Explore a collection of interesting C programming challenges focused on structures with real-world scenarios. From managing a car showroom to tracking airline flights and organizing a smart parking system, these problems make learning fun and practical. Whether you’re a beginner or someone looking to sharpen your coding skills, these structured exercises will help you understand how to work with structures in C in a simple and engaging way
Car Showroom Manager
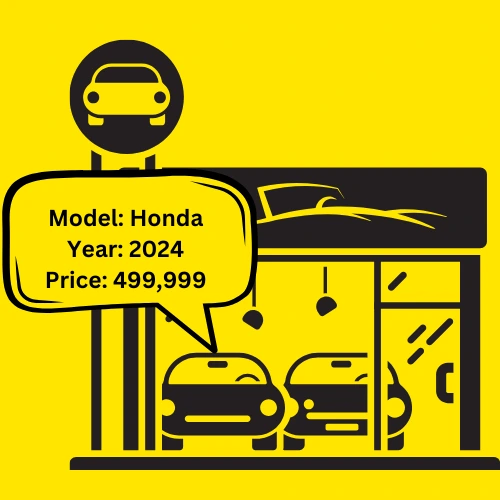
Imagine that you work in a car showroom, and you need to manage details of cars available for sale. Each car has a model name, a year of manufacture, and a price. Write a C program that takes car details as input and displays them in a structured format.
Test Case
Input
Enter car model (single word): Honda
Enter year of manufacture: 2024
Enter car price: 49999.99
Expected Output
Car Details
Model: Honda
Year: 2024
Price: $49999.99
- The program starts by defining a
struct Car
, which includes three fields:model
: A character array to store the car model name.year
: An integer to store the manufacturing year.price
: A float to store the car’s price.
- The program prompts the user to enter the model name, manufacturing year, and price.
- Finally, the program displays the car model, manufacturing year, and price in a structured format.
#include <stdio.h> struct Car { char model[50]; int year; float price; }; int main() { struct Car c; printf("Enter car model (single word): "); scanf("%s", c.model); printf("Enter year of manufacture: "); scanf("%d", &c.year); printf("Enter car price: "); scanf("%f", &c.price); printf("\nCar Details\n"); printf("Model: %s\nYear: %d\nPrice: $%.2f\n", c.model, c.year, c.price); return 0; }
Student Report Card
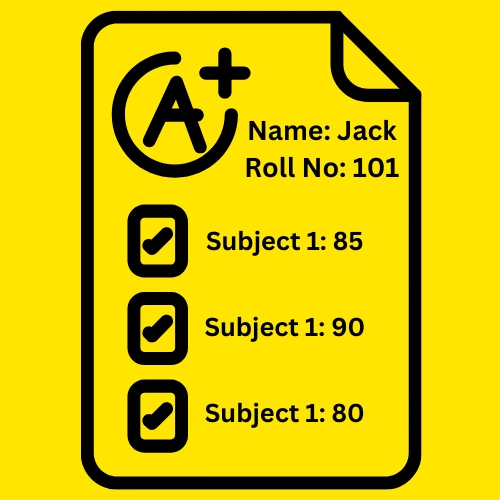
Imagine that you are a teacher in a school, and you need to generate report cards for your students. Each student has a name, a roll number, and marks in three subjects. Your goal is to calculate the student’s average marks and display their report card in a readable format. Write a C program that takes a student’s details as input and prints their report card along with their average marks.
Test Case
Input
Enter student name: Jack
Enter roll number: 101
Enter marks in 3 subjects: 85 90 80
Expected Output
Student Report Card
Name: Jack
Roll Number: 101
Average Marks: 85.00
- The program starts by defining a structure
Student
with three fields:name
(character array) to store the student’s name.roll_no
(integer) to store the roll number.marks
(array of three integers) to store marks in three subjects.
- The program prompts the user to enter the student’s name, roll number, and marks in three subjects. These inputs are stored in the respective structure variables.
- The program calculates the total marks by adding all three subject marks inside a
for
loop. - The average marks are computed by dividing the total marks by
3.0
to ensure floating-point division. - The program then displays the student’s
name
,roll
_number
, and averagemarks
in a structured report card format.
#include <stdio.h> struct Student { char name[50]; int roll_no; int marks[3]; }; int main() { struct Student s; int sum = 0; printf("Enter student name: "); scanf("%s", s.name); printf("Enter roll number: "); scanf("%d", &s.roll_no); printf("Enter marks in 3 subjects: "); for (int i = 0; i < 3; i++) { scanf("%d", &s.marks[i]); sum += s.marks[i]; } float average = sum / 3.0; printf("\nStudent Report Card\n"); printf("Name: %s\nRoll Number: %d\n", s.name, s.roll_no); printf("Average Marks: %.2f\n", average); return 0; }
Employee Salary Calculator
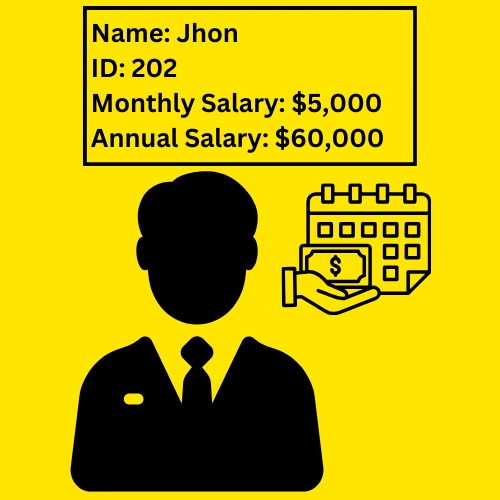
Imagine that you are working in the HR department of a company, and you need to calculate the annual salary of an employee based on their monthly salary. Each employee has a name, an employee ID, and a monthly salary. Write a C program that takes employee details as input, calculates their annual salary, and prints it in a structured format.
Test Case
Input
Enter employee name: Jhon
Enter employee ID: 202
Enter monthly salary: 5000
Expected Output
Employee Details
Name: Jhon
Employee ID: 202
Annual Salary: 60000.00
- The program starts by defining a structure
Employee
with three fields:name
(character array) to store the employee’s name.emp_id
(integer) to store the employee’s ID.salary
(float) to store the monthly salary.
- The program prompts the user to enter the employee’s name, ID, and monthly salary. These values are stored in the structure.
- The
annual_salary
is calculated by multiplying the monthlysalary
by12
. - The program then displays the employee’s name, ID, and annual salary in a structured format.
#include <stdio.h> struct Employee { char name[50]; int emp_id; float salary; }; int main() { struct Employee e; printf("Enter employee name: "); scanf("%s", e.name); printf("Enter employee ID: "); scanf("%d", &e.emp_id); printf("Enter monthly salary: "); scanf("%f", &e.salary); float annual_salary = e.salary * 12; printf("\nEmployee Details\n"); printf("Name: %s\nEmployee ID: %d\n", e.name, e.emp_id); printf("Annual Salary: %.2f\n", annual_salary); return 0; }
Library Book Management
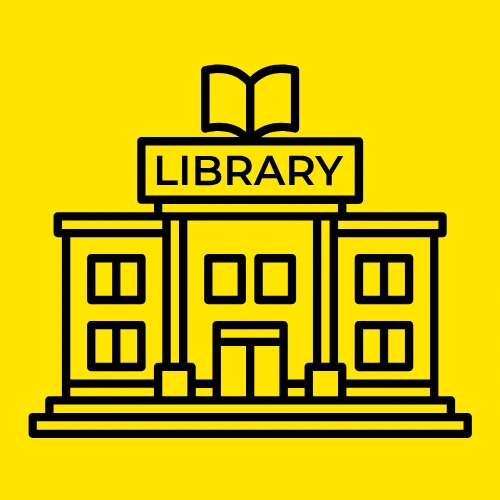
You are managing a small community library. The library keeps records of books, including their title, author, publication year, and availability status. Your task is to write a program in C language that allows users to enter book details and then check all the available books.
Test Case
Input
Enter the number of books: 2
Enter details for book 1:
Title: Gatsby
Author: Fitzgerald
Year of Publication: 1925
Is the book available? (1 for Yes, 0 for No): 1
Enter details for book 2:
Title: Night
Author: Weisel
Year of Publication: 2004
Is the book available? (1 for Yes, 0 for No): 0
Expected Output
Available Books:
Title: Gatsby, Author: Fitzgerald, Year: 1925
- The program starts by asking the user to enter the number of books. This input is stored in the variable
n
. - A structure
Book
is defined to store the book details:title
,author
,year
, andisAvailable
. - The program then uses a
for
loop to collect details for each book. - The user enters the book title, which is stored in
books[i].title
. - The user enters the author’s name, which is stored in
books[i].author
. - The publication year is entered and stored in
books[i].year
. - The user specifies if the book is available (
1
for Yes,0
for No), stored inbooks[i].isAvailable
. - After collecting all the book details, the program displays the list of available books.
- Another
for
loop iterates through all the books and checks ifbooks[i].isAvailable
is 1. - If the book is available, the program prints its title, author, and year of publication.
- The program ends after displaying all available books.
#include <stdio.h> struct Book { char title[50]; char author[50]; int year; int isAvailable; // 1 for available, 0 for not available }; int main() { int n; printf("Enter the number of books: "); scanf("%d", &n); struct Book books[n]; for (int i = 0; i < n; i++) { printf("\nEnter details for book %d:\n", i + 1); printf("Title: "); scanf("%s", books[i].title); printf("Author: "); scanf("%s", books[i].author); printf("Year of Publication: "); scanf("%d", &books[i].year); printf("Is the book available? (1 for Yes, 0 for No): "); scanf("%d", &books[i].isAvailable); } printf("\nAvailable Books:\n"); for (int i = 0; i < n; i++) { if (books[i].isAvailable == 1) { printf("Title: %s, Author: %s, Year: %d\n", books[i].title, books[i].author, books[i].year); } } return 0; }
Car Rental System
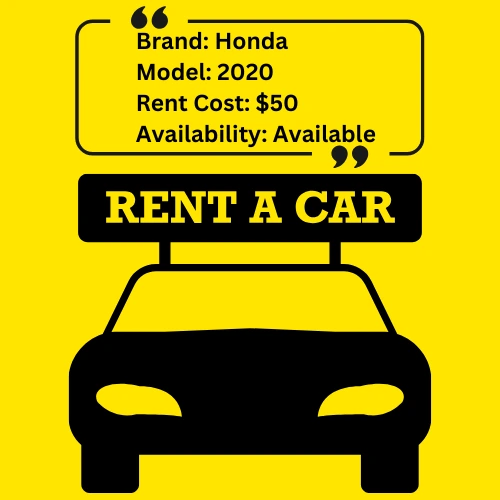
A car rental company wants to automate its rental process. The company keeps records of available cars, including their brand, model, rental cost per day, and availability status. The company allows customers to rent a car if it’s available. Write a C program that lets the user enter car details, checks availability, and calculates the rental cost based on the number of days the car is rented.
Test Case
Input
Enter the number of cars available: 2
Enter details for car 1:
Brand: Honda
Model: 2020
Rental Cost per day: 50
Is the car available? (1 for Yes, 0 for No): 1
Enter details for car 2:
Brand: Tesla
Model: 2025
Rental Cost per day: 100
Is the car available? (1 for Yes, 0 for No): 0
Enter the model of the car you want to rent: 2020
Enter number of days to rent the car: 5
Expected Output
Car Rented Successfully!
Brand: Honda, Model: 2020
Total Rental Cost: 250.00
- The program begins by asking the user to enter the number of cars available for rental. This input is stored in the variable
n
. - A structure
Car
is defined to store:brand
: The brand of the car.model
: The model name of the car.rentalCost
: The rental cost per day.isAvailable
: A flag to indicate if the car is available (1
for available,0
for rented).
- The program then enters a
for
loop to collect details for each car. - The car’s brand is stored in
cars[i].brand
. - The car’s model is stored in
cars[i].model
. - The rental cost per day is stored in
cars[i].rentalCost
. - The availability status is stored in
cars[i].isAvailable
(1 for available, 0 for not available).
- After entering the details of all cars, the program asks the user for the model of the car they want to rent.
- A
for
loop iterates through all cars to check if the requested model is available. - A manual character-by-character comparison is performed directly inside the loop using another
while
loop. - If a matching and available car is found, the program asks for the number of rental days.
- The total rental cost is calculated as
totalCost = rentalDays * cars[i].rentalCost
. - The availability of the car is updated to
0
(rented). The program prints the rental confirmation along with the total cost. The loop stops as soon as a matching available car is found. - If the car is found but already rented, a message is displayed stating that the car is not available.
- If no matching model is found, a message is displayed stating that the car is not available in the system.
- The program terminates after processing the car rental request.
#include <stdio.h> struct Car { char brand[50]; char model[50]; float rentalCost; int isAvailable; // 1 for available, 0 for rented }; int main() { int n; printf("Enter the number of cars available: "); scanf("%d", &n); struct Car cars[n]; for (int i = 0; i < n; i++) { printf("\nEnter details for car %d:\n", i + 1); printf("Brand: "); scanf("%s", cars[i].brand); printf("Model: "); scanf("%s", cars[i].model); printf("Rental Cost per day: "); scanf("%f", &cars[i].rentalCost); printf("Is the car available? (1 for Yes, 0 for No): "); scanf("%d", &cars[i].isAvailable); } char requestedModel[50]; printf("\nEnter the model of the car you want to rent: "); scanf("%s", requestedModel); int found = 0; for (int i = 0; i < n; i++) { int j = 0, match = 1; while (cars[i].model[j] != '\0' && requestedModel[j] != '\0') { if (cars[i].model[j] != requestedModel[j]) { match = 0; break; } j++; } if (match && cars[i].isAvailable == 1) { int rentalDays; printf("Enter number of days to rent the car: "); scanf("%d", &rentalDays); float totalCost = rentalDays * cars[i].rentalCost; cars[i].isAvailable = 0; // Mark as rented printf("\nCar Rented Successfully!\n"); printf("Brand: %s, Model: %s\n", cars[i].brand, cars[i].model); printf("Total Rental Cost: %.2f\n", totalCost); found = 1; break; } } if (!found) { printf("Sorry, the requested car is either not available or already rented.\n"); } return 0; }
Online Shopping Inventory
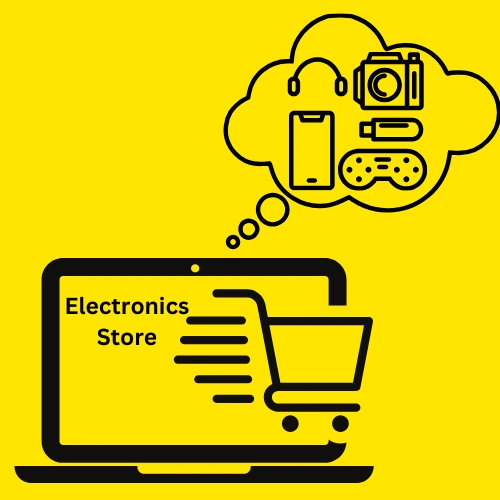
You own an online electronics store that needs an automated inventory system. Every product has a name, price per unit, and available stock. Customers request products by specifying the name and quantity they wish to purchase. Your program should check stock availability, calculate the total purchase cost, update inventory after a successful purchase, and provide confirmation. Write a C program that takes product details from the user, checks availability upon request, calculates total cost, updates stock, and displays transaction confirmation messages.
Test Case
Input
Enter number of products: 2
Enter product name: Laptop
Enter price per unit: 1000
Enter available stock: 20
Enter product name: Phone
Enter price per unit: 700
Enter available stock: 10
Enter product name to buy: Phone
Enter quantity: 8
Expected Output
Purchase confirmed! Total cost: 5600.00
Stock remaining: 2
- The program starts by asking the user to enter the number of products. This input is stored in the
n
variable. - For each product, the program prompts separately for the product name, price per unit, and available stock. These details are stored in an array of structures named
products
. - Next, the user is prompted to enter the product name they want to buy and the quantity desired.
- The program searches through the product array to find a matching product name.
- If the product is found, it checks if the available stock is sufficient.
- If the requested quantity is available, the program calculates the total cost, updates the product’s stock quantity, and displays a confirmation message with transaction details.
- If the stock is insufficient, it displays an appropriate message indicating the available quantity.
- If the product is not found, it displays a message indicating that the product is not found.
#include <stdio.h> struct Product { char name[50]; float price; int stock; }; int main() { int n, quantity, found = 0; char productName[50]; printf("Enter number of products: "); scanf("%d", &n); struct Product products[n]; for (int i = 0; i < n; i++) { printf("Enter product name: "); scanf("%s", products[i].name); printf("Enter price per unit: "); scanf("%f", &products[i].price); printf("Enter available stock: "); scanf("%d", &products[i].stock); } printf("Enter product name to buy: "); scanf("%s", productName); printf("Enter quantity: "); scanf("%d", &quantity); for (int i = 0; i < n; i++) { int j = 0, match = 1; while (products[i].name[j] != '\0' || productName[j] != '\0') { if (products[i].name[j] != productName[j]) { match = 0; break; } j++; } if (match) { found = 1; if (products[i].stock >= quantity) { products[i].stock -= quantity; printf("Purchase confirmed! Total cost: %.2f\n", quantity * products[i].price); printf("Stock remaining: %d\n", products[i].stock); } else { printf("Requested quantity not available. Stock available: %d\n", products[i].stock); } break; } } if (!found) { printf("Product not found.\n"); } return 0; }
Movie Rating System
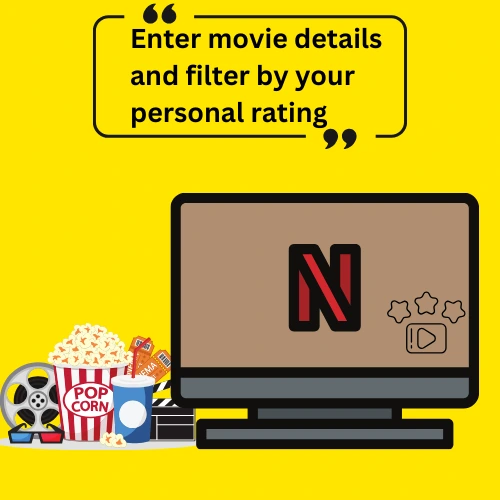
You are creating a simple rating system for a movie enthusiast’s personal database. Each movie entry includes the movie name, genre, release year, and a personal rating. Users can filter and display movies based on a minimum rating threshold. Write a C program that takes movie details as input from the user, asks for a rating threshold, and displays movies rated above this threshold, clearly showing their details.
Test Case
Input
Enter number of movies: 3
Movie 1
Enter movie name: Inception
Enter genre: SciFi
Enter release year: 2010
Enter rating (out of 10): 8.8
Movie 2
Enter movie name: Titanic
Enter genre: Romance
Enter release year: 1997
Enter rating (out of 10): 7.8
Movie 3
Enter movie name: Joker
Enter genre: Drama
Enter release year: 2019
Enter rating (out of 10): 8.5
Enter rating threshold: 8
Expected Output
Movies rated above 8.0:
Movie: Inception
Genre: SciFi
Year: 2010
Rating: 8.8
——————-
Movie: Joker
Genre: Drama
Year: 2019
Rating: 8.5
——————–
- The program starts by asking the user to enter the number of movies. This input is saved in the
n
variable. - For each movie, the program prompts the user to enter the movie name, genre, release year, and personal rating using separate
printf
statements and thefgets()
function for string inputs to handle spaces. These inputs are stored in the structure array namedmovies
. - Next, the user enters a minimum rating threshold.
- The program then iterates through the movies array, comparing each movie’s rating to the threshold.
- If the movie’s rating exceeds the threshold, the program displays the movie details immediately.
#include <stdio.h> struct Movie { char name[50]; char genre[20]; int year; float rating; }; int main() { int n; float threshold; printf("Enter number of movies: "); scanf("%d", &n); getchar(); // to consume newline after number input struct Movie movies[n]; for (int i = 0; i < n; i++) { printf("Movie %d\n",i+1); printf("Enter movie name: "); fgets(movies[i].name, sizeof(movies[i].name), stdin); printf("Enter genre: "); fgets(movies[i].genre, sizeof(movies[i].genre), stdin); printf("Enter release year: "); scanf("%d", &movies[i].year); printf("Enter rating (out of 10): "); scanf("%f", &movies[i].rating); getchar(); } printf("Enter rating threshold: "); scanf("%f", &threshold); printf("Movies rated above %.1f:\n", threshold); for (int i = 0; i < n; i++) { if (movies[i].rating > threshold) { printf("Movie: %s",movies[i].name); printf("Genre: %s",movies[i].genre); printf("Year: %d\n",movies[i].year); printf("Rating: %.1f\n",movies[i].rating); printf("-------------\n"); } } return 0; }
Event Attendance Tracker
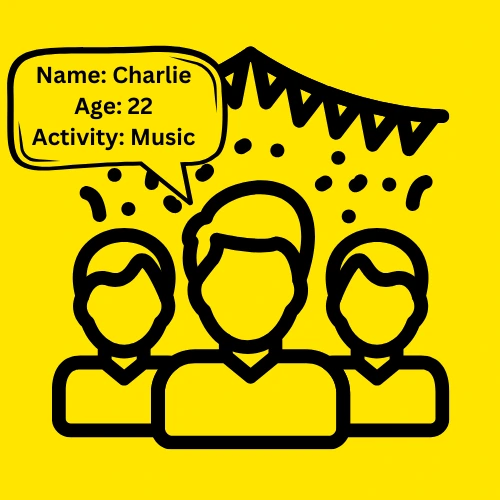
Imagine that you are organizing a community event and want a simple way to track attendee information. Each attendee will register their name, age, and their preferred activity from a list (e.g., sports, arts, music, gaming). After registrations, you want to categorize and display attendees based on their chosen activities. Write a C program that takes attendee details as input, categorizes attendees by their selected activity, and displays lists of attendees grouped by each activity.
Test Case
Input
Enter number of attendees: 3
Enter attendee name: Alice
Enter age: 23
Enter preferred activity (sports/arts/music/gaming): music
Enter attendee name: Bob
Enter age: 30
Enter preferred activity (sports/arts/music/gaming): sports
Enter attendee name: Charlie
Enter age: 22
Enter preferred activity (sports/arts/music/gaming): music
Expected Output
Attendees interested in sports
Bob
Age: 30
Attendees interested in arts
Attendees interested in music
Alice
Age: 23
Charlie
Age: 22
Attendees interested in gaming
- The program starts by asking the user to enter the number of attendees. This input is stored in the
n
variable. - For each attendee, the program prompts the user to enter their name, age, and preferred activity separately.
- For name and activity inputs, the program uses
fgets()
to handle spaces and ensure full line input is captured. - The
getchar()
function is used immediately after numeric inputs (scanf
) to consume the leftover newline character from the input buffer. - All these details are stored in an array of structures called
attendees
. - Next, the program defines an array called
activities
, containing the names of possible activities each attendee might select. - The program then categorizes attendees based on their selected activities.
- It does this by iterating through each activity and comparing the attendees’ chosen activity with the current activity using a manual character-by-character comparison in a nested loop.
- When a match is found, the program prints the attendee’s name and age grouped under the respective activity.
- Finally, the program displays categorized lists of attendees based on their activities.
#include <stdio.h> struct Attendee { char name[50]; int age; char activity[20]; }; int main() { int n; printf("Enter number of attendees: "); scanf("%d", &n); getchar(); struct Attendee attendees[n]; for (int i = 0; i < n; i++) { printf("Enter attendee name: "); fgets(attendees[i].name, sizeof(attendees[i].name), stdin); printf("Enter age: "); scanf("%d", &attendees[i].age); getchar(); printf("Enter preferred activity (sports/arts/music/gaming): "); fgets(attendees[i].activity, sizeof(attendees[i].activity), stdin); } char activities[4][20] = {"sports\n", "arts\n", "music\n", "gaming\n"}; for (int i = 0; i < 4; i++) { printf("\nAttendees interested in %s", activities[i]); for (int j = 0; j < n; j++) { int k = 0, match = 1; while (activities[i][k] != '\0' && attendees[j].activity[k] != '\0') { if (activities[i][k] != attendees[j].activity[k]) { match = 0; break; } k++; } if (match) { printf("%sAge: %d\n", attendees[j].name, attendees[j].age); } } } return 0; }
Weather Record Analysis
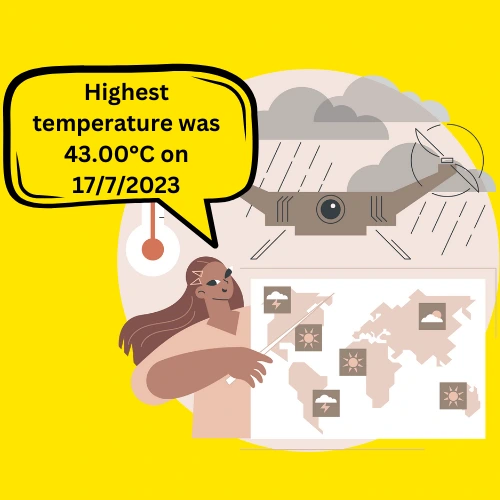
You work for a meteorological department that records daily temperature readings throughout the year. Each day’s record includes the date (day, month, year), highest temperature, and lowest temperature. At the end of the year, the department needs to identify and display the dates on which the highest temperature of the year and the lowest temperature of the year occurred. Write a program in C language that takes daily temperature records as input for an entire year (or a given number of days), finds the dates corresponding to the highest and lowest temperatures, and displays these dates clearly.
Test Case
Input
Enter number of days to record temperatures: 3
Enter details for day 1:
Day: 15
Month: 7
Year: 2023
Highest temperature: 42.5
Lowest temperature: 27.0
Enter details for day 2:
Day: 16
Month: 7
Year: 2023
Highest temperature: 40.0
Lowest temperature: 25.5
Enter details for day 3:
Day: 17
Month: 7
Year: 2023
Highest temperature: 43.0
Lowest temperature: 26.0
Expected Output
Highest temperature was 43.00°C on 17/7/2023
Lowest temperature was 25.50°C on 16/7/2023
- The program starts by asking the user to enter the number of days for which temperature records will be maintained. This input is stored in the
numDays
variable. - For each day, the program prompts separately for the date (day, month, year), highest temperature, and lowest temperature. These details are stored in an array of structures named
weatherRecords
. - The program initializes variables to store the maximum and minimum temperatures along with their corresponding dates using the first day’s data.
- Next, it iterates through the remaining records, comparing each day’s highest and lowest temperatures with the stored maximum and minimum temperatures.
- If a higher or lower temperature is found, the program updates the maximum or minimum temperature and corresponding date.
- Finally, the program displays the dates on which the highest and lowest temperatures occurred.
#include <stdio.h> struct WeatherRecord { int day; int month; int year; float highTemp; float lowTemp; }; int main() { int numDays; printf("Enter number of days to record temperatures: "); scanf("%d", &numDays); struct WeatherRecord weatherRecords[numDays]; for (int i = 0; i < numDays; i++) { printf("\nEnter details for day %d:\n", i + 1); printf("Day: "); scanf("%d", &weatherRecords[i].day); printf("Month: "); scanf("%d", &weatherRecords[i].month); printf("Year: "); scanf("%d", &weatherRecords[i].year); printf("Highest temperature: "); scanf("%f", &weatherRecords[i].highTemp); printf("Lowest temperature: "); scanf("%f", &weatherRecords[i].lowTemp); } struct WeatherRecord highest = weatherRecords[0]; struct WeatherRecord lowest = weatherRecords[0]; for (int i = 1; i < numDays; i++) { if (weatherRecords[i].highTemp > highest.highTemp) { highest = weatherRecords[i]; } if (weatherRecords[i].lowTemp < lowest.lowTemp) { lowest = weatherRecords[i]; } } printf("\nHighest temperature was %.2f°C on %d/%d/%d\n", highest.highTemp, highest.day, highest.month, highest.year); printf("Lowest temperature was %.2f°C on %d/%d/%d\n", lowest.lowTemp, lowest.day, lowest.month, lowest.year); return 0; }
Wizard Spellbook
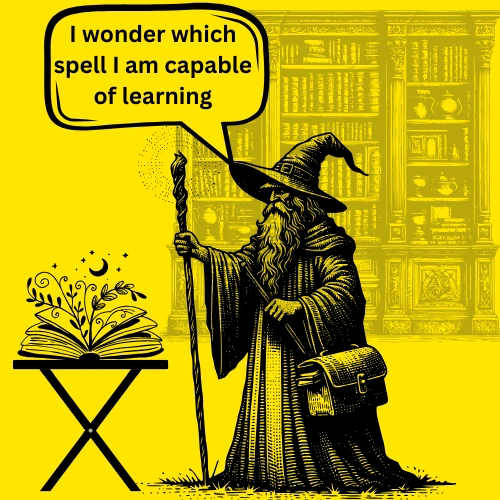
Imagine that you are managing an enchanted library that stores spellbooks for various wizards. Each spellbook contains a unique spell name and its difficulty level. Wizards visit your library to learn spells based on their current magical skill level. You need to display the spells a wizard can learn based on their provided skill level. Write a C program that takes details of spellbooks and the wizard’s skill level as input, and then displays the names and details of all spells whose difficulty level is equal to or less than the wizard’s skill level.
Test Case
Input
Enter number of spellbooks: 3
Enter spell name: Fireball
Enter spell difficulty level: 5
Enter spell name: Teleport
Enter spell difficulty level: 8
Enter spell name: Sheild
Enter spell difficulty level: 4
Enter wizard’s skill level: 5
Expected Output
Spells that the wizard can learn:
Spell: Fireball, Difficulty: 5
Spell: Sheild, Difficulty: 4
- The program begins by asking the user to enter the total number of spellbooks stored in the library. This input is stored in the variable
numSpellbooks
. - For each spellbook, the program separately prompts for the spell name and spell difficulty level. These details are stored in an array of structures called
spellbooks
. - Next, the user is asked to input a wizard’s current magical skill level.
- The program then iterates through the array of spellbooks, comparing the difficulty of each spell to the wizard’s skill level.
- If the spell’s difficulty is equal to or less than the wizard’s skill level, the details of that spell (spell name and difficulty) are displayed immediately.
#include <stdio.h> struct Spellbook { char spellName[50]; int difficulty; }; int main() { int numSpellbooks, wizardSkill; printf("Enter number of spellbooks: "); scanf("%d", &numSpellbooks); struct Spellbook spellbooks[numSpellbooks]; for (int i = 0; i < numSpellbooks; i++) { printf("\nEnter spell name: "); scanf("%s", spellbooks[i].spellName); printf("Enter spell difficulty level: "); scanf("%d", &spellbooks[i].difficulty); } printf("\nEnter wizard's skill level: "); scanf("%d", &wizardSkill); printf("\nSpells that the wizard can learn:\n"); for (int i = 0; i < numSpellbooks; i++) { if (spellbooks[i].difficulty <= wizardSkill) { printf("Spell: %s, Difficulty: %d\n", spellbooks[i].spellName, spellbooks[i].difficulty); } } return 0; }
Adventure Quest
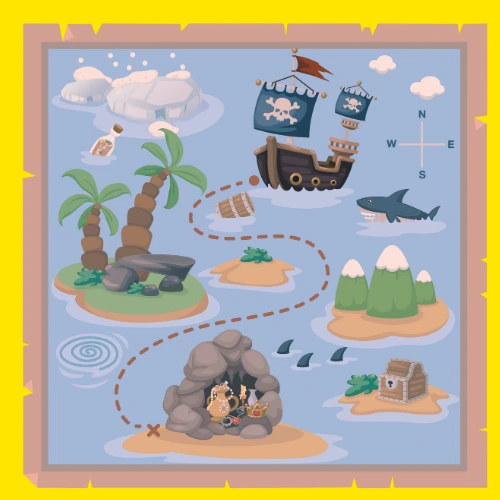
Imagine you are on an adventurous quest where you collect various magical items. Each item has a unique name, weight, and magical power value. You have limited carrying capacity based on weight, and your goal is to choose items to maximize your total magical power without exceeding your carrying capacity. Write a program in C language that takes the details of magical items collected during your quest and your carrying capacity as input, then identifies and displays the items individually that maximize magical power without exceeding your carrying capacity.
Test Case
Input
Enter number of magical items: 3
Enter item name: Amulet
Enter item weight: 3
Enter magical power: 50
Enter item name: Sword
Enter item weight: 5
Enter magical power: 70
Enter item name: Potion
Enter item weight: 2
Enter magical power: 30
Enter your carrying capacity: 5
Expected Output
Selected items for the quest:
Amulet
Potion
Total magical power: 80
Total weight: 5
- The program begins by prompting the user to enter the total number of magical items collected. This input is stored in the integer variable
numItems
. - Next, for each magical item, the program asks the user to enter the item’s name, weight, and magical power. These details are stored within an array of structures named
items
. - Additionally, the program calculates each item’s magical power-to-weight ratio and stores it in the same structure.
- The program then prompts the user to enter the carrying capacity, which is stored in the variable
capacity
. - The program then sorts the array of items in descending order based on the magical power-to-weight ratio using a simple bubble sort algorithm. In this sorting process, a temporary structure variable named
temp
is used to swap items. - Once sorted, the program iteratively checks through the sorted items list and adds each item’s weight to the current total weight (
currentWeight
) only if adding the item does not exceed the carrying capacity. - If the item can be added without exceeding the carrying capacity, the program adds this item’s magical power to the total magical power (
totalPower
) and displays the item’s name. - Finally, the program prints the list of selected items, the total magical power of these items, and the total weight, ensuring the carrying capacity is not exceeded.
#include <stdio.h> struct Item { char name[30]; int weight; int power; float ratio; }; int main() { int numItems, capacity, currentWeight = 0, totalPower = 0; printf("Enter number of magical items: "); scanf("%d", &numItems); struct Item items[numItems], temp; for(int i = 0; i < numItems; i++) { printf("\nEnter item name: "); scanf("%s", items[i].name); printf("Enter item weight: "); scanf("%d", &items[i].weight); printf("Enter magical power: "); scanf("%d", &items[i].power); items[i].ratio = (float)items[i].power / items[i].weight; } printf("\nEnter your carrying capacity: "); scanf("%d", &capacity); for(int i = 0; i < numItems-1; i++) { for(int j = 0; j < numItems-i-1; j++) { if(items[j].ratio < items[j+1].ratio) { temp = items[j]; items[j] = items[j+1]; items[j+1] = temp; } } } printf("\nSelected items for the quest:\n"); for(int i = 0; i < numItems; i++) { if(currentWeight + items[i].weight <= capacity) { printf("%s\n", items[i].name); currentWeight += items[i].weight; totalPower += items[i].power; } } printf("Total magical power: %d\n", totalPower); printf("Total weight: %d\n", currentWeight); return 0; }
Sports League Performance Tracker
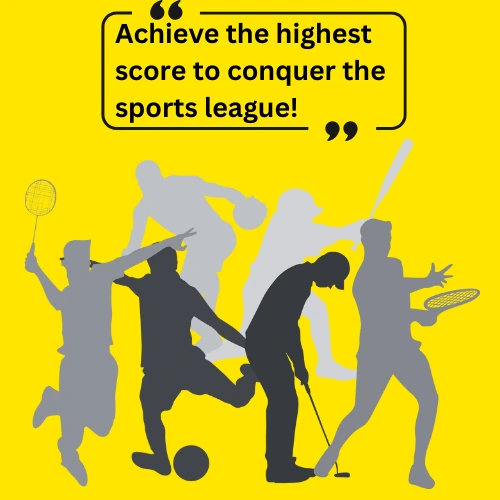
You are developing a sports league performance tracking system. Each team in the league plays multiple matches, and their performance is tracked based on points earned in each match. The system should allow users to input multiple teams, their matches, and the points they earned. The goal is to determine the team with the highest average points per match and display their details. Write a C program that takes the number of teams and their details (team ID, name, number of matches, and points per match) as input. The program should use pointer inside the structure to calculate total score of a team. It should then compute and display the average points for each team and identify the team with the highest average points.
Test Case
Input
Enter number of teams: 2
Enter maximum number of matches per team: 3
Enter Team ID: 101
Enter Team Name: Warriors
Enter points for Match 1: 20
Enter points for Match 2: 25
Enter points for Match 3: 30
Enter Team ID: 102
Enter Team Name: Titans
Enter points for Match 1: 35
Enter points for Match 2: 40
Enter points for Match 3: 32
Expected Output
Team Average Points:
Team: Warriors, Average Points: 25.00
Team: Titans, Average Points: 35.67
Team with Highest Average Points:
Team: Titans, Average Points: 35.67
- The program starts by asking the user to enter the number of teams. This input is saved in the
numTeams
variable. The user is then prompted to enter the maximum number of matches per team, which is stored inmaxMatches
. - For each team, the program prompts the user for the team’s unique ID and name. This information is stored in an array of structures named
teams
. - Next, the program asks the user to enter the points scored by each team in every match using a nested
for
loop. These values are stored in thepoints
array inside the structure. - The total points for each team are calculated by summing the points of all matches using an additional pointer (
totalPoints
) assigned to a separate integer arraytotalPointsArray
. - The program then iterates through the
teams
array, computing the average points per match for each team by dividing the total points by the number of matches. - The highest average points and the corresponding team are identified by comparing the computed averages.
- Finally, the program displays the average points for all teams and highlights the team with the highest average points.
#include <stdio.h> #define MAX_TEAMS 10 #define MAX_MATCHES 10 struct Team { int teamID; char name[50]; int points[MAX_MATCHES]; int *totalPoints; }; int main() { int numTeams, numMatches; struct Team teams[MAX_TEAMS]; int totalPointsArray[MAX_TEAMS]; printf("Enter number of teams: "); scanf("%d", &numTeams); printf("Enter maximum number of matches per team: "); scanf("%d", &numMatches); for(int i = 0; i < numTeams; i++) { teams[i].totalPoints = &totalPointsArray[i]; *teams[i].totalPoints = 0; printf("\nEnter Team ID: "); scanf("%d", &teams[i].teamID); printf("Enter Team Name: "); scanf("%s", teams[i].name); for(int j = 0; j < numMatches; j++) { printf("Enter points for Match %d: ", j + 1); scanf("%d", &teams[i].points[j]); *teams[i].totalPoints += teams[i].points[j]; } } int highestAvgIndex = 0; float highestAvg = 0.0; printf("\nTeam Average Points:\n"); for(int i = 0; i < numTeams; i++) { float avgPoints = (float)(*teams[i].totalPoints) / numMatches; printf("Team: %s, Average Points: %.2f\n", teams[i].name, avgPoints); if(avgPoints > highestAvg) { highestAvg = avgPoints; highestAvgIndex = i; } } printf("\nTeam with Highest Average Points:\n"); printf("Team: %s, Average Points: %.2f\n", teams[highestAvgIndex].name, highestAvg); return 0; }
Airline Flight Management System
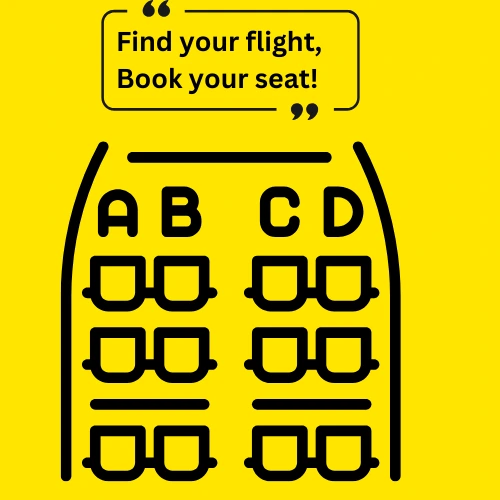
An airline company wants to develop a flight management system to keep track of its flights. Each flight has a unique flight number, a departure city, a destination city, a departure time, an arrival time, and a certain number of available seats. Passengers must search for a flight by entering the flight number, and if the flight exists, they should be able to see the flight details. If seats are available, the passenger should be able to book a seat. If no seats are available, the system should inform the passenger that no booking can be made. Write a program in C language that takes flight details as input, allows a passenger to search for a flight, and processes seat bookings accordingly.
Test Case
Input
Enter the number of flights: 2
Enter details for flight 1:
Flight Number: PK123
Departure City: Lahore
Destination City: Dubai
Departure Time (HH:MM): 10:30
Arrival Time (HH:MM): 14:00
Available Seats: 3
Enter details for flight 2:
Flight Number: PK456
Departure City: Karachi
Destination City: Istanbul
Departure Time (HH:MM): 22:15
Arrival Time (HH:MM): 05:45
Available Seats: 0
Enter Flight Number to search: PK123
Expected Output
Flight Found! Details:
Flight Number: PK123
Departure: Lahore -> Destination: Dubai
Departure Time: 10:30, Arrival Time: 14:00
Available Seats: 3
Seat available! Booking now…
Booking Confirmed! Remaining seats: 2
- The program starts by asking the user to enter the number of flights. This input is saved in the variable
n
. - Next, the program declares an array of structures named
flights[n]
, where each element in the array represents a flight. The structureFlight
is defined to store details such as the flight number, departure and destination cities, departure and arrival times, and the number of available seats. - The program then takes input for each flight using a for loop. The loop iterates from
0
ton-1
, prompting the user to enter:- The flight number (stored in
flightNumber
) - The departure city (stored in
departureCity
) - The destination city (stored in
destinationCity
) - The departure time (stored in
departureTime
) - The arrival time (stored in
arrivalTime
) - The number of available seats (stored in
availableSeats
)
- The flight number (stored in
- Each of these values is stored in the respective index of the
flights
array. - After storing the flight details, the program asks the user to enter a flight number to search for. This input is stored in the variable
searchFlight
. The program then begins searching for a matching flight in theflights
array using another for loop.
- Within the loop, the program performs manual string comparison to check if
searchFlight
matches theflightNumber
of a stored flight. - The variable
isMatch
is initialized to1
, assuming that the flight numbers match. If any character insearchFlight
does not match the corresponding character inflights[i].flightNumber
,isMatch
is set to0
, indicating that the flight numbers are not the same. The comparison continues until the loop reaches the null character\0
at the end of both strings. - If a match is found, the program displays the flight details, including:
- The flight number
- The departure and destination cities
- The departure and arrival times
- The number of available seats
- Next, the program checks if seats are available for booking. If
availableSeats
is greater than0
, the program informs the user that a seat is available, decreases the seat count by 1, and confirms the booking. The updated number of available seats is displayed. - If there are no seats available (
availableSeats == 0
), the program displays a message informing the user that the flight is fully booked and that no seats can be allocated. - If no matching flight is found after the loop completes, the program displays a message indicating that the flight number was not found.
- Finally, the program terminates after processing the search and booking request.
#include <stdio.h> struct Flight { char flightNumber[10]; char departureCity[30]; char destinationCity[30]; char departureTime[10]; char arrivalTime[10]; int availableSeats; }; int main() { int n, i, j, found = 0; char searchFlight[10]; printf("Enter the number of flights: "); scanf("%d", &n); struct Flight flights[n]; for (i = 0; i < n; i++) { printf("\nEnter details for flight %d:\n", i + 1); printf("Flight Number: "); scanf("%s", flights[i].flightNumber); printf("Departure City: "); scanf("%s", flights[i].departureCity); printf("Destination City: "); scanf("%s", flights[i].destinationCity); printf("Departure Time (HH:MM): "); scanf("%s", flights[i].departureTime); printf("Arrival Time (HH:MM): "); scanf("%s", flights[i].arrivalTime); printf("Available Seats: "); scanf("%d", &flights[i].availableSeats); } printf("\nEnter Flight Number to search: "); scanf("%s", searchFlight); for (i = 0; i < n; i++) { int isMatch = 1; for (j = 0; flights[i].flightNumber[j] != '\0' || searchFlight[j] != '\0'; j++) { if (flights[i].flightNumber[j] != searchFlight[j]) { isMatch = 0; break; } } if (isMatch) { found = 1; printf("\nFlight Found! Details:\n"); printf("Flight Number: %s\n", flights[i].flightNumber); printf("Departure: %s -> Destination: %s\n", flights[i].departureCity, flights[i].destinationCity); printf("Departure Time: %s, Arrival Time: %s\n", flights[i].departureTime, flights[i].arrivalTime); printf("Available Seats: %d\n", flights[i].availableSeats); if (flights[i].availableSeats > 0) { printf("\nSeat available! Booking now...\n"); flights[i].availableSeats--; printf("Booking Confirmed! Remaining seats: %d\n", flights[i].availableSeats); } else { printf("\nSorry, No seats available on this flight.\n"); } break; } } if (!found) { printf("\nFlight Not Found!\n"); } return 0; }
Ultimate Road Trip Vehicle Selection System
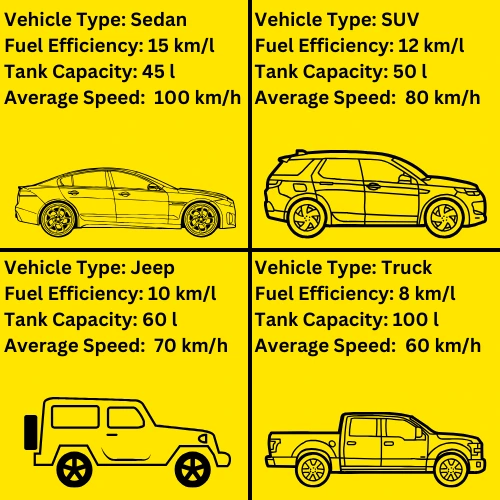
You and your friends are planning the ultimate road trip across multiple cities. You have a set of vehicles available, each with different specifications such as fuel efficiency in kilometers per liter, fuel tank capacity in liters, and average speed in kilometers per hour. Before deciding which vehicle to take on the trip, you need to calculate the maximum distance each vehicle can travel on a full tank, the total time required for a given trip distance using each vehicle, and the most fuel-efficient vehicle for a given trip. Additionally, you should be able to search for a specific vehicle by model name and determine whether the vehicle can complete the trip without refueling, the total time required for the trip using this vehicle, and the total fuel required for the journey if refueling is necessary. Write a program in C language that takes vehicle details as input, calculates the maximum travel distance for each vehicle, determines the total time and fuel required for a given trip, and allows the user to search for a specific vehicle to check its feasibility for the trip.
Test Case
Input
Enter the number of vehicles: 3
Enter details for vehicle 1:
Model Name: SUV
Fuel Efficiency (km per liter): 12
Fuel Tank Capacity (liters): 50
Average Speed (km per hour): 80
Enter details for vehicle 2:
Model Name: Sedan
Fuel Efficiency (km per liter): 15
Fuel Tank Capacity (liters): 45
Average Speed (km per hour): 100
Enter details for vehicle 3:
Model Name: Truck
Fuel Efficiency (km per liter): 8
Fuel Tank Capacity (liters): 100
Average Speed (km per hour): 60
Enter Vehicle Model to check for trip: Sedan
Enter total trip distance (km): 800
Vehicle Found! Details:
Model: Sedan
Maximum Distance on Full Tank: 675.00 km
Speed: 100.00 km/h
This vehicle CANNOT complete the trip without refueling.
Total Fuel Required for the Trip: 53.33 liters
Total Time Required for the Trip: 8.00 hours
Expected Output
Vehicle Details:
Model: SUV | Max Distance: 600.00 km | Speed: 80.00 km/h
Model: Sedan | Max Distance: 675.00 km | Speed: 100.00 km/h
Model: Truck | Max Distance: 800.00 km | Speed: 60.00 km/h
- The program starts by asking the user to enter the number of vehicles to be considered for the road trip. This input is stored in the variable
n
. - Next, the program declares an array of structures named
vehicles[n]
, where each element in the array represents a vehicle. The structureVehicle
is defined to store details such as the vehicle model name, the fuel efficiency in kilometers per liter, the fuel tank capacity in liters, the average speed in kilometers per hour, and the maximum distance the vehicle can travel on a full tank. - The program then takes input for each vehicle using a for loop. The loop iterates from zero to
n-1
, prompting the user to enter the vehicle model name, fuel efficiency, fuel tank capacity, and average speed. - After collecting these inputs, the program calculates the maximum distance the vehicle can travel on a full tank by multiplying the fuel efficiency by the fuel tank capacity. The calculated value is stored in the maxDistance field of the structure for each vehicle.
- Once all vehicle details have been entered, the program displays the details of each vehicle, including the calculated maximum distance.
- After this, the program asks the user to enter a specific vehicle model to check for a trip. The user is also asked to input the total trip distance in kilometers.
- The program then searches for the vehicle model in the list using a manual string comparison. It compares each character of the inputted model with stored vehicle models character by character.
- If a match is found, the program displays the selected vehicle’s maximum distance on a full tank.
- It then checks if the vehicle can complete the trip without refueling.
- If the maximum distance of the vehicle is greater than or equal to the trip distance, the program prints that the vehicle can complete the trip.
- If the maximum distance is less than the trip distance, the program calculates the total fuel required for the entire trip by dividing the trip distance by the fuel efficiency.
- The program then prints this calculated fuel requirement.
- Next, the program calculates the total time required for the trip by dividing the trip distance by the speed of the vehicle.
- It then displays the computed time required for the journey.
- If no matching vehicle model is found, the program displays a message indicating that the vehicle model was not found.
#include <stdio.h> struct Vehicle { char model[20]; float fuelEfficiency; float tankCapacity; float speed; float maxDistance; }; int main() { int n, i, j, found = 0; char searchModel[20]; float tripDistance, totalFuelRequired, totalTime; printf("Enter the number of vehicles: "); scanf("%d", &n); struct Vehicle vehicles[n]; for (i = 0; i < n; i++) { printf("\nEnter details for vehicle %d:\n", i + 1); printf("Model Name: "); scanf("%s", vehicles[i].model); printf("Fuel Efficiency (km per liter): "); scanf("%f", &vehicles[i].fuelEfficiency); printf("Fuel Tank Capacity (liters): "); scanf("%f", &vehicles[i].tankCapacity); printf("Average Speed (km per hour): "); scanf("%f", &vehicles[i].speed); vehicles[i].maxDistance = vehicles[i].fuelEfficiency * vehicles[i].tankCapacity; } printf("\nVehicle Details:\n"); for (i = 0; i < n; i++) { printf("Model: %s | Max Distance: %.2f km | Speed: %.2f km/h\n", vehicles[i].model, vehicles[i].maxDistance, vehicles[i].speed); } printf("\nEnter Vehicle Model to check for trip: "); scanf("%s", searchModel); printf("Enter total trip distance (km): "); scanf("%f", &tripDistance); for (i = 0; i < n; i++) { int isMatch = 1; for (j = 0; vehicles[i].model[j] != '\0' || searchModel[j] != '\0'; j++) { if (vehicles[i].model[j] != searchModel[j]) { isMatch = 0; break; } } if (isMatch) { found = 1; printf("\nVehicle Found! Details:\n"); printf("Model: %s\n", vehicles[i].model); printf("Maximum Distance on Full Tank: %.2f km\n", vehicles[i].maxDistance); printf("Speed: %.2f km/h\n", vehicles[i].speed); if (vehicles[i].maxDistance >= tripDistance) { printf("This vehicle CAN complete the trip without refueling.\n"); } else { totalFuelRequired = tripDistance / vehicles[i].fuelEfficiency; printf("This vehicle CANNOT complete the trip without refueling.\n"); printf("Total Fuel Required for the Trip: %.2f liters\n", totalFuelRequired); } totalTime = tripDistance / vehicles[i].speed; printf("Total Time Required for the Trip: %.2f hours\n", totalTime); break; } } if (!found) { printf("\nVehicle Model Not Found!\n"); } return 0; }
Multi-Level Smart Parking Lot Management System
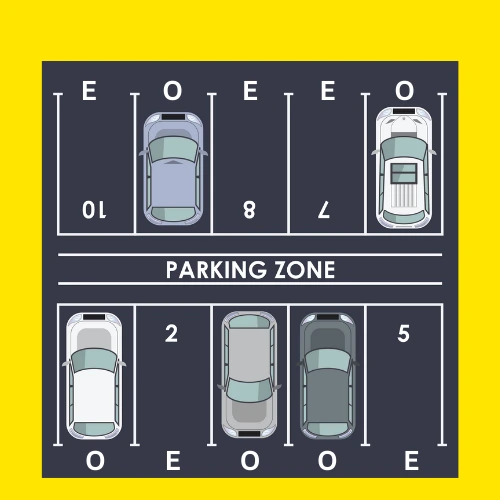
A modern multi-level parking system needs to manage vehicle parking efficiently. The parking lot consists of multiple floors, with each floor containing multiple parking spots. Each parking spot can either be empty (E) or occupied (O). Additionally, each floor has a unique floor ID, a total number of spots, and a current number of occupied spots. The system should allow the user to input the parking status of each floor, search for an available parking spot on a specific floor, and park a vehicle in an empty spot if available. If a spot is occupied, the system should inform the user that parking is not possible at that location. The system should also keep track of how many spots are currently occupied on each floor. Write a program in C language that takes the parking lot details as input, stores them in a structure containing a 2D array, keeps track of the number of occupied spots per floor, allows the user to check for available spots, and updates the parking lot status when a vehicle is parked.
Test Case
Input
Enter the number of floors: 2
Enter the number of spots per floor: 3
Enter parking status for Floor 1 (E for empty, O for occupied):
Floor 1, Spot 1: E
Floor 1, Spot 2: O
Floor 1, Spot 3: E
Enter parking status for Floor 2 (E for empty, O for occupied):
Floor 2, Spot 1: E
Floor 2, Spot 2: O
Floor 2, Spot 3: E
Enter floor number to park on: 1
Enter spot number to park in: 3
Expected Output
Current Parking Lot Status:
Floor 1: Occupied Spots: 1 / 3
Parking Layout: E O E
Floor 2: Occupied Spots: 1 / 3
Parking Layout: E O E
Spot is available! Parking the vehicle…
Updated Parking Lot Status:
Floor 1: Occupied Spots: 2 / 3
Parking Layout: E O O
Floor 2: Occupied Spots: 1 / 3
Parking Layout: E O E
- The program starts by asking the user to enter the number of floors in the parking lot. This input is stored in the variable
floors
. The program then prompts the user to enter the number of spots per floor, which is stored in the variablespots
. - Next, the program defines a structure named
ParkingLot
, which contains the following data members:floorID
stores the identification number for the floor.totalSpots
stores the total number of spots available on the floor.occupiedSpots
keeps track of how many parking spots are occupied.status
is a 2D character array representing the parking layout, where each spot is either ‘E’ (empty) or ‘O’ (occupied).
- After defining the structure, the program initializes an array of
ParkingLot
structures, one for each floor. - It then uses a nested for loop to take user input for each parking spot on each floor. The user is required to enter either ‘E’ for an empty spot or ‘O’ for an occupied spot.
- The program also counts the number of occupied spots on each floor while taking input and stores this count in the
occupiedSpots
variable inside the structure. - Once the parking lot is fully initialized, the program displays the entire parking status floor by floor, showing the number of occupied spots and the parking layout of each floor.
- The program then prompts the user to enter a floor number and a parking spot number to attempt parking a vehicle.
- The program performs bounds checking to ensure that the entered floor and spot numbers are within a valid range.
- If the selected parking spot contains ‘E’, the program updates it to ‘O’, increments the
occupiedSpots
count for that floor, and informs the user that the vehicle has been successfully parked. It then displays the updated parking lot status with the new number of occupied spots. - If the selected spot is already occupied (‘O’), the program informs the user that parking at that location is not possible.
- If the user enters an invalid floor or spot number, the program prints an error message indicating that the selected location does not exist.
- Finally, the program terminates after processing the parking operation.
#include <stdio.h> struct ParkingLot { int floorID; int totalSpots; int occupiedSpots; char status[10][10]; }; int main() { int i, j; int floors, spots; int floorNum, spotNum; printf("Enter the number of floors: "); scanf("%d", &floors); printf("Enter the number of spots per floor: "); scanf("%d", &spots); struct ParkingLot lot[floors]; for (i = 0; i < floors; i++) { lot[i].floorID = i + 1; lot[i].totalSpots = spots; lot[i].occupiedSpots = 0; printf("\nEnter parking status for Floor %d (E for empty, O for occupied):\n", lot[i].floorID); for (j = 0; j < spots; j++) { printf("Floor %d, Spot %d: ", lot[i].floorID, j + 1); scanf(" %c", &lot[i].status[i][j]); if (lot[i].status[i][j] == 'O') { lot[i].occupiedSpots++; } } } printf("\nCurrent Parking Lot Status:\n"); for (i = 0; i < floors; i++) { printf("Floor %d: Occupied Spots: %d / %d\n", lot[i].floorID, lot[i].occupiedSpots, lot[i].totalSpots); printf("Parking Layout: "); for (j = 0; j < spots; j++) { printf("%c ", lot[i].status[i][j]); } printf("\n"); } printf("\nEnter floor number to park on: "); scanf("%d", &floorNum); printf("Enter spot number to park in: "); scanf("%d", &spotNum); if (floorNum < 1 || floorNum > floors || spotNum < 1 || spotNum > spots) { printf("\nInvalid floor or spot number. Please enter a valid location.\n"); } else { if (lot[floorNum - 1].status[floorNum - 1][spotNum - 1] == 'E') { printf("\nSpot is available! Parking the vehicle...\n"); lot[floorNum - 1].status[floorNum - 1][spotNum - 1] = 'O'; lot[floorNum - 1].occupiedSpots++; } else { printf("\nSorry, this spot is already occupied.\n"); } } printf("\nUpdated Parking Lot Status:\n"); for (i = 0; i < floors; i++) { printf("Floor %d: Occupied Spots: %d / %d\n", lot[i].floorID, lot[i].occupiedSpots, lot[i].totalSpots); printf("Parking Layout: "); for (j = 0; j < spots; j++) { printf("%c ", lot[i].status[i][j]); } printf("\n"); } return 0; }