In this tutorial, you will learn how to take input from the user using scanf()
and display the output on the screen using printf()
in C.
Table of Contents
- Input/ Output Streams in C
- Display a Message on Screen Using printf() in C
- Format Specifiers in C
- Display a Variable Using printf() in C
- Display Multiple Variables Using a Single printf() in C
- Input Value from User Using scanf()
- Input Multiple Values from the User in a Single Line in C
- Common Mistakes that Programmers Make in Input/ Output in C
- Conclusion
- FAQs
Input/ Output Streams in C
Input/ Output seems a very easy going process on the front-end, however behind that abstraction, at the back a lot more is going on. That’s where the concept of streams comes in. They are like real-world streams that helps the data to flow in and out of the program.
Display a Message on Screen Using printf() in C
The printf()
function stands for print formatted. It is a part of <stdio.h>
library that is used to display output on the screen in C language,
The printf()
function utilizes the standard output stream (stdout) to send the formatted output from your program to the standard output device i-e monitor. When you call printf()
, it takes the formatted string and the values you want to display. Then it writes that information to the standard output stream, which ultimately displays the message on the console or terminal window.
Syntax
printf(“ Your Message ");
Example Code
#include <stdio.h> int main() { // Printing a simple string printf("Hello, world!"); return 0; }
Output
Hello, World!
Format Specifiers in C
You looked at the basics of printf()
. However, the true power of printf()
comes from the format specifiers. Format specifiers are special placeholders within the string that tell printf()
the type of value you will display and where to display the values you want.
A simple way to understand this is through the example of making a cake. When you’re making a cake, you use measuring spoons to add the ingredients according to the required amount. If you use the wrong amount of ingredients using the wrong spoon size then the result will not be according to your expectations. Similarly, the format specifiers are the measuring spoons just like each data type has its own size. You need to use the correct one to get the right results. Otherwise, you will get runtime or logical errors.
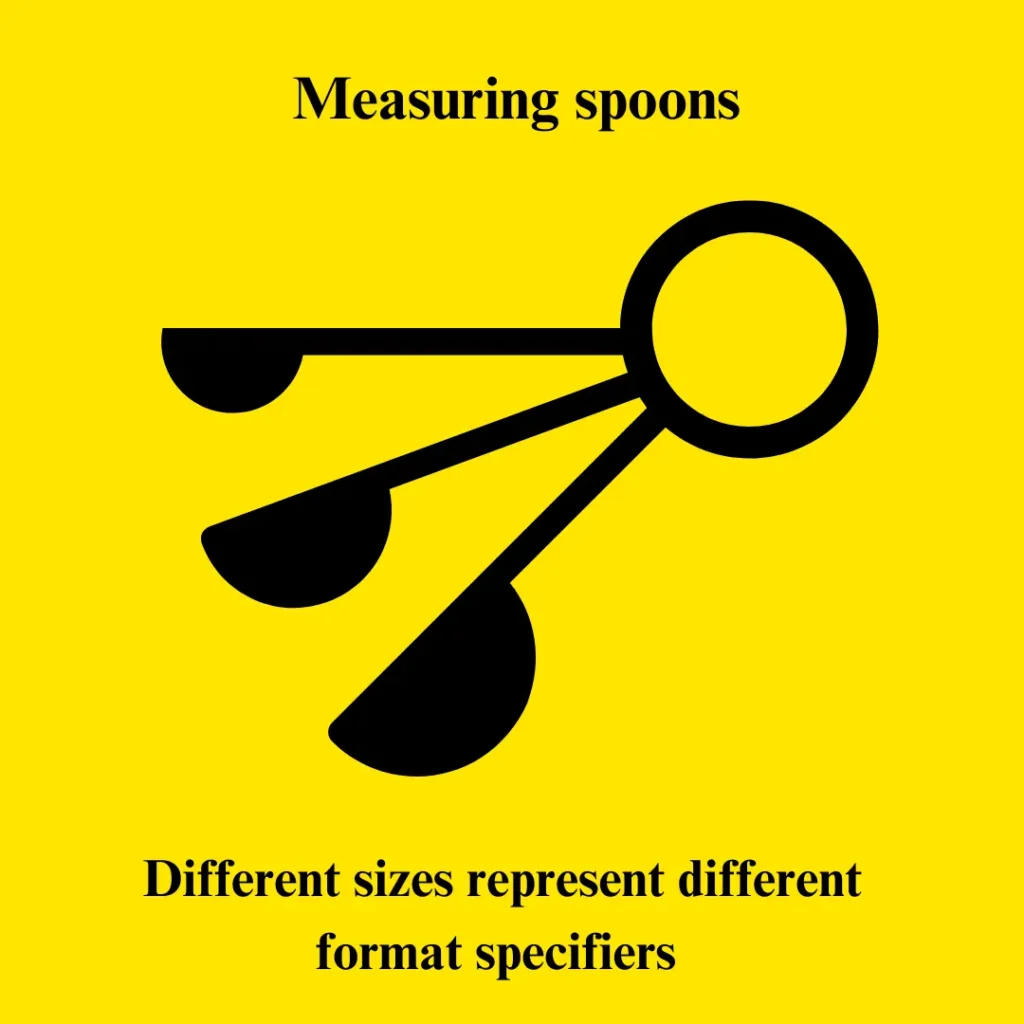
The below table lists the format specifiers for all the data types.
Data Type | Format Specifier |
Int | %d |
Char | %c |
Float | %f |
Double | %lf |
Short int | %hd |
Unsigned int | %u |
Long int | %li |
Long long int | %lli |
Unsigned long int | %lu |
Unsigned long long int | %llu |
Signed char | %c |
Unsigned char | %c |
Long double | %lf |
Display a Variable Using printf() in C
Now that you are clear about format specifiers, let’s print different variables using printf()
with format specifiers. There are multiple data types in the C language. In order to display them you should modify the original syntax of displaying a simple string. Now, you will use the format specifiers in the printf(). The format specifier is a placeholder in the string and is replaced by the value of that variable at runtime.
Let’s display some of the commonly used data types.
Display an Integer
To display an integer variable, you will use the %d
format specifier.
Syntax
int x = value in integers; printf(“ your message %d.", x);
Display a Floating-point Number (float / double)
To display a floating-point number (such as a float or double), you will use the %f format specifier. You can also specify the number of decimal places to display using the format %.<number of decimal places>f
. You can use %f
or %lf
for double when using print()
.
Syntax
double x = value in decimal; printf(“ your message %f.", x);
Display a characters
The %c
format specifier displays a single character, such as letters, digits, or other symbols.
Syntax
char x = ‘a single character’; printf("The letter is %c.", x);
Display Multiple Variables Using a Single printf() in C
To display multiple variables, you can simply add more format specifiers and variable names separated by commas in the printf()
statement. You can display them either in a single line or in multiple lines in the output using the \n
escape sequence.
Example code
#include <stdio.h> int main() { int x = 10; double y = 3.14; char z = 'A'; printf("Int x: %d, Double y: %lf, Char z: %c", x, y, z); printf("\nInt x: %d\nDouble y: %f\nChar z: %c\n", x, y, z); return 0; }
Output
Int x: 10, Double y: 3.140000, Char z: A Int x: 10 Double y: 3.140000 Char z: A
Input Value from User Using scanf()
The scanf()
function is a part of the standard library <stdio.h>
in C programming that takes input from the user into your program and stores it in variables. When you call scanf()
, you provide it with a format string that tells scanf()
how to translate the input provided by the user.
The scanf()
function uses the standard input stream, to read the user’s input from the standard input device i-e keyboard. The format specifier specifies the type of data to be read, and scanf()
will then parse the input accordingly and store the values in the corresponding variables.
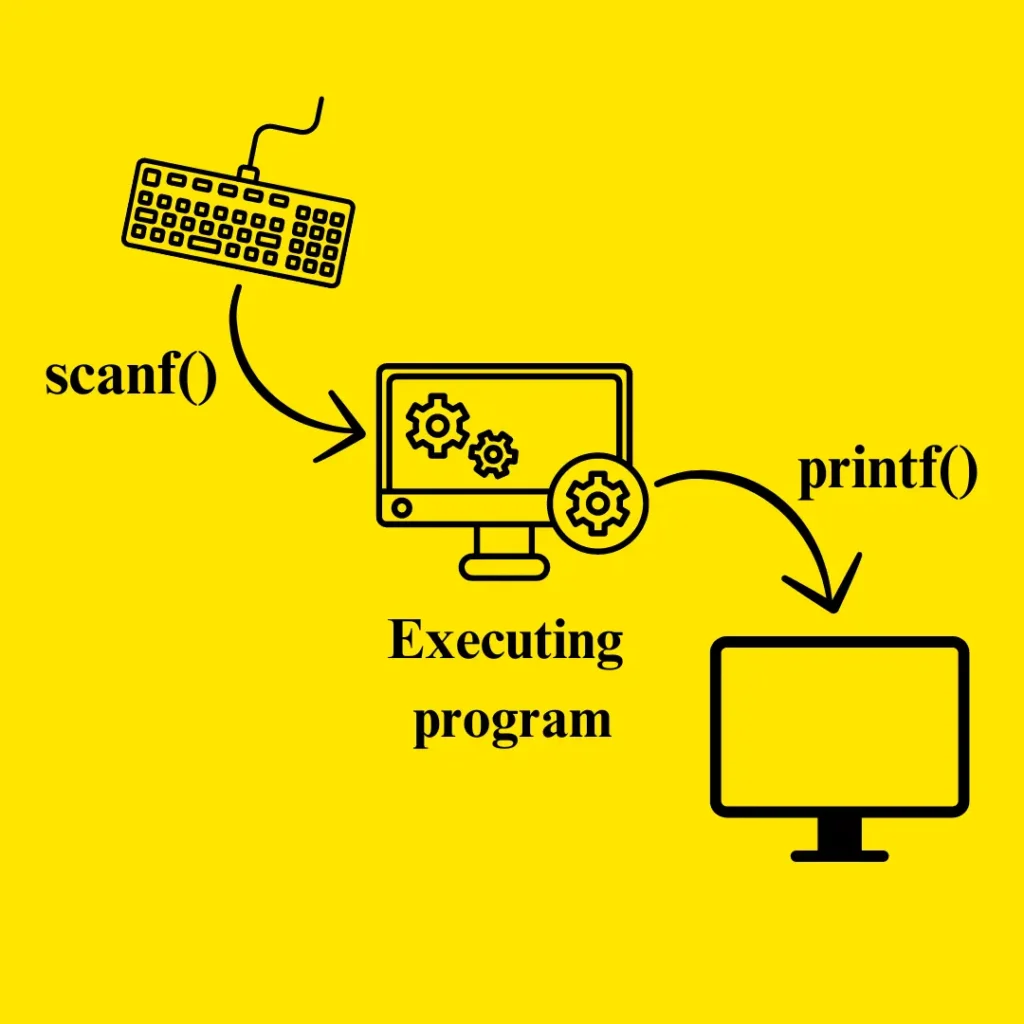
However, there’s a key difference between printf()
and scanf()
. With scanf()
, you need to provide the address of the variable where you want to store the input. This is done using the address-of operator &
, which gives scanf()
the memory location to directly store or update the variable’s value. Actually, there lies the concept of pass-by reference which you will learn later on.
Input an Integer
To input an integer, use the %d
format specifier in scanf()
.
int x; scanf("%d", &x);
Input a Float Number
In case of a float, use the %f
format specifier with scanf()
. However, when you input a double, use the %lf
.
float f; scanf("%f", &f);
Input a Character
When you input a character, use the %c
format specifier.
char x; scanf("%c", &x);
Now let’s input all the types in a single program.
Example code
#include <stdio.h> int main() { int age; char gender; double height; printf("Enter your age: "); scanf("%d", &age); printf("Enter your gender (M/F): "); scanf(" %c", &gender); // Note the space before %c to skip any whitespace printf("Enter your height (in meters): "); scanf("%lf", &height); printf("\nYour information:\n"); printf("Age: %d\n", age); printf("Gender: %c\n", gender); printf("Height: %.2lf meters\n", height); return 0; }
In this program, you have three variables:
- age (an integer to store the user’s age)
- gender (a character to store the user’s gender)
- height (a double to store the user’s height in meters)
The program prompts the user to enter values for each of these variables using scanf() and the appropriate format specifiers (%d
for integer, %c
for character, %lf
for double). After the user has entered all three values, the program displays the information back to the user using printf() statements.
Output
Enter your age: 20 Enter your gender (M/F): F Enter your height (in meters): 1.7 Your information: Age: 20 Gender: F Height: 1.70 meters
Input Multiple Values from the User in a Single Line in C
To take multiple inputs from the user in a single line using scanf()
, you can provide multiple format specifiers in the format string, separated by spaces. Then you should enter the values separated by spaces, and they will be stored in their corresponding variables.
Example code
#include <stdio.h> int main() { int x; double y; char z; printf("Enter an integer, a floating-point number, and a character: "); scanf("%d %lf %c", &x, &y, &z); printf("You entered:\n"); printf("Integer: %d\n", x); printf("Floating-point: %f\n", y); printf("Character: %c\n", z); return 0; }
Output
Enter an integer, a floating-point number, and a character: 2 2.5 f You entered: Integer: 2 Floating-point: 2.500000 Character: f
Common Mistakes that Programmers Make in Input/ Output in C
A common mistake that new programmers make is that they use a different format specifier for a data type in print() or scanf(). It sometimes causes logical or runtime errors. A real-world example of this case is like reaching into labeled jars in a kitchen cabinet. You need to use the correct format specifier to match the type of data, just like using the right label to access the contents of a specific jar. By using the appropriate format specifiers, you can ensure values are stored in the correct format, maintaining the integrity of your data and avoiding issues in your C code, similar to keeping a well-organized kitchen cabinet.
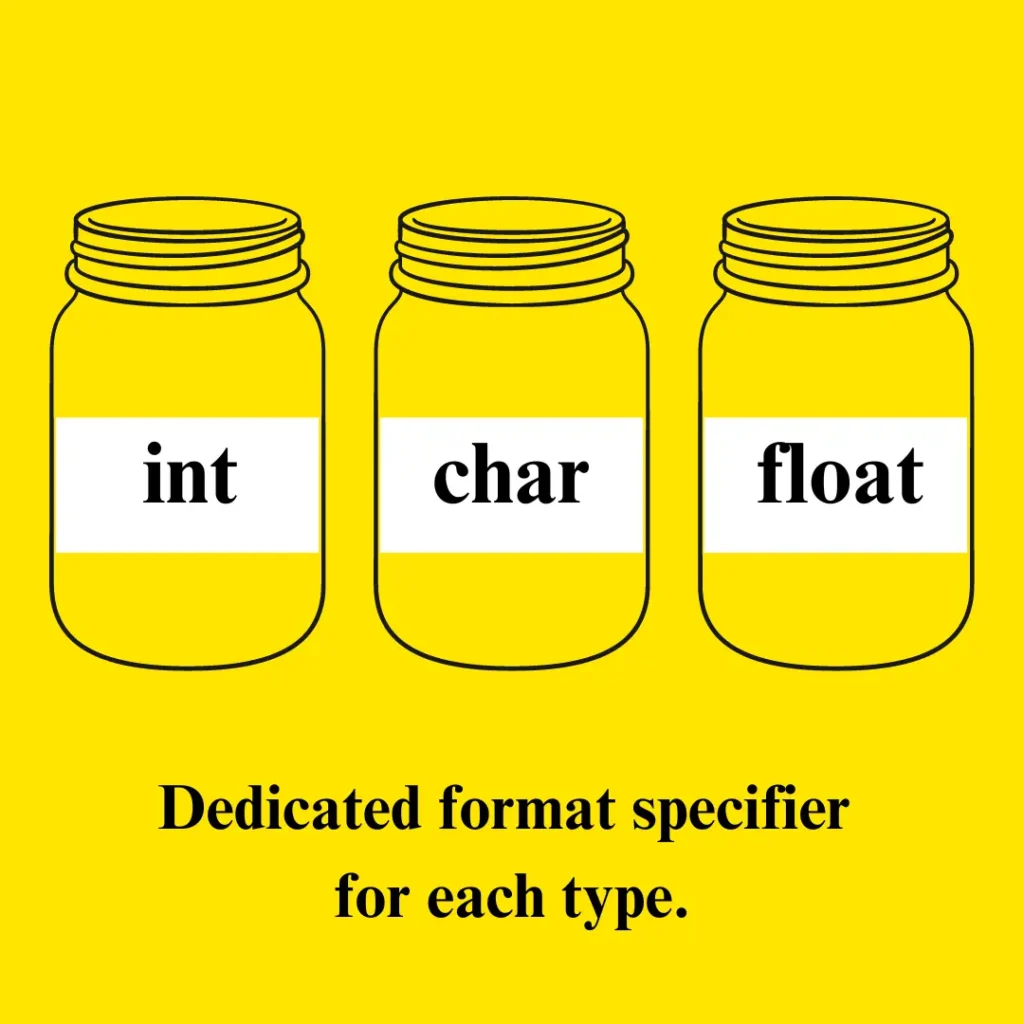
Another common mistake is, that users forget to put the address-of operator %
in scanf()
that causes run-time error or logical error sometimes. Remember that scanf()
always works with addresses so you need to put the address-of operator with the variable name in scanf()
.
Conclusion
To sum up you covered user input and output in C in detail with visuals and real-world examples, including the header file, stdio.h, the printf() and scanf() functions with format specifiers to display output and receive user input, respectively. Understanding these fundamental input/output operations is the base for creating interactive C programs.
FAQs
What is the purpose of printf()
in C programming?
The printf()
is a function that is used to display formatted output on the screen. It takes a string and format specifiers to print messages or variable values to the console.
What are format specifiers in C, and why are they important?
Format specifiers are placeholders used in printf()
and scanf()
to indicate the type of data being handled (e.g., %d
for integers, %f
for floats). They ensure the correct data is processed and displayed.
How can I use scanf()
to take user input in C?
The scanf()
is a function that is used to read input from the user. You provide a format specifier that matches the variable type and use the address-of operator &
to store the input in a variable.
What are common mistakes to avoid when using scanf()
and printf()
?
Common mistakes include using incorrect format specifiers for variables and forgetting to use the address-of operator &
in scanf()
. These errors can cause logical or runtime issues.
How do you display multiple variables in a single printf()
statement in C?
To display multiple variables, include multiple format specifiers in the printf()
string and list the variables separated by commas, like printf("Int: %d, Float: %f", intVar, floatVar);
.