Practicing if-else statements in Mojo helps you learn how to control the flow of your programs based on different conditions. These problems cover situations such as checking if a student is eligible for a discount, determining if someone qualifies for a special offer, or assigning grades based on marks. With clear explanations and examples, you can see how if-else statements allow programs to make decisions, making it easier to manage various cases. By solving these problems, you can improve your ability to write programs that respond dynamically to different inputs.
Check Student Grade
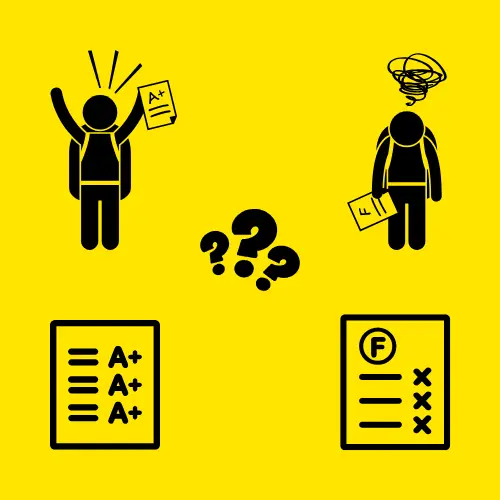
A teacher wants to assign grades to students based on their marks:
90 and above: A
80 to 89: B
70 to 79: C
Below 70: Fail
Write a Mojo program to assign grades easily.
Test Case:
Input:
Enter your marks: 85
Expected Output:
Your grade is: B
Explanation:
This program determines a student’s grade based on the marks they input. It uses simple conditions to categorize the marks into grades like A, B, C, or Fail.
- The program starts by asking the user to enter their marks with
marks = int(input("Enter your marks: "))
. The entered value is stored in themarks
variable as an integer. - Checking for Grade ‘A’: The program then checks if the marks are greater than or equal to 90 using the condition
if marks >= 90
. If this condition is true, it printsYour grade is: A
. - Checking for Grade ‘B’: If the previous condition is false, the program moves to the next condition with
elif marks >= 80
. This checks if the marks are greater than or equal to 80. If this is true, it printsYour grade is: B
. - Checking for Grade ‘C’: If both the previous conditions are false, the program then checks for the
elif marks >= 70
condition. If the marks are greater than or equal to 70, it printsYour grade is: C
. - Failing Condition: If none of the above conditions are true (i.e., the marks are less than 70), the program reaches the
else
statement and printsYour grade is: Fail
.
marks = int(input("Enter your marks: ")) if marks >= 90: print("Your grade is: A") elif marks >= 80: print("Your grade is: B") elif marks >= 70: print("Your grade is: C") else: print("Your grade is: Fail")
Check Voting Eligibility
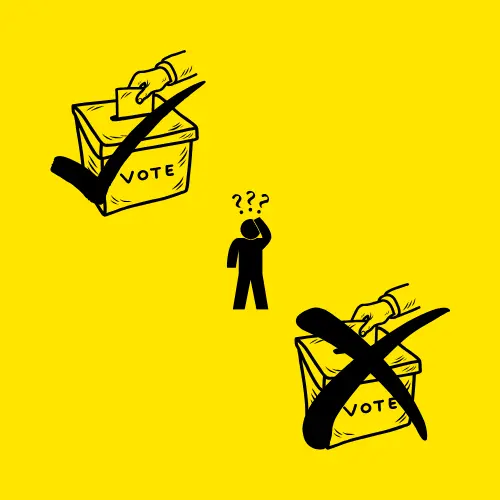
A person is eligible to vote if their age is between 18 and 100 years. Write a program to check if the person is eligible to vote based on their age.
Test Case:
Input:
Enter your age: 20
Expected Output:
Eligible to vote.
Explanation:
This program is designed to check whether the user is eligible based on age.
- The program begins by prompting the user to input their age. The entered age is stored in the
age
variable as an integer. - The program then checks if the
age
is between 18 and 100 using the conditionif age >= 18 and age <= 100:
.- If the condition is true, meaning the user’s age is within this range, it prints
"Eligible to vote."
. - If the condition is false, meaning the age is either below 18 or above 100, it prints
"Not eligible to vote."
.
- If the condition is true, meaning the user’s age is within this range, it prints
age = int(input("Enter your age: ")) # Check if age is between 18 and 100 if age >= 18 and age <= 100: print("Eligible to vote.") else: print("Not eligible to vote.")
Is it Even or Odd?
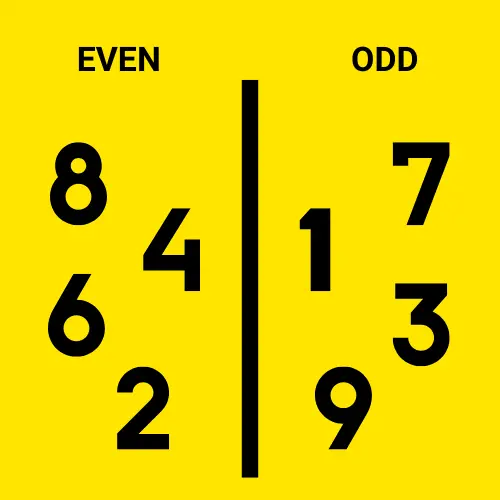
A bakery makes cupcakes with even-numbered toppings. Write a Mojo program to check if the given number of toppings is even or odd.
Test Case:
Input:
Enter the number of toppings: 7
Expected Output:
Odd
Explanation:
This program checks whether the number of toppings the user enters is even or odd.
- First, it asks the user to input the number of toppings, which is stored in the variable
toppings
. - The program then uses an
if
statement to check if the number of toppings is even by calculating the remainder whentoppings
is divided by 2 (toppings % 2
).- If the remainder is 0, it means the number of toppings is even, and the program prints
Even
. - Otherwise, it executes the
else
block and printsOdd
, indicating that the number of toppings is not divisible by 2.
- If the remainder is 0, it means the number of toppings is even, and the program prints
toppings = int(input("Enter the number of toppings: ")) if toppings % 2 == 0: print("Even") else: print("Odd")
Light Intensity Checker
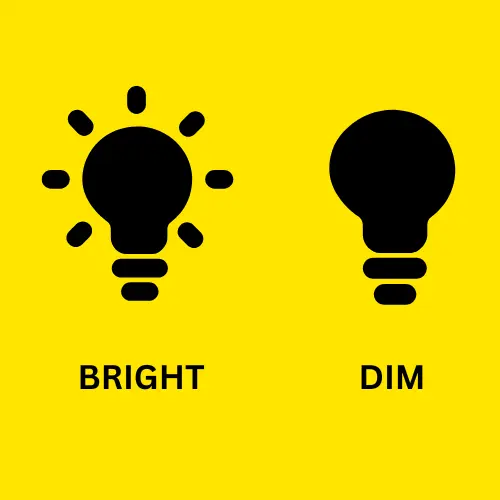
A smart light adjusts its brightness based on intensity levels. If the level is above 50, the light is “Bright” otherwise, it is “Dim.” Write a Mojo program for it.
Test Case:
Input:
Enter the light intensity: 45
Expected Output:
Dim
Explanation:
This program determines whether the light is “Bright” or “Dim” based on the intensity entered by the user.
- First, it prompts the user to input the value of light intensity, which is stored in the variable
intensity
- Next, the program uses an
if
statement to check if the value ofintensity
is greater than 50. If this condition is true (i.e., the light intensity is more than 50), it printsBright
. - If the condition is false (i.e., the intensity is 50 or less), the program moves to the
else
block and printsDim
.
intensity = int(input("Enter the light intensity: ")) if intensity > 50: print("Bright") else: print("Dim")
Parking Fee Calculator
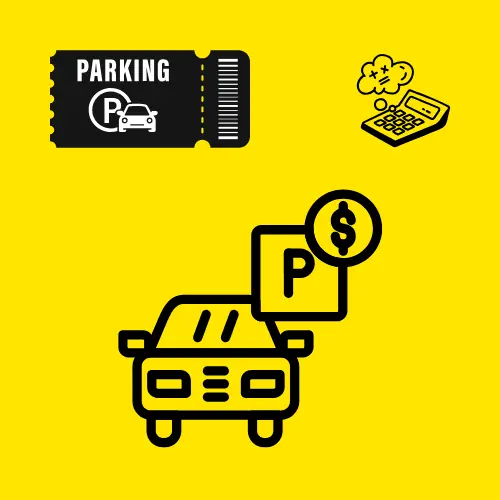
A parking lot charges $20 if the parking time is more than 2 hours, otherwise, it’s free. Write a Mojo program to find out whether parking is free or not.
Test Case:
Input:
Enter parking hours: 3
Expected Output:
You have been charged 20 dollars.
Explanation:
This program calculates the parking charges based on the number of hours a vehicle is parked.
- It first asks the user to input the parking hours. The entered value is stored in the variable
hours
. - The program then uses an
if
statement to check if the value ofhours
is greater than 2.- If the condition
hours > 2
is true, it means the parking duration exceeds the free parking limit, and the program prints the messageYou have been charged 20 dollars.
- If the condition is false, the program proceeds to the
else
block and printsYou have been charged 0 dollars.
- If the condition
hours = int(input("Enter parking hours: ")) if hours > 2: print("You have been charged 20 dollars.") else: print("You have been charged 0 dollars.")
Train Ticket Discount
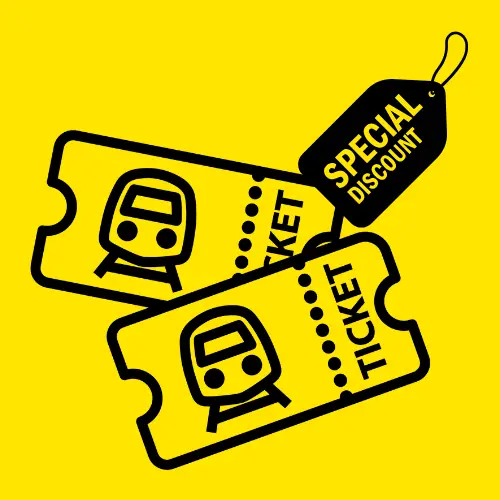
A train offers a 10% discount for passengers aged below 12 or above 60. Write a Mojo program to calculate the ticket price after applying the discount (if applicable). The ticket price is $100.
Test Case:
Input:
Enter your age: 65
Expected Output:
Discounted price: 90
Explanation:
This program calculates the ticket price for a train ride based on the passenger’s age and whether they qualify for a discount.
- The variable
base_price
is set to100
, representing the standard ticket price. The variablediscount_percentage
is set to10
, meaning passengers who qualify will get a 10% discount. - The program asks the user to enter their age. The input is stored in the
age
variable. - The program uses an
if
statement to check if the enteredage
is less than 12 or greater than 60. This is done using the conditionif age < 12 or age > 60:
- If the condition is true, the program calculates the discounted price. The discounted price is stored in the variable
discounted_price
using formula. The formuladiscounted_price = base_price - (base_price * discount_percentage // 100)
calculates the discounted price in two steps. First, it computes the discount amount by multiplying thebase_price
by thediscount_percentage
and dividing by 100. Then, it subtracts this discount from thebase_price
to get the finaldiscounted_price
. - If the condition is false, the program simply prints the full ticket price stored in
base_price
.
- If the condition is true, the program calculates the discounted price. The discounted price is stored in the variable
base_price = 100 discount_percentage = 10 age = Int(input("Enter your age: ")) if age < 12 or age > 60: discounted_price = base_price - (base_price * discount_percentage // 100) print("Discounted price: ", discounted_price) else: print("Full price: ", base_price)
Leap Year Checker
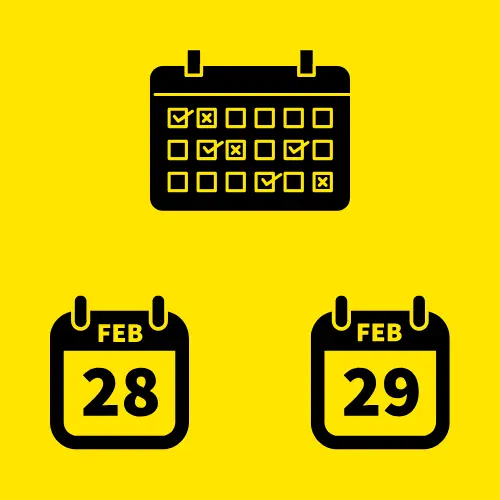
Write a Mojo program to check if a given year is a leap year.
Test Case:
Input:
Enter your year: 2024
Expected Output:
Leap Year
Explanation:
The program checks if a given year is a leap year or not. A leap year is a year that has one extra day, making it 366 days long instead of the usual 365 days. This extra day is added to February, which has 29 days instead of 28. A leap year is checked using a specific rule. First, the year must be divisible by 4. However, if the year is also divisible by 100, it is not a leap year unless it is divisible by 400.
- It first asks the user to input a year and input is stored in
year
variable. - Then, the program uses a
if
condition to check if the year is divisible by 4 (year % 4 == 0
) and either not divisible by 100 (year % 100 != 0
) or divisible by 400 (year % 400 == 0
). If both conditions are met, it printsLeap Year
. If the conditions are not satisfied, it printsNot a Leap Year
.year % 4 == 0
checks if the year is divisible by 4.year % 100 != 0
ensures the year is not divisible by 100, unless…year % 400 == 0
allows the year to be divisible by 400, which makes it a leap year.
year = int(input("Enter the year: ")) if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0): print("Leap Year") else: print("Not a Leap Year")
Triangle Types
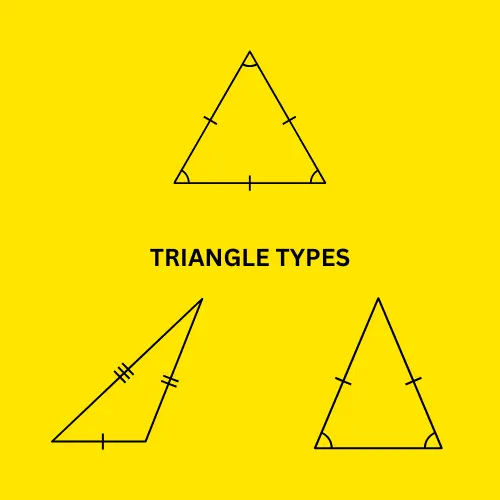
Write a Mojo program to determine the type of a triangle based on its sides:
All sides equal: Equilateral
Two sides equal: Isosceles
All sides different: Scalene
Test Case:
Input:
Enter side 1: 3
Enter side 2: 4
Enter side 3: 4
Expected Output:
Isosceles
Explanation:
This program helps classify a triangle based on the lengths of its sides.
- It starts by asking the user to input the lengths of three sides of a triangle:
side1
,side2
, andside3
. - First, the program checks if all three sides are equal (
side1 == side2 == side3
). If they are, it means the triangle is equilateral, meaning all sides have the same length, so it printsEquilateral
. - If the sides are not all equal, the program checks if any two sides are equal using this condition: (
side1 == side2 or side2 == side3 or side1 == side3
). If two sides are equal, the triangle is isosceles, meaning it has two equal sides, and it printsIsosceles
. - If neither of the above conditions is true, it means all sides are different, so the triangle is scalene, and the program prints
Scalene
.
side1 = int(input("Enter side 1: ")) side2 = int(input("Enter side 2: ")) side3 = int(input("Enter side 3: ")) if side1 == side2 == side3: print("Equilateral") elif side1 == side2 or side2 == side3 or side1 == side3: print("Isosceles") else: print("Scalene")
School Grading System
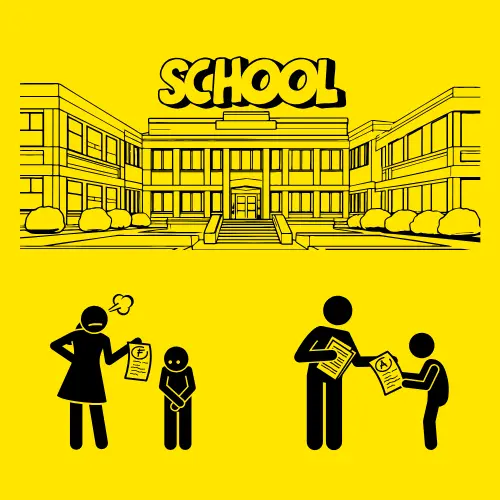
A school uses a grading system based on the student’s marks in three subjects: Mathematics, Science, and English. The grade is determined by the following conditions:
If the average marks are greater than or equal to 90 in all subjects, the grade is “A+”.
If the average marks are between 80 and 89 in all subjects, the grade is “A”.
If the average marks are between 70 and 79 and the student has scored above 60 in all subjects, the grade is “B”.
If the average marks are between 60 and 69, but the student has failed in any one subject, the grade is “C”.
If the average marks are less than 60 or the student has scored below 40 in any subject, the grade is “D”.
Write a Mojo program to calculate the grade based on the given marks in all three subjects.
Test Case:
Input:
Enter your Mathematics marks: 85
Enter your Science marks: 90
Enter your English marks: 80
Expected Output:
Grade: A
Explanation:
This program calculates a student’s grade based on their marks in Mathematics, Science, and English, following certain conditions.
- The program first takes the marks for Mathematics, Science, and English and stores them in the variables
math_marks
,science_marks
, andenglish_marks
. - Then, it calculates the
average_marks
using the formula. - Next, the program checks the following conditions using
if
,elif
, andelse
statements:- First condition: If the average marks and all individual subject marks are 90 or above, the student gets an
"A+"
grade. - Second condition: If the average is 80 or more, and each subject has at least 80 marks, the student gets an
"A"
grade. - Third condition: If the average is between 70 and 80 and the student has scored more than 60 in each subject, the grade is
"B"
. - Fourth condition: If the average is between 60 and 70 but the student has scored less than 60 in any subject, they get a
"C"
grade. - Else condition: If none of the above conditions are met, the student receives a grade
"D"
.
- First condition: If the average marks and all individual subject marks are 90 or above, the student gets an
math_marks = input("Enter your Mathematics marks: ").to_float32() science_marks = input("Enter your Science marks: ").to_float32() english_marks = input("Enter your English marks: ").to_float32() average_marks = (math_marks + science_marks + english_marks) / 3 if average_marks >= 90 and math_marks >= 90 and science_marks >= 90 and english_marks >= 90: grade = "A+" elif average_marks >= 80 and math_marks >= 80 and science_marks >= 80 and english_marks >= 80: grade = "A" elif average_marks >= 70 and average_marks < 80 and math_marks > 60 and science_marks > 60 and english_marks > 60: grade = "B" elif average_marks >= 60 and average_marks < 70 and (math_marks < 60 or science_marks < 60 or english_marks < 60): grade = "C" else: grade = "D" print("Grade:", grade)
University Course Fee Calculation
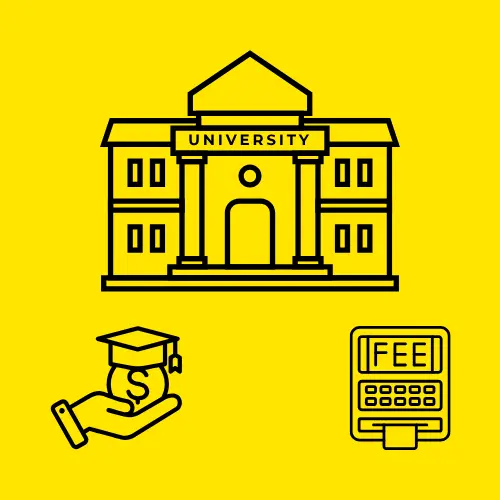
A university charges a fee for courses:
– If a student registers for less than 3 courses, each course costs $500.
– If a student registers between 3 and 5 courses, each course costs $450.
– If a student registers for 6 or more courses, each course costs $400. However, there is a discount if the student is a scholarship holder:
– A scholarship student gets a 20% discount.
– A non-scholarship student gets a 5% discount if they register for 4 or more courses.
Write a Mojo program to calculate the total fee.
Test Case:
Input:
Enter number of courses: 4
Are you a scholarship holder (Yes/No): Yes
Expected Output:
Fee: 1440
Explanation:
This program calculates a student’s total fee based on the number of courses they are taking and whether they hold a scholarship.
- The program asks the user to enter the number of courses, storing the value in the variable
courses
, and then asks if the user is a scholarship holder, storing the response in the variablescholarship
. - Based on the number of courses, it determines the fee per course.
- If the number of courses is less than 3,
fee_per_course = 500
is used. - If the number of courses is between 3 and 5,
fee_per_course = 450
is applied. - If the number of courses is more than 5,
fee_per_course = 400
is set.
- If the number of courses is less than 3,
- Then, the program calculates the total fee the student has to pay. The total fee is determined by multiplying the
fee_per_course
, which is the cost of one course, by the number of courses the student is taking (courses
). This gives the total amount the student would pay if no discounts are applied yet. - Next, the program checks if the student has a scholarship.
- If the student has a scholarship, the line
total_fee -= total_fee * 20 // 100
applies a 20% discount by multiplying the total fee by 20, dividing by 100 to get the discount amount, and then subtracting it from the original total fee. - If the student doesn’t have a scholarship but is enrolled in 4 or more courses, the line
total_fee -= total_fee * 5 // 100
applies a 5% discount. This works by calculating 5% of the total fee, then subtracting it from the original fee.
- If the student has a scholarship, the line
courses = Int(input("Enter number of courses: ")) scholarship = input("Are you a scholarship holder (Yes/No): ") # Determine the fee per course based on the number of courses if courses < 3: fee_per_course = 500 elif courses <= 5: fee_per_course = 450 else: fee_per_course = 400 # Calculate the total fee total_fee = fee_per_course * courses # Apply discount if the user is a scholarship holder if scholarship == "Yes": total_fee -= total_fee * 20 // 100 elif courses >= 4: total_fee -= total_fee * 5 // 100 print("Fee:", total_fee)
Phone Plan Cost
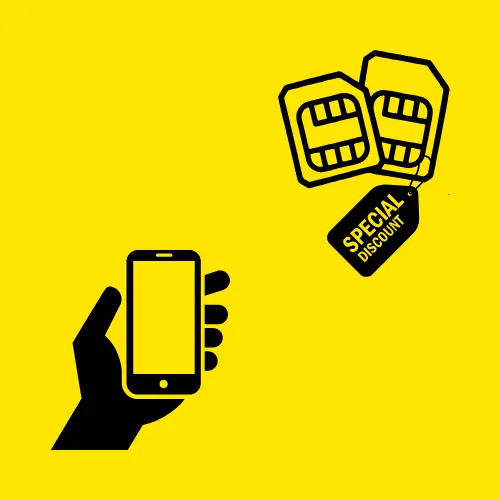
A phone company offers several plans:
Basic Plan: $30/month with 1 GB of data.
Standard Plan: $50/month with 3 GB of data.
Premium Plan: $70/month with 5 GB of data.
Additional charges are applied:
Over 1 GB of data usage costs $5 per GB for Basic.
Over 3 GB of data usage costs $4 per GB for Standard.
Over 5 GB of data usage costs $3 per GB for Premium.
A 10% discount is applied if the customer has been with the company for more than 2 years.
A 5% discount is applied if the customer uses the company’s app to pay.
Write a Mojo program to calculate the total monthly bill based on the selected plan, data usage, loyalty, and payment method.
Test Case:
Input:
Enter plan (Basic/Standard/Premium): Standard
Enter data used in GB: 6 GB
Are you a loyal customer? (Yes/No): Yes
Paid via app? (Yes/No): Yes
Expected Output:
Total Bill: 46.2
Explanation:
This program helps calculate the total mobile plan cost based on the user’s inputs such as their plan, data usage, loyalty status, and payment method.
- The program asks for the plan, the data used, if the user is loyal, and if the payment was via the app, storing the values in the variables
plan
,data_used
,loyalty
, andapp_payment
. - Based on the chosen plan, the program assigns values to base cost, included data, and extra cost using
if-elif-else
statements.- If the plan is “Basic”, the base cost is 30, with 1GB included, and extra data costs 5 per GB.
- If the plan is “Standard”, the base cost is 50, with 3GB included, and extra data costs 4 per GB.
- If the plan is “Premium”, the base cost is 70, with 5GB included, and extra data costs 3 per GB.
- The program calculates how much extra data the user has used beyond the included data. The formula
extra_data = data_used - included_data
is used to find out how much extra data is used. - If there is extra data used, the program calculates the additional cost with
additional_cost = extra_data * extra_cost
. Otherwise, the additional cost is zero. - The program adds the base cost and additional cost to calculate the total bill using
total_cost = base_cost + additional_cost
. - The program checks if the user is a loyal customer. If yes, it applies a 10% discount with
total_cost -= total_cost * 10 // 100
. - It then checks if the user paid via the app. If yes, it applies a 5% discount with
total_cost -= total_cost * 5 // 100
.
plan= input("Enter plan (Basic/Standard/Premium): ") data_used = int(input("Enter data used in GB: ")) loyalty = input("Are you a loyal customer? (Yes/No): ") app_payment = input("Paid via app? (Yes/No): ") if plan == "Basic": base_cost = 30 included_data = 1 extra_cost = 5 elif plan == "Standard": base_cost = 50 included_data = 3 extra_cost = 4 else: base_cost = 70 included_data = 5 extra_cost = 3 extra_data = data_used - included_data if extra_data > 0: additional_cost = extra_data * extra_cost else: additional_cost = 0 total_cost = base_cost + additional_cost if loyalty == "Yes": total_cost -= total_cost * 10 // 100 if app_payment == "Yes": total_cost -= total_cost * 5 // 100 print("Total Bill:", total_cost)
Tax Calculation
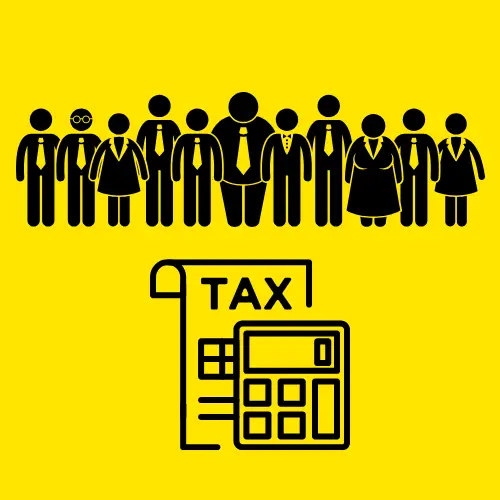
A person’s tax amount is calculated based on their annual income and age. The tax rates depend on the following criteria:
If the annual income is less than $30,000, the tax rate is 10%.
If the annual income is between $30,000 and $60,000, the tax rate is 15%, but if the person is under 30 years of age, the tax rate is 12%.
If the annual income is between $60,000 and $100,000, the tax rate is 20%, but if the person is over 50 years of age, the tax rate is reduced to 18%.
If the annual income exceeds $100,000, the tax rate is 25%, but if the person is over 60 years of age, the tax rate is reduced to 22%.
Additionally, there are deductions and allowances based on the following conditions:
If the person is a student, they are eligible for a deduction of $2,000.
If the person is married, they get a deduction of $5,000.
If the person is over 60 years old, they get an additional deduction of $1,000.
Write a Mojo program to calculate the tax for a person based on their annual income, age, marital status, and student status.
Test Case:
Input:
Enter your annual income: $55,000
Enter your age: 25
Are you a student (yes/no): yes
Are you married (yes/no): no
Expected Output:
Tax Rate: 12%
Total Deductions: $2,000
Final Taxable Income: $53,000
Tax Amount: $6,360
Explanation:
This program calculates the tax based on income, age, student status, marital status, and applicable deductions.
- The program prompts the user to enter their annual income, age, student status (“yes” or “no”), and marital status(“yes” or “no”), storing these values in the variables
income
,age
,student
, andmarried
, respectively. - Based on the entered
income
, the program sets a default tax rate:- If the
income
is below 30,000, thetax_rate
is set to 0.1 (10%). - If the
income
is between 30,000 and 60,000, thetax_rate
is 0.15 (15%). - If the
income
is between 60,000 and 100,000, thetax_rate
is 0.2 (20%). - If the
income
exceeds 100,000, thetax_rate
is 0.25 (25%).
- If the
- However, this rate can be adjusted based on the user’s
age
.- If the
income
is between 30,000 and 60,000 and the user is under 30, thetax_rate
is changed to 0.12 (12%). - If the
income
is between 60,000 and 100,000 and the user is over 50, thetax_rate
is adjusted to 0.18 (18%). - For
income
exceeding 100,000 and users over 60, thetax_rate
becomes 0.22 (22%).
- If the
- The program also calculates deductions based on the user’s status.
- If
student
is “yes”, thedeductions
are increased by 2,000. - If
married
is “yes”, an additional 5,000 is added todeductions
. - If
age
is greater than 60,deductions
are increased by 1,000.
- If
- These
deductions
are subtracted from theincome
to calculate the final taxable income (taxable_income
). Finally, the program computes the tax amount by multiplying thetaxable_income
by thetax_rate
andtax_rate_percentage
by multiplying thetax_rate
by the 100.
income = float(input("Enter your annual income: ")) age = int(input("Enter your age: ")) student = input("Are you a student (yes/no): ") married = input("Are you married (yes/no): ") # Default tax rate based on income range if income < 30000: tax_rate = 0.1 elif income >= 30000 and income <= 60000: tax_rate = 0.15 elif income > 60000 and income <= 100000: tax_rate = 0.2 else: tax_rate = 0.25 # Adjust tax rate based on age without using if in variables if income >= 30000 and income <= 60000 and age < 30: tax_rate = 0.12 elif income > 60000 and income <= 100000 and age > 50: tax_rate = 0.18 elif income > 100000 and age > 60: tax_rate = 0.22 # Calculate deductions deductions = 0 if student: deductions += 2000 # Deduction for students if married: deductions += 5000 # Deduction for married individuals if age > 60: deductions += 1000 # Additional deduction for individuals over 60 taxable_income = income - deductions tax_amount = taxable_income * tax_rate tax_rate_percentage = tax_rate * 100 print(f"Tax Rate: {tax_rate_percentage}") print(f"Total Deductions: ${deductions}") print(f"Final Taxable Income: ${taxable_income}") print(f"Tax Amount: ${tax_amount}")
Electricity Bill Calculation
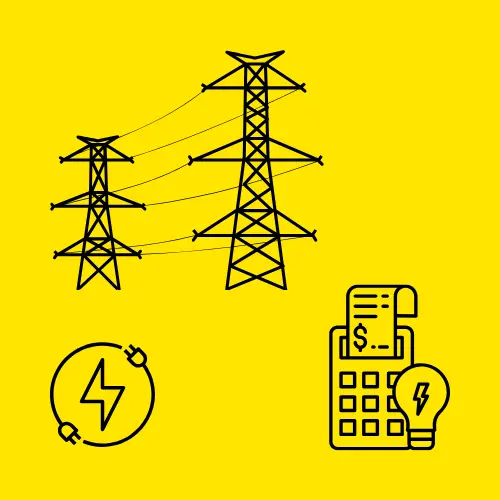
The electricity bill is calculated as follows:
For consumption of up to 100 kWh, the rate is $0.10 per kWh.
For consumption between 101 kWh to 200 kWh, the rate is $0.15 per kWh.
For consumption above 200 kWh, the rate is $0.20 per kWh.
Additional rules:
If the consumption is above 300 kWh, there is a $20 surcharge.
Write a Mojo program to calculate the total electricity bill based on the consumption.
Test Case:
Input:
Enter your electricity consumption in kWh: 250
Expected Output:
Total Bill: 42.5
Explanation:
This program calculates an electricity bill based on the user’s consumption and applies a surcharge for high consumption.
- The program asks the user to enter their electricity usage in kilowatt-hours (kWh). This value is stored in the variable
consumption
. - If the
consumption
is 100 kWh or less, the cost is calculated at $0.10 per kWh:bill = consumption * 0.10
. - If the
consumption
is between 101 and 200 kWh, the cost is calculated at $0.15 per kWh:bill = consumption * 0.15
. - If the
consumption
exceeds 200 kWh, the cost is calculated at $0.20 per kWh:bill = consumption * 0.20
. - If the
consumption
exceeds 300 kWh, an additional surcharge of $20 is added to the bill:bill += 20
.
consumption = int(input("Enter your electricity consumption in kWh: ")) # Calculate bill based on consumption if consumption <= 100: bill = consumption * 0.10 elif consumption > 100 and consumption <= 200: bill = consumption * 0.15 else: bill = consumption * 0.20 # Apply surcharge if applicable if consumption > 300: bill += 20 print("Total Bill:", bill)
Taxi Fare
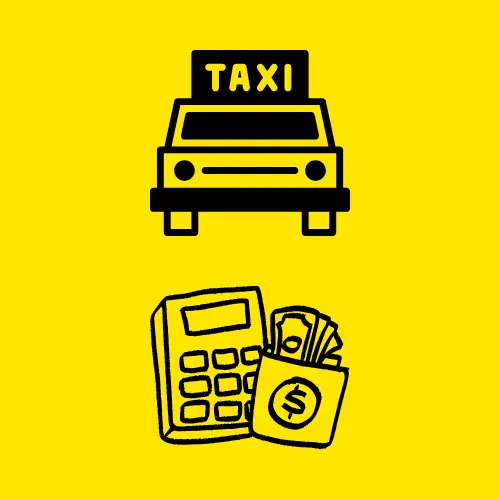
Write a Mojo program to calculate taxi fare based on distance:
≤ 5 km: $10/km.
6–20 km: $8/km.
20 km: $5/km.
Add $15 if travel time > 1 hour.
Test Case:
Input:
Enter distance in km: 25 km
Enter travel time in hours: 2 hours
Expected Output:
Fare: $185
Explanation:
This program calculates the fare for a journey based on the distance traveled and the time taken.
- The program first asks for the distance traveled in kilometers and stores it in the variable
distance
. Then, it asks for the travel time in hours and stores it in the variabletime
. - If the
distance
is 5 km or less, the fare is calculated at $10 per km:fare = distance * 10
. - If the
distance
is between 6 and 20 km, the fare for the first 5 km is $10 per km, and the remaining distance is charged at $8 per km:fare = (5 * 10) + (distance - 5) * 8
. - If the
distance
exceeds 20 km, the fare includes:- $10 per km for the first 5 km,
- $8 per km for the next 15 km (6 to 20 km),
- $5 per km for the remaining distance beyond 20 km:
fare = (5 * 10) + (15 * 8) + (distance - 20) * 5
.
- If the
time
exceeds 1 hour, an additional flat fee of $15 is added to the fare:fare += 15
.
distance = int(input("Enter distance in km: ")) time = int(input("Enter travel time in hours: ")) if distance <= 5: fare = distance * 10 elif distance <= 20: fare = (5 * 10) + (distance - 5) * 8 else: fare = (5 * 10) + (15 * 8) + (distance - 20) * 5 if time > 1: fare += 15 print("Fare: $", fare)
Health Insurance Plan Selector
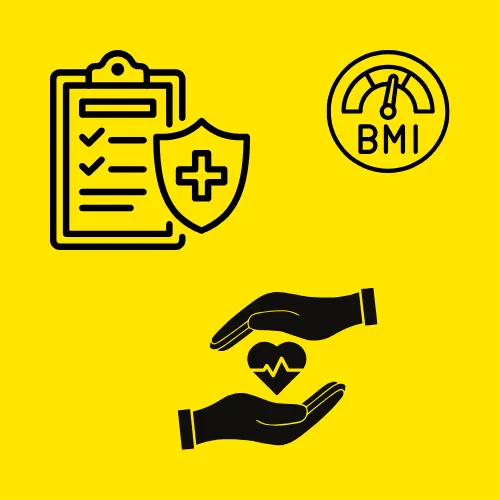
A health insurance company offers plans based on a customer’s age, BMI (Body Mass Index), and habits:
Age ≤ 25: Plan A ($300/year).
Age 26–40: Plan B ($500/year).
Age > 40: Plan C ($800/year).
Additional charges:
Add $200 if BMI > 30 (indicating obesity).
Add $100 if the person smokes.
Write a Mojo program to calculate the total annual cost for the user based on their inputs.
Test Case:
Input:
Enter your age: 35
Enter your BMI: 32
Are you a smoker (Yes/No): Yes
Expected Output:
Total Annual Cost: $800
Explanation:
This program calculates the total annual cost based on the user’s age, BMI, and smoking status.
- The program asks for the user’s age, stores it in
age
, asks for the BMI, and stores it inbmi
, and then checks if the user is a smoker with the response stored insmoker
. - If the
age
is 25 or younger, the base cost is $300:cost = 300
. - If the
age
is between 26 and 40, the base cost is $500:cost = 500
. - If the
age
is above 40, the base cost is $800:cost = 800
. - If the
bmi
is greater than 30, an additional cost of $200 is added:cost += 200
. - If the user is a smoker, an additional cost of $100 is added:
cost += 100
.
age = int(input("Enter your age: ")) bmi = float(input("Enter your BMI: ")) smoker = input("Are you a smoker (Yes/No): ") if age <= 25: cost = 300 elif age <= 40: cost = 500 else: cost = 800 if bmi > 30: cost += 200 if smoker == "Yes": cost += 100 print("Total Annual Cost: $", cost)
In conclusion, learning how to use if-else statements in Mojo is an important skill for making programs that can react to different situations. With if-else statements, your programs can make decisions based on what the user does or the data it gets. This helps in creating programs that are flexible and interactive, like calculating prices or responding to user choices. By practicing, you will get better at solving problems and feel more confident using these statements in different coding challenges.