Learn how to use loops in C programming with these fun and practical coding challenges. This article includes scenario-based questions with test cases, their code solutions, and step-by-step explanations. From managing energy cores to tracking budgets and unlocking treasure chests, it provides clear examples to help you understand and practice loops in real-world scenarios.
Toys Production Factory
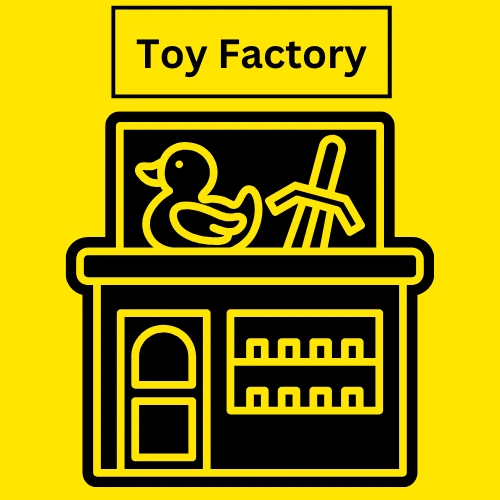
Imagine you own a toy factory where the production rate of toys is not constant. The production rate increases by 2 toys every hour. Write a C program that takes the number of working hours in a day and the initial production rate (toys per hour) as inputs and calculates the total number of toys produced by the end of the day.
Test Case
Input
Enter the number of operating hours: 4
Enter the initial number of toys produced in the first hour: 10
Expected Output
Total toys produced: 52
- The program begins by asking the user to enter the number of operating hours, which is stored in the
hours
variable. It also asks for the initial number of toys produced in the first hour, stored ininitial_toys
. - Next, the program initializes
total_toys
to 0 to hold the sum of all toys produced. - A
for
loop runs from 1 tohours
. During each iteration, the program adds the current production rate(initial_toys
) tototal_toys
. After adding, the program increases theinitial_toys
value by 2 for the next hour. - After the loop finishes, the program prints the total number of toys produced using
printf
.
#include <stdio.h> int main() { int hours; int initial_toys; int total_toys = 0; printf("Enter the number of operating hours: "); scanf("%d", &hours); printf("Enter the initial number of toys produced in the first hour: "); scanf("%d", &initial_toys); for (int i = 1; i <= hours; i++) { total_toys += initial_toys; initial_toys += 2; } printf("Total toys produced: %d\n", total_toys); return 0; }
Book Reading Tracker
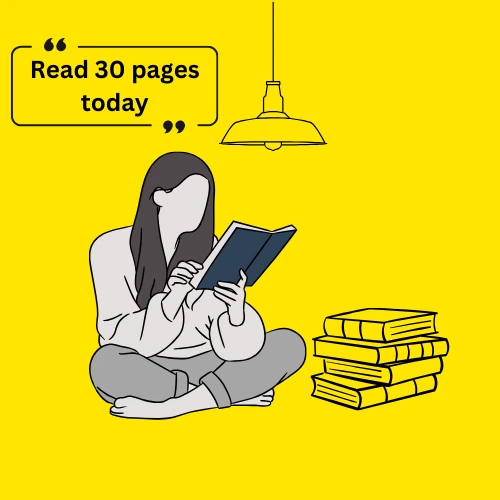
You are taking part in a book reading challenge where the number of pages you read changes from day to day. Write a C program that asks for the number of days you read and the number of pages you read each day, then calculates the total pages you’ve read.
Test Case
Input
Enter the number of reading days: 3
Enter the pages read on day 1: 30
Enter the pages read on day 2: 50
Enter the pages read on day 3: 40
Expected Output
Total number of pages read: 120
- The program asks the user to enter the number of days. This input is stored in
days
variable. total_pages
variable is initialized with value 0. This variable will be used to store the total number of pages read.- A
for
loop runs from 1 todays
, prompting the user to enter the pages read each day which is then stored in the variablepages_read_today
. - The program adds
pages_read_today
tototal_pages
during each iteration. - After the loop ends, the program prints the total number of pages read.
#include <stdio.h> int main() { int days; int pages_read_today; int total_pages = 0; printf("Enter the number of reading days: "); scanf("%d", &days); for (int i = 1; i <= days; i++) { printf("Enter the pages read on day %d: ", i); scanf("%d", &pages_read_today); total_pages += pages_read_today; } printf("Total number of pages read: %d\n", total_pages); return 0; }
Magical Candy Jar
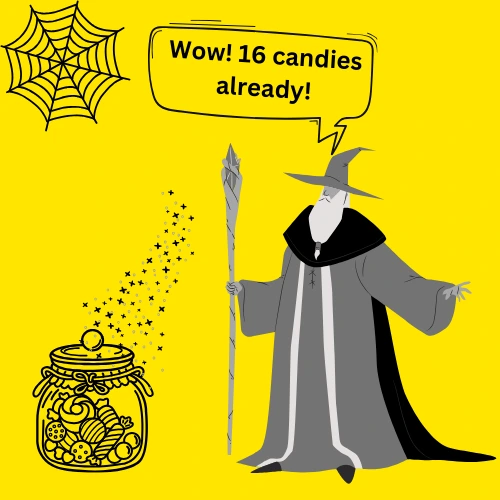
In a wizard’s lair, there’s a magical jar that refills itself with candy in a special way. It starts with 1 candy and doubles the number of candies it adds every minute. This continues until the number of candies in the jar reaches or exceeds the target amount of candies. Write a C program for the magical jar that takes the target candies as input and keeps adding candies until it reaches the target amount.
Test Case
Input
Enter the target number of candies: 30
Expected Output
1 more candies added to the jar
2 more candies added to the jar
4 more candies added to the jar
8 more candies added to the jar
16 more candies added to the jar
The candy jar is full!
- The program begins by asking the user to enter the target number of candies and stores it in
target_candies
variable. - Next, the program initializes
current_candies
to 0 andcandies_added
to an initial value of 1 candy. - A
while
loop is used to keep adding candies untilcurrent_candies
reaches or exceedstarget_candies
. - During each iteration, the program adds
candies_added
tocurrent_candies
and prints the number of candies added to the jar. Then, the program doubles the value ofcandies_added
. - When the
while
loop condition is no longer true, the program prints the message “The candy jar is full!”.
#include <stdio.h> int main() { int target_candies; int current_candies = 0; int candies_added = 1; printf("Enter the target number of candies: "); scanf("%d", &target_candies); while (current_candies < target_candies) { printf("%d more candies added to the jar\n", candies_added); current_candies += candies_added; candies_added *= 2; } printf("The candy jar is full!\n"); return 0; }
Goblin Treasure Chest Unlocking
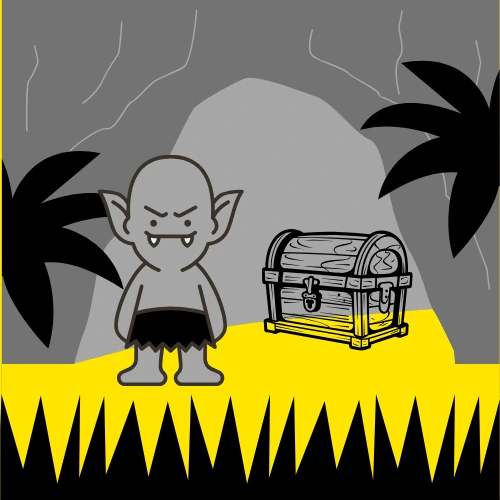
In a goblin’s cave, there’s a magical treasure chest that opens only when a specific sequence of numbers is entered correctly. The numbers in the sequence increase by 3 each time. Write a program in C language that takes the first number and the total number of entries needed, then prints out each number in the sequence.
Test Case
Input
Enter the first number of the sequence: 2
Enter the total number of entries: 5
Expected Output
2 5 8 11 14
The treasure chest opens!
- The program begins by asking the user to enter the first number in the sequence and the total number of entries. These inputs are stored in
first_number
andentries
variables respectively. - Next, the program initializes a loop that iterates
entries
times. - During each iteration, the program prints the current number and increases it by 3.
- After the loop finishes, the program prints a message indicating the sequence is complete.
#include <stdio.h> int main() { int first_number; int entries; printf("Enter the first number of the sequence: "); scanf("%d", &first_number); printf("Enter the total number of entries: "); scanf("%d", &entries); for (int i = 0; i < entries; i++) { printf("%d ", first_number); first_number += 3; } printf("\nThe treasure chest opens!"); return 0; }
Energy Checkpoint Challenge
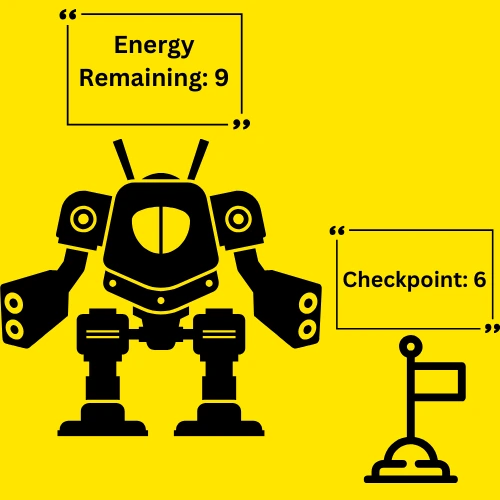
A robot is trying to pass through several checkpoints. At each checkpoint, it uses some energy, and the amount of energy needed increases based on the checkpoint number. Specifically, the energy required to pass a checkpoint is equal to the square of the checkpoint number. Write a C program that takes the initial energy of the robot as input and calculates how many checkpoints it can pass before it runs out of energy.
Test Case
Input
Enter the initial energy level: 100
Expected Output
Checkpoint 1 passed, remaining energy: 99
Checkpoint 2 passed, remaining energy: 95
Checkpoint 3 passed, remaining energy: 86
Checkpoint 4 passed, remaining energy: 70
Checkpoint 5 passed, remaining energy: 45
Checkpoint 6 passed, remaining energy: 9
The robot passed 6 checkpoints before running out of energy.
- The program begins by asking the user to enter the initial energy level and stores it in
energy_available
variable. - Next, the program initializes
checkpoint_number
to 1 and uses awhile
loop to check whether theenergy_available
is less than or equal to the square ofcheckpoint_number
. - During each iteration, the program calculates the energy consumption as
checkpoint_number * checkpoint_number
(square of the checkpoint number) and subtracts it fromenergy_available
. - The loop ends when
energy_available
is not enough to pass the next checkpoint, and the program prints how many checkpoints were passed.
#include <stdio.h> int main() { int energy_available; int checkpoints = 0; int checkpoint_number = 1; printf("Enter the initial energy level: "); scanf("%d", &energy_available); while (energy_available >= checkpoint_number * checkpoint_number) { energy_available -= checkpoint_number * checkpoint_number; checkpoints++; printf("Checkpoint %d passed, remaining energy: %d\n", checkpoint_number, energy_available); checkpoint_number++; } printf("The robot passed %d checkpoints before running out of energy.\n", checkpoints); return 0; }
Enchanted Path Tracker
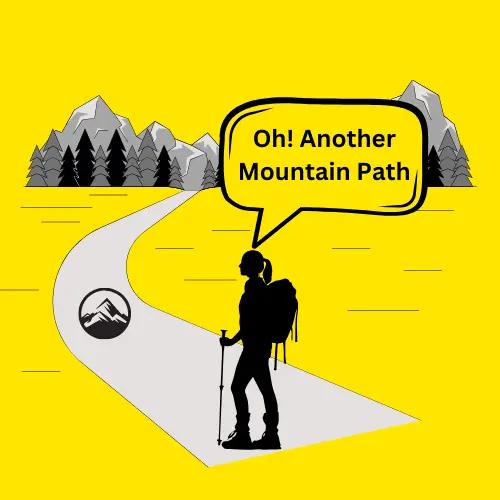
You are traveling through a magical forest, following an ancient trail marked with mysterious symbols. Each symbol represents a specific type of path, such as rivers, mountains, forests, or deserts. Your task is to write a C program that takes a series of numbers as input to represent these path types. The program will count how many times you encounter each type of path during your journey and determine which type of path you encountered the most and the least.
Test Case
Input
Choose a path type:
1- River
2- Mountain
3- Forest
4- Desert
5- Stop
Enter your choice: 1
Enter your choice: 3
Enter your choice: 2
Enter your choice: 4
Enter your choice: 1
Enter your choice: 2
Enter your choice: 5
Expected Output
Path Counts:
River: 2
Mountain: 2
Forest: 1
Desert: 1
Most encountered path: R (2 times)
Least encountered path: F (1 times)
Path analysis complete!
- The program starts by initializing four variables
river
,mountain
,forest
, anddesert
to zero. These variables are used to count the number of times each type of path is encountered. - The program then enters a
while
loop that runs continuously until the user chooses to stop by entering5
. - Inside the loop, the program prompts the user to select a path type by entering a number. The available choices are displayed on the screen, where
1
corresponds to River,2
to Mountain,3
to Forest,4
to Desert, and5
to stop the input process. - If the user enters
1
, the program increments theriver
counter. Similarly, it increments the respective counter for other valid inputs (mountain
,forest
, ordesert
). - If the user enters an invalid input (any number other than
1
to5
), the program displays a message stating that the input is ignored. - When the user enters
5
, the loop exits, and the program proceeds to calculate and display the results. - After the loop is exited, the program displays the counts for each path type, showing how many times the user selected each one.
- Next, the program determines the most encountered path. It initializes a variable
most_encountered
to'R'
(representing River) andmax_count
to the value ofriver
. It then checks ifmountain
,forest
, ordesert
has a higher count thanmax_count
. If a higher count is found,most_encountered
andmax_count
are updated accordingly. - Similarly, the program determines the least encountered path. It initializes a variable
least_encountered
to'R'
andmin_count
to the value ofriver
. It then checks ifmountain
,forest
, ordesert
has a smaller count (greater than 0) thanmin_count
or ifmin_count
is currently zero. If a smaller count is found,least_encountered
andmin_count
are updated. - Finally, the program prints the most and least encountered paths along with their respective counts.
#include <stdio.h> int main() { int input; int river = 0; int mountain = 0; int forest = 0; int desert = 0; while (1) { printf("\nChoose a path type:\n"); printf("1. River\n"); printf("2. Mountain\n"); printf("3. Forest\n"); printf("4. Desert\n"); printf("5. Stop\n"); printf("Enter your choice: "); scanf("%d", &input); if (input == 5) { break; } else if (input == 1) { river++; } else if (input == 2) { mountain++; } else if (input == 3) { forest++; } else if (input == 4) { desert++; } else { printf("Invalid input '%d' ignored.\n", input); } } printf("\nPath Counts:\n"); printf("River: %d\n", river); printf("Mountain: %d\n", mountain); printf("Forest: %d\n", forest); printf("Desert: %d\n", desert); char most_encountered = 'R'; int max_count = river; if (mountain > max_count) { most_encountered = 'M'; max_count = mountain; } if (forest > max_count) { most_encountered = 'F'; max_count = forest; } if (desert > max_count) { most_encountered = 'D'; max_count = desert; } char least_encountered = 'R'; int min_count = river; if ((mountain < min_count && mountain > 0) || min_count == 0) { least_encountered = 'M'; min_count = mountain; } if ((forest < min_count && forest > 0) || min_count == 0) { least_encountered = 'F'; min_count = forest; } if ((desert < min_count && desert > 0) || min_count == 0) { least_encountered = 'D'; min_count = desert; } printf("\nMost encountered path: %c (%d times)\n", most_encountered, max_count); printf("Least encountered path: %c (%d times)\n", least_encountered, min_count); printf("Path analysis complete!\n"); return 0; }
Magical Word Reverser
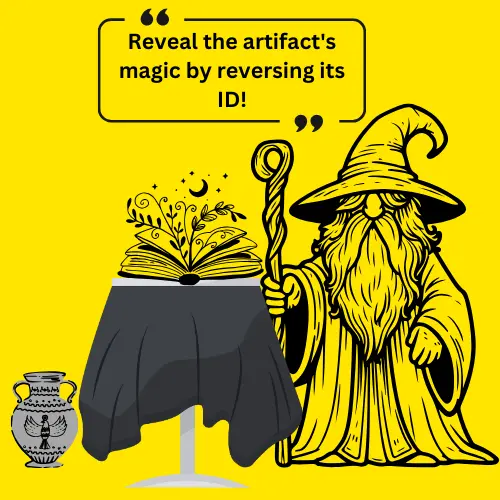
Imagine you are helping a wizard organize a collection of magical artifacts. Each artifact has a special numeric ID engraved on it. To unlock its magical powers, the wizard’s spellbook needs the ID to be read backward. Your task is to write a program in C that takes the artifact’s ID as input and prints it in reverse so the wizard can use it.
Test Case
Input
Enter artifact ID: 12345
Expected Output
Reversed ID: 54321
Reversal complete!
- The program starts by asking the user to enter the artifact’s numeric ID. This number is stored in a variable called
artifact_id
. - To reverse the number, the program uses a
while
loop. - Inside the loop, the last digit of the number is found using the
% 10
operation. This works because when you divide a number by 10, the remainder is always the last digit. For example, if the number is 123, dividing it by 10 gives a remainder of 3, which is the last digit. - Once the last digit is found, the program prints it. After that, the number is updated by removing its last digit. This is done using
/ 10
. For example, if the number is 123, dividing it by 10 gives 12. - The loop keeps repeating these steps until the
artifact_id
becomes 0. - Finally, the program prints a message saying the reversal is complete.
#include <stdio.h> int main() { int artifact_id; printf("Enter artifact ID: "); scanf("%d", &artifact_id); printf("Reversed ID: "); while (artifact_id > 0) { int digit = artifact_id % 10; printf("%d", digit); artifact_id = artifact_id / 10; } printf("\nReversal complete!\n"); return 0; }
Trapped Dungeon Key Finder
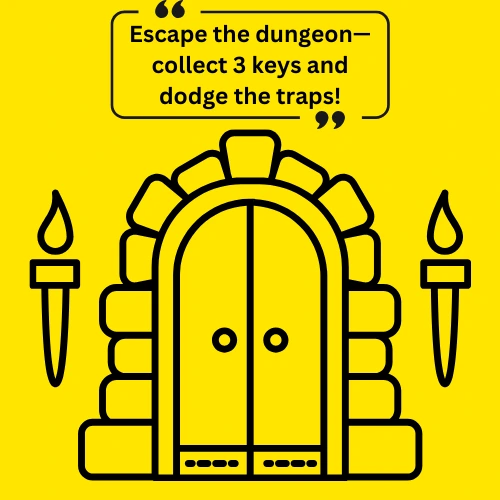
Imagine you are in a dungeon with many rooms, and you need to escape. Some rooms have keys to help you unlock doors, some are empty, and others have traps. If you step into a room with a trap, you lose all the keys you’ve collected so far. You will enter the status of each room one by one. 1
means a key, 0
means an empty room, and -1
means a trap. To escape, you must collect at least 3 keys. Write a program in C language that takes the room status as input in each iteration and calculates how many keys you can safely collect before escaping or failing to escape.
Test Case
Input
Enter the total number of rooms: 6
Enter the status of room 1 (1 for key, 0 for empty, -1 for trap): 1
Enter the status of room 2 (1 for key, 0 for empty, -1 for trap): 0
Enter the status of room 3 (1 for key, 0 for empty, -1 for trap): -1
Enter the status of room 4 (1 for key, 0 for empty, -1 for trap): 1
Enter the status of room 5 (1 for key, 0 for empty, -1 for trap): 1
Enter the status of room 6 (1 for key, 0 for empty, -1 for trap): 1
Expected Output
Room 1: Found a key! Total keys: 1
Room 2: Empty room.
Room 3: Trap triggered! Keys reset to 0.
Room 4: Found a key! Total keys: 1
Room 5: Found a key! Total keys: 2
Room 6: Found a key! Total keys: 3
3 keys collected! Escaped the dungeon!
- The program starts by asking the user to input the total number of rooms and stores it in
total_rooms
variable. - The variable
total_keys
is initialized to 0 to count the keys collected, and the constantescape_keys
is set to 3, representing the number of keys required to escape. - A
for
loop is used to iterate over each room. - In each iteration, the program prompts the user to input the status of the current room and stores it in
room_status
variable. - If the room contains a key
(room_status == 1)
, thetotal_keys
counter is incremented, and a message is displayed showing the total keys collected so far. Iftotal_keys
reaches 3, a success message is displayed, and the program exits usingreturn 0
. - If the room contains a trap
(room_status == -1)
, thetotal_keys
counter is reset to 0, and a message is displayed indicating that all keys have been lost. - If the room is empty
(room_status == 0)
, a message is displayed indicating that the room is empty. - If the input is invalid (anything other than
1
,0
, or-1
), an error message is displayed, and the loop continues. - After the loop completes, if the player has not collected 3 keys, the program displays a message saying that the player failed to escape the dungeon and shows the total number of keys collected.
#include <stdio.h> int main() { int total_rooms; int total_keys = 0; const int escape_keys = 3; int room_status; printf("Enter the total number of rooms: "); scanf("%d", &total_rooms); for (int i = 1; i <= total_rooms; i++) { printf("Enter the status of room %d (1 for key, 0 for empty, -1 for trap): ", i); scanf("%d", &room_status); if (room_status == 1) { total_keys++; printf("Room %d: Found a key! Total keys: %d\n", i, total_keys); if (total_keys == escape_keys) { printf("3 keys collected! Escaped the dungeon!\n"); return 0; } } else if (room_status == -1) { total_keys = 0; printf("Room %d: Trap triggered! Keys reset to 0.\n", i); } else if (room_status == 0) { printf("Room %d: Empty room.\n", i); } else { printf("Invalid input for room %d. Please enter 1, 0, or -1.\n", i); } } printf("Failed to escape the dungeon! Total keys collected: %d\n", total_keys); return 0; }
Secret Code Cracker
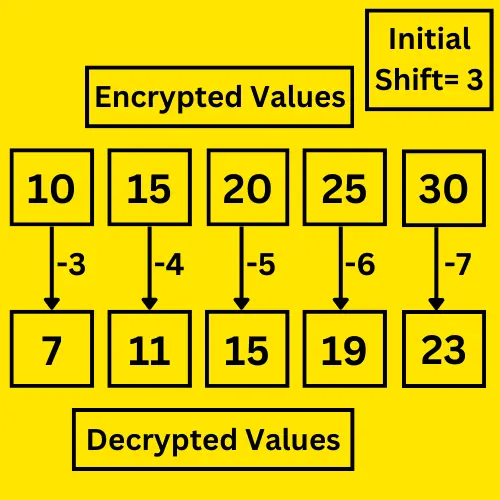
Imagine you need to crack a secret code by decrypting a series of numbers. Each number is locked with a shift value, and the shift increases by one after each step. Write a program in C language that takes the total number of encrypted numbers and a starting shift value as input. The program then asks the user to input each encrypted number one by one, decrypts it immediately by subtracting the shift value, prints the decrypted number, and increments the shift for the next decryption.
Test Case
Input
Enter the total number of encrypted numbers: 5
Enter the initial shift value: 3
Enter encrypted number 1: 10
Enter encrypted number 2: 15
Enter encrypted number 3: 20
Enter encrypted number 4: 25
Enter encrypted number 5: 30
Expected Output
Decrypted number: 7
Decrypted number: 11
Decrypted number: 15
Decrypted number: 19
Decrypted number: 23
Decryption complete!
- The program starts by asking the user to input the total number of encrypted numbers and the initial shift value. These inputs are stored in the
total_numbers
andshift
variables, respectively. - Next, the program enters a
for
loop that runs from 1 tototal_numbers
. This loop processes each encrypted number individually. - Inside the loop, the program prompts the user to input the current encrypted number. The value entered by the user is stored in the
encrypted
variable. - The program then calculates the decrypted value using the formula
decrypted = encrypted - shift
. The decrypted value is printed to the screen immediately after calculation. - After each decryption, the program increments the
shift
variable by 1 to adjust the decryption process for the next number. - When the loop completes, the program prints a final message indicating that the decryption process is complete.
#include <stdio.h> int main() { int total_numbers; int shift; int encrypted; int decrypted; printf("Enter the total number of encrypted numbers: "); scanf("%d", &total_numbers); printf("Enter the initial shift value: "); scanf("%d", &shift); for (int i = 1; i <= total_numbers; i++) { printf("\nEnter encrypted number %d: ", i); scanf("%d", &encrypted); decrypted = encrypted - shift; printf("Decrypted number: %d", decrypted); shift++; } printf("\nDecryption complete!\n"); return 0; }
Dog Shop Inventory Tracker
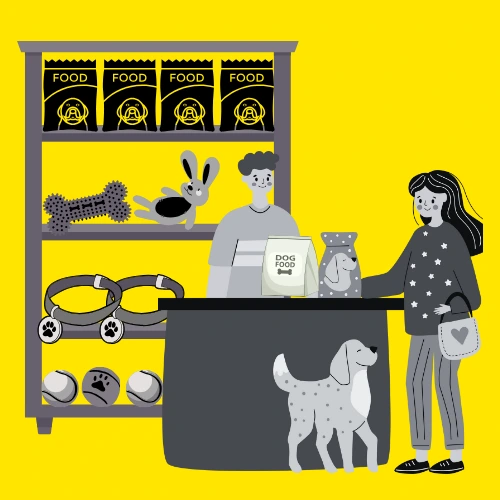
Imagine you own a dog shop that sells various dog accessories, food, and toys. Each day, you track the shop’s inventory to see how much of each item is sold. Every item belongs to a category: 1 for accessories, 2 for food, and 3 for toys. Write a C program that takes the sales data as input and calculates the total number of items sold in each category by the end of the day.
Test Case
Input
Enter the total number of sales transactions: 5
Enter category (1 for accessories, 2 for food, 3 for toys): 1
Enter quantity sold: 10
Enter category (1 for accessories, 2 for food, 3 for toys): 2
Enter quantity sold: 5
Enter category (1 for accessories, 2 for food, 3 for toys): 3
Enter quantity sold: 8
Enter category (1 for accessories, 2 for food, 3 for toys): 4
Enter quantity sold: 12
Enter category (1 for accessories, 2 for food, 3 for toys): 1
Enter quantity sold: 12
Expected Output
Invalid category. Skipping this transaction.
Summary of items sold:
Accessories: 22
Food: 5
Toys: 8
- The program begins by asking the user to enter the total number of sales transactions. This value is stored in the
total_transactions
variable. - Next, the program initializes three counters,
accessories_total
,food_total
, andtoys_total
to track the quantities sold for each category. - A
for
loop is used to take the input for each transaction. - The user is prompted to enter the
category
(1
,2
, or3
) and thequantity
sold, where 1 represents accessories, 2 represents food and 3 represents toys. - An
if
condition checks if thecategory
is1
. If true, the program adds thequantity
toaccessories_total
. - An
else if
condition checks if thecategory
is2
. If true, the program adds thequantity
tofood_total
. - Another
else if
condition checks if thecategory
is3
. If true, the program adds thequantity
totoys_total
. - If the
category
is invalid, the program prints an error message and skips the transaction. - After the loop completes, the program prints the total number of items sold for each category using
printf
.
#include <stdio.h> int main() { int total_transactions; int accessories_total = 0; int food_total = 0; int toys_total = 0; int category; int quantity; printf("Enter the total number of sales transactions: "); scanf("%d", &total_transactions); for (int i = 0; i < total_transactions; i++) { printf("Enter category (1 for accessories, 2 for food, 3 for toys): "); scanf("%d", &category); printf("Enter quantity sold: "); scanf("%d", &quantity); if (category == 1) { accessories_total += quantity; } else if (category == 2) { food_total += quantity; } else if (category == 3) { toys_total += quantity; } else { printf("Invalid category. Skipping this transaction.\n"); } } printf("Summary of items sold:\n"); printf("Accessories: %d\n", accessories_total); printf("Food: %d\n", food_total); printf("Toys: %d\n", toys_total); return 0; }
Trip Budget Planner
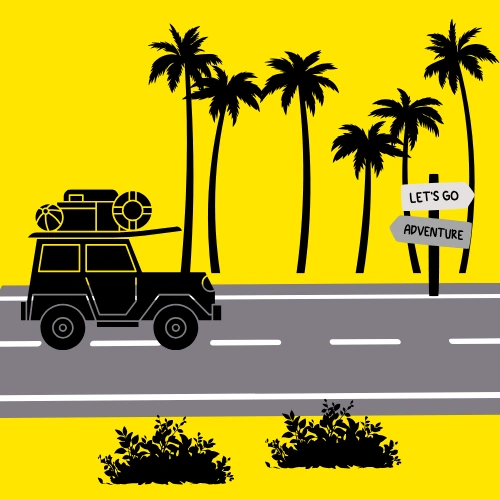
Imagine you are planning a road trip with several stops. At each stop, you record expenses for food, lodging, and fuel. Your trip has a limited budget, and if the expenses go over the budget at any point, the trip must end early. Write a program in C language that takes the initial trip budget and the expenses at each stop as input, calculates the total expenses for the trip, checks if you stayed within the budget, and shows the total cost of food, lodging, and fuel.
Test Case
Input
Enter the total number of stops: 3
Enter the trip budget: 1000
Stop 1:
Enter food expenses: 50
Enter lodging expenses: 100
Enter fuel expenses: 70
Stop 2:
Enter food expenses: 60
Enter lodging expenses: 120
Enter fuel expenses: 80
Stop 3:
Enter food expenses: 70
Enter lodging expenses: 150
Enter fuel expenses: 90
Expected Output
Total expenses for Stop 1: 220.00
Total expenses for Stop 2: 260.00
Total expenses for Stop 3: 310.00
Trip completed within budget!
Total expenses: 790.00
Total cost of food: 180.00
Total cost of lodging: 370.00
Total cost of fuel: 240.00
- The program starts by asking the user to enter the total number of stops for the trip and the trip’s budget. These inputs are stored in the variables
total_stops
andbudget
. - The program initializes
total_expenses
to 0. Additionally, it declares three variables,total_food
,total_lodging
, andtotal_fuel
to keep a record of the food, lodging, and fuel costs separately. Thecurrent_stop
variable is initialized to 1 to start processing the expenses from the first stop. - A
while
loop is used to process the expenses for each stop. The loop runs until all stops are completed or the budget is exceeded. - At the beginning of each loop iteration, the program prompts the user to input the food, lodging, and fuel expenses for the current stop. These values are stored in the variables
food
,lodging
, andfuel
. - The total expenses for the stop are calculated by summing these three values and are added to the running total of
total_expenses
. - The program also updates the totals for food, lodging, and fuel by adding the respective values to
total_food
,total_lodging
, andtotal_fuel
. - The program then checks if the total expenses have exceeded the budget using an
if
condition. - If the total expenses exceed the budget, a message is displayed to inform the user that the budget has been exceeded at the current stop. The program also shows the total expenses so far and ends the trip prematurely by breaking out of the loop.
- If the loop completes without the budget being exceeded, the trip is considered successful.
- After the loop finishes, the program checks if the trip was completed within the budget.
- If the total expenses are less than or equal to the budget, it prints a message stating that the trip was successfully completed within the budget, otherwise, it prints a message indicating that the trip ended prematurely.
- Finally, the program displays the total expenses for the trip. It also prints the total costs for food, lodging, and fuel separately.
#include <stdio.h> int main() { int total_stops; int current_stop = 1; float budget, total_expenses = 0, food, lodging, fuel; float total_food = 0, total_lodging = 0, total_fuel = 0; printf("Enter the total number of stops: "); scanf("%d", &total_stops); printf("Enter the trip budget: "); scanf("%f", &budget); while (current_stop <= total_stops) { printf("\nStop %d:\n", current_stop); printf("Enter food expenses: "); scanf("%f", &food); printf("Enter lodging expenses: "); scanf("%f", &lodging); printf("Enter fuel expenses: "); scanf("%f", &fuel); float stop_expenses = food + lodging + fuel; total_expenses += stop_expenses; total_food += food; total_lodging += lodging; total_fuel += fuel; printf("Total expenses for Stop %d: %.2f\n", current_stop, stop_expenses); if (total_expenses > budget) { printf("Budget exceeded at Stop %d! Trip ends prematurely.\n", current_stop); printf("Total expenses so far: %.2f (Budget: %.2f)\n", total_expenses, budget); break; } current_stop++; } if (total_expenses <= budget) { printf("\nTrip completed within budget!\n"); } else { printf("\nTrip ended prematurely.\n"); } printf("Total expenses: %.2f\n", total_expenses); printf("Total cost of food: %.2f\n", total_food); printf("Total cost of lodging: %.2f\n", total_lodging); printf("Total cost of fuel: %.2f\n", total_fuel); return 0; }
Energy Challenge Tracker
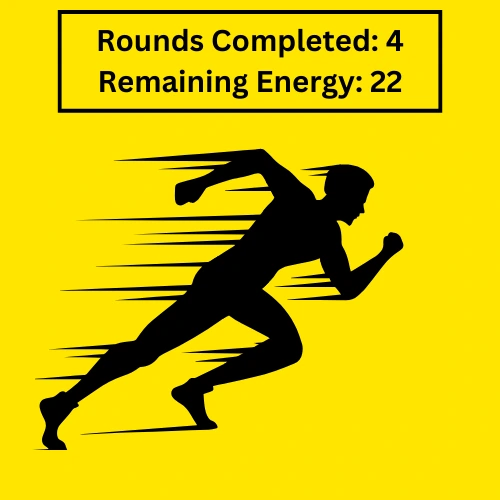
Imagine you’re taking part in a virtual endurance race where your goal is to manage your energy while completing a series of challenges. In each round, the type of challenge determines how your energy is affected. If the challenge is type 1, your energy increases by the round number. If it’s type 2, your energy decreases by the round number divided by 3, using only whole numbers. For type 3 challenges, your energy is multiplied by 1.5 and rounded down to the nearest whole number.
The race continues as long as your energy stays above zero. However, if your energy drops to zero or below at any point, the race ends immediately, and you lose. The program tracks your energy level at the end of each round and determines whether you successfully completed the race or not.
Write a C program that takes the number of rounds, the initial energy level, and the challenge types as input, then calculates and displays the energy level after each round and whether you completed the race.
Test Case
Input
Enter the initial energy level: 10
Enter the total number of rounds: 4
Round 1:
Enter challenge type (1, 2, or 3): 1
Round 2:
Enter challenge type (1, 2, or 3): 3
Round 3:
Enter challenge type (1, 2, or 3): 2
Round 4:
Enter challenge type (1, 2, or 3): 3
Expected Output
Challenge 1: Energy increased by 1. Current energy: 11
Challenge 3: Energy multiplied to 16. Current energy: 16
Challenge 2: Energy decreased by 1. Current energy: 15
Challenge 3: Energy multiplied to 22. Current energy: 22
You completed all 4 rounds! Final energy level: 22
- The program begins by asking the user to input the initial energy level and the total number of rounds for the race. These values are stored in
initial_energy
androunds
respectively. - The
current_energy
variable is initialized with the value ofinitial_energy
to keep track of the energy throughout the race. - The
round_number
variable is set to 1 to indicate the first round of the race. - The program then enters a
do-while
loop, which continues as long as the current round number is less than or equal to the total number of rounds provided by the user. - At the start of each round, the program prompts the user to input the challenge type for that round (1, 2, or 3). This input is stored in the variable
challenge_type
. - If the
challenge_type
is 1, the program increases thecurrent_energy
by the value of theround_number
. A message is displayed showing the energy increase and the current energy level. - If the
challenge_type
is 2, the program decreases thecurrent_energy
by the value of theround_number
divided by 3. A message is displayed showing the energy decrease and the current energy level. - If the
challenge_type
is 3, the program multiplies thecurrent_energy
by 1.5. A message is displayed showing the new energy level. - If the
challenge_type
is invalid (not 1, 2, or 3), the program displays an error message and skips to the next round using thecontinue
statement. - After processing the challenge for the round, the program checks if the
current_energy
has dropped to zero or below. If this condition is true, a message is displayed indicating that the user lost all their energy and the race ends prematurely. The loop is exited using thebreak
statement. - If the energy remains above zero, the program increments the
round_number
and proceeds to the next round. - Once the loop ends, the program checks whether the user completed the race.
- If the user ran out of energy, a message is displayed showing how many rounds they completed before losing all energy.
- If the energy is still above zero, a message is displayed congratulating the user for completing all rounds and showing the final energy level.
#include <stdio.h> int main() { int initial_energy; int rounds; int round_number = 1; int challenge_type; printf("Enter the initial energy level: "); scanf("%d", &initial_energy); printf("Enter the total number of rounds: "); scanf("%d", &rounds); int current_energy = initial_energy; do { printf("\nRound %d:\n", round_number); printf("Enter challenge type (1, 2, or 3): "); scanf("%d", &challenge_type); if (challenge_type == 1) { current_energy += round_number; printf("Challenge 1: Energy increased by %d. Current energy: %d\n", round_number, current_energy); } else if (challenge_type == 2) { current_energy -= round_number / 3; printf("Challenge 2: Energy decreased by %d. Current energy: %d\n", round_number / 3, current_energy); } else if (challenge_type == 3) { current_energy = current_energy * 1.5; printf("Challenge 3: Energy multiplied to %d. Current energy: %d\n", current_energy, current_energy); } else { printf("Invalid challenge type. Skipping this round.\n"); continue; } if (current_energy <= 0) { printf("You lost all your energy in round %d. Race ends!\n", round_number); break; } round_number++; } while (round_number <= rounds); if (current_energy > 0) { printf("\nYou completed all %d rounds! Final energy level: %d\n", rounds, current_energy); } else { printf("\nYou survived %d rounds before running out of energy.\n", round_number - 1); } return 0; }
Advanced Household Budget Manager
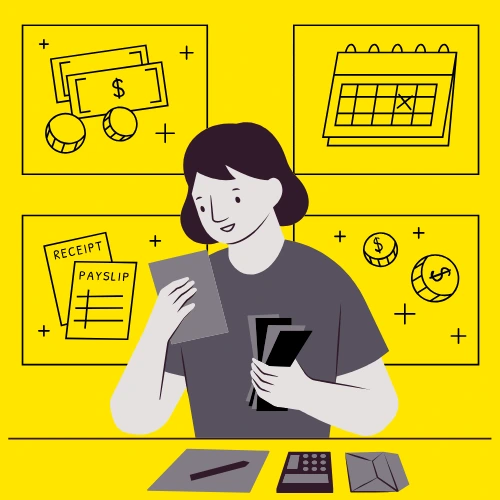
Imagine you are managing a household budget over a set number of days. You start with an initial balance of $1000, and each day, you have different actions to handle your money effectively. You can add income from work or other sources, pay bills like rent and utilities, buy groceries or daily necessities, save money into an emergency fund, or check your current balance. There are some additional rules to keep in mind. Every day, a $5 maintenance fee is automatically deducted to cover daily expenses. If your balance drops below $100 at any point, a $20 penalty is applied, and you get a warning. Your goal is to keep your balance positive, save money for emergencies, and manage your spending wisely.
The program ends either if your balance becomes negative, meaning you run out of money, or if you successfully manage your budget for the entire period. Write a program in C language that simulates this scenario, tracks daily changes in your money, and determines whether you managed your budget successfully.
Test Case
Input
Enter the total number of days to manage the budget: 4
— Day 1 —
Choose an action:
Add Income
Pay Bills
Buy Groceries
Save Money into Emergency Fund
Check Status
Enter your choice: 2
Enter bill amount: 950
— Day 2 —
Choose an action:
Add Income
Pay Bills
Buy Groceries
Save Money into Emergency Fund
Check Status
Enter your choice: 3
Enter grocery amount: 25
Expected Output
Bills paid. Current balance: 50.00
Daily maintenance fee deducted. Current balance: 45.00
Warning! Balance fell below $100. Penalty of $20 applied. Current balance: 25.00
Groceries purchased. Current balance: 0.00
Daily maintenance fee deducted. Current balance: -5.00
Budget Failed! Your balance went negative on day 2.
- The program starts by initializing the
balance
to $1000 and theemergency_fund
to $0. - The user is asked to input the total number of days for managing the budget. This input is stored in
total_days
variable. - The variable
current_day
is set to 1 to track the progress day by day. - The program uses a while loop that runs as long as
current_day
is less than or equal tototal_days
. Each iteration of the loop represents one day of financial management. - At the start of each day, the program displays a menu of actions for the user, which includes
Add income
,Pay bills
,Buy groceries
,Save money into the emergency fund
andCheck the current financial status
. - At the start of each day, the program displays a menu of actions for the user to choose from.
- If the user chooses option 1 (
choice == 1
), the program prompts the user to enter the amount of income. This amount is added to thebalance
, and the updated balance is displayed. - If the user selects option 2 (
choice == 2
), the program asks the user to input the bill amount. This value is subtracted from thebalance
, and the updated balance is shown. - If the user selects option 3 (
choice == 3
), the program prompts the user to enter the cost of groceries. This amount is deducted from thebalance
, and the updated balance is displayed. - If the user selects option 4 (
choice == 4
), the program asks the user how much money they want to save in the emergency fund. If the amount is greater than the currentbalance
, a message is shown saying there isn’t enough money to save. Otherwise, the amount is subtracted from thebalance
, added to theemergency_fund
, and both updated values are displayed. - If the user selects option 5 (
choice == 5
), the program displays the currentbalance
and theemergency_fund
. - If the user enters an invalid choice, the program shows an error message and skips further processing for that day.
- At the end of each day, a daily maintenance fee of $5 is deducted from the
balance
to simulate daily expenses. If thebalance
falls below $100 but remains above $0, the program applies a penalty of $20 and shows a warning message. If thebalance
drops below $0, the program displays a message stating the budget has failed, specifies the day on which this happened, and exits the loop. - If the loop completes successfully, the program congratulates the user for managing their budget wisely and displays the final
balance
and the amount saved in theemergency_fund
.
#include <stdio.h> int main() { float balance = 1000.0; float emergency_fund = 0.0; int total_days; int current_day = 1; int choice; printf("Enter the total number of days to manage the budget: "); scanf("%d", &total_days); while (current_day <= total_days) { printf("\n--- Day %d ---\n", current_day); printf("Choose an action:\n"); printf("1. Add Income\n"); printf("2. Pay Bills\n"); printf("3. Buy Groceries\n"); printf("4. Save Money into Emergency Fund\n"); printf("5. Check Status\n"); printf("Enter your choice: "); scanf("%d", &choice); if (choice == 1) { float income; printf("Enter income amount: "); scanf("%f", &income); balance += income; printf("Income added. Current balance: %.2f\n", balance); } else if (choice == 2) { float bills; printf("Enter bill amount: "); scanf("%f", &bills); balance -= bills; printf("Bills paid. Current balance: %.2f\n", balance); } else if (choice == 3) { float groceries; printf("Enter grocery amount: "); scanf("%f", &groceries); balance -= groceries; printf("Groceries purchased. Current balance: %.2f\n", balance); } else if (choice == 4) { float savings; printf("Enter amount to save in emergency fund: "); scanf("%f", &savings); if (savings > balance) { printf("Not enough balance to save this amount!\n"); } else { balance -= savings; emergency_fund += savings; printf("Saved %.2f into emergency fund. Current emergency fund: %.2f, Current balance: %.2f\n", savings, emergency_fund, balance); } } else if (choice == 5) { printf("Current Financial Status:\n"); printf("Balance: %.2f\n", balance); printf("Emergency Fund: %.2f\n", emergency_fund); } else { printf("Invalid choice. Please select a valid action.\n"); } balance -= 5.0; printf("Daily maintenance fee deducted. Current balance: %.2f\n", balance); if (balance < 100 && balance > 0) { balance -= 20.0; printf("Warning! Balance fell below $100. Penalty of $20 applied. Current balance: %.2f\n", balance); } if (balance < 0) { printf("\nBudget Failed! Your balance went negative on day %d.\n", current_day); break; } current_day++; } if (balance >= 0) { printf("\nBudget spent wisely throughout the days. Final balance: %.2f, Emergency Fund: %.2f\n", balance, emergency_fund); } return 0; }
Efficient Package Transportation
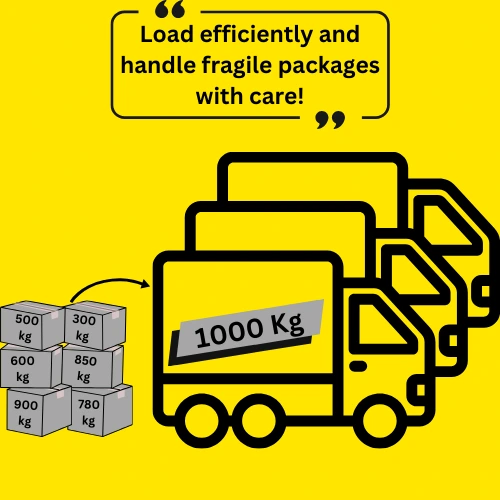
Imagine you are in charge of transporting goods using trucks, and you need to distribute packages as efficiently as possible. Each truck has a maximum weight limit of 1,000 kg. However, fragile packages require special handling and must always be transported alone, even if they weigh much less than the truck’s capacity.
The program will take input from the user for the weights of the packages and whether they are fragile or not. Regular packages can be loaded together in trucks to make the most of the available space, but the total weight in each truck must not exceed 1,000 kg. Fragile packages will be assigned one truck each, regardless of their weight.
Write a program in C that calculates the minimum number of trucks needed to transport all the packages while ensuring that the fragile packages are transported separately and the regular packages are loaded as efficiently as possible.
Test Case
Input
Enter the number of packages: 7
Enter weight of package 1 (max 1000 kg): 500
Is package 1 fragile (0 = No, 1 = Yes)? 0
Enter weight of package 2 (max 1000 kg): 700
Is package 2 fragile (0 = No, 1 = Yes)? 1
Enter weight of package 3 (max 1000 kg): 400
Is package 3 fragile (0 = No, 1 = Yes)? 0
Enter weight of package 4 (max 1000 kg): 600
Is package 4 fragile (0 = No, 1 = Yes)? 0
Enter weight of package 5 (max 1000 kg): 900
Is package 5 fragile (0 = No, 1 = Yes)? 1
Enter weight of package 6 (max 1000 kg): 300
Is package 6 fragile (0 = No, 1 = Yes)? 0
Enter weight of package 7 (max 1000 kg): 200
Is package 7 fragile (0 = No, 1 = Yes)? 0
Expected Output
Package 1 (weight: 500 kg) added to current truck (total weight: 500 kg).
Package 2 (weight: 700 kg) requires a separate truck (fragile).
Package 3 (weight: 400 kg) added to current truck (total weight: 900 kg).
Truck full. Starting a new truck for package 4 (weight: 600 kg).
Package 5 (weight: 900 kg) requires a separate truck (fragile).
Package 6 (weight: 300 kg) added to current truck (total weight: 900 kg).
Truck full. Starting a new truck for package 7 (weight: 200 kg).
Minimum number of trucks required: 5
- The program starts by asking the user to input the total number of packages. This value is stored in the variable
n
. - A variable
truckCount
is initialized to 0 to keep track of the total number of trucks used, andcurrentWeight
is initialized to 0 to track the weight loaded into the current truck. - A
for
loop is used to process each package. Inside the loop, the program asks the user to input the weight of the current package (weight
). - If the weight exceeds 1000 kg, the program displays an error message and decrements the loop counter (
i--
) to ensure the user is prompted to re-enter the weight for the same package. - The program also asks the user if the package is fragile. If the package is fragile
(fragile == 1)
, it is assigned to a separate truck immediately. ThetruckCount
is incremented, and a message is displayed indicating that the package requires a dedicated truck. - If the package is not fragile
(fragile == 0)
, the program checks whether adding the package to the current truck would exceed the 1000 kg limit. If it does not, the package is added to the current truck,currentWeight
is updated, and a message is displayed showing the total weight in the current truck. - If adding the package would exceed the weight limit, the program increments
truckCount
, displays a message indicating that a new truck is being started, and resetscurrentWeight
to the weight of the current package. - After processing all packages, the program checks if there is any remaining weight in the current truck. If
currentWeight > 0
, the program incrementstruckCount
to account for this partially loaded truck. - Finally, the program prints the total number of trucks used
(truckCount)
to transport all the packages.
#include <stdio.h> int main() { int n; int weight; int fragile; int truckCount = 0; int currentWeight = 0; printf("Enter the number of packages: "); scanf("%d", &n); for (int i = 1; i <= n; i++) { printf("\nEnter weight of package %d (max 1000 kg): ", i); scanf("%d", &weight); if (weight > 1000) { printf("Error: Package weight cannot exceed 1000 kg. Please re-enter the weight for package %d.\n", i); i--; continue; } printf("Is package %d fragile (0 = No, 1 = Yes)? ", i); scanf("%d", &fragile); if (fragile == 1) { truckCount++; printf("Package %d (weight: %d kg) requires a separate truck (fragile).\n", i, weight); } else { if (currentWeight + weight <= 1000) { currentWeight += weight; printf("Package %d (weight: %d kg) added to current truck (total weight: %d kg).\n", i, weight, currentWeight); } else { truckCount++; printf("Truck full. Starting a new truck for package %d (weight: %d kg).\n", i, weight); currentWeight = weight; } } } if (currentWeight > 0) { truckCount++; } printf("\nMinimum number of trucks required: %d\n", truckCount); return 0; }
Energy Core Stability
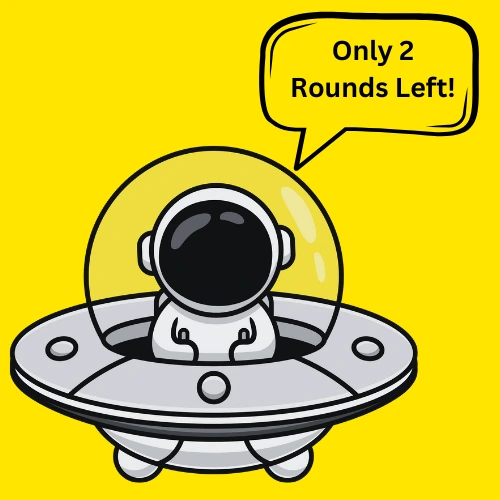
You are in charge of keeping the energy core of a futuristic spaceship stable. The core starts with an initial energy level, and your goal is to maintain its stability for a set number of rounds. During each round, the energy core goes through specific changes based on the round number. If the round number is a multiple of 4, the energy level increases by the square of the round number. If the round number is a multiple of 6, the energy level decreases by twice the round number. If the round number is not a multiple of either, the energy is reduced to half its current value.
The process ends if the energy level falls below zero or exceeds the critical limit of 1000. Write a program in C language that takes the initial energy level and the number of rounds as input, calculates the energy changes for each round, and determines how many rounds the core remains stable.
Test Case
Input
Enter the initial energy level: 200
Enter the total number of stabilization rounds: 10
Expected Output
Round 1: Energy level halved. Current energy level: 100
Round 2: Energy level halved. Current energy level: 50
Round 3: Energy level halved. Current energy level: 25
Round 4: Energy level increased by 16. Current energy level: 41
Round 5: Energy level halved. Current energy level: 20
Round 6: Energy level decreased by 12. Current energy level: 8
Round 7: Energy level halved. Current energy level: 4
Round 8: Energy level increased by 64. Current energy level: 68
Round 9: Energy level halved. Current energy level: 34
Round 10: Energy level halved. Current energy level: 17
Congratulations! You stabilized the energy core for all 10 rounds. Final energy level: 17
- The program begins by asking the user to input the initial energy level of the spaceship’s core and the total number of stabilization rounds. These values are stored in the variables
energy_level
andtotal_rounds
. - The variable
round
is initialized to 1 to represent the first round of the stabilization process. - The program then enters a
while
loop, which runs as long as the current round number is less than or equal to the total number of rounds specified by the user. - At the start of each round, the program checks the round number to determine how the energy level should be updated.
- If the round number is a multiple of 4, the energy level increases by the square of the round number. A message is displayed indicating the energy increase and the updated energy level.
- If the round number is a multiple of 6, the energy level decreases by twice the round number. A message is displayed showing the energy decrease and the updated energy level.
- If the round number is neither a multiple of 4 nor 6, the energy level is halved. A message is displayed showing the new energy level.
- After updating the energy level, the program checks if the energy level has gone out of bounds (less than 0 or greater than 1000). If the energy level is outside these limits, the program prints a message indicating that the stabilization failed in the current round, and the loop is terminated using the
break
statement. - If the energy level remains within bounds, the program increments the
round
variable and proceeds to the next round. - Once the loop ends, the program checks whether all rounds were successfully completed.
- If the loop was terminated early due to the energy level going out of bounds, the program does not display the success message, as stabilization failed.
- If the
round
variable exceeds the total number of rounds, it means the stabilization was successful. A congratulatory message is displayed, showing the total rounds completed and the final energy level.
#include <stdio.h> int main() { int energy_level; int total_rounds; printf("Enter the initial energy level: "); scanf("%d", &energy_level); printf("Enter the total number of stabilization rounds: "); scanf("%d", &total_rounds); int round = 1; while (round <= total_rounds) { printf("\nRound %d: ", round); if (round % 4 == 0) { energy_level += round * round; printf("Energy level increased by %d. Current energy level: %d\n", round * round, energy_level); } else if (round % 6 == 0) { energy_level -= round * 2; printf("Energy level decreased by %d. Current energy level: %d\n", round * 2, energy_level); } else { energy_level /= 2; printf("Energy level halved. Current energy level: %d\n", energy_level); } if (energy_level < 0 || energy_level > 1000) { printf("Energy level out of bounds in round %d. Stabilization failed!\n", round); break; } round++; } if (round > total_rounds) { printf("\nCongratulations! You stabilized the energy core for all %d rounds. Final energy level: %d\n", total_rounds, energy_level); } return 0; }