Ready to dive into some Hands-On Scenario Coding questions? let us tackle some Real life-based Scenario questions testing your logic-building and understanding skills. In this one, you will be focusing on questions based on loops in C++. solve the uniquely designed scenarios with the side of visuals to enhance the learning outcome, maximizing to its best.
The Guessing Game
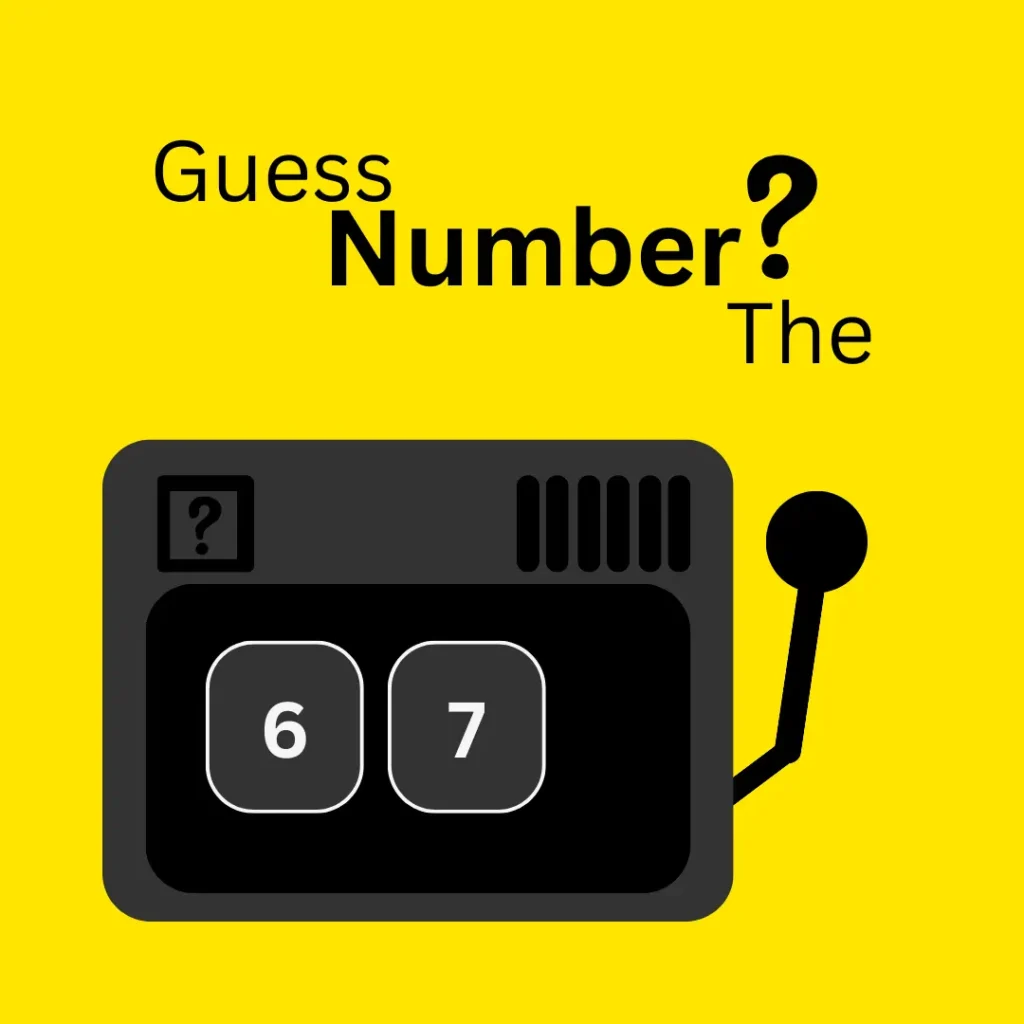
You are a game developer assigned to design a simple game where players have up to 10 chances to guess a secret number between 1 and 100. it also has a function that, after each guess, tells if it is high or low depending on the difference in the guess and the secret number. Now tasked to make this game in C++ language.
Test Case 1:
Input: Secret number = 42, Guesses = 30, 50, 42
Output: “Too low, try again: Too high, try again: Congratulations! You guessed the number!”
Test Case 2:
Input: Secret number = 75, Guesses = 60, 80, 70, 72, 75 (within 10 tries)
Output: “Too low, try again: Too high, try again: Congratulations! You
Explanation:
1. The computer picks a number between 1 and 100.
2. You have 10 chances to guess the number.
3. After each guess, the computer tells you if you need to guess higher or lower.
4. If you guess right, you win. If you don’t guess in 10 tries, you lose.
#include <iostream> #include <cstdlib> using namespace std; int main() { srand(0); // Seed for random number generation int secretNumber = rand() % 100 + 1; // Random number between 1 and 100 int guess; int maxTries = 10; cout << "Guess the number (between 1 and 100). You have 10 tries: " << endl; for (int i = 0; i < maxTries; i++) { cin >> guess; if (guess < secretNumber) { cout << "Too low, try again: "; } else if (guess > secretNumber) { cout << "Too high, try again: "; } else { cout << "Congratulations! You guessed the number!" << endl; return 0; // End the program if the guess is correct } } cout << "Sorry, you've used all your tries. The correct number was " << secretNumber << "." << endl; return 0; }
The ATM Machine
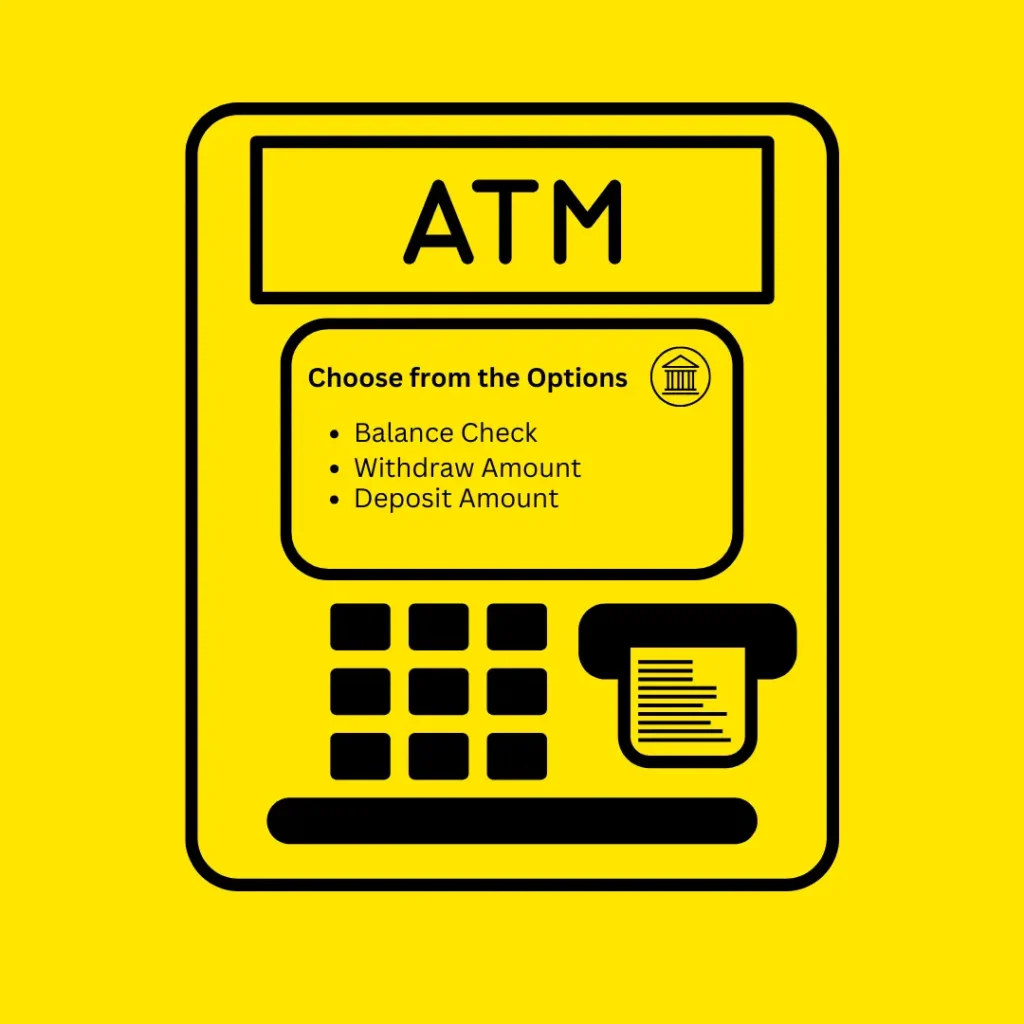
You are a programmer at a software development company and received a project to make the software for an ATM machine that will be installed in their new bank branch. The ATM should allow users to check their balance, deposit money, withdraw money, and exit the program. Write a C++ program that simulates these basic ATM functionalities and keeps them in the loop until the user decides to exit the ATM software.
Test Case 1:
Input: Option 1 (Check Balance)
Output: “Your balance is: 1000”
Test Case 2:
Input: Option 2 (Deposit Money), Amount = 500
Output: “Deposit successful. New Balance: 1500”
Explanation:
1. The program starts with an initial balance.
2. It displays a menu with options to check your balance, deposit money, withdraw money, or exit.
3. Based on the user’s choice, it performs the corresponding operation.
4. The loop continues until the user chooses to exit.
#include <iostream> using namespace std; int main() { int balance = 1000; // Initial balance int option, amount; cout << "Welcome to the ATM!" << endl; cout << "1. Check Balance" << endl; cout << "2. Deposit Money" << endl; cout << "3. Withdraw Money" << endl; cout << "4. Exit" << endl; while (true) { cout << "Enter your option: "; cin >> option; if (option == 1) { cout << "Your balance is: " << balance << endl; } else if (option == 2) { cout << "Enter amount to deposit: "; cin >> amount; balance += amount; cout << "Deposit successful. New balance: " << balance << endl; } else if (option == 3) { cout << "Enter amount to withdraw: "; cin >> amount; if (amount > balance) { cout << "Insufficient funds." << endl; } else { balance -= amount; cout << "Withdrawal successful. New balance: " << balance << endl; } } else if (option == 4) { cout << "Thank you for using the ATM. Goodbye!" << endl; break; } else { cout << "Invalid option. Please try again." << endl; } } return 0; }
Rock Paper Scissors
A great childhood memory is playing the classical game of Rock Paper Scissors, having the functionality that you can do it repeatedly in sets, each having 3 rounds, making it a best of three. As a developer, you have been tasked to replicate the game so that an app can be launched to play online. Write a simple C++ program that lets the user choose Rock, Paper, or Scissors and then displays who wins.
Test Case 1:
Input: User = ‘r’ (Rock)
Output: Computer = ‘s’ (Scissors), “You win!”
Test Case 2:
Input: User = ‘p’ (Paper)
Output: Computer = ‘r’ (Rock), “You win!”
Explanation:
1. Initialize user and computer choices.
2. Display instructions for the user to input ‘r’, ‘p’, or ‘s’.
3. Get the user’s input.
4. Randomly generate the computer’s choice.
5. Display the computer’s choice.
6. Compare the user’s and computer’s choices to decide the winner.
7. Display whether the user wins, loses, or ties.
#include <iostream> #include <cstdlib> using namespace std; int main() { char userChoice, computerChoice; string options = "rps"; // r = Rock, p = Paper, s = Scissors int userWins = 0, computerWins = 0; cout << "Welcome to Rock, Paper, Scissors! Best of 3 rounds." << endl; for (int i = 0; i < 3; i++) { cout << "Round " << i + 1 << ": Enter 'r' for Rock, 'p' for Paper, or 's' for Scissors: "; cin >> userChoice; computerChoice = options[rand() % 3]; // Randomly choose r, p, or s for computer cout << "Computer chose: " << computerChoice << endl; if (userChoice == computerChoice) { cout << "It's a tie!" << endl; } else if ((userChoice == 'r' && computerChoice == 's') || (userChoice == 'p' && computerChoice == 'r') || (userChoice == 's' && computerChoice == 'p')) { cout << "You win this round!" << endl; userWins++; } else { cout << "Computer wins this round!" << endl; computerWins++; } cout << "Current score - You: " << userWins << ", Computer: " << computerWins << endl; } if (userWins > computerWins) { cout << "Congratulations! You win the game!" << endl; } else if (computerWins > userWins) { cout << "Sorry! Computer wins the game!" << endl; } else { cout << "It's a tie game!" << endl; } return 0; }
Keep Tabs on Your Spending
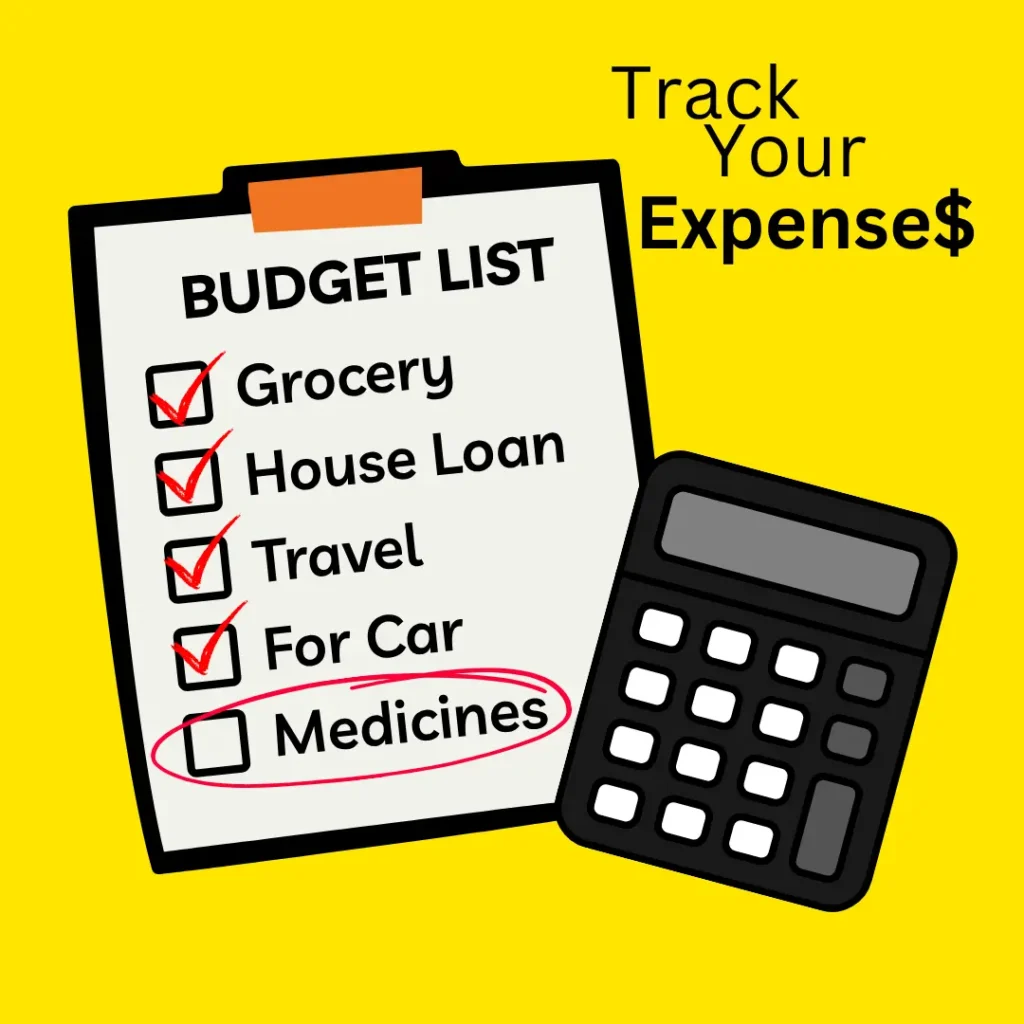
Keeping track of spending is a major part of our lives and as a developer, you are tasked to make a program that allows people to keep track of their spending and calculate what is the total expense to keep things in order. Write a simple C++ program that asks for the number of expenses, takes each expense as input, and then calculates the total amount spent.
Test Case 1:
Input: Number of expenses: 3
Expense 1: 50
Expense 2: 30
Expense 3: 20
Output: “Your total expenses are: $100”
Test Case 2:
Input: Number of expenses: 2
Expense 1: 45.5
Expense 2: 60.75
Output: “Your total expenses are: $106.25”
Explanation:
1. Ask the user for the number of expenses.
2. Initialize the total to zero.
3. Use a for loop to input each expense.
4. Add each expense to the total.
5. Display the total expenses.
#include <iostream> using namespace std; int main() { int numExpenses; float expense, total = 0; cout << "Welcome to Easy Budget Tracker!" << endl; cout << "How many expenses do you have? "; cin >> numExpenses; for (int i = 1; i <= numExpenses; i++) { cout << "Enter expense " << i << ": "; cin >> expense; total += expense; } cout << "Your total expenses are: $" << total << endl; return 0; }
Stock Market Evaluator
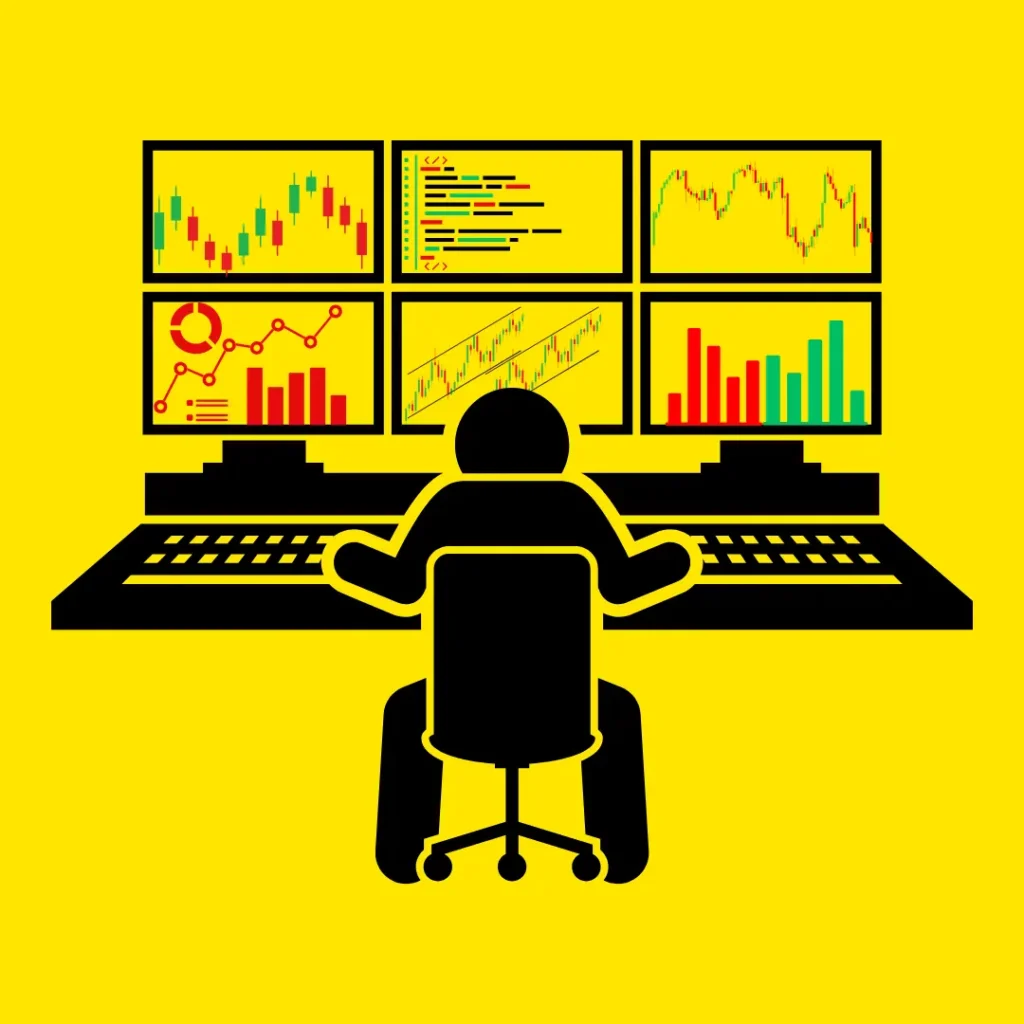
The Stock market is a place where the pricing is never stable. Evaluators collect data of weeks about the pricing keeping tabs but still make a mess when analyzing at the end for the next evaluation. They require something that allows them to take the data from a set number of days with the per-day pricing and in return receive the highest, lowest, and average price of the stock they have been keeping tabs on. Write a C++ program that takes the number of days and stock prices as input and calculates these values.
Test Case 1:
Input: Number of days: 3
Day 1 price: 150
Day 2 price: 200
Day 3 price: 180
Output: Highest stock price: $200
Lowest stock price: $150
Average stock price: $176.67
Test Case 2:
Input: Number of days: 4
Day 1 Price: 100
Day 2 Price: 120
Day 3 Price: 110
Day 4 Price: 105
Output: Highest stock price: $120
Lowest stock price: $100
Average stock price: $108.75
Explanation:
1. Ask the user for the number of days.
2. Initialize variables for highest, lowest, and total prices.
3. Use a for loop to input the stock prices for each day.
4. Compare each price to find the highest and lowest.
5. Calculate the average price and display all results.
#include <iostream> using namespace std; int main() { int numDays; float price, highest = 0, lowest = 0, total = 0; cout << "Welcome to Stock Market Evaluator!" << endl; cout << "Enter the number of days you have stock prices for: "; cin >> numDays; for (int i = 1; i <= numDays; i++) { cout << "Enter the stock price for day " << i << ": "; cin >> price; total += price; if (i == 1) { highest = price; lowest = price; } else { if (price > highest) highest = price; if (price < lowest) lowest = price; } } cout << "Highest stock price: $" << highest << endl; cout << "Lowest stock price: $" << lowest << endl; cout << "Average stock price: $" << total / numDays << endl; return 0; }
The Space Ship Route
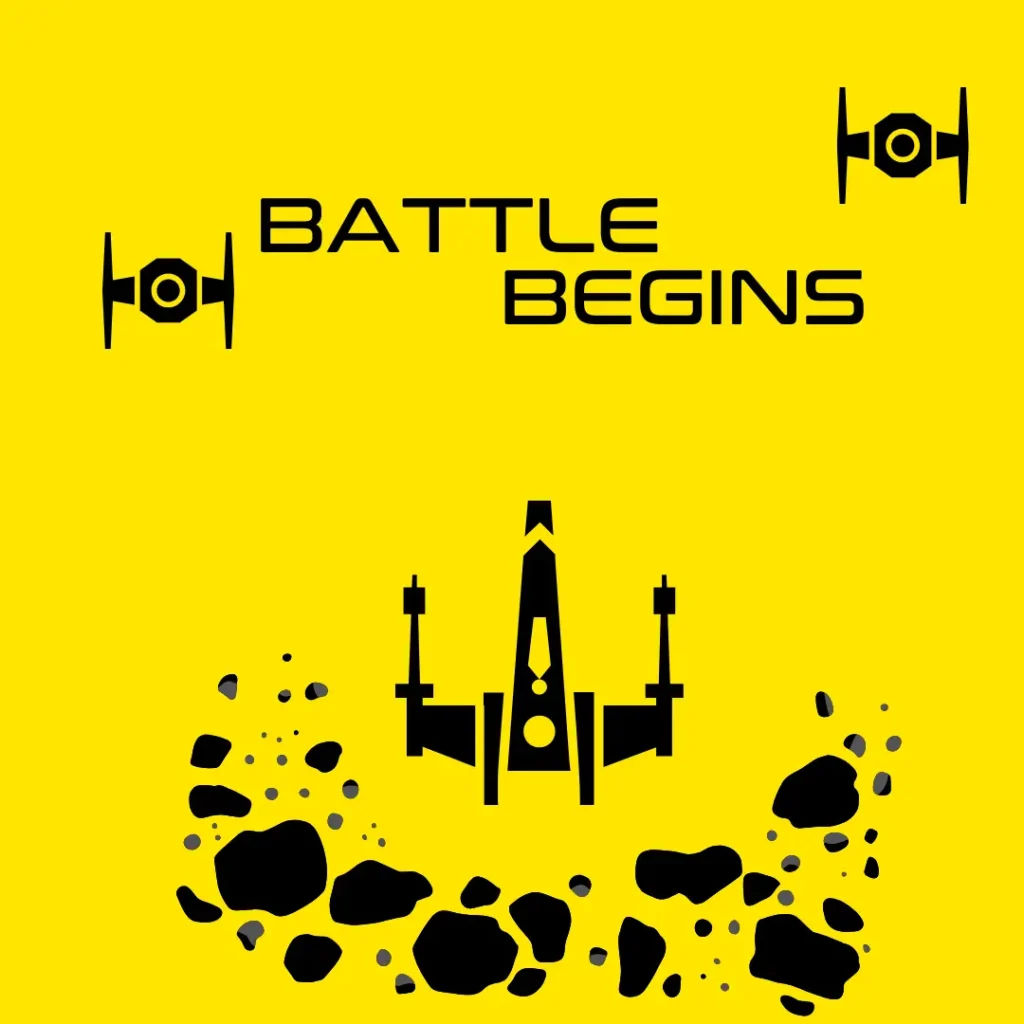
You are a game developer with a friend who is interested in space and hires you to Design a simple game where a spaceship starts with a certain health level. Each round, an alien attack causes random damage to the spaceship. The game continues for a number of rounds, and the player wins if the spaceship survives all rounds. Write a C++ program for this game.
Test Case 1:
Input: Number of rounds: 5
Output: Round 1: Alien attack caused 15 damage!
Spaceship health is now: 85
…
You survived all rounds! Well done!
Test Case 2:
Input: Number of rounds: 3
Output: Round 1: Alien attack caused 18 damage!
Spaceship health is now: 82
…
Your spaceship has been destroyed! Game over.
Explanation:
1. Initialize spaceship health to 100.
2. Ask the player for the number of rounds.
3. Use a for loop to simulate each round.
4. Generate random damage for each round.
5. Deduct the damage from the spaceship’s health.
6. Check if the spaceship’s health is zero or below to end the game.
7. Print game results.
#include <iostream> #include <cstdlib> #include <ctime> using namespace std; int main() { int spaceshipHealth = 100; int alienAttack; int numRounds; cout << "Welcome to the Space Ship Defense Game!" << endl; cout << "How many rounds do you want to play? "; cin >> numRounds; srand(time(0)); // Seed for random number generation for (int i = 1; i <= numRounds; i++) { alienAttack = rand() % 20 + 1; // Random damage between 1 and 20 spaceshipHealth -= alienAttack; cout << "Round " << i << ": Alien attack caused " << alienAttack << " damage!" << endl; cout << "Spaceship health is now: " << spaceshipHealth << endl; if (spaceshipHealth <= 0) { cout << "Your spaceship has been destroyed! Game over." << endl; break; } } if (spaceshipHealth > 0) { cout << "You survived all rounds! Well done!" << endl; } return 0; }
The Robots Greetings
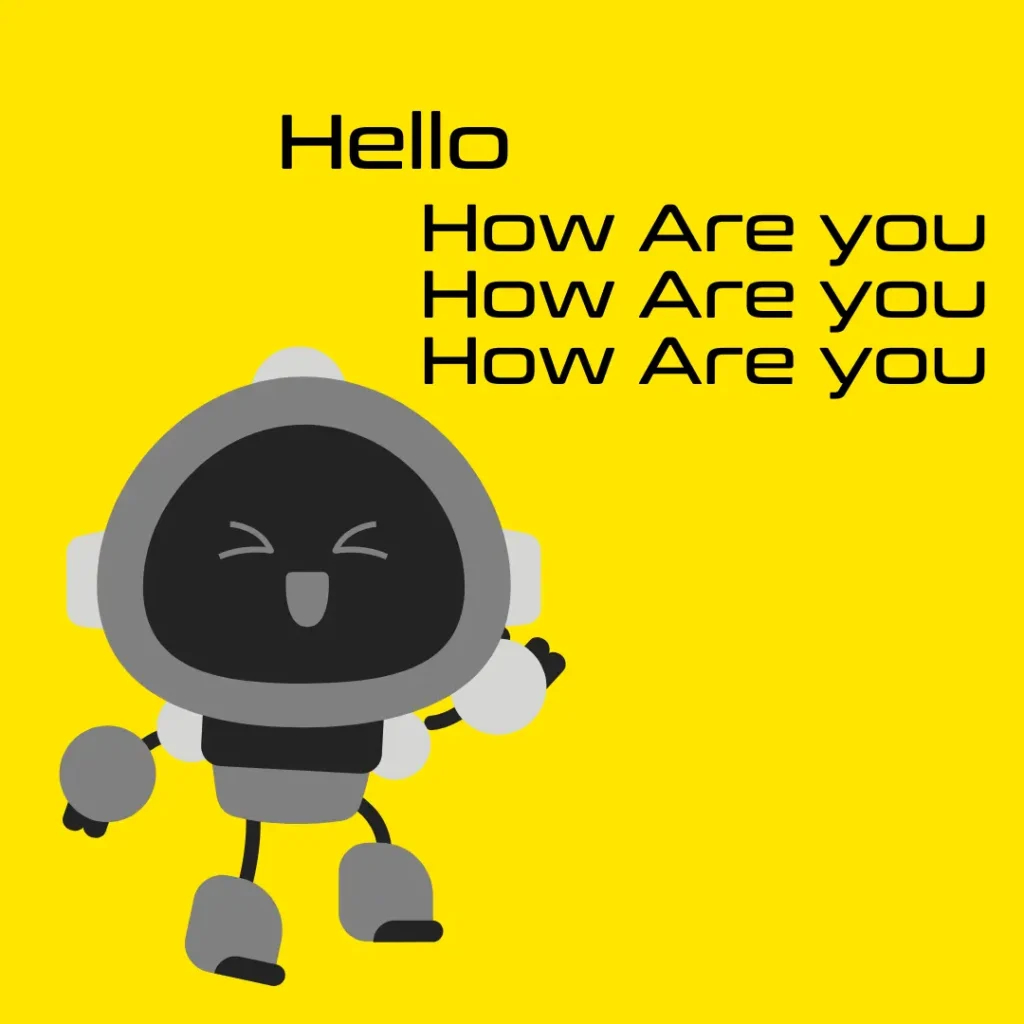
The Chinese are one of the humblest people with advancing technologies. You are part of a team making a greetings robot. You tell the robot your name and how many greetings you want. you are responsible for writing a C++ program that makes the robot greet you accordingly.
Test Case 1:
Input: Number of greetings: 3
Name: Subhan
Output: Greetings, Subhan! This is greeting number 1.
Greetings, Subhan! This is greeting number 2.
Greetings, Subhan! This is greeting number 3.
Test Case 2:
Input: Number of greetings: 2
Name: Hassan
Output: Greetings, Hassan! This is greeting number 1.
Greetings, Hassan! This is greeting number 2.
Explanation:
1. Ask the user for the number of greetings.
2. Ask for the user’s name.
3. Use a for loop to repeat the greeting the specified number of times.
4. Display the personalized greeting with the user’s name.
#include <iostream> #include <string> using namespace std; int main() { int numGreetings; string name; cout << "Hello! I am a friendly robot." << endl; cout << "How many times would you like me to greet you? "; cin >> numGreetings; cout << "What is your name? "; cin >> name; for (int i = 1; i <= numGreetings; i++) { cout << "Greetings, " << name << "! This is greeting number " << i << "." << endl; } return 0; }
Star Exploration Tracking
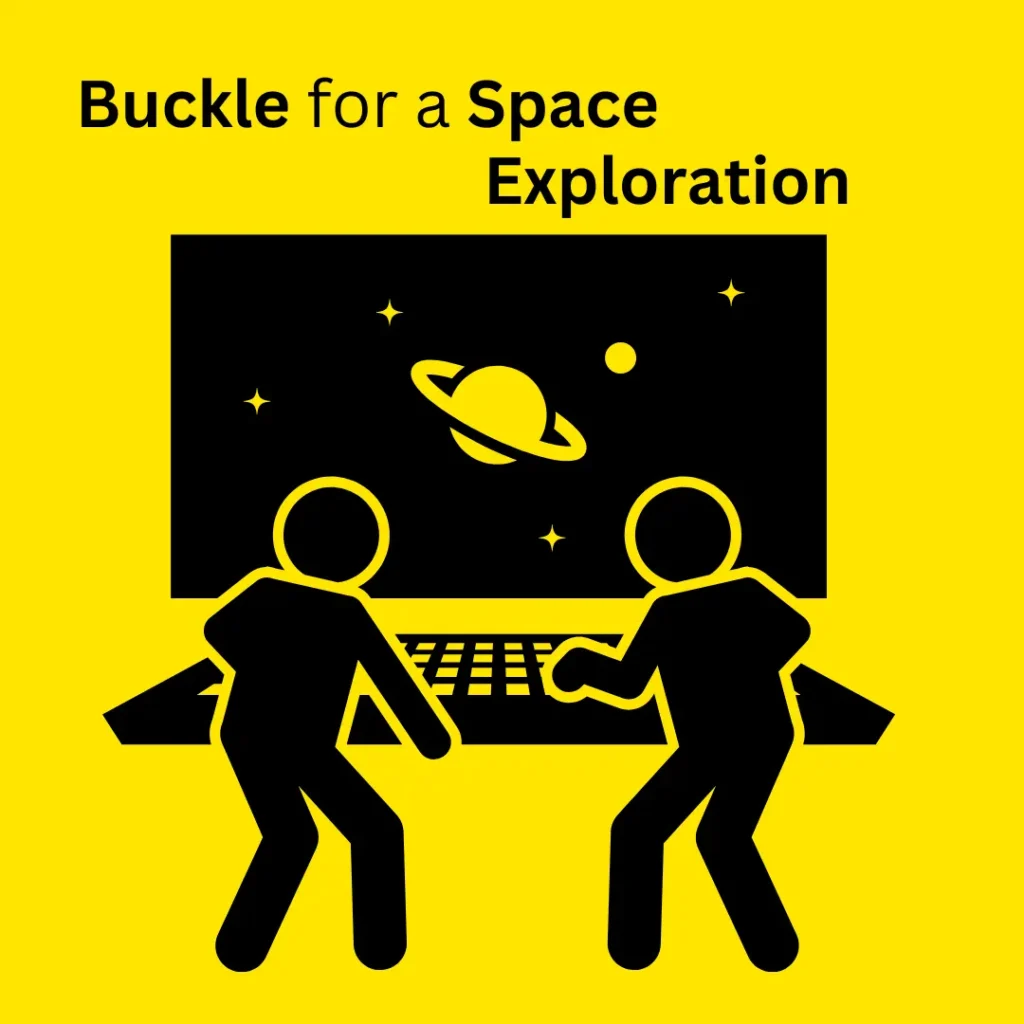
You are a Space Explorer, and you are required to record all the stars that you encounter, along with the names of the stars. In order to help with that, write a C++ program that asks for the number of stars you explored and then records the name of each star.
Test Case 1:
Input: Number of stars: 3
Star names: Vega, Sirius, Betelgeuse
Output: Enter the name of star 1: Vega
Star 1: Vega has been recorded.
Enter the name of star 2: Sirius
Star 2: Sirius has been recorded.
Enter the name of star 3: Betelgeuse
Star 3: Betelgeuse has been recorded.
You have successfully recorded 3 stars. Great job, explorer!
Test Case 2:
Input: Number of stars: 2
Star names: Polaris, Aldebaran
Output: Enter the name of star 1: Polaris
Star 1: Polaris has been recorded.
Enter the name of star 2: Aldebaran
Star 2: Aldebaran has been recorded.
You have successfully recorded 2 stars. Great job, explorer!
Explanation:
1. Ask the user how many stars they explored.
2. Use a for loop to repeat the input process for the specified number of stars.
3: For each iteration, ask for the star’s name.
4. Display a message confirming the recording of each star’s name.
5. Print a final message summarizing the total number of stars recorded.
#include <iostream> #include <string> using namespace std; int main() { int numStars; string starName; cout << "Welcome to the Star Exploration Tracker!" << endl; cout << "How many stars have you explored? "; cin >> numStars; for (int i = 1; i <= numStars; i++) { cout << "Enter the name of star " << i << ": "; cin >> starName; cout << "Star " << i << ": " << starName << " has been recorded." << endl; } cout << "You have successfully recorded " << numStars << " stars. Great job, explorer!" << endl; return 0; }
Travel Luggage Capacity
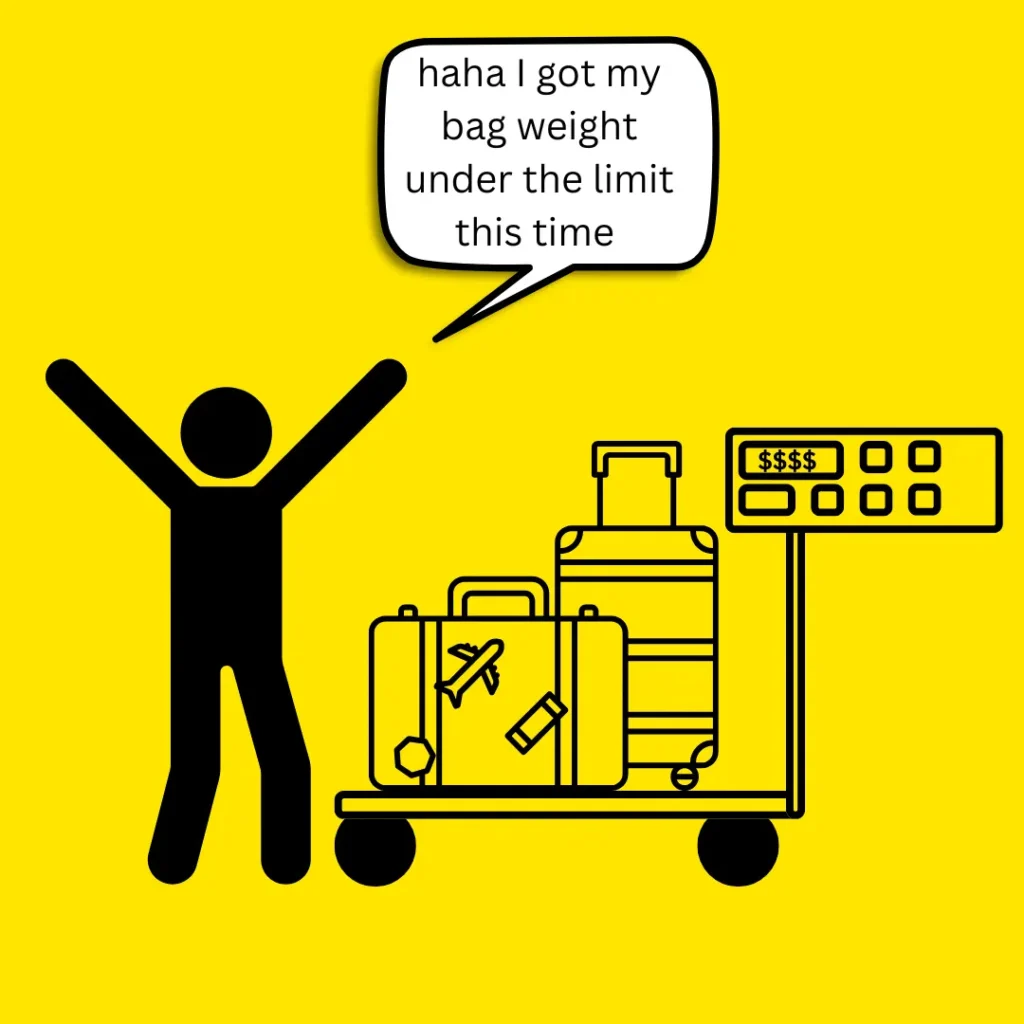
You are Traveling to another country, and every time, you seem to carry overweight luggage, costing you extra for the travel. To solve that problem, you are to write a C++ program that asks for the plane’s maximum luggage capacity, the number of bags, and the weight of each bag. The program should calculate the total luggage weight and indicate if it exceeds the plane’s capacity.
Test Case 1:
Input: Maximum capacity: 1000
Number of bags: 3
Weights: 300, 400, 200
Output: Total luggage weight: 900 kg
Luggage is within the plane’s capacity.
Test Case 2:
Input: Maximum capacity: 800
Number of bags: 3
Weights: 300, 300, 250
Output: Total luggage weight: 850 kg
Warning: Luggage exceeds the plane’s capacity!
Explanation:
1. Ask the user for the plane’s maximum luggage capacity.
2. Ask for the number of bags.
3. Use a for loop to get the weight of each bag and calculate the total weight.
4. Display the total luggage weight.
5. Check if the total weight exceeds the plane’s capacity and show an appropriate message.
#include <iostream> using namespace std; int main() { int numBags; float bagWeight, totalWeight = 0, maxCapacity; cout << "Enter the maximum luggage capacity of the plane (in kg): "; cin >> maxCapacity; cout << "Enter the number of bags: "; cin >> numBags; for (int i = 1; i <= numBags; i++) { cout << "Enter the weight of bag " << i << " (in kg): "; cin >> bagWeight; totalWeight += bagWeight; } cout << "Total luggage weight: " << totalWeight << " kg" << endl; if (totalWeight > maxCapacity) { cout << "Warning: Luggage exceeds the plane's capacity!" << endl; } else { cout << "Luggage is within the plane's capacity." << endl; } return 0; }
Asteroid Emergency
You are a software engineer at a space investigation company and they have discovered that there are asteroids that will impact Earth, you are tasked to write a C++ program that takes the number of asteroids and their sizes as input. The program should calculate the total potential impact force and provide a warning if it exceeds a critical level.
Test Case 1:
Input: Number of asteroids: 3
Sizes: 50, 60, 70
Output: Total potential impact force: 18000 units
Warning: High impact threat detected!
Test Case 2:
Input: Number of asteroids: 2
Sizes: 30, 40
Output: Total potential impact force: 7000 units
The impact threat is manageable.
Explanation:
1. The program asks the user to input the number of asteroids.
2. It then iterates through each asteroid, asking for its size.
3. The total potential impact force is calculated as the sum of all asteroid sizes multiplied by a constant factor (e.g., 100).
4. The program displays the total impact force.
5. If the impact force exceeds a set threshold, a warning is displayed.
#include <iostream> using namespace std; int main() { int numAsteroids; float asteroidSize, totalImpact = 0; cout << "Enter the number of asteroids: "; cin >> numAsteroids; for (int i = 1; i <= numAsteroids; i++) { cout << "Enter the size of asteroid " << i << " (in meters): "; cin >> asteroidSize; totalImpact += asteroidSize * 100; // Assuming impact is size * 100 } cout << "Total potential impact force: " << totalImpact << " units" << endl; if (totalImpact > 10000) { cout << "Warning: High impact threat detected!" << endl; } else { cout << "Impact threat is manageable." << endl; } return 0; }
Customers Ratings Aggregator
You are managing a business and want to know how well your customers are rating your service. Write a C++ program that takes multiple customer ratings as input and calculates the average rating.
Test Case 1:
Input: Number of ratings: 4
Ratings: 4.5, 3.0, 5.0, 4.0
Output: The average customer rating is: 4.125 out of 5
Test Case 2:
Input: Number of ratings: 3
Ratings: 2.0, 3.5, 4.5
Output: The average customer rating is: 3.33333 out of 5
Explanation:
1. The program asks the user to input the number of customer ratings.
2. It then iterates through each rating, adding them to a total sum.
3. The program calculates the average rating by dividing the total sum by the number of ratings.
4. It displays the average rating to the user.
#include <iostream> using namespace std; int main() { int numRatings; float rating, totalRatings = 0, averageRating; cout << "Enter the number of customer ratings: "; cin >> numRatings; for (int i = 1; i <= numRatings; i++) { cout << "Enter rating " << i << " (out of 5): "; cin >> rating; totalRatings += rating; } averageRating = totalRatings / numRatings; cout << "The average customer rating is: " << averageRating << " out of 5" << endl; return 0; }