Ever need to repeat a task over and over while programming in C++? Nested loops in C++ are perfect for that! Whether it’s organizing seats at a theater, calculating late fees at a library, or even building a staircase, nested loops help you manage repeated tasks in a clean and efficient way. Let’s dive into these challenges and see how they work!
Important Note: You can expand the dropdown to see the test case and the solution code for each question.
Multiplication Table Generator
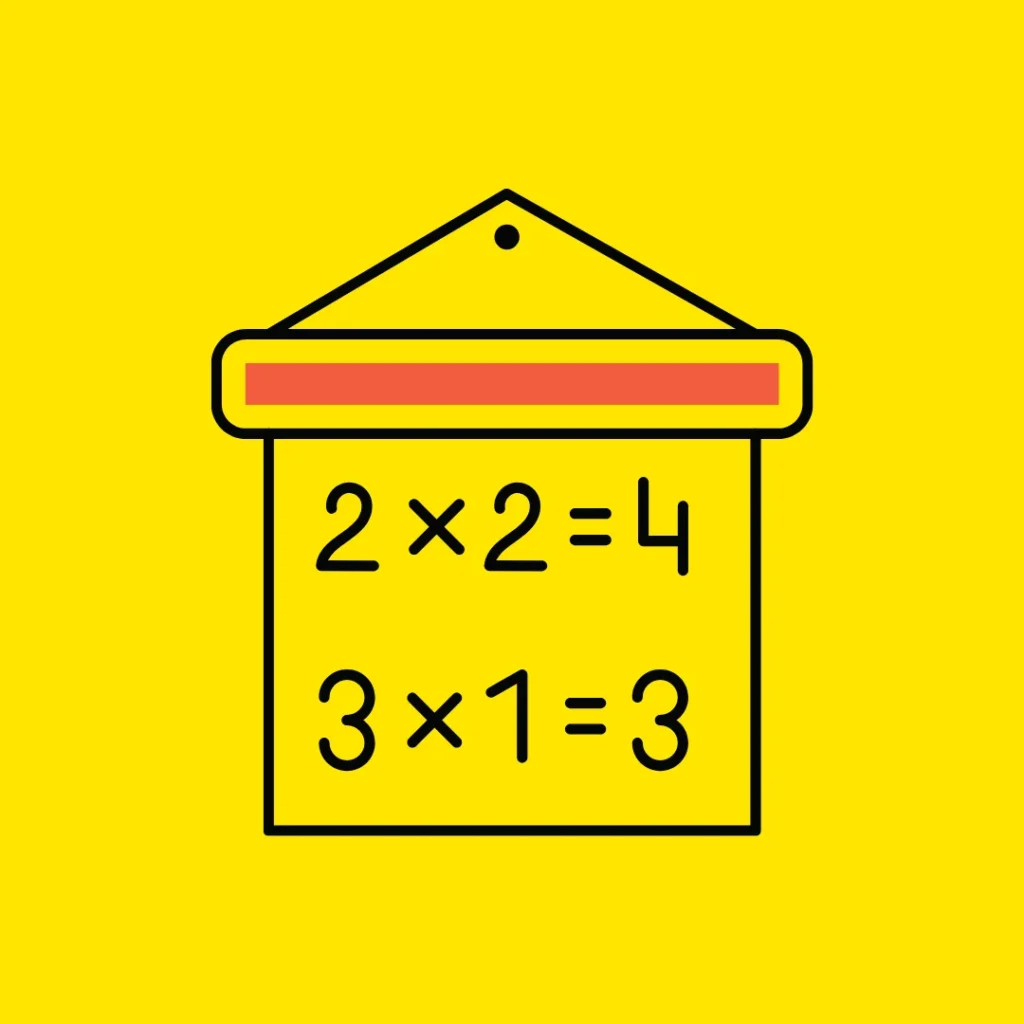
You are a student who is designing a simple multiplication table generator for a school project. The user enters a number, and you need to generate a multiplication table for that number from 1 to 10. Write a C++ program using nested while loops to display the multiplication table in a structured format.
Test Case
Input:
Enter a number: 3
Output:
3 x 1 = 3
3 x 2 = 6
3 x 3 = 9
3 x 4 = 12
3 x 5 = 15
3 x 6 = 18
3 x 7 = 21
3 x 8 = 24
3 x 9 = 27
3 x 10 = 30
Explanation:
- The program asks the user to enter a number, which is 3 in this case.
- The outer
while
loop runs only once becausej
starts at 1 and increases to 2 after the first execution, making the condition false. Inside the outer loop, the innerwhile
loop starts with 1 and goes up to 10. - Each time, it multiplies 3 by the current number and prints the result.
- The numbers increase one by one: first 3 × 1 = 3, then 3 × 2 = 6, then 3 × 3 = 9, and so on until 3 × 10 = 30.Once 10 calculations are printed, the inner loop stops.
- The outer loop also stops since
j
is now 2, and the program ends.
#include <iostream> using namespace std; int main() { int num, i = 1, j = 1; // Taking user input for the number cout << "Enter a number: "; cin >> num; // Outer while loop to iterate from 1 to 1 (just to keep it nested) while (j == 1) { // Inner while loop to iterate from 1 to 10 while (i <= 10) { // Printing the multiplication result cout << num << " x " << i << " = " << num * i << endl; i++; } // Exiting the outer while loop after the inner loop completes j++; } return 0; }
Counting Coins in a Grid
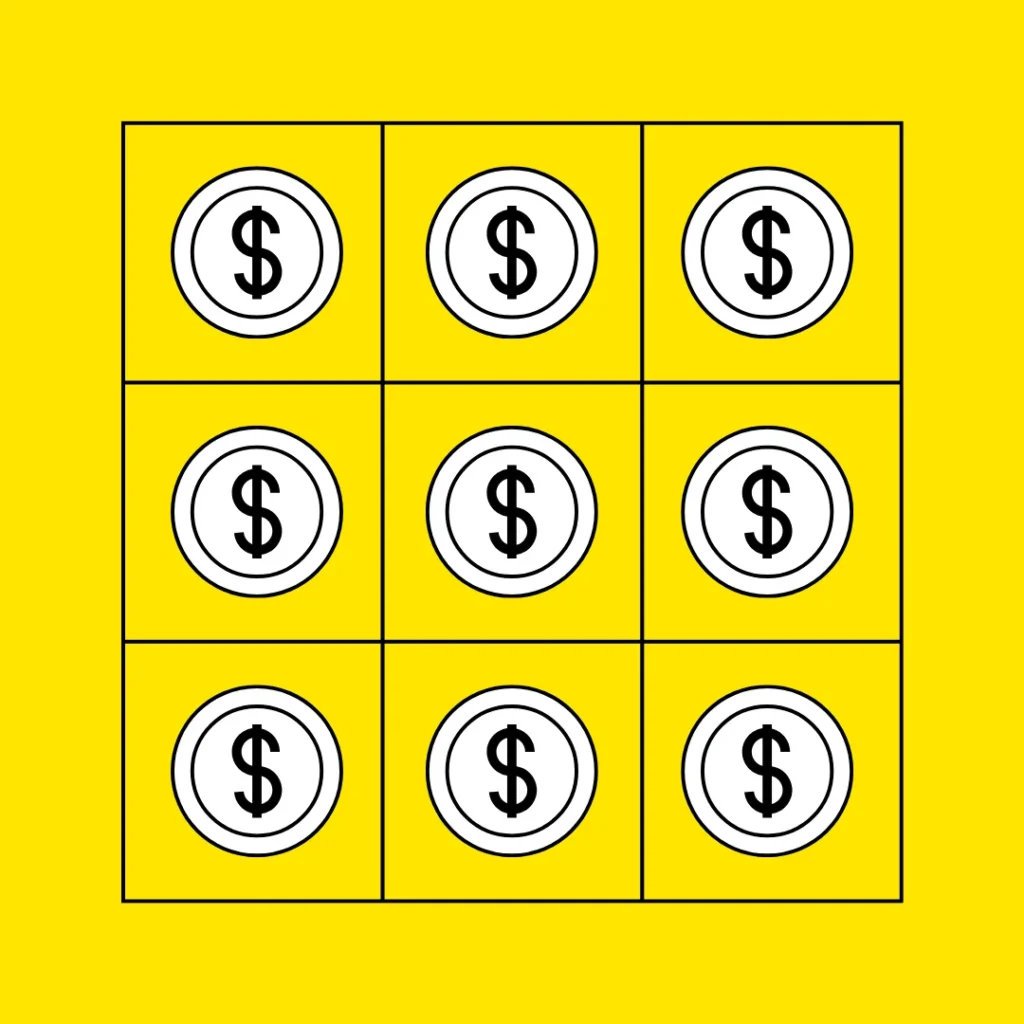
You are playing a treasure hunt game where you have a grid of coins. The grid has multiple rows and columns, and every position contains a coin. You need to display the grid of coins based on the number of rows and columns the user has entered. Write a C++ program using nested do-while loops to generate a grid filled with coins, where each coin is represented by a dollar sign ($).
Test Case
Input:
Enter number of rows: 2
Enter number of columns: 4
Output:
$ $ $ $
$ $ $ $
Explanation:
- Here, the user inputs 2 rows and 4 columns.
- The outer
do-while
loop will run twice (once for each row) because the value ofi
starts at 0 and increments after each iteration. - Inside the outer loop, the inner
do-while
loop runs four times for each row because there are 4 columns. It prints the$
symbol each time. - In the first iteration, the inner loop prints
$ $ $ $
. After the first row is printed, the program moves to the next line and prints the second row:$ $ $ $
. - Once the second row is printed, the outer loop ends, and the program stops.
#include <iostream> using namespace std; int main() { int rows, columns; // Taking user input for the grid size cout << "Enter number of rows: "; cin >> rows; cout << "Enter number of columns: "; cin >> columns; int i = 0; do { int j = 0; do { cout << "$ "; // Print coin symbol j++; } while (j < columns); cout << endl; // Move to the next row i++; } while (i < rows); return 0; }
Building a Staircase
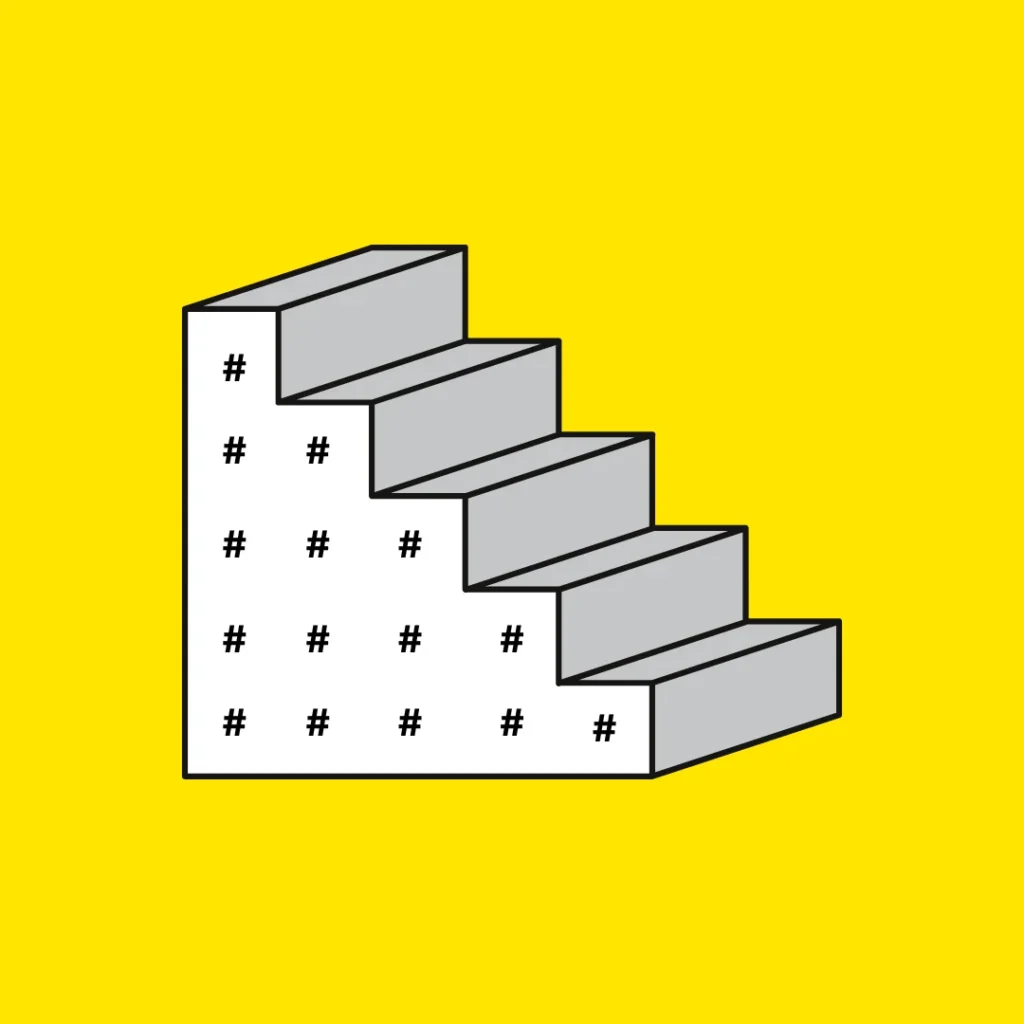
You are a carpenter building a wooden staircase. The staircase consists of steps, and each step has an increasing number of wooden planks. The first step has 1 plank, the second has 2 planks, the third has 3 planks, and so on. Write a C++ program using nested for loops to display a staircase pattern represented by hashtag (#), based on the number of steps provided by the user.
Test Case
Input:
Enter number of steps: 4
Output:
#
# #
# # #
# # # #
Explanation:
- In this case, the user enters 4 steps as input.
- The outer
for
loop runs 4 times, once for each step (since the number of steps is 4). - In the first iteration, the inner
for
loop runs once to print one#
symbol, representing the first step. - The same applies to the other remaining loops: the second iteration prints two
##
symbols, the third prints three###
symbols, and the fourth prints four####
symbols. - Each time the inner loop completes, the outer loop moves to the next line, creating the staircase effect.
#include <iostream> using namespace std; int main() { int steps; // Taking user input for the number of steps cout << "Enter number of steps: "; cin >> steps; // Outer loop for each step for (int i = 1; i <= steps; i++) { // Inner loop for printing the '#' symbols for (int j = 1; j <= i; j++) { cout << "# "; } cout << endl; // Move to the next step } return 0; }
Store Shelf Organization
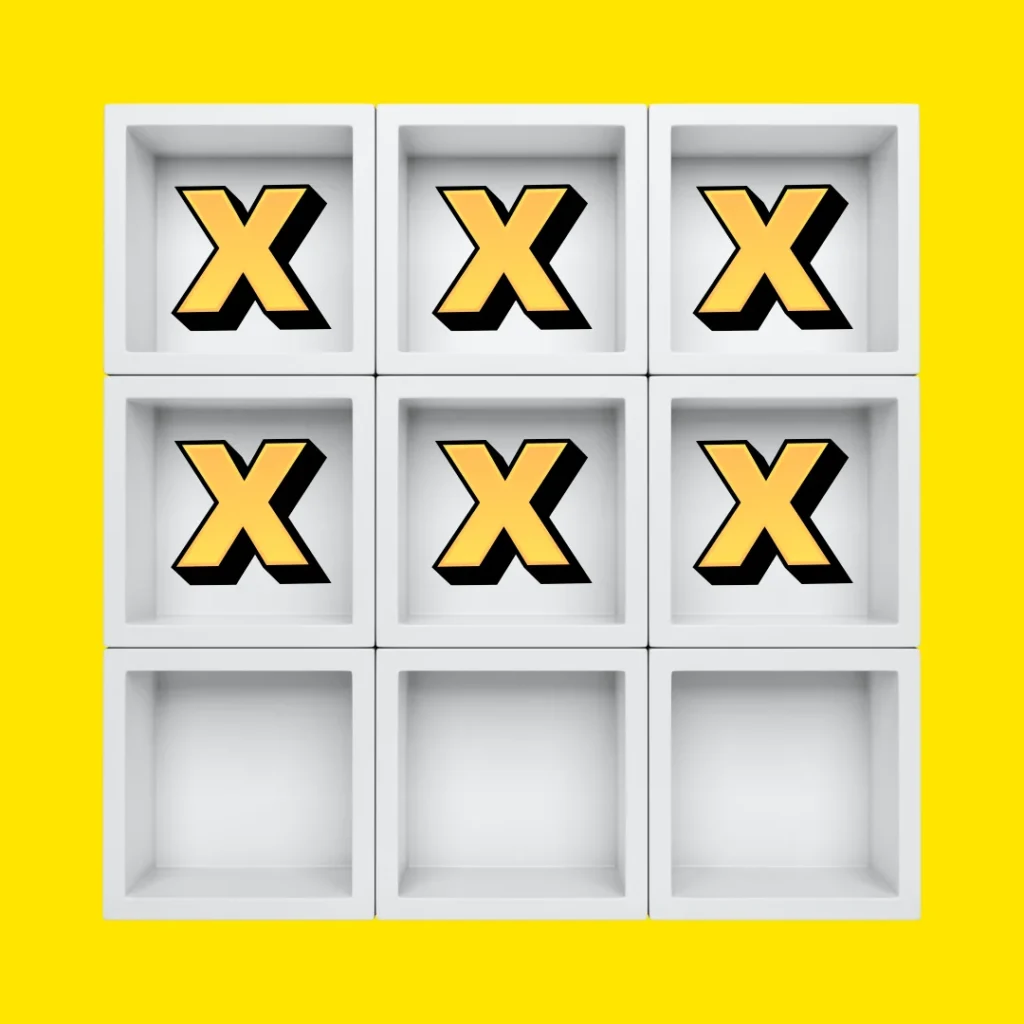
You’re working at a store where you need to organize products on shelves. Each shelf has multiple products, and the store has multiple shelves. The user will input the number of shelves and the number of products per shelf. Write a C++ program using nested do-while loops to display the organization of products on each shelf. Each product is represented by the letter ‘X’.
Test Case
Input:
Shelves = 2
Products per shelf = 3
Output:
X X X
X X X
Explanation:
- Following the user input, there are 2 shelves, and each shelf contains 3 products.
- The outer
do-while
loop manages the shelves. Since there are 2 shelves, it runs 2 times. - For each shelf, the inner
do-while
loop handles the products. Since there are 3 products per shelf, the inner loop runs 3 times for each shelf. - Each time the inner loop runs, it prints the letter ‘X’ to represent a product. After printing the products for one shelf, the program moves to the next line to print the next shelf.
- Once all shelves and products are printed, the program ends.
#include <iostream> using namespace std; int main() { int shelves, products; // Taking input from the user for shelves and products per shelf cout << "Enter number of shelves: "; cin >> shelves; cout << "Enter number of products per shelf: "; cin >> products; // Outer do-while loop for shelves int i = 0; do { // Inner do-while loop for products on each shelf int j = 0; do { cout << "X "; // Print product 'X' j++; // Move to the next product } while (j < products); cout << endl; // Move to the next shelf i++; // Move to the next shelf } while (i < shelves); return 0; }
Movie Theater Seat Reservation
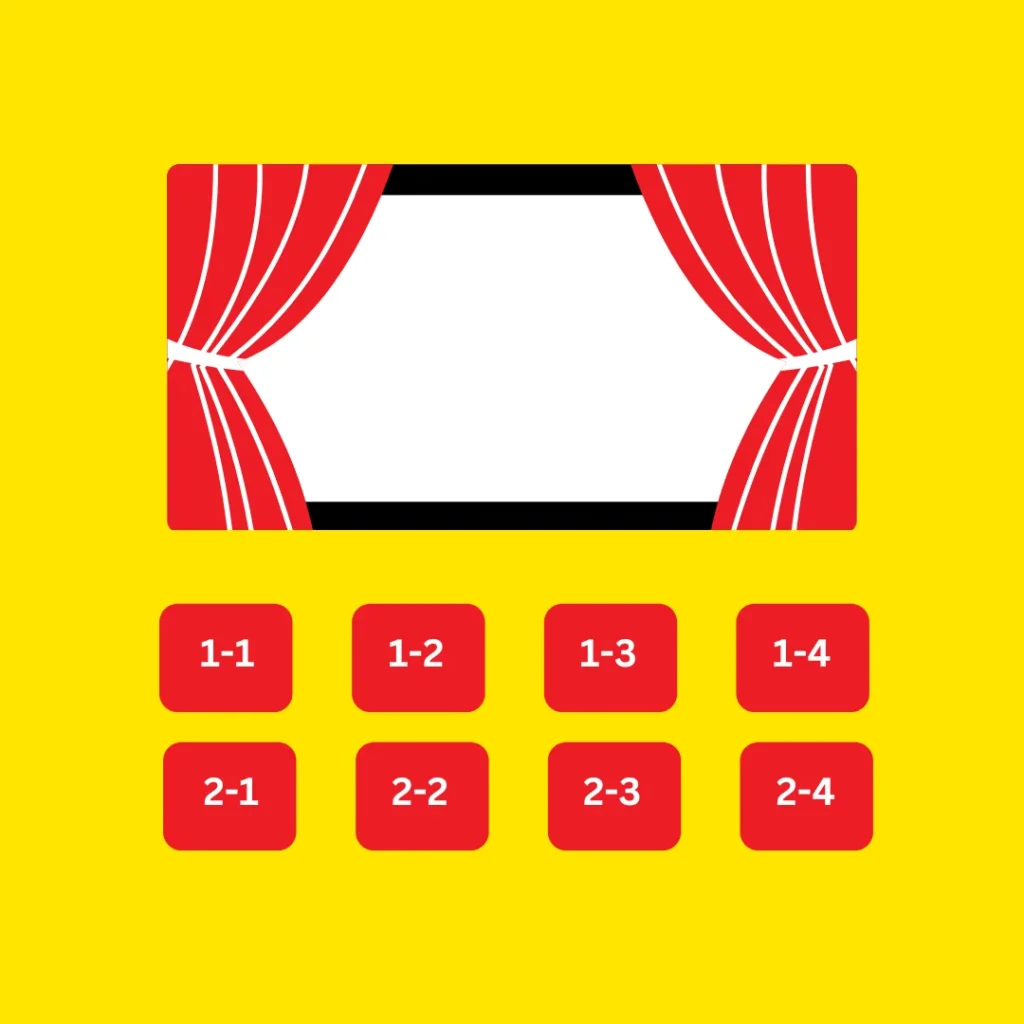
You are developing a movie theater booking system where customers can see the seat arrangement before selecting their seats. The theater has R rows and C columns, where each seat is represented by its row and seat number in the format (row-seat). Write a C++ program using nested for loops to generate a seat layout, where each seat is displayed as (row-seat).
Test Case
Input:
Enter number of rows: 3
Enter number of columns: 4
Output:
(1-1) (1-2) (1-3) (1-4)
(2-1) (2-2) (2-3) (2-4)
(3-1) (3-2) (3-3) (3-4)
Explanation:
- For this case, the theatre has 3 rows and 4 columns.
- The outer
for
loop takes care of the rows. Since there are 3 rows, it runs 3 times. - For each row, the inner
for
loop manages the columns. With 4 columns, the inner loop runs 4 times for each row. - Each time the inner loop runs, it prints the seat in the format (row-column), such as (1-1), (1-2), and so on.
- After printing all the seats for one row, the program moves to the next line and prints the next row’s seats.
- Once all rows and columns are processed, the seat layout is displayed, and the program finishes.
#include <iostream> using namespace std; int main() { int rows, columns; // Taking user input for rows and columns cout << "Enter number of rows: "; cin >> rows; cout << "Enter number of columns: "; cin >> columns; // Outer loop for rows for (int i = 1; i <= rows; i++) { // Inner loop for columns for (int j = 1; j <= columns; j++) { cout << "(" << i << "-" << j << ") "; } cout << endl; // Move to the next row } return 0; }
Traffic Light Countdown System
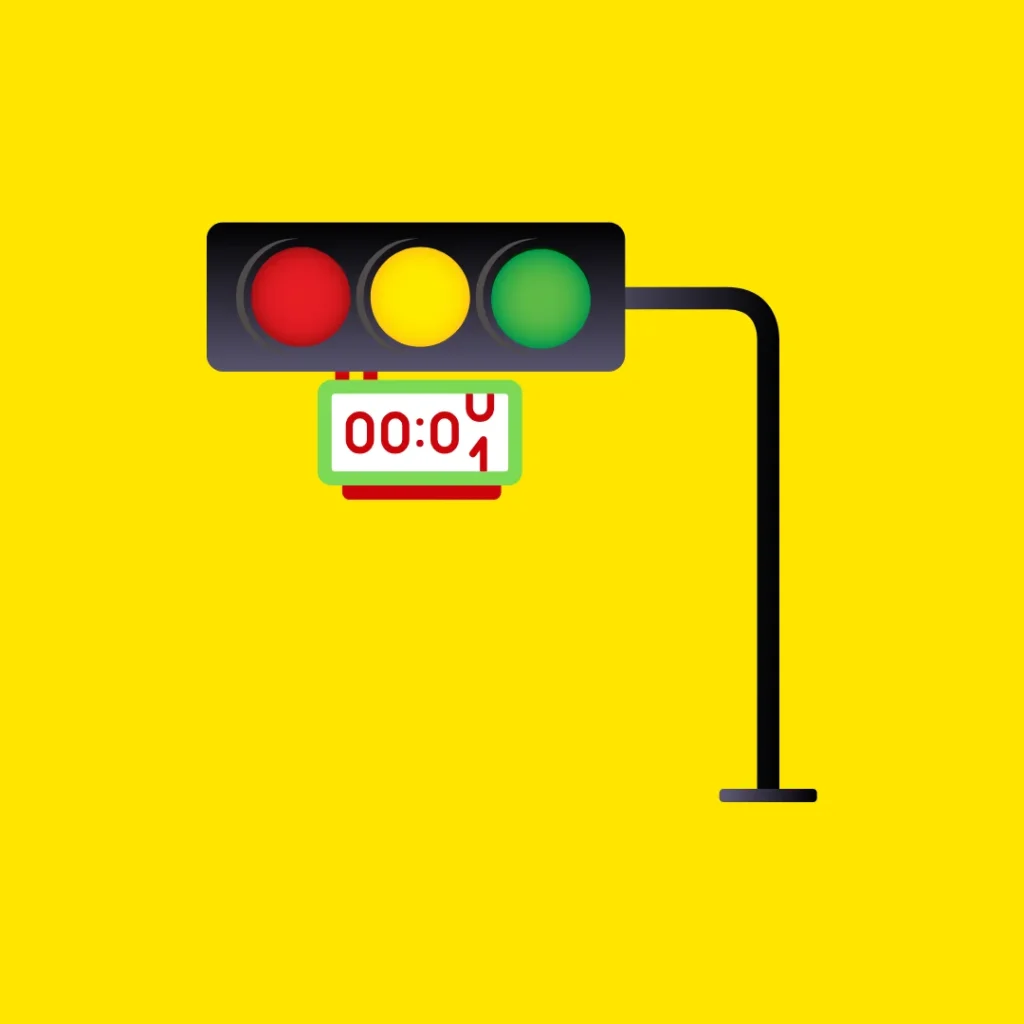
A city installs automated traffic lights at an intersection. The lights stay green for X seconds before switching to red. Write a C++ program using nested do-while loops that ask the user for the green light duration (in seconds), and then count down the remaining seconds of the green light before stopping the countdown.
Test Case
Input:
Enter green light duration (seconds): 5
Output:
Green light active!
Second 1…
Second 2…
Second 3…
Second 4…
Second 5…
Light turns red!
Explanation:
- In our case here, the green light stays on for 5 seconds before turning red.
- The outer
do-while loop
manages the countdown, starting from 1 and running until it reaches 5. - Each time the loop runs, it prints “Second X…”, where X represents the current second.
- Inside the outer loop, the inner
do-while loop
simulates a delay to represent the passing of time. This loop increments a variable until it reaches 1,000,000 (though in real systems, actual timing functions are used). - Once all 5 seconds are counted down, the outer loop stops, and the program prints “Light turns red!” to indicate the signal change.
#include <iostream> using namespace std; int main() { int duration, second = 1; // Taking user input for green light duration cout << "Enter green light duration (seconds): "; cin >> duration; cout << "Green light active!\n"; // Outer do-while loop for counting seconds do { cout << "Second " << second << "...\n"; // Inner do-while loop to simulate a small delay for each second (for example purposes) int delay = 0; do { delay++; } while (delay < 1000000); // Simulate delay second++; } while (second <= duration); // Outer loop for the full duration cout << "Light turns red!\n"; return 0; }
Countdown Number Pyramid
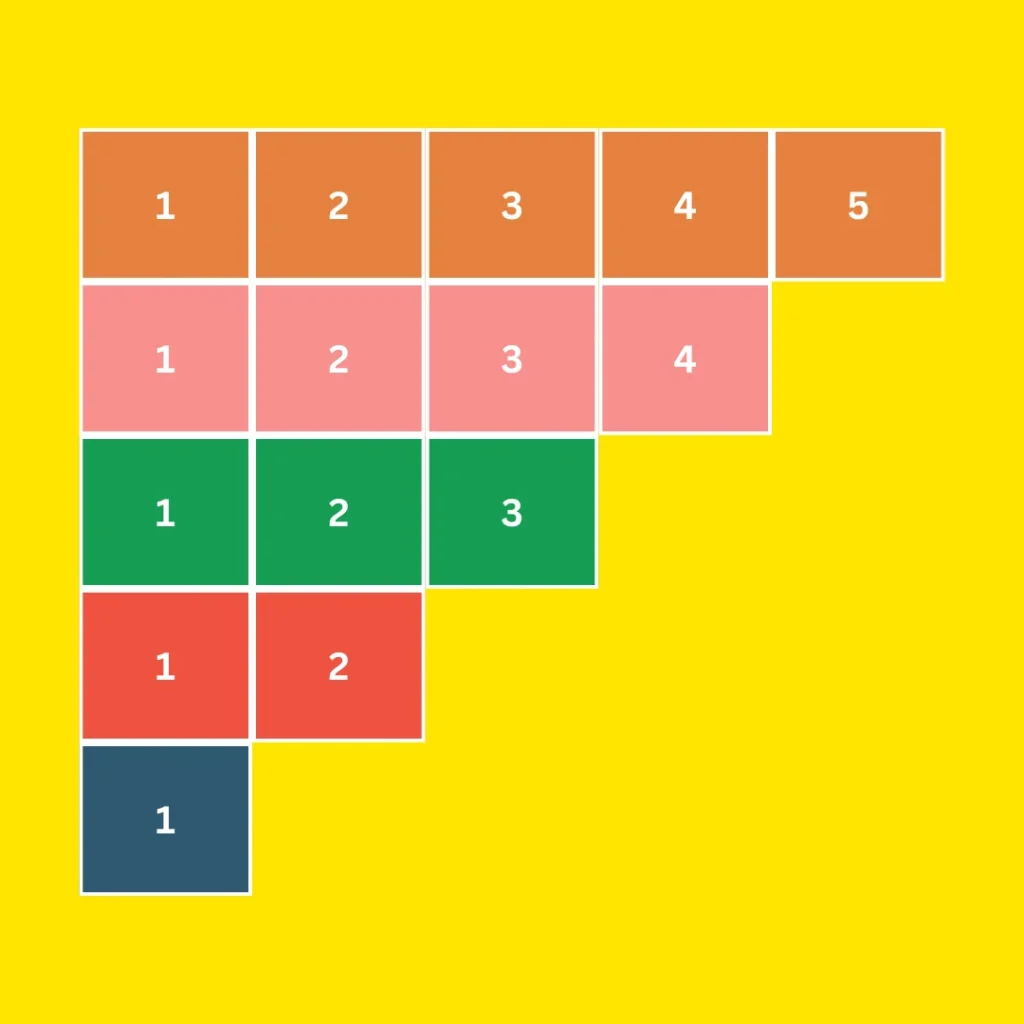
You need to show a countdown pattern. The pattern should be an inverted pyramid of numbers, starting from the row number and decreasing each step. Write a C++ program using nested while loops to display an inverted pyramid pattern where each row starts from 1 and increases to the row number.
Test Case
Input:
Enter number of rows: 5
Output:
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
Explanation:
- If we look at this case, the pyramid starts with 5 rows.
- The outer
while loop
begins with 5 and decreases by 1 after each row, controlling the number of values printed. - Inside this loop, the inner
while loop
prints numbers from 1 to the current row count. - For the first row, numbers 1 to 5 are printed. In the next row, only 1 to 4 appear, and so on.
- This process continues until the last row, which contains only 1, after which the program ends.
#include <iostream> using namespace std; int main() { int rows; // Taking user input for the number of rows cout << "Enter number of rows: "; cin >> rows; int i = rows; while (i >= 1) { // Loop runs from the largest row down to 1 int j = 1; while (j <= i) { // Print numbers from 1 to current row count cout << j << " "; j++; } cout << endl; // Move to the next row i--; } return 0; }
Automated Elevator System
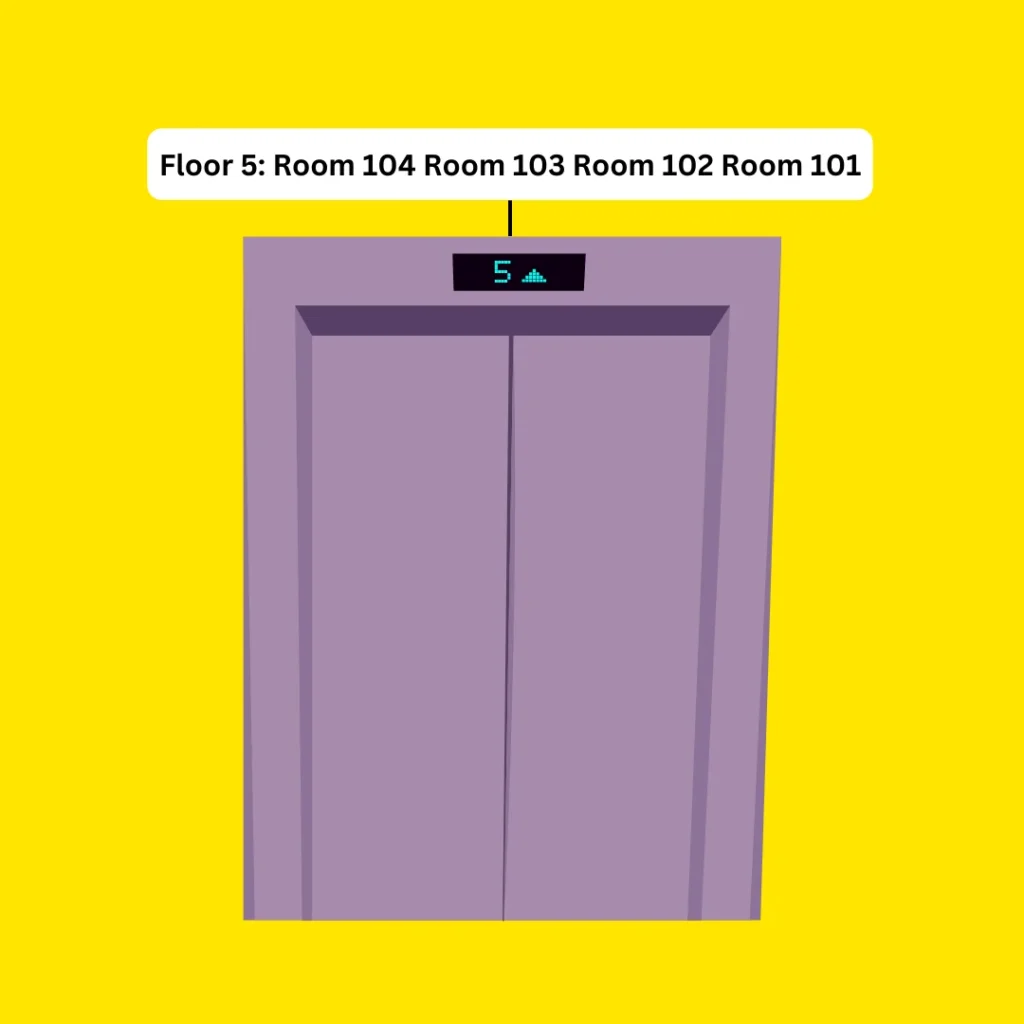
You are designing an automated elevator system for a building with ‘F’ floors and ‘R’ rooms on each floor. The elevator will stop at each floor and announce the available rooms sequentially. Write a C++ program using nested for loops to display an elevator stopping sequence, where each floor lists its rooms in reverse order (from highest to lowest).
Test Case
Input:
Enter number of floors: 3
Enter number of rooms per floor: 4
Output:
Floor 3: Room 304 Room 303 Room 302 Room 301
Floor 2: Room 204 Room 203 Room 202 Room 201
Floor 1: Room 104 Room 103 Room 102 Room 101
Explanation:
- In this case, the number of floors is 3 and rooms per floor are 4.
- The outer
for loop
begins with the top floor (3) and decreases to 1, controlling the floor sequence. - For each floor, the inner
for loop
prints the room numbers in reverse, from Room 4 to Room 1. - On Floor 3, the output is Room 304 Room 303 Room 302 Room 301. The same pattern follows for Floor 2 and Floor 1.
- Once all floors and rooms are displayed, the program ends.
#include <iostream> using namespace std; int main() { int floors, rooms; // Taking user input for floors and rooms cout << "Enter number of floors: "; cin >> floors; cout << "Enter number of rooms per floor: "; cin >> rooms; // Outer loop for floors (reverse order) for (int i = floors; i >= 1; i--) { cout << "Floor " << i << ": "; // Inner loop for rooms (reverse order) for (int j = rooms; j >= 1; j--) { cout << "Room " << i << j << " "; } cout << endl; } return 0; }
School Bell Ringing Schedule
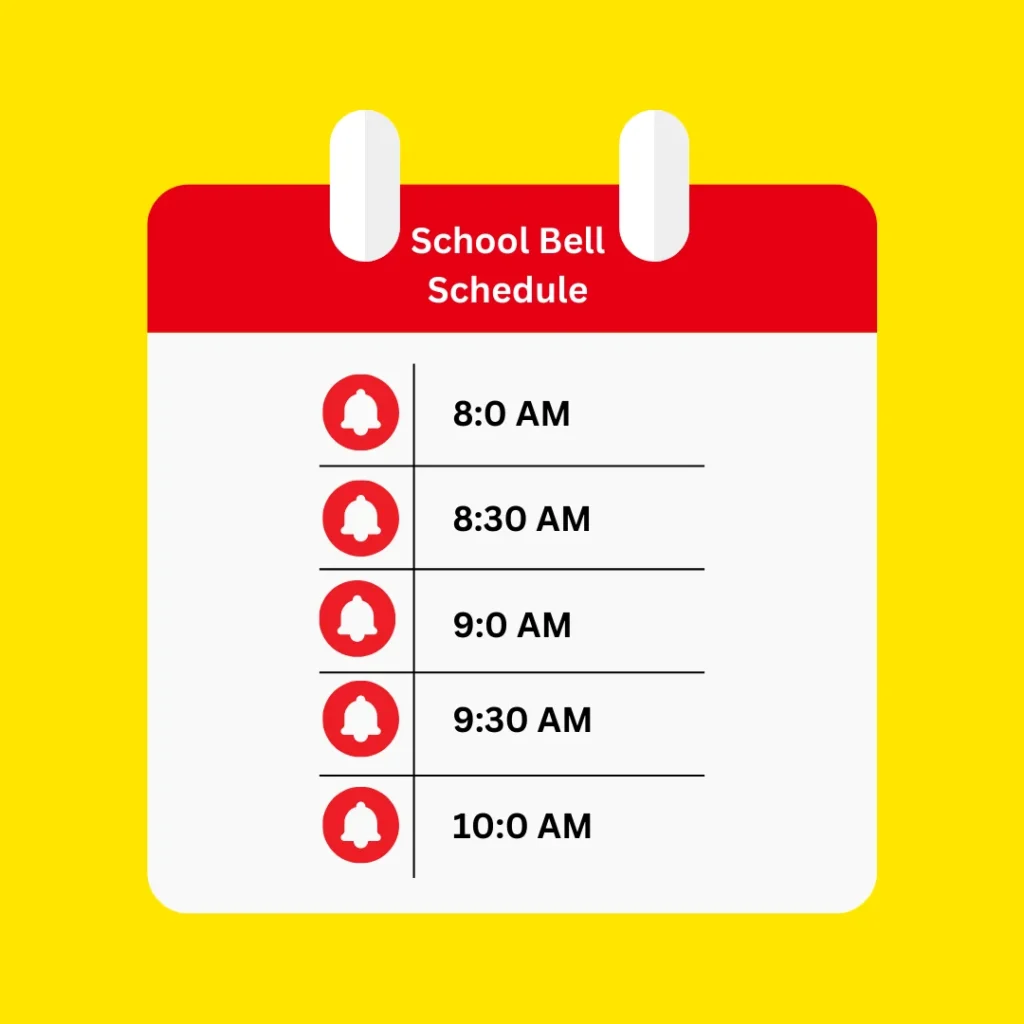
You are tasked with designing a school bell scheduling system. The bell rings every ‘I’ minutes starting at 8 AM, and the school day lasts for ‘H’ hours. Write a C++ program using nested do-while loops to display the bell ringing times in the format hour:minute AM, with no leading zeroes in minutes.
Test Case
Input:
Enter bell interval in minutes: 15
Enter total school hours: 3
Output:
8:0 AM
8:15 AM
8:30 AM
8:45 AM
9:0 AM
9:15 AM
9:30 AM
9:45 AM
10:0 AM
10:15 AM
10:30 AM
10:45 AM
School day over!
Explanation:
- The test case begins with a 15-minute interval and a 3-hour school duration as input.
- The outer
do-while loop
runs through each hour, ensuring the bell rings throughout the school day. Inside it, the innerdo-while loop
starts from 0 minutes and increases by 15 until the hour is up. - The output begins with 8:00 AM, then 8:15 AM, 8:30 AM, and so on. When the minutes reach 60, the hour increments, moving to 9:00 AM and continuing the pattern.
- This process repeats until the final bell at 10:45 AM, after which the program announces “School day over!” and stops.
#include <iostream> using namespace std; int main() { int interval, hours; int time = 0, currentHour = 8; // Start at 8 AM // Taking user input cout << "Enter bell interval in minutes: "; cin >> interval; cout << "Enter total school hours: "; cin >> hours; cout << "Bell Ringing Schedule:\n"; do { int minutes = 0; do { cout << currentHour << ":"; // Print minutes with leading zero without using if-else cout << (minutes / 10) * 10 + (minutes % 10) << " AM\n"; minutes += interval; time += interval; } while (minutes < 60); currentHour += 1; } while (time < hours * 60); cout << "School day over!\n"; return 0; }
Café Order Receipt Generator
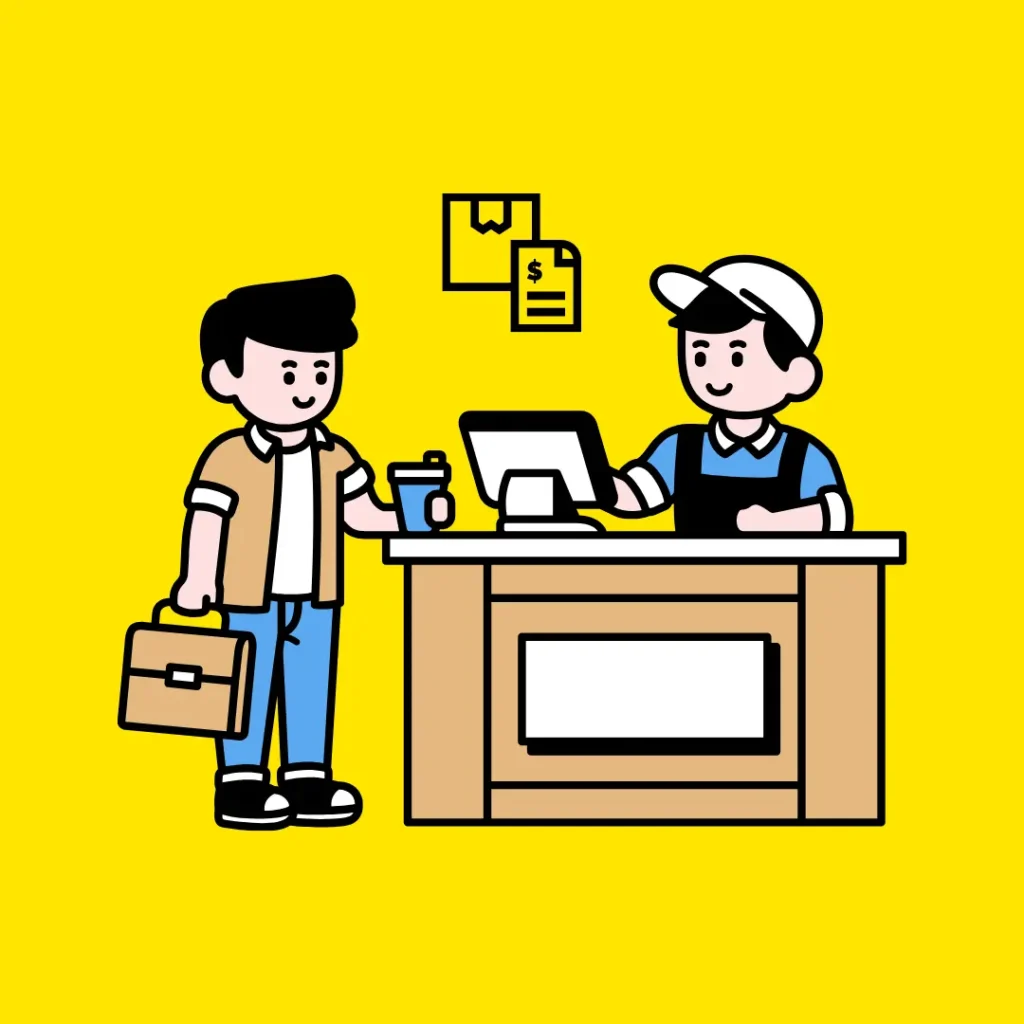
You are developing a billing system for a café. Customers can order multiple cups of coffee, and the receipt must display each cup no. and its total price. The café sells coffee at $5 per cup. Write a C++ program that takes the number of customers and the cups of coffee each customer orders as input, then prints a receipt using nested for loops.
Test Case
Input:
Enter the number of customers: 2
Enter the number of cups for customer 1: 2
Enter the number of cups for customer 2: 4
Output:
Customer 1:
Cup 1 – $5
Cup 2 – $5
Total: $10
Customer 2:
Cup 1 – $5
Cup 2 – $5
Cup 3 – $5
Cup 4 – $5
Total: $20
Explanation:
- In this case, there are 2 customers. The first customer orders 2 cups, while the second orders 4 cups.
- The outer
for loop
runs once per customer, prompting for the number of cups they order. Inside this loop, the innerfor loop
iterates based on the cup count, printing the cup number along with its price. - Each cup is priced at $5, so for Customer 1, the program prints Cup 1 – $5 and Cup 2 – $5, followed by the total price of $10.
- For Customer 2, the process repeats, displaying 4 cups and a total price of $20. Once all customer orders are processed, the program ends.
#include <iostream> using namespace std; int main() { int customers, cups; const int price = 5; // Price per cup cout << "Enter the number of customers: "; cin >> customers; //outer loop for no. of cups a customer ordered for (int i = 1; i <= customers; i++) { cout << "Enter the number of cups for customer " << i << ": "; cin >> cups; int total = 0; cout << "Customer " << i << ":" << endl; //customer no. //inner loop for calculating the price of cups for (int j = 1; j <= cups; j++) { cout << " Cup " << j << " - $" << price << endl; total += price; } cout << " Total: $" << total << "\n" << endl; } return 0; }
Library Late Fee Calculator
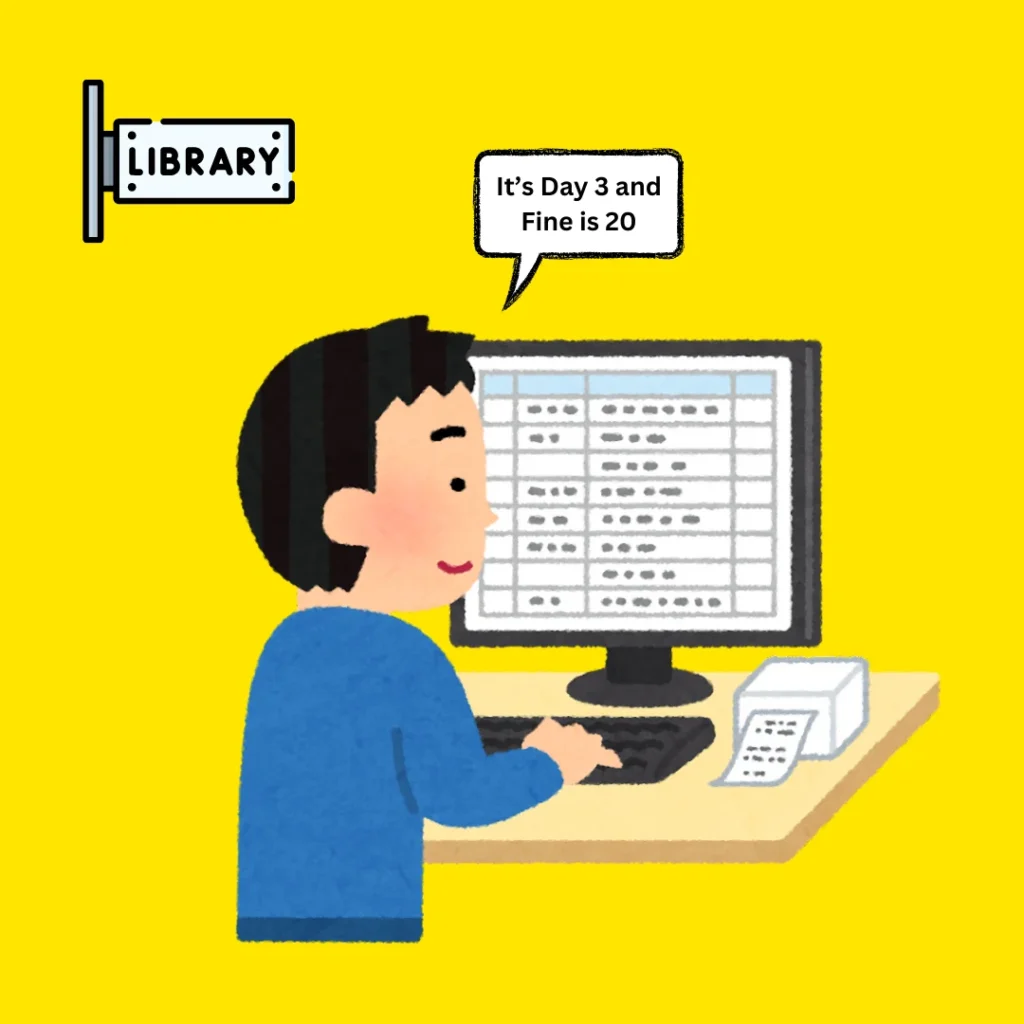
You are developing a library late fee system where a fine increases daily for overdue books. The fine starts at Rs. 5 on day 1 and doubles each day. The library sets a maximum fine of Rs. 1000 per book. Write a C++ program using nested while loops that asks the user for the number of books returned late, and calculates and displays the fine for each book day by day until the maximum fine is reached.
Test Case
Input:
Enter number of books returned late: 1
Output:
Book 1:
Day 1 – Rs. 5
Day 2 – Rs. 10
Day 3 – Rs. 20
Day 4 – Rs. 40
Day 5 – Rs. 80
Day 6 – Rs. 160
Day 7 – Rs. 320
Day 8 – Rs. 640
Max fine reached: Rs. 1000
Explanation:
- The no. of books is 1 in this case.
- The outer
while loop
runs for each book in this code, ensuring every overdue book is processed individually. - The inner
while loop
handles the daily fine calculation. - For Book 1, the fine increases from Rs. 5 to Rs. 640 on Day 8.
- Since the next fine would exceed Rs. 1000, the program stops and displays “Max fine reached: Rs. 1000” before moving to the next book.
#include <iostream> using namespace std; int main() { int books; // Taking user input for number of late books cout << "Enter number of books returned late: "; cin >> books; int book = 1; while (book <= books) { cout << "Book " << book << ":\n"; int day = 1; int fine = 5; // Initial fine while (fine <= 1000) { // Stops at Rs. 1000 cout << "Day " << day << " - Rs. " << fine << endl; fine *= 2; // Fine doubles each day day++; } cout << "Max fine reached: Rs. 1000\n\n"; book++; } return 0; }
Bakery Order System
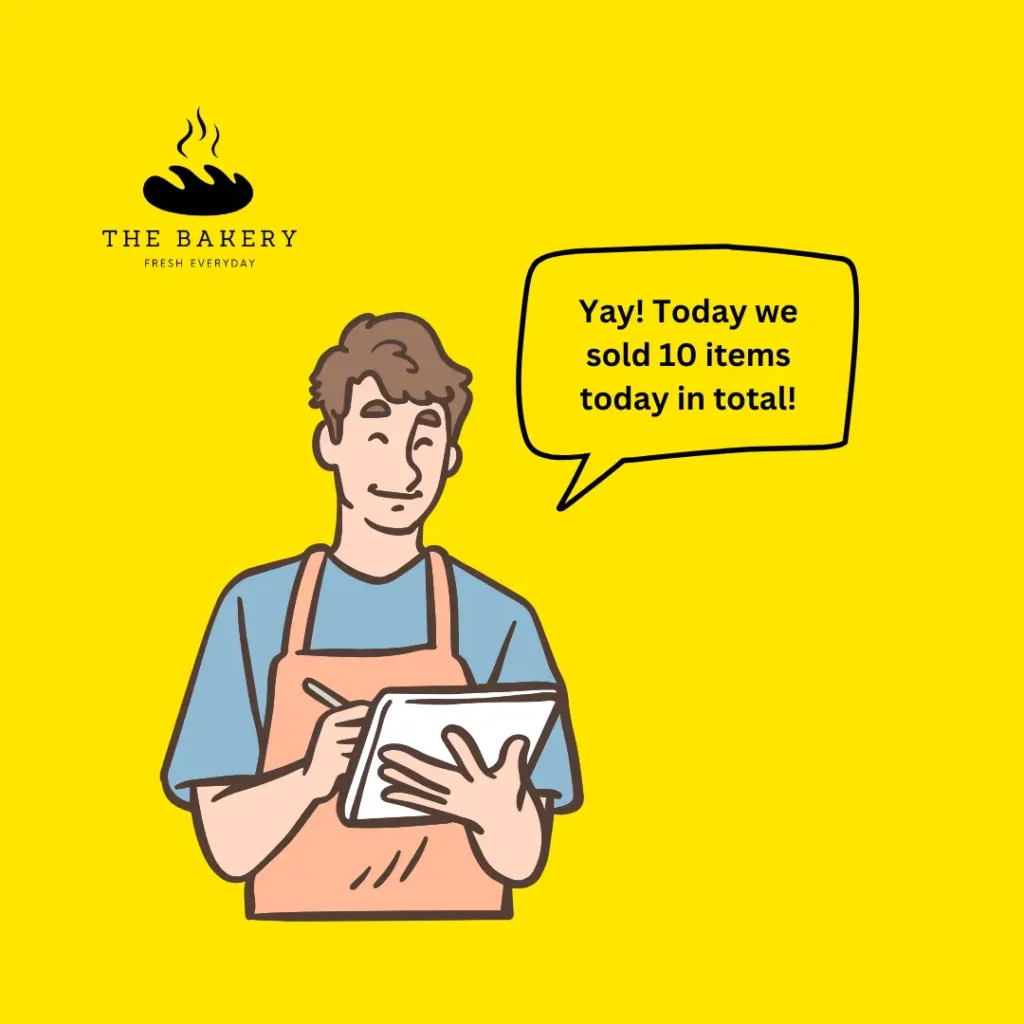
You’re working at a bakery and processing multiple customer orders. Each customer orders multiple items, and you have to compute the total number of items ordered by all customers at the end of the day. Write a C++ program using nested while loops to process the orders and calculate the total no. of items ordered by all customers.
Test Case
Input:
Enter the number of customers: 2
Enter the items for customer 1: 3
Enter the items for customer 2: 5
Output:
Total number of items ordered: 8
Explanation:
- Here, the number of customers is 2.
- The outer
while
loop runs for each customer, ensuring every customer’s order is processed individually. - Inside the outer loop, the program asks how many items each customer ordered.
- For Customer 1, he ordered 3 items, so the inner
while
loop runs 3 times, increasing thetotalItems
count by 1 each time. - For Customer 2, he ordered 5 items, so the inner
while
loop runs 5 times, and thetotalItems
count increases by 1 for each item. - Once all customers are processed, the program displays the total number of items ordered, which is 8 in this case.
#include <iostream> using namespace std; int main() { int customers, totalItems = 0; cout << "Enter the number of customers: "; cin >> customers; int i = 1; //outer loop asking how many items a customer bought while (i <= customers) { int items; cout << "Enter the items for customer " << i << ": "; cin >> items; // inner loop to accumulate items for the current customer int j = 1; while (j <= items) { totalItems++; // Increase the total item count j++; // Move to the next item for this customer } i++; // Move to the next customer } cout << "Total number of items ordered: " << totalItems << endl; return 0; }
Alien Language Translator
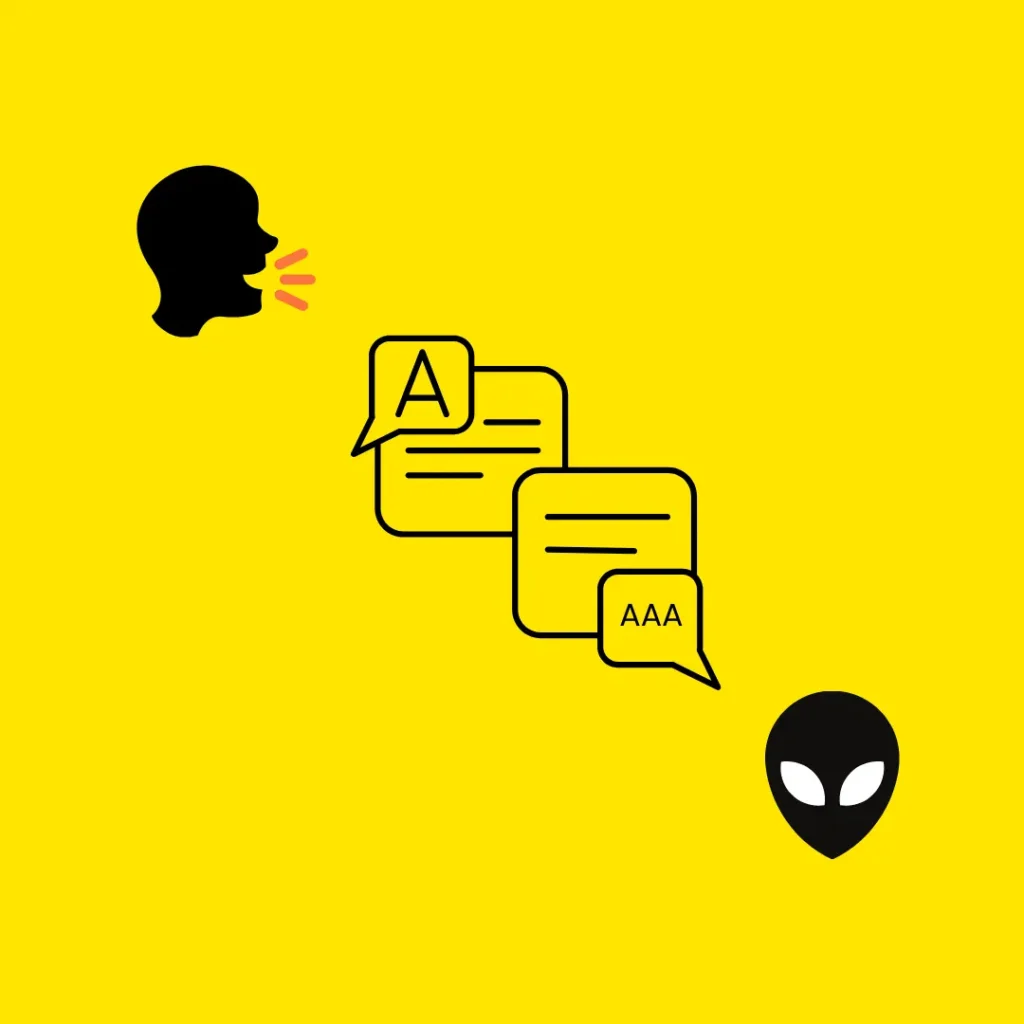
You are working for an intergalactic space agency that is developing a translator for an alien species. The alien language consists of repeating letters in a unique pattern. You must translate any word entered by a human by repeating each letter in a structured grid format. Write a C++ program that prints the alien version of the word using nested for loops.
Test Case
Input:
Enter a word: WOW
Enter repetition count: 3
Output:
WWW OOO WWW
WWW OOO WWW
WWW OOO WWW
Explanation:
- Here, the user inputs the word “WOW” with a repetition count of 3.
- The outer
for
loop runs 3 times, once for each row to be printed. For each iteration of the outer loop, the program loops through each character in the word. - Inside the inner
for
loop, each character is repeated 3 times, based on the repetition count. So, for ‘W’, ‘O’, and ‘W’, it prints “WWW”, “OOO”, and “WWW” respectively. - Once all characters are printed in a row, the program moves to the next row and repeats the process. After 3 rows are printed, the program ends.
#include <iostream> using namespace std; int main() { string word; int repeat; cout << "Enter a word: "; //Word entered for repitition cin >> word; cout << "Enter repetition count: "; //No. of repitition cin >> repeat; // Outer loop to repeat the entire word 'repeat' number of times for (int i = 0; i < repeat; i++) { // Loop through each letter in the word for (char letter : word) { // Inner loop to repeat the current letter 'repeat' times for (int j = 0; j < repeat; j++) { cout << letter; } cout << " "; } cout << endl; } return 0; }
The Secret Vault Password Generator
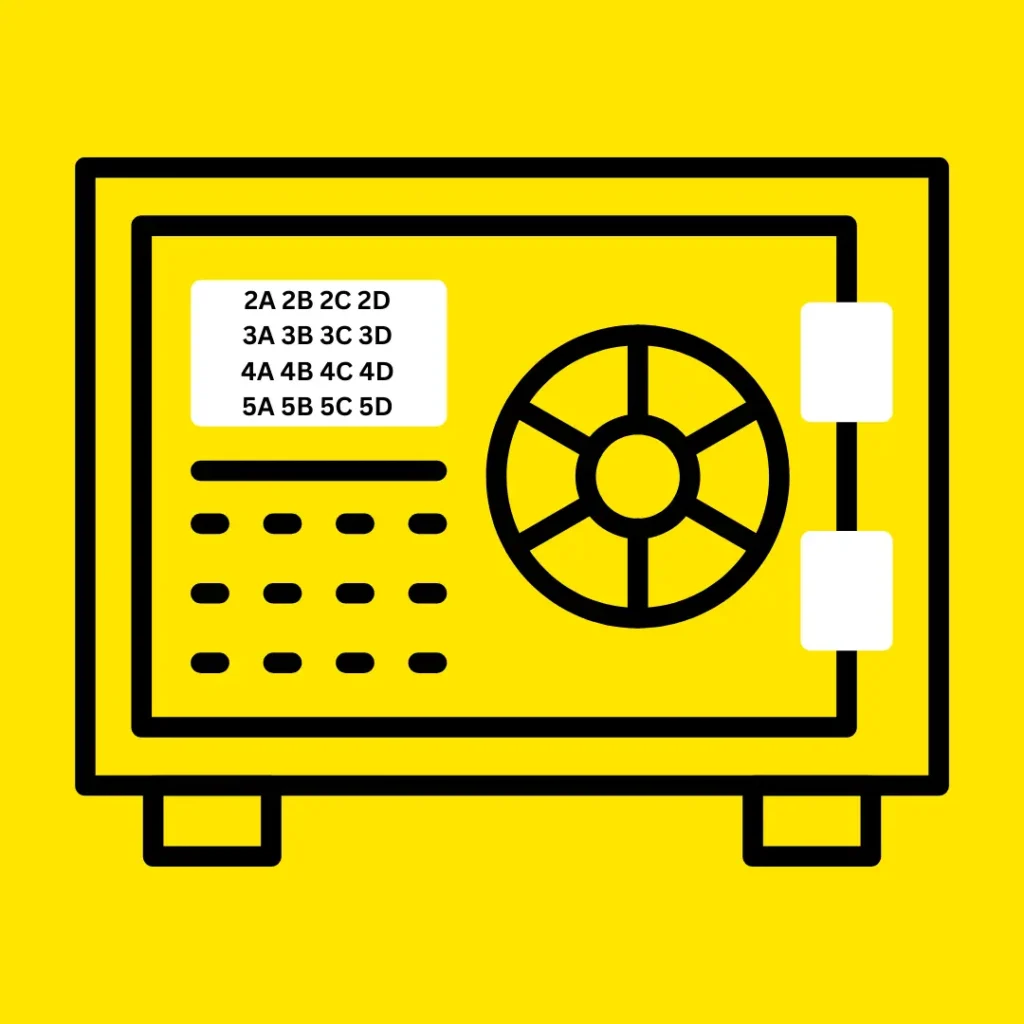
A top-secret underground vault can only be unlocked with a unique password pattern. The password is a combination of numbers and letters and follows a strict format. The vault system generates passwords based on two user inputs: a number ‘N’ and a letter ‘L’. The system then creates multiple passwords, each consisting of a number followed by a letter. Write a C++ program in nested while loops that generates and displays all possible vault passwords based on user input.
Test Case
Input:
Enter a starting number: 2
Enter the last letter (A-Z): D
Output:
2A 2B 2C 2D
3A 3B 3C 3D
4A 4B 4C 4D
5A 5B 5C 5D
Explanation:
- The user inputs a starting number 2 and the last letter D in this case.
- The outer
while
loop runs for 4 iterations, starting from the given number 2 up to 5. - For each iteration, the inner
while
loop starts with the letter A and iterates up to the user-defined letter D. It generates combinations like 2A, 2B, 2C, 2D for the first iteration, then moves on to 3A, 3B, 3C, 3D, and so on. - After the program has printed all the rows, it stops.
#include <iostream> using namespace std; int main() { int startNum; char endLetter; cout << "Enter a starting number: "; cin >> startNum; cout << "Enter the last letter (A-Z): "; cin >> endLetter; int i = startNum; // Outer loop: Generates 4 rows of numbers, starting from the given number while (i < startNum + 4) { // 4 rows of numbers char ch = 'A'; // Inner loop: Iterates through letters from 'A' to the user-defined endLetter while (ch <= endLetter) { // Loop through letters cout << i << ch << " "; ch++; } cout << endl; i++; } return 0; }
Supermarket Checkout System
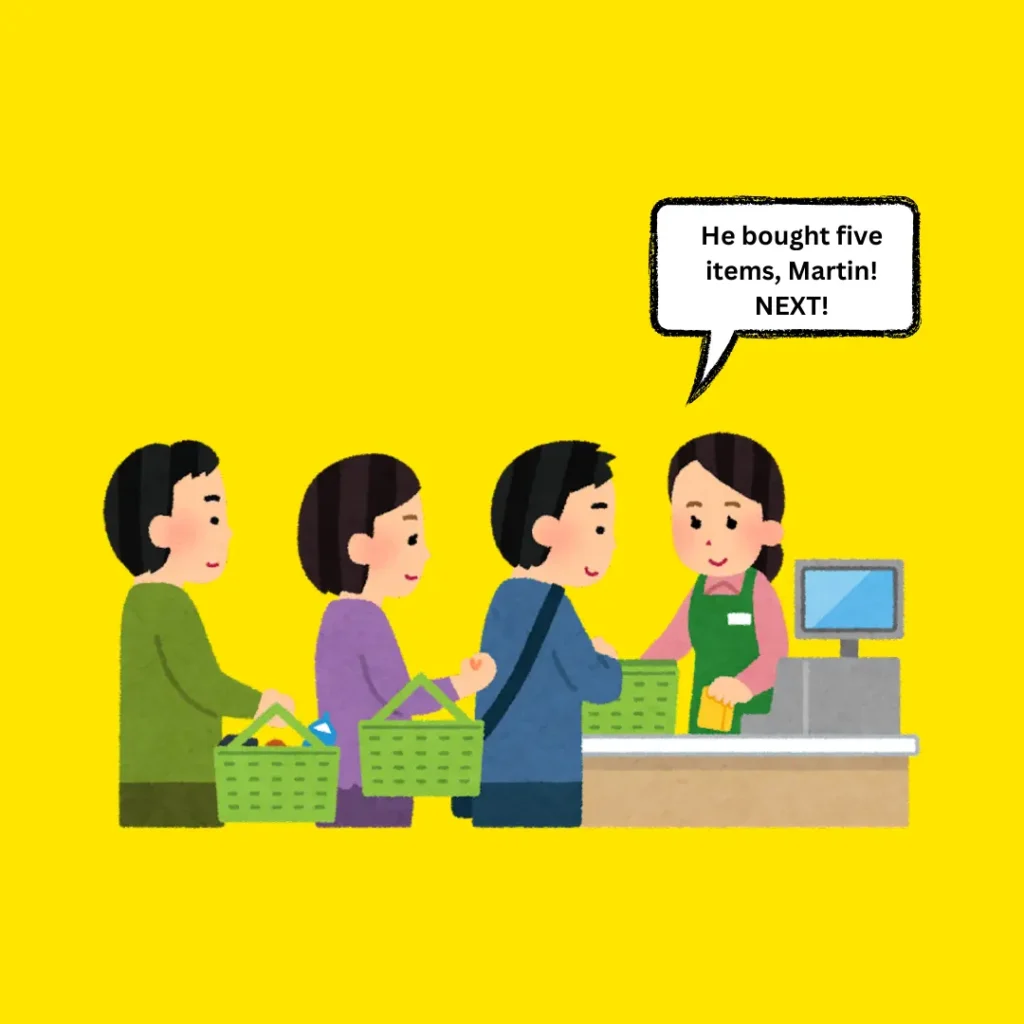
You are building a supermarket checkout system. Customers can add different types of products to their cart, and each product has a specific quantity. The system needs to print out the total quantity of products purchased by each customer. Write a C++ program using nested for loops that asks for the number of customers, and for each customer, asks how many types of products they bought and the quantity for each product. The program should print the total quantity of products each customer bought.
Test Case
Input:
Enter the number of customers: 1
Enter the number of products for customer 1: 2
Enter quantity for product 1: 2
Enter quantity for product 2: 3
Output:
Customer 1 purchased 5 items.
Explanation:
- Here, there is 1 customer, and he bought 2 products.
- The outer
for
loop takes care of the customers. Since there is only 1 customer, it runs once. - For this customer, the inner
for
loop handles the products. With 2 products, the inner loop runs 2 times. - Each time the inner loop runs, it asks for the quantity of each product and adds it to the total quantity for that customer.
- Once all products are processed, the program displays the total number of items purchased by the customer, and the program finishes.
#include <iostream> using namespace std; int main() { int customers; cout << "Enter the number of customers: "; cin >> customers; // Outer loop for customers for (int i = 1; i <= customers; i++) { int products; cout << "Enter the number of products for customer " << i << ": "; cin >> products; int totalQuantity = 0; // Inner loop for products for (int j = 1; j <= products; j++) { int quantity; cout << "Enter quantity for product " << j << ": "; cin >> quantity; totalQuantity += quantity; } cout << "Customer " << i << " purchased " << totalQuantity << " items." << endl; } return 0; }
If you enjoyed solving these scenario-based questions on nested loops in C++, feel free to explore more challenges on C++ programming and continue learning to master the language!