Programming is like solving puzzles, where each problem requires logical thinking and a clear solution. But sometimes, puzzles require you to make different decisions based on various conditions, with each choice leading to a new path. This is where nested if-else statements come in. They let you follow different paths based on multiple conditions, helping you make real-time decisions as your program runs.
If you’ve already worked on basic if-else and operator-based questions in Python, you’ve built a strong foundation. Now, it’s time to test your skills in making decisions at a slightly advanced level with nested if-else. These challenges will show you how to handle more complex decision-making. With simple explanations and easy-to-follow examples, you’ll improve your coding skills and feel more confident tackling logical problems!
Mood-Based Playlist Suggestion
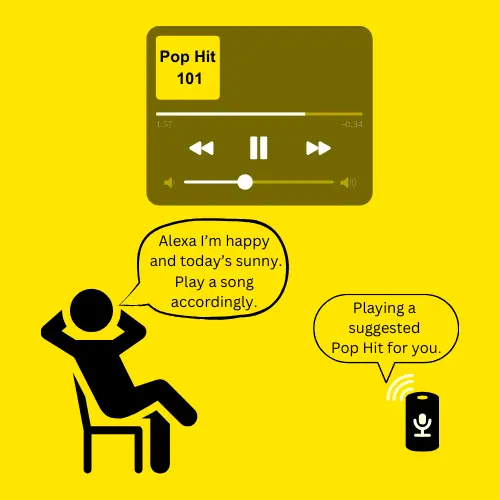
Imagine you’re using a music app that suggests a playlist based on both your mood and the weather outside. The app uses nested if-else statements to select the best playlist for you:
If you’re feeling “Happy” and it’s “Sunny,” the app suggests a fun playlist like “Pop Hits.”
If you’re feeling “Happy” but the weather isn’t “Sunny,” it suggests the “Top Charts” playlist.
If you’re feeling “Sad” and it’s “Rainy,” the app suggests a calming “Sad Acoustic” playlist.
If you’re feeling “Sad” but it’s not “Rainy,” the app suggests the “Top Charts” playlist.
If you’re feeling “Relaxed,” it always suggests the laid-back “Chill Vibes” playlist, regardless of the weather.
If your mood doesn’t match any of the above conditions, the app defaults to suggesting the “Top Charts” playlist.
Write a Python program that takes the user’s mood and the weather as input, and then suggests a playlist based on these conditions.
Test Case:
Input:
Mood = Happy
Weather = Sunny
Expected Output:
Suggested Playlist: Pop Hits.
Explanation:
This program recommends a playlist based on the user’s mood and the current weather conditions.
- First, the program prompts the user to input their current mood
mood = input("How are you feeling (Happy/Sad/Relaxed)? ")
and the weather usingweather = input("What's the weather like (Sunny/Rainy)? ")
. - If the
mood
is"Happy"
, the program checks theweather
.- If the
weather
is"Sunny"
, it printsprint("Suggested Playlist: Pop Hits.")
, recommending upbeat music. - Else, it prints
print("Suggested Playlist: Top Charts.")
, suggesting a general playlist.
- If the
- If the
mood
is"Sad"
, the program again checks theweather
.- If the
weather
is"Rainy"
, it printsprint("Suggested Playlist: Sad Acoustic.")
, aligning with the mood and ambiance. - Else, it prints
print("Suggested Playlist: Top Charts.")
, offering a general playlist to uplift the user.
- If the
- Or if the
mood
is"Relaxed"
, the program skips checking theweather
and directly printsprint("Suggested Playlist: Chill Vibes.")
, recommending calm and soothing music. - If the user enters a mood other than
"Happy"
,"Sad"
, or"Relaxed"
, the program defaults to printingprint("Suggested Playlist: Top Charts.")
, ensuring a recommendation is always given.
mood = input("How are you feeling (Happy/Sad/Relaxed)? ") weather = input("What's the weather like (Sunny/Rainy)? ") if mood == "Happy": if weather == "Sunny": print("Suggested Playlist: Pop Hits.") else: print("Suggested Playlist: Top Charts.") elif mood == "Sad": if weather == "Rainy": print("Suggested Playlist: Sad Acoustic.") else: print("Suggested Playlist: Top Charts.") elif mood == "Relaxed": print("Suggested Playlist: Chill Vibes.") else: print("Suggested Playlist: Top Charts.")
Calculating Shipping Cost
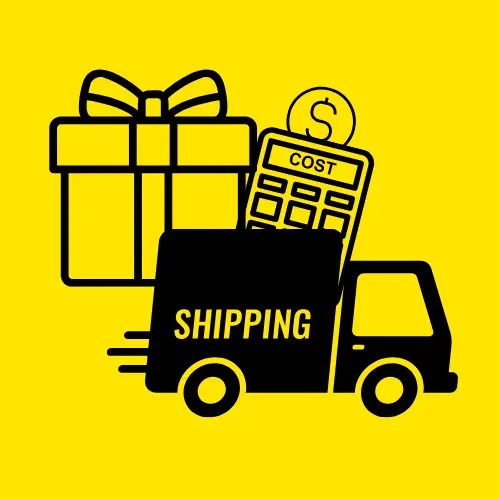
You are sending a gift to a friend, and you need to figure out how much it will cost to ship it. The shipping cost depends on the weight of the gift and the destination. If the weight is less than 5 kg, it costs less to send. If it’s heavier, the cost increases. Additionally, international shipping is more expensive than local shipping.
Write a Python program that calculates the shipping cost based on the weight of the gift and whether the destination is local or international.
Test Case:
Input:
Weight of the gift: 3 kg
Destination: international
Expected Output:
Shipping cost: $15
Explanation:
This program calculates the shipping cost for a gift based on its weight and the destination.
- The user is first prompted to input the gift’s weight using
weight = float(input("Enter the weight of the gift (kg): "))
. Thefloat()
function treats the input as a decimal number. - Next, the program asks for the shipping destination and stores it in
destination
variable. - The program checks if the gift’s weight is less than 5 kg using
if weight < 5:
.- If the weight is less than 5 kg and the destination is
"international"
(checked usingif destination == "international":
), the program printsprint("Shipping cost: $15")
, indicating a lower shipping cost for lightweight international packages. - If the weight is less than 5 kg but the destination is not
"international"
(assumed to be"local"
), it printsprint("Shipping cost: $5")
, assigning a smaller fee for lightweight local deliveries.
- If the weight is less than 5 kg and the destination is
- Else, if the weight is not less than 5 kg (i.e., the gift is heavier), the program moves to the
else
block for the weight.- Within this block, if the destination is
"international"
(checked usingif destination == "international":
), it printsprint("Shipping cost: $30")
, indicating a higher shipping fee for heavy international packages. - If the destination is
"local"
, it printsprint("Shipping cost: $10")
, setting a reduced cost for heavy packages shipped locally.
- Within this block, if the destination is
weight = float(input("Enter the weight of the gift (kg): ")) destination = input("Enter the destination (local, international): ") if weight < 5: if destination == "international": print("Shipping cost: $15") else: print("Shipping cost: $5") else: if destination == "international": print("Shipping cost: $30") else: print("Shipping cost: $10")
Personalized Workout Plan
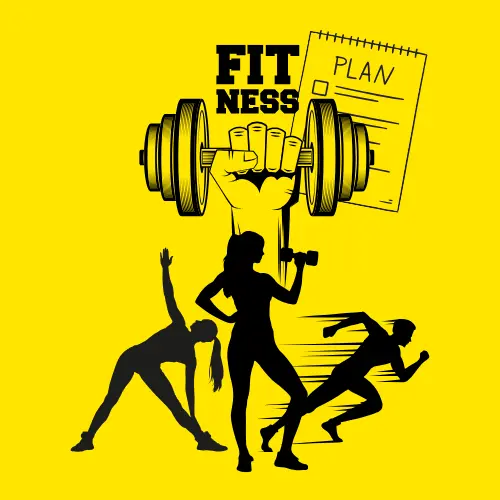
Suppose you’ve joined a fitness center that offers personalized fitness plans based on your BMI, age, and activity level. The center recommends different plans to help you reach your fitness goals, whether it’s losing weight, maintaining weight, gaining muscle, or improving general wellness.
If your BMI is above 25 and you’re between 30 and 50 years old with a “Low” activity level, they recommend a “Weight Loss Bootcamp” with a target weight and tips for starting cardio and light exercises.
If your BMI is between 18.5 and 25 and you have a “High” activity level, they recommend a “Muscle Gain Challenge” with higher calorie intake and intense workouts.
If your BMI is below 18.5 and you’re under 25 or have a “Very High” activity level, they suggest a “Weight Gain Plan” with a target weight and a focus on muscle building.
For everyone else, they provide a “Health Boost Plan” to stay active and eat a balanced diet.
Test Case:
Input:
Weight: 85 kg
Height: 1.75 m
Age: 35
Activity Level: Low
Expected Output:
Weight Loss Bootcamp. Target weight: 67.38 kg. Start with cardio and light exercises!
Explanation:
This program analyzes the user’s health based on their weight, height, age, and activity level and suggests a personalized health plan according to the Body Mass Index (BMI).
- The program starts by asking the user for their
weight
in kilograms (weight
) and theirheight
in meters (height
).Thefloat()
function used here treats the input as a decimal number. - It then asks for the user’s
age
(age
) and their activity level (activity_level
). Theint()
function used for the age variable treats the input as an integer whereas theactivity_level
is treated as astring
. - The BMI is calculated using the formula
bmi = weight / (height ** 2)
determines whether the user is underweight, healthy, or overweight. - If BMI > 25:
- The program further checks if the user’s age is between 30 and 50 and if their activity level is
"Low"
(if 30 <= age <= 50 and activity_level == "Low":
).- If true, it calculates a target weight using the formula
22 * (height ** 2)
and suggests a weight-loss boot camp.
- If true, it calculates a target weight using the formula
- If the conditions are not met, the program suggests a general health boost plan with daily brisk walks and dietary changes
- The program further checks if the user’s age is between 30 and 50 and if their activity level is
- Else, if 18.5 <= BMI <= 25:
- The program checks if the activity level is
"High"
(if activity_level == "High":
).- If true, it suggests a muscle gain challenge and prints
Muscle Gain Challenge. Build strength and gain muscle with intense workouts!
along with the recommended calories.
- If true, it suggests a muscle gain challenge and prints
- If the activity level is not
"High"
, the program suggests a general health boost plan with moderate exercises and recommends standard maintenance calories (calories_needed = 2000
).
- The program checks if the activity level is
- If BMI < 18.5:
- The program checks if the user’s age is less than 25 or if their activity level is
"Very High"
(if age < 25 or activity_level == "Very High":
).- If true, it calculates the required weight using the formula
20 * (height ** 2)
and suggests a weight gain plan. It also recommends higher calories (calories_needed = 3000
).
- If true, it calculates the required weight using the formula
- If these conditions are not met, the program suggests a health boost plan focusing on protein-rich foods and light exercises and recommends slightly more calories (
calories_needed = 2500
).
- The program checks if the user’s age is less than 25 or if their activity level is
- Otherwise, if none of the above conditions are met, the program suggests a general health boost plan to stay active and healthy. It sets the recommended calories to
calories_needed = 2200
.
weight = float(input("Enter your weight in kg: ")) height = float(input("Enter your height in meters: ")) age = int(input("Enter your age: ")) activity_level = input("Enter activity level (Low/Moderate/High/Very High): ") # Calculate BMI bmi = weight / (height ** 2) if bmi > 25: if 30 <= age <= 50 and activity_level == "Low": target_weight = 22 * (height ** 2) # Calculate target weight for weight loss print(f"Weight Loss Bootcamp. Target weight: {target_weight:.2f} kg. Start with cardio and light exercises!") else: print("Health Boost Plan. Try daily brisk walks and small changes to your diet!") elif 18.5 <= bmi <= 25: if activity_level == "High": print("Muscle Gain Challenge. Build strength and gain muscle with intense workouts!") calories_needed = 2500 # Higher calories for muscle gain print(f"Recommended calories: {calories_needed} per day.") else: print("Health Boost Plan. Stay active with moderate exercises like jogging or cycling!") calories_needed = 2000 # Standard maintenance calories print(f"Recommended calories: {calories_needed} per day.") elif bmi < 18.5: if age < 25 or activity_level == "Very High": required_weight = 20 * (height ** 2) # Calculate target weight for weight gain print(f"Weight Gain Plan. Target weight: {required_weight:.2f} kg. Focus on muscle building and nutrient-rich foods!") calories_needed = 3000 # Higher calories for weight gain print(f"Recommended calories: {calories_needed} per day.") else: print("Health Boost Plan. Eat more protein-rich foods and try light exercises!") calories_needed = 2500 # Slightly more for healthy weight gain print(f"Recommended calories: {calories_needed} per day.") else: print("Health Boost Plan. Stay fit, eat healthy, and make every move count!") calories_needed = 2200 # Standard calories for general health print(f"Recommended calories: {calories_needed} per day.")
Study Plan for Exams
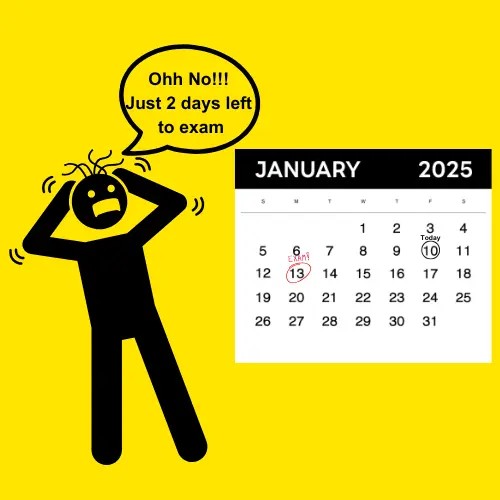
You wake up in a panic—your exams are way closer than you expected! You grab your phone and open a study app to figure out how to prepare in the little time you have. The app asks for two things: your subject and how much time is left. Based on your answers, it gives a study plan:
If your subject is Math:
If you have less than a week, focus on Practice Problems.
Otherwise, review the main Concepts.
If your subject is History:
If you have more than a week, make Detailed Notes.
Otherwise, focus on Memorizing key points.
For any other subject:
If you have more than a week, do a Thorough Study.
Otherwise, do a Quick Revision.
Write a Python program to implement this logic and suggest a study plan.
Test Case:
Input:
Subject: History
Time: More than a week
Expected Output:
Suggested Plan: Detailed Notes.
Explanation:
This program helps suggest a study plan based on the user’s chosen subject and the amount of time left for preparation.
- It begins by asking for the subject, which can be “Math,” “History,” or any other subject entered as “Other.” stored in the variable
subject
. - Then it asks how much time is left for preparation, with options “Less than a week” or “More than a week,” stored in the variable
time
. - The program then uses a series of
if-elif
conditions to determine the appropriate study plan. - If the subject is “Math,” it checks the value of
time
.- If the user has “Less than a week,” it suggests focusing on practicing problems to strengthen problem-solving skills and prints
"Suggested Plan: Practice Problems."
- If the time left is “More than a week,” it advises reviewing concepts for better understanding and outputs
"Suggested Plan: Concept Reviews."
- If the user has “Less than a week,” it suggests focusing on practicing problems to strengthen problem-solving skills and prints
- If the subject is “History,” the program again evaluates the time.
- For “More than a week,” it recommends preparing detailed notes for in-depth understanding and prints
"Suggested Plan: Detailed Notes."
- If the time is “Less than a week,” it suggests focusing on memorization techniques for retaining key facts and dates, displaying
"Suggested Plan: Memorization Techniques."
- For “More than a week,” it recommends preparing detailed notes for in-depth understanding and prints
- For any subject other than “Math” or “History,” the program handles it under the “Other” category.
- If the user has “More than a week,” it advises thorough study and outputs
"Suggested Plan: Thorough Study."
- For “Less than a week,” it recommends quick revision and prioritization of essential topics, printing
"Suggested Plan: Quick Revision."
- If the user has “More than a week,” it advises thorough study and outputs
subject = input("Enter your subject (Math/History/Other): ") time = input("How much time is left (Less than a week/More than a week)? ") if subject == "Math": if time == "Less than a week": print("Suggested Plan: Practice Problems.") else: print("Suggested Plan: Concept Reviews.") elif subject == "History": if time == "More than a week": print("Suggested Plan: Detailed Notes.") else: print("Suggested Plan: Memorization Techniques.") else: if time == "More than a week": print("Suggested Plan: Thorough Study.") else: print("Suggested Plan: Quick Revision.")
Financial Savings Plan
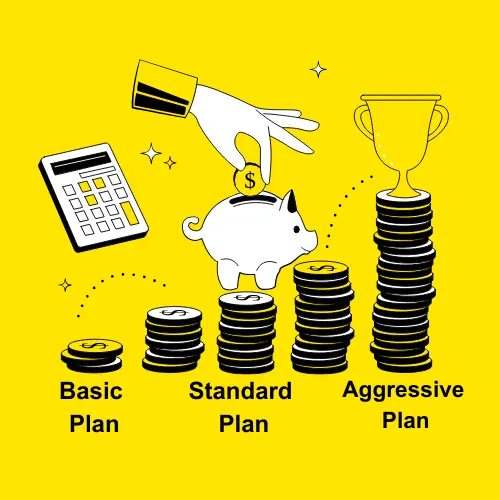
You want to improve your savings, so you use a financial app that provides a savings plan based on your income, age, and spending habits. If your monthly income is less than $2,000, and you are between 20 and 40 years old with “High” spending habits, the app suggests a Basic Savings Plan, calculating how much you need to save each month to reach $10,000 in 2 years. If your monthly income is between $2,000 and $5,000 and your spending habits are “Moderate,” the app recommends a Standard Savings Plan with a target of saving 20% of your income monthly. If your income is above $5,000, and you are younger than 30 or have “Low” spending habits, it suggests an Aggressive Investment Plan and calculates projected savings in 5 years with a 10% yearly return. In any other case, the app offers a Flexible Budget Plan with general tips to improve savings.
Write a Python program using nested if-else to determine the appropriate savings plan and display the calculated amounts where applicable.
Test Case :
Input:
Monthly Income: $3,000
Age: 35
Spending Habits: Moderate
Expected Output:
Standard Savings Plan. Monthly savings target: $600.00.
Explanation:
This program helps you choose a savings or investment plan based on your income, age, and spending habits.
- It asks for three things: your monthly income (
income
), your age (age
), and your spending habits (spending_habits
), which can be “High,” “Moderate,” or “Low”to suggest the best plan for the user. - If your
income
is less than $2000 and yourage
is between 20 and 40, it checks yourspending_habits
.- If the spending habits are “High”, it will suggest a Basic Savings Plan and calculate how much you need to save each month to reach $10,000 in 24 months using the formula
10000 / 24
. - If the spending habits are not “High”, it will suggest a Flexible Budget Plan instead.
- If the spending habits are “High”, it will suggest a Basic Savings Plan and calculate how much you need to save each month to reach $10,000 in 24 months using the formula
- Else, if the
income
is between $2000 and $5000, it checks if thespending_habits
are “Moderate”.- If yes, it calculates 20% of your
income
using the formulaincome * 0.2
and suggests a Standard Savings Plan with the monthly savings target displayed. - If the spending habits are not “Moderate”, it will recommend the Flexible Budget Plan.
- If yes, it calculates 20% of your
- If the
income
is greater than $5000, it checks if yourage
is below 30 or if yourspending_habits
are “Low”.- If either of these is true, it suggests an Aggressive Investment Plan and calculates how much you could save after 5 years using the formula
income * 0.3 * (1.1 ** 5)
showing the savings grow with a 10% annual increase. - If neither condition is met, it will suggest the Flexible Budget Plan.
- If either of these is true, it suggests an Aggressive Investment Plan and calculates how much you could save after 5 years using the formula
- The program uses nested
if-else
statements to choose the right plan using simple math operations like division (/
), multiplication (*
), and exponentiation (**
) to calculate the savings needed for each plan. It then displays the plan and the amounts, formatted to two decimal places for clarity.
income = float(input("Enter your monthly income in dollars: ")) age = int(input("Enter your age: ").strip()) spending_habits = input("Enter your spending habits (High/Moderate/Low): ") if income < 2000: if 20 <= age <= 40: if spending_habits == "High": monthly_savings = 10000 / 24 print(f"Basic Savings Plan. Monthly savings required: ${monthly_savings:.2f}.") else: print("Flexible Budget Plan. Explore tips to improve your savings.") else: print("Flexible Budget Plan. Explore tips to improve your savings.") elif 2000 <= income <= 5000: if spending_habits == "Moderate": savings_target = income * 0.2 print(f"Standard Savings Plan. Monthly savings target: ${savings_target:.2f}.") else: print("Flexible Budget Plan. Explore tips to improve your savings.") elif income > 5000: if age < 30 or spending_habits == "Low": projected_savings = income * 0.3 * (1.1 ** 5) print(f"Aggressive Investment Plan. Projected savings after 5 years: ${projected_savings:.2f}.") else: print("Flexible Budget Plan. Explore tips to improve your savings.") else: print("Flexible Budget Plan. Explore tips to improve your savings.")
Student Grading System
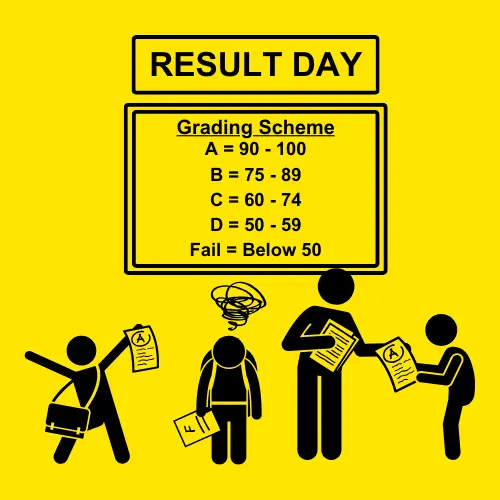
Imagine you’re a teacher, and your students just finished a big test. You want to quickly grade their papers. The grading works like this:
If a student scores 90 or more, they get an A grade.
If their score is between 75 and 89, they get a B grade.
If their score is between 60 and 74, they get a C grade.
If their score is between 50 and 60, they get a D grade.
If their score is below 50, they fail the test.
Write a Python program to determine the grade based on a student’s score.
Test Case:
Input:
Enter the student’s score: 85
Expected Output:
Your grade is: B
Explanation:
This program is designed to assign a letter grade based on the user’s score.
- The program first asks the user to input their score as an integer (using the
int()
andinput()
functions). - It then uses a series of nested conditional statements to determine the grade.
- The program first checks if the
score
is greater than or equal to 90.- If true, it prints
"Your grade is: A"
, since a score of 90 or above qualifies for an A grade. - If the score is below 90, it moves to the next check.
- If true, it prints
- The next condition checks if the
score
is greater than or equal to 75.- If true, it prints
"Your grade is: B"
, as scores between 75 and 89 receive a B grade. I - f the score is still below 75, the program proceeds to the next condition.
- If true, it prints
- Next, the program checks if the
score
is greater than or equal to 60.- If true, it prints
"Your grade is: C"
, indicating a C grade for scores between 60 and 74. - If the score is less than 60, the program checks if the score is greater than or equal to 50.
- If true, it prints
- If the score is greater than or equal to 50, it prints
"Your grade is: D"
, as scores between 50 and 59 receive a D grade. - If the score is below 50, the program finally prints
"You failed!"
, indicating that the user did not pass.
score = int(input("Enter your score: ")) if score >= 90: print("Your grade is: A") else: if score >= 75: print("Your grade is: B") else: if score >= 60: print("Your grade is: C") elif score >= 50: print("Your grade is: D") else: print("You failed!")
Internet Plan Selection
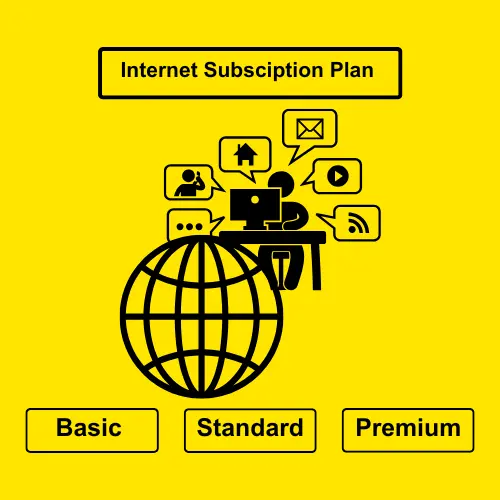
Imagine you’re working at an internet service provider, helping customers choose the best plan based on their data usage, location, and other needs. The available plans are Basic, Standard, and Premium, but there are additional conditions:
Basic Plan: For users who need less than 50GB of data. No special conditions apply.
Standard Plan: For users needing 50GB to 100 GB. However: If the user is local and chooses autopay, they get a 10% discount.
If the user is international or does not choose autopay, no discount applies, and an international surcharge of $15 is added.
Premium Plan: For users needing more than 100GB. However:If the user is local, the base price applies, but if they do not choose autopay, a $10 processing fee is added.
For international users, an international surcharge of $25 is added.
Write a Python program that helps customers determine their internet plan and the total cost.
Test Case:
Input:
Data Usage = 75
Location = local
autopsy = yes
Expected Output:
Your internet plan: Standard Plan
Discount applied: 10%
Final price: $45
Explanation:
The program calculates the final price for an internet plan based on the data usage, location (local or international), and autopay preference, with different charges for each condition.
- In this program, the user is prompted to input their data usage, location, and whether they want to enable autopsy.
- First, the program checks if the user’s
data_usage
is less than 50 GB.- If it is, the user is assigned the “Basic Plan” with a fixed price of $20. No further calculations are needed in this case.
- If the
data_usage
is between 50 and 100 GB, the user is assigned the “Standard Plan.” - The program then checks if the location is “local” or “international.”
- If the location is local and the user has selected autopay (“yes”), a 10% discount is applied to the
base_price_standard
, resulting in a lower price.- If autopay is not selected (“no”), a processing fee of $10 is added to the
base_price_standard
.
- If autopay is not selected (“no”), a processing fee of $10 is added to the
- If the location is international, an international surcharge of $15 is added to the
base_price_standard
.
- If the location is local and the user has selected autopay (“yes”), a 10% discount is applied to the
- If the
data_usage
exceeds 100 GB, the user is assigned the “Premium Plan.”- If the location is “local” and autopay is enabled (“yes”), no extra fees are applied, and the user is charged the base price.
- If autopay is disabled (“no”), a processing fee of $10 is added to the
base_price_premium
.
- If autopay is disabled (“no”), a processing fee of $10 is added to the
- If the location is “international,” an international surcharge of $25 is added to the
base_price_premium
.
- If the location is “local” and autopay is enabled (“yes”), no extra fees are applied, and the user is charged the base price.
- The program then prints out the final price after applying any discounts or additional charges.
data_usage = int(input("Enter your data usage in GB: ")) location = input("Enter your location (local, international): ") autopay = input("Do you want autopay? (yes/no): ") # Initialize variables for prices base_price_standard = 50 base_price_premium = 100 international_surcharge_standard = 15 international_surcharge_premium = 25 processing_fee = 10 discount_rate = 0.1 if data_usage < 50: print("Your internet plan: Basic Plan") print("Price: $20") else: if 50 <= data_usage <= 100: # Standard Plan print("Your internet plan: Standard Plan") if location == "local": if autopay == "yes": final_price = base_price_standard * (1 - discount_rate) print("Discount applied: 10%") else: final_price = base_price_standard + processing_fee print("Processing fee applied: $10") else: # International final_price = base_price_standard + international_surcharge_standard print("International surcharge applied: $15") print(f"Final price: ${final_price:.2f}") else: # Premium Plan print("Your internet plan: Premium Plan") if location == "local": if autopay == "no": final_price = base_price_premium + processing_fee print("Processing fee applied: $10") else: final_price = base_price_premium else: # International final_price = base_price_premium + international_surcharge_premium print("International surcharge applied: $25") print(f"Final price: ${final_price:.2f}")
Calculating Movie Discounts
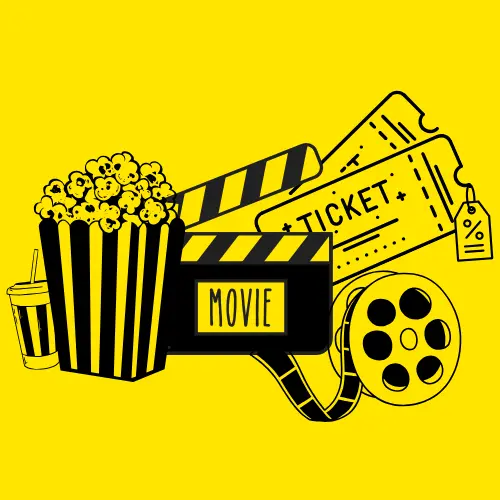
A cinema offers discounts based on age and whether the person is a student. This is how the discount scheme works:
Kids under 12 get a 50% discount.
Students aged 12-18 get a 30% discount.
Adults aged 18+ get no discount unless they are students, in which case they get a 10% discount.
Write a Python program that calculates the ticket price based on these rules. Assume the original ticket price is $20.
Test Case
Input:
Age= 16
Is Student?= yes
Expected Output:
Your ticket price is: $14.00
Explanation:
The program calculates the ticket price after applying a discount (if any) based on age and student status.
- In this program, the user is asked to input their age and whether they are a student and store them in
age
andis_student
respectively. - The base ticket price is set at $20.
- If the age is less than 12, the user receives a 50% discount on the ticket price.
- If the age is between 12 and 18 (exclusive), the discount depends on whether the user is a student:
- Then it checks if the user is a student, then they receive a 30% discount.
- Else, if the user is not a student, there is no discount.
- If the age is 18 or older, the discount is again based on student status:
- If the user is a student, they receive a 10% discount.
- If the user is not a student, there is no discount.
- The final price is calculated by multiplying the base price by the factor
(1 - discount)
.The(1 - discount)
calculates the portion of the base price to be paid after subtracting the discount percentage. - Lastly, the final ticket price is printed with the calculated discount.
age = int(input("Enter your age: ")) is_student = input("Are you a student? (yes/no): ") ticket_price = 20 #Base Price for ticket if age < 12: discount = 0.5 elif 12 <= age < 18: if is_student == "yes": discount = 0.3 else: discount = 0 else: if is_student == "yes": discount = 0.1 else: discount = 0 final_price = ticket_price * (1 - discount) print(f"Your ticket price is: ${final_price:.2f}")
Tax Calculation
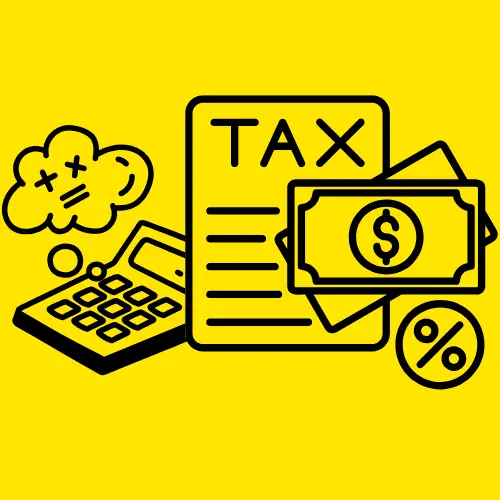
You work as a tax advisor for individuals, helping them calculate their annual income tax. The tax rules are simple: If a person earns less than $20,000 a year, they don’t pay any tax. For incomes between $20,000 and $50,000, a 5% tax is applied, but if they have children, they get a 2% discount on the tax. For those earning more than $50,000, a 10% tax is applied, but if they are married, they get a 3% discount.
To automate the work you are tasked to develop a program that calculates their exact tax based on their income, marital status, and whether they have children.
Test Case
Input:
Annual Income= 30000
Is Married= no
Children= 2
Expected Output:
Your annual tax is: $900.00
Explanation:
This program calculates the annual tax based on the user’s income, marital status, and number of children.
- For the program to begin, the user enters their annual income, marital status (yes/no), and the number of children.
- If the income is less than $20,000, then no tax is applied (
tax_rate = 0
). - For an income between $20,000 and $50,000, the base tax rate is 5% (
tax_rate = 0.05
).- If the user has one or more children, the tax rate is reduced by 2% (
tax_rate -= 0.02
).
- If the user has one or more children, the tax rate is reduced by 2% (
- For an income above $50,000, the base tax rate is 10% (
tax_rate = 0.1
).- If the user is married, the tax rate is reduced by 3% (
tax_rate -= 0.03
).
- If the user is married, the tax rate is reduced by 3% (
- Then the annual tax is calculated as
income * tax_rate
. - Finally, the calculated annual tax is displayed with two decimal places using
annual_tax:.2f
.
income = float(input("Enter your annual income: ")) is_married = input("Are you married? (yes/no): ") children = int(input("Enter the number of children: ")) tax_rate = 0 if income < 20000: tax_rate = 0 elif 20000 <= income <= 50000: tax_rate = 0.05 if children > 0: tax_rate -= 0.02 else: tax_rate = 0.1 if is_married == "yes": tax_rate -= 0.03 annual_tax = income * tax_rate print(f"Your annual tax is: ${annual_tax:.2f}")
Electricity Bill Calculation
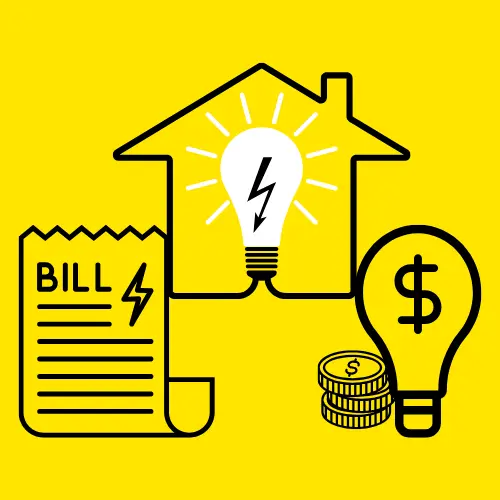
An electricity company charges based on usage with extra conditions for discounts or surcharges. For usage up to 100 units, the cost is $0.50 per unit, and senior citizens get a 10% discount. For usage between 101 and 200 units, the first 100 units cost $0.50 each, and the remaining units cost $0.75 each. If the customer is not a senior citizen and has late payments, a $10 surcharge is added. For usage above 200 units, the first 100 units cost $0.50 each, the next 100 units cost $0.75 each, and any additional units cost $1.00 each. Customers with no late payments or usage under 300 units get a $20 discount.
Automate the calculations by creating a Python script where the bill depends on usage, senior citizen status, and payment history.
Test Case
Input:
Electricity Usage= 250
Senior Citizen= no
Late Payments=no
Expected Output:
Total bill: $162.50
Explanation:
This program calculates the electricity bill based on the user’s usage, senior citizen status, and late payment history.
- The user inputs their electricity usage in units (
usage
), whether they are a senior citizen (senior_citizen
as “yes” or “no”), and if they have late payments (late_payment
as “yes” or “no”). - For
usage <= 100
, the bill is calculated asbill = usage * 0.5
, with a 10% discount applied for senior citizens (bill *= 0.9
). - For
usage
between 101 and 200, - the first 100 units are charged at
$0.50
per unit, additional units at$0.75
per unit, and if the user is not a senior citizen and has late payments, a$10
a surcharge is added (bill += 10
). - For
usage > 200
, the first 100 units are charged at$0.50
per unit, the next 100 at$0.75
per unit, and units above 200 at$1.00
per unit. - If there are no late payments or
usage < 300
, a$20
discount is applied (bill -= 20
). - Finally, the total bill is displayed, formatted to two decimal places (
{bill:.2f}
).
usage = int(input("Enter your electricity usage (in units): ")) senior_citizen = input("Are you a senior citizen? (yes/no): ") late_payments = input("Do you have late payments? (yes/no): ") bill = 0 if usage <= 100: bill = usage * 0.5 if senior_citizen == "yes": bill *= 0.9 # 10% discount else: if usage <= 200: bill = (100 * 0.5) + ((usage - 100) * 0.75) if senior_citizen != "yes" and late_payments == "yes": bill += 10 # $10 surcharge else: bill = (100 * 0.5) + (100 * 0.75) + ((usage - 200) * 1.0) if late_payments == "no" or usage < 300: bill -= 20 # $20 discount print(f"Total bill: ${bill:.2f}")
Robbery Escape Plan
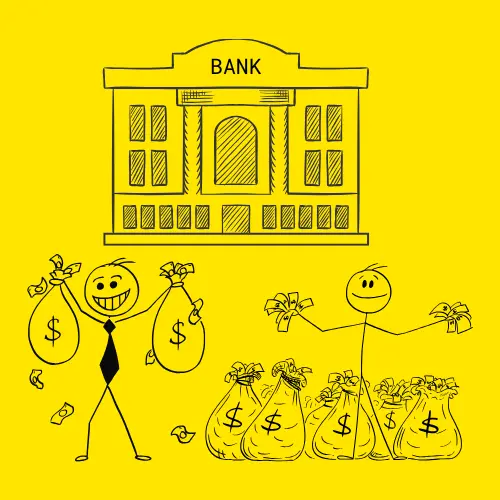
Two robbers plan their escape based on how much money they stole, their getaway vehicle, the time of day, and whether the police are nearby. If they stole more than $50,000 and have a car with no police nearby, they escape on the highway; otherwise, if they have a bike or the police are close, they hide in a nearby alley. If the amount is between $10,000 and $50,000, they escape through the main road at night when the police aren’t nearby; otherwise, they hide in an abandoned house. They blend into the crowd for less than $10,000 if they don’t have a vehicle; otherwise, they take a bus to another city.
Write a Python program to decide the robber’s next move based on the amount stolen, vehicle type, time of day, and police presence.
Test Case:
Input:
Amount Stolen= 55000
Getaway Vehicle= car
Nighttime= no
Police Nearby= no
Expected Output:
Next move: Escape on the highway.
Explanation:
- This program decides the “next move” based on four inputs: the amount stolen (
stolen_amount
), the type of getaway vehicle (getaway_vehicle
as “car,” “bike,” or “none”), whether it is nighttime (is_night
as “yes” or “no”), and whether the police are nearby (police_nearby
as “yes” or “no”). - For
stolen_amount > 50000
,- if the
getaway_vehicle
is “car” andpolice_nearby
is “no,” the suggestion is"Escape on the highway."
- Otherwise, it suggests
"Hide in a nearby alley."
- if the
- For
stolen_amount
between 10000 and 50000,- if
is_night
is “yes” andpolice_nearby
is “no,” the suggestion is"Escape through the main road."
- Otherwise, it recommends
"Hide in an abandoned house."
- if
- For
stolen_amount < 10000
,- if there is no
getaway_vehicle
, the program suggests"Blend in with the crowd."
- Otherwise, it recommends
"Take a bus to a different city."
Based on these conditions, the program outputs the most suitable “next move.”
- if there is no
amount_stolen = int(input("Enter the amount stolen: ")) vehicle = input("Enter the type of getaway vehicle (car/bike/none): ") nighttime = input("Is it nighttime? (yes/no): ") police_nearby = input("Are the police nearby? (yes/no): ") if amount_stolen > 50000: if vehicle == "car" and not police_nearby: print("Next move: Escape on the highway.") else: print("Next move: Hide in a nearby alley.") elif 10000 <= amount_stolen <= 50000: if not police_nearby and nighttime: print("Next move: Escape through the main road.") else: print("Next move: Hide in an abandoned house.") else: # amount_stolen < 10000 if vehicle == "none": print("Next move: Blend in with the crowd.") else: print("Next move: Take a bus to a different city.")
Avengers Mission Reward Points
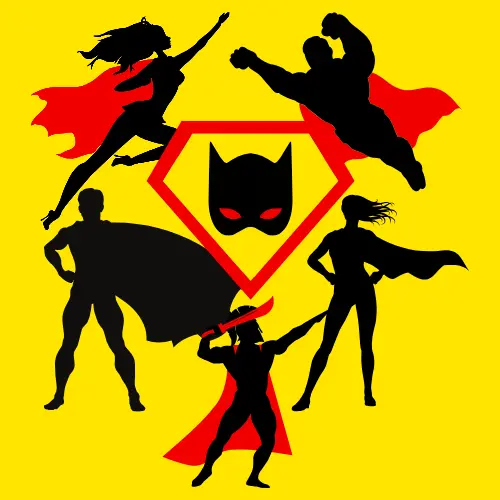
In the Avengers universe, the team members are assigned missions based on the type of threat and their experience level. Each member receives reward points for completing missions, and the points vary depending on the mission and their role. If the mission is “high-risk” and the Avenger has completed 3 or more successful missions, they get 50% more reward points. For “low-risk” missions, if the Avenger has fewer than 3 successful missions, they lose 20% of their points. A veteran Avenger gets a 200-point bonus for “high-risk” or “medium-risk” missions, while a rookie gets 100 points for a “low-risk” mission. If an Avenger has completed more than 5 missions, they earn a loyalty bonus of 150 points.
Based on these conditions, write a Python program to calculate the total reward points for an Avenger based on their mission type, experience, and past missions.
Test Case:
Input:
Mission Type= high-risk
Missions= 6
Hero Rank= veteran
Expected Output:
Reward points: 950
Explanation:
- This program calculates the total reward points for heroes based on the
mission_type
,successful_missions
, andhero_rank
. - It starts with a base reward of
500
points for all missions. - For
mission_type
, high-risk missions add a50%
bonus ifsuccessful_missions >= 3
; otherwise, no bonus is given. - For low-risk missions, if
successful_missions < 3
, the reward is reduced by20%
; otherwise, no changes are made. - Medium-risk missions do not alter the reward.
- The
hero_rank
also impacts the reward: veterans earn an extra200
points for high-risk or medium-risk missions, - while rookies get a flat
100
points for low-risk missions, regardless of other calculations. - Additionally, if
successful_missions > 5
, a loyalty bonus of150
points is added. - The program calculates and displays the total reward points based on these conditions.
mission_type = input("Enter the mission type (high-risk/medium-risk/low-risk): ") missions = int(input("Enter the number of successful missions: ")) hero_rank = input("Enter your hero rank (rookie/veteran): ") reward_points = 500 # Base reward for all missions if mission_type == "high-risk": if missions >= 3: reward_points *= 1.5 # 50% bonus else: reward_points *= 1 # No bonus for high-risk if less than 3 missions completed elif mission_type == "low-risk": if missions < 3: reward_points *= 0.8 # 20% penalty f else: reward_points *= 1 # No penalty else: reward_points *= 1 # No change for medium-risk or unknown mission types # Bonus for veteran heroes if hero_rank == "veteran": if mission_type in ["high-risk", "medium-risk"]: reward_points += 200 # Rookie bonus for low-risk missions if hero_rank == "rookie": if mission_type == "low-risk": reward_points = 100 # Flat reward if missions > 5: reward_points += 150 # Loyalty bonus print(f"Reward points: {reward_points}")
Time Traveler’s Machine Energy
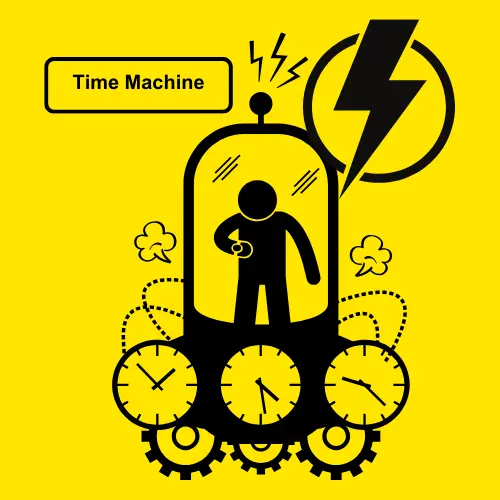
You are designing the power system for a time machine that adjusts energy based on the era selected and the travelers involved. If the era is “future” and there are 3 or more travelers, the machine uses 70% more power, and if they are scientists, it adds another 20%. For the “past” era with fewer than 4 travelers, it saves 40% power, and if they are historians, it saves an extra 10%. For the “distant past,” fewer than 3 travelers use 50% less power, but carrying artifacts increases power by 10%. If more than 5 people travel, the power goes up 30%, and if VIPs are included, it adds 15%.
Develop a Python program to calculate the power consumption based on the era, the number of travelers, and their roles. Can your program handle all these conditions and figure out how much power the machine needs?
Test Case
Input:
Era= future
Travelers= 6
Role= VIPs
Expected Output:
Power consumption: 115% more
Explanation:
This program calculates the power consumption for time travel based on three factors: the era you’re traveling to, the number of travelers, and their role.
- First, the program asks user to input the
era
, the number oftravelers
, and theirrole
. - The base power usage is set to
100
, which is the starting point for the calculation. - Next, the program checks the
era
you choose:- If the
era
isfuture
, and there are3
or more travelers, it increases power usage by70%
(power_usage *= 1.7
).- After that, if the travelers are
scientists
, it adds an additional20%
increase (power_usage *= 1.2
), as scientists need more energy in the future for their research.
- After that, if the travelers are
- If the
era
ispast
, and there are fewer than4
travelers, the power usage is decreased by40%
(power_usage *= 0.6
), making it easier to travel to the past with fewer people.- If the travelers are
historians
, an additional10%
reduction is applied (power_usage *= 0.9
), as historians need less power.
- If the travelers are
- If the
era
isdistant past
, and there are fewer than3
travelers, the power usage is reduced by50%
(power_usage *= 0.5
).- The program also checks if the role is
artifacts
, if valid, it adds a10%
increase for carrying artifacts (power_usage *= 1.1
).
- The program also checks if the role is
- If the
- After that, the program checks if there are more than
5
travelers.- If yes, it adds
30%
more power usage (power_usage *= 1.3
), as more people require more energy. - Then, if the role of the travelers is
vips
, an additional15%
increase is applied to the power usage (power_usage *= 1.15
), because VIPs require extra resources for their luxury.
- If yes, it adds
- Finally, the program calculates the percentage change in power usage by subtracting the initial value (
100
) from the updated value of power usage. This percentage shows how much more or less power is needed for the trip. - The result is printed, showing how much more or less power is consumed, formatted to two decimal places.
era = input("Enter the era (future/past/distant past): ") travelers = int(input("Enter the number of travelers: ")) role = input("Are the travelers scientists/historians/vips/none? ") power_usage = 100 # Base power usage if era == "future": if travelers >= 3: power_usage *= 1.7 # 70% more for future with 3+ travelers if role == "scientists": power_usage *= 1.2 # Additional 20% for scientists elif era == "past": if travelers < 4: power_usage *= 0.6 # 40% less for past with fewer than 4 travelers if role == "historians": power_usage *= 0.9 # Additional 10% decrease for historians elif era == "distant past": if travelers < 3: power_usage *= 0.5 # 50% less for distant past with fewer than 3 travelers if role == "artifacts": power_usage *= 1.1 # Additional 10% for carrying artifacts if travelers > 5: power_usage *= 1.3 # 30% more for more than 5 travelers if role == "vips": power_usage *= 1.15 # Additional 15% for VIPs percentage_change = power_usage - 100 print(f"Power consumption: {percentage_change:.2f}% more")
Laptop Purchase Assistance
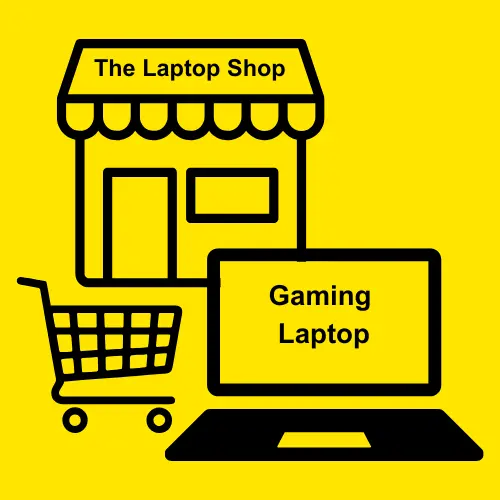
You are creating a program to help customers choose a laptop based on their budget, how they plan to use it, and if they need accessories. If the budget is over $1500, suggest a High-Performance Laptop for $1400 if the usage is “gaming” or “video editing,” or a Business Laptop for $1200 if the usage is “office work.” If the budget is between $800 and $1500, suggest a Budget Gaming Laptop for $1000 for “gaming” or a Mid-Range Office Laptop for $900 for “office work.” If the budget is below $800, suggest a Basic Laptop for $600, and if they need accessories, add $50 to the price. The program should show the laptop choice, total price, and whether it stays within the budget.
Test Case
Input:
Budget= 1000
Usage= gaming
Accessories= no
Expected Output:
Suggested Laptop: Budget Gaming Laptop
Price: $1000
Within Budget: Yes
Explanation:
- The program begins by asking the user to enter their
budget
(the amount of money they can spend), theprimary usage
of the laptop (such as gaming, office work, or video editing), and whether they needaccessories
like a mouse or keyboard. - The program first checks if the
budget
is greater than$1500
. f it is, it further checks theusage
:- if the usage is
gaming
orvideo editing
, the program suggests aHigh-Performance Laptop
priced at$1400
(usinglaptop = "High-Performance Laptop"
andprice = 1400
). - If the usage is
office work
, it suggests aBusiness Laptop
priced at$1200
(usinglaptop = "Business Laptop"
andprice = 1200
).
- if the usage is
- If the
budget
is between$800
and$1500
(usingelif 800 <= budget <= 1500
), the program then checks the usage again.- If the usage is
gaming
, it suggests aBudget Gaming Laptop
priced at$1000
(usinglaptop = "Budget Gaming Laptop"
andprice = 1000
). - If the usage is
office work
, it suggests aMid-Range Office Laptop
priced at$900
(usinglaptop = "Mid-Range Office Laptop"
andprice = 900
).
- If the usage is
- If the
budget
is less than$800
(usingelse
), the program suggests aBasic Laptop
priced at$600
(usinglaptop = "Basic Laptop"
andprice = 600
).- If the user wants
accessories
(withaccessories == "yes"
), it adds$50
to the price (usingprice += 50
).
- If the user wants
- After determining the
price
of the laptop, the program checks if the price iswithin the budget
.- If the laptop’s price is less than or equal to the user’s budget (using
within_budget = "Yes" if price <= budget else "No"
), it setswithin_budget
to"Yes"
. - If the price exceeds the budget,it is set to
"No"
.
- If the laptop’s price is less than or equal to the user’s budget (using
- Finally, the program prints the
suggested laptop
, itsprice
, and whether it iswithin the budget
, providing the user with all the necessary details to decide on their purchase.
budget = int(input("Enter your budget: ")) usage = input("Primary usage (gaming/office work/video editing): ") accessories = input("Do you need accessories? (yes/no): ") if budget > 1500: if usage in ["gaming", "video editing"]: laptop = "High-Performance Laptop" price = 1400 elif usage == "office work": laptop = "Business Laptop" price = 1200 elif 800 <= budget <= 1500: if usage == "gaming": laptop = "Budget Gaming Laptop" price = 1000 elif usage == "office work": laptop = "Mid-Range Office Laptop" price = 900 else: laptop = "Basic Laptop" price = 600 if accessories == "yes": price += 50 # Check if it fits the budget within_budget = "Yes" if price <= budget else "No" print(f"Suggested Laptop: {laptop}") print(f"Price: ${price}") print(f"Within Budget: {within_budget}")
Fruit Selection
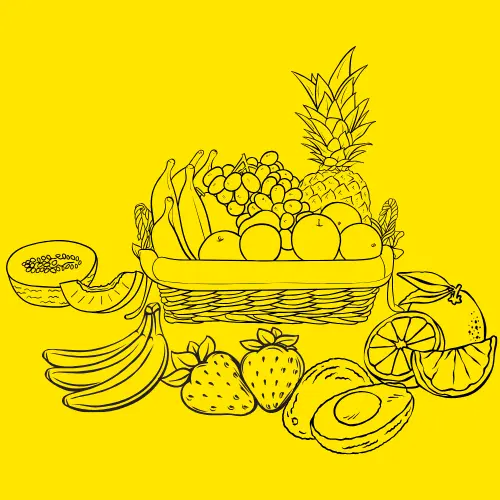
Suppose you are making a program to recommend a fruit based on the customer’s taste (sweet or sour), texture (soft or crunchy), and if they want a low-calorie option. If they like sweet fruits: suggest Watermelon (30 calories per cup) if it’s soft and low-calorie, or Mango (100 calories per cup) if it’s soft but not low-calorie, and Apple (52 calories per medium apple) if it’s crunchy. If they like sour fruits: suggest Kiwi (42 calories per medium kiwi) if it’s soft, Green Apple (50 calories per medium apple) if it’s crunchy and low-calorie, or Pineapple (82 calories per cup) if it’s crunchy but not low-calorie.
The program will show the fruit suggestion and its calories.
Test Case
Input:
Taste= sweet
Texture= soft
low-calorie= yes
Expected Output:
Recommended Fruit: Watermelon
Calories: 30
Explanation:
The program provides a fruit recommendation based on the user’s taste, texture, and calorie preference.
- The program begins by asking for the user’s taste preference (
taste = input("Enter your taste preference (sweet/sour): ")
), texture preference (texture = input("Enter your texture preference (soft/crunchy): ")
), and whether they are looking for low-calorie options (low_calorie = input("Are you looking for low-calorie options? (yes/no): ")
). - The program then checks if the taste is “sweet” (
if taste == "sweet":
). - If it is, the program checks the texture preference (
if texture == "soft":
).- If the texture is soft, it checks if the user wants low-calorie options (
if low_calorie == "yes":
).- If the answer is “yes”, the program suggests Watermelon with 30 calories.
- If the answer is “no”, it suggests Mango with 100 calories.
- If the texture is crunchy, the program suggests Apple with 52 calories.
- If the texture is soft, it checks if the user wants low-calorie options (
- If the taste is “sour” (
elif taste == "sour":
), the program again checks the texture.- If the texture is soft, it suggests Kiwi with 42 calories.
- If the texture is crunchy, the program checks if the user is looking for low-calorie options again.
- If “yes”, it recommends Green Apple with 50 calories.
- If “no”, it suggests Pineapple with 82 calories.
- Finally, the program prints the recommended fruit and the calories of the selected fruit.
taste = input("Enter your taste preference (sweet/sour): ") texture = input("Enter your texture preference (soft/crunchy): ") low_calorie = input("Are you looking for low-calorie options? (yes/no): ") if taste == "sweet": if texture == "soft": if low_calorie == "yes": fruit = "Watermelon" calories = 30 else: fruit = "Mango" calories = 100 elif texture == "crunchy": fruit = "Apple" calories = 52 elif taste == "sour": if texture == "soft": fruit = "Kiwi" calories = 42 elif texture == "crunchy": if low_calorie == "yes": fruit = "Green Apple" calories = 50 else: fruit = "Pineapple" calories = 82 print(f"Recommended Fruit: {fruit}") print(f"Calories: {calories}")
In conclusion, nested if-else statements in Python are a useful tool for making decisions based on multiple conditions. These statements allow you to handle complex situations, like suggesting playlists based on mood, planning finances, or calculating energy usage. By getting better at using nested if-else, you can make your programs more flexible and smart. The more you practice with different examples, the better you’ll understand how to use this tool to solve problems in your code. For more helpful coding tips, be sure to check out Syntax Scenarios.