and angle3 > 0: if angle1 < 90 and angle2 < 90 and angle3 < 90: print("Acute Triangle") elif angle1 == 90 or angle2 == 90 or angle3 == 90: print("Right Triangle") else: print("Obtuse Triangle") else: print("Invalid Triangle: Angles must be greater than 0.") else: print("Invalid Triangle: The sum of angles must be 180°.")
ATM Withdrawal System
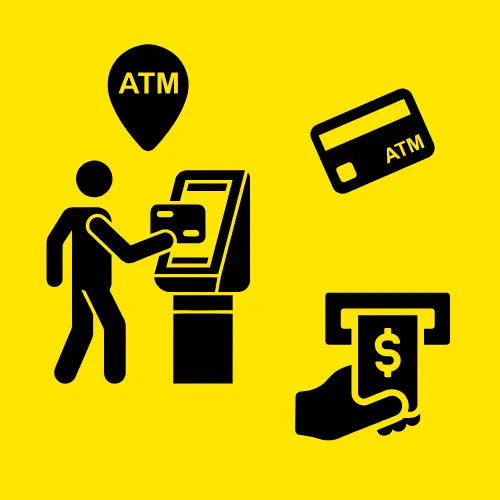
Write a Mojo program that simulates an ATM withdrawal system. The ATM dispenses cash in $50, $20, $10, and $5 denominations. The program should check if the requested withdrawal amount can be dispensed using these denominations.
Conditions:
The requested amount must be a multiple of $5.
The ATM must check if it is possible to dispense the exact requested amount using the available denominations.
The program will take the requested withdrawal amount as input and print out the number of bills for each denomination.
Test Case
Input:
Enter the amount to withdraw: 130
Expected Output:
2 x $50
1 x $20
1 x $10
Explanation:
This program determines how to withdraw a specified amount of money using available denominations of $50, $20, $10, and $5 bills.
- The user inputs an
amount
to withdraw. - The program first checks if the
amount
is a multiple of 5 using the conditionif amount % 5 == 0
.- If the amount is a valid multiple of 5, the program proceeds to calculate the number of bills required for each denomination. It uses integer division (
//
) to determine how many $50 bills can be dispensed by dividing theamount
by 50. - It then updates the remaining
amount
using the modulus operator (%
), which gives the remainder after dividing by 50. The same process is repeated for $20, $10, and $5 bills.
- If the amount is a valid multiple of 5, the program proceeds to calculate the number of bills required for each denomination. It uses integer division (
- After calculating the number of each bill, the program checks if all of the amount has been dispensed using
if amount == 0
.- If true, it prints the number of each denomination that will be dispensed.
- If any denomination is not used, it won’t be printed. If there is any remaining amount, the program prints “Amount cannot be dispensed”.
amount = int(input("Enter the amount to withdraw: ")) if amount % 5 == 0: # Denominations available fifty = amount // 50 amount %= 50 twenty = amount // 20 amount %= 20 ten = amount // 10 amount %= 10 five = amount // 5 amount %= 5 # Checking if all the amount was dispensed if amount == 0: if fifty > 0: print(f"{fifty} x $50") if twenty > 0: print(f"{twenty} x $20") if ten > 0: print(f"{ten} x $10") if five > 0: print(f"{five} x $5") else: print("Amount cannot be dispensed.") else: print("Amount must be a multiple of $5.")
Bank Account Operations
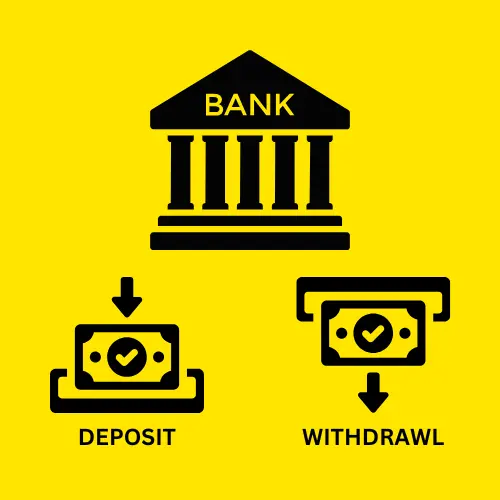
Write a Mojo program to simulate basic bank account operations. The program should allow the user to perform the following operations:
Deposit: Add money to the account balance.
Withdraw: Withdraw money from the account, but only if there is sufficient balance.
Check Balance: Display the current balance.
The program should execute each operation only once based on the user’s choice.
Test Case
Input:
Choose an option (1-3): 200
Expected Output:
Deposited: $200
Explanation:
This program simulates basic bank account operations such as deposit, withdrawal, and balance checking.
- Initially, the
balance
is set to 0. The user is presented with a menu containing three options: deposit (1), withdraw (2), and check balance (3). The program prompts the user to select an option (option
). - If the user selects option 1 (deposit), they are prompted to input a
deposit_amount
. The program then adds this amount to thebalance
usingbalance += deposit_amount
and prints the deposited amount. - If the user selects option 2 (withdraw), they are asked to input a
withdraw_amount
. The program checks if thewithdraw_amount
is less than or equal to thebalance
.- If true, the amount is subtracted from the
balance
usingbalance -= withdraw_amount
, and the program prints the withdrawn amount. If the withdrawal amount exceeds the current balance, it prints “Insufficient balance to withdraw that amount.”
- If true, the amount is subtracted from the
- If the user selects option 3 (check balance), the program simply prints the current
balance
.
# Initialize balance balance = 0 # Get user choice print("--- Bank Account Operations ---") print("1. Deposit") print("2. Withdraw") print("3. Check Balance") option = int(input("Choose an option (1-3): ")) if option == 1: # Deposit money deposit_amount = int(input("Enter the amount to deposit: ")) balance += deposit_amount print(f"Deposited: ${deposit_amount}") elif option == 2: # Withdraw money withdraw_amount = int(input("Enter the amount to withdraw: ")) if withdraw_amount <= balance: balance -= withdraw_amount print(f"Withdrawn: ${withdraw_amount}") else: print("Insufficient balance to withdraw that amount.") elif option == 3: # Check balance print(f"Current balance: ${balance}") else: print("Invalid option. Please choose a valid option.")
Dungeon Trap Decision
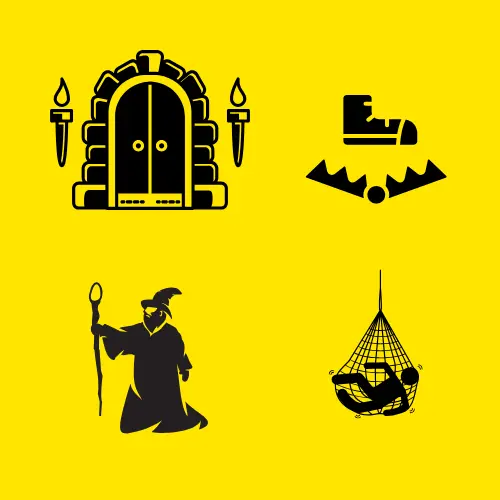
In a dungeon game, the player must decide their survival strategy based on the available items and their conditions:
If the player has a shield:
If the shield’s durability is greater than 50%, the player survives the trap with minimal damage.
If the shield’s durability is 50% or less, the player survives but takes moderate damage.
If the player does not have a shield:
If the player has a health potion:If the potion is strong, the player survives but takes moderate damage.
If the potion is weak, the player dies.
If no potion is available, the player dies instantly.
Write a Mojo program to decide the player’s fate based on the above conditions.
Test Case
Input:
Does the player have a shield? (Yes/No): Yes
Enter shield durability (%): 75
Expected Output:
Player survives with minimal damage.
Explanation:
This program determines whether a player survives an encounter based on their inventory and the condition of their items.
- The user is first asked if the player has a shield (
has_shield == "Yes"
orhas_shield == "No"
).- If the player has a shield, the program asks for its durability (
shield_durability
).
- If the player has a shield, the program asks for its durability (
- If the player does not have a shield (
has_shield == "No"
), the program checks if the player has a health potion (has_potion == "Yes"
orhas_potion == "No"
).- If the player has a potion, the program checks its strength (
potion_strength == "Yes"
orpotion_strength == "No"
).
- If the player has a potion, the program checks its strength (
- If
has_shield == "Yes"
, the program evaluates the shield’s durability:- If
shield_durability > 50
is True, the player survives with minimal damage. - If
shield_durability > 50
is False, the player survives but sustains moderate damage.
- If
- If
has_shield == "No"
:- If
has_potion == "Yes"
andpotion_strength == "Yes"
are both True, the player survives but takes moderate damage. - If
has_potion == "Yes"
andpotion_strength == "Yes"
is False, or ifhas_potion == "No"
, the player dies.
- If
has_shield = input("Does the player have a shield? (Yes/No): ") if has_shield == "Yes": shield_durability = int(input("Enter shield durability (%): ")) else: has_potion = input("Does the player have a health potion? (Yes/No): ") if has_potion == "Yes": potion_strength = input("Is the potion strong? (Yes/No): ") if has_shield == "Yes": if shield_durability > 50: print("Player survives with minimal damage.") else: print("Player survives but takes moderate damage.") else: if has_potion == "Yes": if potion_strength == "Yes": print("Player survives but takes moderate damage.") else: print("Player dies.") else: print("Player dies.")
Battle Arena Damage Calculator
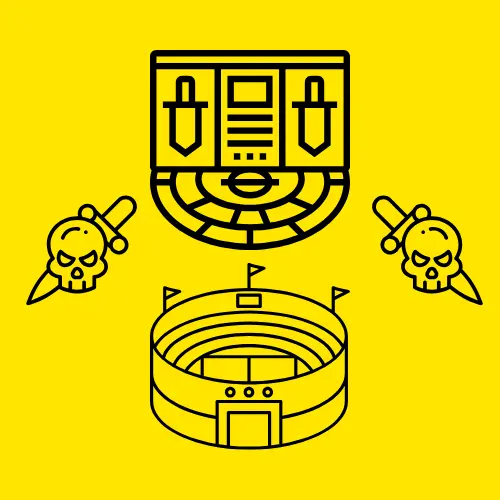
In a turn-based battle game, calculate the final damage dealt by a player’s attack. The rules are as follows:
If the attack type is “Physical”:
Calculate a base damage as strength * 2
.
If the base damage is greater than 100:Subtract the bonus multiplier from the base damage and divide it by 2.
If the base damage is 100 or less: Add 20 to the base damage.
If the attack type is “Ranged”:
Calculate a base damage as (strength + bonus multiplier) * 1.5
.
If the base damage is greater than 150:Multiply the base damage by 0.8 and subtract 10.
If the base damage is 150 or less: Add 15 to the base damage.
If the attack type is “Magical”:
Calculate a base damage as strength ** 2
.
If the base damage exceeds 2000: Subtract 100 and divide it by the bonus multiplier (if the multiplier is non-zero).
If the base damage is 2000 or less: Add bonus multiplier * 2
to the base damage.
Write a Mojo program to calculate the final damage using the above rules.
Test Case
Input:
Enter attack type (Physical/Ranged/Magical): Physical
Enter player strength: 60
Enter bonus multiplier: 10
Expected Output:
Final damage dealt: 115
Explanation:
In this program, the type of attack (Physical, Ranged, or Magical) and the player’s strength and bonus multiplier are used to calculate the final damage dealt.
- The program asks for three inputs:
attack_type
: The type of attack (Physical, Ranged, or Magical).strength
: The player’s strength value.bonus_multiplier
: A value representing a bonus multiplier.
- For a Physical attack, the program calculates the
base_damage
asstrength * 2
.- If
base_damage
is greater than 100, thefinal_damage
is calculated by subtracting thebonus_multiplier
frombase_damage
and dividing the result by 2. Ifbase_damage
is 100 or less, 20 is added tobase_damage
to get thefinal_damage
.
- If
- For a Ranged attack, the program calculates
base_damage
as(strength + bonus_multiplier) * 1.5
.- If
base_damage
is greater than 150, thefinal_damage
is reduced by 20% and 10 is subtracted. Ifbase_damage
is 150 or less, 15 is added tobase_damage
to get thefinal_damage
.
- If
- For a Magical attack, the
base_damage
is calculated asstrength ** 2
.- If
base_damage
is greater than 2000, the program adjusts thefinal_damage
based on thebonus_multiplier
. - If
bonus_multiplier
is non-zero, the program divides(base_damage - 100)
by thebonus_multiplier
. - If
bonus_multiplier
is zero, 100 is subtracted frombase_damage
. Ifbase_damage
is 2000 or less,final_damage
is calculated by addingbonus_multiplier * 2
tobase_damage
.
- If
attack_type = input("Enter attack type (Physical/Ranged/Magical): ") strength = int(input("Enter player strength: ")) bonus_multiplier = int(input("Enter bonus multiplier: ")) if attack_type == "Physical": base_damage = strength * 2 if base_damage > 100: final_damage = (base_damage - bonus_multiplier) // 2 else: final_damage = base_damage + 20 elif attack_type == "Ranged": base_damage = (strength + bonus_multiplier) * 1.5 if base_damage > 150: final_damage = (base_damage * 0.8) - 10 else: final_damage = base_damage + 15 elif attack_type == "Magical": base_damage = strength ** 2 if base_damage > 2000: if bonus_multiplier != 0: final_damage = (base_damage - 100) // bonus_multiplier else: final_damage = base_damage - 100 else: final_damage = base_damage + (bonus_multiplier * 2) print(f"Final damage dealt: {final_damage}")
Salary and Bonus Calculation
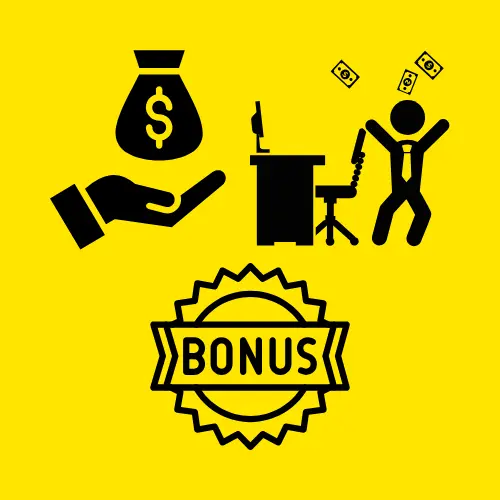
Write a program to calculate the bonus based on the following conditions:
Experience:
If experience < 3 years: No bonus.
If experience ≥ 3 years but < 10 years: Calculate bonus as 5% of base salary.
If the base salary is greater than $50,000, add a 2% extra bonus.
If the salary is between $50,000 and $75,000, add a 1% extra bonus.
If experience ≥ 10 years: Calculate bonus as 10% of base salary.
If the base salary is greater than $100,000, add a 5% extra bonus.
If the salary is between $100,000 and $150,000, add a 3% extra bonus.
If experience is above 15 years, add an additional 2% bonus for loyalty.
Performance Rating:
If the rating is above 4, add 3% of the bonus.
If the rating is exactly 4, add 2% of the bonus.
If the rating is below 3, reduce the bonus by 5%.
Test Case
Input:
Enter years of experience: 8
Enter base salary: 60000
Enter performance rating: 4
Expected Output:
Final bonus: $3300
Explanation:
This program calculates the final bonus based on an employee’s experience
, base_salary
, and performance_rating
.
- First, it asks for three inputs: the
experience
(in years), thebase_salary
, and theperformance_rating
. - For the bonus calculation based on experience, if
experience
is less than 3 years, no bonus is given. Ifexperience
is between 3 and 10 years, a base bonus of 5% of thebase_salary
is added. If thebase_salary
is greater than $50,000, an additional 2%base_salary
is added to the bonus. If thebase_salary
is between $50,000 and $75,000, an additional 1% is added. For employees with more than 10 years of experience, the bonus starts at 10% ofbase_salary
. If thebase_salary
is greater than $100,000, an additional 5% is added, and if thebase_salary
is between $100,000 and $150,000, an additional 3% is added. For employees with over 15 years of experience, an extra 2%base_salary
is added to the bonus. - Next, the program adjusts the bonus based on the
performance_rating
. If theperformance_rating
is greater than 4, the bonus increases by 3%. If it equals 4, the bonus increases by 2%. If theperformance_rating
is below 3, the bonus is reduced by 5%.
experience = int(input("Enter years of experience: ")) base_salary = float(input("Enter base salary: ")) performance_rating = float(input("Enter performance rating: ")) if experience < 3: bonus = 0 elif experience >= 3 and experience < 10: bonus = base_salary * 0.05 if base_salary > 50000: bonus += base_salary * 0.02 elif base_salary >= 50000 and base_salary <= 75000: bonus += base_salary * 0.01 elif experience >= 10: bonus = base_salary * 0.10 if base_salary > 100000: bonus += base_salary * 0.05 elif base_salary >= 100000 and base_salary <= 150000: bonus += base_salary * 0.03 if experience > 15: bonus += base_salary * 0.02 # Adjust the bonus based on performance rating if performance_rating > 4: bonus += bonus * 0.03 elif performance_rating == 4: bonus += bonus * 0.02 elif performance_rating < 3: bonus -= bonus * 0.05 print(f"Final bonus: ${bonus:.2f}")
Job Candidate Eligibility Checker
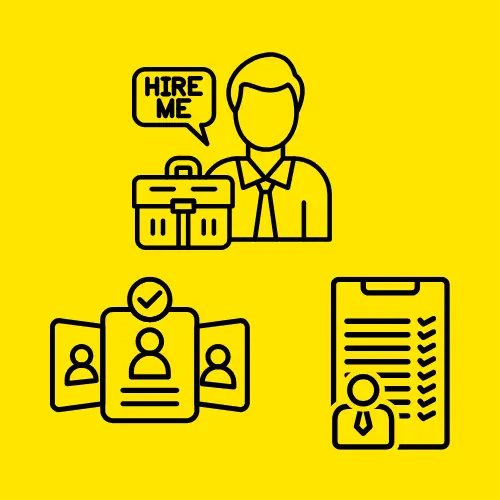
A company is hiring candidates for a technical role based on the following criteria:
Degree:
Candidates must hold either a Bachelor’s or Master’s degree in a relevant field.
CGPA:
For a Bachelor’s degree, candidates must have a CGPA of 3.5 or above.
For a Master’s degree, candidates must have a CGPA of 3.0 or above.
Skill Set:
Candidates must possess at least 3 out of the following 5 required skills:
Python, C++, Machine Learning, Data Analysis, DevOps
Experience:
If the candidate has 2 or more years of experience, they are eligible for an interview directly.
If the candidate has less than 2 years of experience, they need to complete an online test to qualify for the interview.
Task:
Write a Mojo program that checks if a candidate is eligible for the interview based on the following inputs:
Degree: Bachelor’s or Master’s
CGPA: The candidate’s CGPA score
Skills: Three skills from the list
Experience: The number of years of experience the candidate has
Test Case
Input:
Enter degree type (Bachelor’s/Master’s): Bachelor’s
Enter CGPA: 3.6
Enter years of experience: 1
Enter first skill: Python
Enter second skill: DataAnalysis
Enter third skill: MachineLearning
Output:
Candidate must complete an online test to qualify for the interview.
Explanation:
This Mojo code evaluates the eligibility of a candidate for an interview based on their degree, CGPA, years of experience, and required skills
- The program begins by taking input values for
degree
,CGPA
,experience
, and three skills. - First, it checks if the
degree
is either Bachelor’s or Master’s. If thedegree
is valid, it then checks whether the candidate’sCGPA
meets the required minimum: a CGPA of 3.5 for a Bachelor’s degree or 3.0 for a Master’s degree. If this condition is satisfied, the program proceeds to check if the candidate possesses the required skills. The required skills are: Python, C++, MachineLearning, DataAnalysis, or DevOps. All three skills provided by the candidate (skill1
,skill2
,skill3
) must be one of these required skills. - If the candidate’s skills match, the program checks their
experience
. If the candidate has at least 2 years of experience, they are considered eligible for the interview. If their experience is less than 2 years, they are required to complete an online test to qualify for the interview. - If any condition fails, the program prints an appropriate message: the candidate either doesn’t meet the skill set requirement, doesn’t meet the
CGPA
threshold, or doesn’t have a relevant degree.
degree = input("Enter degree type (Bachelor's/Master's): ") cgpa = float(input("Enter CGPA: ")) experience = int(input("Enter years of experience: ")) // Taking each skill one by one skill1 = input("Enter first skill: ") skill2 = input("Enter second skill: ") skill3 = input("Enter third skill: ") // Eligibility check if degree == "Bachelor's" or degree == "Master's": if (degree == "Bachelor's" and cgpa >= 3.5) or (degree == "Master's" and cgpa >= 3.0): // Skill validation: Check if at least 3 required skills match if (skill1 == "Python" or skill1 == "C++" or skill1 == "MachineLearning" or skill1 == "DataAnalysis" or skill1 == "DevOps") and \ (skill2 == "Python" or skill2 == "C++" or skill2 == "MachineLearning" or skill2 == "DataAnalysis" or skill2 == "DevOps") and \ (skill3 == "Python" or skill3 == "C++" or skill3 == "MachineLearning" or skill3 == "DataAnalysis" or skill3 == "DevOps"): // Experience check if experience >= 2: print("Candidate is eligible for the interview.") else: print("Candidate must complete an online test to qualify for the interview.") else: print("Candidate does not have the required skill set.") else: print("Candidate's CGPA does not meet the minimum requirement.") else: print("Candidate's degree is not in a relevant field.")
International Purchase Price Calculation
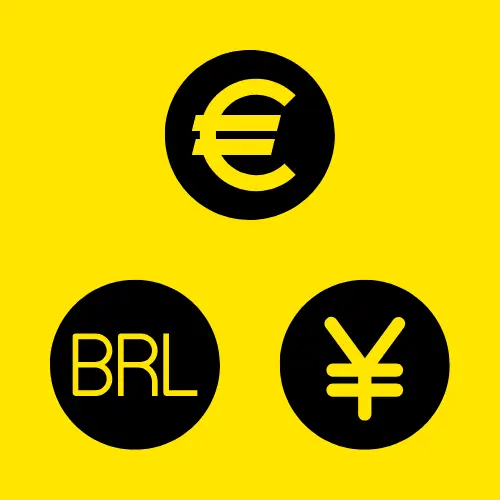
A customer is making an international purchase, and you need to calculate the final price based on various conditions. Write a program that calculates the final price for a customer based on the following conditions:
Product Price:
The customer enters the product price in their local currency.
Customer’s Location:
If the customer is from Europe, the price will be in Euros.
If the customer is from Asia, the price will be in Yen.
If the customer is from South America, the price will be in Brazilian Real.
Currency Conversion Rates:
EUR to USD Conversion Rate: 1 USD = 0.85 EUR.
JPY to USD Conversion Rate: 1 USD = 110 JPY.
BRL to USD Conversion Rate: 1 USD = 5.3 BRL.
Exchange Rate Adjustments:
If the customer is from Europe and their purchase is over €200, apply a 5% surcharge on the price.
If the customer is from Asia and their purchase exceeds ¥15,000, apply a 10% discount on the price.
If the customer is from South America and their purchase exceeds R$500, apply a 15% discount on the price.
Payment Method:
If the customer uses a credit card, add a 3% processing fee.
If the customer uses PayPal, add a 2% processing fee.
Final Price Calculation:
If the final price after adjustments exceeds $500, add a flat $25 shipping fee.
Invalid Inputs:
If the customer enters an invalid location or payment method, output an appropriate error message.
Your task:
Write a Mojo program to calculate the final price for the customer based on these conditions. The program should:Take input for the product price, location, and payment method.
Perform all the necessary calculations and adjustments.
Output the final price in USD.
Test Case
Input:
Enter product price: 250
Enter customer’s location (Europe/Asia/South America): Europe
Enter payment method (credit card/PayPal): credit card
Output:
Final price in USD: $296.06
Explanation:
The provided code calculates the final price of a product in USD, adjusting for the location, payment method, and any additional fees.
- Location Conversion: Based on the customer’s location (
location
), the code converts the product price to USD using specific conversion rates. For customers in Europe, the price is converted from Euros to USD using the conversion rateEUR_to_USD
. If the product price exceeds 200 Euros, a 5% increase is applied to the USD price. For Asia, the conversion from Yen (JPY) to USD is done with the rateJPY_to_USD
, and if the price exceeds 15,000 Yen, a 10% discount is applied. For South America, the conversion from Brazilian Real (BRL) to USD is done using the rateBRL_to_USD
, and if the price exceeds 500 BRL, a 15% discount is applied. If the location is invalid, an error message is printed. - Payment Method Processing Fee: The payment method (
payment_method
) applies a processing fee. If the payment method is credit card, a 3% fee is added to the price in USD. For PayPal, a 2% fee is added. If an invalid payment method is entered, an error message is printed. - Shipping Fee Adjustment: After converting to USD and applying any necessary payment fees, if the resulting price exceeds $500, a $25 shipping fee is added to the final price.
product_price = float(input("Enter product price: ")) location = input("Enter customer's location (Europe/Asia/South America): ") payment_method = input("Enter payment method (credit card/PayPal): ") # Conversion Rates EUR_to_USD = 1 / 0.85 JPY_to_USD = 1 / 110 BRL_to_USD = 1 / 5.3 # Initial price in USD if location == "europe": price_in_usd = product_price * EUR_to_USD if product_price > 200: price_in_usd *= 1.05 elif location == "asia": price_in_usd = product_price * JPY_to_USD if product_price > 15000: price_in_usd *= 0.90 elif location == "south america": price_in_usd = product_price * BRL_to_USD if product_price > 500: price_in_usd *= 0.85 else: print("Invalid location.") # Payment Method Processing Fee if payment_method == "credit card": price_in_usd *= 1.03 elif payment_method == "paypal": price_in_usd *= 1.02 else: print("Invalid payment method.") # Final Price Adjustment based on Shipping Fee if price_in_usd > 500: price_in_usd += 25 print(f"Final price in USD: ${price_in_usd:.2f}")
Question Validation System
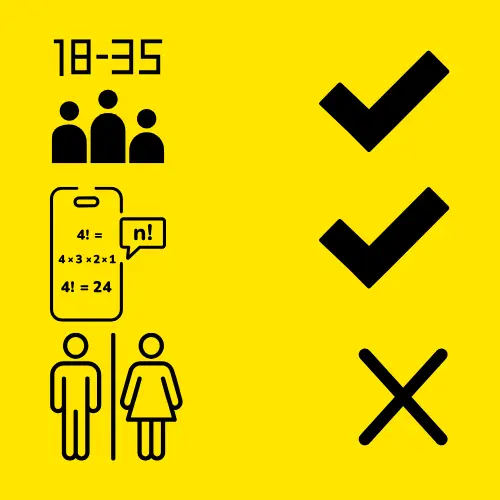
You are designing a validation and quiz system for a new online course platform. The system needs to verify certain user inputs and provide a short quiz with a feedback system. Your task is to implement the following:
Age Validation:
The system should accept the user’s age as input. It should only accept ages between 18 and 100. If the age is valid, proceed to the next step; otherwise, display an error message.
Gender Validation:
After validating the age, prompt the user for their gender. The user should choose one of the following options: “male”, “female”, or “other”. If the gender is valid, proceed to the next step; otherwise, display an error message.
Quiz Question:
Once the gender is validated, present the user with a multiple-choice question about geography:
What is the capital of France? a) Berlin b) Madrid c) Paris d) Rome
The user should choose one of the options. If the answer is correct display a congratulatory message. If the answer is incorrect, display the correct answer.
Factorial Question:
After the quiz, ask the user for the factorial of a number: If the gender is “male”, ask for the factorial of 5.
If the gender is “female”, ask for the factorial of 6.
If the gender is “other”, ask for the factorial of 2.
Validate if the user’s response is correct. If correct, print “Correct!” along with the factorial value; otherwise, display the correct value.
Test Case
Input:
Enter your age (18-100): 30
Enter your gender (male/female/other): abc
Output:
Invalid gender! Please choose ‘male’, ‘female’, or ‘other’.
Explanation:
This program is designed to validate the user’s input based on age, gender, and answers to a quiz.
- The program first asked the user’s age in
age
. It checks ifage
is between 18 and 100. If the condition18 <= age <= 100
is true, the program proceeds. Otherwise, it outputs"Invalid age!"
. - The user’s gender is captured in
gender
. Based on this value, the program:- If
gender
is"male"
, it proceeds with a quiz question and checks the answer stored inanswer
. It also checks the factorial input usingfactorial_input
and compares it to 120 (for factorial of 5). - If
gender
is"female"
, a similar quiz and factorial check occurs, but the factorial input (factorial_input
) is checked against 720 (for factorial of 6). - If
gender
is"other"
, the quiz question is asked again, and the factorial question is checked withfactorial_input
against 2 (for factorial of 2).
- If
- For the quiz, the user must select the correct option (which is
"c"
for “Paris”). If theanswer
is"c"
, the program prints a success message; otherwise, it indicates the incorrect answer. - Depending on the gender, the program asks a factorial question. If the
factorial_input
is correct for the respective gender (120 for male, 720 for female, and 2 for other), the program prints a success message. - If an invalid
gender
is entered (not"male"
,"female"
, or"other"
), the program prints"Invalid gender!"
. If theage
is invalid (outside the range 18-100), it prints"Invalid age!"
.
# Step 1: Age Validation age = int(input("Enter your age (18-100): ")) if age >= 18 and age <= 100: print("Age is valid.") # Step 2: Gender Validation gender = input("Enter your gender (male/female/other): ").lower() if gender == "male": print("Gender is valid.") # Step 3: Quiz Question print("\nWhat is the capital of France?") print("a) Berlin") print("b) Madrid") print("c) Paris") print("d) Rome") answer = input("Choose your answer (a/b/c/d): ").lower() if answer == "a" or answer == "b" or answer == "d": print("Incorrect! The correct answer is 'c'.") elif answer == "c": print("Correct! Paris is the capital of France.") else: print("Invalid choice! Please choose 'a', 'b', 'c', or 'd'.") # Step 4: Factorial Question for Male factorial_input = int(input("What is the factorial of 5? ")) if factorial_input == 120: print("Correct!") else: print("The correct answer is 120.") elif gender == "female": print("Gender is valid.") # Step 3: Quiz Question print("\nWhat is the capital of France?") print("a) Berlin") print("b) Madrid") print("c) Paris") print("d) Rome") answer = input("Choose your answer (a/b/c/d): ").lower() if answer == "a" or answer == "b" or answer == "d": print("Incorrect! The correct answer is 'c'.") elif answer == "c": print("Correct! Paris is the capital of France.") else: print("Invalid choice! Please choose 'a', 'b', 'c', or 'd'.") # Step 4: Factorial Question for Female factorial_input = int(input("What is the factorial of 6? ")) if factorial_input == 720: print("Correct!") else: print("The correct answer is 720.") elif gender == "other": print("Gender is valid.") # Step 3: Quiz Question print("\nWhat is the capital of France?") print("a) Berlin") print("b) Madrid") print("c) Paris") print("d) Rome") answer = input("Choose your answer (a/b/c/d): ").lower() if answer == "a" or answer == "b" or answer == "d": print("Incorrect! The correct answer is 'c'.") elif answer == "c": print("Correct! Paris is the capital of France.") else: print("Invalid choice! Please choose 'a', 'b', 'c', or 'd'.") # Step 4: Factorial Question for Other factorial_input = int(input("What is the factorial of 2? ")) if factorial_input == 2: print("Correct!") else: print("The correct answer is 2.") else: print("Invalid gender! Please choose 'male', 'female', or 'other'.") else: print("Invalid age! Please enter an age between 18 and 100.")
Zodiac Sign Finder & Prediction
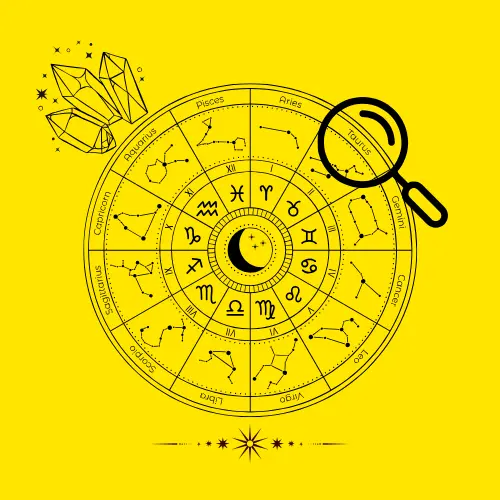
Create a program that takes the user’s birthdate (day and month) as input and determines their Zodiac sign. Based on the user’s Zodiac sign and the day of the month, the program should provide a prediction and a lucky number calculation.
Zodiac Sign: The program should first determine the user’s Zodiac sign based on their birthdate.
Prediction & Calculation: After determining the Zodiac sign, the program should print a prediction based on the specific range of the day (1-10, 11-15, or 16-31) for that sign. Additionally, the program should output a “lucky number” and a fun calculation based on the day and sign.
Zodiac Signs and Date Ranges:
Aries: March 21 – April 19
Taurus: April 20 – May 20
Gemini: May 21 – June 20
Leo: July 23 – August 22
Virgo: August 23 – September 22
Input:
Enter the day of your birth (1-31): 8
Enter the month of your birth (1-12): 4
Output:
Your Zodiac Sign is Aries.
Prediction: The first part of the month will bring new beginnings. Trust in your strength!
Lucky number: 7
Today’s fun calculation: 15 is your lucky sum!
Explanation:
This program is designed to determine a user’s zodiac sign based on their birthdate and provide a daily prediction, lucky number, and fun calculation.
- The program starts by asking the user for their birthdate. The
day
(day of the month) andmonth
(numerical month) are entered as inputs. - The program checks the user’s birthdate using multiple
if-elif
conditions to assign a zodiac sign. For example:- If
month == 3
andday >= 21
ormonth == 4
andday <= 19
, the sign is Aries. - The program continues similarly for other zodiac signs like Taurus, Gemini, Leo, and Virgo, checking the respective day and month conditions.
- If
- Depending on the exact day within the sign’s date range, the program assigns different predictions and lucky numbers:
- If
day <= 10
, it may give a prediction like “new beginnings” for Aries and provide a calculation based on7 + day
. - For days between 11 and 15, the program will offer a new prediction, like personal growth, and provide a different calculation (e.g.,
9 * day
for Aries). - If the day is after the 15th, the program offers a more calming prediction and uses calculations like
4 + day
for Aries.
- If
- Each zodiac sign has its own “fun calculation” that is based on the
day
of birth. For example:- Aries (if day <= 10) has a calculation of
7 + day
. - Taurus (if day <= 10) uses
5 * month
. - Gemini (if day <= 10) uses
day - 3
, and so on.
- Aries (if day <= 10) has a calculation of
- After determining the zodiac sign and providing the prediction, lucky number, and calculation, the program outputs the result for the user.
# Step 1: Input birthdate day = int(input("Enter the day of your birth (1-31): ")) month = int(input("Enter the month of your birth (1-12): ")) # Step 2: Determine zodiac sign based on birthdate and provide prediction and calculation if (month == 3 and day >= 21) or (month == 4 and day <= 19): zodiac_sign = "Aries" print(f"Your Zodiac Sign is {zodiac_sign}.") if day <= 10: # Nested condition for Aries print("Prediction: The first part of the month will bring new beginnings. Trust in your strength!") print("Lucky number: 7") print(f"Today's fun calculation: {7 + day} is your lucky sum!") elif day <= 15: print("Prediction: A time for personal growth and discovery. Take on challenges headfirst!") print("Lucky number: 9") print(f"Today's fun calculation: {9 * day} is your lucky product!") else: print("Prediction: The second half of the month favors calm decision-making. Stay focused!") print("Lucky number: 4") print(f"Today's fun calculation: {4 + day} is your lucky sum!") elif (month == 4 and day >= 20) or (month == 5 and day <= 20): zodiac_sign = "Taurus" print(f"Your Zodiac Sign is {zodiac_sign}.") if day <= 10: print("Prediction: Patience will be your greatest ally in the first half of the month.") print("Lucky number: 5") print(f"Today's fun calculation: {5 * month} is your lucky product!") elif day <= 15: print("Prediction: Focus on your goals and use your strong willpower to make steady progress.") print("Lucky number: 2") print(f"Today's fun calculation: {2 * day} is your lucky product!") else: print("Prediction: The latter part of the month invites calmness, helping you relax and recharge.") print("Lucky number: 8") print(f"Today's fun calculation: {8 + day} is your lucky sum!") elif (month == 5 and day >= 21) or (month == 6 and day <= 20): zodiac_sign = "Gemini" print(f"Your Zodiac Sign is {zodiac_sign}.") if day <= 10: print("Prediction: Social interactions are favored in the early part of the month. You will meet new people!") print("Lucky number: 3") print(f"Today's fun calculation: {day - 3} is your lucky difference!") elif day <= 15: print("Prediction: A time for creative ideas. Let your imagination run wild!") print("Lucky number: 6") print(f"Today's fun calculation: {6 * day} is your lucky product!") else: print("Prediction: The later half of the month brings wisdom and introspection. Take some time for yourself.") print("Lucky number: 7") print(f"Today's fun calculation: {7 + day} is your lucky sum!") elif (month == 7 and day >= 23) or (month == 8 and day <= 22): zodiac_sign = "Leo" print(f"Your Zodiac Sign is {zodiac_sign}.") if day <= 10: print("Prediction: The start of the month brings confidence and leadership. Take charge of opportunities!") print("Lucky number: 9") print(f"Today's fun calculation: {9 * day} is your lucky product!") elif day <= 15: print("Prediction: You will shine brighter with your creativity. Embrace your uniqueness!") print("Lucky number: 5") print(f"Today's fun calculation: {5 + day} is your lucky sum!") else: print("Prediction: The second half of the month may bring challenges. Use your wit and courage to overcome them.") print("Lucky number: 3") print(f"Today's fun calculation: {3 * day} is your lucky product!") elif (month == 8 and day >= 23) or (month == 9 and day <= 22): zodiac_sign = "Virgo" print(f"Your Zodiac Sign is {zodiac_sign}.") if day <= 10: print("Prediction: Early in the month, your focus and attention to detail will be your superpower.") print("Lucky number: 4") print(f"Today's fun calculation: {4 + day} is your lucky sum!") elif day <= 15: print("Prediction: Mid-month brings clarity. Trust your instincts and make important decisions.") print("Lucky number: 7") print(f"Today's fun calculation: {7 - day} is your lucky difference!") else: print("Prediction: Later in the month, you'll find peace through organization and simplicity.") print("Lucky number: 6") print(f"Today's fun calculation: {6 * day} is your lucky product!") else: print("Sorry, we couldn't determine your zodiac sign from the provided date.")
Nested if-else coding questions in Mojo test a candidate’s ability to handle complex decision-making. They challenge problem-solving skills and help sharpen logical thinking, ensuring candidates can write efficient and effective code for real-world scenarios.