Scientific notation is widely used in fields like physics, engineering, and astronomy to work with extremely large or small numbers. Instead of writing out long strings of zeros, scientific notation helps keep numbers clean and manageable. Luckily, C++ offers simple ways to handle these numbers right in your code.
- What Is Scientific Notation? A Simple Analogy
- C++ Syntax for Scientific Notation
- How C++ Displays Numbers by Default?
- Formatting Numbers in C++ (Scientific vs. Fixed-Point)
- How to Convert Scientific Notation to Regular Numbers in C++?
- Prevent Scientific Notation in C++
- When Should You Use Scientific Notation in C++?
- FAQs
- Conclusion
- Related Resources
What Is Scientific Notation? A Simple Analogy
Imagine you’re working at a warehouse with huge product codes. Instead of writing “0000000123”, wouldn’t it be easier to write “1.23 × 10⁸”? Scientific notation is like a shortcut that makes long numbers easier to read, write, and calculate.
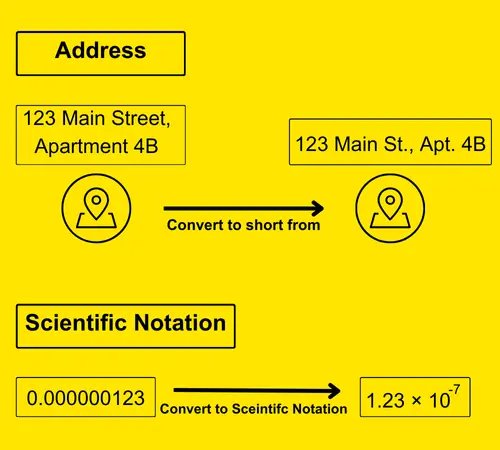
In short:
- Scientific notation expresses numbers as a product of a decimal (between 1 and 10) and a power of 10.
- Example: 0.0000456 becomes 4.56 × 10⁻⁵.
This makes it perfect for programming when handling very large or very small values without losing precision.
C++ Syntax for Scientific Notation
In C++, scientific notation uses the letter e
or E
to stand for “×10 to the power of.”
This is most often used with floating-point numbers (like float
, double
, or long double
).
Syntax:
double number = aEb;
Where:
a
is the base (a decimal number).E
ore
means “times 10 raised to the power of.”b
is the exponent (an integer).
Example:
Here, 6.022e23
means 6.022 × 10²³, a number commonly known from chemistry (Avogadro’s number).We store the number in a double
because it’s large.
#include <iostream> using namespace std; int main() { double sci_num = 6.022e23; // 6.022 × 10^23 cout << "Scientific number: " << sci_num << endl; return 0; }
Output:
The +23
means the decimal moves 23 places to the right.
Scientific number: 6.022e+23
How C++ Displays Numbers by Default?
In C++, very large or very small numbers may automatically be shown in scientific notation when printed.
But, we can control the display format using <iomanip>
, which lets us switch between:
fixed
→ Shows standard decimal notationscientific
→ Forces scientific notation
Formatting Numbers in C++ (Scientific vs. Fixed-Point)
C++ gives you full control over how numbers appear in your output:
Format | Use Case | Example |
---|---|---|
scientific | Forces scientific notation | cout << scientific; |
fixed | Shows standard decimal notation | cout << fixed; |
Example:
In the following code:
scientific
makes sure the number is shown like 1.234568e+08.fixed
forces standard decimal form like 123456789.00.setprecision(2)
limits the number to 2 decimal places after the dot.
#include <iostream> #include <iomanip> using namespace std; int main() { double number = 123456789.0; cout << scientific << "Scientific Notation: " << number << endl; cout << fixed << setprecision(2) << "Fixed-Point Notation: " << number << endl; return 0; }
Output:
Scientific Notation: 1.234568e+08 Fixed-Point Notation: 123456789.00
setprecision(n)
controls the number of digits after the decimal point.
How to Convert Scientific Notation to Regular Numbers in C++?
In reality, when you use a number like 1.23e5
in C++, it’s already stored as a normal floating-point number internally.
To display it in standard decimal form, we simply print it using fixed
formatting.
Use fixed
Formatting
Example:
#include <iostream> #include <iomanip> using namespace std; int main() { double sci_num = 1.23e5; // This is 123,000 cout << fixed << setprecision(0) << "Standard Number: " << sci_num << endl; return 0; }
Output:
Standard Number: 123000
Cast to Different Data Types
Sometimes, you may want to convert scientific notation numbers into:
float
→ single-precision numbersint
→ whole numbers (no decimal)
#include <iostream> using namespace std; int main() { double sci_num = 9.876e3; // 9876.0 int int_val = static_cast<int>(sci_num); // Cast to int float float_val = static_cast<float>(sci_num); // Cast to float cout << "Integer: " << int_val << endl; cout << "Float: " << float_val << endl; return 0; }
Output:
Integer: 9876 Float: 9876
Prevent Scientific Notation in C++
What if you want to always show numbers as full decimals, no matter how small or large?
Use std::fixed
with setprecision()
.
Example:
#include <iostream> #include <iomanip> using namespace std; int main() { double small_num = 0.000000123; cout << fixed << setprecision(9) << "Standard Form: " << small_num << endl; return 0; }
Output:
Standard Form: 0.000000123
When Should You Use Scientific Notation in C++?
Use scientific notation when:
- Dealing with extremely large or small values.
- You want consistent formatting in scientific calculations.
- Working on projects like physics simulations, astronomy data, or large datasets.
Avoid scientific notation when:
- You need human-readable outputs (like reports or invoices).
- You are working with financial numbers where precision matters.
FAQs
Why is scientific notation useful in C++?
Scientific notation simplifies handling very large or small numbers, keeping your code clean and calculations accurate, especially in scientific or engineering programs.
How do you write scientific notation in C++?
You can use e
or E
in your number. For example, 6.022e23
means 6.022 × 10²³.
How can I avoid scientific notation in output?
Use std::fixed
along with std::setprecision()
to force decimal (standard) notation.
Can you control how many decimals are shown?
Yes! std::setprecision(n)
allows you to specify the number of digits after the decimal point.
Is scientific notation only for doubles?
No, but it’s most commonly used with floating-point types (float
, double
, long double
).
Conclusion
Scientific notation in C++ is a powerful tool to handle numbers of all sizes without cluttering your code. Whether you’re displaying astronomical distances or microscopic measurements, C++ gives you the flexibility to control exactly how those numbers appear. By understanding how to write, format, and convert scientific notation, you’ll make your C++ programs cleaner, more readable, and better suited for real-world data.