Learn how to truncate a string in Python using different techniques such as string slicing, shorten()
, rsplit()
, regular expressions and manually.
- What is Truncate?
- How To Truncate a String in Python?
- Scenarios Where You Need to Truncate Strings
- Conclusion
- FAQs
- Why would you want to truncate a string in Python?
- What is the simplest way to truncate a string in Python?
- How does the textwrap module help in truncating strings?
- Can I truncate a string based on a specific character or substring?
- What are regular expressions, and how can they be used for truncating strings?
- Is it possible to truncate a string character by character using a loop?
What is Truncate?
Truncate means to cut off part of a string to make it shorter. It’s useful when you want to store or display only a portion of a string. Imagine you have a small table that’s 20 inches long, but you buy a tablecloth that’s 50 inches. The tablecloth won’t fit properly until you cut it down. To make the cloth fit on the table, you would need to cut off 30 inches from the original cloth. That’s a real worls analogy of truncation.
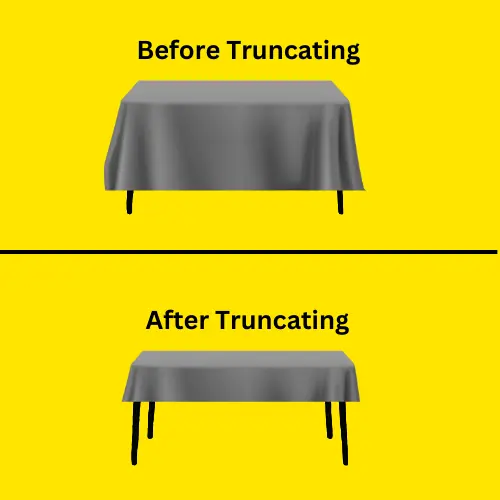
Same is the case with strings in Python. We use truncate to trim a part of the string to store it or print it. Truncation is commonly used in everyday programming, especially when we need to shorten a long string.
How To Truncate a String in Python?
In Python, there are multiple methods to truncate a string. We will be discussing all the possible ways to truncate a string in python using code, real life examples and visual analogies.
Use String Slicing
In Python, the simplest way to trim a string is to use string slicing. The slicing method allows you to cut a specific portion of a string based on its index. In Python, string indices start at 0. The first character of a string is at index 0, the second character is at index 1, and so on.
Imagine you’ve cooked a pizza, but you want to save some for your friend who is not available at the moment. You cut the pizza into slices and store a slice of specific size in the refrigerator so that your friend can eat it later.
Same is the case with string slicing. We select a specific part of the string and cut it. Then we can either store that part of the string for later use or print it.
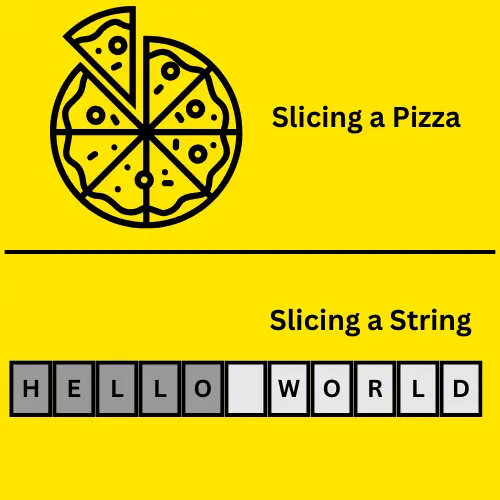
To use string slicing, we write the starting index of the portion of the string we want to extract, followed by the ending index, enclosed in square brackets ‘[]
’after the string name. We can store the extracted string in a variable or can print it directly.
If we leave the starting index empty, the first index of the string (which is the zero index) will be selected by default. Similarly, If we leave the ending index empty, the last index of the string will be selected by default.
Code
my_string = "Syntax Scenarios is an amazing website for learning fundamental programming concepts" sliced_string = my_string[23:38] print(sliced_string)
Output
amazing website
Trim a String Using textwrap Module
The textwrap
is a built-in module in Python which is used to format and manipulate strings. This module contains a function called ‘shorten()
’ used to truncate a string. This function trims the string at index which is provided by the user. Keep in mind that it always starts from the zero index. We only provide the maximum width(which is the ending index) of the string to the function.
Imagine you have a long wooden ladder. If you want to shorten it, you can cut it after a specific number of steps.
Same is the case with the shorten()
function. Just as you select the last step of the ladder at which you want to cut, you select a width of the substring and pass it to the shorten()
function. The shorten()
function trims the string at that width and returns the substring by placing a placeholder at the end of the substring to indicate that it has been truncated. The default placeholder is three dots ‘…
’ but it can be changed by passing placeholder of your choice to the function.
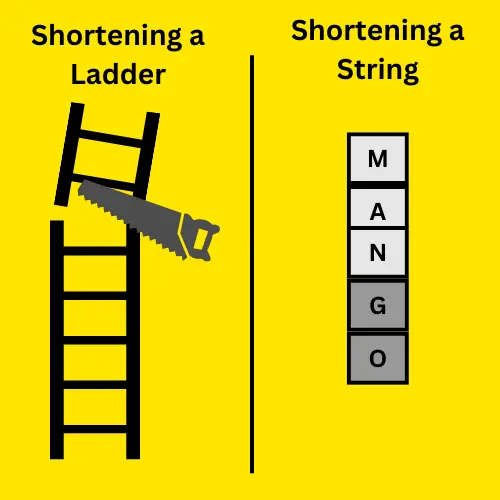
To use the shorten()
method, first you need to import the textwrap
module. Once the module is imported, you can use the shorten()
method by placing a dot operator ‘.
’ after the module. The shorten()
method takes three parameters. The first parameter is the string that you want to truncate. The second parameter is the width at which you want to trim the string and the third parameter is the place holder that will be placed at the end of returned substring. If you don’t pass the placeholder to the function, it will select three dots ‘…
’ as a placeholder by default.
Code
import textwrap my_string = "Syntax Scenarios is an amazing website for learning fundamental programming concepts" truncated_string = textwrap.shorten(my_string, width=38,placeholder="") print(truncated_string)
Output
Syntax Scenarios is an amazing website
Use rsplit() Method
The rsplit()
function is primarily used to split a string into multiple substrings whenever a specific character or substring is found in the string , but it can be also used to cut off a string. You can truncate a string by passing a character or a substring to the rsplit()
method. The rsplit()
function will cut the string whenever that character or substring is found and will return a list containing all the extracted substrings. You can then access the desired substring by the index of the list. The first trimmed substring will be stored at the 0 index of the list, the second will be stored at the 1 index of the list, and so on.
Code
my_string = "Syntax Scenarios is an amazing website for learning fundamental programming concept" substrings = my_string.rsplit('for') truncated_string = substrings[0] print(truncated_string)
Output
Syntax Scenarios is an amazing website
Truncate Strings Using Regular Expressions
Regular Expressions (Regex) are powerful tools used for pattern matching. We can truncate a string by matching its pattern. In regex, we define a pattern and then compare it with a string. To use regex, we first need to import the re
module. Then, we can use the functions of the re module by placing a dot operator after the module name. We will be using the sub()
function of regex to search for the substring that we want to remove from the string. The remaining part of the string will be the substring that we need.
To truncate a string at a specific length, you can define a pattern that counts the number of characters from the end of the string. Then, you can replace those characters with an empty space. This will remove all the specified characters, allowing you to store the desired substring in a variable.
In this pattern ‘.{33}
’ matches any 33 characters. The dot ‘.
’ Is called a wild card and it represents any character (except a newline). The {33}
indicates that it should match exactly 33 of those characters. The $
makes sure that the matched characters must be at the end of the string. This pattern will select the part of the string that we want to remove and the sub()
function will replace this substring with an empty string.
Code
my_string = "Syntax Scenarios is an amazing website for learning fundamental programming concepts" pattern=r'.{33}$' truncated_string = re.sub(pattern," ", my_string) print(truncated_string)
Output
Syntax Scenarios is an amazing website for learning
Use for Loop to Manually Cut Strings
A for
loop is used to perform a task iteratively and it can be used to truncate a string. Strings cannot be changed directly in Python because they are immutable, but you can still create a truncated version of the string by using a for
loop. The for
loop will go through each character of the original string one by one. In every loop cycle, a new string is created by adding the current character of the original string to the previously created substring. The process will start with an empty substring and by the end of the for
loop, you will get a truncated string.
To use a for
loop , you define the minimum range and the maximum range. Inside the loop, the minimum range will be working as the starting index of the substring that you want to trim and the maximum range will work as the ending index.
Code
my_string = "Syntax Scenarios is an amazing website for learning fundamental programming concepts" truncated_string = "" for i in range(23, 38): truncated_string += my_string[i] print(truncated_string)
Output
amazing website
Scenarios Where You Need to Truncate Strings
Here are a few practical scenarios where you need to truncate strings.
- Displaying previews or summaries:
Imagine you’re building a blog or a news website. You don’t want to show the entire article on the homepage; instead, you show a brief summary. You can use string truncation to show just the first 100 characters with a “Read More” link.
Example:article[:100] + '...'
- Limiting text in social media posts:
Think about platforms like Twitter, where there’s a character limit (e.g., 280 characters). You would truncate the user’s post to make sure it fits the limit, preventing them from posting overly long messages.
Example:post[:280]
- Fitting text in UI elements:
Suppose you’re building a mobile app or website, and you have a button with a limited space. If the button label is too long, it might break the layout. Truncating the string fits the text neatly within the button or other UI components.
Example:label[:10] + '...'
(for a button label) - Handling user bio or profile descriptions:
On platforms like Instagram or Twitter, users can write bios, but there’s usually a limit to how long it can be (e.g., 150 characters). You’d truncate any text beyond the limit so that users don’t exceed it.
Example:bio[:150]
- Cleaning up long logs or messages:
When you’re working with server logs or error messages, they can get extremely long. You might want to truncate them to show only the most relevant part, especially when displaying them in a dashboard or log viewer.
Example:log_message[:500] + '...'
Conclusion
String truncation is commonly used in everyday programming to manage and manipulate text data. It allows you to cut off unnecessary parts of a string, making it easier to display, store, or process. In this article, you learned all the possible ways to strip a specific part of a string.
FAQs
Why would you want to truncate a string in Python?
Truncating a string is useful when you only need a portion of a string, such as displaying a preview or saving memory. It helps in managing long strings by making them easier to read and handle.
What is the simplest way to truncate a string in Python?
The simplest method is using string slicing. You can easily specify the starting and ending indices to cut the string to the desired length.
How does the textwrap module help in truncating strings?
The textwrap
module provides a shorten()
function that allows you to truncate a string to a specific width while adding a placeholder (like …) to indicate that the string has been cut off.
Can I truncate a string based on a specific character or substring?
Yes, you can use the rsplit()
function to truncate a string based on a specific character or substring. This method splits the string into parts to easily access and select the desired portion.
What are regular expressions, and how can they be used for truncating strings?
Regular expressions (regex) are powerful tools for pattern matching. You can use regex to define a specific pattern and truncate the string by replacing unwanted parts with an empty string. This method provides flexibility for complex string manipulation.
Is it possible to truncate a string character by character using a loop?
Yes, you can use a for
loop to iterate through each character of the string and copy the desired number of characters into a new string. This method gives you precise control over the truncation process.