Explore C programming through simple and engaging real-life scenarios, with a focus on nested if-else statements. Work through problems like school admissions, tax calculations, energy management, and game creation using nested if-else structures. Each example comes with test cases, clear explanations, and sample code perfect for beginners and those who want to enhance their coding skills step by step.
Admission Based on Age and Grade
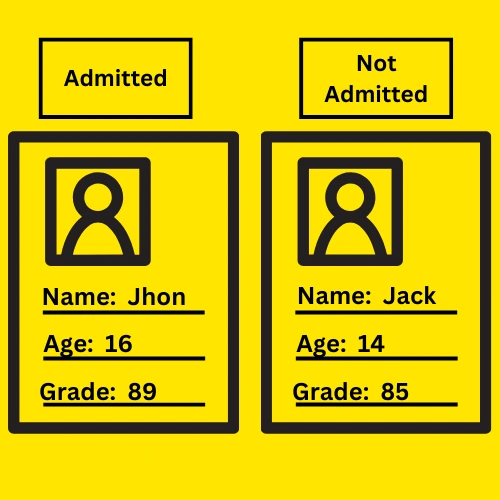
Imagine that you are writing a program for a school to help the admission team decide if a student can be admitted based on their age and grade. If a student is older than 15 and their grade is above 80, they will be admitted. If the student is 15 or younger and their grade is above 90, they will be admitted under a special case. In any other situation, the student will not be admitted. Write a C program to take the student’s age and grade as input and decide if they should be admitted, admitted under special consideration, or not admitted.
Test Case
Input
Enter age: 14
Enter grade: 92
Expected Output
Admitted under special case.
- The program begins by prompting the user to input their age and grade, which are stored in the variables
age
andgrade
respectively. Then The program uses a nested if-else structure to evaluate the admission criteria. - An
if
statement checks if theage
is greater than 15. If this condition is true, the program further checks if thegrade
is greater than 80. - If both conditions are true, the program prints “
Admitted
.” If the grade condition fails, it prints “Not admitted.
“ - If the initial
age
condition fails, the program useselse
condition to evaluate if thegrade
is greater than 90. - If this condition is true, the program prints “
Admitted under special case
.” Otherwise, it prints “Not admitted.
“
#include <stdio.h> int main() { int age; int grade; printf("Enter age: "); scanf("%d", &age); printf("Enter grade: "); scanf("%d", &grade); if (age > 15) { if (grade > 80) { printf("Admitted.\n"); } else { printf("Not admitted.\n"); } } else { if (grade > 90) { printf("Admitted under special case.\n"); } else { printf("Not admitted.\n"); } } return 0; }
Bank Loan Approval Decision Program
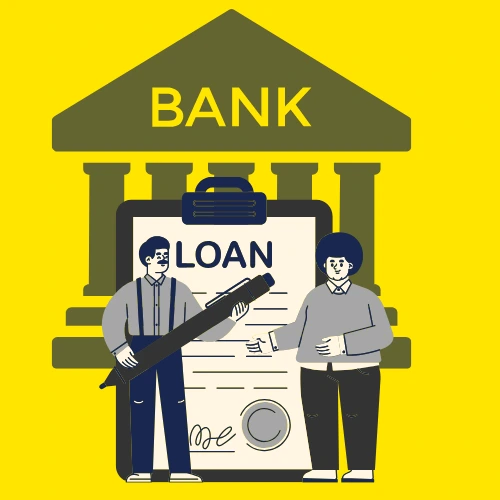
Imagine that you are writing a program for a bank to decide if a customer qualifies for a loan based on their annual income and credit score. If a customer’s income is more than $50,000 and their credit score is above 700, their loan will be approved. If their income is less than $50,000 but their credit score is above 750, their loan will be approved under special conditions. In any other case, the loan will be denied.
Write a C program to take the customer’s income and credit score as input and decide if their loan should be approved, approved under special conditions, or denied.
Test Case
Input
Enter annual income: 52000
Enter credit score: 750
Expected Output
Loan Approved under special conditions.
- The program begins by prompting the user to input their annual income and credit score, which are stored in the variables
income
andcreditScore
respectively. - The program uses a nested if-else structure to evaluate loan eligibility.
- An
if
statement checks if theincome
is greater than $50,000. If this condition is true, it further checks if the credit score is above 700. - If both conditions are met, the program prints “
Loan Approved.
” If the credit score condition fails, it prints “Loan Denied.
“. - If the initial income condition fails, the program evaluates if the credit score is above 750.
- If this condition is true, the program prints “
Loan Approved under special conditions.
” Otherwise, it prints “Loan Denied.
“.
#include <stdio.h> int main() { int income; int creditScore; printf("Enter annual income: "); scanf("%d", &income); printf("Enter credit score: "); scanf("%d", &creditScore); if (income > 50000) { if (creditScore > 700) { printf("Loan Approved.\n"); } else { printf("Loan Denied.\n"); } } else { if (creditScore > 750) { printf("Loan Approved under special conditions.\n"); } else { printf("Loan Denied.\n"); } } return 0; }
Customer Reward Points
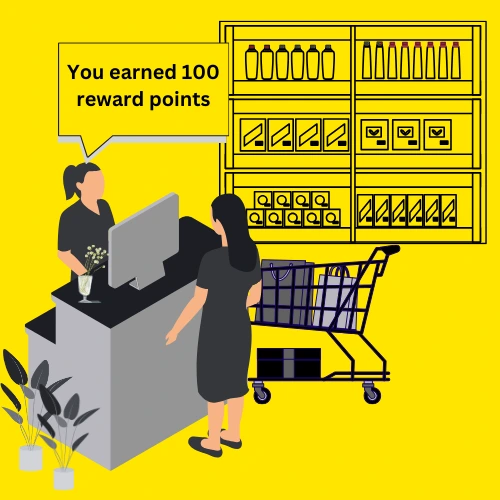
Imagine a store wants to calculate reward points for its customers based on their spending, membership type, and whether they shop during a promotion period. For premium members, if they spend more than $500, they earn 150 reward points during a promotion or 100 points if it is not a promotion period. If their spending is $500 or less, they earn 75 points during a promotion or 50 points otherwise. For regular members, if they spend more than $500, they earn 50 reward points during a promotion or 25 points if it is not a promotion. However, if they spend $500 or less, no points are awarded, regardless of whether it is a promotion or not. Write a C program that takes the customer’s spending, membership type, and promotion status as input and calculates the number of reward points they will receive.
Test Case
Input
Enter total purchase amount: 550
Enter membership type (1 for Premium, 0 for Regular): 1
Is it a promotion period? (1 for Yes, 0 for No): 0
Expected Output
Reward Points Awarded: 100
- The program starts by asking the user to enter their total purchase amount, membership type (1 for premium, 0 for regular), and whether the purchase was made during a promotion (1 for Yes, 0 for No). These inputs are saved in the variables
purchaseAmount
,membership
, andpromotion
variables respectively. - The program then uses a nested if-else structure to decide how many reward points the customer should get.
- First, an
if
condition is used to check whether the customer has a premiummembership
(membership == 1)
. - Once this condition is true, the program uses a nested condition to check whether the
purchaseAmount
is greater than 500(purchaseAmount > 500)
. - Once this condition is also true, the program checks whether it is the
promotion
period(promotion == 1)
. - When the purchase is made during a promotion, the program awards 150 reward points to the customer. Otherwise, the customer is awarded 100 points.
- When the
purchaseAmount
is not greater than 500, the program checks whether the purchase is made during thepromotion
period. - During the
promotion
, the program awards 75 reward points. Otherwise, the customer gets 50 reward points. - When the customer is not a premium member
(membership == 0)
, the program checks whether the customer has spent more than $500(purchaseAmount > 500)
. - Once this condition is true, the program further checks whether the purchase was made during a
promotion
. - If true, the customer gets 50 reward points. Otherwise, the customer gets 25 reward points.
- For customers who spent $500 or less, no reward points are given.
- Finally, the program shows the total reward points to the user using the
printf
function.
#include <stdio.h> int main() { int purchaseAmount, membership, promotion; int rewardPoints = 0; printf("Enter total purchase amount: "); scanf("%d", &purchaseAmount); printf("Enter membership type (1 for Premium, 0 for Regular): "); scanf("%d", &membership); printf("Is it a promotion period? (1 for Yes, 0 for No): "); scanf("%d", &promotion); if (membership == 1) { if (purchaseAmount > 500) { if (promotion == 1) { rewardPoints = 150; } else { rewardPoints = 100; } } else { if (promotion == 1) { rewardPoints = 75; } else { rewardPoints = 50; } } } else if (membership == 0) { if (purchaseAmount > 500) { if (promotion == 1) { rewardPoints = 50; } else { rewardPoints = 25; } } else { rewardPoints = 0; } } printf("Reward Points Awarded: %d\n", rewardPoints); return 0; }
Travel Permission Decision
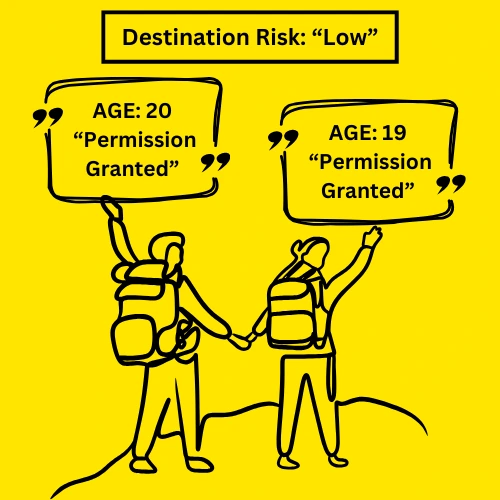
Imagine that you are writing a program for a travel agency to decide whether a traveler can be granted permission based on their age and the destination’s risk level. If a traveler is older than 18 and the risk level of the destination is low or very low, then permission is granted. If the traveller is younger than 18 and the risk level is very low, then permission is granted with parental consent. In all other cases, permission is denied. Write a C program to take the traveler’s age and the destination’s risk level as input and decide if permission should be granted, granted with parental consent, or denied.
Test Case
Input
Enter age: 19
Enter destination risk level (1 for Very Low, 2 for Low, 3 for High): 2
Expected Output
Permission Granted.
- The program begins by asking the user to input the traveller’s age and the destination’s risk level (1 for Very Low, 2 for Low, 3 for High). These inputs are stored in
age
andriskLevel
variables respectively. - An
if
condition checks if the traveller’sage
is greater than 18. - If the condition is true, then another
if
condition checks if theriskLevel
is Very Low (1) or Low (2). - If this is true, the program prints “
Permission Granted.
” - If this condition is false, then the
else
the block is executed, and the program prints “Permission Denied.
” - If the traveller’s age is 18 or less, then the initial if condition fails, and the
else
block of the firstif
condition is executed. - Inside this block, another
if
condition checks if the risk level is Very Low (1). - If true, the program prints “
Permission Granted with Parental Consent.
” - Otherwise, the
else
block executes, and it prints “Permission Denied.
” - The program ends after printing the appropriate result.
#include <stdio.h> int main() { int age; int riskLevel; printf("Enter age: "); scanf("%d", &age); printf("Enter destination risk level (1 for Very Low, 2 for Low, 3 for High): "); scanf("%d", &riskLevel); if (age > 18) { if (riskLevel == 1 || riskLevel == 2) { printf("Permission Granted.\n"); } else { printf("Permission Denied.\n"); } } else { if (riskLevel == 1) { printf("Permission Granted with Parental Consent.\n"); } else { printf("Permission Denied.\n"); } } return 0; }
Electricity Rate Calculation
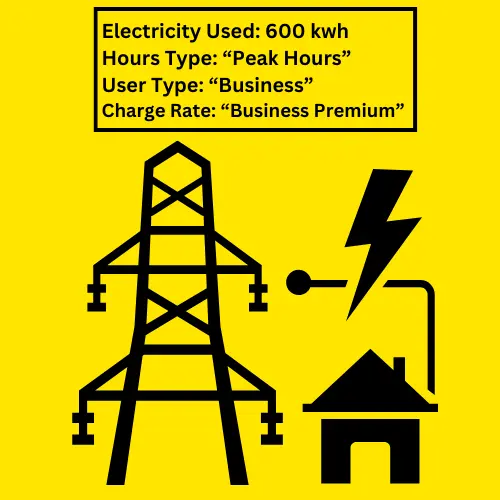
Imagine that you are writing a program for an energy company to determine the appropriate rate plan for its customers based on their electricity usage, the time of day, and whether the customer is a residential or business user. If the customer’s usage exceeds 500 kWh and it is during peak hours, a premium rate applies. Business users are charged a business premium rate, while residential users are charged a residential premium rate. If the usage exceeds 500 kWh but it is not during peak hours, then the standard rate applies. If the customer’s usage is 500 kWh or less and it is during off-peak hours, the customer qualifies for a discounted rate. Business users are charged a business discount rate, while residential users are charged a residential discount rate. If the usage is 500 kWh or less and it is during peak hours, the standard rate applies. Write a program in C language that takes the electricity usage, the time of day, and the user type as input and determines if the customer should be charged a premium rate, a discounted rate, or the standard rate.
Test Case
Input
Enter electricity usage (in kWh): 600
Is it during peak hours? (1 for Yes, 0 for No): 1
Enter user type (1 for residential, 2 for business): 2
Expected Output
Business Premium Rate Applied.
- The program starts by asking the user to enter their electricity usage in kilowatt-hours (kWh), the time of day (1 for peak hours, 0 for off-peak), and the user type (1 for residential, 2 for business). These inputs are stored in the variables
usage
,isPeak
, anduserType
respectively. - The program then uses a nested if-else structure to determine the appropriate rate plan.
- First, an
if
condition is used to check whether the electricityusage
is greater than 500 kWh(usage > 500)
. - Once this condition is true, the program uses a nested condition to check whether the time of day is during peak hours
(isPeak == 1)
. - Once this condition is also true, the program checks whether the
userType
is business(userType == 2)
. - If the user is a business customer, the program applies the business premium rate and prints “
Business Premium Rate Applied.
” Otherwise, the residential premium rate is applied and “Residential Premium Rate Applied
” is printed. - If the time of day is not during peak hours, the program applies the standard rate and prints “
Standard Rate Applied.
“ - When the electricity
usage
is 500 kWh or less, the program checks whether the time of day is off-peak(isPeak == 0)
. - If this condition is true, the program checks whether the
userType
is residential(userType == 1)
. - If the user is residential, the program applies the residential discount rate and prints
"Residential Discount Rate Applied.
” Otherwise, the business discount rate is applied and “Business Discount Rate Applied
” is printed. - If the time of day is during peak hours, the program applies the standard rate and prints “
Standard Rate Applied.
“
#include <stdio.h> int main() { int usage; int isPeak; int userType; printf("Enter electricity usage (in kWh): "); scanf("%d", &usage); printf("Is it during peak hours? (1 for Yes, 0 for No): "); scanf("%d", &isPeak); printf("Enter user type (1 for residential, 2 for business): "); scanf("%d", &userType); if (usage > 500) { if (isPeak == 1) { if (userType == 2) { printf("Business Premium Rate Applied.\n"); } else { printf("Residential Premium Rate Applied.\n"); } } else { printf("Standard Rate Applied.\n"); } } else { if (isPeak == 0) { if (userType == 1) { printf("Residential Discount Rate Applied.\n"); } else { printf("Business Discount Rate Applied.\n"); } } else { printf("Standard Rate Applied.\n"); } } return 0; }
Income Tax calculation
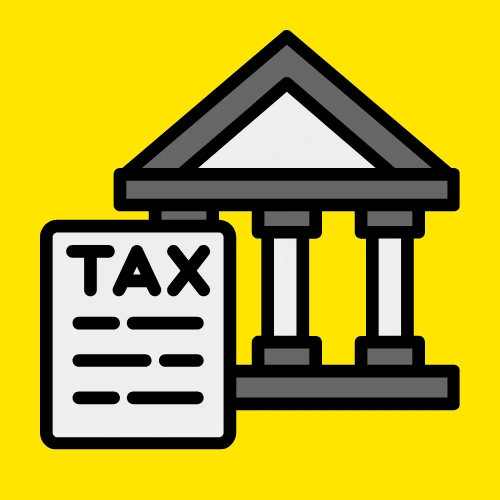
Imagine that you are writing a program for a government tax system to calculate income tax based on a person’s annual income and the number of dependents. If the income is more than $80,000 and there are no dependents, the tax rate is 30%. If the income is more than $80,000 but there are dependents, the tax rate is reduced to 20%. If the income is $80,000 or below, the tax rate is 10%, regardless of the number of dependents. Design a program in C language to take the annual income and the number of dependents as input and determine the applicable tax rate.
Test Case
Input
Enter annual income: 100000
Enter number of dependents: 1
Expected Output
Tax Amount: $20000.00
- The program begins by asking the user to input their annual income and the number of dependents. These values are stored in the
income
anddependents
variables, respectively. - An
if
condition checks if the income is greater than $80,000. If this condition is true, then another condition checks if the number of dependents is 0. - If this is true, the
tax
is calculated as 30% of the income. - Otherwise, the
else
block is executed, and the tax is calculated as 20% of the income. - If the income is $80,000 or below, the
else
block of the first condition is executed and thetax
is calculated as 10% of the income. - Finally, the program prints the calculated
tax
amount using aprintf
function.
#include <stdio.h> int main() { float income; float tax; int dependents; printf("Enter annual income: "); scanf("%f", &income); printf("Enter number of dependents: "); scanf("%d", &dependents); if (income > 80000) { if (dependents == 0) { tax = income * 0.30; } else { tax = income * 0.20; } } else { tax = income * 0.10; } printf("Tax Amount: $%.2f\n", tax); return 0; }
Water Bill Calculation
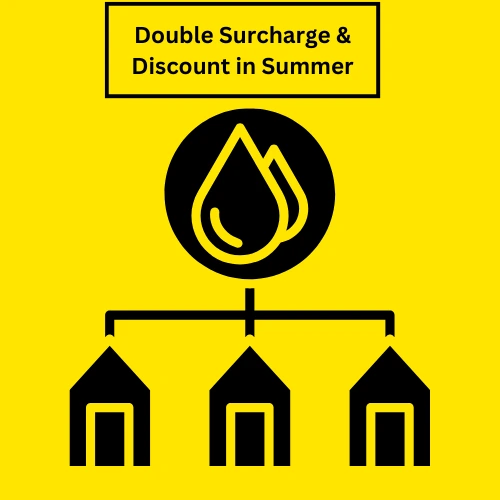
Imagine that you are writing a program for a utility company to calculate water bills based on a household’s water consumption, family size, and the current season. During the summer, if the water consumption per person is more than 100 liters per day, a 20% surcharge is applied. If the consumption per person is between 50 and 100 liters per day, a flat summer fee of $10 is added. If the consumption per person is less than 50 liters per day, a 10% discount is applied. For all other seasons, the surcharge and discount rates are reduced to half, and no flat fee is added. Write a C program to take the total water consumption, family size, and current season as input and determine whether the bill should include a surcharge, a discount, or any additional fees.
Test Case
Input
Enter total water consumption (liters/day): 600
Enter number of residents: 5
Enter base bill amount ($): 100
Enter season (1 for summer, 2 for other seasons): 1
Expected Output
Final Bill Amount: $120.00
- The program starts by asking the user to input the total water consumption (in liters per day), the number of residents in the household, the base bill amount (in dollars), and the current season. These inputs are stored in
totalConsumption
,residents
,bill
, andseason
variables respectively. - The program then calculates the per-person water consumption by dividing the total water consumption by the number of residents and stores it in
perPersonConsumption
. - An
if
condition checks if theseason
is summer (1). - If true, another condition checks if the
perPersonConsumption
is more than 100 liters. - If this is true, a 20% surcharge is applied to the
bill
. - If the
perPersonConsumption
is between 50 and 100 liters, the program adds a flat fee of $10 to thebill
. - Otherwise, if the
perPersonConsumption
is less than 50 liters, a 10% discount is applied to thebill
. - If the
season
is not summer (any value ofseason
other than 1), then theelse
block is executed. - Inside this block, another
if
condition checks if theperPersonConsumption
is more than 100 liters. If this is true, a 10% surcharge is applied to thebill
, which is half of the surcharge applied during summer. - If the
perPersonConsumption
is between 50 and 100 liters, thebill
remains unchanged. - Otherwise, if the
perPersonConsumption
is less than 50 liters, a 5% discount is applied to thebill
, which is half of the discount applied during summer. - Finally, the program prints the final
bill
amount after applying any applicable surcharge, flat fee, or discount, and then ends.
#include <stdio.h> int main() { float totalConsumption; float bill; float perPersonConsumption; int residents; int season; printf("Enter total water consumption (liters/day): "); scanf("%f", &totalConsumption); printf("Enter number of residents: "); scanf("%d", &residents); printf("Enter base bill amount ($): "); scanf("%f", &bill); printf("Enter season (1 for summer, 2 for other seasons): "); scanf("%d", &season); perPersonConsumption = totalConsumption / residents; if (season == 1) { if (perPersonConsumption > 100) { bill = bill + (bill * 0.20); } else { if (perPersonConsumption >= 50) { bill = bill + 10; } else { bill = bill - (bill * 0.10); } } } else { if (perPersonConsumption > 100) { bill = bill + (bill * 0.10); } else { if (perPersonConsumption >= 50) { bill=bill; } else { bill = bill - (bill * 0.05); } } } printf("Final Bill Amount: $%.2f\n", bill); return 0; }
Fuel Efficiency Categorization
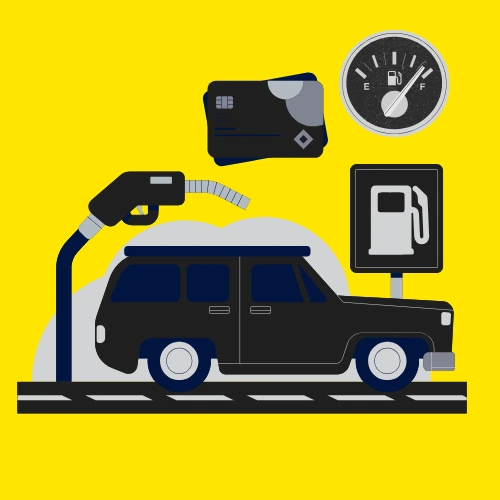
Imagine that you are writing a program to determine a vehicle’s fuel efficiency category based on its mileage and the amount of fuel used. If the vehicle’s mileage is more than 20 km/l and the fuel used is less than 40 litres, it is categorized as “Highly Efficient.” If the mileage is more than 15 km/l but less than 20 km/l , and the fuel used is between 40 and 60 litres, it is categorized as “Moderately Efficient.” In all other cases, the vehicle is categorized as “Low Efficiency.” Write a C program to take the vehicle’s mileage and fuel used as input and determine its fuel efficiency category.
Test Case
Input
Enter mileage (km/l): 18
Enter fuel used (liters): 45
Expected Output
Moderately Efficient.
- The program begins by prompting the user to input the mileage of the vehicle and the amount of fuel used, stored in
mileage
andfuelUsed
. The program uses nested if-else to determine the efficiency category. - An
if
statement checks if themileage
is above 20. - If true, another
if
condition checks if thefuelUsed
is less than 40. - If yes, it prints “
Highly Efficient.
” Otherwise, it prints “Moderately Efficient.
“ - If
mileage
is between 15 to 20, another nestedif
checks if thefuelUsed
is between 40 and 60 liters. - If true, it prints “
Moderately Efficient.
” Otherwise, it prints “Low Efficiency.
“ - If neither condition is met, the last
else
block is executed and the program directly prints “Low Efficiency.
“
#include <stdio.h> int main() { float mileage; float fuelUsed; printf("Enter mileage (km/l): "); scanf("%f", &mileage); printf("Enter fuel used (liters): "); scanf("%f", &fuelUsed); if (mileage > 20) { if (fuelUsed < 40) { printf("Highly Efficient.\n"); } else { printf("Moderately Efficient.\n"); } } else if (mileage > 15 && mileage <= 20) { if (fuelUsed >= 40 && fuelUsed <= 60) { printf("Moderately Efficient.\n"); } else { printf("Low Efficiency.\n"); } } else { printf("Low Efficiency.\n"); } return 0; }
Damage Calculation Program for a Fantasy Game
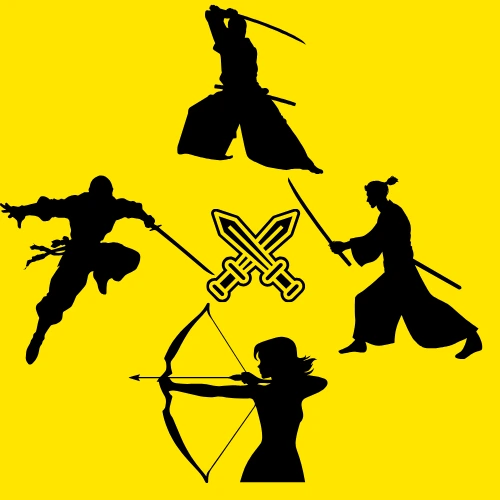
Imagine that you are writing a program for a fantasy game to calculate the damage dealt by a character based on their weapon type and whether they land a critical hit. If the weapon type is “Sword,” the base damage is 50. If the weapon type is “Bow,” the base damage is 30. If a critical hit is landed, the base damage is multiplied by 1.5. If no critical hit is landed, the damage remains unchanged. Write a C program to take the weapon type and whether a critical hit occurred as input and calculate the total damage dealt by the character.
Test Case
Input
Enter weapon type (1 for Sword, 2 for Bow): 1
Did you land a critical hit? (1 for Yes, 0 for No): 1
Expected Output
Damage dealt: 75.00
- The program begins by asking the user to enter the weapon type and whether they landed a critical hit. The weapon type is stored in the
weaponType
variable, and the critical hit status is stored in thecriticalHit
variable. - First, the program checks the
weaponType
. If theweaponType
is Sword(weaponType == 1)
, it sets the basedamage
to 50. If theweaponType
is Bow(weaponType == 2)
, it sets the basedamage
to 30. If neither condition is true, then theelse
block is executed and the program prints “Invalid weapon type” and ends the program. - Within each
weaponType
condition, the program checks whether a critical hit occurred using the condition(criticalHit == 1)
. If this condition is true, the program multiplies the basedamage
by 1.5. Otherwise, the basedamage
remains unchanged. - Finally, the program prints the total
damage
dealt using aprintf
statement.
#include <stdio.h> int main() { int weaponType; int criticalHit; float damage; printf("Enter weapon type (1 for Sword, 2 for Bow): "); scanf("%d", &weaponType); printf("Did you land a critical hit? (1 for Yes, 0 for No): "); scanf("%d", &criticalHit); if (weaponType == 1) { damage = 50; if (criticalHit == 1) { damage = damage * 1.5; } } else if (weaponType == 2) { damage = 30; if (criticalHit == 1) { damage = damage * 1.5; } } else { printf("Invalid weapon type.\n"); return 1; } printf("Damage dealt: %.2f\n", damage); return 0; }
Penalty Kick Success Prediction
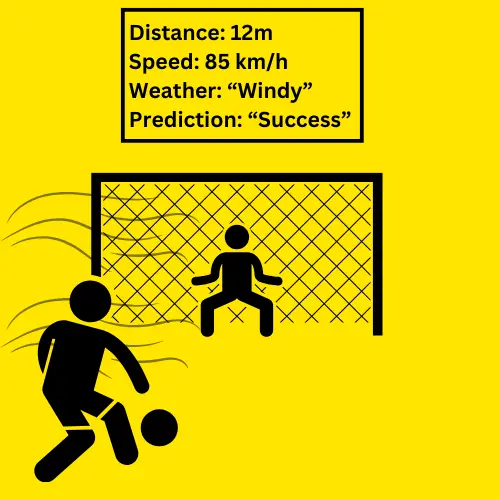
Imagine that you are writing a program for a soccer game to determine whether a penalty kick is successful based on the distance of the shot, the speed of the ball, and the weather conditions. In rainy weather, the shot always results in a miss. In clear weather, the required speed for distances less than 10 meters is 50 km/h, and for distances of 10 meters or more, it starts at 70 km/h and increases by 2 km/h for every meter beyond 10. In windy weather, the required speed is 10 km/h higher in all cases: 60 km/h for distances less than 10 meters and 80 km/h plus 2 km/h for every meter beyond 10 meters.
Write a program in C language that takes the distance of the shot, the speed of the ball, and the weather conditions as input and determines whether the penalty kick is successful or missed.
Test Case
Input
Enter the distance of the shot (in meters): 12
Enter the speed of the ball (in km/h): 85
Enter the weather condition (1 for clear, 2 for windy, 3 for rainy): 2
Expected Output
Penalty Kick Outcome: Successful
- The program starts by asking the user to enter the distance of the shot, the speed of the ball, and the weather condition (1 for clear, 2 for windy, 3 for rainy). These inputs are saved in the variables
distance
,speed
, andweather
respectively. - First, the program checks if the
weather
condition is rainy(weather == 3)
. If this condition is true, the program outputs that the shot is missed due to rain. - If the
weather
condition is not rainy, the program checks if theweather
is clear(weather == 1)
. - If the
weather
is clear and thedistance
is less than 10 meters(distance < 10)
, the program checks whether thespeed
is greater than 50 km/h(speed > 50)
. - When the
speed
is greater than 50 km/h, the shot is successful otherwise, the shot is missed. - If the
distance
is 10 meters or more, the program calculates the required speed as70 + (distance - 10) * 2
and stores it inrequiredSpeed
variable. Then it checks whether the ball’sspeed
exceeds this value. - When the
speed
exceeds therequiredSpeed
, the shot is successful, otherwise, the shot is missed. - If the
weather
is windy(weather == 2)
, the program follows similar steps but with adjustedspeed
thresholds due to wind. - If the
distance
is less than 10 meters, the program checks whether thespeed
is greater than 60 km/h(speed > 60)
. - When the
speed
is greater than 60 km/h, the shot is successful, otherwise, the shot is missed. - If the
distance
is 10 meters or more, the program calculates therequiredSpeed
as80 + (distance - 10) * 2
and checks whether the ball’sspeed
exceeds this value. - When the
speed
exceeds therequiredSpeed
, the shot is successful, otherwise, the shot is missed. - Finally, if the user enters a
weather
condition other than 1, 2, or 3, the program outputs an error message indicating that theweather
condition is invalid.
#include <stdio.h> int main() { float distance; float speed; int weather; float requiredSpeed; printf("Enter the distance of the shot (in meters): "); scanf("%f", &distance); printf("Enter the speed of the ball (in km/h): "); scanf("%f", &speed); printf("Enter the weather condition (1 for clear, 2 for windy, 3 for rainy): "); scanf("%d", &weather); if (weather == 3) { printf("Penalty Kick Outcome: Missed (due to rain)\n"); } else { if (weather == 1) { if (distance < 10) { if (speed > 50) { printf("Penalty Kick Outcome: Successful\n"); } else { printf("Penalty Kick Outcome: Missed\n"); } } else { requiredSpeed = 70 + (distance - 10) * 2; if (speed > requiredSpeed) { printf("Penalty Kick Outcome: Successful\n"); } else { printf("Penalty Kick Outcome: Missed\n"); } } } else if (weather == 2) { if (distance < 10) { if (speed > 60) { printf("Penalty Kick Outcome: Successful\n"); } else { printf("Penalty Kick Outcome: Missed\n"); } } else { requiredSpeed = 80 + (distance - 10) * 2; if (speed > requiredSpeed) { printf("Penalty Kick Outcome: Successful\n"); } else { printf("Penalty Kick Outcome: Missed\n"); } } } else { printf("Invalid weather condition entered.\n"); } } return 0; }
Construction Cost Calculation
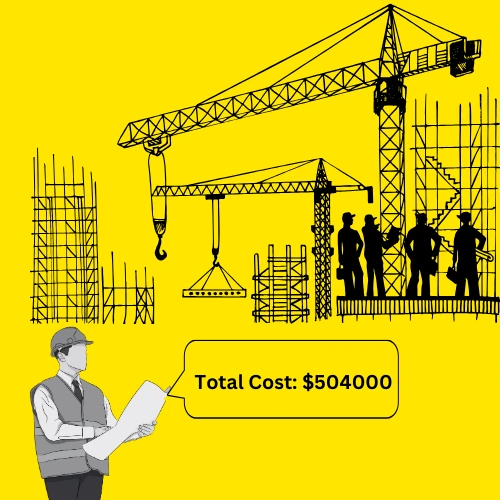
Imagine that you are writing a program for a construction project to calculate the total cost based on the type of material used and the total area of construction. If the material type is “Standard” and the area is less than 500 square meters, the cost is determined by multiplying the area by 1200 and adding 5% of that value as an additional cost for wastage. For “Standard” material with an area of 500 square meters or more, the cost is calculated by multiplying the area by 1200 and adding 3% of that value for wastage. If the material type is “Premium” and the area is less than 500 square meters, the cost is calculated by multiplying the area by 1800 and adding 7% of that value for wastage. For “Premium” material with an area of 500 square meters or more, the cost is determined by multiplying the area by 1800 and adding 5% of that value for wastage. Write a C program to take the material type and total construction area as input and calculate the total construction cost.
Test Case
Input
Enter material type (1 for Standard, 2 for Premium): 1
Enter the area of construction (in square meters): 400
Expected Output
Total construction cost: 504000.00
- The program begins by asking the user to enter the material type and the area of construction. The material type is entered as 1 for “Standard” and 2 for “Premium,” and these values are stored in the variable
materialType
. The area is stored in the variablearea
. - The program then checks the
materialType
using the condition(materialType == 1)
. If this condition is true, it means that “Standard” material is being used. The program further checks thearea
. - If the
area
is less than 500 square meters(area < 500)
, thecost
is calculated using the formula,cost = (area * 1200) + (area * 0.05 * 1200)
. This covers the base rate of 1200 per square meter along with an extra 5% for wastage. - If the
area
is 500 square meters or more, thecost
is calculated using the formula,cost = (area * 1200) + (area * 0.03 * 1200)
. Here, the wastagecost
is reduced to 3%. - If the
materialType
is “Premium” (materialType == 2
), the program checks the area again. - If the
area
is less than 500 square meters(area < 500)
, thecost
is calculated using the formula,cost = (area * 1800) + (area * 0.07 * 1800)
.This includes a base rate of 1800 per square meter and a higher wastagecost
of 7%. - If the
area
is 500 square meters or more, thecost
is calculated using the formula,cost = (area * 1800) + (area * 0.05 * 1800)
.Here, the wastagecost
is slightly reduced to 5%. - Finally, if an invalid
materialType
is entered (any value other than 1 or 2), the program prints an error message and exits. For valid inputs, the total constructioncost
is printed using aprintf
statement.
#include <stdio.h> int main() { int materialType; float area; float cost; printf("Enter material type (1 for Standard, 2 for Premium): "); scanf("%d", &materialType); printf("Enter the area of construction (in square meters): "); scanf("%f", &area); if (materialType == 1) { if (area < 500) { cost = (area * 1200) + (area * 0.05 * 1200); } else { cost = (area * 1200) + (area * 0.03 * 1200); } } else if (materialType == 2) { if (area < 500) { cost = (area * 1800) + (area * 0.07 * 1800); } else { cost = (area * 1800) + (area * 0.05 * 1800); } } else { printf("Invalid material type.\n"); return 1; } printf("Total construction cost: %.2f\n", cost); return 0; }
Time Management Challenge Game
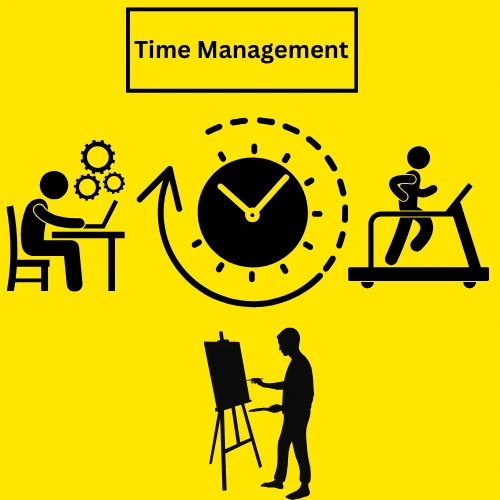
Imagine that you are writing a program for a time management challenge game where players have 24 hours to distribute among three tasks, “Work,” “Exercise,” and “Fun.” The player’s score is determined by specific rules. If the hours allocated to “Work” exceed 10 and “Exercise” hours are less than 2, the player loses 5 points for neglecting health. If “Exercise” hours are 2 or more but “Fun” hours are less than 1, the player gains 10 points for fitness but loses 2 points for stress. If the tasks are well balanced with “Work” hours between 6 and 8, “Exercise” hours at least 2, and “Fun” hours at least 1, the player earns 20 points for perfect balance. If none of these conditions are met, the player gains 5 points simply for participation. Write a C program to take the hours allocated to each task as input and calculate the player’s score based on these rules.
Test Case
Input
Enter hours allocated to Work: 7
Enter hours allocated to Exercise: 3
Enter hours allocated to Fun: 2
Expected Output
Total score: 20
- The program begins by asking the user to enter the number of hours allocated to “Work,” “Exercise,” and “Fun.” These values are stored in the variables
workHours
,exerciseHours
, andfunHours
, respectively. - The program first checks if
workHours
is greater than 10(workHours > 10)
. If this condition is true, it further checks theexerciseHours
. - If exerciseHours is less than 2
(exerciseHours < 2)
, the player loses 5 points for neglecting health. - If exerciseHours is 2 or more
(exerciseHours >= 2)
, the program checksfunHours
. - If
funHours
is less than 1(funHours < 1)
, the player gains 10 points for fitness but loses 2 points for stress, resulting in a net gain of 8 points. - If
workHours
is between 6 and 8(workHours >= 6 && workHours <= 8)
, then the program checks for a balance. - If
exerciseHours
is at least 2(exerciseHours >= 2)
andfunHours
is at least 1(funHours >= 1)
, the player gains 20 points for a perfect balance. - If none of the above conditions are met, then the last
else
block is executed and the player gains 5 points for participation. - Finally, the program prints the total score using a
printf
statement
#include <stdio.h> int main() { float workHours; float exerciseHours; float funHours; int score = 0; printf("Enter hours allocated to Work: "); scanf("%f", &workHours); printf("Enter hours allocated to Exercise: "); scanf("%f", &exerciseHours); printf("Enter hours allocated to Fun: "); scanf("%f", &funHours); if (workHours > 10) { if (exerciseHours < 2) { score -= 5; } else if (exerciseHours >= 2) { if (funHours < 1) { score += 10; score -= 2; } } } else if (workHours >= 6 && workHours <= 8) { if (exerciseHours >= 2 && funHours >= 1) { score += 20; } } else { score += 5; } printf("Total score: %d\n", score); return 0; }
Car Customization
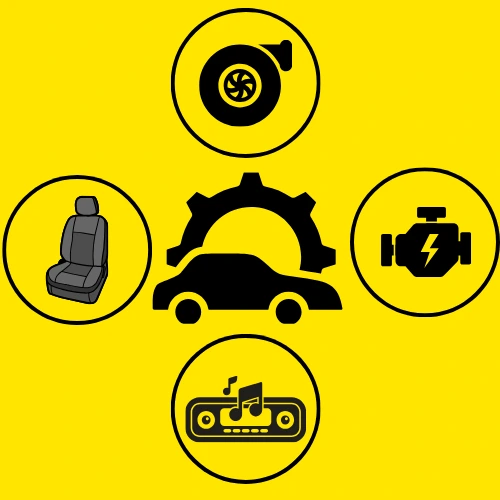
Imagine you are creating a program for a car customization shop to calculate the total cost based on the upgrades a customer selects. If the customer chooses an engine upgrade, it costs $5000, and adding a turbo boost increases the total cost by $2000. For an interior upgrade, the cost is $3000, with an extra $1500 if luxury seats are included. If the customer only adds premium audio without luxury seats, it costs an extra $1000. When both engine and interior upgrades are selected, the customer gets a $1000 discount. If no upgrades are chosen, a basic inspection fee of $500 is applied. Write a C program to calculate the total cost based on the customer’s choices.
Test Case
Input
Do you want an Engine Upgrade? (1 for Yes, 0 for No): 1
Do you want a Turbo Boost? (1 for Yes, 0 for No): 1
Do you want an Interior Upgrade? (1 for Yes, 0 for No): 1
Do you want Luxury Seats? (1 for Yes, 0 for No): 1
Do you want Premium Audio? (1 for Yes, 0 for No): 0
Expected Output
Total cost of customization: $10500
- The program begins by asking the user to input their choices for upgrades, whether they want an “Engine Upgrade,” “Turbo Boost,” “Interior Upgrade,” “Luxury Seats,” or “Premium Audio.” The user inputs are stored in integer variables
engineUpgrade
,turboBoost
,interiorUpgrade
,luxurySeats
andpremiumAudio
respectively, where1
indicates “Yes” and0
indicates “No.” - First, the program checks if the
engineUpgrade
is selected(engineUpgrade == 1)
usingif
condition. If yes, $5000 is added to the totalcost
. Inside this block, anotherif
condition is used to check ifturboBoost
is also selected(turboBoost == 1)
. If this is also true, another $2000 is added to thecost
. - Next, the program checks if the
interiorUpgrade
is selected(interiorUpgrade == 1)
. If yes, $3000 is added to the totalcost
. Then, it checks forluxurySeats
(luxurySeats == 1)
using a nestedif
condition. IfluxurySeats
are also selected by the user, $1500 is added to the totalcost
. If theluxurySeats
are not selected, then theelse if
condition checks forpremiumAudio
(premiumAudio == 1)
, and $1000 is added if the condition is true. - Another
if
condition is used to check if bothengineUpgrade
andinteriorUpgrade
are selected(engineUpgrade == 1 && interiorUpgrade == 1)
. If this condition is true, then a discount of $1000 is applied to the totalcost
. - If no upgrades are selected
(cost == 0)
, a basic inspection fee of $500 is added to the totalcost
. - finally, the total
cost
is printed using theprintf
function.
#include <stdio.h> int main() { int engineUpgrade, turboBoost, interiorUpgrade, luxurySeats, premiumAudio; int cost = 0; printf("Do you want an Engine Upgrade? (1 for Yes, 0 for No): "); scanf("%d", &engineUpgrade); printf("Do you want a Turbo Boost? (1 for Yes, 0 for No): "); scanf("%d", &turboBoost); printf("Do you want an Interior Upgrade? (1 for Yes, 0 for No): "); scanf("%d", &interiorUpgrade); printf("Do you want Luxury Seats? (1 for Yes, 0 for No): "); scanf("%d", &luxurySeats); printf("Do you want Premium Audio? (1 for Yes, 0 for No): "); scanf("%d", &premiumAudio); if (engineUpgrade == 1) { cost += 5000; if (turboBoost == 1) { cost += 2000; } } if (interiorUpgrade == 1) { cost += 3000; if (luxurySeats == 1) { cost += 1500; } else if (premiumAudio == 1) { cost += 1000; } } if (engineUpgrade == 1 && interiorUpgrade == 1) { cost -= 1000; } if (cost == 0) { cost += 500; } printf("Total cost of customization: $%d\n", cost); return 0; }
Dragon Quest
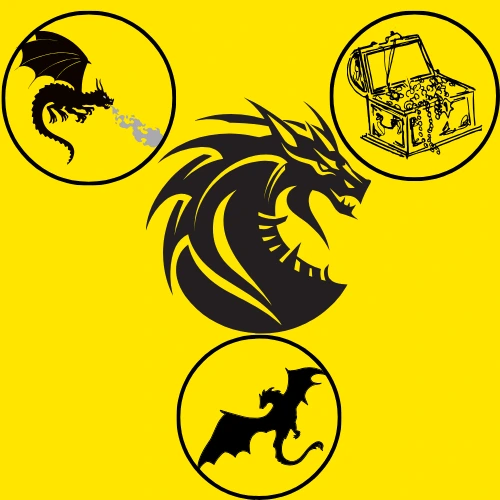
Imagine you are creating a program for a Dragon Quest game where the player decides how to allocate their dragon’s 100 energy points across three activities: flying, combat training, and treasure hunting. The journey’s success depends on how the energy is distributed. If more than 50 points are given to flying and more than 30 to combat training, the dragon gets exhausted, reducing the success score by 20 points. If flying gets between 30 and 50 points and treasure hunting gets at least 20 points, the success score increases by 25 points. If more than 40 points are allocated to combat training, the success score increases by 30 points, but if it gets between 20 and 40 points, the success score increases by 15 points. However, if the total energy allocated is not exactly 100, the player gets a penalty of 50 points. Write a C program to calculate the success score based on how the energy is allocated.
Test Case
Input
Enter energy points for flying: 40
Enter energy points for combat training: 25
Enter energy points for treasure hunting: 35
Expected Output
Success score: 40
- The program first asks the player to enter how many energy points they want to assign to flying, combat training, and treasure hunting. These inputs are stored in the variables
flying
,combat
, andtreasure
variables respectively. - The program calculates the total energy by adding
flying
,combat
, andtreasure
and stores it intotalEnergy
variable. - If the
totalEnergy
is not exactly 100, the program applies a penalty of 50 points to thesuccessScore
(successScore -= 50)
. - If the
totalEnergy
is valid (equal to 100), the program checks ifflying
has more than 50 points andcombat
training has more than 30 points using two if conditions. If both these conditions are true, the dragon gets tired, and thesuccessScore
is reduced by 20 points(successScore -= 20)
. - Next, the program checks if
flying
has between 30 and 50 points andtreasure
hunting has at least 20 points. If this condition is true, thesuccessScore
increases by 25 points(successScore += 25)
. - The program then checks the points of
combat
training. If it is more than 40 points, thesuccessScore
increases by 30 points(successScore += 30)
. - If it is between 20 and 40 points, the
successScore
increases by 15 points(successScore += 15)
. - After processing all the conditions, the program prints the final
successScore
usingprintf
.
#include <stdio.h> int main() { int flying, combat, treasure; int successScore = 0; printf("Enter energy points for flying: "); scanf("%d", &flying); printf("Enter energy points for combat training: "); scanf("%d", &combat); printf("Enter energy points for treasure hunting: "); scanf("%d", &treasure); int totalEnergy = flying + combat + treasure; if (totalEnergy != 100) { successScore -= 50; } else { if (flying > 50) { if (combat > 30) { successScore -= 20; } } else if (flying >= 30 && flying <= 50) { if (treasure >= 20) { successScore += 25; } } if (combat > 40) { successScore += 30; } else if (combat >= 20 && combat <= 40) { successScore += 15; } } printf("Success score: %d\n", successScore); return 0; }
Futuristic City Energy Distribution
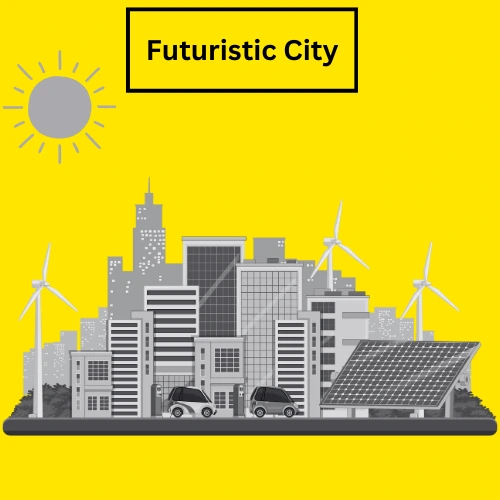
Imagine you are managing the energy resources for a futuristic city during a critical 24-hour operation. The city uses three energy sources: solar, wind, and nuclear. Your task is to distribute the energy load efficiently to avoid a blackout and achieve maximum performance. Solar energy must provide at least 30% of the total energy. If solar energy exceeds 50%, nuclear energy usage must be reduced by 10%. If solar energy is below 30%, a penalty of 20 points is applied for insufficient solar energy. If wind energy exceeds 40% when solar energy is below 30%, an additional penalty of 5 points is applied. If nuclear energy also exceeds 50% during this condition, another 5 points are deducted. Wind energy must contribute between 20% and 40% of the total energy. If wind energy exceeds 40%, a penalty of 15 points is applied to the performance score. If solar energy is also below 30%, another 10 points are deducted. If nuclear energy is below 20%, an additional 10 points are deducted due to insufficient backup. If wind energy is below 20%, and nuclear energy exceeds 30%, another 10 points are deducted. Nuclear energy must not exceed 50%. If nuclear energy is between 30% and 50%, the performance score drops by 10 points. If wind energy is below 20% and solar energy is below 30%, an additional 15 points are deducted for insufficient load balancing. If nuclear energy exceeds 50%, the performance score drops by 25 points due to a safety limit breach. The total energy from solar, wind, and nuclear must add up to exactly 100%. If this condition is not met, the system will trigger an emergency shutdown, deducting 50 points from the performance score. Write a C program to calculate the final performance score based on how the energy is distributed across these sources.
Test Case
Input
Enter percentage of energy from Solar: 25
Enter percentage of energy from Wind: 50
Enter percentage of energy from Nuclear: 25
Expected Output
Final Performance Score: 50
- The program starts by asking the user to enter the percentage of energy from solar, wind, and nuclear sources. These inputs are stored in the variables
solar
,wind
, andnuclear
, respectively. - The
performanceScore
is initialized with 100 points. - The program then calculates the
totalEnergy
by summing the values ofsolar
,wind
, andnuclear
. - First, an
if
condition is used to check whether thetotalEnergy
is equal to 100%. If this condition is false(totalEnergy != 100)
, the program deducts 50 points from theperformanceScore
for an emergency shutdown. - If this condition is true , the program proceeds to evaluate each energy sources.
- The program then checks whether
solar
energy is at least 30%(solar >= 30)
. - If this condition is true, the program checks if
solar
energy exceeds 50%(solar > 50)
. - If
solar
energy exceeds 50%,nuclear
energy is reduced by 10% to maintain balance. - If
solar
energy is less than 30%, the program deducts 20 points for insufficientsolar
energy. - It further checks if
wind
energy exceeds 40%(wind > 40)
. - If this condition is true, an additional 5 points are deducted for poor load balancing.
- The program also checks if
nuclear
energy exceeds 50%(nuclear > 50)
. - If true, another 5 points are deducted due to excessive
nuclear
usage. - Next, the program checks whether
wind
energy is between 20% and 40%(wind >= 20 && wind <= 40)
. - If this condition is true, the program adds 10 points as a bonus for optimal
wind
usage. - If
wind
energy exceeds 40%, the program deducts 15 points for excesswind
energy. - It then checks if
solar
energy is below 30%(solar < 30)
. If true, another 10 points are deducted. - The program further checks if
nuclear
energy is below 20%(nuclear < 20)
. - If true, another 10 points are deducted due to insufficient
nuclear
backup. - If
wind
energy is below 20%(wind < 20)
, the program checks ifnuclear
energy exceeds 30%(nuclear > 30)
. If true, 10 points are deducted due to insufficientwind
support. - The program then evaluates
nuclear
energy. - If
nuclear
energy is 50% or less(nuclear <= 50)
, the program checks ifnuclear
energy is between 30% and 50%. If true, 10 points are deducted. - It further checks if both
wind
andsolar
energy are low(wind < 20 && solar < 30)
. If true, an additional 15 points are deducted. - If
nuclear
energy exceeds 50% , the program deducts 25 points for exceeding the safety limit. - Finally, the program prints the final performance score using the
printf
function.
#include <stdio.h> int main() { float solar; float wind; float nuclear; int performanceScore = 100; printf("Enter percentage of energy from Solar: "); scanf("%f", &solar); printf("Enter percentage of energy from Wind: "); scanf("%f", &wind); printf("Enter percentage of energy from Nuclear: "); scanf("%f", &nuclear); float totalEnergy = solar + wind + nuclear; if (totalEnergy != 100) { performanceScore -= 50; } else { if (solar >= 30) { if (solar > 50) { nuclear -= nuclear * 0.1; } } else { performanceScore -= 20; if (wind > 40) { performanceScore -= 5; if (nuclear > 50) { performanceScore -= 5; } } } if (wind >= 20 && wind <= 40) { performanceScore += 10; } else if (wind > 40) { performanceScore -= 15; if (solar < 30) { performanceScore -= 10; if (nuclear < 20) { performanceScore -= 10; } } } else if (wind < 20) { if (nuclear > 30) { performanceScore -= 10; } } if (nuclear <= 50) { if (nuclear >= 30) { performanceScore -= 10; if (wind < 20 && solar < 30) { performanceScore -= 15; } } } else { performanceScore -= 25; } } printf("Final Performance Score: %d\n", performanceScore); return 0; }