In this article, you will learn conditional statements in C++ for decision-making. The blog covers if, if-else, and if-else-if statements with real-world examples and engaging visuals in our beginner-friendly guide. To understand them correctly, you require prior knowledge of variables & data types, operators, and also user input and output in some cases.
Table of Contents
What are Conditional Statements in C++?
Conditional statements are also called decision statements. They allow specific actions to be taken based on whether certain conditions are met or not. This means the program can make decisions and respond differently in various situations. Conditional statements make your program interactive and user-friendly by changing the program’s output according to the situation.
For example, we can set up the program to do something when a condition is true or do something else when it’s false. Additionally, if neither condition is met, we can provide an alternative action. This way, the program can handle different scenarios smoothly and make appropriate choices depending on the conditions.
If Statement in C++
The if statement in C++ is the simplest type of decision statement. The if statement executes a code block when a specific condition is true. If statement is similar to asking, “Is this condition true? if so, then it executes that code. Otherwise, it ignores what’s written inside if block and continues with the rest of the code below it.
Real-world Example of If Statement
A simple way to understand if statement is to relate it to real life. A real-world example is that you make all your decisions in life based on some conditions. Such as, when you are at a traffic light, you make a decision based on some conditions like:
- When the light is Green -> You go
- When the light is Yellow -> You start your Engine
- When the light is Red -> You stop
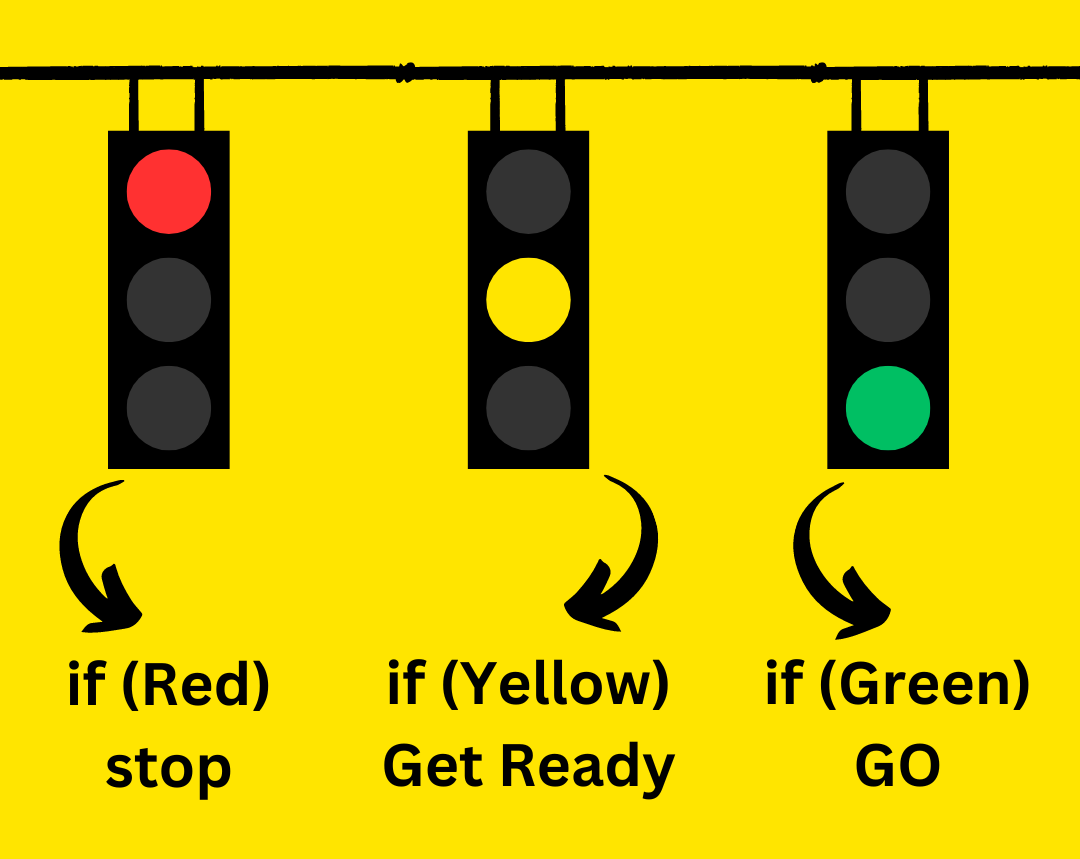
Flow Chart of If statement
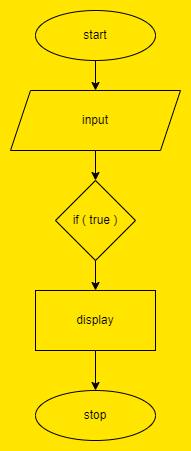
Syntax
if (condition) { // code to be executed if the condition is true }
Example Code
In the following code:
- The program sets the variable
x
to 10. - It checks if
x
is greater than 5. - Since
x = 10
, this condition evaluates to true. The program executes the if block and prints “x is greater than 5”. - After the
if
statement, the program prints “Statement outside if” regardless of the condition, going in the normal flow of execution.
#include <iostream> using namespace std; int main() { int x = 10; if (x > 5) { cout << "x is greater than 5" << endl; } cout << "Statement outside if" << endl; return 0; }
Output
x is greater than 5 Statement outside if
If else in C++
You have used the if statement to execute a block of code if a specific condition is true, but what if we want to happen if you want to do something false? This is where else comes into play. You can use else after an if to execute a code block when a specific condition is false. It’s like giving your program a choice between two actions based on a condition. Remember that, in if-else one block will always execute.
Real-world Example of If-else Statement
Imagine a situation in daily life where if it is raining, you would take an umbrella with you; otherwise, you would not, which demonstrates the use of having only two situations.
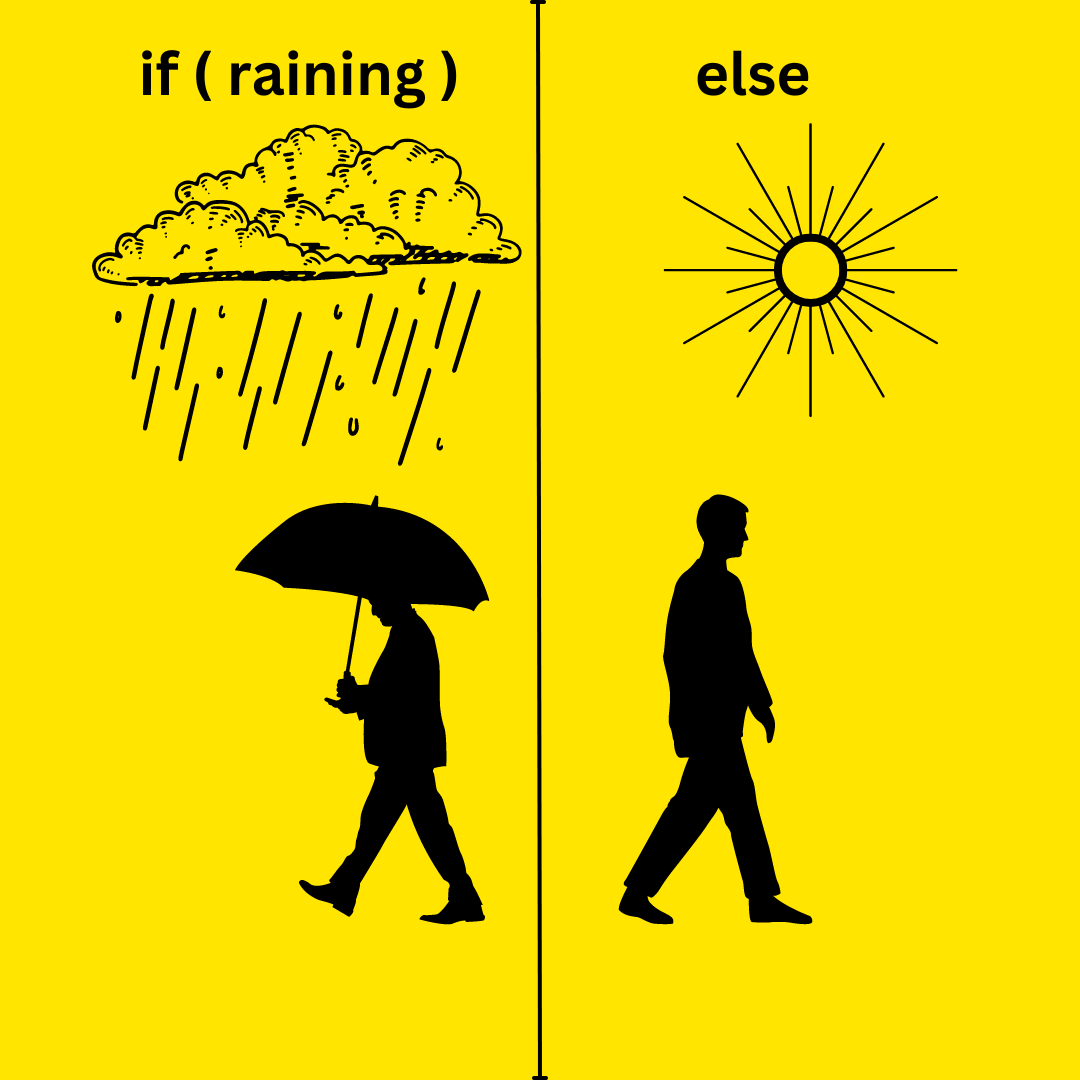
Flow Chart of if-else
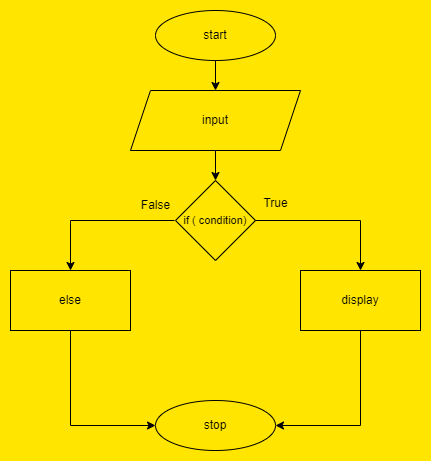
Syntax
if (condition) { // code to be executed if the condition is true } else { // code to be executed if the condition is false }
Example
In the following code:
- The program sets the variable
x
to 10. - The if statement checks if
x
is greater than 5. - Since
x = 10
, this condition evaluates to true. The program prints “x is greater than 5”. If the condition were false, it would print “x is 5 or less” instead. - After the
if-else
block, the program prints “statement after else block” regardless of the condition, going in the normal flow of execution.
#include <iostream> using namespace std; int main() { int x = 10; if (x > 5) { cout << "x is greater than 5" << endl; } else { cout << "x is 5 or less" << endl; } cout << "statement after else block" << endl; return 0; }
Output
x is greater than 5 statement after else block
Else if in C++
Just like the if-else statement gives your program a choice between two actions based on a condition, the else-if statement allows you to add more conditions to check after an initial if statement. This is helpful when you have multiple conditions to consider in sequence, and only one of them can be true. Whenever there are multiple else-if statements in a program, the program starts checking from top to bottom to find which condition is true. Whenever it finds that condition to be true, it does not check the conditions below it and directly jumps to the end of the else or else if.
The else statement is optional here and it executes when none of the above conditions are true. The else-if statements is also called else-if ladder because it works like a ladder. Where, in each step you check whether the current condition is true or not. That’s why note that the flowchart of else if looks like a ladder.
Real-world Example of Else-if Statement
You can relate to it else if it is in your real-life situation. When you are headed out, you wear different types of footwear based on what you will do (running, hiking, formal events, casual).
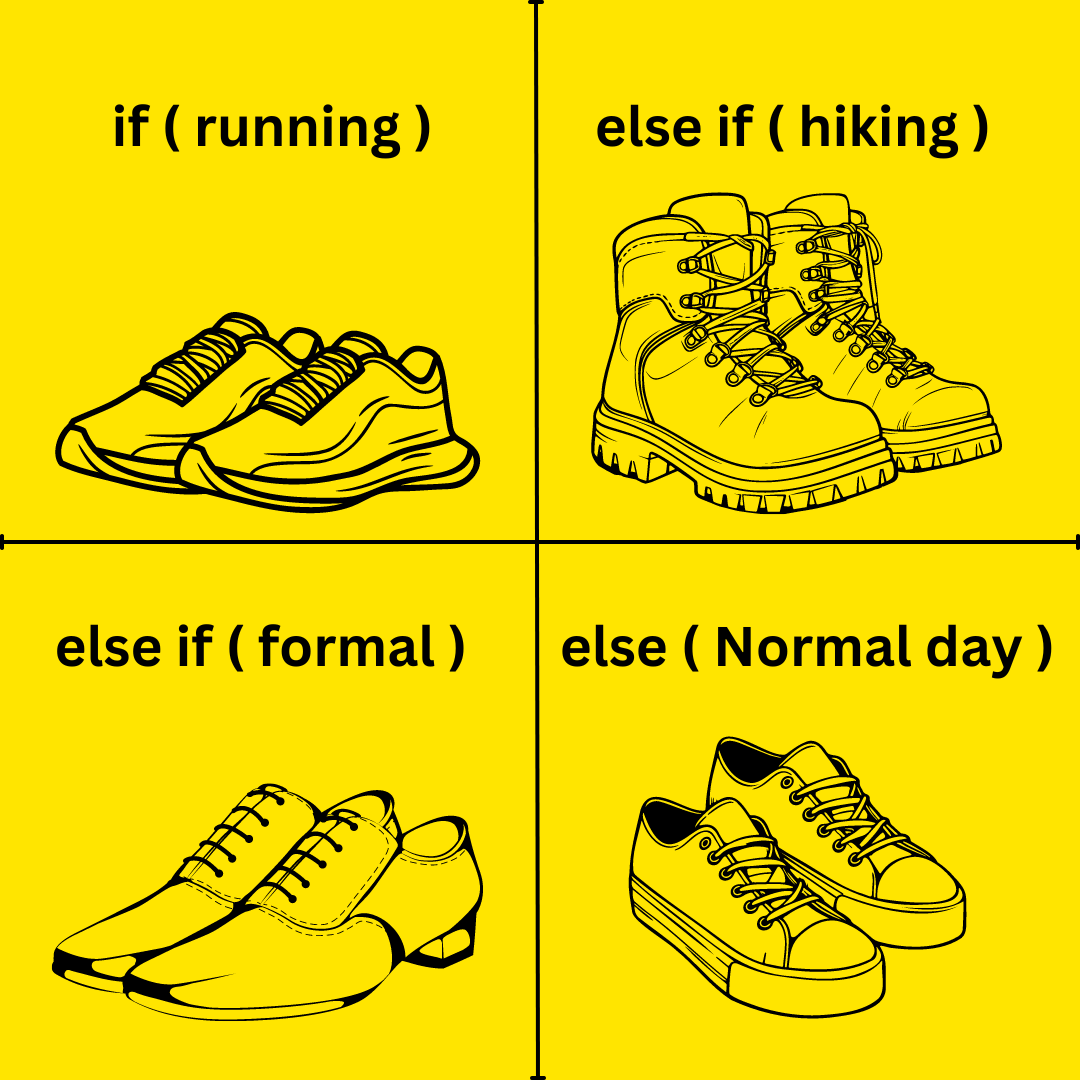
Flow Chart of else-if
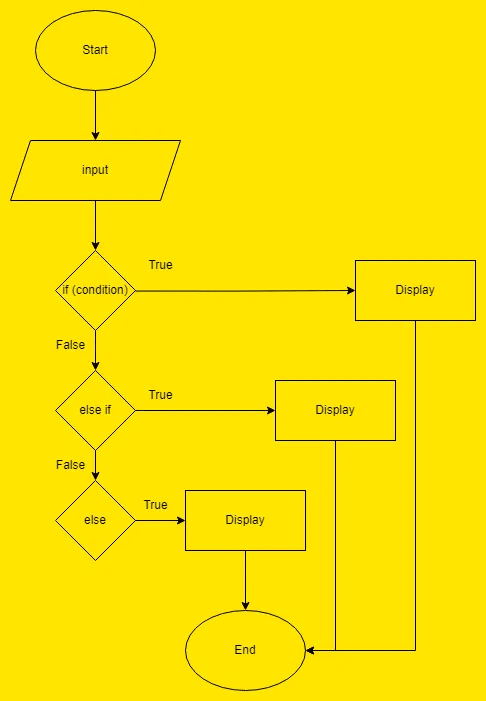
Syntax
if (condition1) { // code to be executed if condition1 is true } else if (condition2) { // code to be executed if condition1 is false and condition2 is true } else { // code to be executed if both condition1 and condition2 are false }
Example Code
In the following code:
- The program sets the variable
x
to 10. - It first checks if
x
is greater than 10. Sincex = 10
, this condition is false. - It then checks if
x
is exactly 10. Sincex = 10
, this condition is true. The program prints “x is exactly 10”. - If neither condition were true, it would print “x is less than 10”.
#include <iostream> using namespace std; int main() { int x = 10; if (x > 10) { cout << "x is greater than 10" << endl; } else if (x == 10) { cout << "x is exactly 10" << endl; } else { cout << "x is less than 10" << endl; } return 0; }
Output
x is exactly 10
Real-world Example Program on All Conditional Statements
Here is a simple real-world example a program that tells the user what to do based on the day of the week, weather, and free time.
Example Code
- The program sets
dayOfWeek
to “Sunday”,weather
to “sunny”, andfreeTime
to 3. - The program checks the first
if
statement to see ifdayOfWeek
is “Sunday”. SincedayOfWeek
is “Sunday”, it prints “It’s the weekend! Time to relax.” - The program then checks the second
if-else
statement to see ifweather
is “rainy”. Sinceweather
is “sunny”, it prints “The weather is nice, a great day to be outdoors!” - The program then evaluates the
else if
ladder forfreeTime
. - Since
freeTime
is 3, it matches the conditionfreeTime >= 3
and prints “You have some free time. Maybe watch a movie or read a book?”
Now for more interactive learning, copy the code, try changing variable values and observe the output based on the conditions. For this, you can utilize an excellent tool Python Tutor that let’s you visualize the code
#include <iostream> using namespace std; int main() { string dayOfWeek = "Sunday"; string weather = "sunny"; int freeTime = 3; // free time in hours // Example of if statement if (dayOfWeek == "Sunday") { cout << "It's the weekend! Time to relax." << endl; } // Example of if-else statement if (weather == "rainy") { cout << "Since it's raining, staying indoors is a good idea." << endl; } else { cout << "The weather is nice, a great day to be outdoors!" << endl; } // Example of else if statement if (freeTime >= 5) { cout << "You have plenty of free time. How about going on a day trip?" << endl; } else if (freeTime >= 3) { cout << "You have some free time. Maybe watch a movie or read a book?" << endl; } else if (freeTime >= 1) { cout << "You have a little free time. Perhaps go for a walk or exercise?" << endl; } else { cout << "Not much free time. Just relax at home for a bit." << endl; } return 0; }
Output
It's the weekend! Time to relax. The weather is nice, a great day to be outdoors! You have some free time. Maybe watch a movie or read a book?
Try Some Variations on Your Own and Visualize the Code
As Syntax Scenarios aims to learn coding using creativity, so go ahead and visualize the code using an amazing online tool called Python Tutor. Copy the code, and paste there. Then using the next and previous buttons execute and visualze each step, see the current value of variables, and the flow of execution. Through this, you will have a more clear idea of what’s happening on the back-end. Be creative! Be smart!
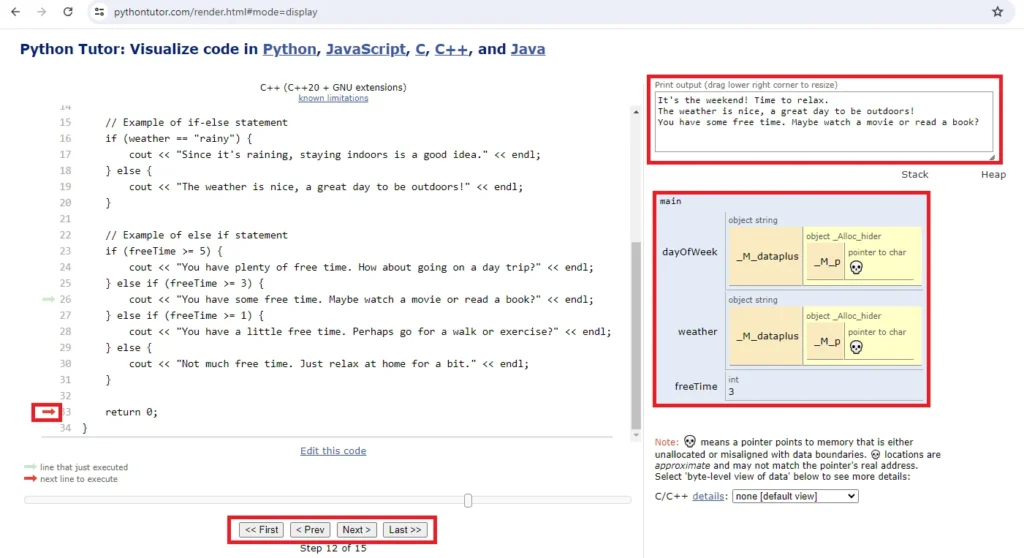
Time to Implement What You’ve Learned!
Now that you have learned conditional statements in C++, their syntax, and code snippets, it’s time to reinforce what you’ve learned. Try our quiz on conditional statements that includes multiple choice, fill-in-the-blanks, true-false, and drag-and-drop.
Remember, the best way to learn programming is to practice it. So, for hands-on practice, solve our scenario-based coding problems on if-else, which include real-world scenarios and engaging visuals.
Conclusion
In this article, you learned about if, if-else, and else-if conditional statements, along with their syntax, flowchart, real-world examples, engaging visuals, and code snippets specially designed for beginner programmers. Conditional statements in C++ allow you to make your programs more interactive and user-friendly and are the building blocks to developing large-scale applications,