Understand the various data types in Python, including integers, floats, strings, and more, with examples and visuals for beginners.
- What are Data Types in Python?
- Why are Data Types Important?
- Types of Data Types in Python
- Numeric Data Types in Python
- Sequence Data Type in Python
- Mapping Data Type in Python
- Set Data Types in Python
- Boolean Data Type in Python
- Binary Data Types in Python
- How to Check the Type of a Variable in Python
- Python 3 Data Types with Sizes
- Conclusion
What are Data Types in Python?
Data types in Python categorize the kind of data a variable holds, similar to how a debit card stores specific information. For example, the account number on a debit card is stored as an int
(short for integer), which means it’s a whole number. The variable accountNumber
is of type int
. Similarly, the cardholder’s name is stored as a string
, which means it’s text. The variable name
is of type string
. This way, you know exactly what type of information is in each variable, helping you use it correctly.
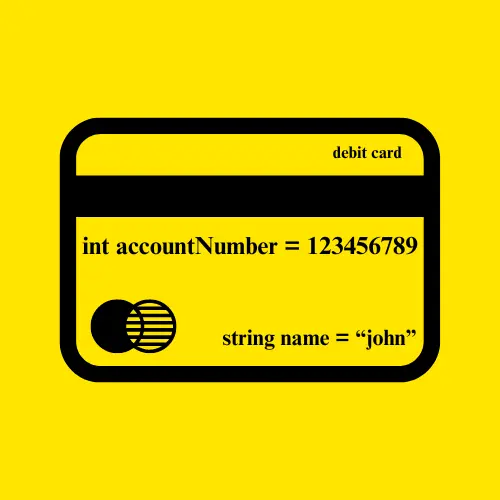
Why are Data Types Important?
Understanding data types is important for several reasons:
- Memory Management: Different data types use different amounts of memory. Knowing the type of data you’re working with helps make your program more efficient.
- Error Prevention: Many programming errors happen because of using the wrong data types. Understanding data types can help you avoid these mistakes.
- Efficiency: Some operations are faster with specific data types. For example, math operations are quicker with numbers than with text.
- Clarity: Using the right data types makes your code easier to read and understand, which is especially helpful when working with others.
Types of Data Types in Python
Python has six primary categories of data types, each with its own subtypes.
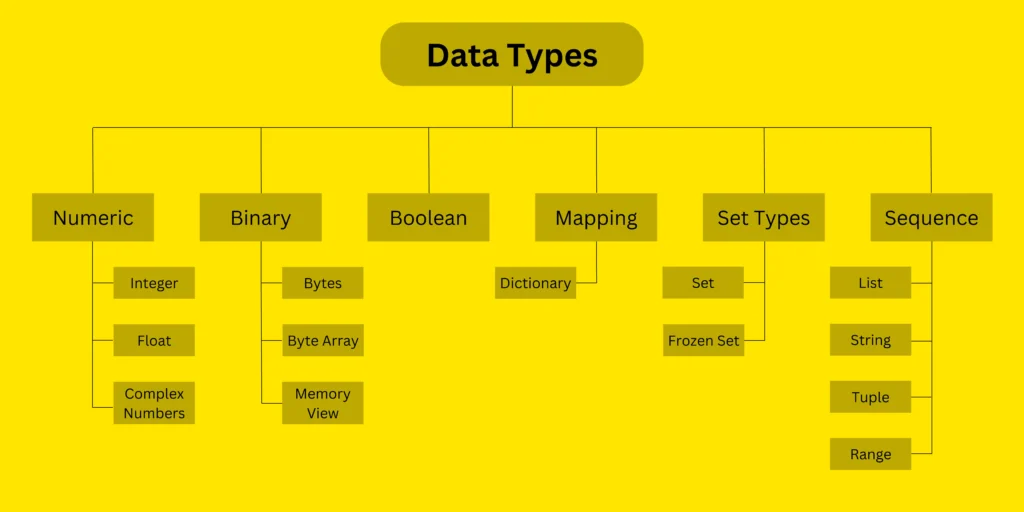
Numeric Data Types in Python
Numeric data types in Python represent numbers and are used for calculations and operations. They are divided into three main types: integers, floats, and complex numbers. Each type is suited for different numerical tasks.
Integer (int)
Represents whole numbers without any decimal point.
Syntax
variable_name = value
Example
age = 25 distance = -100
Float (float)
Represents real numbers with a decimal point.
Syntax
variable_name = value
Example
price = 19.99 temperature = -3.5
Complex Data Type in Python
Complex data type represents complex numbers with a real part
and an imaginary part
. First, the real part
is written as a
. Next, the imaginary part
is written as b
, followed by j
. Together, they form a + bj
. The value of a and b depends on the user. Complex numbers are useful in advanced mathematical computations.
Syntax
variable_name = a + bj
Example
first_complex = 3 + 4j # Here, 3 is the real part, and 4 is the imaginary part second_complex = 5 - 2j # Here, 5 is the real part (a), and -2 is the imaginary part (b)
These numeric data types allow you to perform a wide range of mathematical operations in Python, making it a versatile language for scientific and engineering calculations.
Sequence Data Type in Python
Sequence data types in Python represent ordered collections of items. They allow you to store multiple values in an organized manner. The main sequence data types in Python are lists, tuples, ranges, and strings.
List (list)
List in Python is an ordered collection of items.
Syntax
list_name = [item1, item2, item3]
Example
fruits = ['apple', 'banana', 'cherry']
Tuple (tuple)
A tuple in Python is an ordered, immutable collection of items.
Syntax:
tuple_name = (item1, item2, item3)
Example
coordinates = (10, 20)
Range (range)
A range represents a sequence of numbers. The range()
built-in function generates numbers from a start value to an end value, with an optional step size.
Syntax
range_name = range(start, stop, step)
Example
numbers = range(1, 5) # Generates numbers from 1 to 4 even_numbers = range(0, 11, 2) # Generates numbers from 0 to 10, stepping by 2
String (str)
A String in Python represents a sequence of characters. These sequence data types help you organize and manage collections of items in a systematic way, making it easier to perform operations on them.
Syntax
string_name = "your text"
Example
message = "Hello, World!"
Mapping Data Type in Python
Mapping data types in Python are used to store data in key-value pairs. The primary mapping type in Python is the dictionary.
Dictionary (dict)
A dictionary in Python is an unordered collection of key-value pairs. The keys must be unique and immutable, while values can be of any type. Dictionaries are powerful for storing and manipulating data in key-value pairs, making them essential for tasks involving lookup tables, databases, and associative arrays.
Syntax
dict_name = {key1: value1, key2: value2}
Example
student = { "name": "John", "age": 21, "grades": [88, 92, 79] }
Set Data Types in Python
Set data types in Python are used to store collections of unique items. They are unordered, meaning the items do not have a specific order.
Set (set)
A set is an unordered collection of unique items.
Syntax
set_name = {item1, item2, item3}
Example
fruits = {'apple', 'banana', 'cherry'}
Frozen Set (frozenset)
An immutable version of a set. Once created, its items cannot be changed. A frozenset is created using the frozenset()
function in Python. These set data types are useful for handling collections of items where uniqueness is important and order is not.
Syntax
frozenset_name = frozenset([item1, item2, item3])
Example
frozen_fruits = frozenset(['apple', 'banana', 'cherry'])
Boolean Data Type in Python
Boolean data types in Python are used to represent truth values. They can have only two possible values: True and False. Boolean values are commonly used in conditional statements, loops, and logical expressions to make decisions and control the program’s behavior.
Syntax
variable_name = True # OR Variable_name = False
Example
is_sunny = True is_raining = False
Binary Data Types in Python
Binary data types in Python are used to handle data in binary format. You use built-in functions to create and work with these types.
Bytes (bytes)
An immutable sequence of bytes, typically used for binary data.
Syntax
bytes_object = b'byte_string'
Example
binary_data = b'\x68\x65\x6c\x6c\x6f' # Represents the string "hello" in bytes
Bytearray (bytearray)
A mutable sequence of bytes. Created using the bytearray()
function. This allows you to modify the binary data after creation.
Syntax
bytearray_object = bytearray(iterable)
Example
mutable_binary_data = bytearray(b'hello') mutable_binary_data[0] = 72 # Change first byte to 72 (ASCII for 'H')
Memoryview (memoryview)
Memoryview provides a view into the memory of a byte-oriented object without copying it. Created using the memoryview()
function. This enables efficient access and manipulation of binary data.
Syntax
memoryview_object = memoryview(bytes_object)
Example
byte_data = bytes([1, 2, 3, 4, 5]) view = memoryview(byte_data)
How to Check the Type of a Variable in Python
In Python, determining the type of a variable is essential for understanding how to work with it. The built-in type()
function is used to check the type of any variable. Below is a comprehensive code snippet demonstrating how to check the types of different variables, along with comments indicating the variable type being checked.
# Integer (int) x = 10 print(type(x)) # Output: <class 'int'> # Float (float) y = 10.5 print(type(y)) # Output: <class 'float'> # String (str) z = "Hello" print(type(z)) # Output: <class 'str'> # List (list) my_list = [1, 2, 3] print(type(my_list)) # Output: <class 'list'> # Dictionary (dict) my_dict = {'a': 1, 'b': 2} print(type(my_dict)) # Output: <class 'dict'> # Set (set) my_set = {1, 2, 3} print(type(my_set)) # Output: <class 'set'> # Tuple (tuple) my_tuple = (1, 2, 3) print(type(my_tuple)) # Output: <class 'tuple'> # Boolean (bool) flag = True print(type(flag)) # Output: <class 'bool'> # Bytes (bytes) byte_data = b'hello' print(type(byte_data)) # Output: <class 'bytes'> # Bytearray (bytearray) byte_array_data = bytearray(b'hello') print(type(byte_array_data)) # Output: <class 'bytearray'> # Memoryview (memoryview) byte_data = bytes([1, 2, 3]) memory_view = memoryview(byte_data) print(type(memory_view)) # Output: <class 'memoryview'> # Range (range) range_data = range(1, 10) print(type(range_data)) # Output: <class 'range'> # Frozen Set (frozenset) frozen_set_data = frozenset([1, 2, 3]) print(type(frozen_set_data)) # Output: <class 'frozenset'>
In this snippet:
- The
type()
function is used to determine and print the type of each variable. - Different data types are demonstrated, including integers, floats, strings, lists, dictionaries, sets, tuples, booleans, bytes, bytearrays, memoryviews, ranges, and frozen sets.
- Comments are included to indicate the type of each variable being checked.
Using the type()
function helps you understand the nature and behavior of the data you’re working with, ensuring you apply the correct operations and functions to your variables.
Python 3 Data Types with Sizes
The sizes listed in the table are based on Python 3, the currently recommended version. Python 2, being outdated and no longer maintained, has different sizes and behavior for data types.
Category | Data Types | Size |
Numeric | Integer (int) | 28 bytes |
Float (float) | 24 bytes | |
Complex (complex) | 32 bytes | |
Sequence | String (str) | 49 bytes + 1 byte per character |
List (list) | 64 bytes + 8 bytes per item | |
Tuple (tuple) | 48 bytes + 8 bytes per item | |
Range (range) | 48 bytes | |
Mapping | Dictionary (dict) | 240 bytes + 8 bytes per item |
Set | Set (set) | 216 bytes + 8 bytes per item |
Frozen Set (frozenset) | 216 bytes + 8 bytes per item | |
Boolean | Boolean (bool) | 24 bytes |
Binary | Bytes (bytes) | 33 bytes + 1 byte per item |
Bytearray (bytearray) | 56 bytes + 1 byte per item | |
Memoryview (memoryview) | 48 bytes (minimum, varies with object) |
Conclusion
In summary, understanding data types in Python is essential for effective programming. Each type, whether it’s numbers, text, or complex structures, has a specific purpose. Knowing their sizes helps you manage memory more efficiently. This understanding allows you to select the right data type for different tasks. Consequently, your code will run smoother and perform better.
For more detailed information on data types, visit the official Python documentation.