Ready to dive into some hands-on coding? After solving scenario-based questions on if-else in C++ (with single conditions), Let’s tackle exciting scenario-based problems that bring real-world situations to life. This time, you’ll be focusing on scenario-based coding questions designed around conditional statements in C++ with multiple conditions, using AND (&) and OR (||) operators. Solve our uniquely designed scenario-based coding questions along with visuals to enhance your programming skills by creating interactive and user-friendly programs.
Note: You can see the answer code along with its explanation by clicking on the question (or the drop down icon).
Treasure Hunt
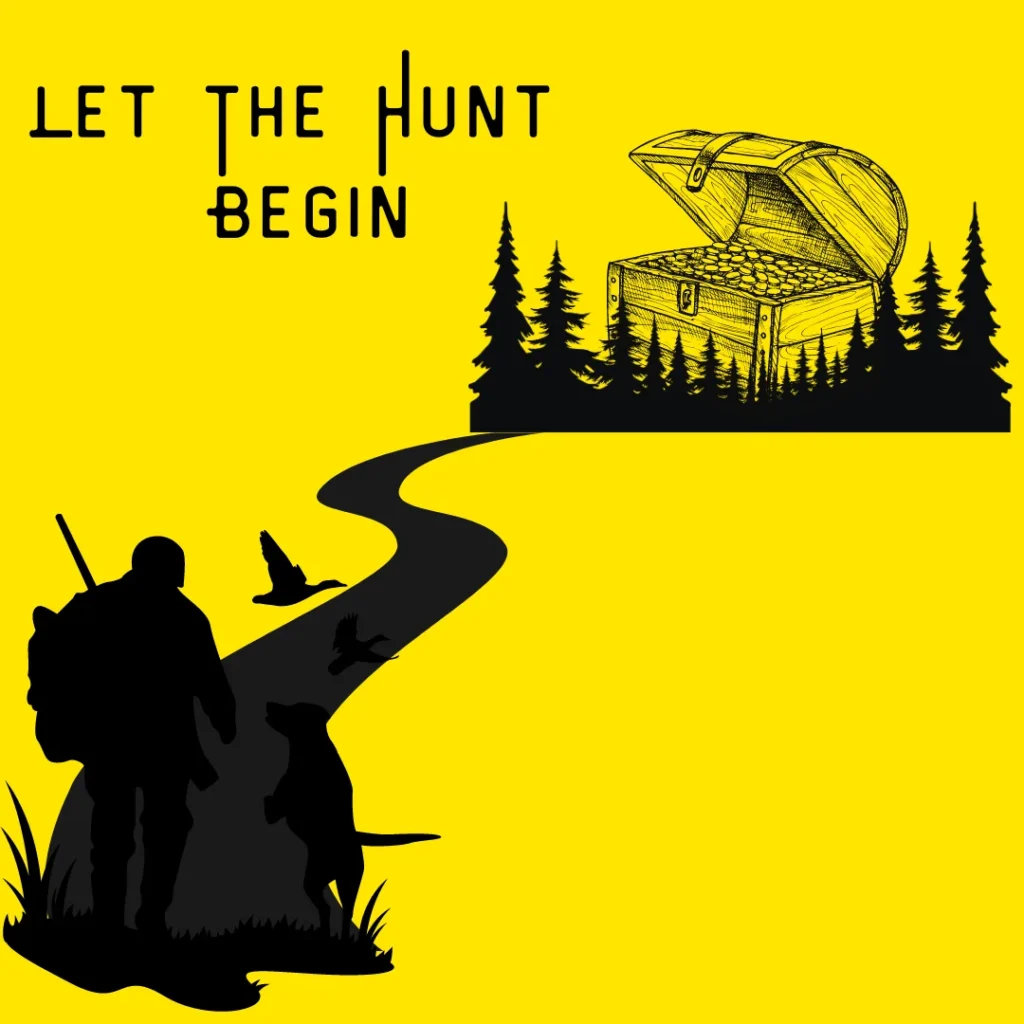
You are a developer tasked with creating a treasure hunt game. In this game, the player searches for a hidden treasure at one of four locations. The player has a couple of tools to find the treasure a key and a map but their success in finding the treasure depends on whether they have these tools.
Locations:
Location 1: The player can search here, but the treasure is not located there.
Location 2: The map indicates that the treasure might be here, but only players with a map will receive this clue.
Location 3: This is a decoy location where the treasure is not hidden.
Location 4: The treasure might be locked behind a door, and the player will need a key to access it.
Rules:
1. The player must first indicate whether they have a key and a map.
2. If the player has a map, they will receive a clue that the treasure may be hidden at Location
3. If the player chooses Location 4, they will need a key to unlock the door and collect the treasure.
4. If the player searches in the wrong location (Location 1 or Location 3), they will be told that the treasure is not there, and they can try again.
The player’s decisions on where to search for the treasure will determine whether they succeed or not.
Test Case 1:
Input:
Has Key: y
Has Map: y
Location chosen: 2
Expected Output:
Do you have a key? (y/n): y
Do you have a map? (y/n): y
You have a map! The map shows the treasure might be at Location 2.
Choose a location to search for the treasure (1, 2, 3, or 4): 2
Congratulations! You found the treasure at Location 2 using the map!
Test Case 2:
Input:
Has Key: n
Has Map: y
Location chosen: 4
Expected Output:
Do you have a key? (y/n): n
Do you have a map? (y/n): y
You have a map! The map shows the treasure might be at Location 2.
Choose a location to search for the treasure (1, 2, 3, or 4): 4
The treasure is locked behind a door. You need a key to access Location 4!
Explanation
Explanation of the Code:
The game starts by asking the player if they have a key and a map. Based on their answers, the program sets two boolean variables, hasKey and hasMap, to track whether the player possesses these items.
If the player has a map, they receive a clue that the treasure might be at Location 2. Without the map, they get no clues and must guess.
The player is then prompted to choose one of four locations (1, 2, 3, or 4) to search for the treasure.
Location 2: If the player chooses this location and has a map, they find the treasure. If they don’t have the map, they won’t find it.
Location 4: If the player chooses Location 4, they need a key. If they have the key, they find the treasure. If not, they are informed that the treasure is locked.
Locations 1 and 3: These locations do not contain the treasure, and the player is informed that the treasure is not there.
The game uses simple conditional statements (if-else) with logical operators to handle all the different outcomes without using nested conditions.
#include <iostream> using namespace std; int main() { int location; bool hasKey = false, hasMap = false; char answer; // Ask the player if they have a key cout << "Do you have a key? (y/n): "; cin >> answer; hasKey = (answer == 'y' || answer == 'Y'); // Ask the player if they have a map cout << "Do you have a map? (y/n): "; cin >> answer; hasMap = (answer == 'y' || answer == 'Y'); // Provide a clue based on whether the player has a map if (hasMap) { cout << "You have a map! The map shows the treasure might be at Location 2." << endl; } else { cout << "You don't have a map, so you must guess where the treasure is hidden!" << endl; } // Choose a location to search for the treasure cout << "Choose a location to search for the treasure (1, 2, 3, or 4): "; cin >> location; // Check conditions for finding the treasure if (location == 4 && !hasKey) { cout << "The treasure is locked behind a door. You need a key to access Location 4!" << endl; } else if (location == 2 && hasMap) { cout << "Congratulations! You found the treasure at Location 2 using the map!" << endl; } else if (location == 4 && hasKey) { cout << "Congratulations! You used the key and found the treasure at Location 4!" << endl; } else if (location == 3 || location == 1) { cout << "Sorry, the treasure is not at this location. Try again!" << endl; } return 0; }
The Alien Ship
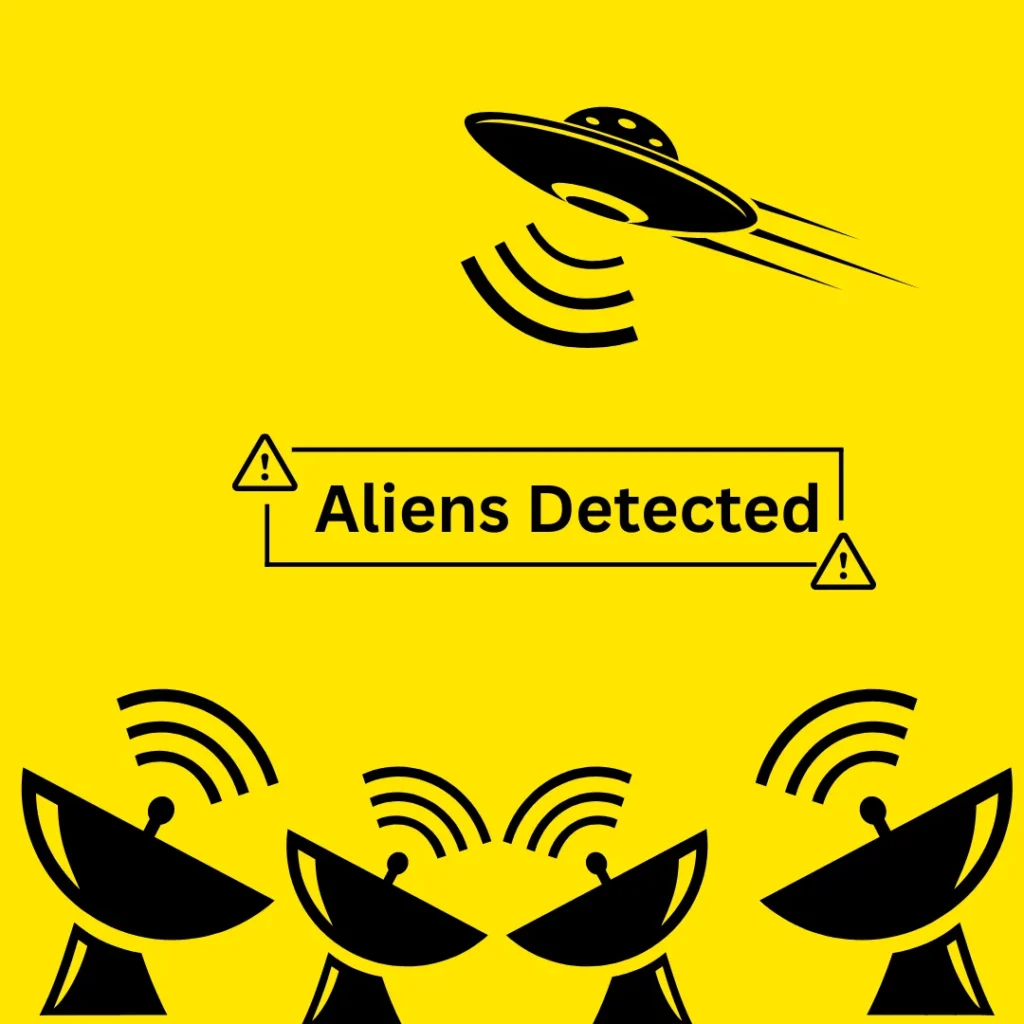
You have discovered a mysterious alien ship with three adjustable settings: Power Level, Frequency, and Mode. The artifact’s effect is determined by the combination of these settings. The ship can produce various effects based on specific ranges and combinations of settings. The settings might not always align perfectly due to possible defects in the dials.
Task: Write a program to determine the ship effect based on the following settings:
Power Level: An integer ranging from 1 to 20.
Frequency: An integer ranging from 40 to 100.
Mode: A string which can be “Basic”, “Standard”, or “Advanced”.
Conditions:
In the case Power Level is more than 15, Frequency is greater than 70, and Mode is “Advanced”, the effect is “Quantum Leap”.On the other hand when Power Level is higher than 15, Frequency is 70 or less, and Mode is “Standard”, the effect is “Energy Surge”.Also, if the Power Level is greater than 10, Frequency is greater than 60, and Mode is “Basic”, the effect is “Temporal Shift”. When Power Level is 15 or less and Frequency is 60 or less, or Mode is “Basic” and Power Level is greater than 5, the effect is “Energy Shield”. But when the Power Level is greater than 10, Frequency is greater than 50, and Mode is “Standard”, the effect is “Stability Boost”. Besides that, if none of the above conditions are met, the effect is an “Unknown Signal”.
User provides values for Power Level, Frequency, and Mode.
Display the effect of the ship based on the input settings.
Test Case 1
Input:
Power Level: 18
Frequency: 75
Mode: “Advanced”
Expected Output:
Effect: Quantum Leap
Test Case 2
Input:
Power Level: 12
Frequency: 55
Mode: “Standard”
Expected Output:
Effect: Stability Boost
Explanation
- The program initializes variables for powerLevel, frequency, and mode.
- It prompts the user to enter values for these variables.
- The program then evaluates the conditions to determine the artifact’s effect:
- If Power Level > 15, Frequency > 70, and Mode is “Advanced”, it outputs “Quantum Leap”.
- If Power Level > 15, Frequency ≤ 70, and Mode is “Standard”, it outputs “Energy Surge”.
- If Power Level > 10, Frequency > 60, and Mode is “Basic”, it outputs “Temporal Shift”.
- If either Power Level ≤ 15 and Frequency ≤ 60 or Mode is “Basic” and Power Level > 5, it outputs “Energy Shield”.
- If Power Level > 10, Frequency > 50, and Mode is “Standard”, it outputs “Stability Boost”.
- If none of the conditions match, it outputs “Unknown Signal”.
- The output is determined by matching the user’s input against these conditions.
#include <iostream> #include <string> using namespace std; int main() { int powerLevel; int frequency; string mode; // Input settings cout << "Enter power level (1-20): "; cin >> powerLevel; cout << "Enter frequency (40-100): "; cin >> frequency; cout << "Enter mode (Basic/Standard/Advanced): "; cin >> mode; // Determine the artifact’s effect based on settings if (powerLevel > 15 && frequency > 70 && mode == "Advanced") { cout << "Effect: Quantum Leap" << endl; } else if (powerLevel > 15 && frequency <= 70 && mode == "Standard") { cout << "Effect: Energy Surge" << endl; } else if (powerLevel > 10 && frequency > 60 && mode == "Basic") { cout << "Effect: Temporal Shift" << endl; } else if ((powerLevel <= 15 && frequency <= 60) || (mode == "Basic" && powerLevel > 5)) { cout << "Effect: Energy Shield" << endl; } else if (powerLevel > 10 && frequency > 50 && mode == "Standard") { cout << "Effect: Stability Boost" << endl; } else { cout << "Effect: Unknown Signal" << endl; } return 0; }
The Ancient Codebreaker
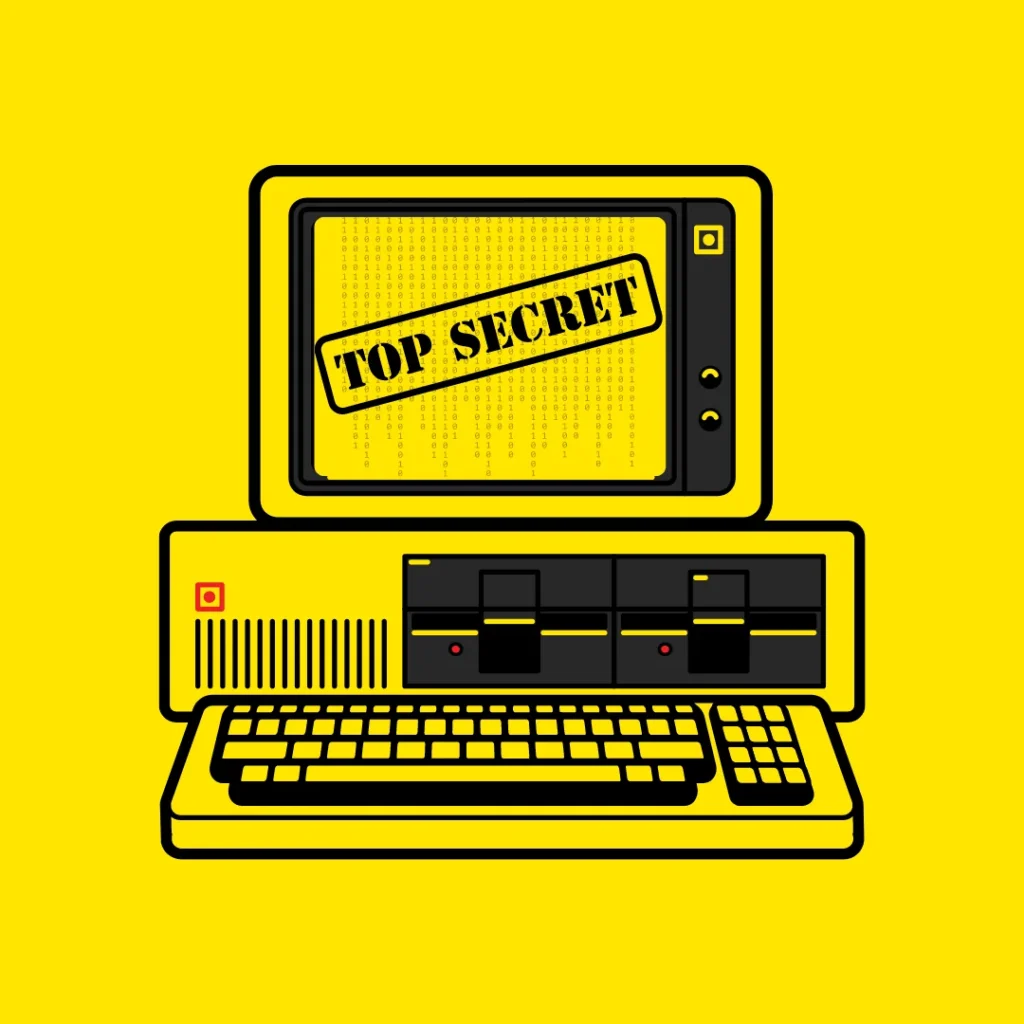
Imagine you have an ancient codebreaking device that helps you decode secret messages. This device has three important settings: Difficulty Level, Tool Quality, and Expertise. The Difficulty Level is a number between 1 and 10 that shows how challenging the code is to crack. Tool Quality, also a number between 1 and 10, indicates how good your tools are for decoding. Lastly, Expertise refers to your skill level, which can be “Beginner,” “Intermediate,” “Advanced,” or “Expert.”
Depending on these settings, the device will suggest different methods to decode the message. For example, if the Difficulty Level is above 8, Tool Quality is above 9, and your Expertise is “Expert,” the device will recommend a “Quantum Decryption” method. On the other hand, if the Difficulty Level is above 8, Tool Quality is 9 or less, and your Expertise is “Advanced,” it will suggest a “Complex Algorithm.” There are other combinations as well, such as “Advanced Technique,” “Optimized Approach,” and more, depending on the values you enter.
Your task is to write a program that takes these three settings as input and tells you which decoding method the device will suggest. If none of the predefined combinations match, the program should indicate that the decoding method is “Unknown.”
Test Case 1:
Input:
Difficulty Level: 9
Tool Quality: 10
Expertise: “Expert”
Expected Output:
“Decode Method: Quantum Decryption”
Test Case 2:
Input:
Difficulty Level: 6
Tool Quality: 4
Expertise: “Intermediate”
Expected Output:
“Decode Method: Practical Method”
Explanation
- The program initializes three variables: difficultyLevel, toolQuality, and expertise, with specific values representing the settings of the codebreaking device.
- The first if statement checks if the difficultyLevel is greater than 8, toolQuality is greater than 9, and expertise is “Expert”. If all these conditions are true, the output will be “Quantum Decryption”.
- The else if statement then checks if the difficultyLevel is greater than 8, toolQuality is 9 or less, and expertise is “Advanced”. If these conditions are met, it outputs “Complex Algorithm”.
- Another else if statement verifies if the difficultyLevel is greater than 5, toolQuality is greater than 7, and expertise is “Expert”. If true, it outputs “Advanced Technique”.
- The next else if checks if the difficultyLevel is greater than 5, toolQuality is 7 or less, and expertise is “Intermediate”. If this condition is met, it outputs “Optimized Approach”.
- Another else if checks if the difficultyLevel is 5 or less, toolQuality is greater than 5, and expertise is “Expert”. If true, it outputs “Strategic Solution”.
- The following else if verifies if the difficultyLevel is 5 or less, toolQuality is 5 or less, and expertise is “Intermediate”. If all these conditions are true, it outputs “Practical Method”.
- The next else if checks if the difficultyLevel is 5 or less, toolQuality is 5 or less, and expertise is “Beginner”. If true, it outputs “Basic Approach”.
- If none of these conditions match, the else block outputs “Decode Method: Unknown”, indicating that the settings do not fit any predefined decoding method.
#include <iostream> #include <string> using namespace std; int main() { int difficultyLevel = 5; int toolQuality = 7; string expertise = "Expert"; if (difficultyLevel > 8 && toolQuality > 9 && expertise == "Expert") { cout << "Decode Method: Quantum Decryption" << endl; } else if (difficultyLevel > 8 && toolQuality <= 9 && expertise == "Advanced") { cout << "Decode Method: Complex Algorithm" << endl; } else if (difficultyLevel > 5 && toolQuality > 7 && expertise == "Expert") { cout << "Decode Method: Advanced Technique" << endl; } else if (difficultyLevel > 5 && toolQuality <= 7 && expertise == "Intermediate") { cout << "Decode Method: Optimized Approach" << endl; } else if (difficultyLevel <= 5 && toolQuality > 5 && expertise == "Expert") { cout << "Decode Method: Strategic Solution" << endl; } else if (difficultyLevel <= 5 && toolQuality <= 5 && expertise == "Intermediate") { cout << "Decode Method: Practical Method" << endl; } else if (difficultyLevel <= 5 && toolQuality <= 5 && expertise == "Beginner") { cout << "Decode Method: Basic Approach" << endl; } else { cout << "Decode Method: Unknown" << endl; } return 0; }
The Loan Approval System
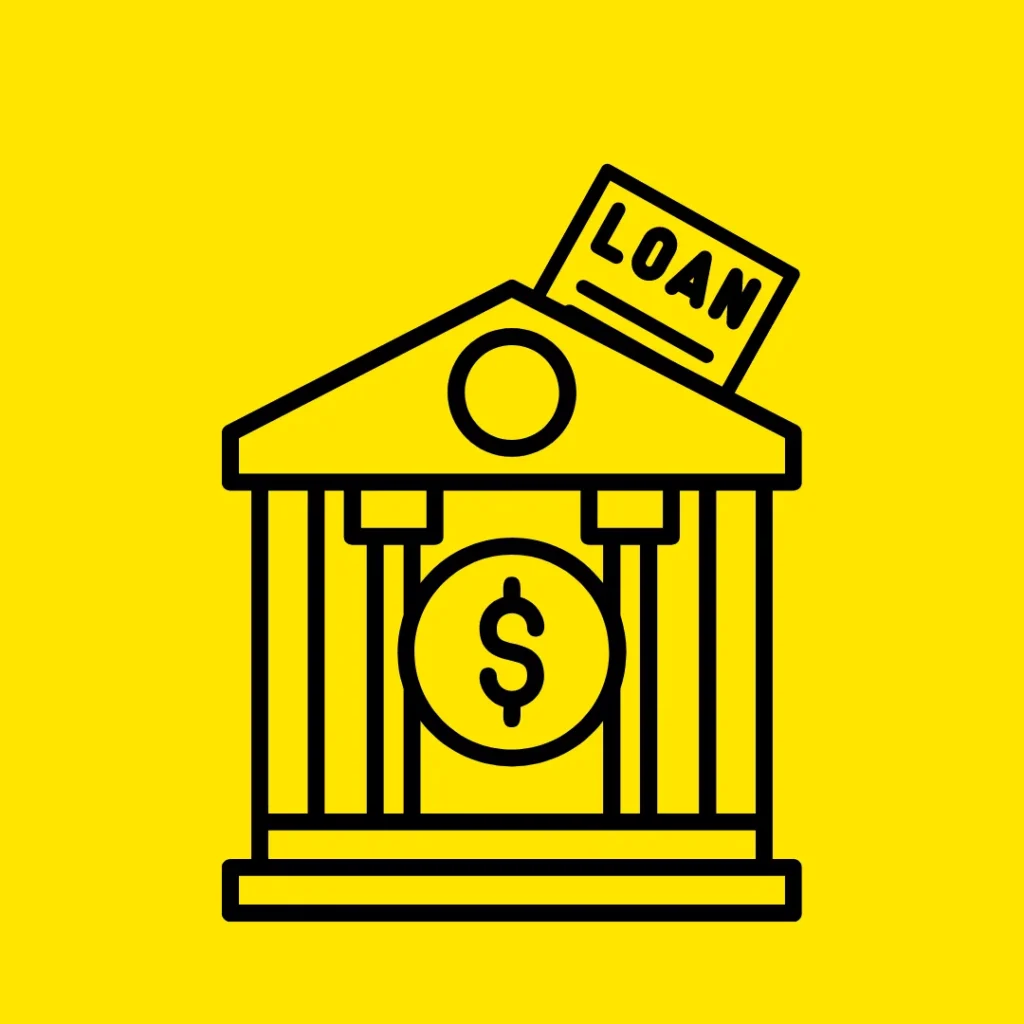
Imagine you’re working for a bank, and you need to decide if a customer qualifies for a loan based on their financial details. The decision depends on three factors: their credit score, their existing debt, and the amount of loan they require. First, the bank checks the debt-to-income ratio by dividing the customer’s total debt by their income. If the debt-to-income ratio exceeds 40%, the loan is denied due to a high debt load. If the ratio is within acceptable limits, the credit score is checked. Customers with a credit score of 750 or higher are approved with the best interest rate, while those with a credit score between 700 and 749 receive a standard interest rate. If the credit score is below 700, the loan is denied. Finally, if the loan amount requested exceeds 50% of the customer’s income, the loan is denied even if other conditions are met. Your task is to write a program that inputs these values and outputs the loan status and interest rate based on these criteria.
Test Case 1:
Input: Credit Score: 780
Income: $80,000
Debt: $20,000
Loan Amount Requested: $30,000
Expected Output: “Loan Status: Approved with best interest rate”
Test Case 2:
Input: Credit Score: 690
Income: $50,000
Debt: $30,000
Loan Amount Requested: $10,000
Expected Output: “Loan Status: Denied due to high debt-to-income ratio”
Explanation
The program prompts the user to enter their credit score, income, total debt, and the amount of loan they are requesting.It calculates the debt-to-income ratio by dividing the user’s debt by their income, ensuring that the result is a float for accurate calculations.First, it checks if the debt-to-income ratio exceeds 0.4 (or 40%). If so, the loan is denied due to a high debt load.Next, it checks if the requested loan amount exceeds 50% of the user’s income. If it does, the loan is denied due to requesting too much in relation to income.If both the debt-to-income ratio and loan amount are within limits, the program checks the user’s credit score:
- If the credit score is 750 or above, the loan is approved with the best interest rate.
- If the credit score is between 700 and 749, the loan is approved with a standard interest rate.
- If the credit score is below 700, the loan is denied due to insufficient credit history.
#include <iostream> using namespace std; int main() { int creditScore = 720; int income = 60000; int debt = 25000; float debtToIncomeRatio = (float)debt / income; if (debtToIncomeRatio > 0.4) { cout << "Loan Status: Denied due to high debt-to-income ratio" << endl; } else if (creditScore >= 750) { cout << "Loan Status: Approved with best interest rate" << endl; } else if (creditScore >= 700 && creditScore < 750) { cout << "Loan Status: Approved with standard interest rate" << endl; } else { cout << "Loan Status: Denied due to low credit score" << endl; } return 0; }
Electric Vehicle Charging Station
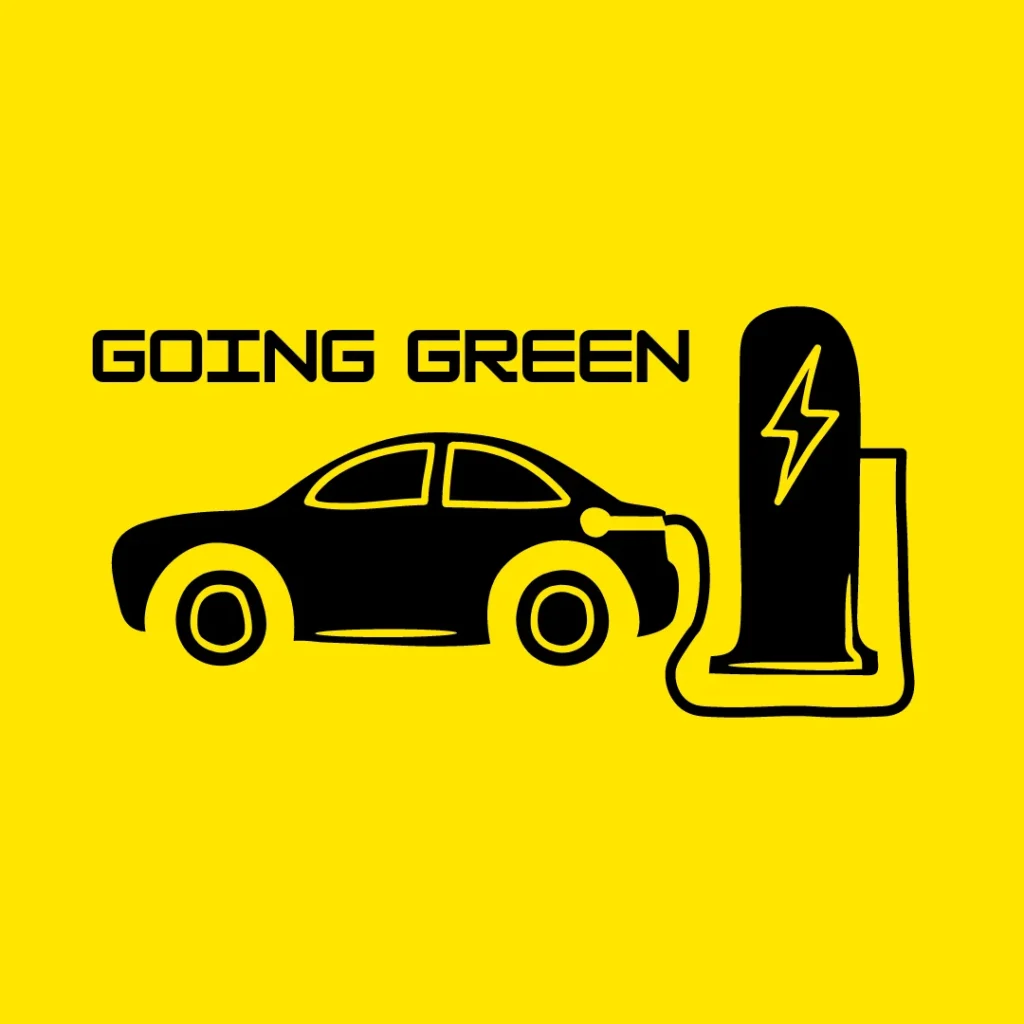
You are tasked with designing a smart charging system for electric vehicles in a futuristic city. The system calculates the appropriate charging rate based on three key factors: the type of vehicle, its battery level, and the time of day. Here’s how the charging rate is determined:
Vehicle Types:
Sedan: Discounted charging rate during Off-Peak hours.
SUV: Standard charging rate regardless of other factors.
Battery Levels:
Below 15%: Prioritized charging (higher rate).
50% or more: Increased rate during Peak hours.
Time of Day:
Off-Peak: Discounted rate for Sedans.
Peak: Increased rate if battery is 50% or more.
Charging Rates:
Standard Rate: $10 per kWh
Discounted Rate: $7 per kWh
Increased Rate: $15 per kWh
Prioritized Charging Rate: $20 per kWh
Test Case 1:
Input: Vehicle Type: Sedan
Battery Level: 10
Time of Day: Off-Peak
Expected Output: Final Charging Rate: $5 per unit
Test Case 2:
Input: Vehicle Type: SUV
Battery Level: 60
Time of Day: Peak
Expected Output: Final Charging Rate: $4 per unit
Explanation:
- The program first initializes the vehicle type, battery level, and time of day variables by asking for user input.
- Based on the battery level, vehicle type, and time of day, different conditions are checked to determine the charging rate.
- If the battery level is less than 15%, the vehicle is given a priority charging rate of $5 per unit to ensure it doesn’t run out of charge.
- If the vehicle is a “Sedan” and it’s “Off-Peak” hours, the charging rate is discounted to $2 per unit as a reward for charging during less busy times.
- If the vehicle is an “SUV,” the program applies a flat rate of $4 per unit, regardless of other conditions.
- If the battery level is 50% or more and it’s “Peak” hours, the rate increases to $6 per unit due to high demand.
- For any other combination of inputs, the program applies a normal charging rate of $3 per unit.
- Finally, the charging rate is printed to the screen.
#include <iostream> #include <string> using namespace std; int main() { string vehicleType; float batteryLevel; string timeOfDay; float chargingRate; // Input cout << "Enter vehicle type (Sedan/SUV): "; cin >> vehicleType; cout << "Enter battery level (in %): "; cin >> batteryLevel; cout << "Enter time of day (Peak/Off-Peak): "; cin >> timeOfDay; // Charging logic if (batteryLevel < 15) { chargingRate = 5.0; // Prioritized charging rate } else if (vehicleType == "Sedan" && timeOfDay == "Off-Peak") { chargingRate = 2.0; // Discounted rate for Sedan in Off-Peak hours } else if (vehicleType == "SUV") { chargingRate = 4.0; // Standard rate for SUV } else if (batteryLevel >= 50 && timeOfDay == "Peak") { chargingRate = 6.0; // Increased rate for high demand } else { chargingRate = 3.0; // Normal charging rate } // Output final charging rate cout << "Final Charging Rate: $" << chargingRate << " per unit" << endl; return 0; }
The Wizard’s Spells
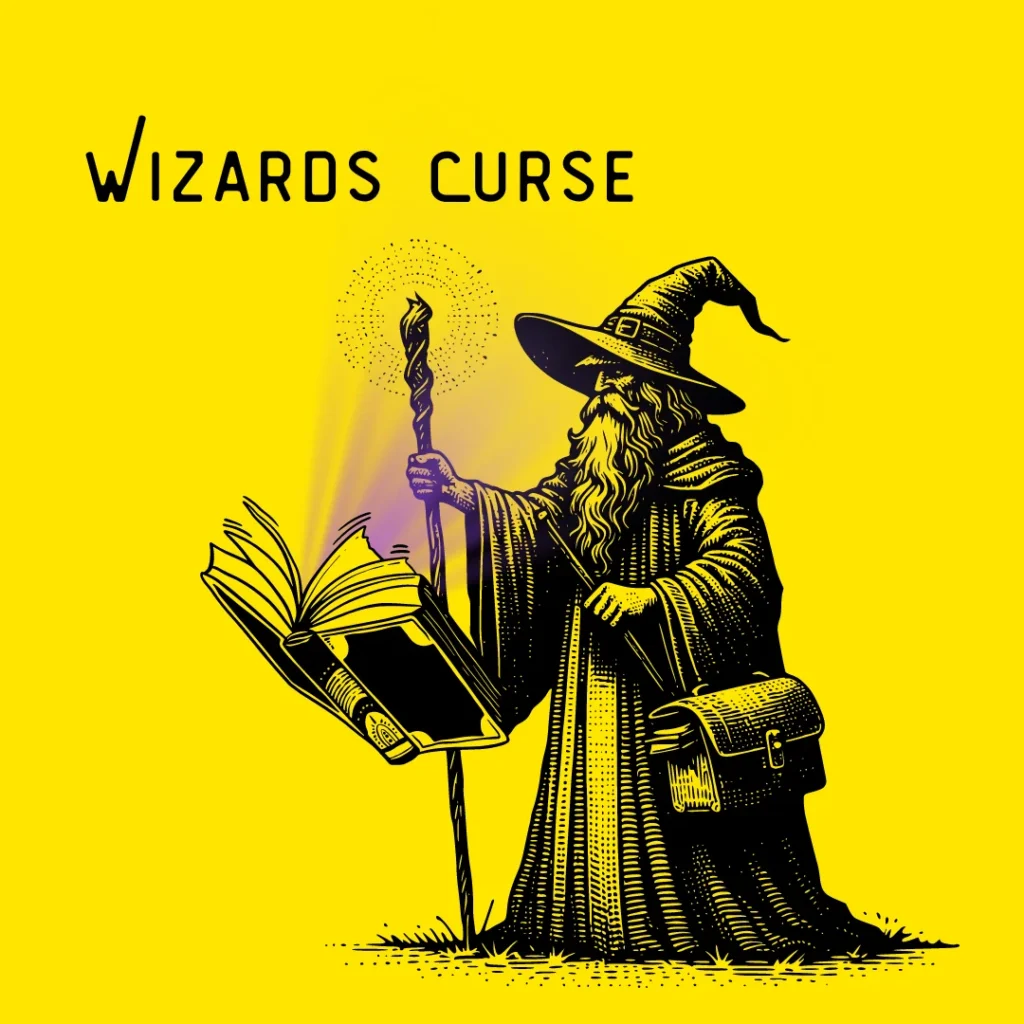
In a magical realm where wizards regularly cast spells, calculating the cost of casting a spell involves several key factors. Each type of spell has a base cost associated with it, and depending on the number of spells, the use of rare runes, the rank of the wizard performing the spell, and whether the spell is for a special occasion, additional charges are applied. Here’s how the pricing works:
Spell Type:
Offensive: $300 base cost.
Defensive: $400 base cost.
Utility: $500 base cost.
Number of Spells:
Few (1-3 spells): Add $50.
Moderate (4-6 spells): Add $100.
Many (7 or more spells): Add $200.
Additional Charges:
Rare Runes: If rare runes are used, add $150 to the total cost.
High-Ranking Wizard: If the spell is cast by a high-ranking wizard, add $200 to the total cost.
Special Occasion: If the spell is cast for a special event or occasion, add $250 to the total cost.
The program you are tasked with building should take these factors into account to calculate the final enchantment cost. The user will input the type of spell, the number of spells, whether rare runes are used, if a high-ranking wizard is involved, and whether the spell is for a special occasion. Based on this information, the program should calculate and display the final cost of the enchantment.
Test Case 1:
Input: Spell Type: Defensive
Number of Spells: Many
Rare Runes: Yes
High-Ranking Wizard: No
Special Occasion: Yes
Expected Output: Final Enchantment Cost: $850
Test Case 2:
Input: Spell Type: Offensive
Number of Spells: Few
Rare Runes: No
High-Ranking Wizard: Yes
Special Occasion: No
Expected Output: Final Enchantment Cost: $500
Explanation:
The program begins by asking the user to input the type of spell they want to cast: either Offensive, Defensive, or Utility. Based on the user’s choice, the program sets the base cost:
- Offensive: $300
- Defensive: $400
- Utility: $500
Next, the user inputs the number of spells they want to cast, and the program adds an extra charge based on this:
- Few spells (1-3): Add $50
- Moderate spells (4-6): Add $100
- Many spells (7 or more): Add $200
Then, the user is asked if rare runes will be used. If the answer is “Yes,” the program adds $150 to the total cost.
The user is also asked if the spell will be performed by a high-ranking wizard. If the answer is “Yes,” an additional $200 is added.
Lastly, if the spell is for a special occasion, another $250 is added to the cost.
After gathering all this information, the program adds up the base cost and all the additional charges, then prints the final enchantment cost to the user.
This step-by-step process ensures that the final cost is tailored to the specific spell type, number of spells, and any extra conditions like rare runes or special occasions.
#include <iostream> #include <string> using namespace std; int main() { string spellType; string numberOfSpells; string rareRunes; string highRankingWizard; string specialOccasion; int baseCost; int finalCost; // Input cout << "Enter spell type (Offensive/Defensive/Utility): "; cin >> spellType; cout << "Enter number of spells (Few/Moderate/Many): "; cin >> numberOfSpells; cout << "Are rare runes used? (Yes/No): "; cin >> rareRunes; cout << "Is a high-ranking wizard performing the spell? (Yes/No): "; cin >> highRankingWizard; cout << "Is the spell for a special occasion? (Yes/No): "; cin >> specialOccasion; // Determine base cost by spell type if (spellType == "Offensive") { baseCost = 300; } else if (spellType == "Defensive") { baseCost = 400; } else if (spellType == "Utility") { baseCost = 500; } else { cout << "Invalid spell type." << endl; return 1; // Exit the program for invalid input } finalCost = baseCost; // Adjust cost based on the number of spells if (numberOfSpells == "Few") { finalCost += 50; } else if (numberOfSpells == "Moderate") { finalCost += 100; } else if (numberOfSpells == "Many") { finalCost += 200; } else { cout << "Invalid number of spells." << endl; return 1; // Exit the program for invalid input } // Add cost for rare runes if (rareRunes == "Yes") { finalCost += 150; } // Add cost for high-ranking wizard if (highRankingWizard == "Yes") { finalCost += 200; } // Add cost for special occasion if (specialOccasion == "Yes") { finalCost += 250; } // Display final cost cout << "Final Enchantment Cost: $" << finalCost << endl; return 0; }
Magical Creatures
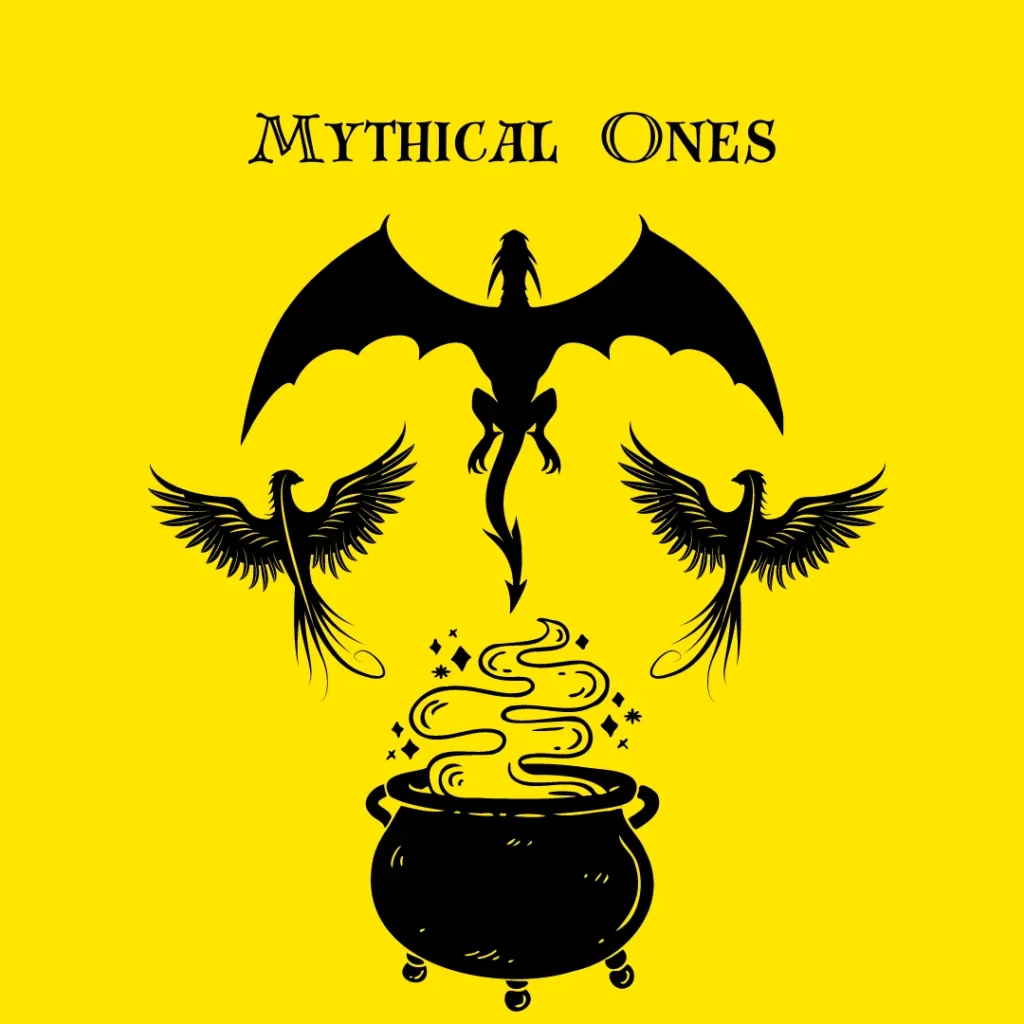
You are tasked with designing a program to calculate the total cost of setting up a magical shop for mystical creatures. The base cost for establishing the shop is $400, but additional charges will depend on several factors, such as the type of creature, the number of creatures, special habitat requirements, whether the shop is located in a mystical forest, and if the creatures are classified as endangered.
The cost of the shop is influenced by these elements:
Creature Type:
Dragons add $300 to the base cost.
Unicorns add $200 to the base cost.
Phoenixes add $150 to the base cost.
Number of Creatures:
Few (1-3 creatures) adds $100.
Several (4-6 creatures) adds $200.
Many (7 or more creatures) adds $300.
Special Habitats: If special habitats are required, it adds $250.
Mystical Forest: If the shop includes a mystical forest, it adds $100.
Endangered Creatures: If the creatures are endangered, it adds $400.
Your task is to create a program that takes inputs for the creature type, the number of creatures, and other features (such as special habitats, mystical forest, and endangered status) to compute and display the total cost of setting up the shop.
Test Case 1
Inputs:
Creature Type: Unicorns
Number of Creatures: Several
Special Habitats: Yes
Mystical Forest: No
Endangered: Yes
Expected Output:
Final Shop Expenses: $1150
Test Case 2
Inputs:
Creature Type: Dragons
Number of Creatures: Few
Special Habitats: No
Mystical Forest: Yes
Endangered: No
Expected Output:
Final Shop Expenses: $800
Explanation:
The program starts by initializing the base cost of $400. It then prompts the user to input key details, including the creature type, number of creatures, and additional features such as special habitats, mystical forest, and whether the creatures are endangered.
How the calculations work:
- The creature type is checked first, and depending on whether the creature is a Dragon, Unicorn, or Phoenix, the program adds an extra amount ($300, $200, or $150) to the base cost.
- The program then checks the number of creatures. For a few creatures, $100 is added. For several creatures, $200 is added, and for many creatures, $300 is added.
- If special habitats are required, the cost is increased by $250.
- If a mystical forest is included, an additional $100 is added.
- Lastly, if the creatures are endangered, the program adds $400 to the cost.
After adjusting for all these factors, the program computes the final cost by summing the base cost and the additional charges and then displays the result.
#include <iostream> #include <string> using namespace std; int main() { string creatureType; string numberOfCreatures; string specialHabitats; string mysticalForest; string endangered; // Initialize the base cost int baseCost = 400; int finalCost = baseCost; // Get inputs cout << "Enter Creature Type (Dragon, Unicorn, Phoenix): "; cin >> creatureType; cout << "Enter Number of Creatures (Few, Several, Many): "; cin >> numberOfCreatures; cout << "Special Habitats Required? (Yes/No): "; cin >> specialHabitats; cout << "Mystical Forest Included? (Yes/No): "; cin >> mysticalForest; cout << "Are the Creatures Endangered? (Yes/No): "; cin >> endangered; // Adjust cost based on creature type if (creatureType == "Dragon") { finalCost += 300; } else if (creatureType == "Unicorn") { finalCost += 200; } else if (creatureType == "Phoenix") { finalCost += 150; } // Adjust cost based on number of creatures if (numberOfCreatures == "Few") { finalCost += 100; } else if (numberOfCreatures == "Several") { finalCost += 200; } else if (numberOfCreatures == "Many") { finalCost += 300; } // Adjust cost for special habitats if (specialHabitats == "Yes") { finalCost += 250; } // Adjust cost for mystical forest if (mysticalForest == "Yes") { finalCost += 100; } // Adjust cost for endangered creatures if (endangered == "Yes") { finalCost += 400; } // Output the final cost cout << "Final Shop Expenses: $" << finalCost << endl; return 0; }
Adventure Park Ticket Pricing
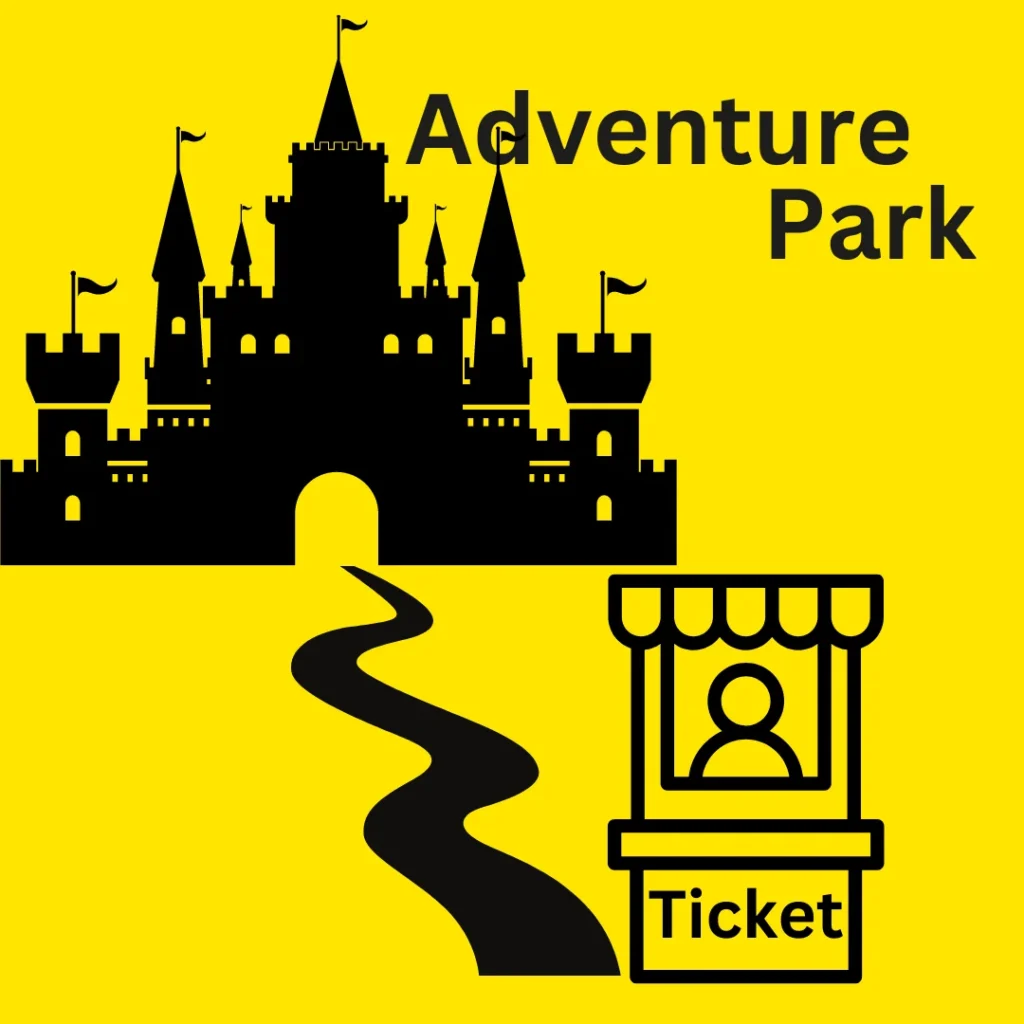
You’re planning a day at an amusement park and need to calculate the total cost of tickets. The park offers different ticket types: Regular, Fast-Pass, and Group, each with its own price adjustment. Additionally, the price can vary depending on the time of day (Peak or Off-Peak), whether it’s a Weekend or Weekday, and whether you purchase tickets for multiple attractions. The base price for each ticket is $100, and several factors will adjust this price to determine the final amount you need to pay.
The ticket pricing is determined as follows:
Ticket Type:
Regular: No change (remains $100).
Fast-Pass: Adds $30 to the base price.
Group: Subtracts $20 from the base price.
Time of Day:
Peak: Adds $20.
Off-Peak: No change.
Day of Visit:
Weekend: Adds $15.
Weekday: No change.
Multi-Attraction Purchase:
Yes: Subtracts $25.
No: No change.
Your task is to write a program that calculates the final ticket price by considering these factors.
Test Case 1
Inputs:
Ticket Type: Fast-Pass
Time of Day: Peak
Day: Weekend
Multi-Attraction Purchase: Yes
Expected Output:
Final Ticket Price: $120
Test Case 2
Inputs:
Ticket Type: Group
Time of Day: Off-Peak
Day: Weekday
Multi-Attraction Purchase: No
Expected Output:
Final Ticket Price: $80
Explanation:
The program begins with a base ticket price of $100 and adjusts this price based on several factors such as the type of ticket, the time of day, the day of the week, and whether multiple attractions are included in the purchase.
- The ticket type determines the first price adjustment:
- A Fast-Pass adds $30 to the base price.
- A Group ticket subtracts $20.
- A Regular ticket leaves the price unchanged.
- The time of day is then checked:
- If it’s Peak time, $20 is added to the price.
- If it’s Off-Peak, no additional cost is added.
- The day of visit further adjusts the price:
- If it’s a Weekend, an extra $15 is added.
- For Weekdays, there is no extra charge.
- Finally, if the purchase includes multiple attractions, a discount of $25 is applied.
The program sums up all these adjustments and displays the final ticket price to the user.
#include <iostream> #include <string> using namespace std; int main() { string ticketType; string timeOfDay; string day; string multiAttractionPurchase; // Initialize base price int basePrice = 100; int finalPrice = basePrice; // Input for ticket type cout << "Enter Ticket Type (Regular, Fast-Pass, Group): "; cin >> ticketType; // Input for time of day cout << "Enter Time of Day (Peak, Off-Peak): "; cin >> timeOfDay; // Input for day cout << "Enter Day (Weekend, Weekday): "; cin >> day; // Input for multi-attraction purchase cout << "Multiple Attraction Purchase (Yes/No): "; cin >> multiAttractionPurchase; // Adjust price based on ticket type if (ticketType == "Fast-Pass") { finalPrice += 30; } else if (ticketType == "Group") { finalPrice -= 20; } // Adjust price based on time of day if (timeOfDay == "Peak") { finalPrice += 20; } // Adjust price based on day of visit if (day == "Weekend") { finalPrice += 15; } // Adjust price based on multi-attraction purchase if (multiAttractionPurchase == "Yes") { finalPrice -= 25; } // Output the final ticket price cout << "Final Ticket Price: $" << finalPrice << endl; return 0; }
Hotel Reservation System
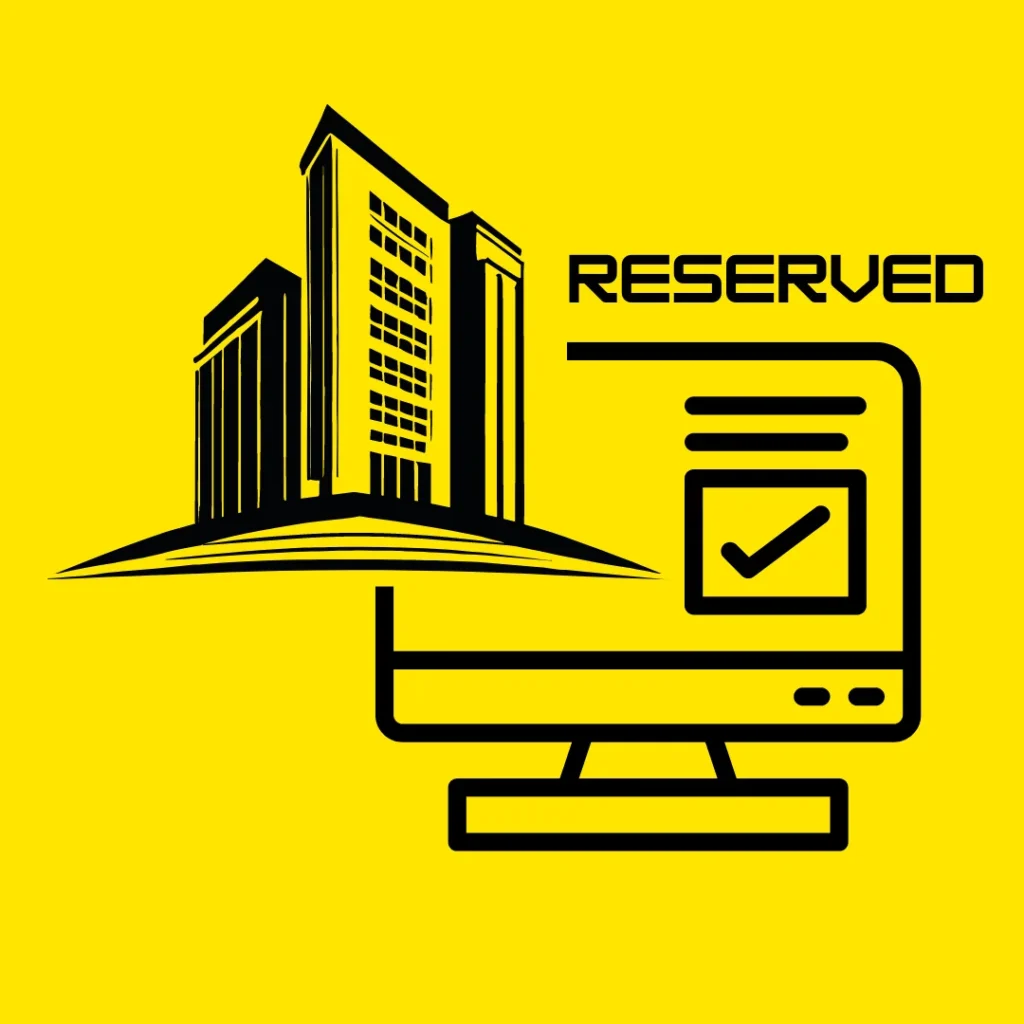
You’re managing a hotel reservation system that needs to calculate the final room cost based on several factors. The hotel offers different room types—Standard, Deluxe, and Suite—each with its own base price. The total price is adjusted based on the time of year (season), the guest’s loyalty status, the duration of stay, whether the reservation is for a weekend or weekday, and the selected meal plan. Guests can also opt for additional services such as spa treatments or guided tours, which add a flat fee to the total cost.
The price adjustments are as follows:
Room Type:
Standard: $150 per night.
Deluxe: $250 per night.
Suite: $400 per night.
Season:
Off-Season: Subtract $50 per night.
Peak: No change.
Loyalty Status:
Loyal: Subtract $30 per night.
New: No change.
Duration of Stay:
If the stay is more than 5 nights, subtract $100 from the total.
Day of Reservation:
Weekend: Add $40 per night.
Weekday: No change.
Meal Plan:
None: No change.
Breakfast: Add $20 per night.
Full-Board: Add $50 per night.
Additional Services:
Yes: Add a flat fee of $150.
No: No change.
Test Case 1
Inputs:
Room Type: Deluxe
Season: Peak
Loyalty Status: Yes
Stay Duration: 5 nights
Day of Reservation: Weekend
Meal Plan: Full-Board
Additional Services: Yes
Expected Output:
Final Room Cost: $960
Test Case 2
Inputs:
Room Type: Standard
Season: Off-Season
Loyalty Status: No
Stay Duration: 3 nights
Day of Reservation: Weekday
Meal Plan: Breakfast Only
Additional Services: No
Expected Output:
Final Room Cost: $375
Explanation:
The program calculates the final cost of a hotel room based on several factors: room type, season, loyalty status, stay duration, day of the week, meal plan, and additional services. Here’s how it works:
- Base Price Calculation: The cost starts with a base price depending on the room type. For “Standard” rooms, it’s $150 per night; “Deluxe” rooms cost $250 per night; and “Suite” rooms are $400 per night. This base price is multiplied by the number of nights to get the initial cost.
- Season Adjustment: If the reservation is made for the off-season, $50 is deducted from the total cost for each night to reflect lower prices during this time.
- Loyalty Discount: Guests with loyalty status receive a discount of $30 per night, reducing the total cost further.
- Length of Stay Discount: If the stay exceeds 5 nights, an additional discount of $100 is applied to the total cost to reward longer stays.
- Weekend Surcharge: For stays that include weekends, an extra $40 per night is added to the cost due to higher demand during these times.
- Meal Plan Costs: The choice of meal plan affects the total cost. “Breakfast” adds $20 per night, while “Full-Board” (which includes all meals) adds $50 per night.
- Additional Services Fee: If the guest opts for extra services, such as spa treatments or guided tours, a flat fee of $150 is added to the final cost.
By applying all these factors, the program calculates the total amount the guest needs to pay and displays this final room cost.
#include <iostream> #include <string> using namespace std; int main() { // Input values string roomType = "Deluxe"; // Room Type: Standard, Deluxe, Suite string season = "Peak"; // Season: Off-Season, Peak string loyaltyStatus = "Yes"; // Loyalty Status: Yes, No string dayType = "Weekend"; // Day: Weekday, Weekend string mealPlan = "Full-Board"; // Meal Plan: None, Breakfast, Full-Board int nights = 5; // Number of nights bool additionalServices = true; // Additional services: Yes/No // Initialize base price based on room type int basePrice = 0; if (roomType == "Standard") { basePrice = 150; } else if (roomType == "Deluxe") { basePrice = 250; } else if (roomType == "Suite") { basePrice = 400; } // Calculate initial price (base price * number of nights) int finalPrice = basePrice * nights; // Apply season discount for Off-Season if (season == "Off-Season") { finalPrice -= 50 * nights; } // Apply loyalty discount for loyal customers if (loyaltyStatus == "Yes") { finalPrice -= 30 * nights; } // Apply long stay discount (more than 5 nights) if (nights > 5) { finalPrice -= 100; } // Apply weekend surcharge if (dayType == "Weekend") { finalPrice += 40 * nights; } // Apply meal plan cost if (mealPlan == "Breakfast") { finalPrice += 20 * nights; } else if (mealPlan == "Full-Board") { finalPrice += 50 * nights; } // Add cost for additional services if (additionalServices) { finalPrice += 150; } // Display the final room cost cout << "Final Room Cost: $" << finalPrice << endl; return 0; }
Travel Booking System
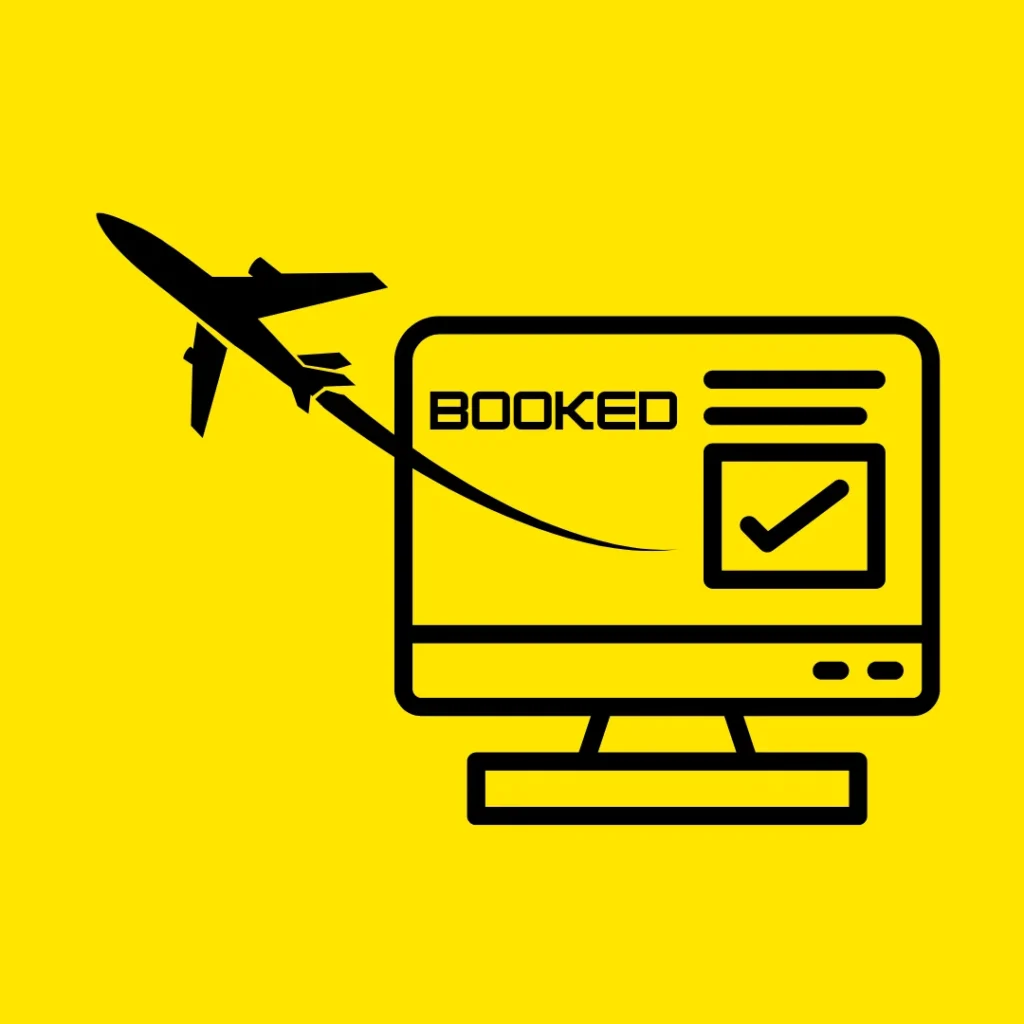
You are tasked with calculating the total cost of a travel package offered by an agency. The cost varies based on several factors including the destination type, the season, additional services, and loyalty membership. The base price of the travel package is $500. Here are the adjustments:
Destination Type:
Domestic: No additional cost
International: +$300
Season:
Peak: +$100
Off-Peak: No additional cost
Additional Services:
Guided Tours: +$150
Luggage Handling: +$50
Loyalty Membership:
Loyalty Member: -$100 discount
Non-Member: No discount
Your goal is to compute the final cost of the package by applying these conditions.
Test Case 1:
Inputs: Destination Type: International
Season: Peak
Guided Tours: Yes
Luggage Handling: Yes
Loyalty Member: Yes
Expected Output: Final Travel Package Cost: $1000
Test Case 2:
Inputs: Destination Type: Domestic
Season: Off-Peak
Guided Tours: No
Luggage Handling: No
Loyalty Member: No
Expected Output: Final Travel Package Cost: $500
Explanation:
Initialize Base Cost: The program starts with a base cost of $500 for the travel package.
- If the destination is “Domestic,” the cost remains at $500.
- If the destination is “International,” an additional $300 is added to the base cost, making the cost $800 for international destinations.
- If the season is “Peak,” $100 is added to the current total cost.
- If the season is “Off-Peak,” there is no additional charge.
- If guided tours are selected, an additional $150 is added.
- If luggage handling is chosen, an additional $50 is added.
- If the traveler is a loyalty member, a discount of $100 is subtracted from the total cost.
- If the traveler is not a loyalty member, no discount is applied.
- The final cost is computed by summing the base cost, adjustments for destination and season, costs for additional services, and applying the loyalty discount.
- The final cost is then displayed to the user.
#include <iostream> #include <string> using namespace std; int main() { string destinationType = "International"; // Options: "Domestic", "International" string season = "Peak"; // Options: "Peak", "Off-Peak" string guidedTours = "Yes"; // Options: "Yes", "No" string luggageHandling = "No"; // Options: "Yes", "No" string loyaltyMember = "Yes"; // Options: "Yes", "No" int baseCost = 500; int finalCost; // Calculate cost based on destination if (destinationType == "Domestic") { finalCost = baseCost; } else if (destinationType == "International") { finalCost = baseCost + 300; } // Adjust for season if (season == "Peak") { finalCost += 100; } // Additional services if (guidedTours == "Yes") { finalCost += 150; } if (luggageHandling == "Yes") { finalCost += 50; } // Loyalty discount if (loyaltyMember == "Yes") { finalCost -= 100; } cout << "Final Travel Package Cost: $" << finalCost << endl; return 0; }
To really get the concept of using AND and OR to combine multiple conditions in if statements, you need to apply them to real-life situations. That’s what these scenarios are all about—taking something complex and making it practical and engaging. At Syntax Scenarios, we believe in making coding fun and interactive. By working through these problems, you’ll improve your coding skills and start thinking like a programmer.