In Python, packages are the building blocks of your projects. They come with built-in features that save time and effort. However, new versions can sometimes cause issues by changing how your code works. To avoid this, you can install a specific version of a package. In this article, you’ll learn how to use pip
to install a specific version, so you won’t have to worry about the changes introduced in newer package releases.
What is Pip?
Pip (stands for “Pip Installs Packages”) is the default package manager for Python. It allows you to install, upgrade, and manage third-party libraries from the Python Package Index (PyPI). Think of Pip as an app store for Python libraries—whenever you need a new tool or dependency, pip
is your go-to command.
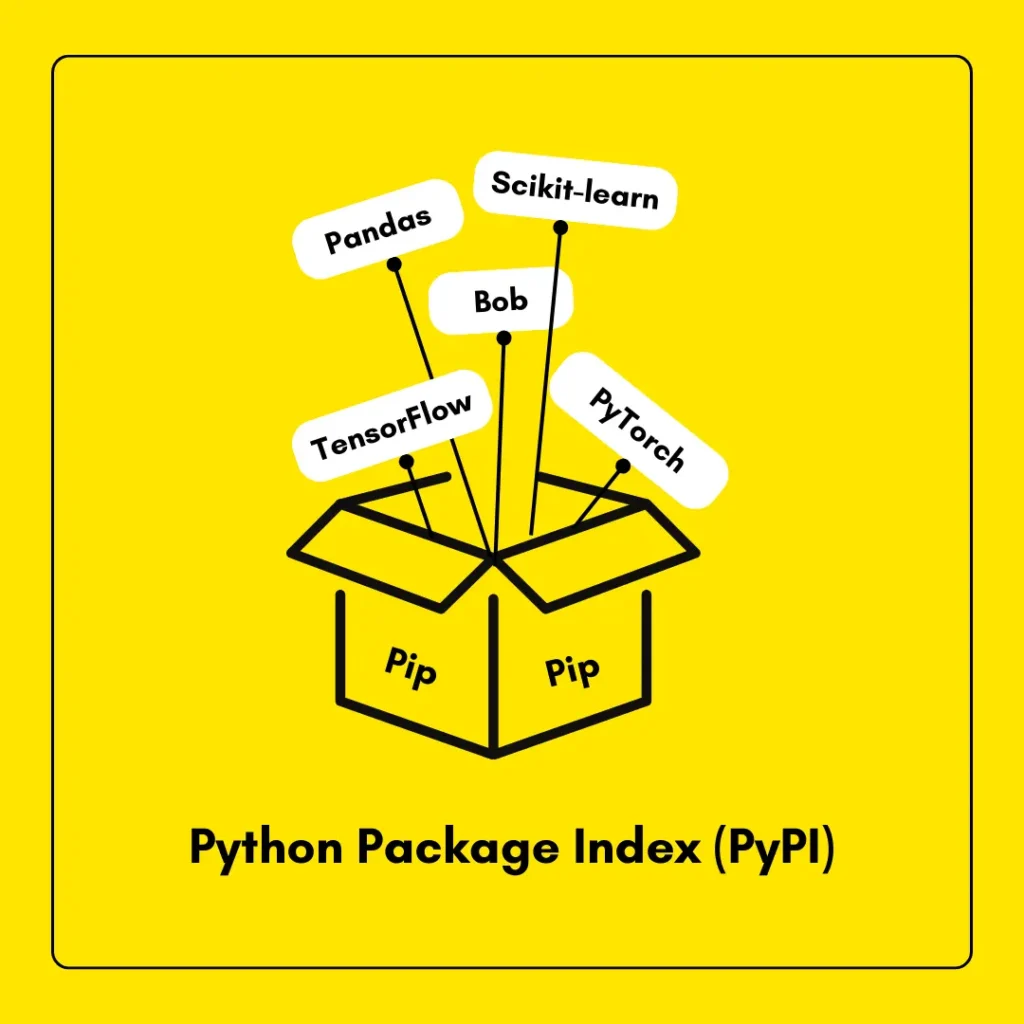
Different Methods to Install a Specific Version of a Package with Pip
Method 1: Using pip install with Version Number
If you know the exact version number, this method is for you. It prevents unwanted updates that might break your code.
Command Syntax
pip install package-name==version-number
Example Command
Let’s say you want to install version 1.2.3 of the requests
package. You would run:
pip install requests==1.2.3
Command Explanation
In this command, requests
is the package name, and 1.2.3
is the exact version you want to install. Pip will fetch that version from PyPI and install it for you. If the version isn’t available or doesn’t exist, Pip will throw an error.
Method 2: Installing a Version Greater or Lesser than a Specific Version
Sometimes, you don’t need an exact version but a version within a certain range. This method is useful when you want a package that’s newer than or older than a specific release.
Command Syntax
For installing version X or higher:
pip install package-name>=version-number
For installing version X or lower:
pip install package-name<=version-number
Example Command
To install a requests
package that is greater than or equal to version 1.2.3 but less than or equal to 1.5.0, you would use:
pip install requests>=1.2.3,<=1.5.0
Command Explanation
Here, Pip will install any version of requests
between 1.2.3
and 1.5.0
, including those exact versions. It ensures that you don’t accidentally upgrade beyond the version you need.
Method 3: Install the Latest Version of a Package
If you’re working on a project that should always stay up-to-date with the latest version of a package, you can use Pip’s --upgrade
flag.
Command Syntax
pip install --upgrade <package-name>
Example Command
To upgrade requests
to the latest version, use:
pip install --upgrade requests
Command Explanation
The --upgrade
flag here tells Pip to check if there’s a newer version of the package requests
installed. If there is, it will replace the older version with the new one. If you’re running the latest version already, Pip will simply let you know.
How to Check the Installed Version of Package with Pip
You may want to confirm that you’ve installed the correct version of a package. Pip provides a command show
to list all installed packages and their versions.
Command Syntax
pip show <package-name>
Example Command
To check the installed version of requests
, you can use:
pip show requests
Command Explanation
In this command, the pip show
gives detailed information about the package, including its version, location, and dependencies.
How to Uninstall a Package with Pip
Sometimes, you may want to remove a package from your environment. Uninstalling a package is as easy as installing one.
Command Syntax
pip uninstall <package-name>
Example Command
To uninstall requests
, you can run the command:
pip uninstall requests
Command Explanation
Here, the pip
uninstall command will remove the requests
package from your Python environment.
This command will ask for confirmation before uninstalling the requests
package. You can also use the -y
flag to skip the confirmation step:
pip uninstall -y requests
Conclusion
You’ve now learned various ways to install, manage, and uninstall specific versions of Python packages using Pip. Whether you’re dealing with package compatibility issues, managing multiple environments, or working on a long-term project, knowing how to install and manage packages will help you make your development process smooth. So, next time you run into a package-related issue, you’ll know exactly how to handle it!
If you want to learn more about Python in a simple, easy-to-understand way, check out our Python series at Syntax Scenarios.