Every day, we make decisions based on different situations. For example, if it’s raining, we grab an umbrella; if it’s sunny, we may leave it at home. In programming, we use if-else statements to make similar decisions in our code. These statements help us decide what to do based on certain conditions. To help you practice, Syntax Scenarios has designed scenario-based questions that will guide you in applying if-else statements to solve real-world problems. It’s time to see how you can make decisions in Python!
Note: You can view the answer code and its explanation by clicking on the question (or the drop-down icon).
Check If You Are Eligible to Vote
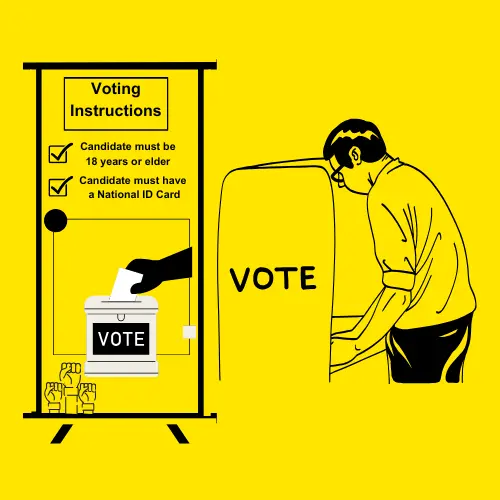
You work at the Election Commission office, and you’ve been asked to create a simple Python program to check if someone is eligible to vote. To vote, a person must be at least 18 years old and have a National ID card. Your task is to write a program that checks whether both conditions are met.
Test Case:
Input:
Age = 20
Has Id = yes
Expected Output:
You qualify to vote.
Explanation:
- The program asks the user to input their age and checks if it’s at least 18 years old.
- It then asks if the user has a National ID card, expecting the response to be either “yes” or “no”.
- The program checks two conditions:
1. whether the user is 18 or older
2. and whether the user has a National ID card. - The program will show “You qualify to vote” if both conditions are met.
- The program will display “You can not vote” if either condition is false.
age = int(input("Enter your age: ")) has_id = input("Do you have a National ID card? (yes/no): ").lower() if age >= 18 and has_id == "yes": print("You qualify to vote.") else: print("You can not vote.")
Even or Odd Number of Players
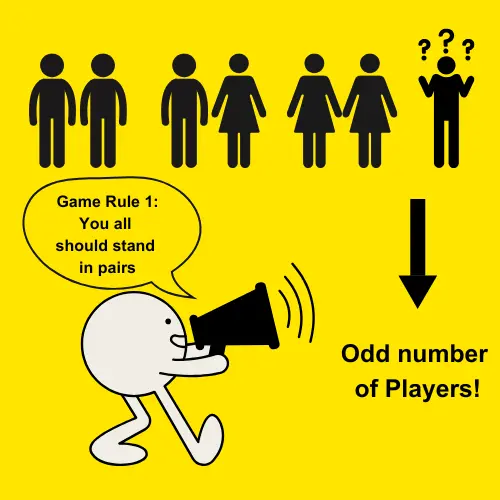
Imagine you’re in charge of organizing a game where players have to stand in pairs. To make sure the game works, you need to check if the number of players is even or odd. Everyone gets a partner if the number is even, but if it’s odd, one person will be left without a partner.
Develop a Python code for the scenario above.
Test Case:
Input:
Number = 7
Expected Output:
Game can not proceed as there is odd number of players.
Explanation:
- The program prompts the user to input the number of players.
- It checks if the number of players is divisible by 2 using the modulo operator (
%
). - If the number of players is divisible by 2 (i.e., it’s even), the program prints “Game can proceed as there is even number of players.”
- Otherwise, if the number is not divisible by 2 (i.e., it’s odd), the program prints “Game can not proceed as there is odd number of players.”
number = int(input("Enter the number of players: ")) if number % 2 == 0: print("Game can proceed as there is even number of players.") else: print("Game can not proceed as there is odd number of players.")
Verify if a Year is a Leap Year
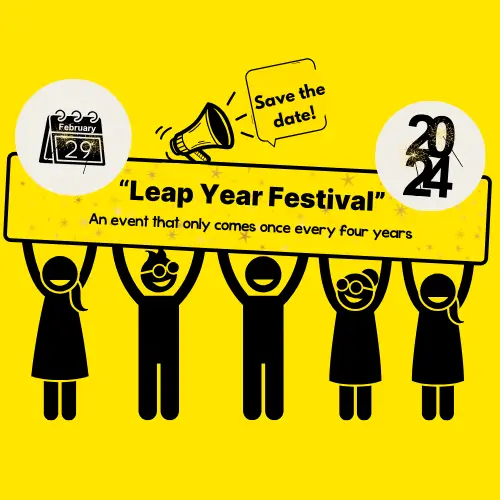
Imagine you’re organizing the “Leap Year Festival,” a fun event that only happens on February 29th during a leap year. To plan the festival, you need to find out if a year has this extra day in February. A year is considered a leap year if it is divisible by 4, except when it is divisible by 100, unless it is also divisible by 400. To make sure your plans are correct, you need to write a Python program to check if a year is a leap year.
Test Case:
Input:
Leap Check Year = 2024
Expected Output:
2024 is a leap year.
Explanation:
- The program starts by asking the user to input a year and converts it into a number using
int()
. - To check whether a year is a leap year:
- The year should be divisible by 4.
- If it’s divisible by 100, it also needs to be divisible by 400 to qualify.
- If the year satisfies these rules, it’s considered a leap year, and the program outputs the result.
- Otherwise, it states that the year is not a leap year.
leap_check_year = int(input("Enter a year to check: ")) if (leap_check_year % 4 == 0 and leap_check_year % 100 != 0) or (leap_check_year % 400 == 0): print(f"{leap_check_year} qualifies as a leap year.") else: print(f"{leap_check_year} does not qualify as a leap year.")
Alarm for the Weekend
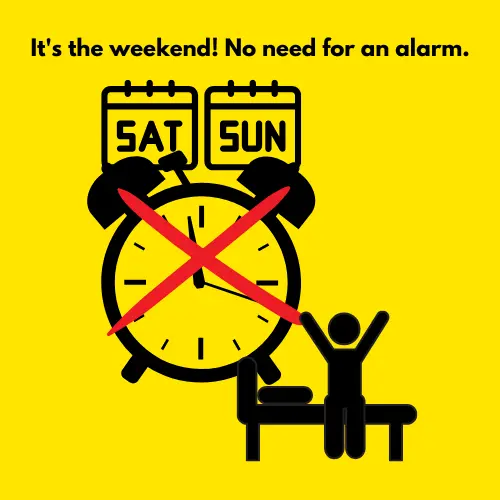
Imagine you have a regular alarm to wake up for school, but you don’t need it on weekends. You wish to determine whether today is a weekend or a weekday to decide whether you should set the alarm.If it’s the weekend, you get to sleep longer. If it’s a weekday, you should set the alarm to wake up early.
Write a Python program to check if you need to set an alarm or not.
Test Case:
Input:
Day = Saturday
Expected Output:
It’s the weekend! No need for an alarm.
Explanation:
- The program starts by asking the user to enter the day of the week.
- It then checks if the entered day is “Saturday” or “Sunday” using the
if
condition. - If the day is either Saturday or Sunday, the program tells you it’s the weekend, so no alarm is needed.
- If the day is not Saturday or Sunday (i.e., it’s a weekday), the program reminds you to set the alarm to wake up early.
day = input("Enter the day: ").lower() if day == "saturday" or day == "sunday": print("It's the weekend! No need for an alarm.") else: print("It's a weekday. Set the alarm!")
Checking GPA to Determine Graduation Status
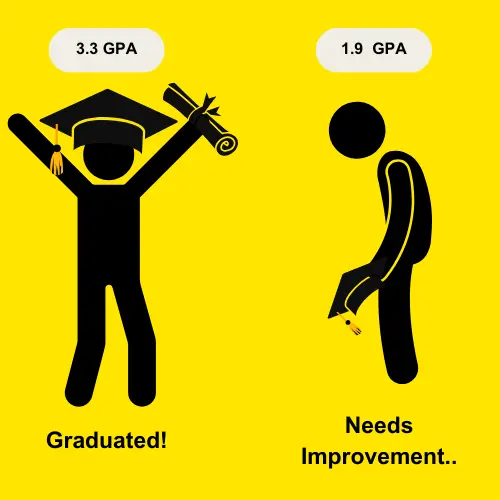
Suppose you’re a student and you need to check if your GPA qualifies you for graduation. To graduate, you must have a GPA of 2.0 or higher. You want to write a program that checks your GPA and tells you whether you can graduate or if you need to improve your grades.
Write a Python program that checks if the GPA entered is greater than or equal to 2.0. If it is, the program will tell you that you can graduate. If the GPA is below 2.0, the program will remind you to improve your grades.
Test Case:
Input:
GPA = 1.9
Expected Output:
You need to improve your GPA to graduate.
Explanation:
- The program begins by asking the user to enter their GPA.
- It checks if the GPA is 2.0 or greater using an if statement.
- If the GPA is 2.0 or more, it prints “You can graduate!”.
- If the GPA is below 2.0, it prints “Your GPA needs to be higher for graduation.”
gpa = float(input("Enter your GPA: ")) if gpa >= 2.0: print("You can graduate!") else: print("Your GPA needs to be higher for graduation.")
Are Your Savings Sufficient To Buy A Phone?
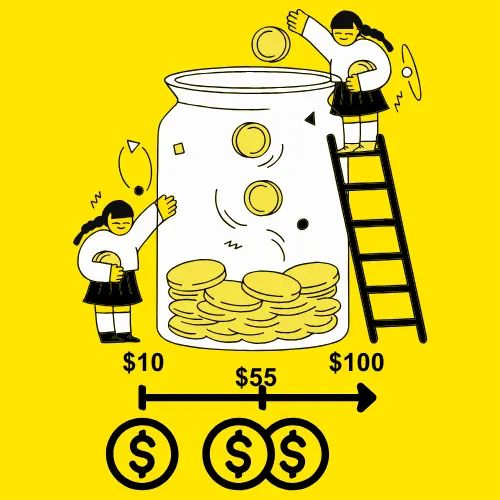
You’re saving money to buy a new phone, and you’ve set a target amount between $10 and $100. You want to check if your current savings are enough to meet this target range. If your savings fall within this range, you’re on track to buy the phone. If not, you’ll need to save more or adjust your goal.
Write a Python program that checks if your savings (the number you enter) fall between $10 and $100. The program should let you know if you’re on track or need to save more.
Test Case:
Input:
Savings = 50
Expected Output:
Your savings are within the target range.
Explanation:
- The program starts by asking the user to input their savings amount (how much money they’ve saved).
- It then checks if the savings are between $10 and $100 (inclusive).
- If the savings are within the range, it prints: “Your savings are within the target range.”
- If the savings are not within this range, it prints: “Your savings are out of the target range.”
savings = int(input("Enter your savings amount: ")) if 10 <= savings <= 100: print("Your savings are within the target range.") else: print("Your savings are out of the target range.")
Divisible by 2 & 3 in a Group Assignment
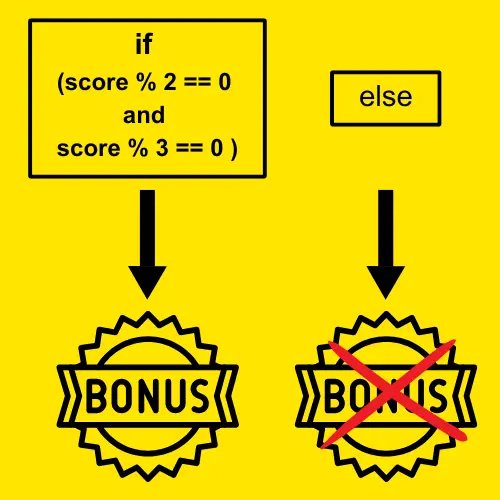
You are working on a group assignment, and your teacher says that each group member must contribute a score that meets several criteria to qualify for extra credit. These criteria are:
The score must be divisible by both 2 and 3.
The score must be at least 5.
The score must not be equal to 9.
If the score is prime, it still needs to be divisible by both 2 and 3.
Write a Python program to check if the score qualifies based on these conditions.
Test Case:
Input: Score = 6
Expected Output:
Divisible by 2 and 3, meets other conditions
Explanation:
- The program asks the user to input their score.
- It then checks the following conditions:
1. Is the score divisible by both 2 and 3?
2. Is the score at least 5?
3. Is the score not equal to 9? - If the score meets all of these conditions, it tells the user that they are eligible for extra credit.
- If any of the conditions is not met, it encourages the user to keep working on improving their score.
score = int(input("Enter your score: ")) if score % 2 == 0 and score % 3 == 0 and score >= 5 and score != 9: print("You're eligible for extra credit!") else: print("You are not eligible for extra credit. Keep working on improving your score!")
Eligibility for 20% Discount
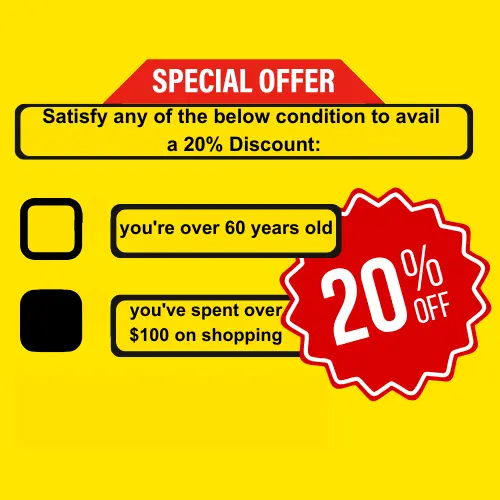
You’re going shopping for clothes and want to know if you qualify for a special discount. There are two ways to get a 20% discount:
If you’re over 60 years old.
If you’ve spent over $100 on your shopping, you qualify for a “big spender” 20% discount.
You want to check if you’re eligible for either of these discounts before paying through a Python program.
Test Case:
Input:
Age = 65
Purchase = 80
Expected Output:
You are eligible for a 20% discount.
Explanation:
- The program asks for the age and the amount spent.
- It checks if the age is over 60 or if the amount spent is more than $100.
- If either condition is true, a 20% discount is given and displays “You are eligible for a 20% discount.”
- Otherwise, it will say: “You are not eligible for a discount.”
age = int(input("Enter your age: ")) purchase = float(input("Enter your purchase amount: ")) if age > 60 or purchase > 100: print("You are eligible for a 20% discount.") else: print("You are not eligible for a discount.")
Securing Your Online Accounts
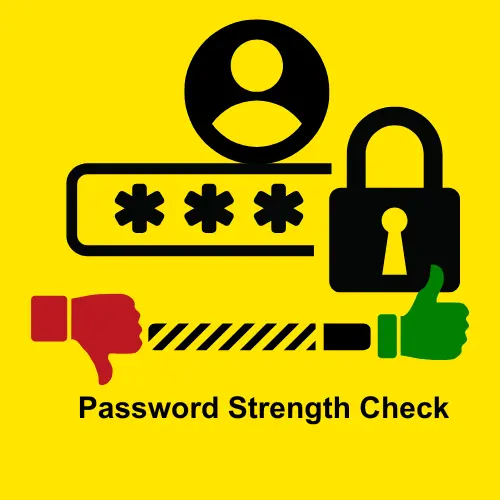
You are creating a new account and want to set a strong password to keep it secure. For the password to be strong, it must follow these simple rules:
It should be at least 8 characters long.
The first character must be an uppercase letter.
The last character must be a lowercase letter.
If your password meets all these rules, it will be accepted as “Strong.” Otherwise, you’ll need to create a better one.
Write a Python program to check if a password is strong based on these rules.
Test Case:
Input:
Password = Securepass
Expected Output:
Strong password! Your account is secure.
Explanation:
- The program prompts the user to create a password.
- It checks three conditions:
1. The password should have at least 8 characters.
2. The first letter must be an uppercase letter.
3. The last letter must be a lowercase letter. - If the password satisfies these rules, it displays: “Strong password! Your account is secure.”
- Otherwise, it shows:“Weak password! Follow the rules to improve it.”
password = input("Create your password: ") has_min_length = len(password) >= 8 # At least 8 characters long starts_with_uppercase = password[0].isupper() # First character is uppercase ends_with_lowercase = password[-1].islower() # Last character is lowercase if has_min_length and starts_with_uppercase and ends_with_lowercase: print("Strong password! Your account is secure.") else: print("Weak password! Ensure it is at least 8 characters long, starts with an uppercase letter, and ends with a lowercase letter.")
Triangle Validity Check
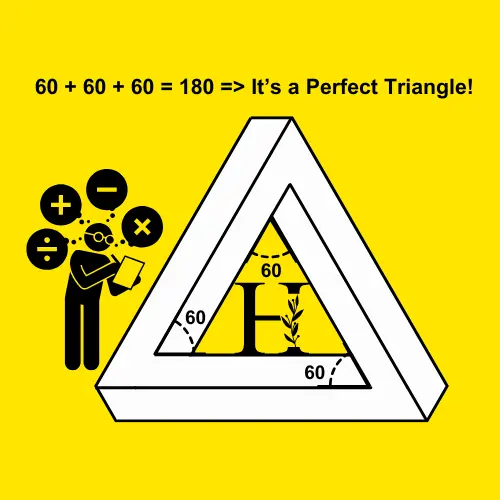
Imagine you’re helping a friend design a triangular signboard for their store. To make sure the angles form a proper triangle, you need to check two things:
Each angle should be a positive number (greater than 0).
The sum of all three angles must be exactly 180 degrees.
Write a Python program to check if the angles you enter can form a valid triangle.
Test Case:
Input:
Angle1 = 60
Angle2 = 70
Angle3 = 50
Expected Output:
The angles form a triangle.
Explanation:
- The program asks you to enter three angles.
- It adds the three angles together.
- Then, it checks if the total is exactly 180 degrees.
- Also, it checks if each angle is greater than 0 (positive).
- If both conditions are true, it tells you that the angles can form a triangle.
- If either condition is false, it tells you that the angles cannot form a triangle.
angle1 = int(input("Enter first angle: ")) angle2 = int(input("Enter second angle: ")) angle3 = int(input("Enter third angle: ")) if angle1 > 0 and angle2 > 0 and angle3 > 0 and angle1 + angle2 + angle3 == 180: print("The angles form a triangle.") else: print("The angles do not form a triangle.")
Eligibility for Driving License
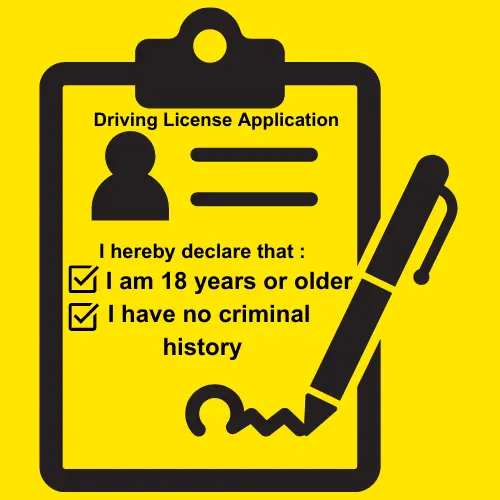
You’re helping a friend check if they can apply for a driving license. To qualify, the following must be true:
They must be at least 18 years old.
They must not have a criminal record.
They must pass a vision test with a score of 6 or above.
They must have completed at least 20 hours of driving practice.
However, if they are 21 or older, they do not need to meet the vision test requirement. Similarly, if they have completed more than 50 hours of driving practice, they are considered eligible regardless of the other conditions (except for age).
Test Case:
Input:
Age = 22
Criminal Record = Yes
Vision Test Score = 4
Driving Practice Hours = 55
Expected Output:
You are eligible for a driving license.
Explanation:
- The program asks for the following inputs:
1. Age
2. Criminal record (Yes/No)
3. Vision test score (0-10)
4. Total driving practice hours - It checks the following conditions:
1. The age is 18 or older, and there is no criminal record.
2. The vision test score is 6 or higher, or the age is 21 or older.
3. The user has at least 20 hours of driving practice. - If the user has more than 50 driving practice hours, they are automatically eligible.
- If all conditions are true, the program will print “You are eligible for a driving license.”
- If any condition is false, it will print “You are not eligible for a driving license.”
age = int(input("Enter your age: ")) criminal_record = input("Do you have a criminal record? (Yes/No): ").lower() vision_test_score = int(input("Enter your vision test score (0-10): ")) driving_practice_hours = int(input("Enter your total driving practice hours: ")) if (age >= 18 and not criminal_record == "yes" and (vision_test_score >= 6 or age >= 21) and driving_practice_hours >= 20) or driving_practice_hours > 50: print("You are eligible for a driving license.") else: print("You are not eligible for a driving license.")
Valid Login
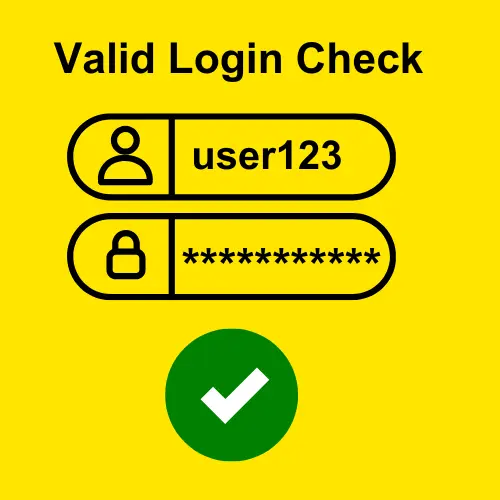
Suppose you’re trying to log in to a website, and the website has a special username and password that you need to type in to get in. The website’s username is always “user123” and the password is “password123”. If you type them exactly right, you’ll be allowed in. If you type anything differently, you won’t be able to log in.
Develop a Python program to implement this scenario.
Test Case:
Input:
Input Username = user111
Input Password = password321
Expected Output:
Invalid username or password.
Explanation:
- The program has a special username (“user123”) and password (“password123”).
- It asks you to enter your username and password.
- It checks if the username and password you entered match the special ones stored in the program.
- If both match, the program says “Login successful.”
- If either one is wrong, the program says “Invalid username or password.”
#values for username and password username = "user123" password = "password123" input_username = input("Enter your username: ") input_password = input("Enter your password: ") if input_username == username and input_password == password: print("Login successful.") else: print("Invalid username or password.")
Blood Donation Eligibility
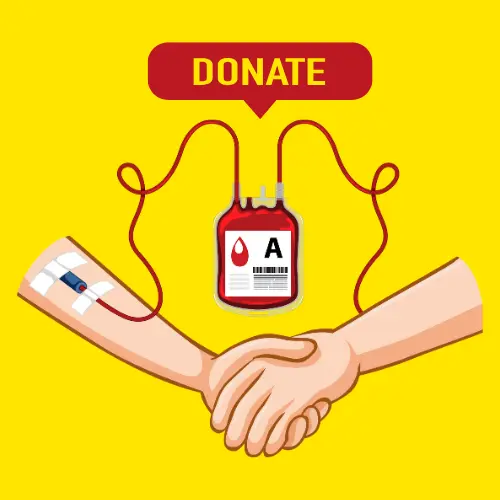
You are organizing a blood donation event and need to check if the donor is eligible. In addition to checking for certain medical conditions, we have additional criteria:
The person must not have any of these conditions: HIV, Hepatitis, or Malaria.
They must also be 18 years or older to donate.
They must not have a history of recent surgeries (within the last 6 months).
If they are on any medications, they must confirm whether those medications are approved by the donation center.
If any of these conditions are violated, the system will say “Not eligible.” If all the conditions are met, the system will say “Eligible to donate.”
Test Case:
Input:
Medical condition = Hepatitis
Age = 25
Recent surgery = No
Medications = No
Expected Output:
Not eligible
Explanation:
- The program first asks for the user’s medical condition.
- It checks if the condition is HIV, Hepatitis, or Malaria.
- Then, it checks the user’s age to ensure they are 18 or older.
- The program also checks if the user has had any recent surgery (within the last 6 months).
- Additionally, it checks if the user is currently taking any medications, ensuring they are approved for donation.
- If any condition is not met (i.e., they have one of the restricted conditions, are under 18, have had recent surgery, or are on unapproved medications), it prints “Not eligible.”
- If all conditions are met, it prints “Eligible to donate.”
medical_condition = input("Enter your medical condition (HIV, Hepatitis, Malaria, None): ").lower() age = int(input("Enter your age: ")) recent_surgery = input("Have you had any surgery in the last 6 months? (Yes/No): ").lower() medications = input("Are you currently taking any medications? (Yes/No): ").lower() if (medical_condition != "hiv" and medical_condition != "hepatitis" and medical_condition != "malaria") and \ (age >= 18) and \ (recent_surgery != "yes") and \ (medications != "yes" or medications == "no"): print("Eligible to donate") else: print("Not eligible")
Check if a Package Can be Delivered
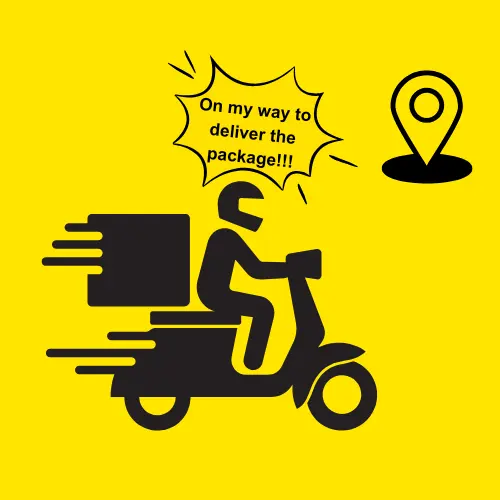
Imagine you work for a delivery company, and your task is to check if a package can be sent to a customer. There are two simple rules for deliveries:
The delivery location must be within 100 miles of the warehouse.
The package should weigh no more than 50 pounds.
If both rules are followed, the package can be delivered. But if even one rule is broken, the package cannot be delivered. Write a Python Program to check whether a package can be delivered or not.
Test Case:
Input:
Distance = 90 miles
Weight = 40 pounds
Expected Output:
The Package can be delivered
Explanation:
- First, the program asks for the delivery distance and the weight of the package.
- It then checks if the distance is 100 miles or less.
- Next, it checks if the package weight is 50 pounds or less.
- If both checks are true, it says “The Package can be delivered.”
- If either check fails, it says “The Package cannot be delivered.”
distance = int(input("Enter the distance (in miles): ")) weight = int(input("Input the package weight (in pounds): ")) if distance <= 100 and weight <= 50: print("The Package can be delivered") else: print("The package cannot be delivered.") }
Is the Temperature Suitable for Growing a Plant?
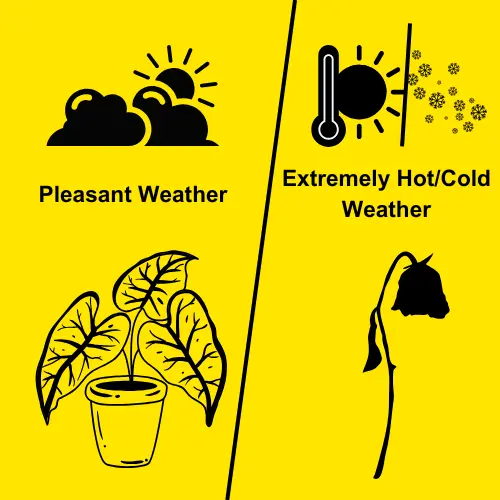
You want to grow a plant, but before you start, you need to check if the temperature is right for it.
If the temperature is too hot (above 35°C) or too cold (below 0°C), the plant cannot grow.
The plant will grow at a suitable temperature.
Write a Python program that checks if the current temperature is suitable for growing the plant.
Test Case:
Input:
Temperature = 40°C
Expected Output:
The temperature is not suitable for the plant to grow.
Explanation:
- The program asks the user to input the temperature in Celsius.
- It then checks two conditions:
If the temperature is greater than 35°C (too hot) or less than 0°C (too cold). - If either condition is true, it prints: “The temperature is not suitable for the plant to grow.”
- If both conditions are false (temperature is between 0°C and 35°C), it prints: “Plant can grow in this temperature.”
temperature = int(input("Enter the temperature in Celsius: ")) if temperature > 35 or temperature < 0: print("The temperature is not suitable for the plant to grow.") else: print("Plant can grow in this temperature.")
At SyntaxScenarios, we make learning Python fun with real-life examples. By solving these if-else problems, you’ve learned how to think logically and use operators to make decisions in your code. Keep practicing, and you’ll soon master Python!”