In this article, you will learn nested if-else in C++, along with real-world examples, engaging visuals, and easy code snippets on real-world scenarios.
Table of Contents
Conditional Statements in C++ ( if-else )
Conditional statements are also called decision statements. They allow specific actions to be taken based on whether certain conditions are met. This means the program can make decisions and respond differently in various situations.
if-else
statements in C++ are a type of conditional statement, they allow the program to make the decision based on the condition, if
conditions runs a specified block of code when the condition is true, and the else
condition runs when the if
condition is found to be false.
What are Nested Conditions?
Nested conditions mean when you use a conditional statement inside another conditional statement, just like using an if-else
condition as the specified block of code of another if-else
statement. This allows checking multiple conditions that can be true or false in a sequence.
Real Life Example of Nested Conditions
You walk into a coffee shop, ready to order your drink. First, you decide on the flavor, and after looking at the menu, you pick a vanilla latte. Then, you think about whether you want it hot or cold. Since it’s cold outside, you choose a hot one. Lastly, you consider the size: a small would be enough, but you’re in the mood for more, so you go for a large hot vanilla latte. As you wait, you realize that each choice—flavor, temperature, and size—is like using nested if-else conditions in programming, where each decision leads to the next.
- Choose a coffee flavor
if ( coffee == vanilla latte )
- Choose hot or cold
if ( coffee temperature)
- Choose size
if ( coffee size )
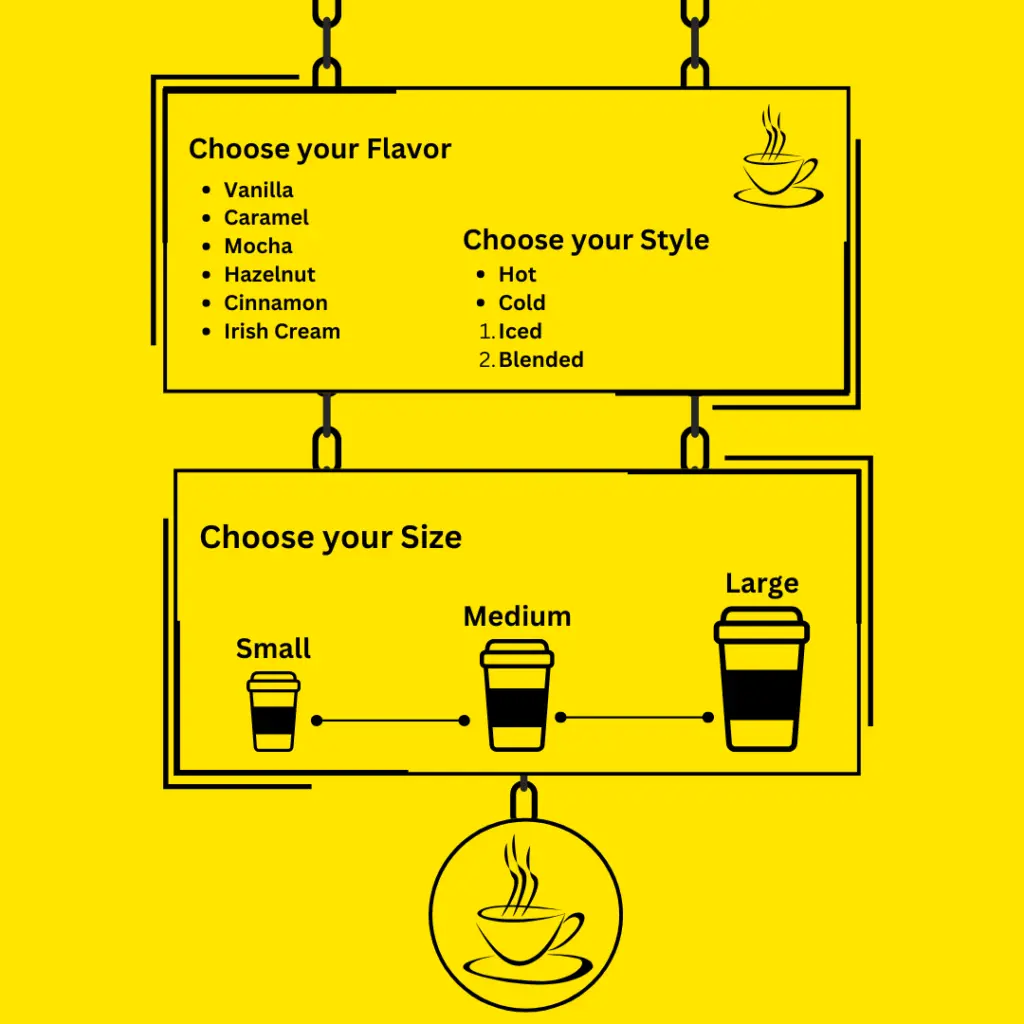
Nested If Statement
Nested if
statement can handle multiple layers of decision-making in your code. They work by placing one if
statement inside another. First, the program checks an initial condition with an if
statement. If this condition is true, it then proceeds to check another condition inside the first if
statement. In other words, you place an if
statement inside the block of another if
statement. By using nested if
statements, you can manage complex scenarios where each decision depends on the outcome of the previous one.
Real-World Analogy of Nested If Condition – Catching a Bus
A simple example is when you’re about to catch a bus to your destination. First, you check if the bus goes to that location. If it does, you then check if you have enough balance on your card to board the bus.
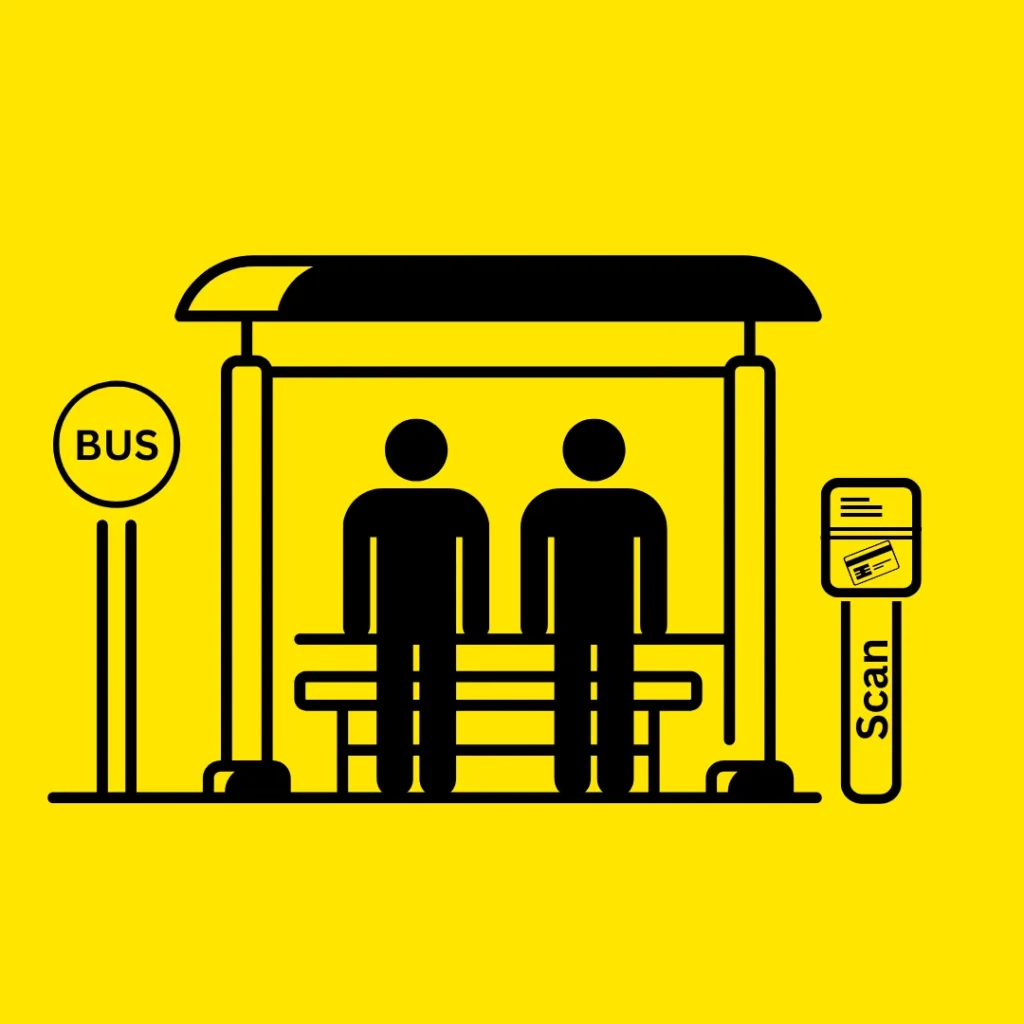
Flow Chart of Nested If
The program first evaluates the initial if
statement. If it is true, the program then checks the nested if
statement. If the nested if
is also true, it executes the corresponding statements. If the initial if
is false, the program skips to the else
block and executes its statements before ending.
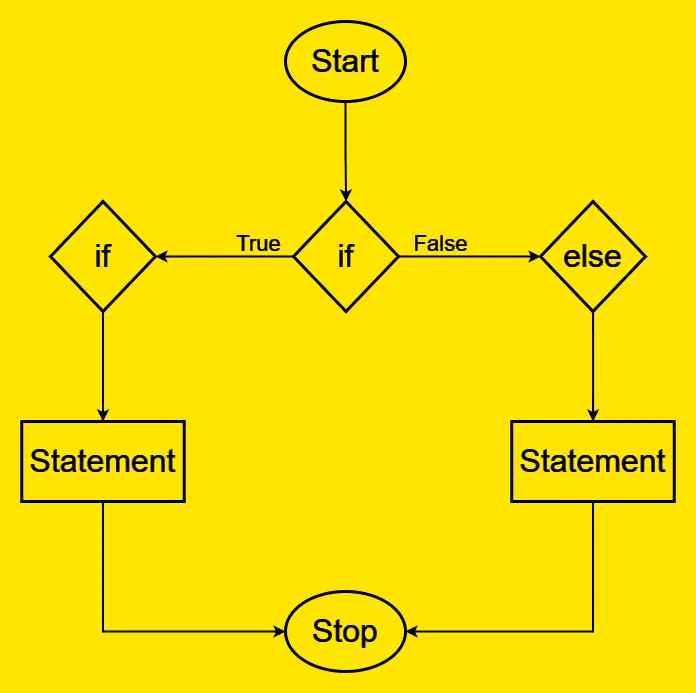
Syntax
if (condition1) { // Code to execute if condition1 is true if (condition2) { // Code to execute if condition2 is also true } }
Example Code
- The program asks the user to enter a number.
- It first checks if the number is positive (greater than 0).
If
the number is positive, it prints"The number is positive."
- Then, it checks if the number is even (
divisible by 2
).- If the number is even, it prints
"The number is even."
- If the number is even, it prints
- If the number is not positive, the program doesn’t check if it’s even and ends without printing anything.
This step-by-step process checks two conditions: first, whether the number is positive, then if it’s even, but only if the first condition is true.
#include <iostream> using namespace std; int main() { int number; cout << "Enter a number: "; cin >> number; if (number > 0) { // First condition checks if the number is positive cout << "The number is positive." << endl; if (number % 2 == 0) { // Second condition checks if the number is even cout << "The number is even." << endl; } } return 0; }
Output
Enter a number: 6 The number is positive. The number is even.
Nested if-else Statement
Nested if-else
statements are a way to make more detailed decisions in your program. Before this, you were checking only if the conditions were true now using nested if-else
conditional statements you can check multiple ones with options for both true and false outcomes. This helps when you need to handle different possibilities based on the results of previous checks, making your code more flexible.
Real-World Analogy of Nested If-else – Online Education
Imagine you want to enroll in an online course. The program will guide you through three steps: first, you select a course category like Technology, Arts, or Business. If your choice is valid, you then further pick a level such as Beginner, Intermediate, or Advanced. Finally, you choose whether you want Live Classes or Recorded Sessions. The program will then confirm your enrollment based on these choices.
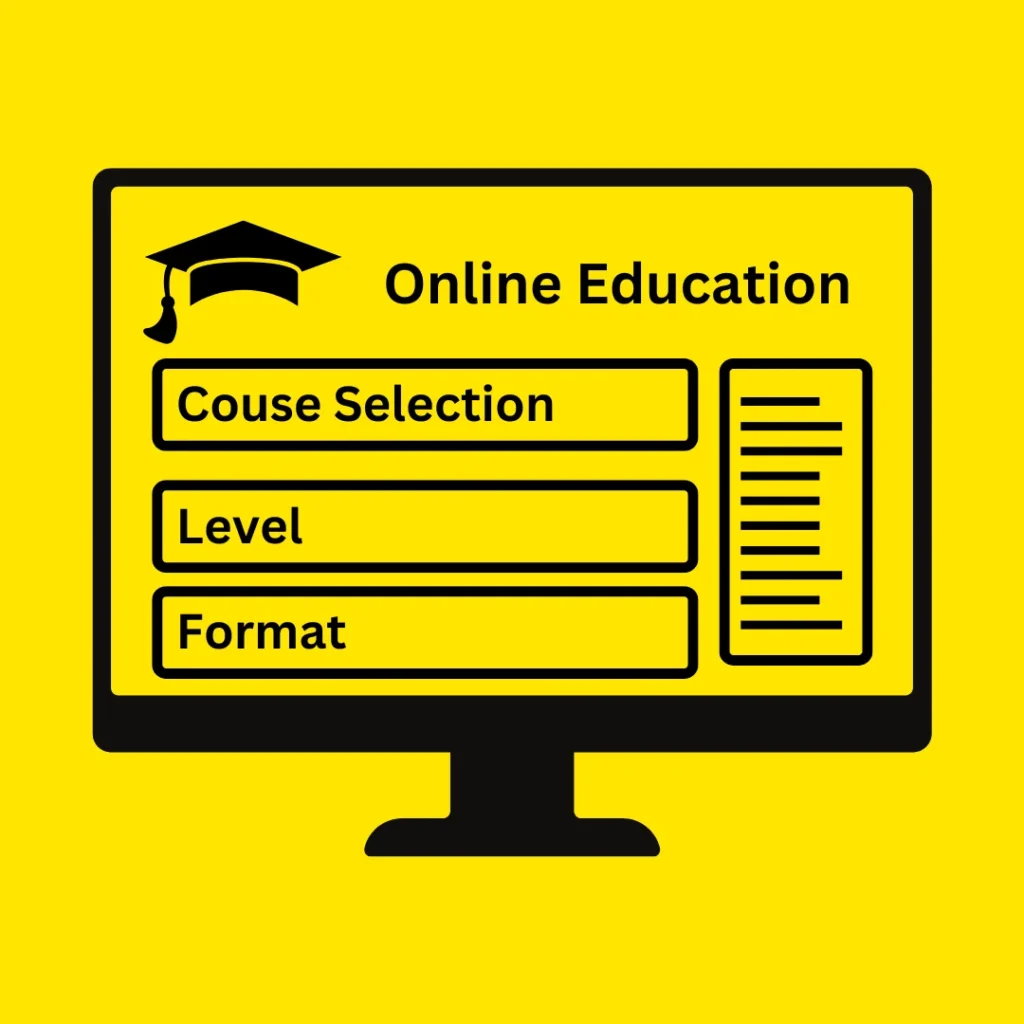
Flow Chart of Nested If-else
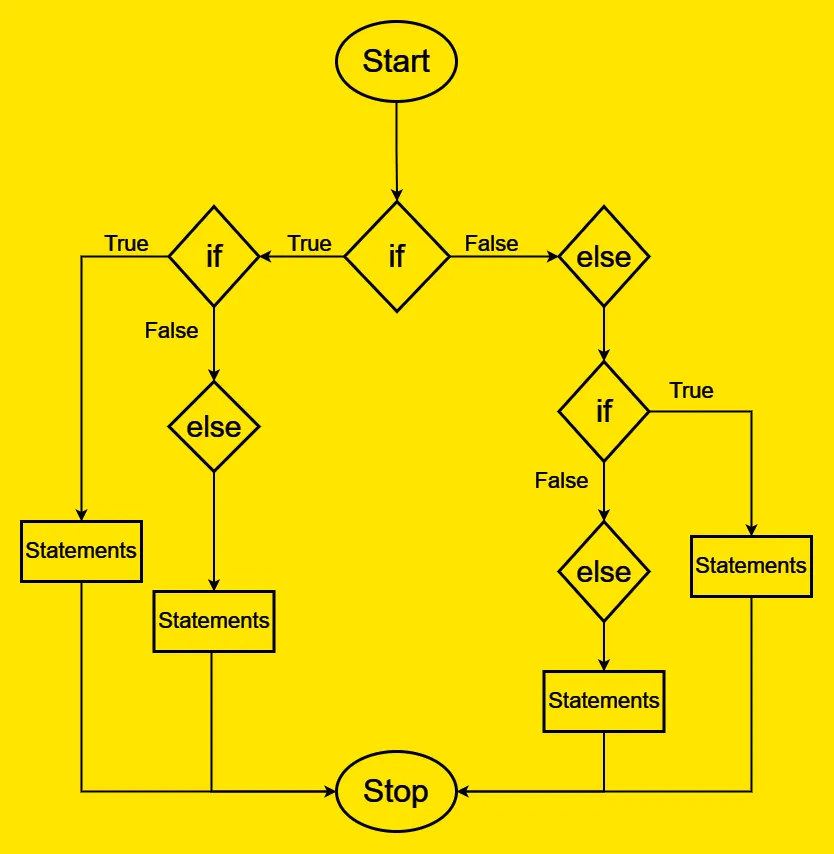
Syntax
if (condition1) { // Code for condition1 being true if (condition2) { // Code for condition2 being true inside condition1 } else { // Code for condition2 being false inside condition1 } } else { // Code for condition1 being false if (condition3) { // Code for condition3 being true inside condition1's else } else { // Code for condition3 being false inside condition1's else } }
Example Code
Below is a nested if-else statement in C++ example program. The program asks the user to enter their age using cin.
It first checks if
the age is between 13 and 19, to see if the person is a teenager.
If
the person is a teenager, it then checksif
they are 16 or older to determine if they are eligible to apply for a driver’s license.If
the person is younger than 16, it outputs that they are not yet eligible to apply for a license.
If the person is not a teenager, the program continues with further checks:
if
the age is between 20 and 64, it identifies the person as an adult.if
the age is 65 or older, the program recognizes the person as a senior citizen.
if
the age is below 13, it concludes that the person is a child.
#include <iostream> using namespace std; int main() { int age; cout << "Enter your age: "; cin >> age; if (age >= 13 && age <= 19) { // First check: is the person a teenager (13-19)? cout << "You are a teenager." << endl; if (age >= 16) { // Check if the teenager is 16 or older cout << "You are eligible to apply for a driver's license." << endl; } else { // If the teenager is younger than 16 cout << "You are not yet eligible to apply for a driver's license." << endl; } } else { // Second check: not a teenager cout << "You are not a teenager." << endl; if (age >= 20 && age <= 64) { // Check if the person is an adult cout << "You are an adult." << endl; } else if (age >= 65) { // Check if the person is a senior cout << "You are a senior citizen." << endl; } else { // If the person is a child (below 13) cout << "You are a child." << endl; } } return 0; }
Output
Enter your age: 18 You are a teenager. You are eligible to apply for a driver's license.
Real-World Example of Nested if-else Statement
You’re building a program to calculate the cost of gym memberships with some additional options. The gym offers three membership types: basic ($20/month), standard ($30/month), and premium ($50/month). The user will also select a training type (strength/cardio/yoga) and indicate whether they need a personal trainer (yes/no). These extra options won’t affect the cost, but they will be stored as part of the selection process.
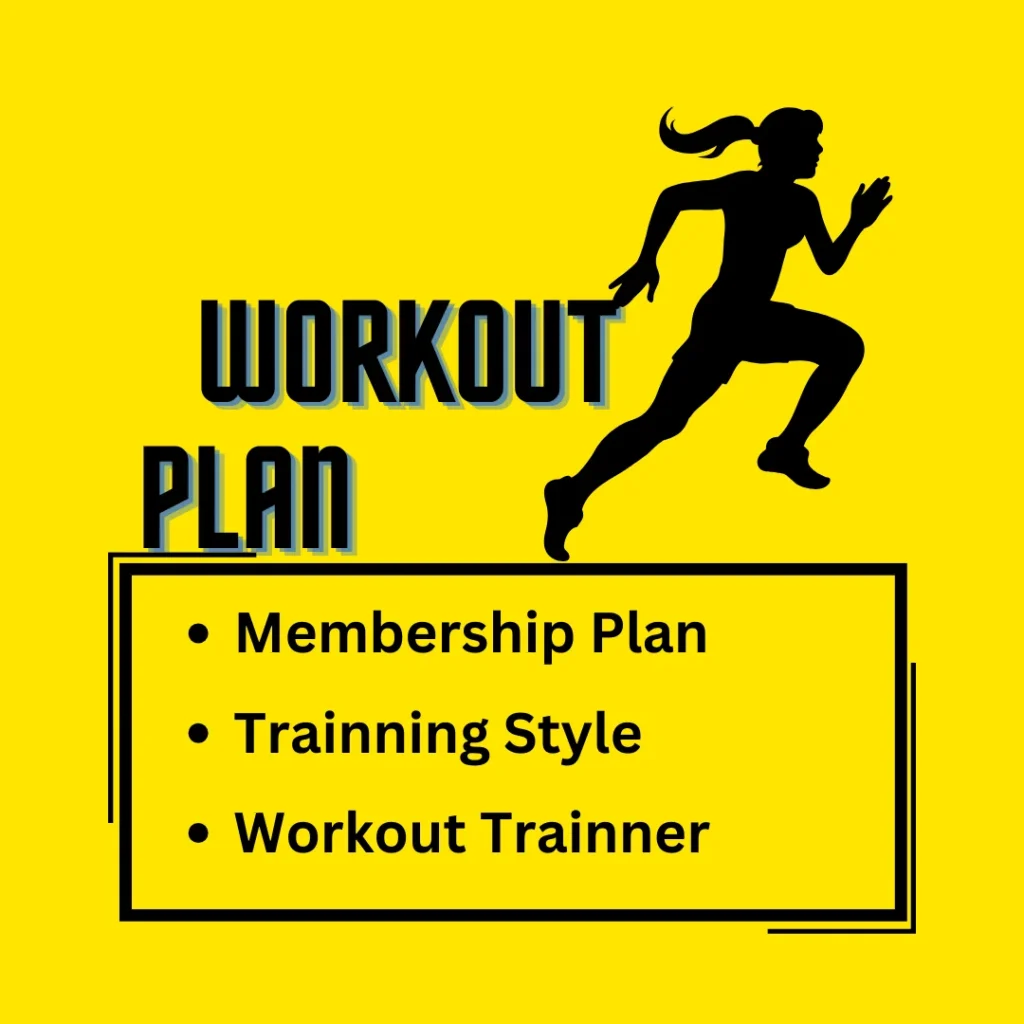
Example Code
- First, the program asks for the membership type and number of months.
- It uses a nested if-else structure inside each membership type to select the training type and whether a trainer is needed.
- The membership type determines the base cost (basic, standard, premium).
- Inside each membership type condition, the user is prompted to choose a training type.
- Another nested condition is used to check if the training type is valid, and then the user is asked whether they need a trainer.
- The program outputs the total cost along with the chosen options.
- This way, the nested if-else statements make the choices more specific within each membership type!
#include <iostream> using namespace std; int main() { string membershipType, trainingType, trainerNeeded; int months; float totalCost; // Input from user cout << "Enter membership type (basic/standard/premium): "; cin >> membershipType; cout << "Enter number of months: "; cin >> months; // Nested if-else structure for membership type and further choices if (membershipType == "basic") { totalCost = months * 20; cout << "Enter training type (strength/cardio/yoga): "; cin >> trainingType; if (trainingType == "strength" || trainingType == "cardio" || trainingType == "yoga") { cout << "Do you need a trainer? (yes/no): "; cin >> trainerNeeded; } else { cout << "Invalid training type!" << endl; return 1; } } else if (membershipType == "standard") { totalCost = months * 30; cout << "Enter training type (strength/cardio/yoga): "; cin >> trainingType; if (trainingType == "strength" || trainingType == "cardio" || trainingType == "yoga") { cout << "Do you need a trainer? (yes/no): "; cin >> trainerNeeded; } else { cout << "Invalid training type!" << endl; return 1; } } else if (membershipType == "premium") { totalCost = months * 50; cout << "Enter training type (strength/cardio/yoga): "; cin >> trainingType; if (trainingType == "strength" || trainingType == "cardio" || trainingType == "yoga") { cout << "Do you need a trainer? (yes/no): "; cin >> trainerNeeded; } else { cout << "Invalid training type!" << endl; return 1; } } else { cout << "Invalid membership type!" << endl; return 1; } // Output selections and total cost cout << "Membership Type: " << membershipType << endl; cout << "Training Type: " << trainingType << endl; cout << "Trainer Needed: " << trainerNeeded << endl; cout << "Total Cost: $" << totalCost << endl; return 0; }
Output
Enter membership type (basic/standard/premium): basic Enter number of months: 3 Enter training type (strength/cardio/yoga): strength Do you need a trainer? (yes/no): yes Membership Type: basic Training Type: strength Trainer Needed: yes Total Cost: $60
Conclusion
In conclusion, you have learned what is nesting, and how you can use nested if-conditional statements and nested if-else statements in C++, you looked at different visuals and example codes. In the end, you looked at a scenario-based question that was used nesting and how you can use it to the best of your abilities. There are many more situations where you can use nested conditional statements to make your program more interactive, user-friendly, and efficient.