Using arithmetic operators in solving scenario questions helps you understand how to apply basic calculations on different data types in C++ . This set of C++ problems includes scenarios like figuring out grocery bills, converting distances, and calculating savings. Each problem comes with a clear explanation and sample code to make it easy to practice and see how arithmetic operations work in real-life situations.
Grocery Bill Calculator
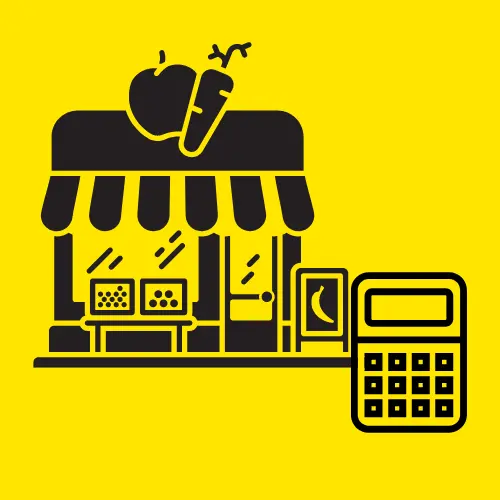
You’re at a grocery store buying apples, bananas, and oranges. The prices per kilogram are as follows: apples cost $3, bananas cost $2, and oranges cost $4. Write a C++ program to calculate your total bill based on the weight of each fruit you’re buying.
Test Case
Input:
Enter the weight of apples (kg): 2
Enter the weight of bananas (kg): 1
Enter the weight of oranges (kg): 1.5
Expected Output: Total bill: $14
Explanation:
To determine the total grocery bill, first input the weight of each fruit. Then, compute the cost for each fruit by multiplying the weight by its price per kilogram. Sum these costs to get the total bill, which is then displayed to the user.
- The program starts by asking the user to input the weight of
apples
,bananas
, andoranges
. - Each fruit has a specific price per kilogram:
- Apples are
$3 per kg
. - Bananas are
$2 per kg
. - Oranges are
$4 per kg
.
- Apples are
- The program alculates the total cost by multiplying the weight of each fruit by its price per kilogram and adding these amounts to get the total bill, stored in
totalBill
.
#include <iostream> using namespace std; int main() { double apples, bananas, oranges; double totalBill; const double priceApple = 3.0, priceBanana = 2.0, priceOrange = 4.0; cout << "Enter the weight of apples (kg): "; cin >> apples; cout << "Enter the weight of bananas (kg): "; cin >> bananas; cout << "Enter the weight of oranges (kg): "; cin >> oranges; totalBill = (apples * priceApple) + (bananas * priceBanana) + (oranges * priceOrange); cout << "Total bill: $" << totalBill << endl; return 0; }
Movie Ticket Cost
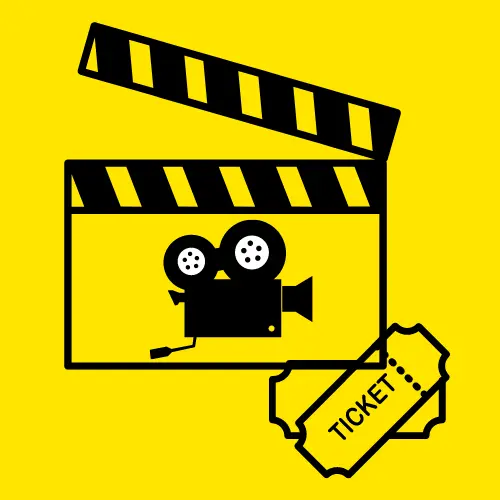
You’re going to the movies and need to calculate the total cost of tickets. Each adult ticket costs $12 and each child ticket costs $8. Write a C++ program to calculate the total cost based on the number of adult and child tickets.
Test Case
Input:
Enter the number of adult tickets: 2
Enter the number of child tickets: 3
Expected Output:Total cost: $48
Explanation:
To find out the total cost, input the number of adult and child tickets. Multiply the number of adult tickets by $12 and the number of child tickets by $8. Add these amounts together to get the total cost, then display it.
- The program first asks how many adult and child tickets you want to buy.
- The cost for each type of ticket is predefined:
adultPrice
is set to$12.0
.childPrice
is set to$8.0
.
- The program calculates the total cost:
- Adult cost:
adultTickets * adultPrice
- Child cost:
childTickets * childPrice
- Adult cost:
- The total cost is calculated by adding the costs for adult and child tickets, which are stored in
totalCost
.
#include <iostream> using namespace std; int main() { int adultTickets, childTickets; const double adultPrice = 12.0, childPrice = 8.0; double totalCost; cout << "Enter the number of adult tickets: "; cin >> adultTickets; cout << "Enter the number of child tickets: "; cin >> childTickets; totalCost = (adultTickets * adultPrice) + (childTickets * childPrice); cout << "Total cost: $" << totalCost << endl; return 0; }
Birthday Party Budget
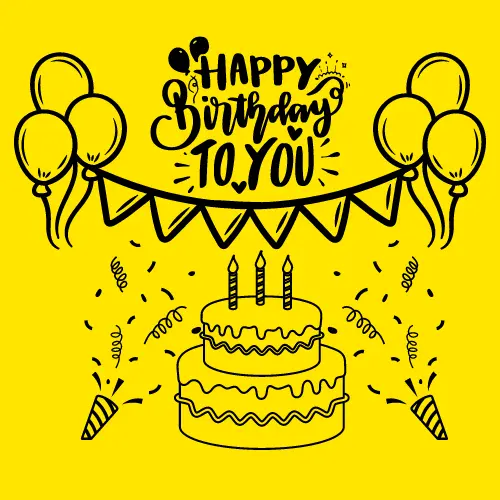
You’re organizing a birthday party and need to calculate the total cost of party items. Each party item costs $4, and you plan to buy 15 items. Write a C++ program to compute the total cost of the party items.
Test Case
Input: 15
Expected Output:Total cost: $60.00
Explanation:
This program calculates the total cost of party items based on the number of items you want to purchase. It multiplies the number of items by a fixed cost per item and then displays the total amount.
- The program first requests the user to input the number of party items, which is then recorded in the variable
numberOfItems
. - The cost per item is predefined and set to
$4.0
in the variablecostPerItem
. - After entering the number of items, the program calculates the total cost by multiplying
numberOfItems
bycostPerItem
and stores the result intotalCost
.
#include <iostream> using namespace std; int main() { int numberOfItems; const double costPerItem = 4.0; double totalCost; cout << "Enter the number of party items: "; cin >> numberOfItems; totalCost = numberOfItems * costPerItem; cout << "Total cost: $" << totalCost << endl; return 0; }
Splitting the Bill
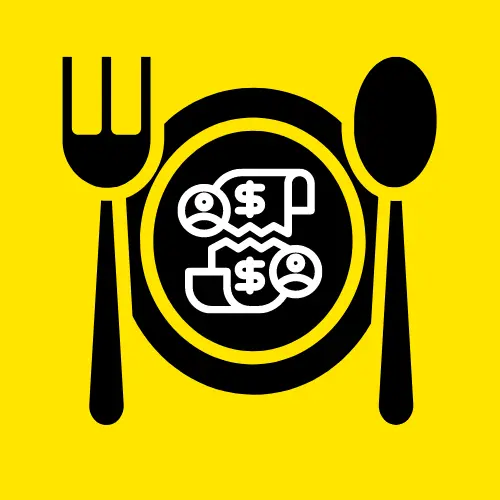
You and your friends went out to dinner, and the total bill needs to be split equally among all the diners. Write a C++ program that asks for the total bill amount and the number of people. The program should calculate and print the amount each person needs to pay.
Test Case
Input:
Enter the total bill amount: $150
Enter the total bill amount: $150
Enter the number of people: 5
Expected Output: Each person needs to pay: $30
Explanation:
To evenly split the bill among friends, input the total bill amount and the number of people. Divide the total bill by the number of people to find out how much each person owes.
- The program prompts the user for the total bill amount and stores it as
totalBill
. It then asks for the number of people splitting the bill and stores it asnumberOfPeople
. - The program calculates how much each person should pay by dividing
totalBill
bynumberOfPeople
. The result is stored asamountPerPerson
.
#include <iostream> using namespace std; int main() { double totalBill; int numberOfPeople; double amountPerPerson; cout << "Enter the total bill amount: $"; cin >> totalBill; cout << "Enter the number of people: "; cin >> numberOfPeople; amountPerPerson = totalBill / numberOfPeople; cout << "Each person needs to pay: $" << amountPerPerson << endl; return 0; }
Calculate Total Monthly Savings
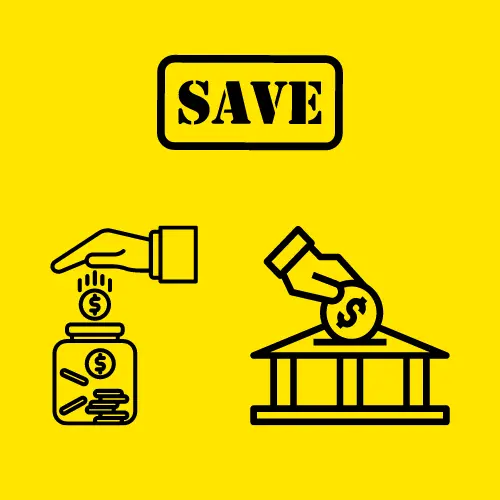
You have multiple sources of income and want to calculate your total savings for the month after deducting expenses. First, calculate your total income by adding up the income from different sources. Then, subtract the total expenses from this total income to determine your monthly savings. Write a C++ program to perform these calculations.
Test Case
Input:
Enter the amount of Income 1: $2000
Enter the amount of Income 2: $1500
Enter the amount of Income 3: $800
Enter the total expenses: $2500
Expected Output: Total Monthly Savings: $1800
Explanation:
In this scenario, you’re trying to find your monthly savings by calculating the total income from different sources and then subtracting your total expenses. This helps you understand how much money you have left after covering all your costs for the month.
- The program asks for the amount of income from each source, stored in
income1
,income2
, andincome3
, as well as the total expenses, stored inexpenses
. - Next, the total income is calculated by summing the amounts from
income1
,income2
, andincome3
, and is stored intotalIncome
. - To calculate the monthly savings, the total expenses are subtracted from the total income, and the result is stored in
savings
.
#include <iostream> using namespace std; int main() { double income1, income2, income3, expenses, totalIncome, savings; cout << "Enter the amount of Income 1: $"; cin >> income1; cout << "Enter the amount of Income 2: $"; cin >> income2; cout << "Enter the amount of Income 3: $"; cin >> income3; cout << "Enter the total expenses: $"; cin >> expenses; totalIncome = income1 + income2 + income3; savings = totalIncome - expenses; cout << "Total Monthly Savings: $" << savings << endl; return 0; }
Fuel Cost Estimator
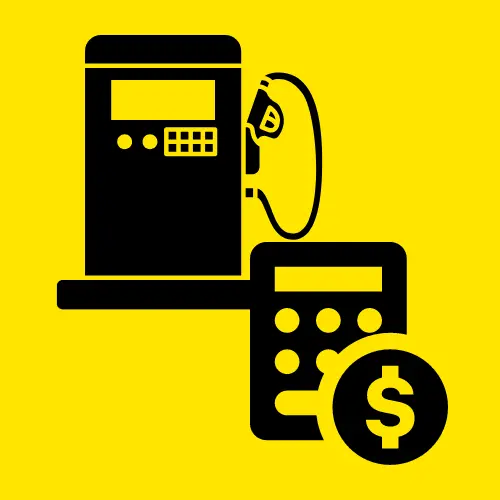
You’re planning a road trip and need to estimate the fuel cost. Write a C++ program that asks for the trip’s distance, the car’s fuel efficiency (in kilometers per liter), and the price of fuel per liter. The program should calculate the total fuel cost.
Test Case
Input:
Enter the distance of the trip (in km): 300
Enter the fuel efficiency of the car (km per liter): 15
Enter the price of fuel per liter: 2.5
Expected Output: Total fuel cost: $50
Explanation:
This program calculates the cost of fuel for a trip based on the distance, fuel efficiency, and fuel price.
- The code takes user input for total distance, fuel efficiency, and fuel price, storing them in
distance
,fuelEfficiency
, andfuelPrice
. - The program calculates the amount of fuel needed for the trip by dividing the total distance by the car’s fuel efficiency using the formula:
fuelNeeded = distance / fuelEfficiency
- It then calculates the total fuel cost by multiplying the amount of fuel needed by the price per liter using the formula:
totalCost = fuelNeeded * fuelPrice
#include <iostream> using namespace std; int main() { double distance, fuelEfficiency, fuelPrice, fuelNeeded, totalCost; cout << "Enter the distance of the trip (in km): "; cin >> distance; cout << "Enter the fuel efficiency of the car (km per liter): "; cin >> fuelEfficiency; cout << "Enter the price of fuel per liter: "; cin >> fuelPrice; fuelNeeded = distance / fuelEfficiency; totalCost = fuelNeeded * fuelPrice; cout << "Total fuel cost: $" << totalCost << endl; return 0; }
Area of a Room
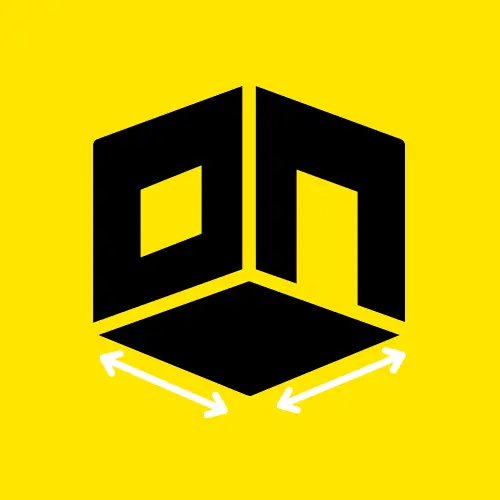
You’re working on a home improvement project and need to calculate the area of a rectangular room. Write a C++ program that asks for the length and width of the room and calculates the area.
Test Case
Input:
Enter the length of the room (in meters): 5
Enter the width of the room (in meters): 4
Expected Output: Area of the room: 20 square meters
Explanation:
This program calculates the area of a room using its length and width measurements. By multiplying these dimensions, the program determines the total floor area of the room.
- The first step requires inputting the room’s length and storing it as
length
. It then prompts for the room’s width, which is stored aswidth
. - The program calculates the area of the room by multiplying
length
bywidth
:Area = length * width
#include <iostream> using namespace std; int main() { double length, width, area; cout << "Enter the length of the room (in meters): "; cin >> length; cout << "Enter the width of the room (in meters): "; cin >> width; area = length * width; cout << "Area of the room: " << area << " square meters" << endl; return 0; }
Monthly Loan Payment for a New Laptop
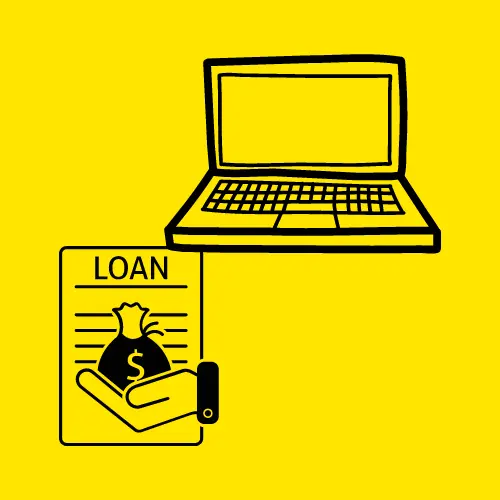
Imagine you’ve bought a new laptop for your studies and decided to finance it through a loan. You need to figure out your monthly payment based on the total amount of the loan, the monthly interest rate, and the number of months over which you’ll repay the loan. Use the formula: M=(P×(1+r))/N where M is the monthly payment, P is the total amount of the loan, r is the monthly interest rate, and N is the number of months. Write a program in C++ to calculate this.
Test Case
Input:
Enter the total loan amount for the laptop: $1200
Enter the monthly interest rate (as a decimal): 0.015
Enter the number of months for repayment: 12
Expected Output: Monthly payment: $101.5
Explanation:
This program calculates the monthly payment required for a loan to finance a new laptop. It takes into account the total loan amount, the monthly interest rate, and the number of months for repayment to determine the monthly payment.
- The program starts by entering the total loan amount as
loanAmount
, then the monthly interest rate asmonthlyRate
, and the number of months asmonths
. - The formula
monthlyPayment = (loanAmount * (1 + monthlyRate)) / months
calculates your monthly payment by adding a bit of interest to the loan amount and then splitting it into equal payments over the months. Interest is the extra money you pay on top of the borrowed amount.1 + monthlyRate
: This adds the interest to 1, showing how much more you will owe each month because of the interest. For example, if the interest rate is 0.015, adding it to 1 gives you 1.015.loanAmount * (1 + monthlyRate)
: This calculates the total amount you’ll owe after adding interest to the loan. For example, if you borrowed $1200 and the interest rate is 0.015, you multiply $1200 by 1.015 to get the total amount with interest.(loanAmount * (1 + monthlyRate)) / months
: This divides the total amount (including interest) by the number of months. This gives you the amount you need to pay each month. If the total with interest is $1218 and you’re paying over 12 months, dividing $1218 by 12 tells you the monthly payment.
#include <iostream> using namespace std; int main() { double loanAmount, monthlyRate, monthlyPayment; int months; cout << "Enter the total loan amount for the laptop: $"; cin >> loanAmount; cout << "Enter the monthly interest rate (as a decimal): "; cin >> monthlyRate; cout << "Enter the number of months for repayment: "; cin >> months; monthlyPayment = (loanAmount * (1 + monthlyRate)) / months; cout << "Monthly payment: $" << monthlyPayment << endl; return 0; }
Painting a Room
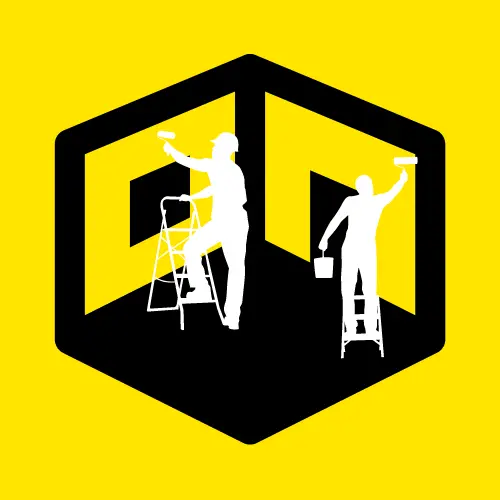
You’ve decided to paint your bedroom, and you want to calculate the total amount of paint needed. You know that one can of paint covers a specific area in square feet. Given the dimensions of your room (length, width, and height) and the area that one can of paint covers, determine how many cans of paint you need to buy. Assume all four walls are to be painted and you will not paint the ceiling or the floor. Write a program in C++ to perform this calculation.
Test Case
Input:
Enter the length of the room in feet: 12
Enter the width of the room in feet: 10
Enter the height of the room in feet: 8
Enter the coverage area per can in square feet: 350
Expected Output: Cans of paint needed: 1.00571
Explanation:
This program calculates the exact number of paint cans required to cover the walls of a room based on its dimensions and the coverage provided by each can. It ensures that you know precisely how much paint you’ll need for your project.
- The program inputs the room’s length, width, and height as
length
,width
, andheight
, along with the coverage area per can of paint, stored ascoveragePerCan
. - The program calculates the total area to be painted by considering the walls of the room:
- Multiply the length of the room by the height to get the area of one of the long walls:
length * height
. - Multiply the width of the room by the height to get the area of one of the short walls:
width * height
. - Add these two areas together:
length * height + width * height
- Since there are two of each type of wall (two long walls and two short walls), multiply the sum by 2:
2 * (length * height + width * height)
.
- Multiply the length of the room by the height to get the area of one of the long walls:
- The program then calculates the exact number of cans of paint needed using the formula:
- Cans of paint needed =
totalArea / coveragePerCan
- Cans of paint needed =
#include <iostream> using namespace std; int main() { double length, width, height, coveragePerCan, totalArea, cansNeeded; cout << "Enter the length of the room in feet: "; cin >> length; cout << "Enter the width of the room in feet: "; cin >> width; cout << "Enter the height of the room in feet: "; cin >> height; cout << "Enter the coverage area per can in square feet: "; cin >> coveragePerCan; totalArea = 2 * (length * height + width * height); cansNeeded = totalArea / coveragePerCan; cout << "Cans of paint needed: " << cansNeeded << endl; return 0; }
The Farmer’s Harvest Calculation
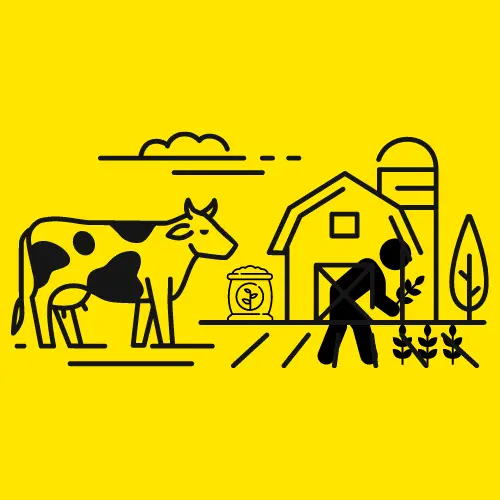
A farmer wants to calculate the total revenue from selling his crops: wheat, corn, and barley. He computes the revenue for each crop by multiplying the amount harvested by the price per kilogram. After finding the revenue for each type of crop, he adds them together to get the total revenue. Write a C++ program to perform these calculations.
Test Case
Input:
Enter the amount of wheat harvested (in kg): 100
Enter the price per kg of wheat: $2
Enter the amount of corn harvested (in kg): 150
Enter the price per kg of corn: $1.5
Enter the amount of barley harvested (in kg): 200
Enter the price per kg of barley: $1.8
Expected Output:Total Revenue: $785
Explanation:
In this program, the farmer is figuring out his total income by calculating the earnings from each type of crop he sells at the market.
- The program prompts the user to enter the amount of wheat, corn, and barley harvested, which are stored in the variables
wheatAmount
,cornAmount
, andbarleyAmount
, respectively. It also asks for the prices per kilogram for each crop, stored inwheatPrice
,cornPrice
, andbarleyPrice
. - The revenue from each crop is calculated by multiplying the harvested amount by the price per kilogram:
- Wheat Revenue: Stored in
wheatRevenue
aswheatAmount * wheatPrice
. - Corn Revenue: Stored in
cornRevenue
ascornAmount * cornPrice
. - Barley Revenue: Stored in
barleyRevenue
asbarleyAmount * barleyPrice
.
- Wheat Revenue: Stored in
- The revenues from wheat, corn, and barley are then added together to calculate the total revenue, which is stored in
totalRevenue
.
#include <iostream> using namespace std; int main() { double wheatAmount, wheatPrice, cornAmount, cornPrice, barleyAmount, barleyPrice, totalRevenue; cout << "Enter the amount of wheat harvested (in kg): "; cin >> wheatAmount; cout << "Enter the price per kg of wheat: $"; cin >> wheatPrice; cout << "Enter the amount of corn harvested (in kg): "; cin >> cornAmount; cout << "Enter the price per kg of corn: $"; cin >> cornPrice; cout << "Enter the amount of barley harvested (in kg): "; cin >> barleyAmount; cout << "Enter the price per kg of barley: $"; cin >> barleyPrice; double wheatRevenue = wheatAmount * wheatPrice; double cornRevenue = cornAmount * cornPrice; double barleyRevenue = barleyAmount * barleyPrice; totalRevenue = wheatRevenue + cornRevenue + barleyRevenue; cout << "Total Revenue: $" << totalRevenue << endl; return 0; }
Space Equipment Purchase
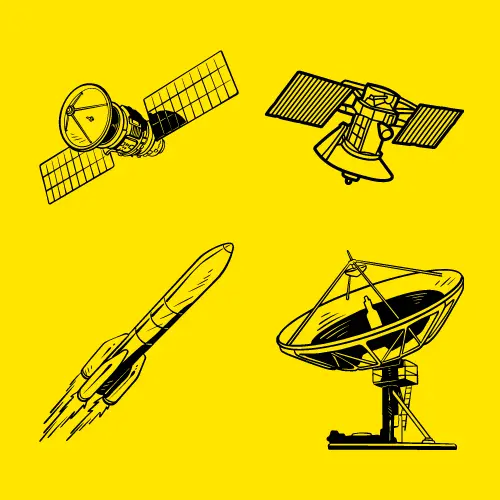
You’re preparing for an exciting adventure to a faraway planet, where you need special equipment to succeed. Each unit you buy has a price, and you know exactly how many units you need to take. Luckily, the supplier offers a discount of $60.00 for every 5 units you purchase. Before you complete your order, you need to figure out the total cost after using this discount, ensuring you have everything you need for the journey without spending too much. Write a C++ program to calculate the final cost after applying the discount.
Test Case
Input:
Enter the price per unit: 550
Enter the number of units: 12
Expected Output:
Final cost after discount: $6480
Explanation:
The program calculates the total cost by multiplying the unit price by the number of units. It then applies a $60 discount for every 5 units purchased and subtracts this total discount from the initial cost. Finally, it outputs the final cost after applying the discount.
- The program asks you to enter the
unitPrice
(price for each unit) andnumberOfUnits
(how many units you want to buy). - Next, it calculates the
totalCostBeforeDiscount
by multiplyingunitPrice
withnumberOfUnits
. - To determine the discount, the program calculates how many groups of 5 units you are buying using integer division (
numberOfUnits / 5
), giving you a discount for each group. - The total discount is then found by multiplying the number of groups by
$60
(the discount value). - Finally, the
finalCost
is calculated by subtractingtotalDiscount
fromtotalCostBeforeDiscount
.
#include <iostream> using namespace std; int main() { const float DISCOUNT_PER_BULK = 60.00; float unitPrice, totalCostBeforeDiscount, totalDiscount, finalCost; int numberOfUnits; cout << "Enter the price per unit: "; cin >> unitPrice; cout << "Enter the number of units: "; cin >> numberOfUnits; totalCostBeforeDiscount = unitPrice * numberOfUnits; int numberOfDiscounts = numberOfUnits / 5; totalDiscount = numberOfDiscounts * DISCOUNT_PER_BULK; finalCost = totalCostBeforeDiscount - totalDiscount; cout << "Final cost after discount: $" << finalCost << endl; return 0; }
Land Plot Calculator
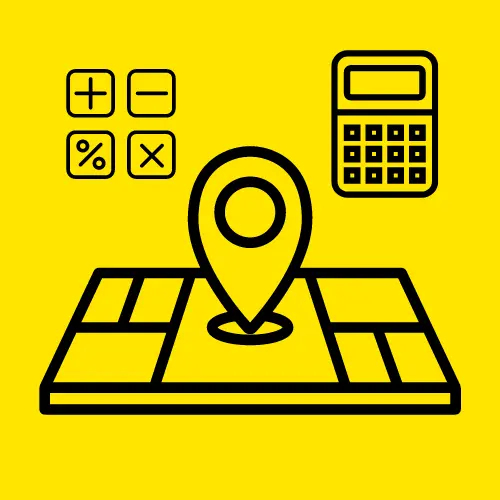
Imagine a farmer who wants to divide a big rectangular field into smaller square plots for planting. The farmer knows the total area of the field and the size of each square plot. To plan the planting, the farmer needs to find out how many of these square plots can fit in the field and how much area will be left over. Write a program in C++ to help the farmer calculate the number of complete square plots that can fit and the leftover area.
Test Case
Input:
Enter the total area of the land: 1000
Enter the side length of one square plot: 15
Expected Output:
Number of complete square plots: 4
Remaining area: 100
Explanation:
The code computes how many square plots fit into a given land area and calculates the leftover space. It does this by dividing the total area by the plot area and subtracting the space used by the plots. It then displays the number of plots and the remaining area.
- The code begins by taking user inputs for
total_area
andside_length
of the square plots. - It calculates the area of one square plot using
plot_area = side_length * side_length
. This is done by multiplyingside_length
by itself. - The number of complete square plots that can fit into the total area is computed with
full_plots = total_area / plot_area
. This uses integer division to determine how many whole plots fit into thetotal_area
. - The remaining area that cannot be used for a complete plot is calculated with
remaining_area = total_area - (full_plots * plot_area)
. This subtracts the area occupied by the full plots from the total land area.
#include <iostream> using namespace std; int main() { double total_area, side_length; cout << "Enter the total area of the land: "; cin >> total_area; cout << "Enter the side length of one square plot: "; cin >> side_length; double plot_area = side_length * side_length; int full_plots = total_area / plot_area; double remaining_area = total_area - (full_plots * plot_area); cout << "Number of complete square plots: " << full_plots << endl; cout << "Remaining area: " << remaining_area << endl; return 0; }
Digital Clock Seconds Calculation
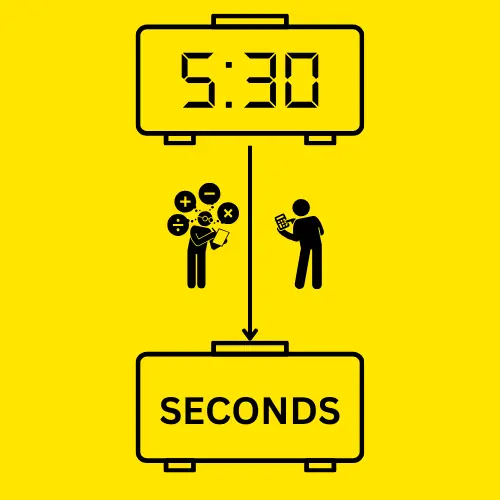
You have a digital clock that shows hours, minutes, and seconds. Each time you set the clock, you need to know how many total seconds have passed since midnight. Write a program in C++ to convert the given time into the total number of seconds from the start of the day, making it easier to manage and track time with your clock.
Test Case
Input:
Enter hours: 2
Enter minutes: 30
Enter seconds: 45
Expected Output:
Total seconds since midnight: 9045
Explanation:
The code converts hours, minutes, and seconds into total seconds since midnight. It multiplies hours by 3600
(seconds per hour) and minutes by 60
(seconds per minute) to get the total seconds for each unit. The formula combines these values with the remaining seconds to give the total number of seconds since midnight.
- The code takes user inputs for
hours
,minutes
, andseconds
. - It calculates the total number of seconds since midnight by:
- Converting hours to seconds by multiplying
hours
by3600
(since there are3600
seconds in an hour: 60 minutes/hour * 60 seconds/minute). - Converting minutes to seconds by multiplying
minutes
by60
(since there are60
seconds in a minute). - Adding the remaining
seconds
.
- Converting hours to seconds by multiplying
- Finally, it outputs the total number of seconds since midnight.
#include <iostream> using namespace std; int main() { int hours, minutes, seconds; cout << "Enter hours: "; cin >> hours; cout << "Enter minutes: "; cin >> minutes; cout << "Enter seconds: "; cin >> seconds; int total_seconds = (hours * 3600) + (minutes * 60) + seconds; cout << "Total seconds since midnight: " << total_seconds << endl; return 0; }
Construction Materials Calculation
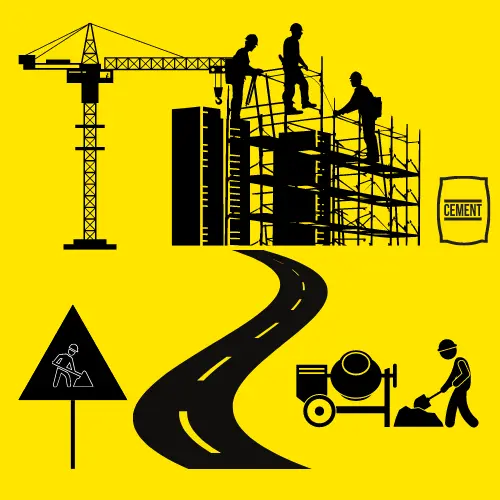
As a project manager for a large construction site, you need to determine the amount of cement required to complete the foundation of a building. The foundation needs a certain volume of concrete to be poured. Each bag of cement contributes a specific volume to the concrete mix, and you can only order cement in full pallets, where each pallet contains a fixed number of bags. To meet or exceed the required concrete volume, you need to calculate how many pallets of cement are necessary. Additionally, because you can only order full pallets, there will be some excess concrete due to the rounding up of pallets.
Write a C++ program to:
1. Calculate the minimum number of pallets needed to meet or exceed the required volume of concrete.
2. Determine the amount of excess concrete produced due to ordering full pallets.
Test Case
Input:
Enter the required volume of concrete in cubic meters: 1000
Enter the volume contributed by one bag of cement in cubic meters: 2
Enter the number of bags in one pallet: 40
Expected Output:
Total pallets required: 13
Excess concrete produced: 40 cubic meters
Explanation:
The code prompts the user for the required concrete volume, cement volume per bag, and bags per pallet. It calculates the total number of bags and pallets needed, rounding up as necessary. The excess concrete is then determined by comparing the total concrete from the pallets to the required volume, and the results are displayed.
- The code begins by prompting the user to enter three input values: the total volume of concrete needed (
requiredConcreteVolume
), the volume contributed by each cement bag (cementVolumePerBag
), and the number of bags per pallet (bagsPerPallet
). - Next, the program calculates how many cement bags are required. It does this by dividing the total concrete volume needed by the volume provided by one bag of cement. Since you can’t order a fraction of a bag, it rounds up to ensure there are enough bags. This calculation uses the formula
(requiredConcreteVolume + cementVolumePerBag - 1) / cementVolumePerBag
. - After determining the total number of bags, the program calculates how many pallets of cement are needed. Since pallets come in whole numbers and each pallet contains a specific number of bags, it again rounds up to the nearest whole number. This is done with the formula
(totalBagsRequired + bagsPerPallet - 1) / bagsPerPallet
. - To calculate the excess concrete, it finds out how much concrete the total number of pallets will produce and subtracts the required volume, resulting in
(palletsRequired * bagsPerPallet * cementVolumePerBag) - requiredConcreteVolume
.
#include <iostream> using namespace std; int main() { int requiredConcreteVolume; int cementVolumePerBag; int bagsPerPallet; cout << "Enter the required volume of concrete in cubic meters: "; cin >> requiredConcreteVolume; cout << "Enter the volume contributed by one bag of cement in cubic meters: "; cin >> cementVolumePerBag; cout << "Enter the number of bags in one pallet: "; cin >> bagsPerPallet; int totalBagsRequired = (requiredConcreteVolume + cementVolumePerBag - 1) / cementVolumePerBag; int palletsRequired = (totalBagsRequired + bagsPerPallet - 1) / bagsPerPallet; int excessConcreteProduced = (palletsRequired * bagsPerPallet * cementVolumePerBag) - requiredConcreteVolume; cout << "Total pallets required: " << palletsRequired << endl; cout << "Excess concrete produced: " << excessConcreteProduced << " cubic meters" << endl; return 0; }
Inventory Code Analysis
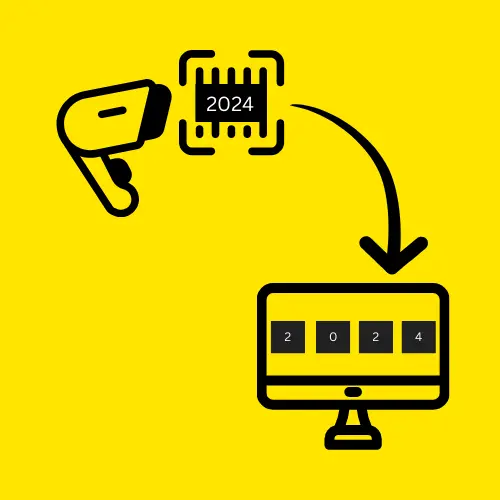
You are working on a system that processes inventory codes, where each code is a four-digit number used to identify different product categories. Each product gets a unique four-digit code to help categorize it accurately within the inventory. To improve the system’s functionality, you need to write a C++ program. This program should read a four-digit inventory code from the user, separate the code into its individual digits, and then display each digit separately. This process will help in better managing and analyzing the product information.
Test Case
Input:
Enter a four-digit inventory code: 2024
Expected Output:
Digits of the inventory code are:
Thousands place: 2
Hundreds place: 0
Tens place: 2
Ones place: 4
Explanation:
The program reads a four-digit inventory code and separates each digit. It calculates the thousands place by dividing the code by 1000, the hundreds place by dividing the code by 100 and then taking the remainder when divided by 10, the tens place by dividing the code by 10 and taking the remainder when divided by 10, and the ones place by taking the remainder when divided by 10. Finally, it displays each digit separately.
- The program starts by reading a four-digit inventory code from the user. This code is stored in the variable
inventoryCode
. - To get the thousands place digit, the program performs integer division by 1000:
inventoryCode / 1000
. This operation removes the last three digits, leaving only the thousands place digit. - To obtain the hundreds place digit, the program first divides
inventoryCode
by 100:inventoryCode / 100
. This operation removes the last two digits, leaving the hundreds place digit along with the tens and ones places. To get just the hundreds place, the program then calculates the remainder when divided by 10:(inventoryCode / 100) % 10
. This operation removes the tens and ones places, leaving only the hundreds place digit. - The tens place digit is found by dividing
inventoryCode
by 10:inventoryCode / 10
. It removes the ones place digit, leaving the tens and hundreds places. To get just the tens place, the program calculates the remainder when divided by 10:(inventoryCode / 10) % 10
. This operation removes the hundreds place, leaving only the tens place digit. - The ones place digit is obtained directly by calculating the remainder when
inventoryCode
is divided by 10:inventoryCode % 10
. This operation isolates the ones place digit, leaving it as the result.
#include <iostream> using namespace std; int main() { // Declare variable for the inventory code int inventoryCode; // Input the inventory code from the user cout << "Enter a four-digit inventory code: "; cin >> inventoryCode; // Extract each digit from the inventory code int thousands = inventoryCode / 1000; // Extract the thousands place int hundreds = (inventoryCode / 100) % 10; // Extract the hundreds place int tens = (inventoryCode / 10) % 10; // Extract the tens place int ones = inventoryCode % 10; // Extract the ones place // Output each digit separately cout << "Digits of the inventory code are:" << endl; cout << "Thousands place: " << thousands << endl; cout << "Hundreds place: " << hundreds << endl; cout << "Tens place: " << tens << endl; cout << "Ones place: " << ones << endl; return 0; }
Profit Margin Calculation
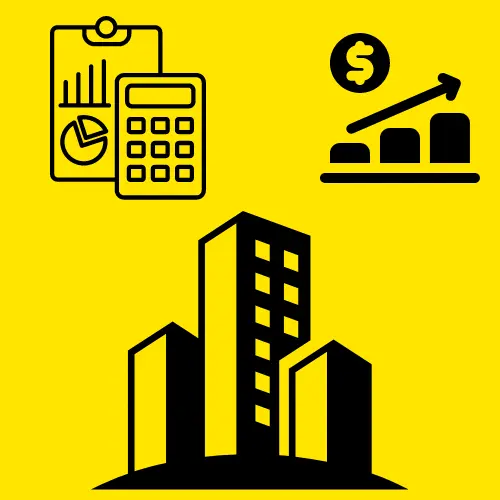
A company sells a product for a certain amount. The costs to make the product and extra expenses like shipping and taxes are also known. You need to determine the profit margin percentage, which shows how much profit is made compared to the selling price. This percentage shows the profit as a part of the selling price. Write a program in C++ that takes the selling price, production cost, and additional expenses as input, and then calculates and displays the profit margin percentage.
Test Case
Input:
Enter the selling price of the product: 200
Enter the production cost of the product: 120
Enter additional costs (shipping, taxes, etc.): 30
Expected Output:
Profit Margin Percentage: 25%
Explanation:
The user inputs the selling price, production cost, and additional costs. The program calculates the total costs, and then determines the profit by subtracting these costs from the selling price. Finally, it computes the profit margin percentage and displays the result, showing the profit as a percentage of the selling price.
- First, the user inputs the selling price (
sellingPrice
), production cost (productionCost
), and additional costs (additionalCosts
). - Next, the total costs are calculated by adding
productionCost
andadditionalCosts
with the linetotalCosts = productionCost + additionalCosts
. - To determine the profit, the program subtracts the total costs from the selling price using
profit = sellingPrice - totalCosts
. - The profit margin percentage is then calculated by dividing the profit by the selling price and multiplying by 100 with
profitMarginPercentage = (profit * 100) / sellingPrice
.
#include <iostream> using namespace std; int main() { int sellingPrice, productionCost, additionalCosts; cout << "Enter the selling price of the product: "; cin >> sellingPrice; cout << "Enter the production cost of the product: "; cin >> productionCost; cout << "Enter additional costs (shipping, taxes, etc.): "; cin >> additionalCosts; int totalCosts = productionCost + additionalCosts; int profit = sellingPrice - totalCosts; int profitMarginPercentage = (profit * 100) / sellingPrice; cout << "Profit Margin Percentage: " << profitMarginPercentage << "%" << endl; return 0; }
Subscription Budget Planner
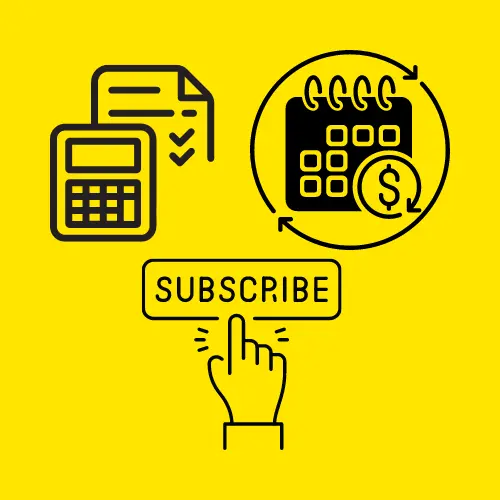
You want to enroll in an online course platform that requires a monthly subscription fee. You have some initial savings set aside and plan to save a fixed amount each month to cover the subscription cost. To manage your finances effectively, you want to calculate your total savings after a certain number of months, determine how many full months of subscriptions you can afford, and find out how much money will be left after paying for those months. Additionally, you want to know how much more you need to save to afford another full month of subscription. This way, you can plan your savings to maintain the subscription as long as possible and understand what additional savings are required to extend it further. Write C++ code to perform these calculations and display the results based on the inputs for initial savings, monthly savings, subscription fees, and the number of months you plan to save.
Test Case
Input:
Enter initial savings: 100
Enter monthly savings: 50
Enter monthly subscription fee: 60
Enter the number of months you plan to save: 6
Expected Output:
After 6 months:
Total Savings: 400
Full Months Affordable: 6
Remaining Money: 40
Additional Savings Needed for Next Month: 20
Explanation:
It calculates how many full months of a subscription can be covered based on initial and monthly savings combined with the subscription fee. First, it computes the total savings after a given number of months, then determines the number of full months that can be afforded by dividing this total by the subscription fee. Finally, it calculates the remaining money after these months and how much additional savings are needed for the next month.
- The program prompts the user to input four values:
initial_savings
,monthly_savings
,monthly_subscription_fee
, andmonths
, which represent the initial amount of money saved, the amount saved each month, the cost of the monthly subscription, and the number of months planned for saving, respectively. - It calculates the total savings after the given number of months using the formula
total_savings = initial_savings + months * monthly_savings
where the total savings are computed by adding the initial savings to the product of the number of months and the amount saved each month. - The code then determines how many full months of subscription can be afforded with the total savings by performing integer division:
full_months_affordable = total_savings / monthly_subscription_fee
. This calculation ignores any fractional months by storing the result as an integer. - After that, it calculates how much money is left after paying for the full month using
remaining_money = total_savings - (full_months_affordable * monthly_subscription_fee
, which subtracts the total cost of the full month of subscription from the total savings. - The program also determines how much additional savings are needed to afford another full month of subscription using
additional_savings_needed = monthly_subscription_fee - remaining_money
, which calculates the difference between the monthly subscription fee and the remaining money.
#include <iostream> using namespace std; int main() { double initial_savings; double monthly_savings; double monthly_subscription_fee; int months; cout << "Enter initial savings: "; cin >> initial_savings; cout << "Enter monthly savings: "; cin >> monthly_savings; cout << "Enter monthly subscription fee: "; cin >> monthly_subscription_fee; cout << "Enter the number of months you plan to save: "; cin >> months; double total_savings = initial_savings + months * monthly_savings; int full_months_affordable = total_savings / monthly_subscription_fee; double remaining_money = total_savings - (full_months_affordable * monthly_subscription_fee); double additional_savings_needed = monthly_subscription_fee - remaining_money; cout << "\nAfter " << months << " months:\n"; cout << "Total Savings: " << total_savings << "\n"; cout << "Full Months Affordable: " << full_months_affordable << "\n"; cout << "Remaining Money: " << remaining_money << "\n"; cout << "Additional Savings Needed for Next Month: " << additional_savings_needed << "\n"; return 0; }
Total Travel Time Calculation
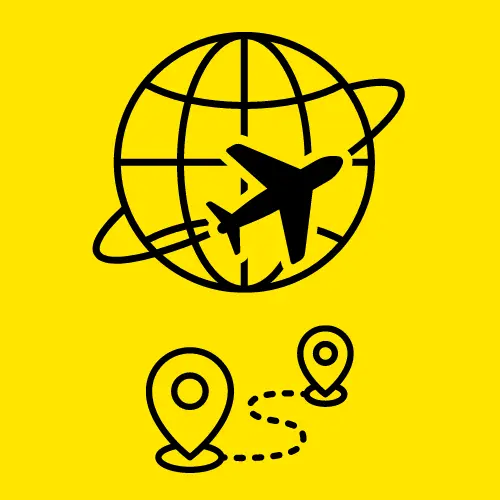
You are a travel coordinator who needs to prepare a detailed schedule for a passenger’s trip. The passenger’s journey includes a main flight, a layover, and a connecting flight. To help the passenger understand how long their entire trip will take, you need to add up the time of the main flight, the layover, and the connecting flight. Each part of the trip is given in hours and minutes. Your task is to write code in C++ to calculate the total travel time and show it in hours and minutes.
Test Case
Input:
Enter the main flight duration in hours: 3
Enter the main flight duration in minutes: 45
Enter the layover duration in hours: 2
Enter the layover duration in minutes: 30
Enter the connecting flight duration in hours: 1
Enter the connecting flight duration in minutes: 15
Expected Output:
Total time spent traveling, including layovers and connecting flights, is: 7 hours and 30 minutes.
Explanation:
To determine the total travel time, first convert each duration into minutes by multiplying hours by 60 and adding minutes for the main flight, layover, and connecting flight. Next, sum these total minutes to get the overall travel time. Finally, convert the total minutes back into hours and minutes by dividing by 60 to get the hours and using the modulus operator to find the remaining minutes.
- The program prompts the user to input the required six values.
- To calculate the total minutes for the main flight, first convert the hours into minutes. Multiply
mainFlightHours
by 60, which is done with(mainFlightHours * 60)
. Then add themainFlightMinutes
to this result, as intotalMainFlightMinutes = (mainFlightHours * 60) + mainFlightMinutes
. - For the layover, perform a similar conversion. Multiply
layoverHours
by 60 to get the minutes, which is done with(layoverHours * 60)
. AddlayoverMinutes
to this, resulting intotalLayoverMinutes = (layoverHours * 60) + layoverMinutes
. - Convert the connecting flight duration into total minutes by multiplying
connectingFlightHours
by 60, and then addconnectingFlightMinutes
. This is represented bytotalConnectingFlightMinutes = (connectingFlightHours * 60) + connectingFlightMinutes
. - Sum the minutes for all segments to get the total travel time. Add
totalMainFlightMinutes
,totalLayoverMinutes
, andtotalConnectingFlightMinutes
together withtotalTimeInMinutes = totalMainFlightMinutes + totalLayoverMinutes + totalConnectingFlightMinutes
. - To find the total hours, divide
totalTimeInMinutes
by 60 withtotalHours = totalTimeInMinutes / 60
. For the remaining minutes, use the modulus operator to get the remainder after dividing by 60, likeremainingMinutes = totalTimeInMinutes % 60
.
#include <iostream> using namespace std; int main() { int mainFlightHours, mainFlightMinutes; int layoverHours, layoverMinutes; int connectingFlightHours, connectingFlightMinutes; cout << "Enter the main flight duration in hours: "; cin >> mainFlightHours; cout << "Enter the main flight duration in minutes: "; cin >> mainFlightMinutes; cout << "Enter the layover duration in hours: "; cin >> layoverHours; cout << "Enter the layover duration in minutes: "; cin >> layoverMinutes; cout << "Enter the connecting flight duration in hours: "; cin >> connectingFlightHours; cout << "Enter the connecting flight duration in minutes: "; cin >> connectingFlightMinutes; int totalMainFlightMinutes = (mainFlightHours * 60) + mainFlightMinutes; int totalLayoverMinutes = (layoverHours * 60) + layoverMinutes; int totalConnectingFlightMinutes = (connectingFlightHours * 60) + connectingFlightMinutes; int totalTimeInMinutes = totalMainFlightMinutes + totalLayoverMinutes + totalConnectingFlightMinutes; int totalHours = totalTimeInMinutes / 60; int remainingMinutes = totalTimeInMinutes % 60; cout << "Total time spent traveling, including layovers and connecting flights, is: " << totalHours << " hours and " << remainingMinutes << " minutes." << endl; return 0; }
Car Loan Plan
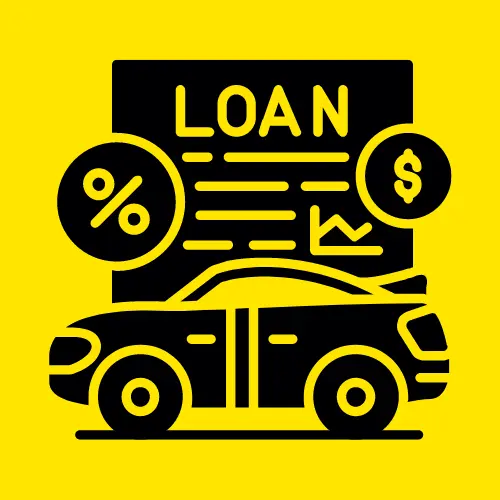
You’ve decided to buy your first car and need to take out a loan to finance it. You have a specific budget in mind for your monthly installment and know the total cost of the car. You want to calculate how many months it will take to pay off the loan based on your monthly installment and the interest rate applied to the loan. Additionally, you want to know the total cost of the loan, including interest. Write a C++ program that calculates the number of months required to repay the loan and the total cost, including the interest.
Test Case
Input:
Car cost: 20000
Down payment: 5000
Interest rate: 5
Monthly installment: 300
Expected Output:
Total loan amount (including interest): 15750
Number of months to pay off the loan: 52.5
Explanation:
- The program begins by taking input from the user for the total cost of the car, the down payment, the annual interest rate, and the desired monthly installment amount. These values are stored in the variables
carCost
,downPayment
,interestRate
, andmonthlyInstallment
. - To calculate the amount of the loan required, the program subtracts the down payment from the total cost of the car.
- Next, the program calculates the interest by multiplying the loan amount with the interest rate and dividing it by 100 to find how much extra you need to pay due to interest.
- The program then adds the calculated interest to the original loan amount to find the total amount that needs to be repaid.
- To determine how many months it will take to pay off the loan, the program divides the total loan amount by the monthly installment amount. This gives the number of months required to repay the loan fully.
- Finally, the program outputs the total loan amount including interest and the number of months required to pay off the loan, providing the user with a clear understanding of their financial obligation.
#include <iostream> using namespace std; int main() { double carCost, downPayment, interestRate, monthlyInstallment; cout << "Enter the total cost of the car: "; cin >> carCost; cout << "Enter the down payment: "; cin >> downPayment; cout << "Enter the annual interest rate (in %): "; cin >> interestRate; cout << "Enter your planned monthly installment: "; cin >> monthlyInstallment; double loanAmount = carCost - downPayment; double interestAmount = (loanAmount * interestRate) / 100; double totalLoanAmount = loanAmount + interestAmount; double monthsToPayOff = totalLoanAmount / monthlyInstallment; cout << "Total loan amount (including interest): " << totalLoanAmount << endl; cout << "Number of months to pay off the loan: " << monthsToPayOff << endl; return 0; }
Pocket Money Saver
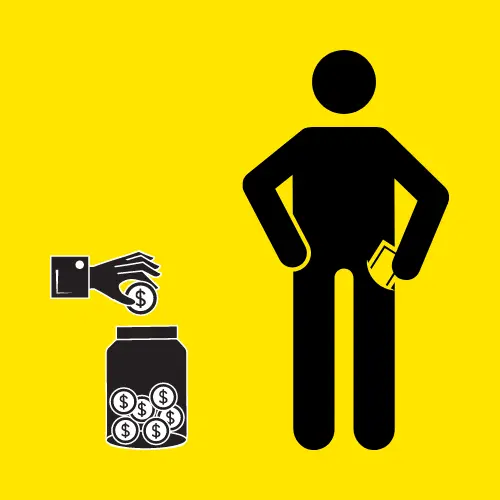
You receive a base pocket money amount each week, which you enter into the system, and you have a weekly expense. For the week, a performance multiplier of 1.25 is applied to your base pocket money. Additionally, if your total savings after considering the expense exceed $120, you receive a fixed bonus of $30. On the other hand, if your total savings fall below $60, you face a fixed penalty of $20. Calculate your total savings after one week. Write a program in C++ to perform this calculation.
Test Case
Input:
Enter your base pocket money: $110
Enter your weekly expense: $10
Expected Output: Total savings after one week: $151.875
Explanation:
The program calculates weekly savings by multiplying the base pocket money by a performance multiplier of 1.25 to reflect a 25% increase in earnings. After this adjustment, the weekly expense is subtracted to determine the net savings. Additionally, bonuses or penalties are applied based on whether the final savings exceed or fall below certain thresholds.
- The user inputs their base pocket money and weekly expenses which are stored in
base_money
andexpense
, respectively. - The formula
weekly_savings = base_money * multiplier - expense
calculates your weekly savings by first boosting your base money by 25% using a multiplier of 1.25 to reflect improved performance, then subtracting your expenses to determine the remaining savings. The multiplier (1.25) is used to increase the base pocket money by 25% to reflect improved performance or additional earnings. It boosts the base amount to reward the user based on their performance. - The formula
bonus_or_penalty = bonus * ((weekly_savings - bonus_threshold) / bonus_threshold) - penalty * ((penalty_threshold - weekly_savings) / penalty_threshold)
adjusts your weekly savings based on how much your savings are above or below certain limits.- First, it calculates a bonus if
weekly_savings
is more thanbonus_threshold
. The partbonus * ((weekly_savings - bonus_threshold) / bonus_threshold)
finds out how much your savings are above the threshold. It does this by subtractingbonus_threshold
fromweekly_savings
and then dividing that number bybonus_threshold
to get a percentage. This percentage is used to calculate the bonus amount. - Next, it calculates a penalty if
weekly_savings
is less thanpenalty_threshold
. The partpenalty * ((penalty_threshold - weekly_savings) / penalty_threshold)
figures out how much less your savings are compared to the threshold. It subtractsweekly_savings
frompenalty_threshold
, divides that bypenalty_threshold
to get a percentage, and uses this to calculate the penalty amount. - The formula then subtracts the penalty from the bonus to get the final adjustment. This adjustment is added to your
weekly_savings
to show the total amount. If the bonus is higher than the penalty, your savings go up. If the penalty is higher, your savings go down.
- First, it calculates a bonus if
- Calculate the final savings by adding or subtracting the bonus or penalty to/from
weekly_savings
. This is given by:final_savings = weekly_savings + bonus_or_penalty
.
#include <iostream> using namespace std; int main() { const double multiplier = 1.25; const int bonus = 30; const int penalty = 20; const int bonus_threshold = 120; const int penalty_threshold = 60; int base_money; int expense; cout << "Enter your base pocket money: $"; cin >> base_money; cout << "Enter your weekly expense: $"; cin >> expense; double weekly_savings = base_money * multiplier - expense; double bonus_or_penalty = bonus * ((weekly_savings - bonus_threshold) / bonus_threshold) - penalty * ((penalty_threshold - weekly_savings) / penalty_threshold); double final_savings = weekly_savings + bonus_or_penalty; cout << "Total savings after one week: $" << final_savings << endl; return 0; }
Using arithmetic operators in scenario-based coding questions in C++ is an excellent way to apply your programming skills to real-life situations. Practicing these scenarios will teach you how to handle various calculations, making your code more practical and effective. Keep challenging yourself and trying out new ideas to get better at solving real-life problems.