In this article, you will learn how to fix NullPointerException in Java. What are the different reasons that cause this exception? And ways to fix them.
Table of Contents
- What Is a Pointer?
- What is an Exception?
- What is a NullPointerException in Java?
- Causes and Solutions for NullPointerException in Java
- Case 1: Accessing a Method on a Null Object
- Solution: Check if the Object Is Null Before Accessing Its Methods
- Case 2: Accessing an Array Element on a Null Array
- Solution: Ensure the Array Is Initialized Before Accessing Its Elements.
- Case 3: Accessing a Field on a Null Object
- Solution: Check if the Object Is Null Before Accessing Its Fields
- Case 4: Calling a Method on a Null Object Returned by Another Method
- Solution:Check if the Return Value Is Null Before Calling Methods on It.
- Case 5: Incorrect Handling of Wrapper Classes
- Solution: Check if the Wrapper Class Instance Is Null Before Unboxing.
- Conclusion
What Is a Pointer?
Pointers are variables that store the memory address of another variable. However, in Java, direct pointers are not used for safety and simplicity. Instead, Java uses reference variables to objects, which indirectly handle memory addresses. This makes programming in Java safer and less error-prone for beginners. When a reference is null, it means it does not point to any object in memory.
What is an Exception?
After getting started with Java, facing exceptions in code is quite common. An exception in Java is an event that interrupts the normal flow of a program. It occurs during execution when something unexpected happens, like dividing by zero or accessing an array element out of bounds. When an exception occurs, Java creates an error object and throws it, stopping the current process and looking for error handling. Properly handling exceptions ensures the program can recover from errors or terminate gracefully.
What is a NullPointerException in Java?
A NullPointerException in Java happens when you try to use an object reference that points to null, meaning it hasn’t been assigned an actual object. This error means you’re trying to call a method or access a property on a null object. For example, if String str = null; and you try str.length()
, it will throw NullPointerException. A good practice is to always check if an object is null before using it to avoid null pointer exception.
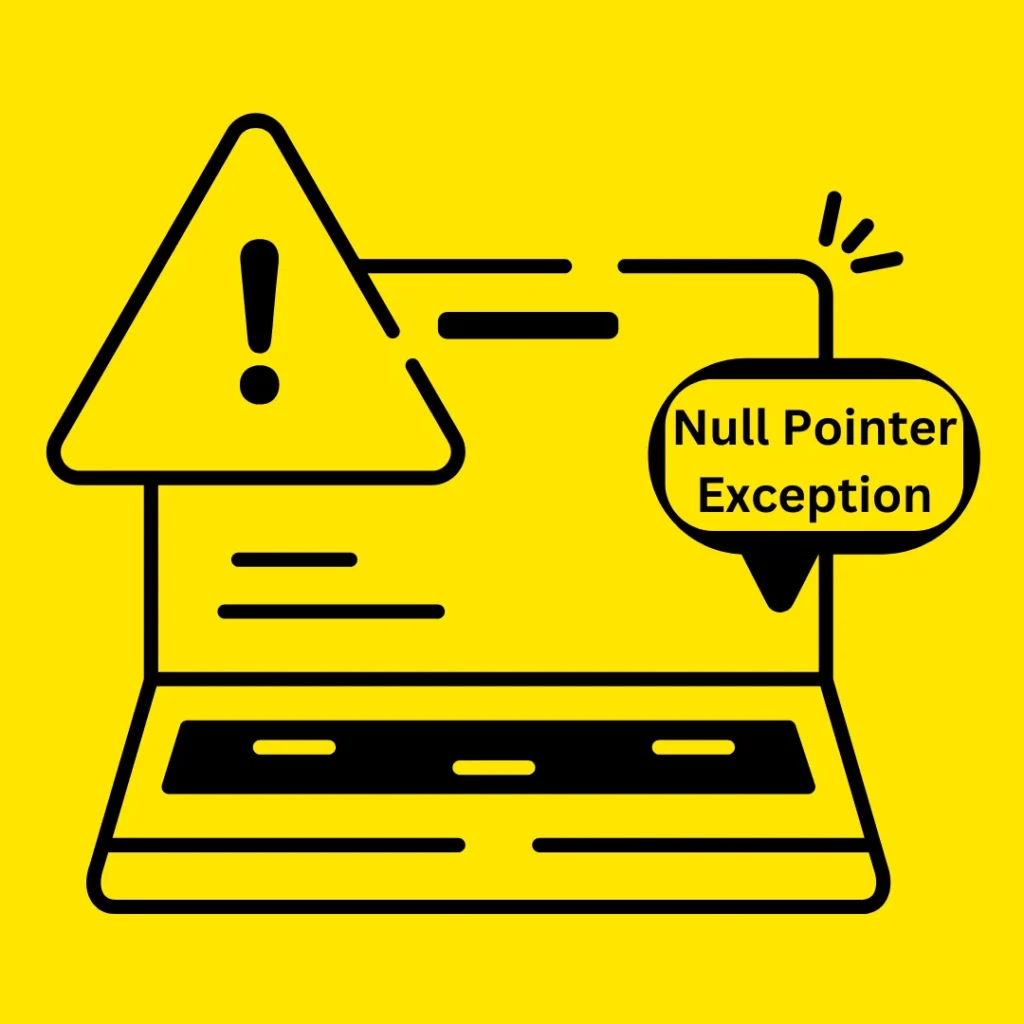
Causes and Solutions for NullPointerException in Java
Following are some common causes of NullPointerException that can occur while programming in Java.
Case 1: Accessing a Method on a Null Object
Accessing a method on a null object causes a NullPointerException. This issue occurs when you try to call a method on a null object. Since there is no object to invoke the method on, it results in a NullPointerException. In this example, str is null, so calling str.length() results in Exception in thread "main" java.lang.NullPointerException
. However even if you declare a reference variable and assign it to nothing, still, it will cause NullPointerException because the default value of a reference variable in Java is null.
Example Code
public class Main { public static void main(String[] args) { String str = null; // or String str; System.out.println(str.length()); // This will cause a NullPointerException } }
Output
ERROR! Exception in thread "main" java.lang.NullPointerException: Cannot invoke "String.length()" because "<local2>" is null at Main.main(Main.java:5)
A simple way to explain this situation is when you call someone and the number you dialed does not exist, so it responds with doesn’t exist, similarly since there are no object or the number in use it results in Null pointer exception
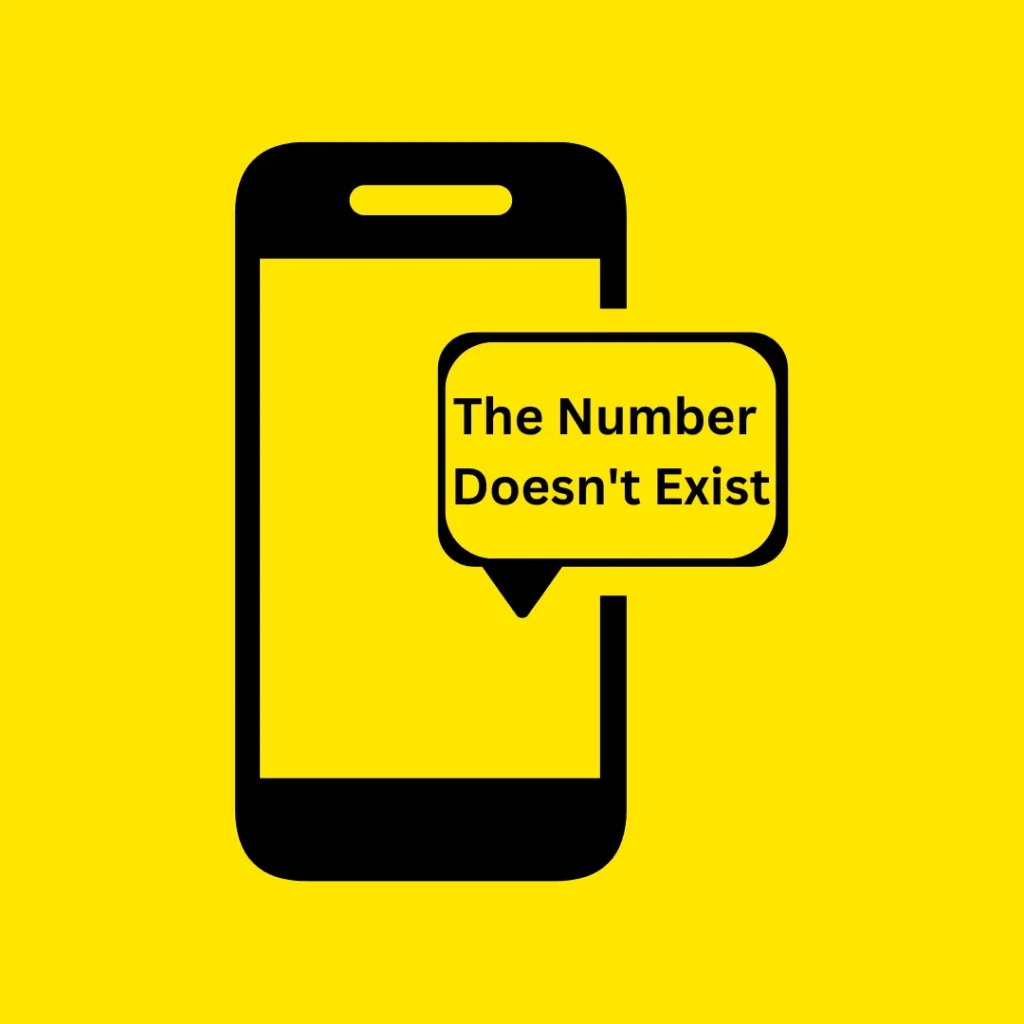
Solution: Check if the Object Is Null Before Accessing Its Methods
To avoid a NullPointerException in Java, always check if an object is null before calling its methods. This ensures that you only interact with valid objects. For example, use an if statement to verify that the object isn’t null before proceeding with method calls. Or either you can initialize the reference variable to an object if you forgot to.
Solution Code
public class Main { public static void main(String[] args) { String str = null; if (str != null) { System.out.println(str.length()); } else { System.out.println("String is null"); } } }
Output
String is null
Case 2: Accessing an Array Element on a Null Array
If you try to access an element in an array that hasn’t been initialized (i.e., it’s null), Java will throw a NullPointerException. This happens because you’re trying to work with an array that doesn’t exist in memory yet. Always make sure to initialize your arrays before accessing their elements.
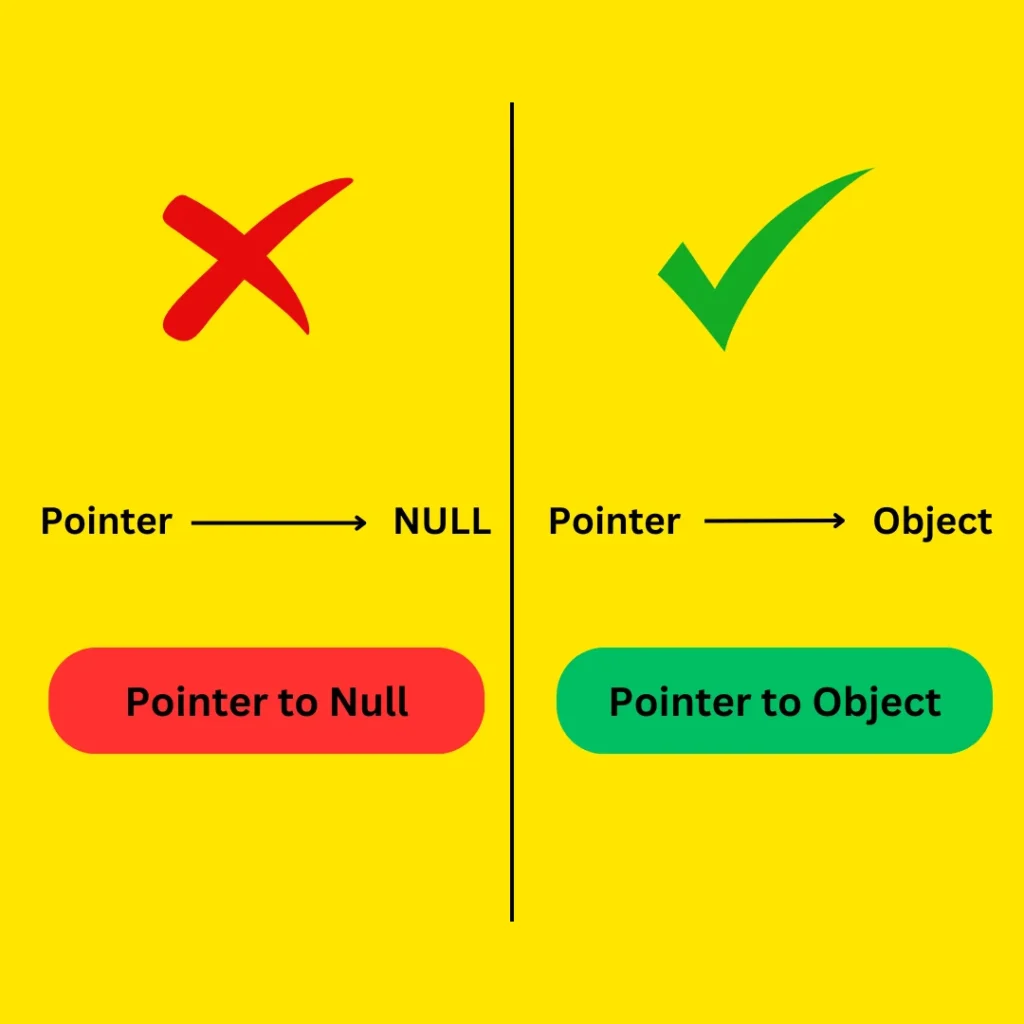
Example Code
Here, arr is null, so accessing arr[0] throws an exception.
public class Main { public static void main(String[] args) { int[] arr = null; System.out.println(arr[0]); // This will cause a NullPointerException } }
Output
ERROR! Exception in thread "main" java.lang.NullPointerException: Cannot load from int array because "<local1>" is null at Main.main(Main.java:4)
Solution: Ensure the Array Is Initialized Before Accessing Its Elements.
To avoid a NullPointerException with arrays, make sure the array is properly initialized before you try to use it. Initializing the array means setting it up so you can store and access its elements. If you don’t initialize it, the array is null, and trying to access it will lead to an error.
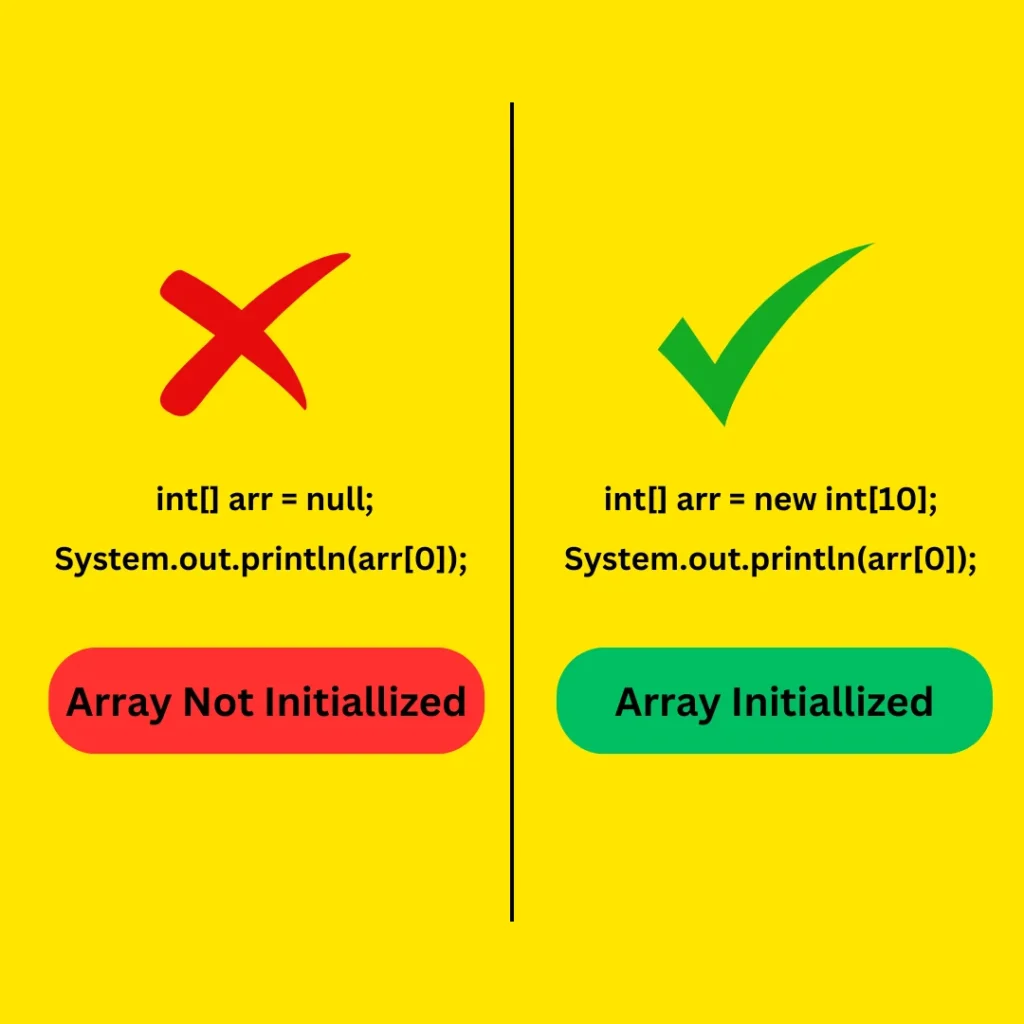
Solution Code
In the following code, array is now properly initialized with ten elements and the default value is zero for each element.
public class Main { public static void main(String[] args) { int[] arr = new int[10]; System.out.println(arr[0]); // This will not cause an exception } }
Output
0
Case 3: Accessing a Field on a Null Object
Similar to accessing methods on a null object, accessing a field on a null object also causes a NullPointerException. This happens when you try to use an object that hasn’t been created yet. Always check if the object is not null before trying to access its fields to avoid this error.
In the following code the object of the class person is assigned to null or not, it will cause a null pointer exception because the default value of a reference variable is null in Java. And when you try to access the field name on the reference variable pointing to null, it will cause a null pointer exception.
Example Code
public class Main { public static void main(String[] args) { Person person = null; // or Person p; System.out.println(person.name); // This will cause a NullPointerException } } class Person { String name; }
Output
ERROR! Exception in thread "main" java.lang.NullPointerException: Cannot read field "name" because "<local1>" is null at Main.main(Main.java:4)
Solution: Check if the Object Is Null Before Accessing Its Fields
Before accessing a field in an object, always check if the object is null. This means making sure the object has been properly created. If it’s null and you try to access its fields, you’ll get an error.
Solution Code
public class Main { public static void main(String[] args) { Person person = null; if (person != null) { System.out.println(person.name); } else { System.out.println("Person is null"); } } } class Person { String name; }
Output
Person is null
Case 4: Calling a Method on a Null Object Returned by Another Method
If a method returns null and you try to call another method on that result, you’ll get an error. To avoid this issue, always check if the result is null before using it. In the following code, the getMessage() method returns null and is assigned to str in main. So when we call the str.length() it will cause the NullPointerException because the str is pointing to null.
Example Code
public class Main { public static void main(String[] args) { String str = getMessage(); System.out.println(str.length()); // This will cause a NullPointerException } public static String getMessage() { return null; } }
Output
ERROR! Exception in thread "main" java.lang.NullPointerException: Cannot invoke "String.length()" because "<local2>" is null at Main.main(Main.java:4)
Solution:Check if the Return Value Is Null Before Calling Methods on It.
Before calling methods on a value returned from another method, make sure it’s not null. Checking this helps prevent errors from happening.
Solution Code
public class Main { public static void main(String[] args) { String str = getMessage(); if(str != null) { System.out.println(str.length()); // This will cause a NullPointerException } else { System.out.println("Returned string is null"); } } public static String getMessage() { return null; } }
Output
Returned string is null
Case 5: Incorrect Handling of Wrapper Classes
When you try to unbox a null value from a wrapper class (like Integer or Double), it causes a NullPointerException. Unboxing means converting the wrapper class back to a primitive type. If the wrapper is null, there’s nothing to convert, leading to an error.
Example Code
public class Main { public static void main(String[] args) { Integer num = null; int value = num; // This will cause a NullPointerException } }
Output
ERROR! Exception in thread "main" java.lang.NullPointerException: Cannot invoke "java.lang.Integer.intValue()" because "<local1>" is null at Main.main(Main.java:4)
Solution: Check if the Wrapper Class Instance Is Null Before Unboxing.
Before unboxing a wrapper class (like Integer or Double), always check if the instance is null. If it is null, you can’t convert it to a primitive type and will get an error. Checking for null helps avoid this problem.
Solution Code
public class Main { public static void main(String[] args) { Integer num = null; if (num != null) { int value = num; System.out.println(value); } else { System.out.println("Integer is null"); } } }
Output
Integer is null
An interesting way to flag this issue is to visualize the code using tools like Python tutor to see what’s happening in each step of execution, where the reference variable are pointing to? And much more.
Conclusion
In conclusion, you have learned the top five causes of NullPointerException in Java. The concepts of pointers, null
, and exceptions, with clear explanations and code examples to improve your understanding. Visual aids were included to make these concepts easier to Understand. While there are many reasons for NullPointerExceptions, Learning these five fundamental cases will help you avoid many beginner Mistakes. This foundation will assist you in removing errors and writing in Java you continue learning.