Explore exciting, real-world coding challenges based on pointers in C. This article presents fun, scenario-based problems like swapping cups, decoding secret messages, tracking cricket scores, and navigating submarines, all while mastering pointer operations. Perfect for students and programmers looking to strengthen their C programming skills with interactive examples and step by step explanations. Whether you’re new to pointers or looking for a challenge, these problems will enhance your understanding in a fun and practical way
Swapping Cups
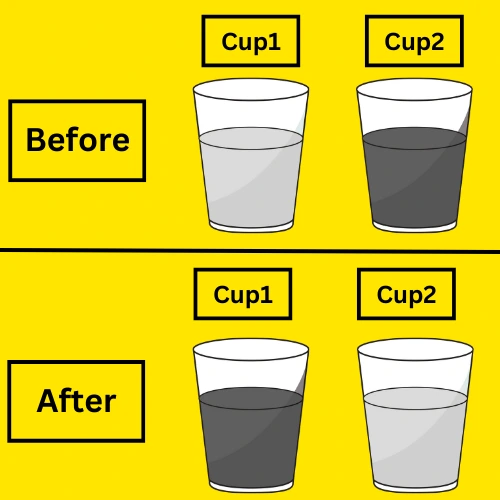
You are performing a magic trick where you swap the contents of two cups without touching them directly. Write a C program that takes two integers as input, representing the contents of two cups, and swaps their values using pointers.
Test Case
Input
Enter contents of cup1: 5
Enter contents of cup2: 10
Expected Output
Before swapping:
Cup1: 5
Cup2: 10
After swapping:
Cup1: 10
Cup2: 5
- The program starts by asking the user to enter two integer values, which are stored in
cup1
andcup2
variables. - Two integer pointers
ptr1
andptr2
are assigned to store the addresses ofcup1
andcup2
. - Before swapping, the program prints the initial values of the cups using pointers
*ptr1
and*ptr2
. - A temporary variable
temp
stores the value at*ptr1
. - The value at
*ptr2
is assigned to*ptr1
. - The value stored in
temp
is assigned to*ptr2
. - After swapping, the updated values are printed using pointers.
#include <stdio.h> int main() { int cup1, cup2; int *ptr1, *ptr2; printf("Enter contents of cup1: "); scanf("%d", &cup1); printf("Enter contents of cup2: "); scanf("%d", &cup2); ptr1 = &cup1; ptr2 = &cup2; printf("Before swapping:\n"); printf("Cup1: %d\n", *ptr1); printf("Cup2: %d\n", *ptr2); int temp = *ptr1; *ptr1 = *ptr2; *ptr2 = temp; printf("After swapping:\n"); printf("Cup1: %d\n", *ptr1); printf("Cup2: %d\n", *ptr2); return 0; }
Finding the Secret Message Length
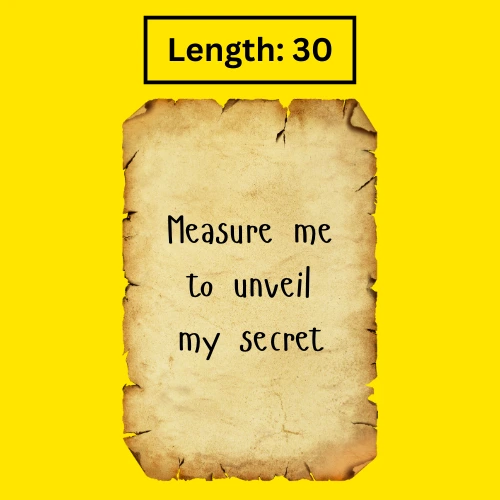
Imagine you found a secret message on an old parchment and need to determine its length using pointers. Write a C program that takes a string as input and calculates its length using pointers.
Test Case
Input
Enter a secret message: HelloWorld
Expected Output
The length of the message is: 10
- The program starts by asking the user to enter a string. The input is stored in a character array called
message
. - A pointer
ptr
is initialized to the starting address of the stringmessage
. - A loop iterates through the string using the pointer.
- The pointer starts at the first character of the string.
- It moves forward one character at a time (
ptr++
). - Each time the pointer moves, the length counter
length
is increased. - The loop continues until the null character (
\0
) is reached. - Finally, the
length
of the string is printed.
#include <stdio.h> int main() { char message[100]; char *ptr; int length = 0; printf("Enter a secret message: "); scanf("%s", message); ptr = message; while (*ptr != '\0') { length++; ptr++; } printf("The length of the message is: %d\n", length); return 0; }
The Rising Temperatures
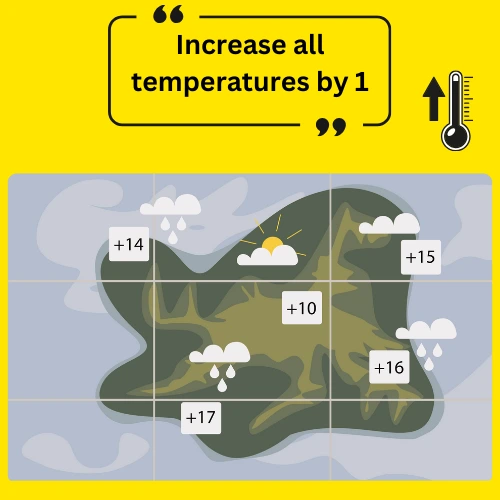
A weather station records temperature readings at different times of the day. However, due to an error, all readings need to be increased by 1°C. Write a program in C language that takes five temperature readings as input, modifies them by increasing each by 1°C using pointers, and prints the updated values
Test Case
Input
Enter 5 temperature readings:
25
30
28
31
29
Expected Output
Updated Temperatures:
26 31 29 32 30
- The program starts by asking the user to enter five temperature readings. These values are stored in an integer array called
temps
. - A pointer variable
ptr
is declared and initialized to point to the first element of thetemps
array. - A
for
loop is used to take input for the five temperature readings. - In each iteration,
scanf("%d", ptr + i);
is used to store the user input at the corresponding memory location. ptr + i
moves the pointer to thei
-th element of the array.- After the input is taken, the program modifies each temperature value by increasing it by 1°C using another
for
loop. - Inside the loop,
(*(ptr + i))++;
is used to increment the value stored at the memory address pointed to byptr + i
.ptr + i
moves the pointer to thei
-th element of the array.*(ptr + i)
accesses the value stored at that memory location.(*(ptr + i))++
increases that value by 1°C.
- Finally, another
for
loop prints the updated temperatures using pointer dereferencing.
#include <stdio.h> int main() { int temps[5], i; int *ptr = temps; printf("Enter 5 temperature readings:\n"); for (i = 0; i < 5; i++) { scanf("%d", ptr + i); } for (i = 0; i < 5; i++) { (*(ptr + i))++; } printf("Updated Temperatures:\n"); for (i = 0; i < 5; i++) { printf("%d ", *(ptr + i)); } return 0; }
Finding the Highest Score
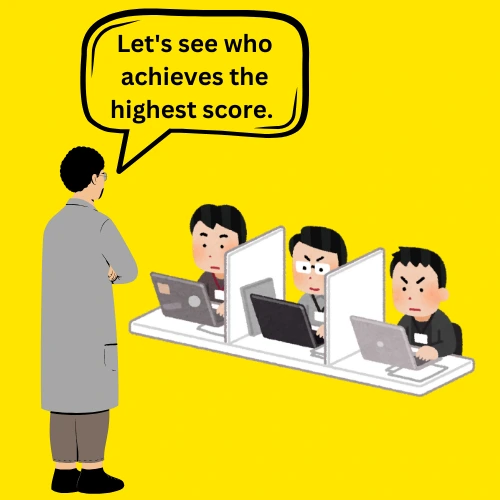
A school is conducting a programming competition where five students have participated. Each student is given a score based on their performance. Write a C program that takes five scores as input and determines the highest score using pointers.
Test Case
Input
Enter 5 student scores:
78
92
85
67
99
Expected Output
The highest score is: 99
- The program starts by asking the user to enter five student scores. These values are stored in an integer array called
scores
. - Next, a pointer variable
ptr
is declared and initialized to point to the first element of thescores
array. - The program initializes the variable
max
with the value of the first element of the array using pointer dereferencing (*ptr
). This variable is used to store the highest score. - A
for
loop is used to iterate through the remaining four scores. - In each iteration,
ptr
is incremented (ptr++
) to move to the next element in the array. - The value at the new pointer location (
*ptr
) is compared withmax
. - If
*ptr
is greater thanmax
, the value ofmax
is updated to*ptr
. - After all five scores have been processed, the program prints the highest score.
#include <stdio.h> int main() { int scores[5], *ptr, max, i; printf("Enter 5 student scores:\n"); for (i = 0; i < 5; i++) { scanf("%d", &scores[i]); } ptr = scores; max = *ptr; for (i = 1; i < 5; i++) { ptr++; if (*ptr > max) { max = *ptr; } } printf("The highest score is: %d\n", max); return 0; }
Counting Heavy and Light Boxes
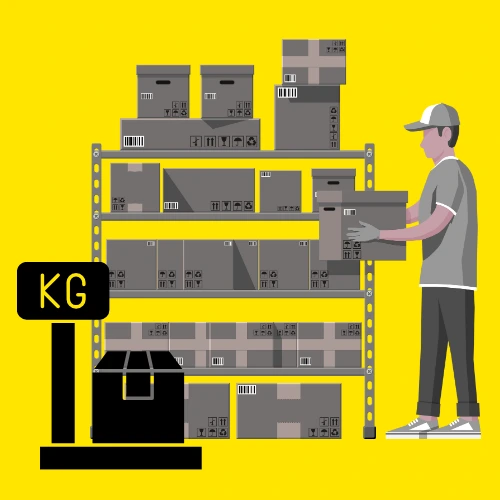
A warehouse manager receives a shipment of boxes with different weights. To keep track of the inventory, the manager wants to count how many boxes have even weights (heavy boxes) and how many have odd weights (light boxes). Write a C program that takes the number of boxes and their weights as input and counts how many of them have even weights and how many have odd weights using pointers.
Test Case
Input
Enter the number of boxes: 6
Enter the weights of 6 boxes:
23
12
9
44
31
18
Expected Output
Total heavy (even weight) boxes: 3
Total light (odd weight) boxes: 3
- The program starts by asking the user to enter the number of boxes. This input is stored in the variable
n
. - An integer array
weights[n]
is declared to store the weights of the boxes. - A pointer variable
ptr
is declared and initialized to point to the first element of theweights
array. This pointer is used to traverse the array. - A
for
loop is used to take the weights ofn
boxes as input. scanf("%d", ptr + i);
is used to store the input values in the array.ptr + i
moves the pointer to thei
-th position, and the input is stored at that memory location.- The program initializes two integer variables,
evenCount
andoddCount
, to 0 to count heavy (even) and light (odd) boxes. - Another
for
loop iterates through alln
weights to determine whether each box is heavy or light. - If
*ptr
is divisible by 2 (*ptr % 2 == 0
), it is a heavy box (even weight), soevenCount
is incremented. - Otherwise, it is a light box (odd weight), so
oddCount
is incremented. - The pointer is incremented (
ptr++
) to move to the next weight. - After counting, the program prints the total number of heavy and light boxes.
#include <stdio.h> int main() { int n, i, evenCount = 0, oddCount = 0; printf("Enter the number of boxes: "); scanf("%d", &n); int weights[n]; int *ptr = weights; printf("Enter the weights of %d boxes:\n", n); for (i = 0; i < n; i++) { scanf("%d", ptr + i); } for (i = 0; i < n; i++) { if (*(ptr + i) % 2 == 0) { evenCount++; } else { oddCount++; } } printf("Total heavy (even weight) boxes: %d\n", evenCount); printf("Total light (odd weight) boxes: %d\n", oddCount); return 0; }
The Mysterious Vault Security System
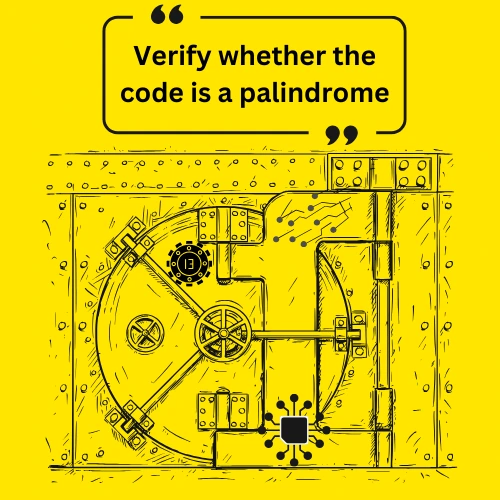
A high-tech vault is protected by a multi-digit security code. However, due to a malfunction, the system can only process the code by accessing its digits through memory without using direct indexing. The security team must verify whether the code is a palindrome, meaning it reads the same forward and backward. Since the vault’s system does not support direct indexing, the team must rely solely on pointer-based operations to access and check the digits. Write a C program that takes a security code as input, checks whether the code is a palindrome, and prints the result using pointers.
Test Case
Input
Enter the number of digits in the security code: 5
Enter the 5-digit security code:
1
2
3
2
1
Expected Output
The security code is a palindrome
- The program starts by asking the user to enter the number of digits in the security code. This value is stored in the variable
n
. - A memory block is used to store the security code, and a pointer
start
is initialized to reference the first digit. Another pointerend
is initialized to reference the last digit. - A
for
loop is used to take input for the digits of the security code. The pointerstart
is incremented (start + i
) to store values sequentially in memory. - To check whether the code is a palindrome, a while loop is used.
- The values at
start
andend
are compared. - If they are not equal, the code is not a palindrome, and the program exits the loop.
- If they match,
start
moves forward (start++
), andend
moves backward (end--
) to check the next pair of digits. - This continues until all digits have been checked or a mismatch is found.
- Finally, the program prints whether the security code is a palindrome or not.
#include <stdio.h> int main() { int n, i, isPalindrome = 1; printf("Enter the number of digits in the security code: "); scanf("%d", &n); int code[n]; int *start = code; int *end; printf("Enter the %d-digit security code:\n", n); for (i = 0; i < n; i++) { scanf("%d", start + i); } end = start + (n - 1); while (start < end) { if (*start != *end) { isPalindrome = 0; break; } start++; end--; } if (isPalindrome) { printf("The security code is a palindrome.\n"); } else { printf("The security code is NOT a palindrome.\n"); } return 0; }
Navigating the Deep-Sea Submarine
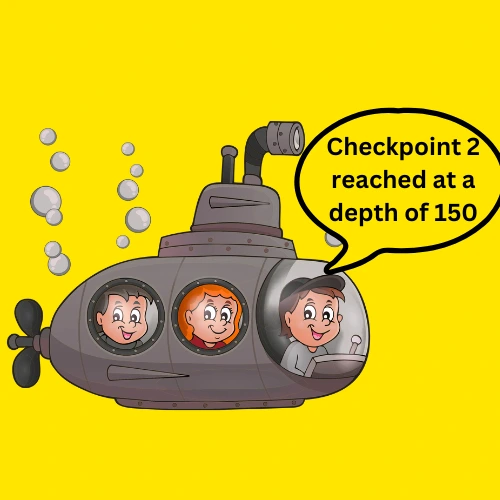
A deep-sea research submarine is navigating through a series of checkpoints in the ocean. Each checkpoint has a unique depth measurement. The submarine logs these depths in memory, but due to limited screen space, it can only display depths in reverse order. Write a C program that takes the number of checkpoints and their respective depths as input, then prints the depths in reverse order using pointers.
Test Case
Input
Enter the number of checkpoints: 4
Enter the depth at each checkpoint:
50
100
150
200
Expected Output
Reversed order of depths:
200 150 100 50
- The program starts by asking the user to enter the number of checkpoints in the ocean. This input is stored in the variable
n
. - Next, the program declares an integer array named
depths
of sizen
to store the depth values at each checkpoint. - A pointer variable
ptr
is assigned the address ofdepths
. - A
for
loop is used to take the depth values for each checkpoint from the user. The loop starts fromi = 0
and continues untili < n
. - In each iteration:
- The pointer expression
ptr + i
moves to thei
-th position in memory. - The
scanf
function stores the depth value at the corresponding memory location.
- The pointer expression
- After all the depth values are entered, the program prints the depths in reverse order using another
for
loop. This loop starts from the last index (i = n - 1
) and iterates backward untili >= 0
. - The pointer expression
*(ptr + i)
retrieves the value at thei
-th position. - The
printf
function prints each depth value, effectively displaying the depths in reverse order. - Once all the depths are printed, the program terminates.
#include <stdio.h> int main() { int n, i; printf("Enter the number of checkpoints: "); scanf("%d", &n); int depths[n]; int *ptr = depths; printf("Enter the depth at each checkpoint:\n"); for (i = 0; i < n; i++) { scanf("%d", ptr + i); } printf("Reversed order of depths:\n"); for (i = n - 1; i >= 0; i--) { printf("%d ", *(ptr + i)); } return 0; }
The Encrypted Scroll
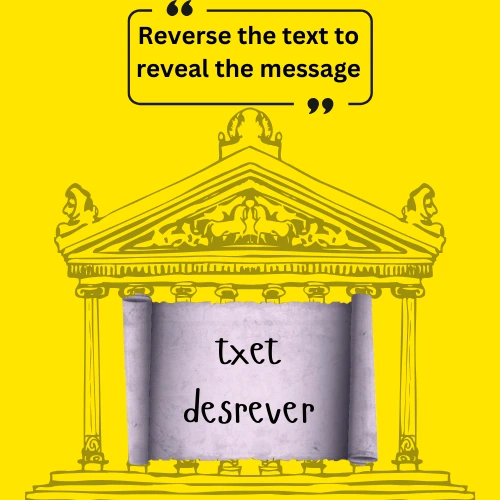
Deep in an ancient temple, an explorer discovers a mysterious encrypted scroll. The text on the scroll appears reversed, making it unreadable. According to legend, the scroll’s contents hold the key to an ancient treasure. The explorer needs a program that can reverse the text to decode the message. However, due to the fragile nature of the scroll, the text must be processed in memory using pointer-based operations, without using indexing. Write a C program that takes a secret message as input, reverses it using only pointers, and prints the decoded message.
Test Case
Input
Enter the encrypted message: drowssap
Expected Output
Decoded message: password
- The program starts by asking the user to enter the encrypted message, which is stored in a character array named
message
. - A pointer variable
start
is assigned the first character of the message, while another pointerend
is assigned the last character. - A
while
loop is used to swap characters in memory without using array indexing. - The loop continues as long as
start
is beforeend
. - The characters at
start
andend
are swapped using a temporary variable. start
moves forward (start++
), andend
moves backward (end--
).- This process continues until all characters have been reversed in memory.
- After the reversal is complete, the program prints the decoded message.
#include <stdio.h> int main() { char message[100], *start, *end, temp; int length = 0; printf("Enter the encrypted message: "); scanf("%s", message); start = message; while (*(start + length) != '\0') { length++; } end = message + (length - 1); while (start < end) { temp = *start; *start = *end; *end = temp; start++; end--; } printf("Decoded message: %s\n", message); return 0; }
The Lost Spaceship Coordinates
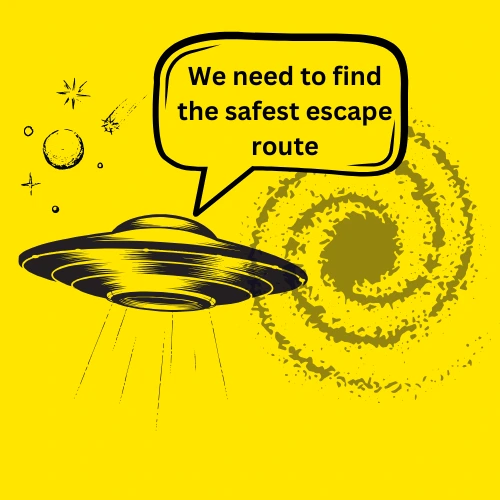
A spaceship traveling through deep space has been caught in a gravitational anomaly near a black hole. The ship’s computer has recorded the last known coordinates of its path before losing signal. However, due to the intense gravity, the coordinates have been scrambled, and they must be reorganized in ascending order for the ship’s navigation system to predict the safest escape route. The onboard computer can only access memory through pointers, meaning the crew must sort the coordinates using pointer-based operations. Write a C program that takes a set of recorded spaceship coordinates, sorts them in ascending order using pointers, and prints the corrected path.
Test Case
Input
Enter the number of recorded coordinates: 5
Enter the coordinates:
300
150
400
100
500
Expected Output
Corrected spaceship path:
100 150 300 400 500
- The program starts by asking the user to enter the number of recorded coordinates. This input is stored in the variable
n
. coordinates
array of sizen
is created to store the coordinates, and a pointer variableptr
is assigned to its starting position.- A
for
loop is used to take input for the coordinates, where values are stored in memory usingptr + i
. - Once all coordinates are entered, the program uses pointer-based sorting to arrange them in ascending order.
- A nested
for
loop is used for sorting.- A pointer
ptr1
is assigned the first element and another pointerptr2
moves through the list comparing values. - If the value at
ptr1
is greater than the value atptr2
, they are swapped using a temporary variable. - This process continues until all coordinates are sorted in memory.
- A pointer
- After sorting, another loop prints the corrected path of the spaceship.
#include <stdio.h> int main() { int n, i, j, temp; printf("Enter the number of recorded coordinates: "); scanf("%d", &n); int coordinates[n]; int *ptr = coordinates; printf("Enter the coordinates:\n"); for (i = 0; i < n; i++) { scanf("%d", ptr + i); } for (i = 0; i < n - 1; i++) { for (j = i + 1; j < n; j++) { if (*(ptr + i) > *(ptr + j)) { temp = *(ptr + i); *(ptr + i) = *(ptr + j); *(ptr + j) = temp; } } } printf("Corrected spaceship path:\n"); for (i = 0; i < n; i++) { printf("%d ", *(ptr + i)); } return 0; }
The Cybersecurity Firewall
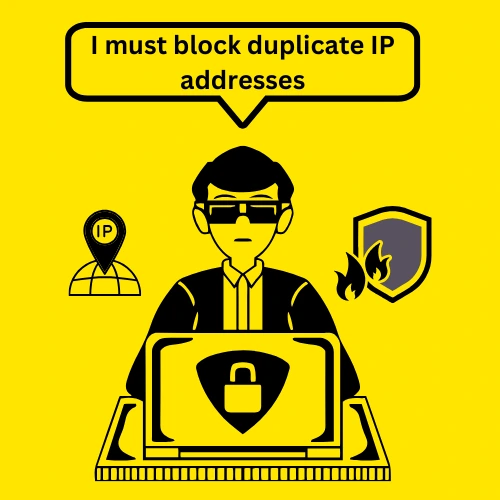
A cybersecurity expert is monitoring a firewall that protects a high-security server. The firewall logs multiple incoming IP addresses and needs to identify and block duplicate IPs to prevent cyberattacks. The expert must analyze the log file and remove duplicate IP addresses from memory to keep only unique connections. Due to security restrictions, the firewall software can only access and manipulate IP logs using pointers. Write a C program that takes a list of logged IP addresses, removes duplicates using pointer-based operations, and prints the filtered list of unique IPs.
Test Case
Input
Enter the number of logged IP addresses: 6
Enter the IP addresses (as integers for simplicity):
192 168 10 192 172 168
Expected Output
Filtered unique IP addresses:
192 168 10 172
- The program starts by asking the user to enter the number of logged IP addresses. This input is stored in the variable
n
. - An integer array is used to store the IP addresses, and a pointer variable
ptr
is assigned to its starting position. - A
for
loop is used to take input for the IP addresses, storing them in memory usingptr + i
. - To remove duplicate IPs, a nested loop is used:
- A pointer
ptr1
is assigned the first element, while another pointerptr2
moves through the remaining IPs to check for duplicates. - If a duplicate is found, a while loop is used to shift values left, effectively removing the duplicate entry in memory.
- The process continues until all duplicates are removed.
- A pointer
- After filtering out duplicate IPs, the program prints the list of unique IP addresses.
#include <stdio.h> int main() { int n, i, j, k; printf("Enter the number of logged IP addresses: "); scanf("%d", &n); int ipLogs[n]; int *ptr = ipLogs; printf("Enter the IP addresses (as integers for simplicity):\n"); for (i = 0; i < n; i++) { scanf("%d", ptr + i); } for (i = 0; i < n; i++) { j = i + 1; while (j < n) { if (*(ptr + i) == *(ptr + j)) { k = j; while (k < n - 1) { *(ptr + k) = *(ptr + k + 1); k++; } n--; } else { j++; } } } printf("Filtered unique IP addresses:\n"); for (i = 0; i < n; i++) { printf("%d ", *(ptr + i)); } return 0; }
The AI Network
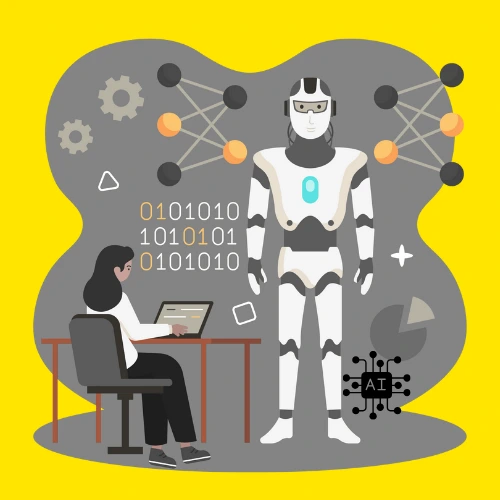
In a futuristic artificial intelligence lab, engineers are building a self-learning neural network. The AI grid consists of interconnected nodes, each of which stores a dynamic weight. However, due to a recent system failure, some of the neural pathways are broken, and the AI is unable to function correctly. The engineers must find and remove the broken pathways (weights marked as negative values) from memory while preserving the integrity of the remaining network. However, direct array access is not allowed in this system. The program must scan and shift weights using pointer-based operations to maintain a continuous neural connection. Write a C program that takes a list of neural weights, removes all broken pathways (negative values) using pointers, and prints the repaired neural grid.
Test Case
Input
Enter the number of neural weights: 7
Enter the weights of the neural connections:
5 -3 12 8 -7 4 10
Expected Output
Repaired neural grid:
5 12 8 4 10
- The program starts by asking the user to enter the number of neural weights that need to be processed. This input is stored in the variable
n
. - An array is created to store the weights, and a pointer variable
ptr
is assigned to its starting position. - A
for
loop is used to take input for the neural weights, where values are stored dynamically in memory usingptr + i
. - To remove broken pathways (negative values), a
while
loop is used to scan through the weights. - A pointer
ptr1
is assigned the first weight, while another pointerptr2
moves through the list to locate valid (non-negative) values. - If a negative value is found, a shifting process moves all remaining weights one position left in memory to overwrite the negative value.
- The process continues until all broken pathways are removed, and the AI neural network is fully connected.
- After processing, another loop prints the repaired neural grid.
#include <stdio.h> int main() { int n, i, j; printf("Enter the number of neural weights: "); scanf("%d", &n); int weights[n]; int *ptr = weights; printf("Enter the weights of the neural connections:\n"); for (i = 0; i < n; i++) { scanf("%d", ptr + i); } i = 0; while (i < n) { if (*(ptr + i) < 0) { j = i; while (j < n - 1) { *(ptr + j) = *(ptr + j + 1); j++; } n--; } else { i++; } } printf("Repaired neural grid:\n"); for (i = 0; i < n; i++) { printf("%d ", *(ptr + i)); } return 0; }
The Shifting Sand Puzzle
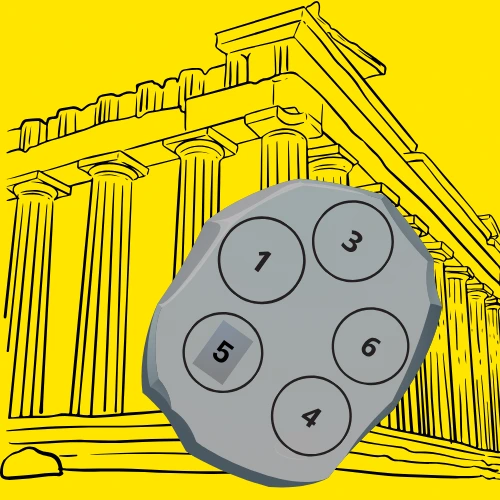
An ancient temple has an inscription made of numbers carved into a stone slab. Due to centuries of erosion, the slab has shifted, displacing the numbers in a cyclic pattern. The temple’s guardian reveals that the numbers must be realigned by rotating them to the right by a specific number of positions. However, the alignment process must be done manually using a special tool that allows only pointer-based manipulations. The only way to restore the inscription is to read the sequence of numbers, shift them rightward by the given number of positions, and place them back in the correct order without disturbing their integrity. Write a C program that takes an array of numbers and the number of right shifts as input and outputs the correctly realigned sequence.
Test Case
Input
Enter the number of elements in the array: 6
Enter the elements of the array:
1 2 3 4 5 6
Enter the number of right shifts: 2
Expected Output
Shifted Array:
5 6 1 2 3 4
- The program starts by asking the user to enter the number of elements in the array. This input is stored in the variable
n
. - Next, the program reads the elements of the array one by one and stores them in the array
arr
using pointer. - The pointer
ptr
is used to access memory locations instead of using array indexing. - The program then asks for the number of positions the array should be shifted to the right. The value entered is stored in
shifts
, and it is adjusted using the modulus operator to handle cases where the number of shifts is greater than the array size. - A second pointer
ptrShifted
is used to store the shifted values in a temporary arrayshifted
. - The new position of each element is calculated using modular arithmetic
(i + shifts) % n
. - After shifting, the values from
shifted
are copied back into the original array using pointer dereferencing. - Finally, the program prints the shifted array by accessing elements using pointer arithmetic.
#include <stdio.h> int main() { int arr[100], shifted[100], n, shifts, *ptr, *ptrShifted, i; printf("Enter the number of elements in the array: "); scanf("%d", &n); printf("Enter the elements of the array:\n"); ptr = arr; for (i = 0; i < n; i++) { scanf("%d", (ptr + i)); } printf("Enter the number of right shifts: "); scanf("%d", &shifts); shifts = shifts % n; ptrShifted = shifted; for (i = 0; i < n; i++) { *(ptrShifted + ((i + shifts) % n)) = *(ptr + i); } for (i = 0; i < n; i++) { *(ptr + i) = *(ptrShifted + i); } printf("Shifted Array:\n"); for (i = 0; i < n; i++) { printf("%d ", *(ptr + i)); } return 0; }
Feeding Frenzy at the Zoo
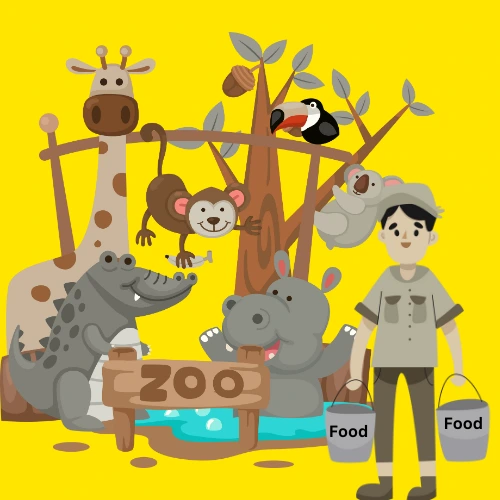
It’s feeding time at the zoo, and the zookeepers need to track how much food each animal consumes. Each animal has a specific appetite, and the zookeepers have prepared a total food supply to be distributed among the animals. The goal is to track how much food is left after each animal has eaten. The zookeeper has a list of animals, and each animal requires a certain amount of food. The animals eat one by one, reducing the total food supply. If there is not enough food left for an animal, it gets only the remaining food before the supply runs out. The zookeeper needs to monitor how much food remains after each animal is fed and display the results. The zoo’s feeding system only allows pointer-based operations, so the program must process the food consumption using pointers instead of array indexing. Write a C program that takes the number of animals, their respective food requirements, and the total available food as input, and outputs how much food each animal receives along with how much food is left after each feeding.
Test Case
Input
Enter the number of animals in the zoo: 4
Enter the total amount of food available: 50
Enter the food requirements for each animal:
10 20 15 30
Expected Output
Food distribution:
Animal 1 received 10 units of food. Remaining food: 40
Animal 2 received 20 units of food. Remaining food: 20
Animal 3 received 15 units of food. Remaining food: 5
Animal 4 received 5 units of food (only remaining amount). Food supply depleted.
- The program starts by asking the user to enter the number of animals. This value is stored in
numAnimals
. - Next, the program asks the user to enter the total amount of food available, which is stored in
totalFoodSupply
. - The program then prompts the user to enter the amount of food required by each animal. These values are stored in an array
foodRequirements
, and a pointerfoodPtr
is assigned to its first element. Instead of using array indexing, pointer arithmetic is used to store and access each value. - A loop iterates through the array, feeding each animal one by one.
- If there is enough food left in
totalFoodSupply
, the animal receives its required portion. Otherwise, the animal gets only the remaining food, and the supply becomes zero. - After each feeding, the remaining food supply is printed. If the food runs out, the remaining animals do not receive any food.
- Finally, the program prints the total food left after all animals have been served.
#include <stdio.h> int main() { int foodRequirements[100], numAnimals, totalFoodSupply; int *foodPtr; printf("Enter the number of animals in the zoo: "); scanf("%d", &numAnimals); printf("Enter the total amount of food available: "); scanf("%d", &totalFoodSupply); printf("Enter the food requirements for each animal:\n"); foodPtr = foodRequirements; for (int i = 0; i < numAnimals; i++) { scanf("%d", (foodPtr + i)); } printf("Food distribution:\n"); for (int i = 0; i < numAnimals; i++) { if (totalFoodSupply >= *(foodPtr + i)) { totalFoodSupply -= *(foodPtr + i); printf("Animal %d received %d units of food. Remaining food: %d\n", i + 1, *(foodPtr + i), totalFoodSupply); } else { printf("Animal %d received %d units of food (only remaining amount). Food supply depleted.\n", i + 1, totalFoodSupply); totalFoodSupply = 0; } } return 0; }
Secret Message Retrieval
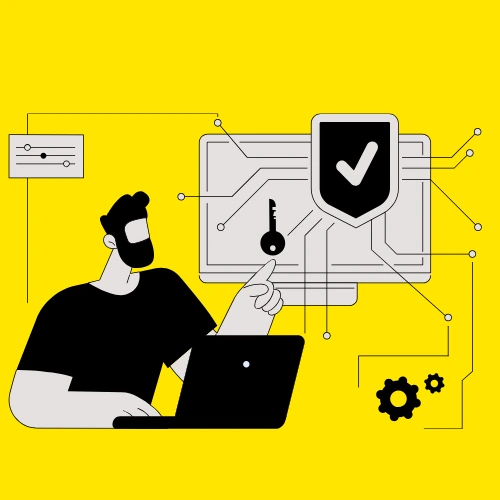
A top-secret agency has intercepted an encrypted message stored in a square grid of numbers. However, due to an unknown system error, some numbers within the grid have been misplaced. The agency’s cryptographers have identified two positions where the values were swapped and need your help in restoring the original message. Since this message is classified, it must be decrypted using low-level memory operations, meaning only pointer-based manipulations can be used to swap the numbers back into their correct positions. Write a C program that takes a matrix of numbers as input, swaps the values at two specified positions using pointers, and prints the corrected matrix.
Test Case
Input
Enter number of rows and columns: 3 3
Enter the matrix values:
1 2 3
4 5 6
7 8 9
Enter the two positions to be swapped (row1 col1 row2 col2): 1 1 3 3
Expected Output
Corrected Matrix:
9 2 3
4 5 6
7 8 1
- The program starts by asking the user to enter the number of rows and columns in the matrix. These values are stored in the variables
numRows
andnumCols
. - Next, the program prompts the user to enter the elements of the matrix. The pointer
matrixPtr
is assigned to the first element of the matrix (&matrix[0][0]
). Instead of using traditional array indexing, pointer arithmetic is used to store each element at the correct position in memory. The program calculates each memory location using:matrixPtr+i×numCols+j
, wherei
represents the row index andj
represents the column index. - Once the matrix is stored, the program asks the user to enter the row and column indices of two positions that need to be swapped. These positions are stored in:
firstRow
andfirstCol
: Represents the first element to be swapped.secondRow
andsecondCol
: Represents the second element to be swapped.
- Since user input is 1-based indexing (starting from 1), the program converts these values to 0-based indexing by subtracting 1 from each.
- Using pointer dereferencing, the program swaps the values.
- The value at
(firstRow, firstCol)
is stored intemp
. - The value at
(secondRow, secondCol)
is assigned to(firstRow, firstCol)
. - The stored value in
temp
is assigned to(secondRow, secondCol)
. - Finally, the program prints the corrected matrix by iterating through it using pointer arithmetic. Each value is accessed and printed without using array indexing.
#include <stdio.h> int main() { int matrix[10][10], numRows, numCols; int firstRow, firstCol, secondRow, secondCol, temp; int *matrixPtr; printf("Enter number of rows and columns: "); scanf("%d %d", &numRows, &numCols); printf("Enter the matrix values:\n"); matrixPtr = &matrix[0][0]; for (int i = 0; i < numRows; i++) { for (int j = 0; j < numCols; j++) { scanf("%d", (matrixPtr + i * numCols + j)); } } printf("Enter the two positions to be swapped (row1 col1 row2 col2): "); scanf("%d %d %d %d", &firstRow, &firstCol, &secondRow, &secondCol); firstRow--; firstCol--; secondRow--; secondCol--; temp = *(matrixPtr + firstRow * numCols + firstCol); *(matrixPtr + firstRow * numCols + firstCol) = *(matrixPtr + secondRow * numCols + secondCol); *(matrixPtr + secondRow * numCols + secondCol) = temp; printf("Corrected Matrix:\n"); for (int i = 0; i < numRows; i++) { for (int j = 0; j < numCols; j++) { printf("%d ", *(matrixPtr + i * numCols + j)); } printf("\n"); } return 0; }
Cricket Scoreboard
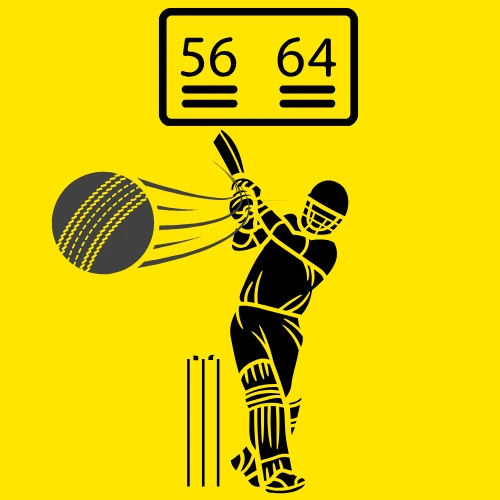
During a thrilling cricket match, the scoreboard operator is responsible for tracking the performance of batsmen and bowlers. The match involves multiple batsmen who each have a score (runs) and a status (whether they are still batting or have been dismissed). Additionally, bowlers record wickets taken and runs conceded. To ensure accurate scorekeeping, the scoreboard operator needs to dynamically update the scoreboard whenever a run is scored, a batsman is dismissed, or a bowler takes a wicket. However, to efficiently handle this information, the scoreboard must be managed using only pointers. Write a C program that keeps track of the runs scored by each batsman and the wickets taken by bowlers, updating the scoreboard dynamically using only pointers.
Test Case
Input
Enter the number of batsmen: 3
Enter the number of bowlers: 2
=== Cricket Scoreboard ===
1. Update batsman score
2. Mark batsman as out
3. Update bowler wickets
4. Update bowler runs conceded
5. Display scoreboard
6. Exit
Enter your choice: 1
Enter batsman number (1-3): 1
Enter runs scored: 20
Enter your choice: 1
Enter batsman number (1-3): 2
Enter runs scored: 35
Enter your choice: 2
Enter batsman number (1-3): 1
Enter your choice: 3
Enter bowler number (1-2): 1
Enter your choice: 4
Enter bowler number (1-2): 2
Enter runs conceded: 15
Enter your choice: 5
Enter your choice: 6
Exiting scoreboard…
Expected Output
=== Scoreboard ===
Batsmen:
Batsman 1: 20 runs (Out)
Batsman 2: 35 runs (Batting)
Batsman 3: 0 runs (Batting)
Bowlers:
Bowler 1: 1 wickets, 0 runs conceded
Bowler 2: 0 wickets, 15 runs conceded
- The program starts by asking the user to enter the number of batsmen and bowlers. These values are stored in the variables
numBatsmen
andnumBowlers
, respectively. - Next, the program initializes two arrays to store the runs scored and status of each batsman. The
batsmenRuns
array holds the runs scored by each batsman, whilebatsmenStatus
keeps track of whether a batsman is still batting (1
) or out (0
). - Similarly, two arrays are initialized for bowlers,
bowlerWickets
to store the number of wickets taken andbowlerRuns
to track the total runs conceded by each bowler. - The program then uses pointers (
batsmenRunsPtr
,batsmenStatusPtr
,bowlerWicketsPtr
, andbowlerRunsPtr
) to point to the respective arrays, ensuring that all operations are performed using pointer arithmetic instead of direct array indexing. - A
for
loop is used to initialize the batsmen’s statistics. Each batsman’s runs are set to0
, and their status is marked as1
(indicating they are still batting). - Another
for
loop initializes the bowlers’ statistics, setting their wickets and runs conceded to0
. - After initialization, the program enters a menu-driven loop, allowing the user to update the scoreboard dynamically.
- The user is presented with multiple options: updating a batsman’s score, marking a batsman as out, updating a bowler’s wickets, updating a bowler’s runs conceded, displaying the scoreboard, or exiting the program.
- If the user chooses to update a batsman’s score, the program prompts them to enter the batsman number and the number of runs scored. Using pointer arithmetic, the program accesses the appropriate batsman’s runs and increments the value.
- Before updating, the program checks whether the batsman is already out (
batsmenStatusPtr + (batsmanNumber - 1) == 0
). If the batsman is out, a message is displayed indicating that the update is not possible. - If the user chooses to mark a batsman as out, the program asks for the batsman number, then updates the batsman’s status to
0
using the pointer (batsmenStatusPtr + (batsmanNumber - 1)
). - When the user selects the option to update a bowler’s wickets taken, the program asks for the bowler number, then increments the corresponding wickets count using pointer arithmetic.
- Similarly, when updating a bowler’s runs conceded, the program asks for the bowler number and the number of runs conceded, then updates the value using pointers.
- If the user chooses to display the scoreboard, the program first prints the list of batsmen along with their runs scored and status (
Batting
if their status is1
,Out
if their status is0
). The program then prints the list of bowlers along with their wickets taken and runs conceded. - This process continues in a loop until the user selects the Exit option (
choice == 6
), at which point the program terminates.
#include <stdio.h> int main() { int const MAX_PLAYERS = 11; int numBatsmen, numBowlers; int batsmenRuns[MAX_PLAYERS], batsmenStatus[MAX_PLAYERS]; // 1 = Batting, 0 = Out int bowlerWickets[MAX_PLAYERS], bowlerRuns[MAX_PLAYERS]; int *batsmenRunsPtr = batsmenRuns; int *batsmenStatusPtr = batsmenStatus; int *bowlerWicketsPtr = bowlerWickets; int *bowlerRunsPtr = bowlerRuns; printf("Enter the number of batsmen: "); scanf("%d", &numBatsmen); printf("Enter the number of bowlers: "); scanf("%d", &numBowlers); for (int i = 0; i < numBatsmen; i++) { *(batsmenRunsPtr + i) = 0; *(batsmenStatusPtr + i) = 1; } for (int i = 0; i < numBowlers; i++) { *(bowlerWicketsPtr + i) = 0; *(bowlerRunsPtr + i) = 0; } int choice; do { printf("\n=== Cricket Scoreboard ===\n"); printf("1. Update batsman score\n"); printf("2. Mark batsman as out\n"); printf("3. Update bowler wickets\n"); printf("4. Update bowler runs conceded\n"); printf("5. Display scoreboard\n"); printf("6. Exit\n"); printf("Enter your choice: "); scanf("%d", &choice); if (choice == 1) { int batsmanNumber, runs; printf("Enter batsman number (1-%d): ", numBatsmen); scanf("%d", &batsmanNumber); printf("Enter runs scored: "); scanf("%d", &runs); if (*(batsmenStatusPtr + (batsmanNumber - 1)) == 1) { *(batsmenRunsPtr + (batsmanNumber - 1)) += runs; } else { printf("Batsman %d is already out!\n", batsmanNumber); } } else if (choice == 2) { int batsmanNumber; printf("Enter batsman number (1-%d): ", numBatsmen); scanf("%d", &batsmanNumber); *(batsmenStatusPtr + (batsmanNumber - 1)) = 0; } else if (choice == 3) { int bowlerNumber; printf("Enter bowler number (1-%d): ", numBowlers); scanf("%d", &bowlerNumber); *(bowlerWicketsPtr + (bowlerNumber - 1)) += 1; } else if (choice == 4) { int bowlerNumber, runs; printf("Enter bowler number (1-%d): ", numBowlers); scanf("%d", &bowlerNumber); printf("Enter runs conceded: "); scanf("%d", &runs); *(bowlerRunsPtr + (bowlerNumber - 1)) += runs; } else if (choice == 5) { printf("\n=== Scoreboard ===\n"); printf("Batsmen:\n"); for (int i = 0; i < numBatsmen; i++) { printf("Batsman %d: %d runs (%s)\n", i + 1, *(batsmenRunsPtr + i), *(batsmenStatusPtr + i) ? "Batting" : "Out"); } printf("\nBowlers:\n"); for (int i = 0; i < numBowlers; i++) { printf("Bowler %d: %d wickets, %d runs conceded\n", i + 1, *(bowlerWicketsPtr + i), *(bowlerRunsPtr + i)); } } else if (choice == 6) { printf("Exiting scoreboard...\n"); } else { printf("Invalid choice! Try again.\n"); } } while (choice != 6); return 0; }