In this article, you will learn to use if statement with multiple conditions using AND, OR, and NOT operators with real-world examples and visuals for more interactive learning. Before this, you need to know conditional statements, variables and data types, and also user input-output in some cases.
Table of Contents
In programming, there are some situations where you need to make decisions based on multiple factors. This is where an If Statement with Multiple Conditions comes in handy. It allows you to check several conditions at once and execute certain actions only if all or some of those conditions are met.
For example, you might want to ensure that a user has entered valid information in a form or that certain criteria are met in a game before moving to the next level. By using logical operators like “and” and “or,” you can create flexible and powerful conditional statements that help your program make smart decisions.
Multiple Conditions in if Statement: Using AND operator
The AND &&
operator allows you to check if statements with multiple conditions together. It makes sure that all of these conditions must be true for the code inside the if block to run. Using the AND operator makes the code simpler and easier to follow. This is useful when you need to do something only if all the conditions you care about are met.
Check Whether a Person is a Teenager or Not
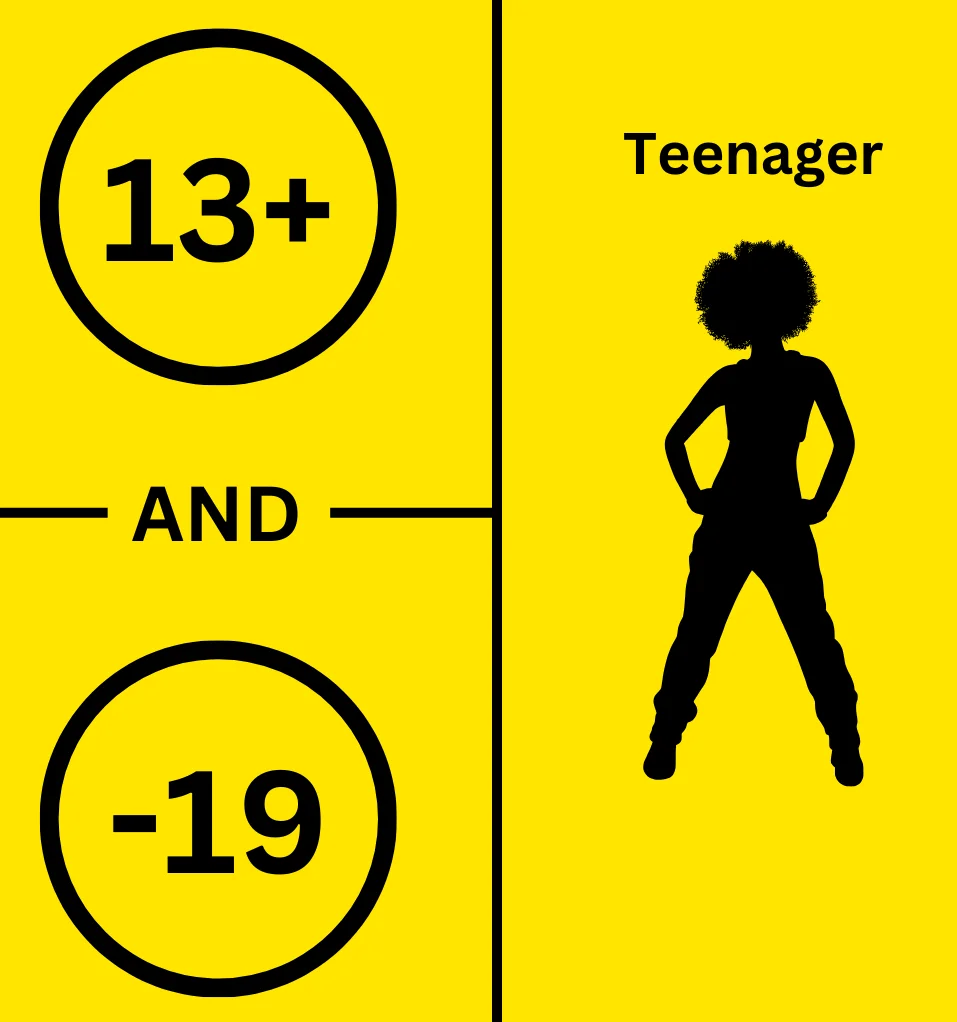
Code
In the following code:
int age = 16;
Sets the age to 16.if (age >= 13 && age <= 19)
Checks if theage
is between 13 and 19 (inclusive) by checking both left and right conditions with the AND operator. If both conditions are true, the program displays “The person is a teenager”.- If the condition evaluates to false, the program displays “The person is not a teenager”.
#include <iostream> using namespace std; int main() { int age = 16; // Example age if (age >= 13 && age <= 19) { cout << "The person is a teenager." << endl; } else { cout << "The person is not a teenager." << endl; } return 0; }
Output
The person is a teenager.
Multiple Conditions in if Statement: OR operator
The OR ||
operator lets you check multiple conditions in if statements. The code inside the if block will run if any conditions are true
. Using the OR operator makes the code simpler and easier to read, which is helpful when we want to do something if at least one of the conditions is met.
Program to Check Whether to Go on Hiking or Not Based on the Day
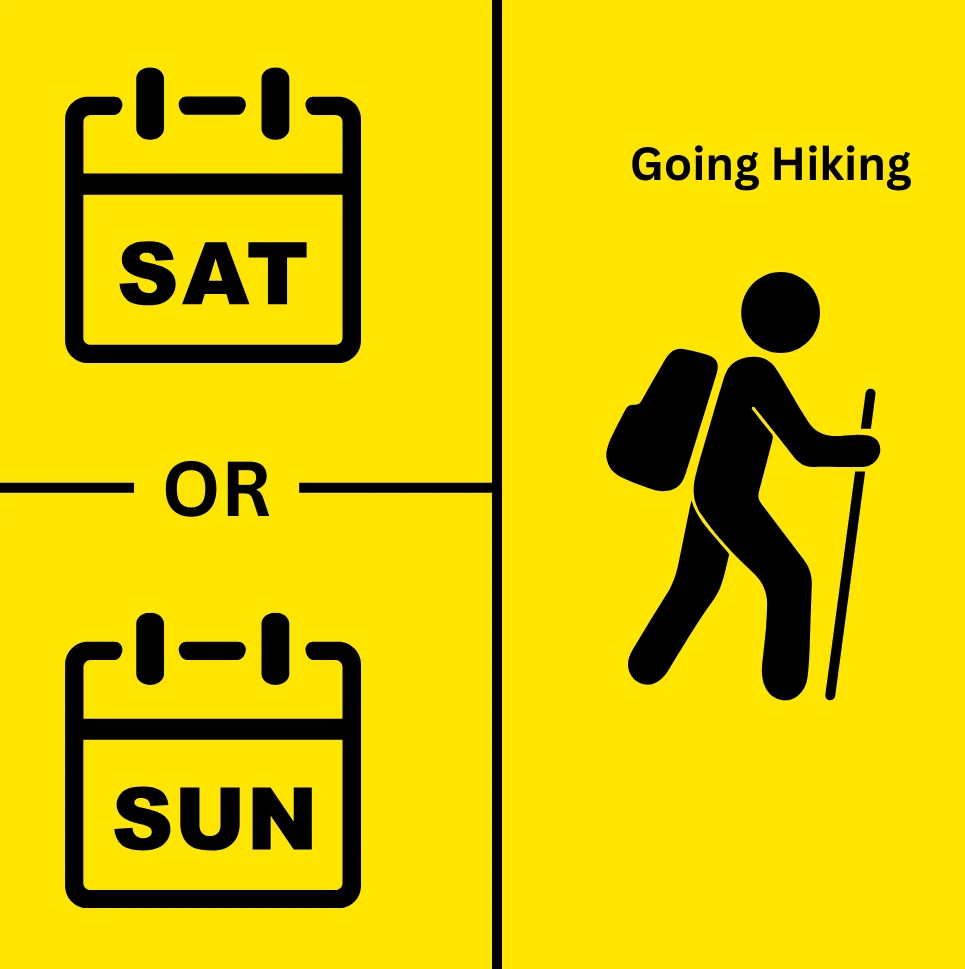
Code
In the following code:
- The program sets the value to
Sunday
in the variabledayofWeek
. If
statement checks if it isSaturday
orSunday
.If
any of the condition is true, it prints “It’s the weekend! Let’s go on a hike!”If
they are nottrue
thenelse
is executed and the program prints “It’s not the weekend. No hike today.”.
#include <iostream> using namespace std; int main() { string dayOfWeek = "Sunday"; // Change to "Saturday" or "Sunday" to see different outputs // Checking if it's Saturday OR Sunday to decide on hiking if (dayOfWeek == "Saturday" || dayOfWeek == "Sunday") { cout << "It's the weekend! Let's go on a hike!" << endl; } else { cout << "It's not the weekend. No hike today." << endl; } return 0; }
Sample Output
It's the weekend! Let's go on a hike!
Using NOT Operator with a Condition in If Statement
The NOT !
operator in if statements lets you check if a condition is false
. It works by evaluating the condition inside parenthesis first and revert its result. Means, if the condition is true, it makes the result as false and vice versa. If the condition is false
, the code inside the if block will run. Using the NOT operator makes the code easier to understand when we want to do something only if a specific condition is false
. This is useful when we want to take action if something is not happening.
Program to Check Critical Battery Level
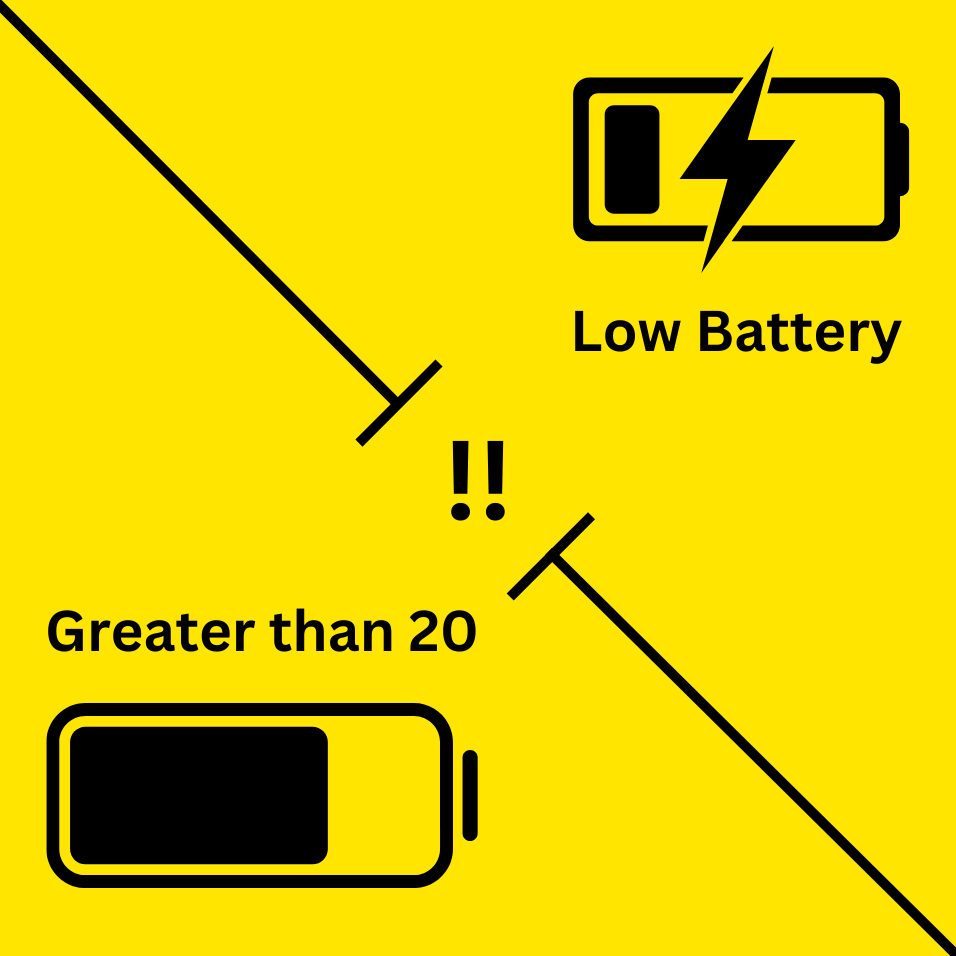
Code
#include <iostream> using namespace std; int main() { int batteryLevel = 75; // Example battery level // Check if battery level is not low if (!(batteryLevel < 20)) { cout << "Battery level is not low. Continue normal operation." << endl; } else { cout << "Battery level is low. Please recharge soon." << endl; } return 0; }
Output
Battery level is not low. Continue normal operation.
Explanation
- Set
batteryLevel
to 75% to represent the current battery status. - Check
if
the battery level is not below 20% using!(batteryLevel < 20).
If !(batteryLevel < 20)
evaluates totrue
, indicate that the battery level is not low and normal operation can continue.If !(batteryLevel < 20)
isfalse
, prompt the user to recharge the device soon.
Real-World example Using If Statement with Multiple Conditions
Now look at this simple example of a sports team selection eligibility, where the person needs to be in the age limit, have some sports experience or certification also check if there is a spot open in the sports team that remains.
Code
In the following code:
- The program initializes variables with sample values.
- Then using a single if statement with multiple conditions that uses AND, OR, and NOT operator the program checks if:
- The
age
is between 8 and 18 inclusive, using the&&
(AND) operator. - The person has either experience of a training certificate using the || (OR) operator.
- The team is not full (using the ! (NOT) operator.
- Outputs “You are eligible to join the sports team!” if all the above conditions are satisfied.
- Outputs “You are not eligible to join the sports team.” if any of the eligibility conditions fail.
#include <iostream> using namespace std; int main() { int age = 12; // Example age bool hasExperience = true; // Example skill level bool hasTrainingCertificate = false; // Example training certificate bool teamFull = false; // Example team capacity // Combine all conditions to determine eligibility if (age >= 8 && age <= 18 && (hasExperience || hasTrainingCertificate) && !teamFull) { cout << "You are eligible to join the sports team!" << endl; } else { cout << "You are not eligible to join the sports team." << endl; } return 0; }
Sample Output
You are eligible to join the sports team!
Try Some Scenario-Based Questions on Conditional Statements (with Multiple Conditions) in C++
Now that you’ve learned the basic concepts of using multiple conditions in if using logical operators, its time to apply that knowledge by solving some real-world coding exercise on conditional statements in C+ with multiple conditions to reinforce your learning.
Conclusion
In conclusion, you learned how to use if statement with multiple conditions using interesting visuals, real world examples and easy code snippets. Along with that, why it is not advised to use multiple if statements to check multiple conditions. Using multiple conditions in if statement using the AND, OR, and NOT operators makes your programs more efficient, readable and short. In the end you looked at a real-world example of a Sports Team Eligibility System that used all the different operators to combine multiple conditions together in a single if statement.